[ENGLISH](./README_EN.md)
<h1 align="center">CRender</h1>
<p align="center">
<a href="https://travis-ci.com/jiaming743/CRender"><img src="https://img.shields.io/travis/com/jiaming743/CRender.svg" alt="Travis CI"></a>
<a href="https://github.com/jiaming743/CRender/blob/master/LICENSE"><img src="https://img.shields.io/github/license/jiaming743/CRender.svg" alt="LICENSE" /></a>
<a href="https://www.npmjs.com/package/@jiaminghi/c-render"><img src="https://img.shields.io/npm/v/@jiaminghi/c-render.svg" alt="version" /></a>
</p>
### CRender是干什么的?
- 它是一个基于**canvas**的**矢量**图形渲染插件。
- 它对图形提供动画和鼠标事件支持。
### npm安装
```shell
$ npm install @jiaminghi/c-render
```
### 快速体验
```html
<!--资源位于个人服务器仅供体验和测试,请勿在生产环境使用-->
<!--调试版-->
<script src="http://lib.jiaminghi.com/crender/crender.map.js"></script>
<!--压缩版-->
<script src="http://lib.jiaminghi.com/crender/crender.min.js"></script>
<script>
const { CRender, extendNewGraph } = window.CRender
// do something
</script>
```
详细文档及示例请移步[HomePage](http://crender.jiaminghi.com).
- [使用](#使用)
- [Class CRender](#class-crender)
- [Class Graph](#class-graph)
- [Class Style](#class-style)
- [示例](#示例)
- [扩展新图形](#扩展新图形)
- [相关支持](#相关支持)
------
<h3 align="center">使用</h3>
```javascript
import CRender from '@jiaminghi/c-redner'
const canvas = document.getElementById('canvas')
// 实例化 CRender
const render = new CRender(canvas)
// 向render中添加图形
const circle = render.add({ name: 'circle', ... })
```
<h3 align="center">Class CRender</h3>
### 类
```javascript
/**
* @description Class of CRender
* @param {Object} canvas Canvas 节点
* @return {CRender} CRender实例
*/
class CRender {
// ...
}
```
### 实例属性
- [ctx](#ctx)
- [area](#area)
- [animationStatus](#animationStatus)
- [graphs](#graphs)
- [color](#color)
- [bezierCurve](#bezierCurve)
#### ctx
```javascript
/**
* @description canvas context
* @type {Object}
* @example ctx = canvas.getContext('2d')
*/
```
#### area
```javascript
/**
* @description canvas宽高
* @type {Array<Number>}
* @example area = [300,100]
*/
```
#### animationStatus
```javascript
/**
* @description render是否处于动画渲染中
* @type {Boolean}
* @example animationStatus = true|false
*/
```
#### graphs
```javascript
/**
* @description 已添加的图形
* @type {Array<Graph>}
* @example graphs = [Graph, Graph, ...]
*/
```
#### [color](https://github.com/jiaming743/color)
```javascript
/**
* @description 颜色插件
* @type {Object}
*/
```
#### [bezierCurve](https://github.com/jiaming743/BezierCurve)
```javascript
/**
* @description 贝塞尔曲线插件
* @type {Object}
*/
```
### 原型方法
- [add](#add)
向render中添加图形
- [clone](#clone)
克隆一个图形
- [delGraph](#delGraph)
删除render中的一个图形
- [delAllGraph](#delAllGraph)
删除render中所有的图形
- [drawAllGraph](#drawAllGraph)
渲染render中所有的图形
- [clearArea](#clearArea)
擦除canvas绘制区域
- [launchAnimation](#launchAnimation)
使动画队列不为空且animationPause不为false的图形进行动画
#### add
```javascript
/**
* @description 向render中添加图形
* @param {Object} config 图形配置
* @return {Graph} 图形实例
*/
CRender.prototype.add = function (config = {}) {
// ...
}
```
#### Clone
```javascript
/**
* @description 克隆一个图形
* @param {Graph} graph 将要被克隆的图形
* @return {Graph} 克隆的图形
*/
CRender.prototype.clone = function (graph) {
}
```
#### delGraph
```javascript
/**
* @description 删除render中的一个图形
* @param {Graph} graph 将要删除的图形实例
* @return {Undefined} 无返回值
*/
CRender.prototype.delGraph = function (graph) {
// ...
}
```
#### delAllGraph
```javascript
/**
* @description 删除render中所有的图形
* @return {Undefined} 无返回值
*/
CRender.prototype.delAllGraph = function () {
// ...
}
```
#### drawAllGraph
```javascript
/**
* @description 渲染render中所有的图形
* @return {Undefined} 无返回值
*/
CRender.prototype.drawAllGraph = function () {
// ...
}
```
#### clearArea
```javascript
/**
* @description 擦除canvas绘制区域
* @return {Undefined} 无返回值
*/
CRender.prototype.clearArea = function () {
// ...
}
```
#### launchAnimation
```javascript
/**
* @description 使动画队列不为空且animationPause不为false的图形进行动画
* @return {Promise} Animation Promise
*/
CRender.prototype.launchAnimation = function () {
// ...
}
```
<h3 align="center">Class Graph</h3>
### 实例属性
**当添加一个图形时,你可以配置这些属性。**
- [visible](#visible)
- [shape](#shape)
- [style](#style)
- [drag](#drag)
- [hover](#hover)
- [index](#index)
- [animationDelay](#animationDelay)
- [animationFrame](#animationFrame)
- [animationCurve](#animationCurve)
- [animationPause](#animationPause)
- [hoverRect](#hoverRect)
- [mouseEnter](#mouseEnter)
- [mouseOuter](#mouseOuter)
- [click](#click)
#### visible
```javascript
/**
* @description 该图形是否可被渲染
* @type {Boolean}
* @default visible = true
*/
```
#### shape
```javascript
/**
* @description 图形形状数据
* @type {Object}
*/
```
#### [style](#Class-Style)
```javascript
/**
* @description 图形样式数据 (Style实例)
* @type {Style}
*/
```
#### drag
```javascript
/**
* @description 是否启用拖拽功能
* @type {Boolean}
* @default drag = false
*/
```
#### hover
```javascript
/**
* @description 是否启用悬浮检测
* @type {Boolean}
* @default hover = false
*/
```
#### index
```javascript
/**
* @description 图形渲染层级,层级高者优先渲染
* @type {Number}
* @default index = 1
*/
```
#### animationDelay
```javascript
/**
* @description 动画延迟时间(ms)
* @type {Number}
* @default animationDelay = 0
*/
```
#### animationFrame
```javascript
/**
* @description 动画帧数
* @type {Number}
* @default animationFrame = 30
*/
```
#### [animationCurve](https://github.com/jiaming743/transition)
```javascript
/**
* @description 动画缓动曲线
* @type {String}
* @default animationCurve = 'linear'
*/
```
#### animationPause
```javascript
/**
* @description 是否暂停图形动画
* @type {Boolean}
* @default animationPause = false
*/
```
#### hoverRect
```javascript
/**
* @description 矩形悬浮检测盒,配置该项则优先使用其进行鼠标悬浮检测
* @type {Null|Array<Number>}
* @default hoverRect = null
* @example hoverRect = [0, 0, 100, 100] // [矩形起始点 x, y 坐标, 矩形宽, 高]
*/
```
#### mouseEnter
```javascript
/**
* @description 鼠标进入图形事件处理器
* @type {Null|Function}
* @default mouseEnter = null
*/
```
#### mouseOuter
```javascript
/**
* @description 鼠标移出图形事件处理器
* @type {Null|Function}
* @default mouseOuter = null
*/
```
#### click
```javascript
/**
* @description 鼠标点击图形事件处理器
* @type {Null|Function}
* @default click = null
*/
```
#### Tip
启用图形的**mouseEnter**,**mouseOuter**,**click**等事件支持需要将`hover`属性配置为`true`。扩展的新图形需要配置**hoverCheck**方法以提供事件支持。
### 原型方法
- [attr](#attr)
更新图形状态
- [animat
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
官网直接安装的不支持vite+vue2的 主要修复: 1.build或者dev项目时不报错,兼容vite2,vue2; 2.加入deep监听watch,直接在父组件中修改图表中的config参数即可完成图表中的数据变更。 yarn npm cnpm pnpm可通用的,其实就是底层node_modules位置不一样而已,不过修改原理跟代码基本都是一直的,大家可以自行下载研究,windows macos linux均兼容。如果下载错了请私信我。 下面说下安装步骤: 1.在项目更目录中安装,执行pnpm install @jiaminghi/data-view即可; 2.https://github.com/jiaming743/Color查看最新的color版本号,压缩包里版本号未1.1.3,如果不是,这替换.pnpm下@jiaminghi+color@1.1.3文件夹名称,改为最新版本号。 3.将other_modules压缩包解压并覆盖到项目的node_modules文件夹下即可 调用方法,请参考官网文档http://datav.jiaminghi.com/
资源推荐
资源详情
资源评论
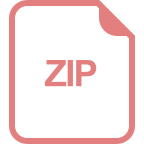
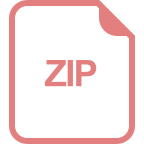
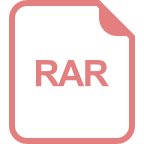
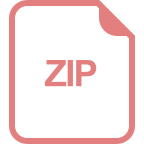
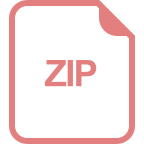
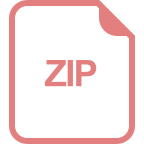
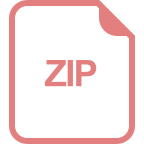
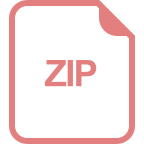
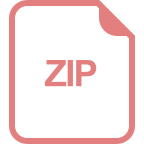
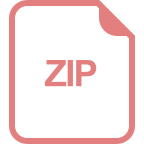
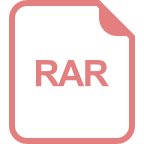
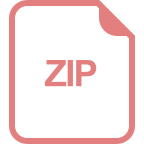
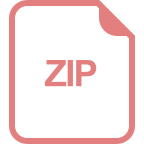
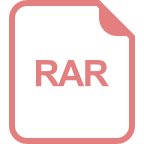
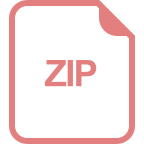
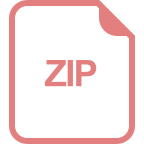
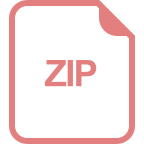
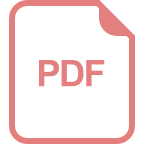
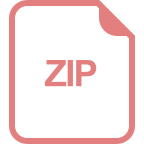
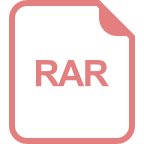
收起资源包目录

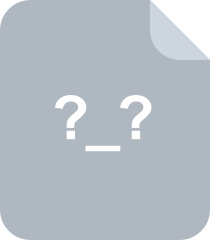
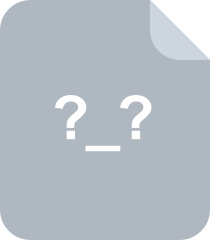
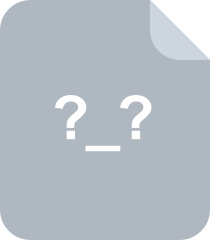
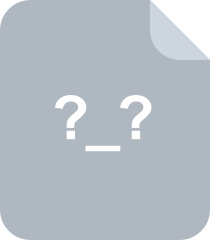
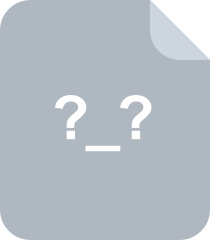
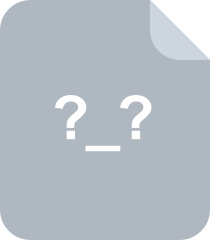
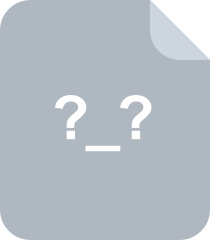
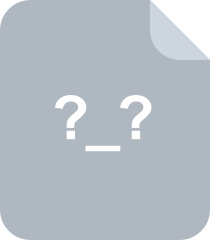
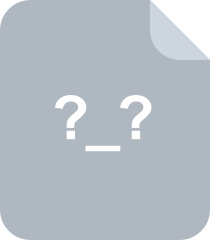
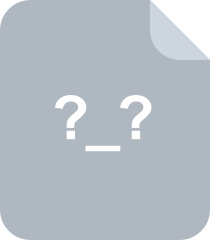
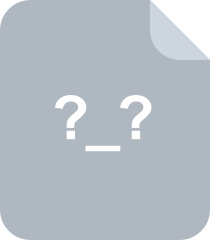
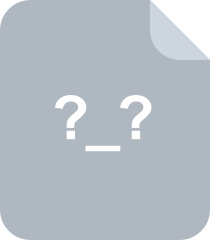
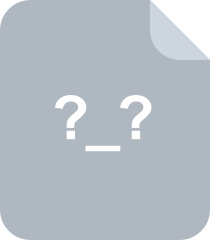
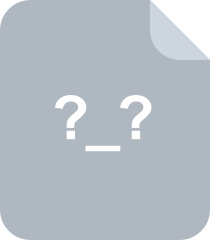
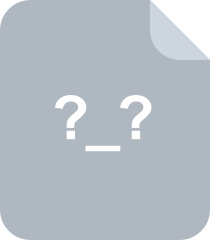
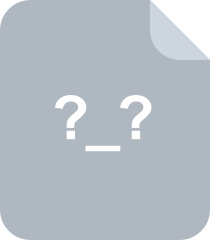
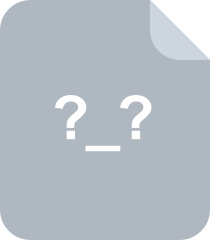
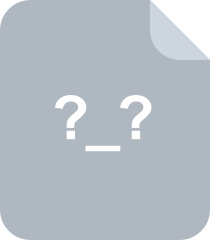
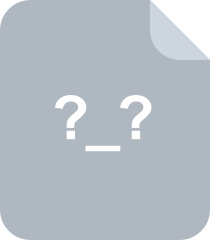
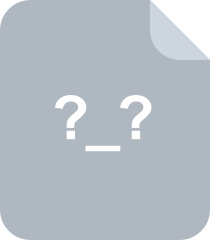
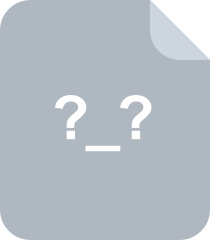
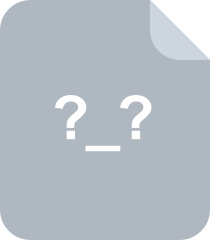
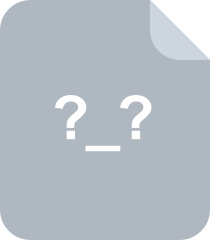
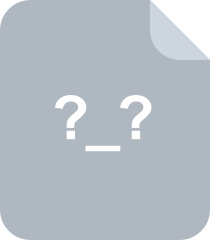
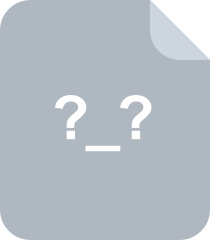
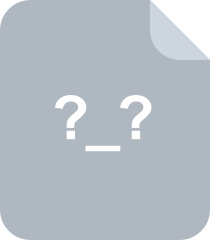
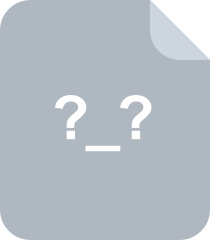
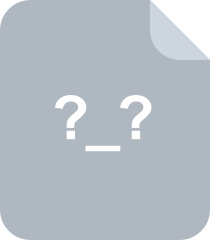
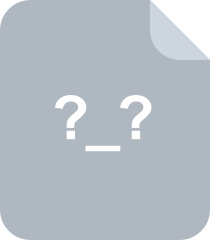
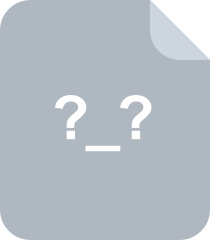
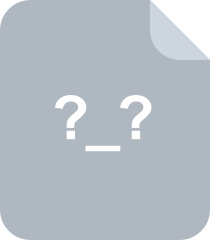
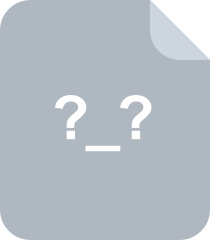
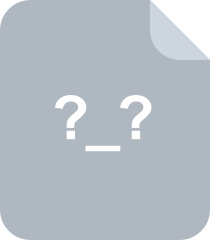
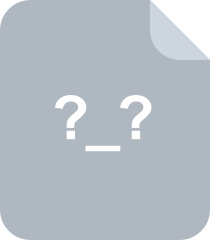
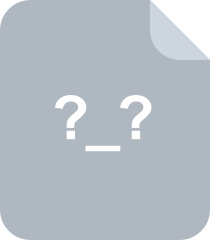
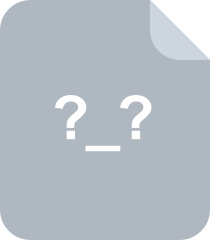
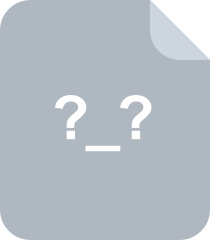
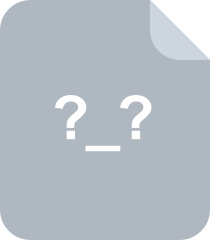
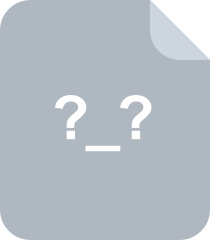
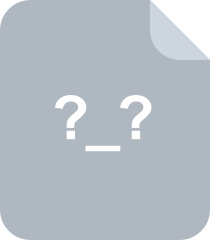
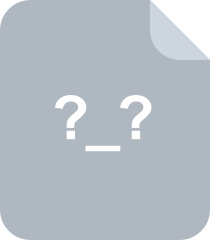
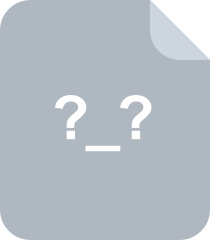
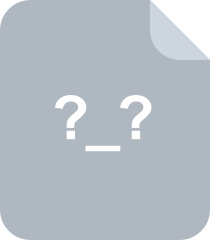
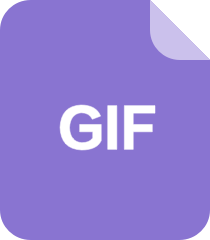
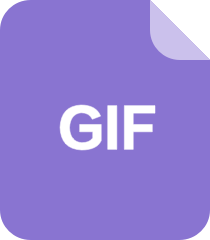
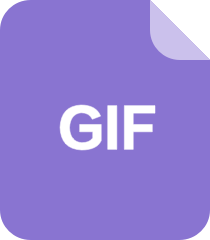
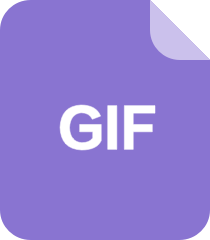
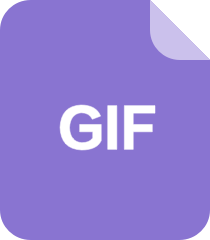
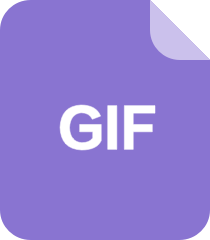
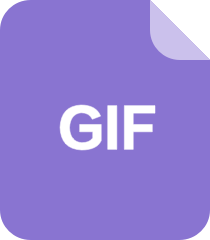
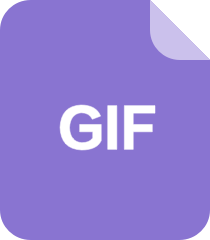
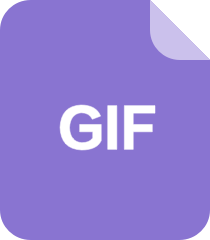
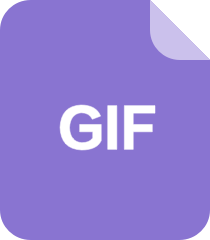
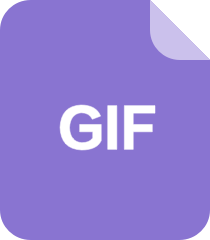
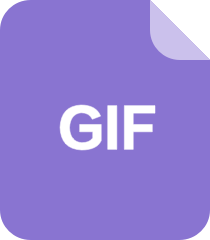
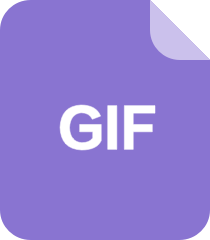
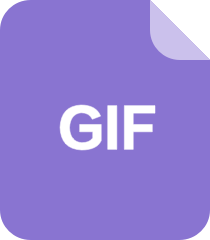
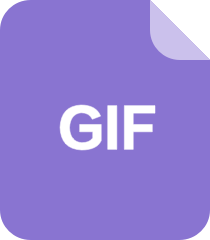
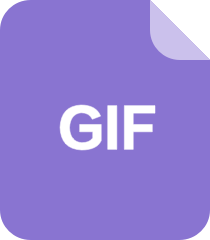
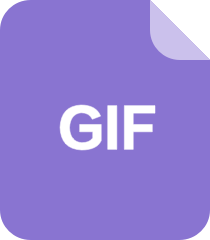
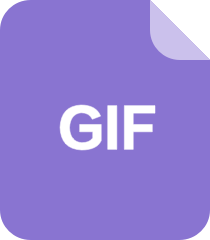
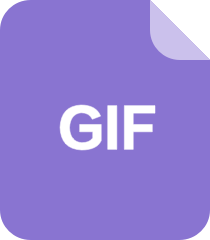
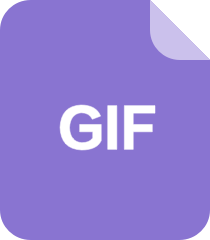
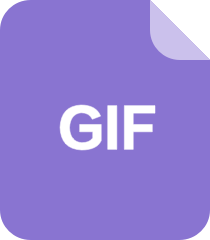
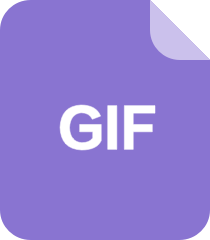
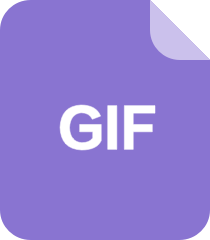
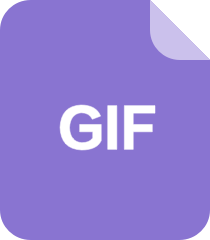
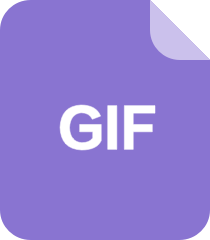
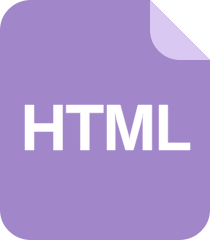
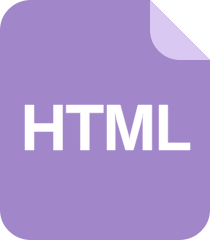
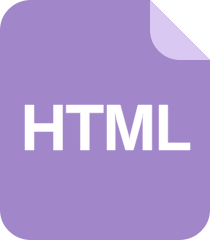
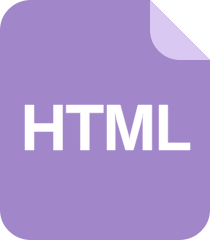
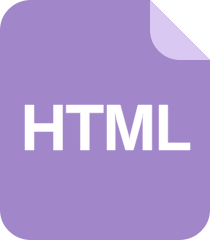
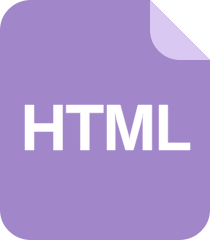
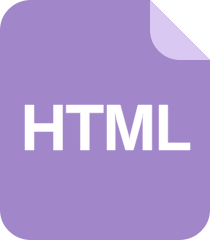
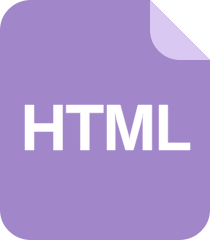
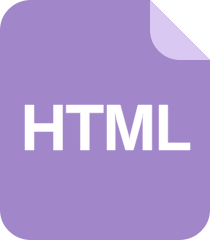
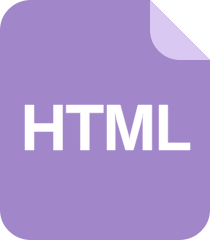
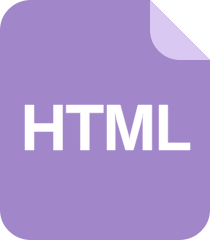
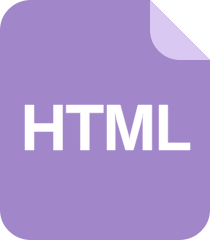
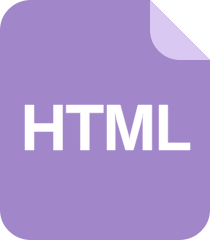
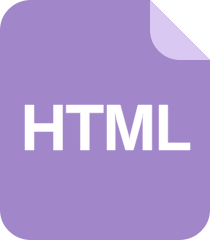
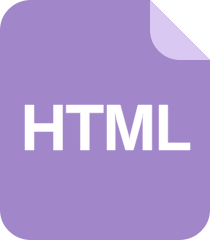
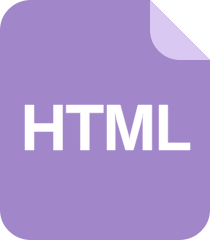
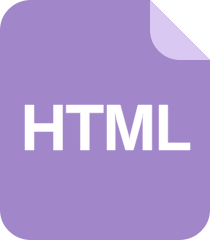
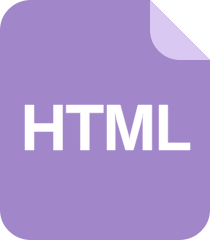
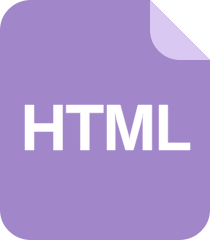
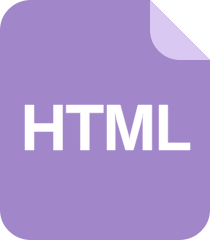
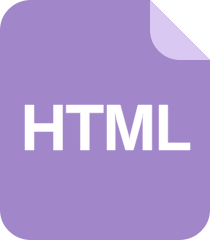
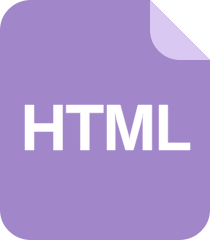
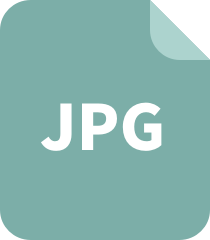
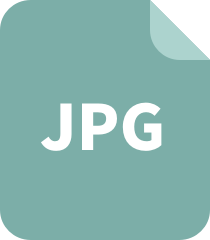
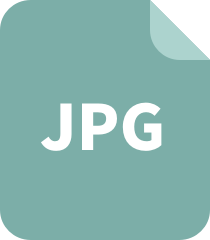
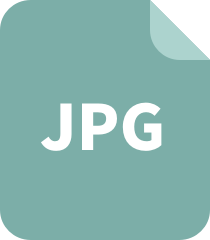
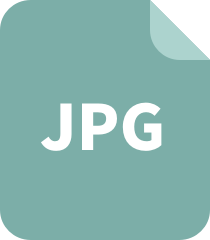
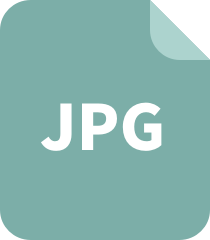
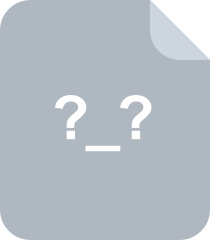
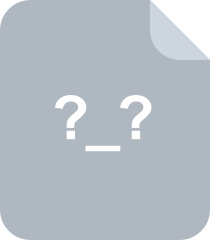
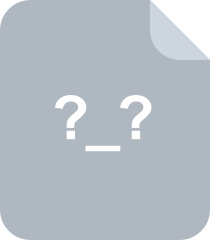
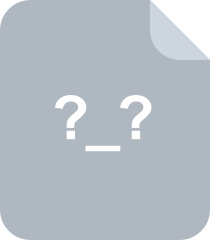
共 455 条
- 1
- 2
- 3
- 4
- 5
资源评论
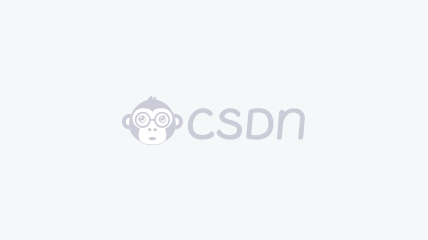

多留活口少挖坑
- 粉丝: 17
- 资源: 33
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

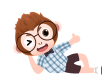
安全验证
文档复制为VIP权益,开通VIP直接复制
