<p align="center"><img width="140"src="https://raw.githubusercontent.com/SortableJS/Vue.Draggable/master/logo.svg?sanitize=true"></p>
<h1 align="center">vue.draggable.next</h1>
[](https://circleci.com/gh/SortableJS/Vue.Draggable)
[](https://codecov.io/gh/SortableJS/Vue.Draggable)
[](https://codebeat.co/projects/github-com-sortablejs-vue-draggable-master)
[](https://github.com/SortableJS/Vue.Draggable/issues?q=is%3Aopen+is%3Aissue)
[](https://www.npmjs.com/package/vuedraggable)
[](https://www.npmjs.com/package/vuedraggable)
[](https://www.npmjs.com/package/vuedraggable/v/next)
[](https://github.com/SortableJS/vue.draggable.next/blob/master/LICENSE)
Vue component (Vue.js 3.0) allowing drag-and-drop and synchronization with view model array.
For Vue 2 and Vue 1 version check: https://github.com/SortableJS/Vue.Draggable
Based on and offering all features of [Sortable.js](https://github.com/RubaXa/Sortable)
## Demo
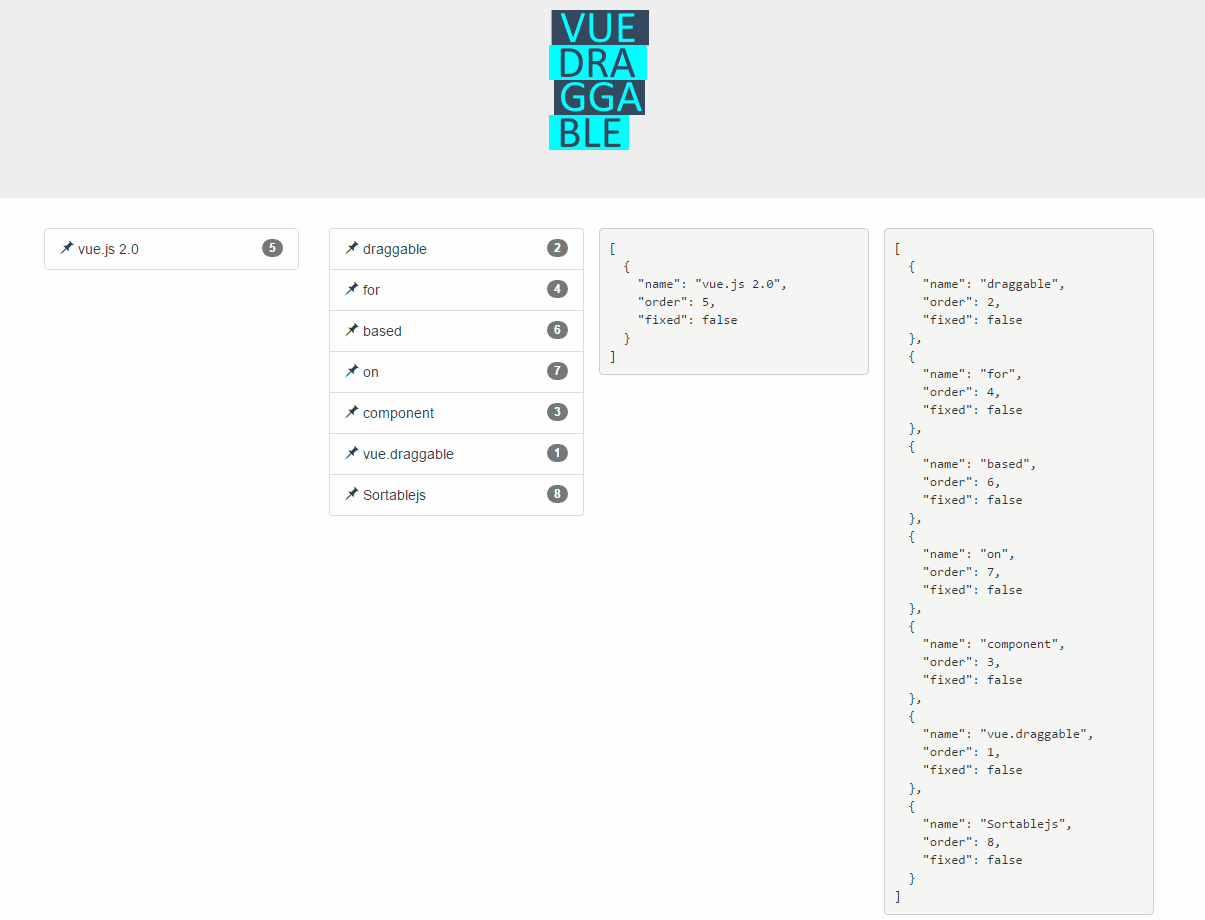
## Live Demos
https://sortablejs.github.io/vue.draggable.next/
## Features
* Full support of [Sortable.js](https://github.com/RubaXa/Sortable) features:
* Supports touch devices
* Supports drag handles and selectable text
* Smart auto-scrolling
* Support drag and drop between different lists
* No jQuery dependency
* Keeps in sync HTML and view model list
* Compatible with Vue.js 3.0 transition-group
* Cancellation support
* Events reporting any changes when full control is needed
* Reuse existing UI library components (such as [vuetify](https://vuetifyjs.com), [element](http://element.eleme.io/), or [vue material](https://vuematerial.io) etc...) and make them draggable using `tag` and `componentData` props
## Donate
Find this project useful? You can buy me a :coffee: or a :beer:
[](https://www.paypal.com/cgi-bin/webscr?cmd=_donations&business=GYAEKQZJ4FQT2¤cy_code=USD&source=url)
## Installation
### With npm or yarn
```bash
yarn add vuedraggable@next
npm i -S vuedraggable@next
```
### with direct link
```html
<script src="//cdnjs.cloudflare.com/ajax/libs/vue/3.0.2/vue.min.js"></script>
<!-- CDNJS :: Sortable (https://cdnjs.com/) -->
<script src="//cdn.jsdelivr.net/npm/sortablejs@1.10.2/Sortable.min.js"></script>
<!-- CDNJS :: Vue.Draggable (https://cdnjs.com/) -->
<script src="//cdnjs.cloudflare.com/ajax/libs/Vue.Draggable/4.0.0/vuedraggable.umd.min.js"></script>
```
[cf example section](https://github.com/SortableJS/Vue.Draggable/tree/master/example)
## Typical use:
``` html
<draggable
v-model="myArray"
group="people"
@start="drag=true"
@end="drag=false"
item-key="id">
<template #item="{element}">
<div>{{element.name}}</div>
</template>
</draggable>
```
``` js
import draggable from 'vuedraggable'
...
export default {
components: {
draggable,
},
data() {
return {
drag: false,
}
},
...
```
The `item` slot should be used to display items of the list. It receives the element value and the element index as slot-props.
### With `transition-group`:
``` html
<draggable v-model="myArray" tag="transition-group" item-key="id">
<template #item="{element}">
<div> {{element.name}} </div>
</template>
</draggable>
```
### With footer slot:
``` html
<draggable v-model="myArray" item-key="id">
<template #item="{element}">
<div> {{element.name}} </div>
</template>
<template #footer>
<button @click="addPeople">Add</button>
</template>
</draggable>
```
### With header slot:
``` html
<draggable v-model="myArray" item-key="id">
<template #item="{element}">
<div> {{element.name}} </div>
</template>
<template #header>
<button @click="addPeople">Add</button>
</template>
</draggable>
```
### With Vuex:
```html
<draggable v-model='myList'>
```
```javascript
computed: {
myList: {
get() {
return this.$store.state.myList
},
set(value) {
this.$store.commit('updateList', value)
}
}
}
```
### Migrate from vue 2 version:
Breaking changes:
1) Use `item` slot instead of default to display elements
2) Provide a key for items using `itemKey` props
From:
``` html
<!-- vue 2 version -->
<draggable v-model="myArray">
<div v-for="element in myArray" :key="element.id">{{element.name}}</div>
</draggable>
```
To:
``` html
<draggable v-model="myArray" item-key="id">
<template #item="{element}">
<div>{{element.name}}</div>
</template>
</draggable>
```
Breaking changes:
3) When using transition, you should now use the `tag` props and `componentData` to create the transition
From
``` html
<!-- vue 2 version -->
<draggable v-model="myArray">
<transition-group name="fade">
<div v-for="element in myArray" :key="element.id">
{{element.name}}
</div>
</transition-group>
</draggable>
```
to
``` html
<draggable v-model="myArray" tag="transition-group" :component-data="{name:'fade'}">
<template #item="{element}">
<div>{{element.name}}</div>
</template>
</draggable>
```
### Props
#### modelValue
Type: `Array`<br>
Required: `false`<br>
Default: `null`
Input array to draggable component. Typically same array as referenced by inner element v-for directive.<br>
This is the preferred way to use Vue.draggable as it is compatible with Vuex.<br>
It should not be used directly but only though the `v-model` directive:
```html
<draggable v-model="myArray">
```
#### list
Type: `Array`<br>
Required: `false`<br>
Default: `null`
Alternative to the `modelValue` prop, list is an array to be synchronized with drag-and-drop.<br>
The main difference is that `list` prop is updated by draggable component using splice method, whereas `modelValue` is immutable.<br>
**Do not use in conjunction with modelValue prop.**
#### itemKey
Type: `String` or `Function`<br>
Required: `true`<br>
The property to be used as the element key. Alternatively a function receiving an element of the list and returning its key.
#### All sortable options
Sortable options can be set directly as vue.draggable props since version 2.19.
This means that all [sortable option](https://github.com/RubaXa/Sortable#options) are valid sortable props with the notable exception of all the method starting by "on" as draggable component expose the same API via events.
kebab-case property are supported: for example `ghost-class` props will be converted to `ghostClass` sortable option.
Example setting handle, sortable and a group option:
```HTML
<draggable
v-model="list"
handle=".handle"
:group="{ name: 'people', pull: 'clone', put: false }"
ghost-class="ghost"
:sort="false"
@change="log"
>
<!-- -->
</draggable>
```
#### tag
Type: `String`<br>
Default: `'div'`
HTML node type of the element that draggable component create as outer element for the included slot.<br>
It is also possible to pass the name of vue comp