package com.action;
import java.io.*;
import java.util.List;
import java.util.StringTokenizer;
import org.apache.struts.action.*;
import com.dao.SongDAO;
import com.model.SongForm;
import com.model.SongTypeForm;
import javax.servlet.RequestDispatcher;
import javax.servlet.ServletException;
import javax.servlet.http.*;
import com.tools.MyPagination;
import com.tools.StringUtils;
public class SongAction extends Action {
private SongDAO songDAO = null;
MyPagination pagination = null;
StringUtils su=new StringUtils();
public SongAction() {
this.songDAO = new SongDAO();
}
public ActionForward execute(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
String action = request.getParameter("action");
// System.out.println("\nscrip*********************action="+action);
if ("main".equals(action)) {
return main(mapping, form, request, response); // 前台首页
} else if ("songQuery".equals(action)) {
return songQuery(mapping, form, request, response); // 查询歌曲信息
} else if ("tryListen".equals(action)) {
return tryListen(mapping, form, request, response); // 查询试听歌曲信息
} else if ("continuePlay".equals(action)) {
return continuePlay(mapping, form, request, response); // 进行歌曲连播
} else if ("songSort".equals(action)) {
return songSort(mapping, form, request, response); // 歌曲排行(试听和下载)
} else if ("navigation".equals(action)) {
return navigation(mapping, form, request, response); // 查询导航栏信息
} else if ("search".equals(action)) {
return search(mapping, form, request, response); // 按条件查询歌曲
} else if ("download".equals(action)) {
return download(mapping, form, request, response); // 文件下载
} else if ("songType".equals(action)) {
return songType(mapping, form, request, response); // 查询歌曲类别
} else if ("adm_search".equals(action)) {
return adm_search(mapping, form, request, response); // 后台查询歌曲信息
} else if ("add".equals(action)){
return adm_add(mapping,form,request,response); //添加歌曲信息
} else if ("checkMusic".equals(action)){
return checkMusic(mapping,form,request,response); //检测歌曲是否已经添加
} else if ("del".equals(action)) {
return del(mapping, form, request, response); // 删除歌曲信息
} else {
request.setAttribute("error", "操作失败!");
return mapping.findForward("error");
}
}
// 主页中的新歌速递
public ActionForward main(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
List<SongTypeForm> list = songDAO.queryType(); // 获取歌曲类别
int songTypeId = 0;
String[][] typeName = new String[6][2];
for (int i = 0; i < list.size(); i++) {
songTypeId = list.get(i).getId();
typeName[i][0] = String.valueOf(list.get(i).getId());
typeName[i][1] = list.get(i).getTypeName();
request.setAttribute("newSongList" + i, songDAO
.query("WHERE songType=" + songTypeId
+ " ORDER BY upTime DESC",5)); // 获取最新上传的5首歌曲
}
request.setAttribute("typeArray", typeName); // 保存类别信息数组到Request中
return mapping.findForward("main");
}
// 歌曲排行
public ActionForward songSort(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
String type = request.getParameter("sortType"); //获取表示是试听排行还是下载排行的参数值
if ("hits".equals(type)) {
request.setAttribute("sortType", songDAO
.query(" ORDER BY hits DESC",8)); // 获取试听排行信息
} else if ("download".equals(type)) {
request.setAttribute("sortType", songDAO
.query(" ORDER BY download DESC",8)); // 获取下载排行信息
}
request.setAttribute("sortTypeName", type);
RequestDispatcher requestDispatcher = request
.getRequestDispatcher("/songSort.jsp"); //将页面重定向到歌曲排行榜页面
try {
requestDispatcher.include(request, response); // 此处不能使用forward()方法
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
// 获取导航栏信息
public ActionForward navigation(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
request.setAttribute("navigation", songDAO.queryType()); // 获取歌曲类别信息
RequestDispatcher requestDispatcher = request
.getRequestDispatcher("/navigation.jsp");
try {
requestDispatcher.include(request, response); // 此处不能使用forward()方法
} catch (ServletException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
// 查找歌曲列表
public ActionForward songQuery(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
String strPage = (String) request.getParameter("Page");
int songType = Integer.parseInt(request.getParameter("songType_more")); // 要查看的歌曲类别
int Page = 1;
List<SongForm> list = null;
if (strPage == null) {
pagination = new MyPagination();
list = songDAO.query(" WHERE s.songType=" + songType
+ " ORDER BY s.upTime DESC"); // 获取歌曲信息
int pagesize = 2; // 指定每页显示的记录数
list = pagination.getInitPage(list, Page, pagesize); // 初始化分页信息
request.getSession().setAttribute("pagination", pagination);
} else {
pagination = (MyPagination) request.getSession().getAttribute(
"pagination");
Page = pagination.getPage(strPage);
list = pagination.getAppointPage(Page); // 获取指定页数据
}
if (list.size() > 0) {
request.setAttribute("typeName", list.get(0).getSongType()); // 获取歌曲类别
request.setAttribute("typeID", list.get(0).getSongTypeId()); // 获取歌曲类别ID
}
request.setAttribute("songList", list); // 保存当前页的歌曲信息
request.setAttribute("Page", Page); // 保存的当前页码
return mapping.findForward("songQuery");
}
// 按条件查询歌曲
public ActionForward search(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
String strPage = (String) request.getParameter("Page");
int songType = Integer.parseInt(request.getParameter("songType_more")); // 要查看的歌曲类别
String fieldName = request.getParameter("fieldName"); // 获取查询依据
String key = request.getParameter("key"); // 获取查询关键字
System.out.println("获取的查询条件:" + key + fieldName + songType);
String condition = "";
String fieldName_cn = "";
if (songType > 0 && fieldName != null) {
condition = " WHERE s.songType=" + songType + " AND s." + fieldName
+ " LIKE '%" + key + "%' ORDER BY s.upTime DESC";
} else if (songType > 0) {
condition = " WHERE s.songType=" + songType
+ " ORDER BY s.upTime DESC";
} else if (fieldName != null) {
condition = " WHERE s." + fieldName + " LIKE '%" + key
+ "%' ORDER BY s.upTime DESC";
} else {
condition = " ORDER BY s.upTime DESC";
}
if ("songName".equals(fieldName)) {
fieldName_cn = "歌曲名";
} else if ("specialName".equals(fieldName)) {
fieldName_cn = "专辑";
} else if ("singer".equals(fieldName)) {
fieldName_cn = "歌手";
}
int Page = 1;
List<SongForm> list = null;
if (strPage == null) {
pagination = new MyPagination();
list = songDAO.query(condition); // 获取歌曲信息
int pagesize = 2; // 指定每页显示的记录数
list = pagination.getInitPage(list, Page, pagesize); // 初始化分页信息
request.getSession().setAttribute("pagination", pagination);
} else {
pagination = (MyPagination) request.getSession().getAttribute(
"pagination");
Page = pagination.getPage(strPage);
list = pagination.getAppointPage(Page); // 获取指定页数据
}
if (list.size() > 0) {
if (songType > 0) {
// request.set
没有合适的资源?快使用搜索试试~ 我知道了~
JavaWeb项目-悦声音乐网站.zip
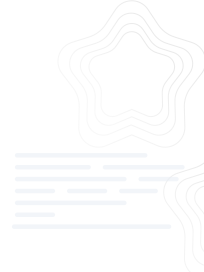
共107个文件
jsp:29个
jar:16个
java:11个

需积分: 14 2 下载量 26 浏览量
2023-03-22
11:18:35
上传
评论 1
收藏 10.58MB ZIP 举报
温馨提示
JSP项目,可作为小项目练习使用
资源推荐
资源详情
资源评论
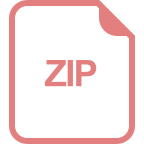
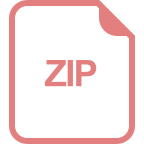
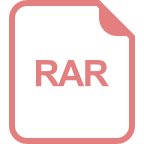
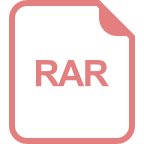
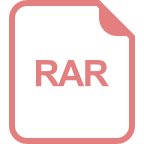
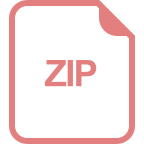
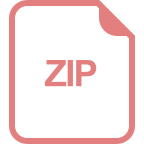
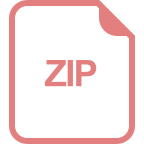
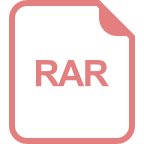
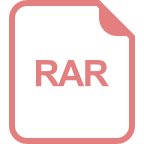
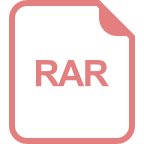
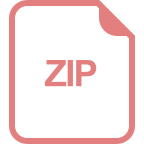
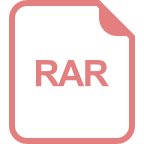
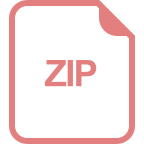
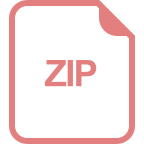
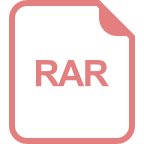
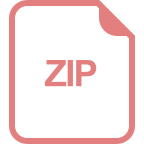
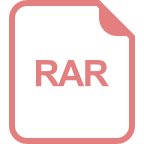
收起资源包目录

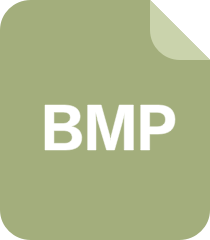
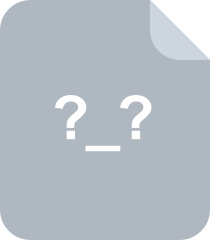
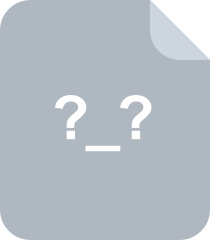
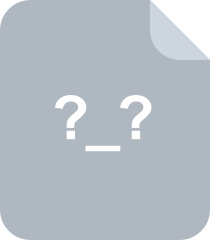
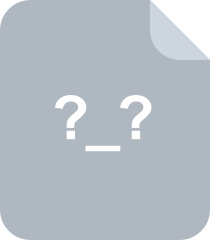
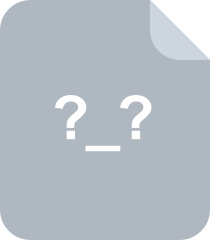
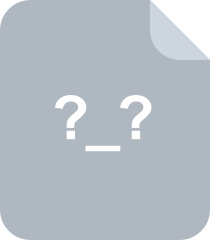
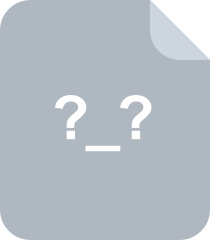
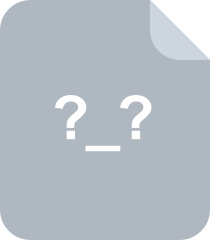
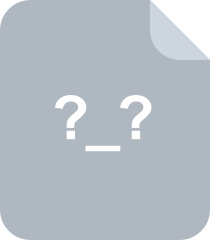
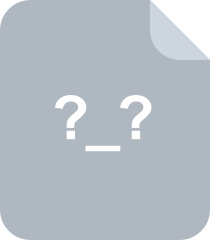
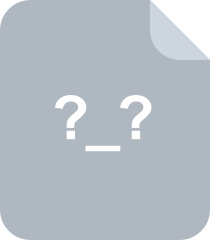
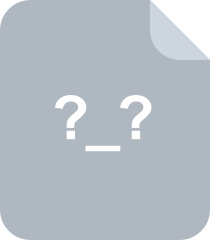
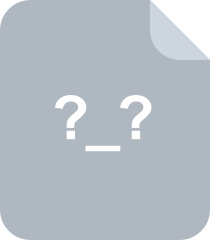
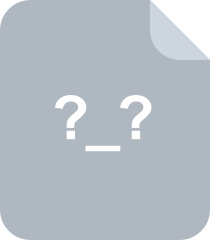
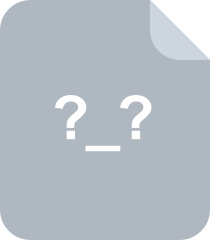
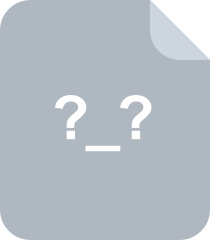
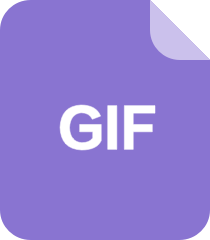
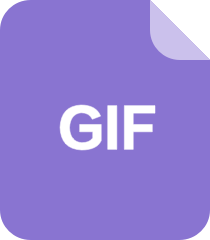
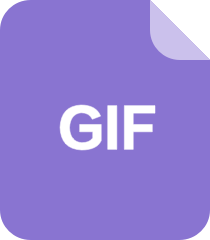
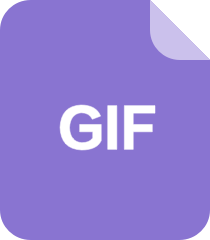
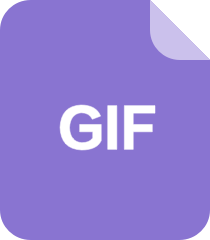
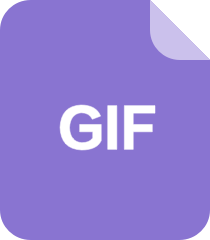
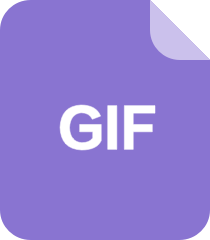
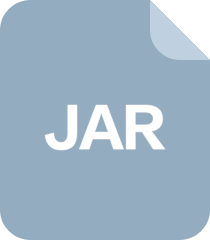
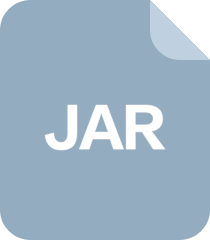
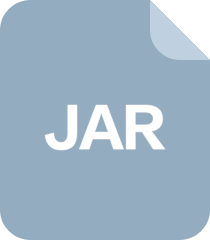
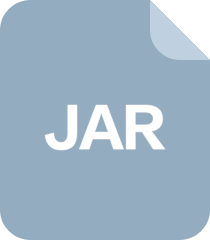
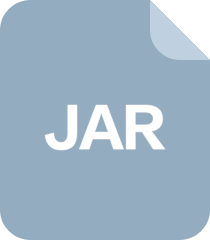
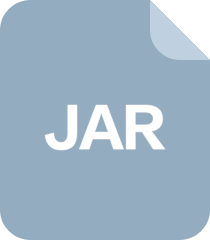
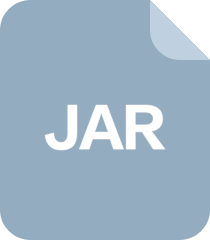
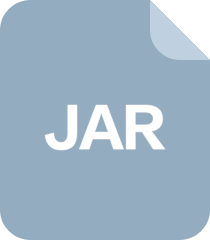
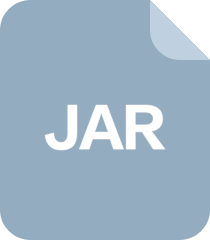
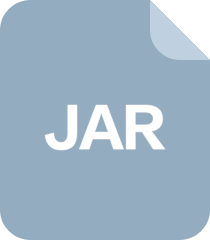
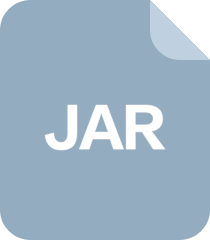
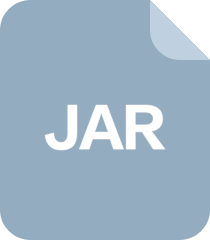
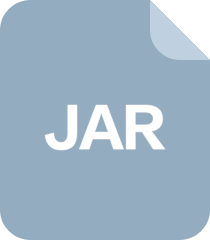
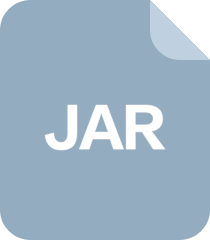
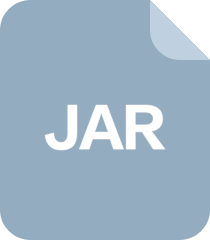
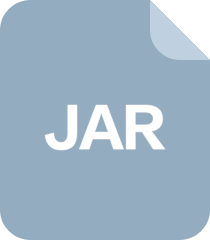
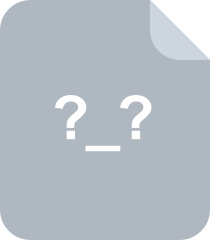
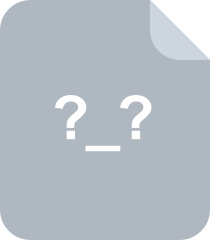
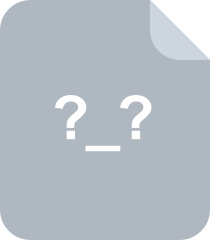
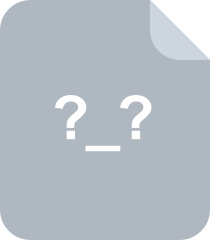
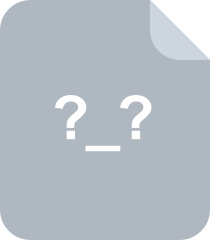
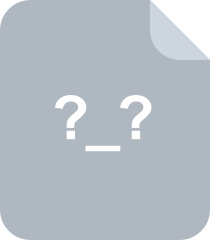
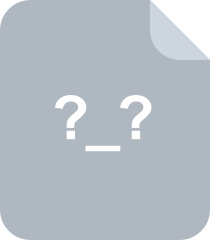
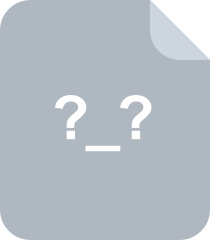
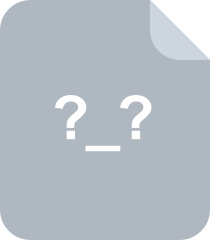
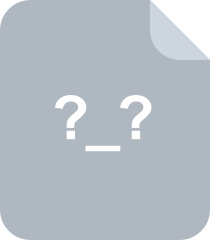
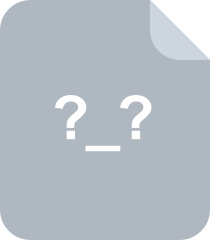
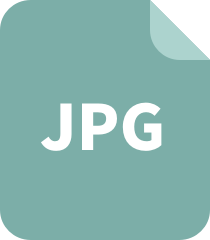
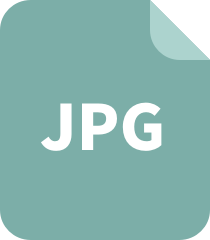
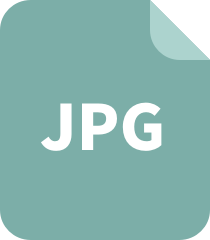
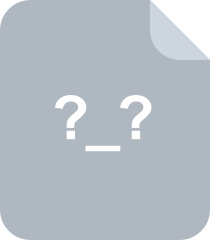
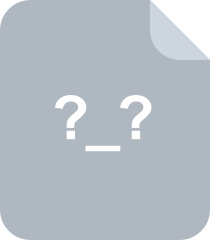
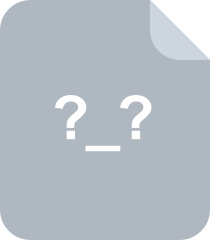
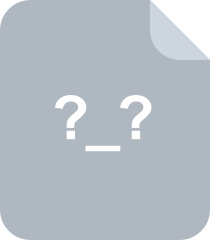
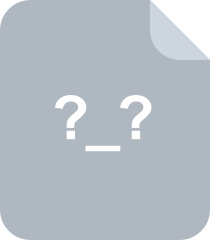
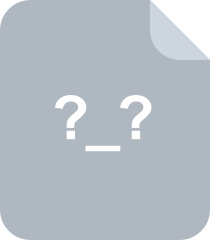
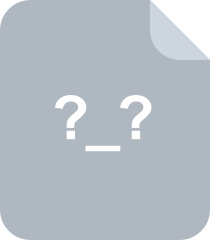
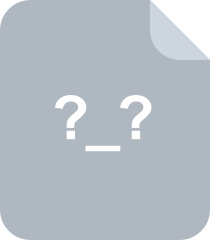
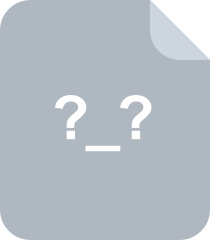
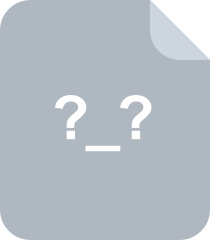
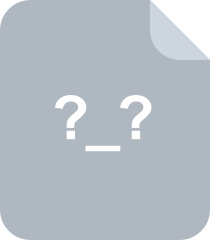
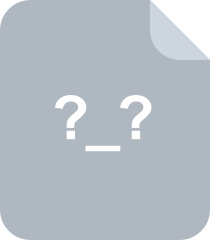
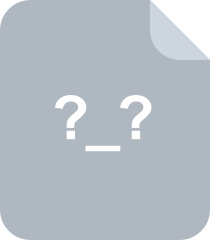
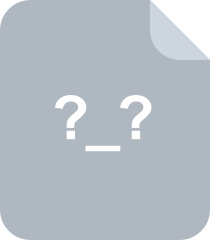
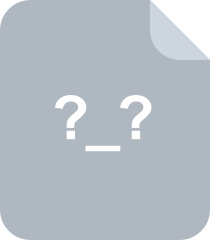
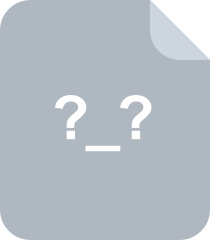
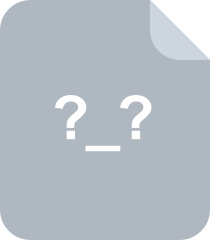
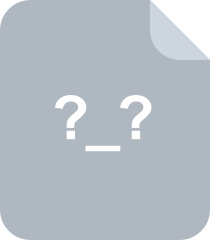
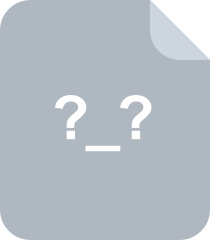
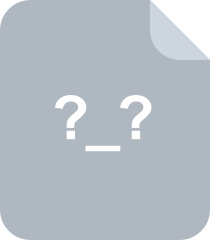
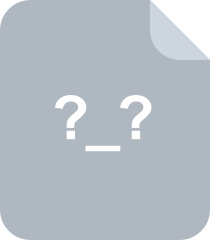
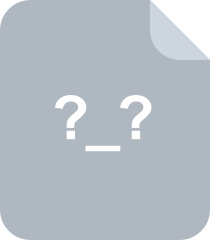
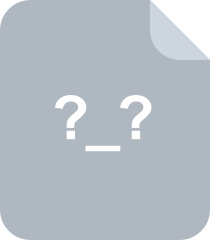
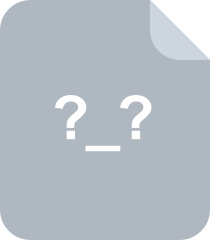
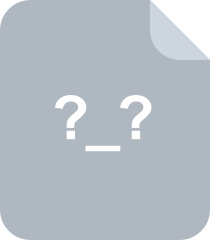
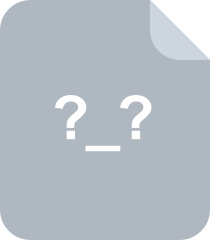
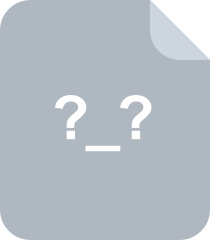
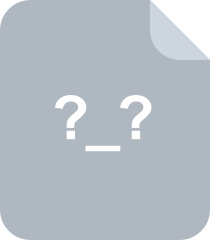
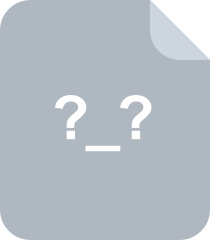
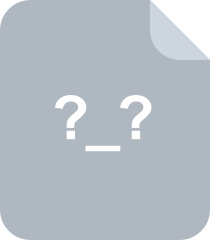
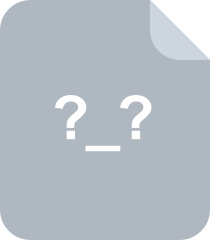
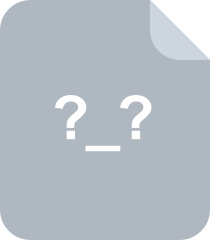
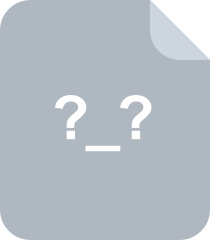
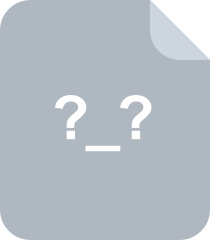
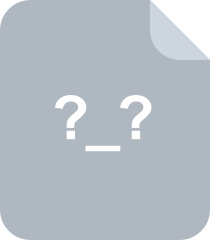
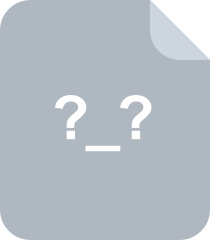
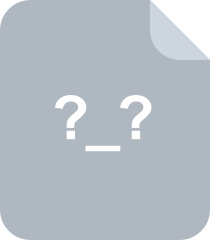
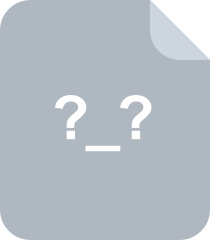
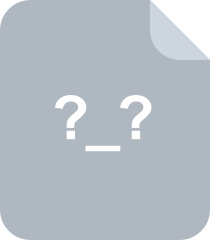
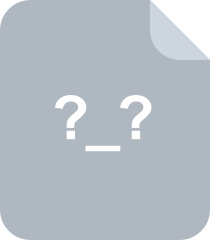
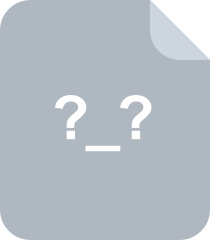
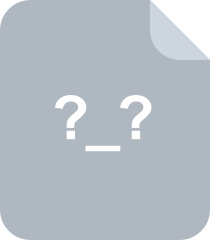
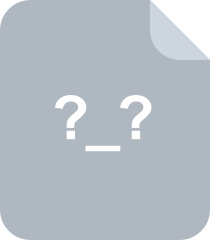
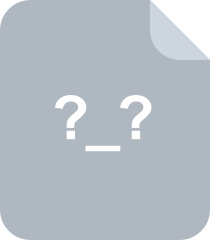
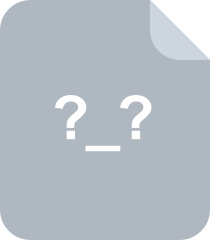
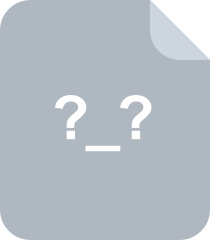
共 107 条
- 1
- 2
资源评论
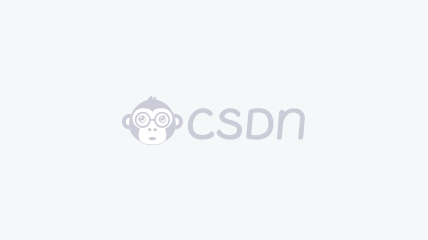

疏竹
- 粉丝: 21
- 资源: 30
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

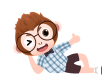
最新资源
- 单片机常用芯片和器件手册.zip
- C51论坛离线浏览资料.chm
- 单片机指令速查表.doc
- 设定80C51串行异步通讯的波特率.zip
- 创新MOM培训文档_物料主数据之包材_240625.pptx
- 医学图像分类数据集:CT胸部扫描癌症分类(4分类)【包括划分好的数据、类别字典文件、python数据可视化脚本 】
- 基于C51单片机设计四位数字频率计数码管显示实验Proteus仿真及软件实例源码.zip
- 基于C51单片机设计MAX7221数码管动态显示程序Proteus仿真及软件实例源码.zip
- DS18B20温度传感器实战应用与源码解析.zip
- python-leetcode面试题解之第384题打乱数组.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


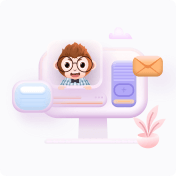
安全验证
文档复制为VIP权益,开通VIP直接复制
