/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.struts2.jasper.compiler;
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.Enumeration;
import java.util.Hashtable;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Vector;
import javax.el.MethodExpression;
import javax.el.ValueExpression;
import javax.servlet.jsp.tagext.TagAttributeInfo;
import javax.servlet.jsp.tagext.TagInfo;
import javax.servlet.jsp.tagext.TagVariableInfo;
import javax.servlet.jsp.tagext.VariableInfo;
import org.apache.struts2.jasper.Constants;
import org.apache.struts2.jasper.JasperException;
import org.apache.struts2.jasper.JspCompilationContext;
import org.apache.struts2.jasper.compiler.Node.NamedAttribute;
import org.apache.struts2.jasper.runtime.JspRuntimeLibrary;
import org.apache.struts2.JSPRuntime;
import org.xml.sax.Attributes;
/**
* Generate Java source from Nodes
*
* @author Anil K. Vijendran
* @author Danno Ferrin
* @author Mandar Raje
* @author Rajiv Mordani
* @author Pierre Delisle
*
* Tomcat 4.1.x and Tomcat 5:
* @author Kin-man Chung
* @author Jan Luehe
* @author Shawn Bayern
* @author Mark Roth
* @author Denis Benoit
*
* Tomcat 6.x
* @author Jacob Hookom
* @author Remy Maucherat
*/
class Generator {
private static final Class[] OBJECT_CLASS = { Object.class };
private static final String VAR_EXPRESSIONFACTORY =
System.getProperty("org.apache.struts2.jasper.compiler.Generator.VAR_EXPRESSIONFACTORY", "_el_expressionfactory");
private static final String VAR_ANNOTATIONPROCESSOR =
System.getProperty("org.apache.struts2.jasper.compiler.Generator.VAR_ANNOTATIONPROCESSOR", "_jsp_annotationprocessor");
private ServletWriter out;
private ArrayList methodsBuffered;
private FragmentHelperClass fragmentHelperClass;
private ErrorDispatcher err;
private BeanRepository beanInfo;
private JspCompilationContext ctxt;
private boolean isPoolingEnabled;
private boolean breakAtLF;
private String jspIdPrefix;
private int jspId;
private PageInfo pageInfo;
private Vector<String> tagHandlerPoolNames;
private GenBuffer charArrayBuffer;
/**
* @param s
* the input string
* @return quoted and escaped string, per Java rule
*/
static String quote(String s) {
if (s == null)
return "null";
return '"' + escape(s) + '"';
}
/**
* @param s
* the input string
* @return escaped string, per Java rule
*/
static String escape(String s) {
if (s == null)
return "";
StringBuffer b = new StringBuffer();
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if (c == '"')
b.append('\\').append('"');
else if (c == '\\')
b.append('\\').append('\\');
else if (c == '\n')
b.append('\\').append('n');
else if (c == '\r')
b.append('\\').append('r');
else
b.append(c);
}
return b.toString();
}
/**
* Single quote and escape a character
*/
static String quote(char c) {
StringBuffer b = new StringBuffer();
b.append('\'');
if (c == '\'')
b.append('\\').append('\'');
else if (c == '\\')
b.append('\\').append('\\');
else if (c == '\n')
b.append('\\').append('n');
else if (c == '\r')
b.append('\\').append('r');
else
b.append(c);
b.append('\'');
return b.toString();
}
private String createJspId() throws JasperException {
if (this.jspIdPrefix == null) {
StringBuffer sb = new StringBuffer(32);
String name = ctxt.getServletJavaFileName();
sb.append("jsp_").append(Math.abs(name.hashCode())).append('_');
this.jspIdPrefix = sb.toString();
}
return this.jspIdPrefix + (this.jspId++);
}
/**
* Generates declarations. This includes "info" of the page directive, and
* scriptlet declarations.
*/
private void generateDeclarations(Node.Nodes page) throws JasperException {
class DeclarationVisitor extends Node.Visitor {
private boolean getServletInfoGenerated = false;
/*
* Generates getServletInfo() method that returns the value of the
* page directive's 'info' attribute, if present.
*
* The Validator has already ensured that if the translation unit
* contains more than one page directive with an 'info' attribute,
* their values match.
*/
public void visit(Node.PageDirective n) throws JasperException {
if (getServletInfoGenerated) {
return;
}
String info = n.getAttributeValue("info");
if (info == null)
return;
getServletInfoGenerated = true;
out.printil("public String getServletInfo() {");
out.pushIndent();
out.printin("return ");
out.print(quote(info));
out.println(";");
out.popIndent();
out.printil("}");
out.println();
}
public void visit(Node.Declaration n) throws JasperException {
n.setBeginJavaLine(out.getJavaLine());
out.printMultiLn(new String(n.getText()));
out.println();
n.setEndJavaLine(out.getJavaLine());
}
// Custom Tags may contain declarations from tag plugins.
public void visit(Node.CustomTag n) throws JasperException {
if (n.useTagPlugin()) {
if (n.getAtSTag() != null) {
n.getAtSTag().visit(this);
}
visitBody(n);
if (n.getAtETag() != null) {
n.getAtETag().visit(this);
}
} else {
visitBody(n);
}
}
}
out.println();
page.visit(new DeclarationVisitor());
}
/**
* Compiles list of tag handler pool names.
*/
private void compileTagHandlerPoolList(Node.Nodes page)
throws JasperException {
class TagHandlerPoolVisitor extends Node.Visitor {
private Vector names;
/*
* Constructor
*
* @param v Vector of tag handler pool names to populate
*/
TagHandlerPoolVisitor(Vector v) {
names = v;
}
/*
* Gets the name of the tag handler pool for the given cu
没有合适的资源?快使用搜索试试~ 我知道了~
STRUTS2源码解析
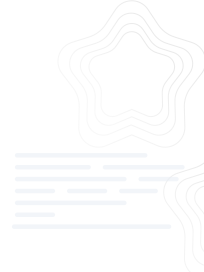
共3984个文件
java:1844个
js:476个
txt:276个


温馨提示
STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析STRUTS2源码解析
资源推荐
资源详情
资源评论
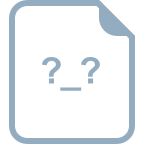
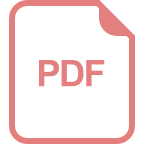
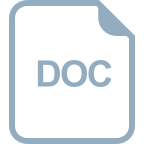
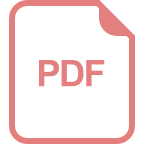
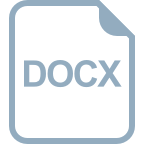
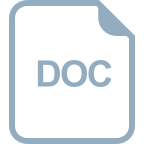
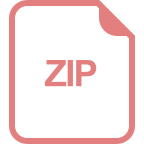
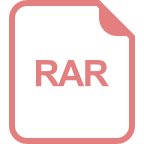
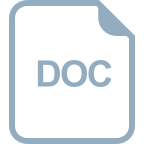
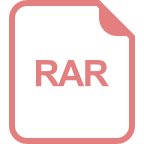
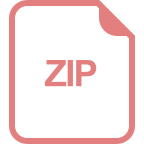
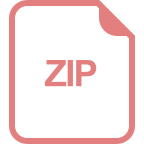
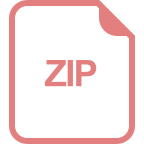
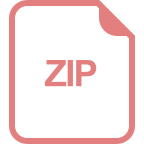
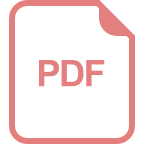
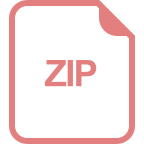
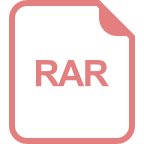
收起资源包目录

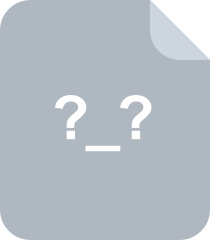
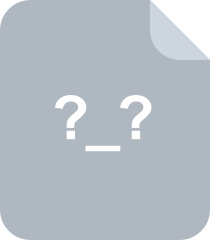
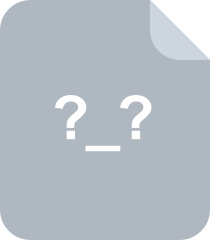
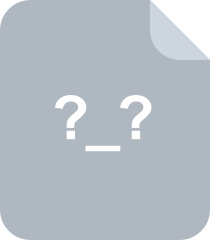
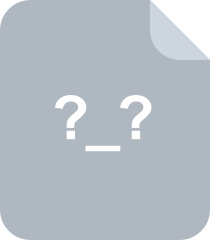
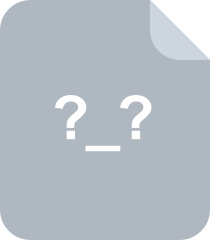
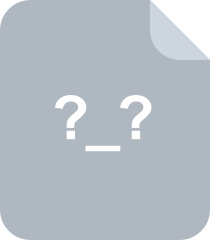
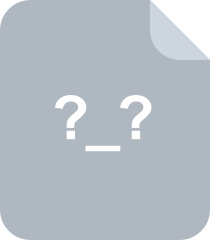
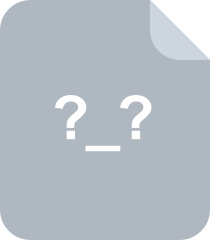
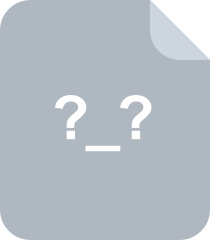
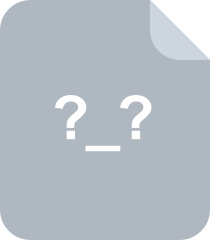
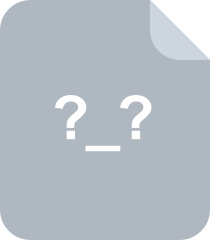
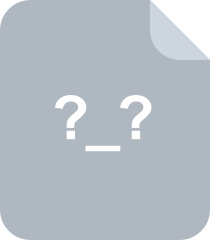
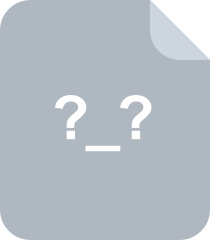
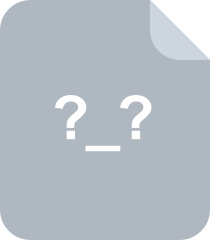
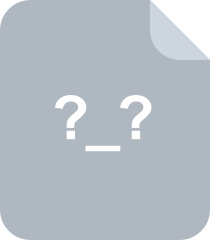
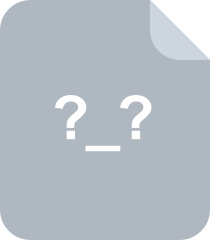
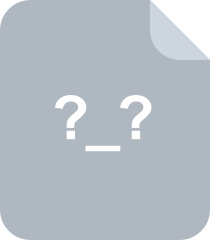
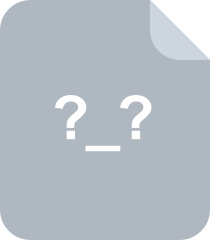
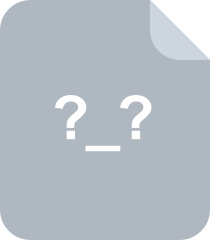
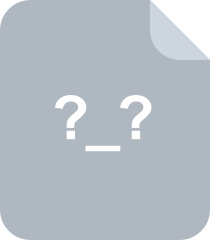
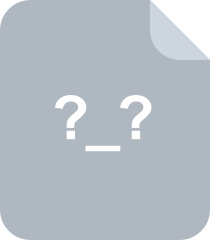
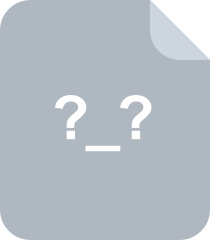
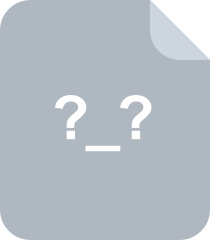
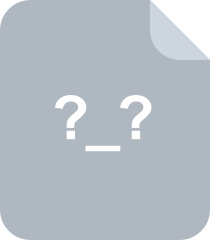
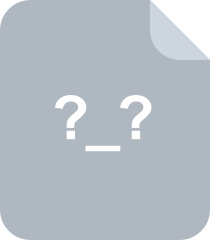
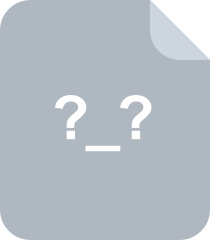
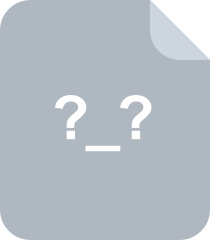
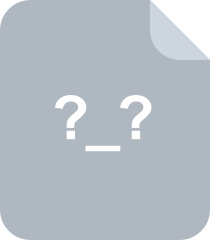
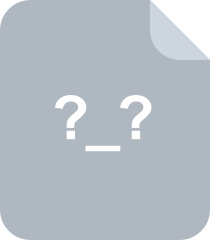
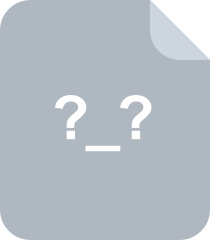
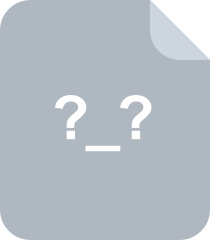
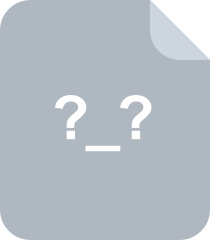
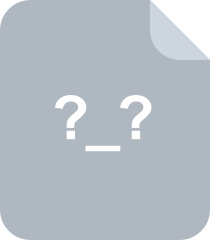
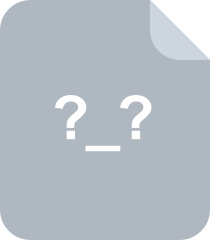
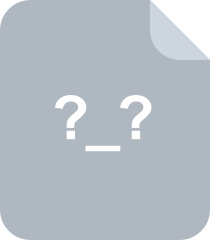
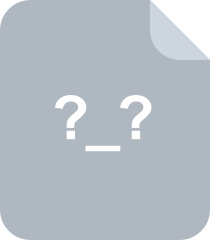
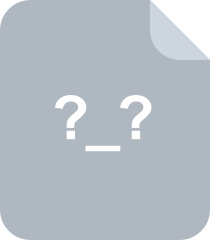
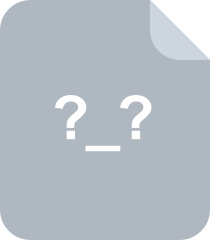
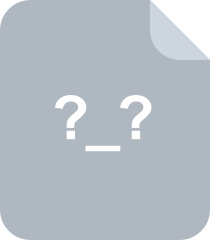
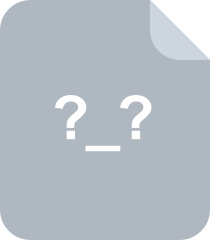
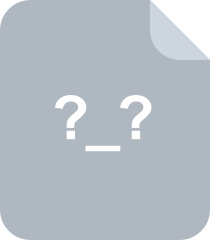
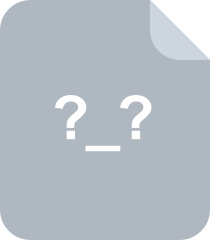
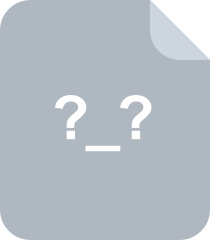
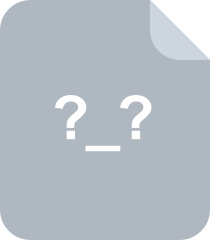
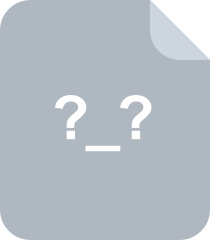
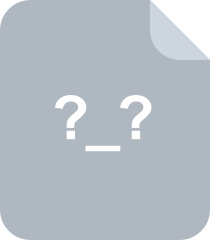
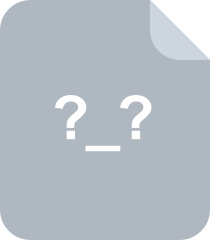
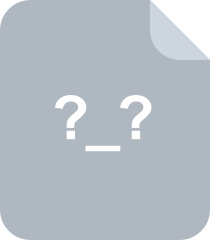
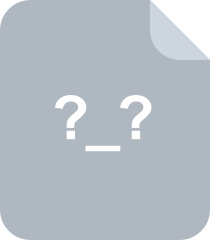
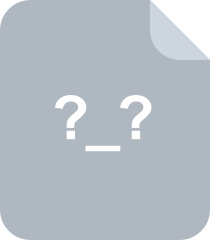
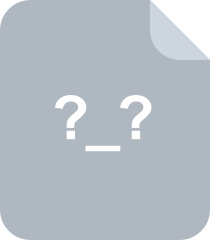
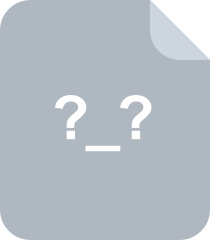
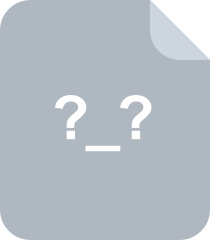
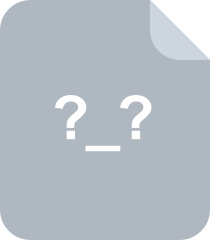
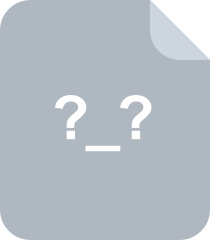
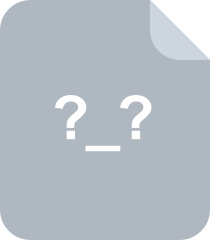
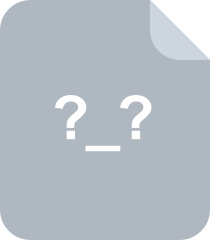
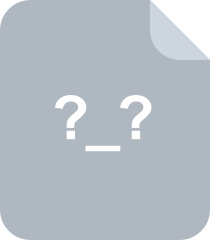
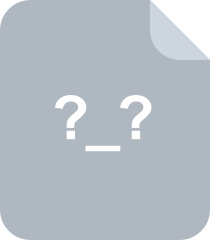
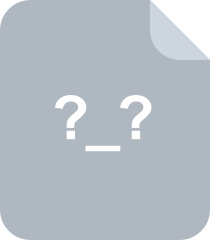
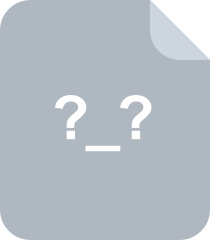
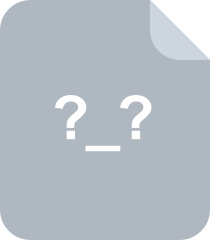
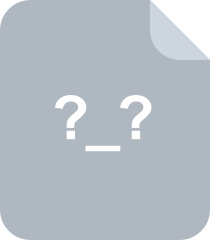
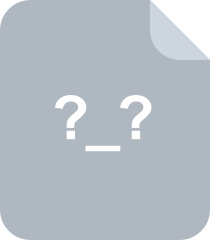
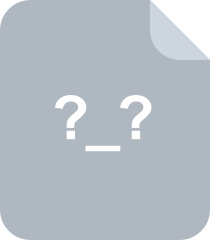
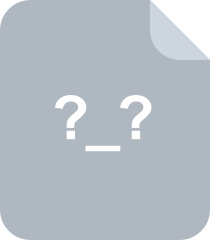
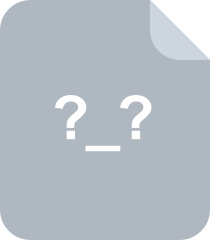
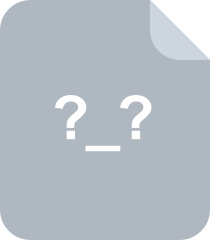
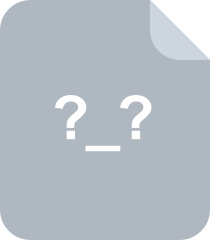
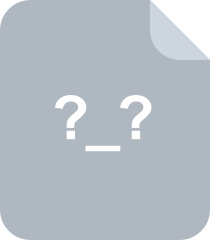
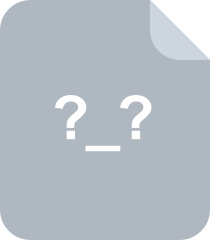
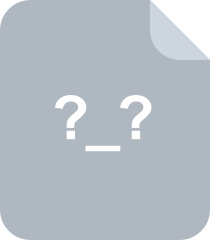
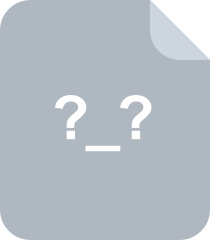
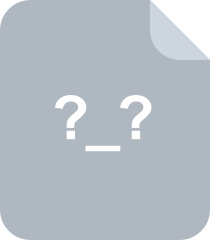
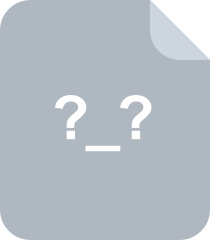
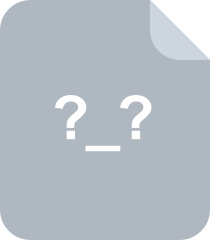
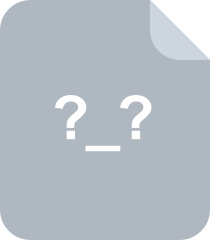
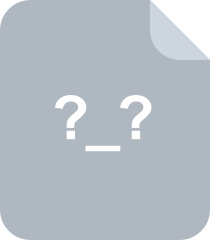
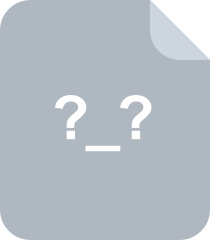
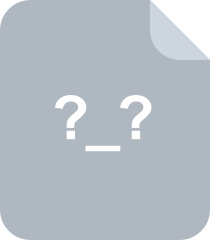
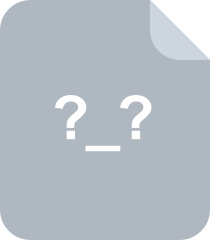
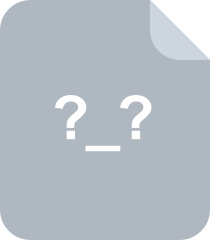
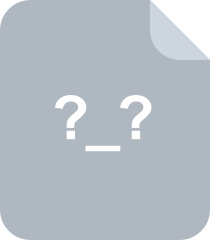
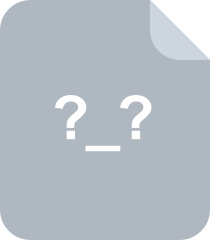
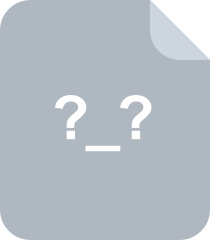
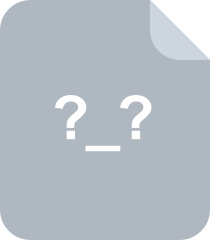
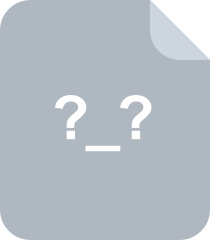
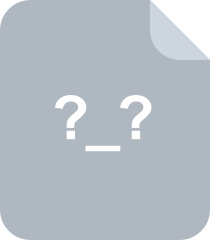
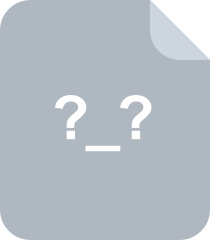
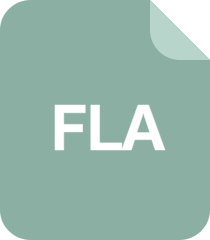
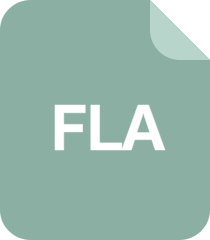
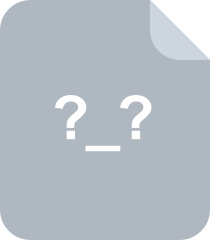
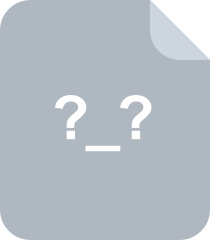
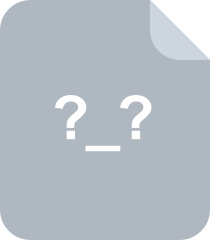
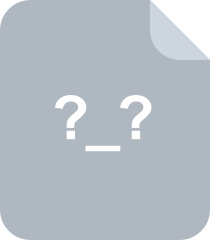
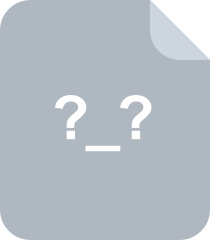
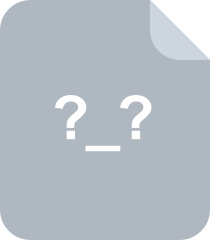
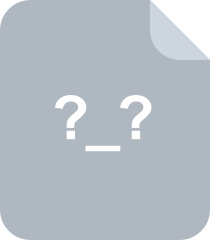
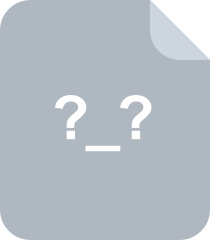
共 3984 条
- 1
- 2
- 3
- 4
- 5
- 6
- 40
资源评论
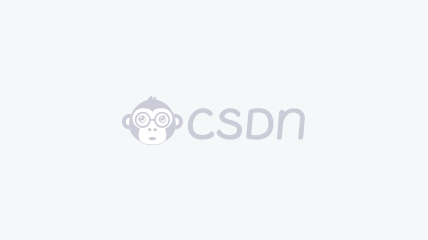
- 钟瀚林2012-11-19struts源码,哈哈,关联了eclipse就可以看到了。
- nimeijian2012-11-09就是Struts2的源码,不是什么源码解析。
- GRANCY很冷静2012-03-06这是struts真正的源码,在eclipse关联后可以看struts的源码的。
- gougou8511292012-05-02struts-2.2.3.1版本,但是缺少archetypes子工程,mvn跑不起来。

haojia1985
- 粉丝: 0
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

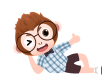
最新资源
- (源码)基于ESP8266的WebDAV服务器与3D打印机管理系统.zip
- (源码)基于Nio实现的Mycat 2.0数据库代理系统.zip
- (源码)基于Java的高校学生就业管理系统.zip
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
- (源码)基于计算机系统原理与Arduino技术的学习平台.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


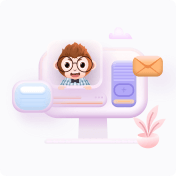
安全验证
文档复制为VIP权益,开通VIP直接复制
