java https POST/GET取数据
需积分: 12 132 浏览量
2018-04-16
17:33:07
上传
评论
收藏 1.09MB RAR 举报

hengfeiyu1
- 粉丝: 1
- 资源: 20
最新资源
- Fortran语言语法快速入门.pdf
- 明日方舟 年 鼠标指针.rar
- 全国银行经济监管可视化系统
- e商小二-供应链管理物流交仓创业商业计划书.rar
- Edge浏览器下载文件提示 “无法安全下载” 的解决方法
- 基于springboot+layui的医院日常耗材管理系统.zip
- Emkex亿迈克思新型互联网磁材供应链商业计划书.rar
- 计算机毕业设计-ASP.NET某店POS积分管理系统-销售情况,会员卡再发行数据生成(源代码+)-毕设源码实例.zip
- 计算机毕业设计-asp.net某店POS积分管理系统-清除履历表、日志表、月购买额(源代码+)-毕设源码实例.zip
- 计算机毕业设计-ASP.NET某店POS积分管理系统-积分实绩更新及销售状况统计(源代码+)-毕设源码实例.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


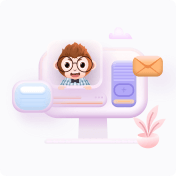