httpclient 工具类
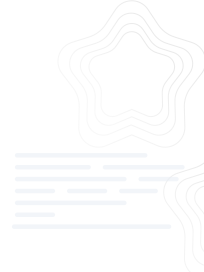

HttpClient是Apache基金会下的一个开源HTTP客户端库,广泛用于Java开发者执行HTTP请求。它提供了丰富的API,使得我们可以方便地实现GET、POST以及其他HTTP方法的操作。在本文中,我们将深入探讨HttpClient工具类的创建与使用,以及如何自定义返回的对象。 我们需要引入HttpClient的相关依赖。在Maven项目中,可以在pom.xml文件中添加以下依赖: ```xml <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.13</version> </dependency> ``` 接下来,我们创建一个名为`HttpUtilClient`的工具类,这个类将包含执行HTTP请求的方法。以下是一个基础的实现: ```java import org.apache.http.HttpEntity; import org.apache.http.client.config.RequestConfig; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.entity.StringEntity; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import org.apache.http.util.EntityUtils; import java.io.IOException; public class HttpUtilClient { private static final CloseableHttpClient httpClient = HttpClients.createDefault(); /** * 执行GET请求 * * @param url 请求的URL * @return 返回的HTTP响应体 * @throws IOException IO异常 */ public static String sendGetRequest(String url) throws IOException { HttpGet httpGet = new HttpGet(url); CloseableHttpResponse response = httpClient.execute(httpGet); try { HttpEntity entity = response.getEntity(); if (entity != null) { return EntityUtils.toString(entity, "UTF-8"); } else { return null; } } finally { response.close(); } } /** * 执行POST请求 * * @param url 请求的URL * @param param 请求参数 * @return 返回的HTTP响应体 * @throws IOException IO异常 */ public static String sendPostRequest(String url, String param) throws IOException { HttpPost httpPost = new HttpPost(url); httpPost.setEntity(new StringEntity(param, "UTF-8")); httpPost.setHeader("Content-type", "application/x-www-form-urlencoded"); CloseableHttpResponse response = httpClient.execute(httpPost); try { HttpEntity entity = response.getEntity(); if (entity != null) { return EntityUtils.toString(entity, "UTF-8"); } else { return null; } } finally { response.close(); } } // 可以根据需求扩展其他HTTP方法,如PUT、DELETE等 } ``` 在上述代码中,我们创建了两个静态方法:`sendGetRequest`和`sendPostRequest`。这两个方法分别用于执行GET和POST请求。它们都使用了`CloseableHttpClient`的`execute`方法来发送请求,并获取响应。注意,我们在处理完响应后要关闭资源,防止内存泄漏。 在实际应用中,你可能需要对请求进行更多的配置,例如设置超时时间、重试机制、添加请求头等。这时,你可以使用`RequestConfig`来定制请求配置。例如: ```java RequestConfig config = RequestConfig.custom() .setConnectTimeout(5000) .setSocketTimeout(5000) .build(); HttpGet httpGet = new HttpGet(url); httpGet.setConfig(config); ``` 至于返回的对象,描述中提到可以自定义。在上述示例中,我们简单地将响应体转换为字符串返回。如果需要更复杂的处理,例如解析JSON或XML,可以使用诸如Gson或Jackson这样的库来处理响应实体。 总结一下,HttpClient工具类为我们提供了一种简洁的方式去发送HTTP请求。通过它可以方便地执行GET、POST请求,并对请求进行各种定制。同时,我们可以根据需求自定义返回的对象,以便更好地处理请求结果。在实际开发中,HttpClient是实现Web服务交互的一个强大工具。
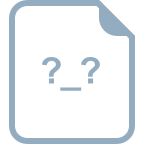
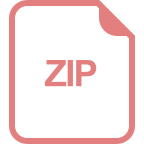
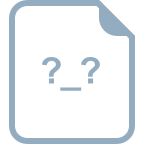
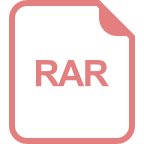
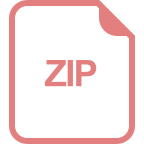
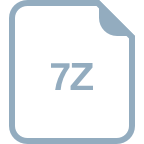
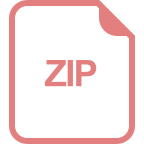
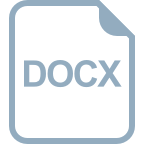
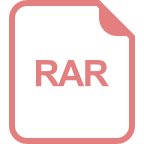
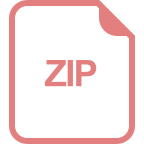
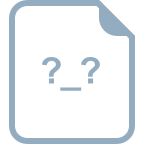
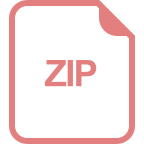

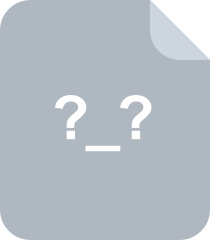
- 1
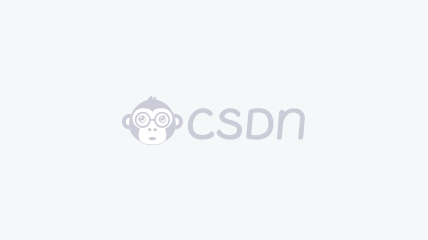

- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

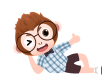
最新资源

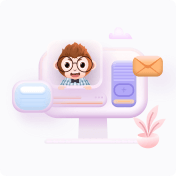
