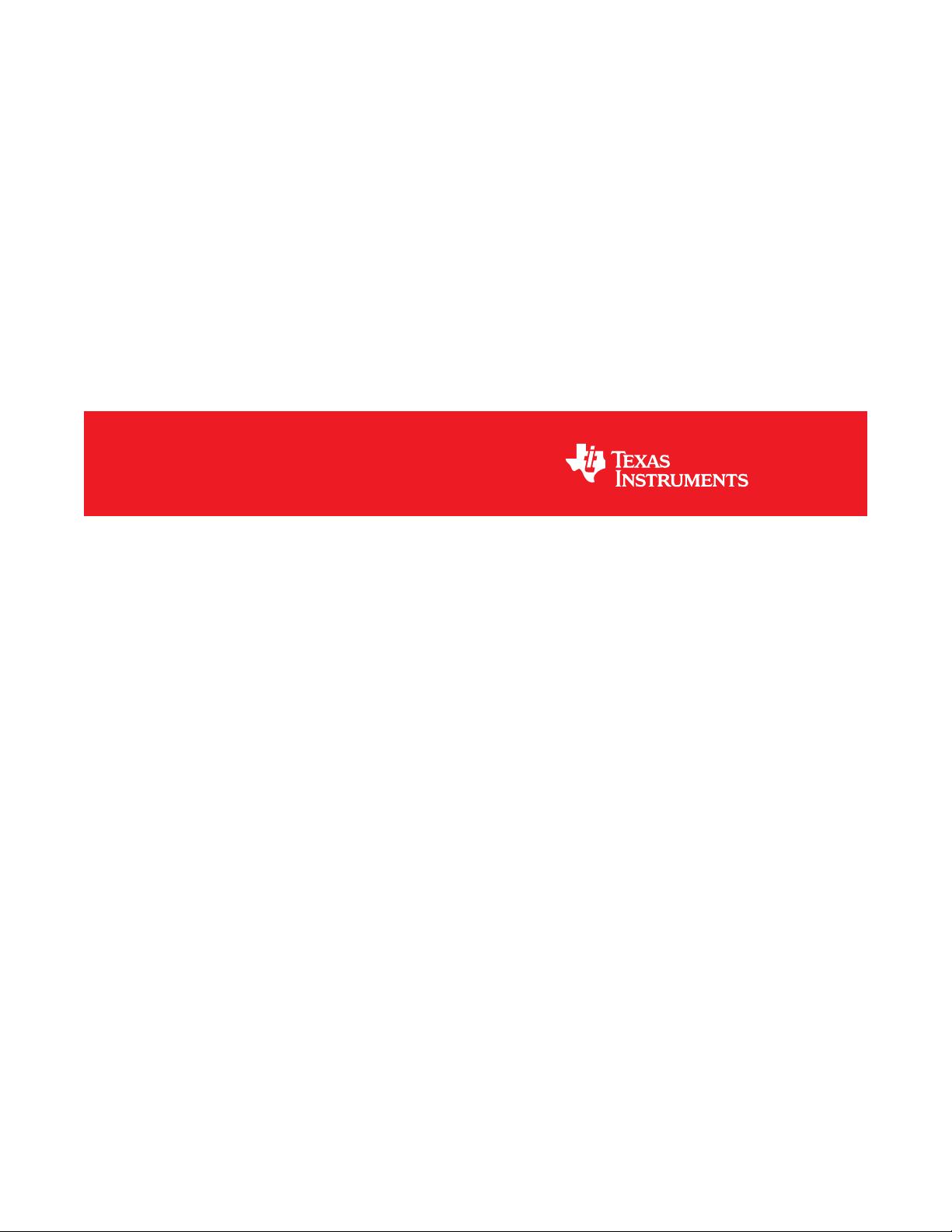
TMS320C6000 Optimizing Compiler
v7.6
User's Guide
Literature Number: SPRU187V
March 2014
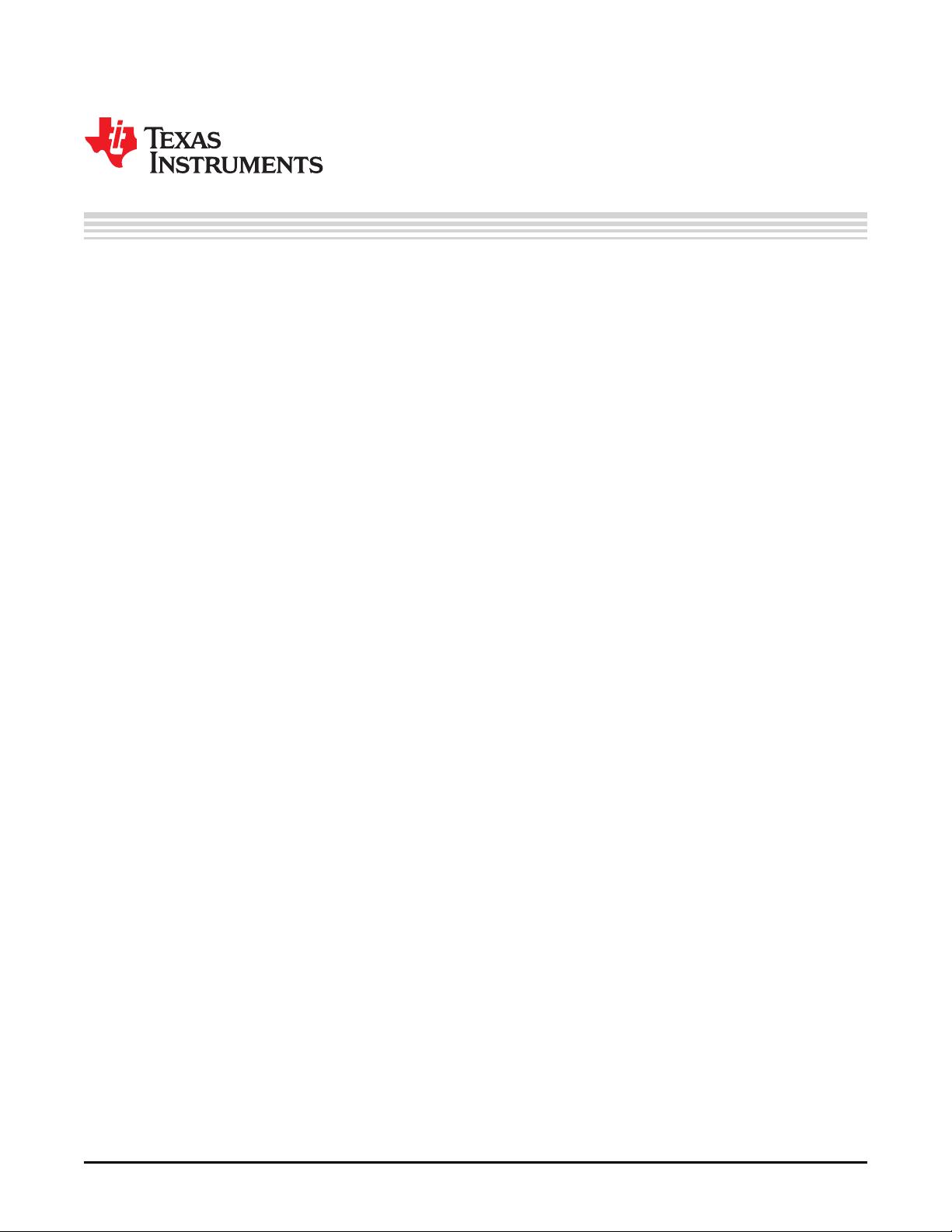
Contents
Preface ...................................................................................................................................... 11
1 Introduction to the Software Development Tools ................................................................... 14
1.1 Software Development Tools Overview ................................................................................ 15
1.2 Compiler Interface ......................................................................................................... 16
1.3 ANSI/ISO Standard ........................................................................................................ 16
1.4 Output Files ................................................................................................................ 17
1.5 Utilities ...................................................................................................................... 17
2 Using the C/C++ Compiler .................................................................................................. 18
2.1 About the Compiler ........................................................................................................ 19
2.2 Invoking the C/C++ Compiler ............................................................................................ 19
2.3 Changing the Compiler's Behavior with Options ...................................................................... 20
2.3.1 Frequently Used Options ........................................................................................ 29
2.3.2 Miscellaneous Useful Options .................................................................................. 31
2.3.3 Run-Time Model Options ........................................................................................ 33
2.3.4 Selecting Target CPU Version (--silicon_version Option) ................................................... 34
2.3.5 Symbolic Debugging and Profiling Options ................................................................... 35
2.3.6 Specifying Filenames ............................................................................................ 36
2.3.7 Changing How the Compiler Interprets Filenames ........................................................... 36
2.3.8 Changing How the Compiler Processes C Files ............................................................. 37
2.3.9 Changing How the Compiler Interprets and Names Extensions ........................................... 37
2.3.10 Specifying Directories ........................................................................................... 37
2.3.11 Assembler Options .............................................................................................. 38
2.3.12 Dynamic Linking ................................................................................................. 39
2.3.13 Deprecated Options ............................................................................................. 40
2.4 Controlling the Compiler Through Environment Variables ........................................................... 40
2.4.1 Setting Default Compiler Options (C6X_C_OPTION) ....................................................... 40
2.4.2 Naming an Alternate Directory (C6X_C_DIR) ................................................................ 41
2.5 Controlling the Preprocessor ............................................................................................. 42
2.5.1 Predefined Macro Names ....................................................................................... 42
2.5.2 The Search Path for #include Files ............................................................................ 43
2.5.3 Support for the #warning and #warn Directives .............................................................. 44
2.5.4 Generating a Preprocessed Listing File (--preproc_only Option) .......................................... 44
2.5.5 Continuing Compilation After Preprocessing (--preproc_with_compile Option) .......................... 44
2.5.6 Generating a Preprocessed Listing File with Comments (--preproc_with_comment Option) .......... 44
2.5.7 Generating a Preprocessed Listing File with Line-Control Information (--preproc_with_line Option) . 45
2.5.8 Generating Preprocessed Output for a Make Utility (--preproc_dependency Option) ................... 45
2.5.9 Generating a List of Files Included with the #include Directive (--preproc_includes Option) ........... 45
2.5.10 Generating a List of Macros in a File (--preproc_macros Option) ........................................ 45
2.6 Understanding Diagnostic Messages ................................................................................... 45
2.6.1 Controlling Diagnostic Messages ............................................................................... 46
2.6.2 How You Can Use Diagnostic Suppression Options ........................................................ 47
2.7 Other Messages ........................................................................................................... 48
2.8 Generating Cross-Reference Listing Information (--gen_acp_xref Option) ........................................ 48
2.9 Generating a Raw Listing File (--gen_acp_raw Option) .............................................................. 48
2.10 Using Inline Function Expansion ........................................................................................ 50
2
Contents SPRU187V–March 2014
Submit Documentation Feedback
Copyright © 2014, Texas Instruments Incorporated
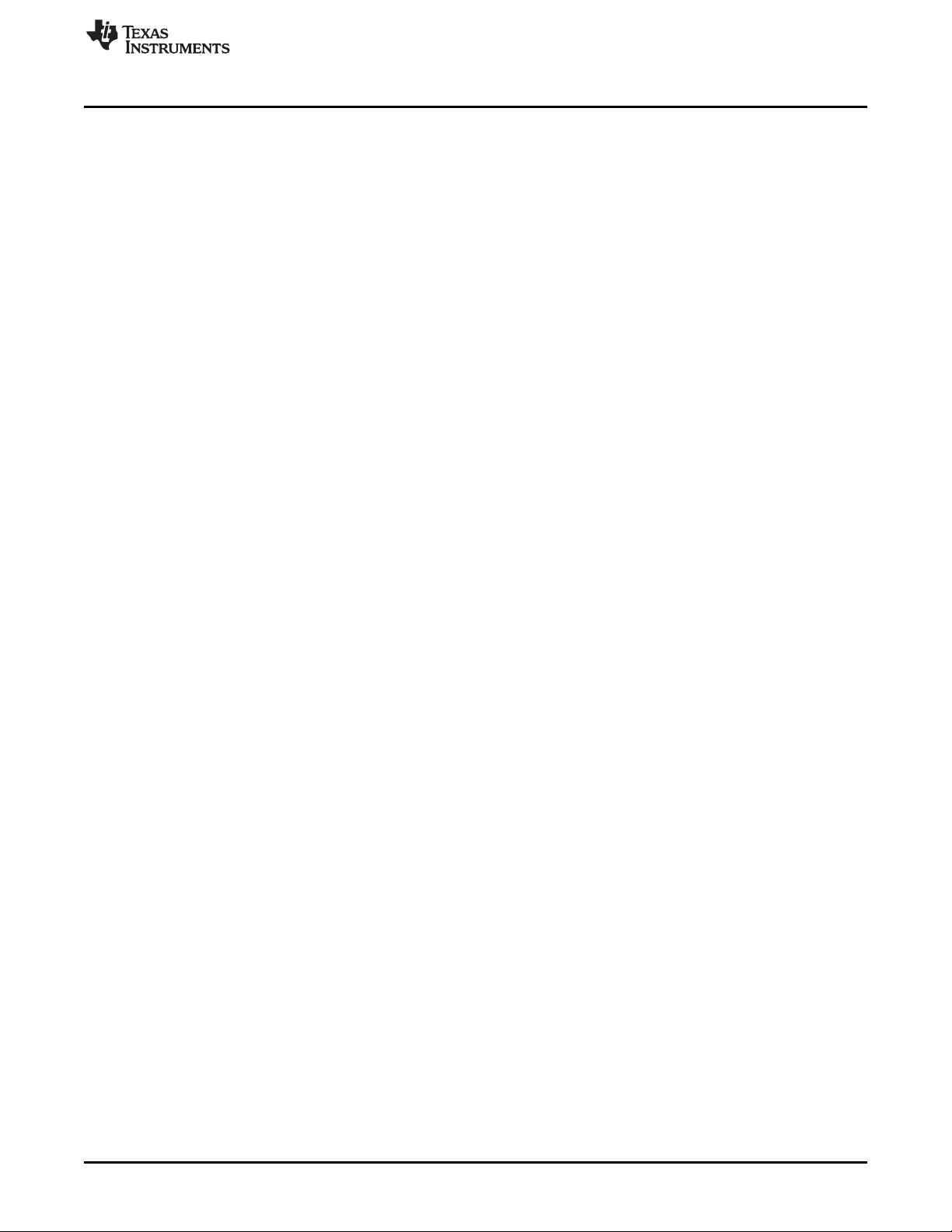
www.ti.com
2.10.1 Inlining Intrinsic Operators ..................................................................................... 51
2.10.2 Automatic Inlining ................................................................................................ 51
2.10.3 Unguarded Definition-Controlled Inlining ..................................................................... 51
2.10.4 Guarded Inlining and the _INLINE Preprocessor Symbol .................................................. 51
2.10.5 Inlining Restrictions ............................................................................................. 53
2.11 Interrupt Flexibility Options (--interrupt_threshold Option) ........................................................... 54
2.12 Linking C6400 Code with C6200/C6700/Older C6400 Object Code ............................................... 55
2.13 Using Interlist ............................................................................................................... 55
2.14 Generating and Using Performance Advice ........................................................................... 56
2.15 Controlling Application Binary Interface ................................................................................ 57
2.16 Enabling Entry Hook and Exit Hook Functions ........................................................................ 58
3 Optimizing Your Code ........................................................................................................ 59
3.1 Invoking Optimization ..................................................................................................... 60
3.2 Optimizing Software Pipelining .......................................................................................... 61
3.2.1 Turn Off Software Pipelining (--disable_software_pipelining Option) ...................................... 62
3.2.2 Software Pipelining Information ................................................................................. 62
3.2.3 Collapsing Prologs and Epilogs for Improved Performance and Code Size .............................. 67
3.3 Redundant Loops .......................................................................................................... 68
3.4 Utilizing the Loop Buffer Using SPLOOP on C6400+, C6740, and C6600 ........................................ 69
3.5 Reducing Code Size (--opt_for_space (or -ms) Option) .............................................................. 70
3.6 Performing File-Level Optimization (--opt_level=3 option) ........................................................... 70
3.6.1 Controlling File-Level Optimization (--std_lib_func_def Options) ........................................... 70
3.6.2 Creating an Optimization Information File (--gen_opt_info Option) ........................................ 71
3.7 Performing Program-Level Optimization (--program_level_compile and --opt_level=3 options) ................ 71
3.7.1 Controlling Program-Level Optimization (--call_assumptions Option) ..................................... 72
3.7.2 Optimization Considerations When Mixing C/C++ and Assembly ......................................... 72
3.8 Using Feedback Directed Optimization ................................................................................. 74
3.8.1 Feedback Directed Optimization ............................................................................... 74
3.8.2 Profile Data Decoder ............................................................................................. 76
3.8.3 Feedback Directed Optimization API .......................................................................... 77
3.8.4 Feedback Directed Optimization Summary ................................................................... 77
3.9 Using Profile Information to Get Better Program Cache Layout and Analyze Code Coverage ................. 78
3.9.1 Background and Motivation ..................................................................................... 78
3.9.2 Code Coverage ................................................................................................... 79
3.9.3 What Performance Improvements Can You Expect to See? ............................................... 80
3.9.4 Program Cache Layout Related Features and Capabilities ................................................ 80
3.9.5 Program Instruction Cache Layout Development Flow ...................................................... 81
3.9.6 Comma-Separated Values (CSV) Files with Weighted Call Graph (WCG) Information ................ 84
3.9.7 Linker Command File Operator - unordered() ................................................................ 84
3.9.8 Things to be Aware of ........................................................................................... 87
3.10 Indicating Whether Certain Aliasing Techniques Are Used .......................................................... 88
3.10.1 Use the --aliased_variables Option When Certain Aliases are Used ..................................... 88
3.10.2 Use the --no_bad_aliases Option to Indicate That These Techniques Are Not Used .................. 88
3.10.3 Using the --no_bad_aliases Option With the Assembly Optimizer ........................................ 89
3.11 Prevent Reordering of Associative Floating-Point Operations ....................................................... 89
3.12 Use Caution With asm Statements in Optimized Code .............................................................. 90
3.13 Automatic Inline Expansion (--auto_inline Option) .................................................................... 90
3.14 Using Performance Advice to Optimize Your Code ................................................................... 91
3.14.1 Advice #27000 ................................................................................................... 92
3.14.2 Advice #27001: Increase Optimization Level ................................................................ 92
3.14.3 Advice #27002: Do not turn off software pipelining ......................................................... 93
3.14.4 Advice #27003: Avoid compiling with debug options ....................................................... 93
3.14.5 Advice #27004: No Performance Advice generated ........................................................ 93
3
SPRU187V–March 2014 Contents
Submit Documentation Feedback
Copyright © 2014, Texas Instruments Incorporated
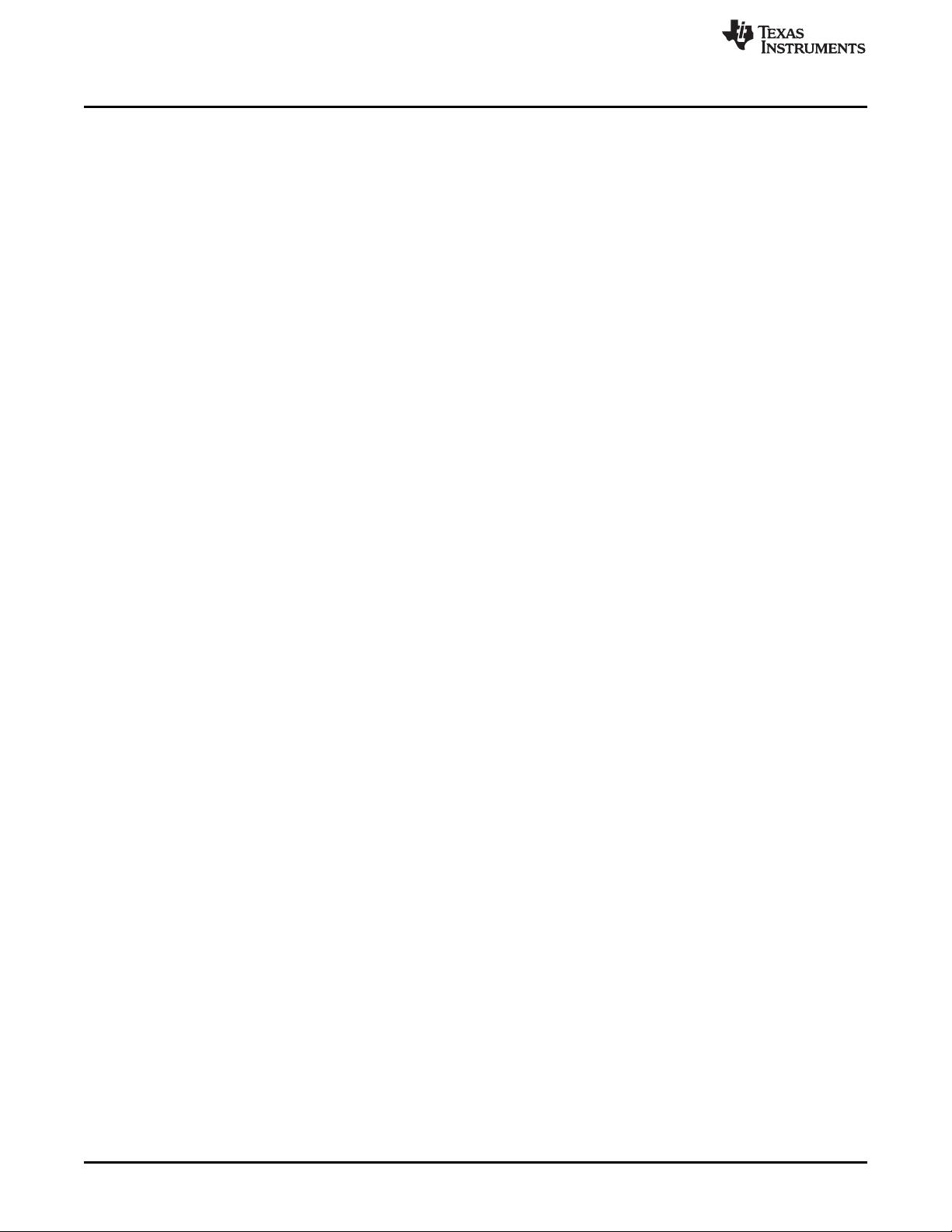
www.ti.com
3.14.6 Advice #30000: Prevent Loop Disqualification due to call ................................................. 93
3.14.7 Advice #30001: Prevent Loop Disqualification due to rts-call ............................................. 94
3.14.8 Advice #30002: Prevent Loop Disqualification due to asm statement ................................... 94
3.14.9 Advice #30003: Prevent Loop Disqualification due to complex condition ................................ 94
3.14.10 Advice #30004: Prevent Loop Disqualification due to switch statement ................................ 95
3.14.11 Advice #30005: Prevent Loop Disqualification due to arithmetic operation ............................ 95
3.14.12 Advice #30006: Prevent Loop Disqualification due to call(2) ............................................ 95
3.14.13 Advice #30007: Prevent Loop Disqualification due to rts-call(2) ........................................ 96
3.14.14 Advice #30008: Improve Loop; Qualify with restrict ....................................................... 96
3.14.15 Advice #30009: Improve Loop; Add MUST_ITERATE pragma .......................................... 97
3.14.16 Advice #30010: Improve Loop; Add MUST_ITERATE pragma(2) ...................................... 97
3.14.17 Advice #30011: Improve Loop; Add _nasssert() ........................................................... 97
3.15 Using the Interlist Feature With Optimization .......................................................................... 97
3.16 Debugging and Profiling Optimized Code .............................................................................. 99
3.16.1 Profiling Optimized Code ....................................................................................... 99
3.17 Controlling Code Size Versus Speed ................................................................................. 100
3.18 What Kind of Optimization Is Being Performed? ..................................................................... 100
3.18.1 Cost-Based Register Allocation .............................................................................. 101
3.18.2 Alias Disambiguation .......................................................................................... 101
3.18.3 Branch Optimizations and Control-Flow Simplification .................................................... 102
3.18.4 Data Flow Optimizations ...................................................................................... 102
3.18.5 Expression Simplification ..................................................................................... 102
3.18.6 Inline Expansion of Functions ................................................................................ 102
3.18.7 Function Symbol Aliasing ..................................................................................... 102
3.18.8 Induction Variables and Strength Reduction ............................................................... 103
3.18.9 Loop-Invariant Code Motion .................................................................................. 103
3.18.10 Loop Rotation ................................................................................................. 103
3.18.11 Instruction Scheduling ....................................................................................... 103
3.18.12 Register Variables ............................................................................................ 103
3.18.13 Register Tracking/Targeting ................................................................................. 103
3.18.14 Software Pipelining ........................................................................................... 103
4 Using the Assembly Optimizer .......................................................................................... 104
4.1 Code Development Flow to Increase Performance ................................................................. 105
4.2 About the Assembly Optimizer ......................................................................................... 106
4.3 What You Need to Know to Write Linear Assembly ................................................................. 107
4.3.1 Linear Assembly Source Statement Format ................................................................. 108
4.3.2 Register Specification for Linear Assembly .................................................................. 109
4.3.3 Functional Unit Specification for Linear Assembly .......................................................... 111
4.3.4 Using Linear Assembly Source Comments .................................................................. 111
4.3.5 Assembly File Retains Your Symbolic Register Names ................................................... 112
4.4 Assembly Optimizer Directives ......................................................................................... 113
4.4.1 Instructions That Are Not Allowed in Procedures ........................................................... 126
4.5 Avoiding Memory Bank Conflicts With the Assembly Optimizer ................................................... 127
4.5.1 Preventing Memory Bank Conflicts ........................................................................... 128
4.5.2 A Dot Product Example That Avoids Memory Bank Conflicts ............................................ 129
4.5.3 Memory Bank Conflicts for Indexed Pointers ................................................................ 132
4.5.4 Memory Bank Conflict Algorithm .............................................................................. 133
4.6 Memory Alias Disambiguation .......................................................................................... 133
4.6.1 How the Assembly Optimizer Handles Memory References (Default) ................................... 133
4.6.2 Using the --no_bad_aliases Option to Handle Memory References ..................................... 133
4.6.3 Using the .no_mdep Directive ................................................................................. 133
4.6.4 Using the .mdep Directive to Identify Specific Memory Dependencies .................................. 134
4.6.5 Memory Alias Examples ....................................................................................... 135
4
Contents SPRU187V–March 2014
Submit Documentation Feedback
Copyright © 2014, Texas Instruments Incorporated
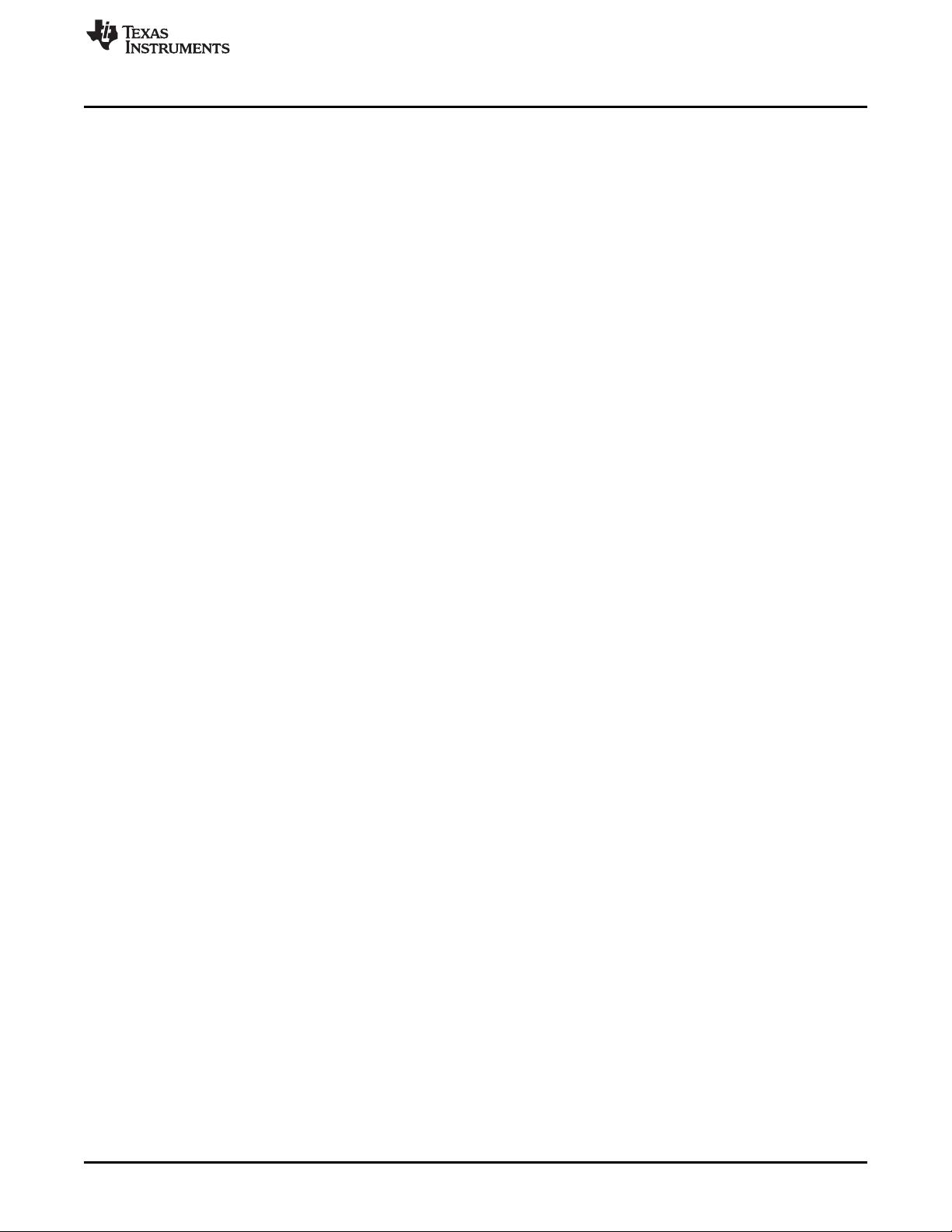
www.ti.com
5 Linking C/C++ Code ......................................................................................................... 137
5.1 Invoking the Linker Through the Compiler (-z Option) .............................................................. 138
5.1.1 Invoking the Linker Separately ................................................................................ 138
5.1.2 Invoking the Linker as Part of the Compile Step ............................................................ 139
5.1.3 Disabling the Linker (--compile_only Compiler Option) .................................................... 139
5.2 Linker Code Optimizations .............................................................................................. 140
5.2.1 Generating Function Subsections (--gen_func_subsections Compiler Option) ......................... 140
5.2.2 Conditional Linking .............................................................................................. 140
5.3 Controlling the Linking Process ........................................................................................ 141
5.3.1 Including the Run-Time-Support Library ..................................................................... 141
5.3.2 Run-Time Initialization .......................................................................................... 142
5.3.3 Global Object Constructors .................................................................................... 142
5.3.4 Specifying the Type of Global Variable Initialization ....................................................... 143
5.3.5 Specifying Where to Allocate Sections in Memory ......................................................... 143
5.3.6 A Sample Linker Command File .............................................................................. 145
6 TMS320C6000C/C++ Language Implementation ................................................................... 146
6.1 Characteristics of TMS320C6000 C ................................................................................... 147
6.1.1 Implementation-Defined Behavior ............................................................................ 147
6.2 Characteristics of TMS320C6000 C++ ................................................................................ 151
6.3 Using MISRA-C:2004 .................................................................................................... 152
6.4 Data Types ................................................................................................................ 153
6.4.1 Size of Enum Types ............................................................................................ 154
6.5 Keywords .................................................................................................................. 155
6.5.1 The complex Keyword .......................................................................................... 155
6.5.2 The const Keyword ............................................................................................. 155
6.5.3 The __cregister Keyword ...................................................................................... 156
6.5.4 The __interrupt Keyword ....................................................................................... 157
6.5.5 The __near and __far Keywords .............................................................................. 158
6.5.6 The restrict Keyword ............................................................................................ 159
6.5.7 The volatile Keyword ........................................................................................... 160
6.6 C++ Exception Handling ................................................................................................ 161
6.7 Register Variables and Parameters ................................................................................... 161
6.8 The __asm Statement ................................................................................................... 162
6.9 Pragma Directives ....................................................................................................... 163
6.9.1 The CHECK_MISRA Pragma ................................................................................. 164
6.9.2 The CLINK Pragma ............................................................................................. 164
6.9.3 The CODE_SECTION Pragma ............................................................................... 164
6.9.4 The DATA_ALIGN Pragma .................................................................................... 165
6.9.5 The DATA_MEM_BANK Pragma ............................................................................. 166
6.9.6 The DATA_SECTION Pragma ................................................................................ 167
6.9.7 The Diagnostic Message Pragmas ........................................................................... 168
6.9.8 The FUNC_ALWAYS_INLINE Pragma ...................................................................... 168
6.9.9 The FUNC_CANNOT_INLINE Pragma ...................................................................... 169
6.9.10 The FUNC_EXT_CALLED Pragma ......................................................................... 169
6.9.11 The FUNC_INTERRUPT_THRESHOLD Pragma ......................................................... 170
6.9.12 The FUNC_IS_PURE Pragma ............................................................................... 170
6.9.13 The FUNC_IS_SYSTEM Pragma ............................................................................ 171
6.9.14 The FUNC_NEVER_RETURNS Pragma ................................................................... 171
6.9.15 The FUNC_NO_GLOBAL_ASG Pragma ................................................................... 171
6.9.16 The FUNC_NO_IND_ASG Pragma ......................................................................... 172
6.9.17 The FUNCTION_OPTIONS Pragma ........................................................................ 172
6.9.18 The INTERRUPT Pragma .................................................................................... 172
6.9.19 The LOCATION Pragma ...................................................................................... 173
5
SPRU187V–March 2014 Contents
Submit Documentation Feedback
Copyright © 2014, Texas Instruments Incorporated