### 深入学习C++ String 2.1版 #### C++的`string`的使用 ##### 1.1 C++ `string`简介 在C++中,`string`是一个非常重要的容器类,用于处理文本数据。它提供了一系列丰富的接口来支持字符串的基本操作和高级功能,比如拼接、搜索、替换等。`string`类是标准模板库(STL)的一部分,定义在`<string>`头文件中。 ##### 1.2 `string`的成员函数 **1.2.1 append** `append`函数用于将一个或多个字符串添加到当前`string`对象的末尾。例如: ```cpp std::string s = "hello"; s.append(" world"); ``` 这会使得`s`变为"hello world"。 **1.2.2 assign** `assign`函数用于改变`string`对象的内容。它可以接受多种参数类型,包括单个字符、字符数组、另一个`string`对象等。例如: ```cpp std::string s; s.assign(5, 'a'); // 将s设置为"aaaaa" ``` **1.2.3 at** `at`函数返回指定索引处的字符,并且如果索引超出范围会抛出异常。例如: ```cpp std::string s = "hello"; char c = s.at(1); // c 是'e' ``` **1.2.4 begin** `begin`返回指向`string`第一个元素的迭代器。例如: ```cpp std::string s = "hello"; auto it = s.begin(); ``` **1.2.5 c_str** `c_str`返回一个指向`string`内容的常量指针,该指针可以被用作C风格字符串。例如: ```cpp std::string s = "hello"; const char* cs = s.c_str(); ``` **1.2.6 capacity** `capacity`返回`string`对象当前能够容纳的字符的最大数量。例如: ```cpp std::string s = "hello"; size_t cap = s.capacity(); // 返回当前容量 ``` **1.2.7 clear** `clear`函数用于清空`string`对象中的所有内容。例如: ```cpp std::string s = "hello"; s.clear(); // 清空s ``` **1.2.8 compare** `compare`函数用于比较两个`string`对象的内容。如果它们相同则返回0;如果当前对象小于另一个则返回负值;反之,则返回正值。例如: ```cpp std::string s1 = "hello"; std::string s2 = "world"; int res = s1.compare(s2); // res < 0 ``` **1.2.9 copy** `copy`函数用于复制一个`string`对象的部分内容到另一个字符数组中。例如: ```cpp std::string s = "hello"; char buf[10]; s.copy(buf, 3); // buf 现在包含"hel" ``` **1.2.10 _Copy_s** `_Copy_s`是一个非标准的函数,不推荐使用。它主要用于复制字符串,但在安全性方面存在问题。 **1.2.11 data** `data`返回一个指向`string`内部存储区的指针。例如: ```cpp std::string s = "hello"; char* p = s.data(); ``` **1.2.12 empty** `empty`检查`string`对象是否为空。例如: ```cpp std::string s = ""; bool b = s.empty(); // b 是true ``` **1.2.13 end** `end`返回一个指向`string`最后一个元素之后位置的迭代器。例如: ```cpp std::string s = "hello"; auto it = s.end(); ``` **1.2.14 erase** `erase`函数用于删除`string`对象的一部分内容。例如: ```cpp std::string s = "hello"; s.erase(1, 2); // 删除"el", s 变为"ho" ``` **1.2.15 find** `find`函数用于在`string`对象中查找子串的位置。如果找到则返回子串的起始位置;否则返回`npos`。例如: ```cpp std::string s = "hello"; size_t pos = s.find("ll"); // pos 是2 ``` **1.2.16 find_first_not_of** `find_first_not_of`函数用于在`string`对象中查找第一个不属于给定字符集的字符的位置。例如: ```cpp std::string s = "hello"; size_t pos = s.find_first_not_of("eo"); // pos 是1 ``` **1.2.17 find_first_of** `find_first_of`函数用于在`string`对象中查找第一个属于给定字符集的字符的位置。例如: ```cpp std::string s = "hello"; size_t pos = s.find_first_of("eo"); // pos 是1 ``` **1.2.18 find_last_not_of** `find_last_not_of`函数用于在`string`对象中查找最后一个不属于给定字符集的字符的位置。例如: ```cpp std::string s = "hello"; size_t pos = s.find_last_not_of("eo"); // pos 是3 ``` **1.2.19 find_last_of** `find_last_of`函数用于在`string`对象中查找最后一个属于给定字符集的字符的位置。例如: ```cpp std::string s = "hello"; size_t pos = s.find_last_of("eo"); // pos 是1 ``` **1.2.20 get_allocator** `get_allocator`返回用于创建`string`对象的分配器对象。通常情况下,这个分配器对象不需要显式使用。 **1.2.21 insert** `insert`函数用于在`string`对象的指定位置插入新的内容。例如: ```cpp std::string s = "hello"; s.insert(1, "e"); // s 变为"heello" ``` **1.2.22 length** `length`返回`string`对象中字符的数量。例如: ```cpp std::string s = "hello"; size_t len = s.length(); // len 是5 ``` **1.2.23 max_size** `max_size`返回`string`对象最大可能的长度。例如: ```cpp std::string s; size_t maxSize = s.max_size(); ``` **1.2.24 push_back** `push_back`函数用于在`string`对象的末尾添加一个字符。例如: ```cpp std::string s = "hello"; s.push_back('!'); // s 变为"hello!" ``` **1.2.25 rbegin** `rbegin`返回一个反向迭代器,指向`string`对象的最后一个元素。例如: ```cpp std::string s = "hello"; auto rit = s.rbegin(); ``` **1.2.26 rend** `rend`返回一个反向迭代器,指向`string`对象的第一个元素之前的位置。例如: ```cpp std::string s = "hello"; auto rit = s.rend(); ``` **1.2.27 replace** `replace`函数用于替换`string`对象的一部分内容。例如: ```cpp std::string s = "hello"; s.replace(1, 2, "a"); // 替换"el"为"a", s 变为"hallo" ``` **1.2.28 reserve** `reserve`函数用于预留空间,以便`string`对象能够在不重新分配内存的情况下扩展。例如: ```cpp std::string s; s.reserve(100); // 预留100个字符的空间 ``` **1.2.29 resize** `resize`函数用于改变`string`对象的大小。如果新大小比当前大小大,则会填充默认字符(通常是`\0`)。例如: ```cpp std::string s = "hello"; s.resize(10); // 填充'\0'到10个字符 ``` **1.2.30 rfind** `rfind`函数用于从`string`对象的末尾开始查找子串的位置。如果找到则返回子串的起始位置;否则返回`npos`。例如: ```cpp std::string s = "hello"; size_t pos = s.rfind("ll"); // pos 是2 ``` **1.2.31 size** `size`返回`string`对象中字符的数量。例如: ```cpp std::string s = "hello"; size_t sz = s.size(); // sz 是5 ``` **1.2.32 substr** `substr`函数用于提取`string`对象的一个子串。例如: ```cpp std::string s = "hello"; std::string sub = s.substr(1, 2); // sub 是"el" ``` **1.2.33 swap** `swap`函数用于交换两个`string`对象的内容。例如: ```cpp std::string s1 = "hello"; std::string s2 = "world"; s1.swap(s2); // 交换s1和s2的内容 ``` **1.3 `string`的构造** `string`提供了多种构造方式,包括默认构造、基于字符数组的构造、基于另一个`string`对象的构造等。例如: ```cpp std::string s1; // 默认构造 std::string s2("hello"); // 基于C风格字符串的构造 std::string s3(s2); // 基于另一个string对象的构造 ``` **1.4 `string`的重载运算符** `string`类重载了一些运算符,如`+`用于连接两个`string`对象,`==`用于比较两个`string`对象是否相等。例如: ```cpp std::string s1 = "hello"; std::string s2 = "world"; std::string s3 = s1 + " " + s2; // s3 是"hello world" ``` **1.5 `string`与`algorithm`相结合的使用** `string`类可以结合`algorithm`头文件中的函数使用,以实现更复杂的功能。例如: - **1.5.1 `string`与`remove`** - 使用`remove`移除`string`中的某些字符。 - **1.5.2 `string`与`unique`、`sort`** - 结合使用`unique`和`sort`去除重复字符并排序。 - **1.5.3 `string`与`search`** - 使用`search`在`string`中查找模式。 - **1.5.4 `string`和`find`、`find_if`** - 使用`find`和`find_if`搜索特定字符或满足条件的字符。 - **1.5.5 `string`与`copy`、`copy_if`** - 使用`copy`和`copy_if`复制满足条件的字符。 - **1.5.6 `string`与`count`、`count_if`** - 使用`count`和`count_if`统计特定字符或满足条件的字符的数量。 **1.6 `string`与`wstring`** `wstring`是宽字符版本的`string`,用于处理宽字符数据。它使用`wchar_t`类型作为其内部表示。 **1.6.1 简介** `wstring`提供了类似于`string`的功能,但使用宽字符。 **1.6.2 `wstring`实例** ```cpp std::wstring ws(L"hello"); ``` **1.6.3 `wstring`与控制台** 在Windows环境下,可以使用`SetConsoleOutputCP`和`SetConsoleCP`来配置控制台以显示宽字符。 **1.6.4 `string`与`wstring`的相互转换** 可以通过`std::use_facet<std::ctype<wchar_t> >`等工具进行转换。 **1.7 `string`与C++流** `string`可以与C++流一起使用,用于读写数据。 **1.7.1 C++流简介** C++流是一组用于输入输出操作的对象,如`cin`、`cout`。 **1.7.2 `string`与`iostream`、`fstream`** 可以使用`iostream`和`fstream`读取或写入`string`。 **1.8 格式化字符串** `string`可以通过各种方法进行格式化,如使用C语言的`snprintf`或者C++的`std::stringstream`。 **1.9 `string`与`CString`** 在MFC中,`CString`是一个广泛使用的类,用于处理字符串。它提供了一些额外的功能,如多字节和宽字符之间的转换。 --- 以上是对`string`类及其成员函数的详细介绍,涵盖了从基本使用到高级应用的各个方面。熟练掌握这些知识点对于高效地使用C++进行字符串处理至关重要。
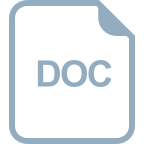
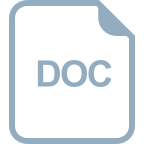
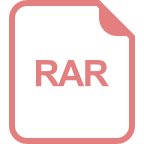
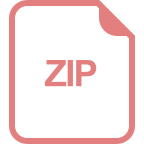
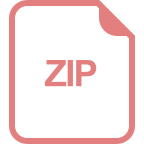
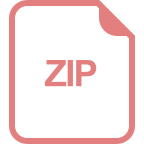
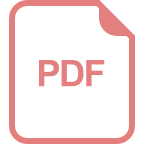
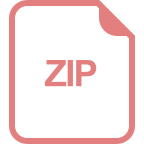
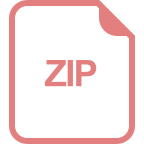
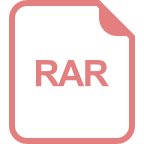
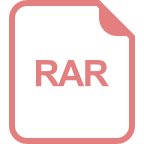
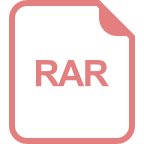
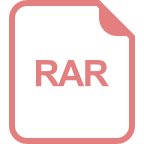
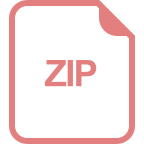
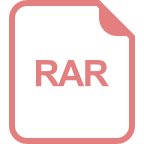
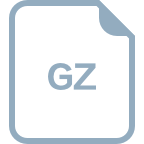
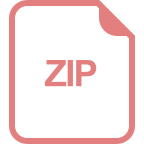
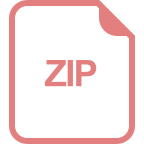
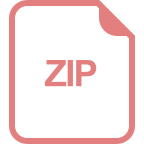
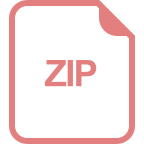
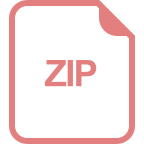
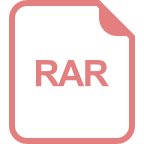
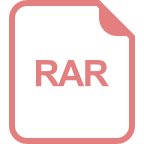
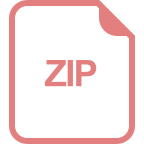
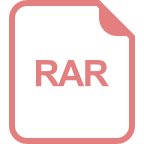
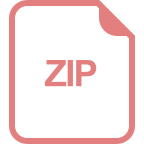
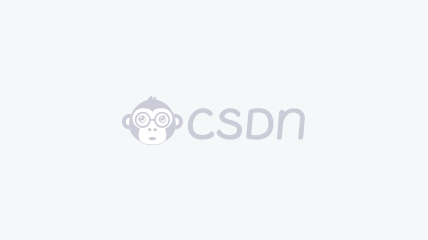
- aoa1122013-12-29普及基础知识,不错

- 粉丝: 0
- 资源: 12
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

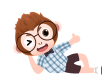
最新资源

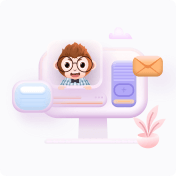
