This put-selector/put module/package provides a high-performance, lightweight
(~1.5KB minified, ~0.7KB gzipped with other code) function for creating
and manipulating DOM elements with succinct, elegant, familiar CSS selector-based
syntax across all browsers and platforms (including HTML generation on NodeJS).
The single function from the module creates or updates DOM elements by providing
a series of arguments that can include reference elements, selector strings, properties,
and text content. The put() function utilizes the proven techniques for optimal performance
on modern browsers to ensure maximum speed.
Installation/Usage
----------------
The put.js module can be simply downloaded and used a plain script (creates a global
put() function), as an AMD module (exports the put() function), or as a NodeJS (or any
server side JS environment) module.
It can also be installed with <a href="https://github.com/kriszyp/cpm">CPM</a>:
cpm install put-selector
and then reference the "put-selector" module as a dependency.
or installed for Node with NPM:
npm install put-selector
and then:
put = require("put-selector");
Creating Elements
----------------
Type selector syntax (no prefix) can be used to indicate the type of element to be created. For example:
newDiv = put("div");
will create a new <div> element. We can put a reference element in front of the selector
string and the <div> will be appended as a child to the provided element:
put(parent, "div");
The selector .class-name can be used to assign the class name. For example:
put("div.my-class")
would create an element <div class="my-class"> (an element with a class of "my-class").
The selector #id can be used to assign an id and [name=value] can be used to
assign additional attributes to the element. For example:
newInput = put(parent, "input.my-input#address[type=checkbox]");
Would create an input element with a class name of "my-input", an id of "address",
and the type attribute set to "checkbox". The attribute assignment will always use
setAttribute to assign the attribute to the element. Multiple attributes and classes
can be assigned to a single element.
The put function returns the last top level element created or referenced. In the
examples above, the newly create element would be returned.
Modifying Elements
----------------
One can also modify elements with selectors. If the tag name is omitted (and no
combinators have been used), the reference element will be modified by the selector.
For example, to add the class "foo" to element, we could write:
put(element, ".foo");
Likewise, we could set attributes, here we set the "role" attribute to "presentation":
put(element, "[role=presentation]");
And these can be combined also. For example, we could set the id and an attribute in
one statement:
put(element, "#id[tabIndex=2]");
One can also remove classes from elements by using the "!" operator in place of a ".".
To remove the "foo" class from an element, we could write:
put(element, "!foo");
We can also use the "!" operator to remove attributes as well. Prepending an attribute name
with "!" within brackets will remove it. To remove the "role" attribute, we could write:
put(element, "[!role]");
Deleting Elements
--------------
To delete an element, we can simply use the "!" operator by itself as the entire selector:
put(elementToDelete, "!");
This will destroy the element from the DOM (using parent innerHTML destruction that reduces memory leaks in IE).
Text Content
-----------
The put() arguments may also include a subsequent string (or any primitive value including
boolean and numbers) argument immediately
following a selector, in which case it is used as the text inside of the new/referenced element.
For example, here we could create a new <div> with the text "Hello, World" inside.
newDiv = put(parent, "div", "Hello, World");
The text is escaped, so any string will show up as is, and will not be parsed as HTML.
Children and Combinators
-----------------------
CSS combinators can be used to create child elements and sibling elements. For example,
we can use the child operator (or the descendant operator, it acts the same here) to
create nested elements:
spanInsideOfDiv = put(reference, "div.outer span.inner");
This would create a new span element (with a class name of "inner") as a child of a
new div element (with a class name of "outer") as a child of the reference element. The
span element would be returned. We can also use the sibling operator to reference
the last created element or the reference element. In the example we indicate that
we want to create sibling of the reference element:
newSpan = put(reference, "+span");
Would create a new span element directly after the reference element (reference and
newSpan would be siblings.) We can also use the "-" operator to indicate that the new element
should go before:
newSpan = put(reference, "-span");
This new span element will be inserted before the reference element in the DOM order.
Note that "-" is valid character in tags and classes, so it will only be interpreted as a
combinator if it is the first character or if it is preceded by a space.
The sibling operator can reference the last created element as well. For example
to add two td element to a table row:
put(tableRow, "td+td");
The last created td will be returned.
The parent operator, "<" can be used to reference the parent of the last created
element or reference element. In this example, we go crazy, and create a full table,
using the parent operator (applied twice) to traverse back up the DOM to create another table row
after creating a td element:
newTable = put(referenceElement, "table.class-name#id tr td[colSpan=2]<<tr td+td<<");
We also use a parent operator twice at the end, so that we move back up two parents
to return the table element (instead of the td element).
Finally, we can use the comma operator to create multiple elements, each basing their selector
scope on the reference element. For example we could add two more rows to our table
without having to use the double parent operator:
put(newTable, "tr td,tr td+td");
Appending/Inserting Existing Elements
---------------------------------
Existing elements may be referenced in the arguments after selectors as well as before.
If an existing element is included in the arguments after a selector, the existing element will
be appended to the last create/referenced element or it will be inserted according to
a trailing combinator. For example, we could create a <div> and then append
the "child" element to the new <div>:
put("div", child);
Or we can do a simple append of an existing element to another element:
put(parent, ">", child);
We could also use sibling combinators to place the referenced element. We could place
the "second" element after (as the next sibling) the "first" element:
put(first, "+", second);
Or we could create a <div> and place "first" before it using the previous sibling combinator:
put(parent, "div.second -", first);
The put() function takes an unlimited number of arguments, so we could combine as
many selectors and elements as we want:
put(parent, "div.child", grandchild, "div.great-grandchild", gggrandchild);
Variable Substitution
-------------------
The put() function also supports variable substitution, by using the "$" symbol in selectors.
The "$" can be used for attribute values and to represent text content. When a "$"
is encountered in a selector, the next argument value is consumed and used in it's
place. To create an element with a title that comes from the variable "title", we could write:
put("div[title=$]", title);
The value of title may have any characters (including ']'), no escaping is needed.
This approach can simplify selector string construction and avoids the need for complicated
escaping mechanisms.
The "$" may be used as a child entity to in
没有合适的资源?快使用搜索试试~ 我知道了~
ArcGIS Server JavaScript API 3.1离线包
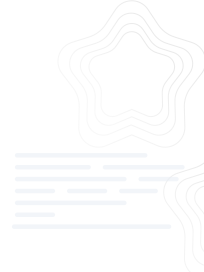
共4939个文件
js:2975个
css:624个
png:478个


温馨提示
ArcGIS Server Javascript API 3.1 jsapi普通模式的离线包,里面有API和新增的功能说明,包括CSS文件夹、image文件夹,js文件夹等 不包含jsapicompact包
资源推荐
资源详情
资源评论
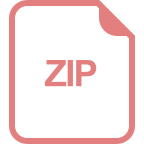
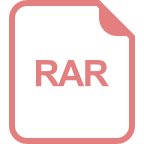
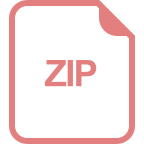
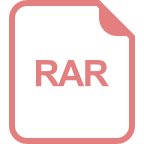
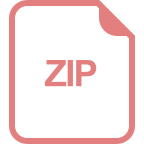
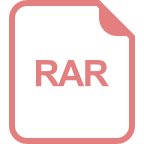
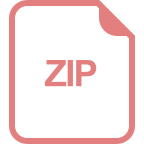
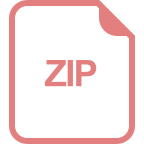
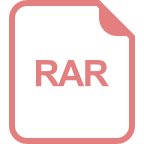
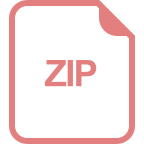
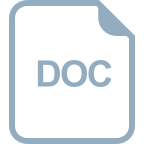
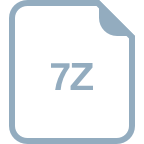
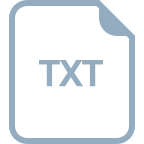
收起资源包目录

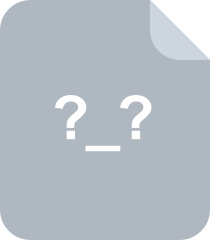
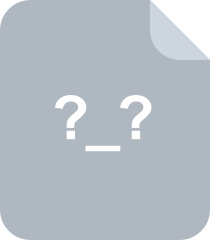
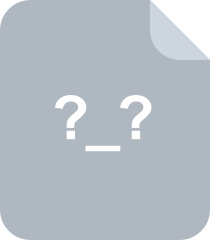
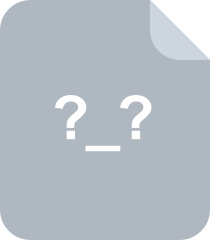
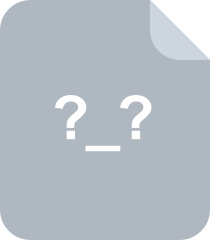
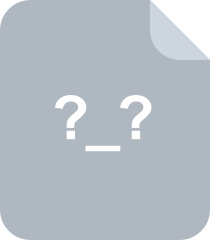
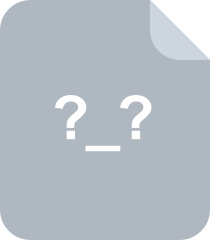
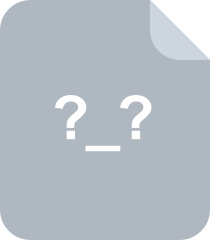
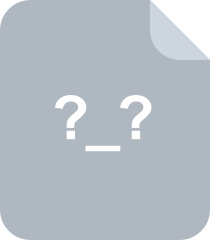
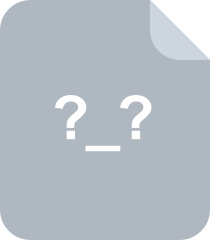
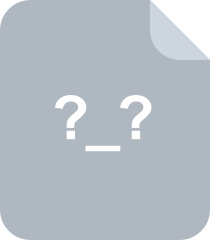
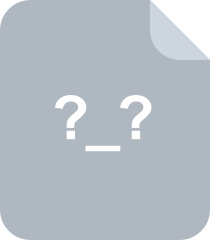
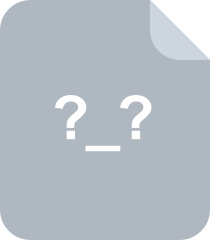
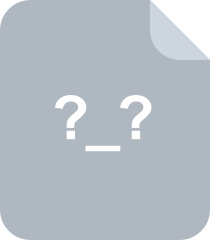
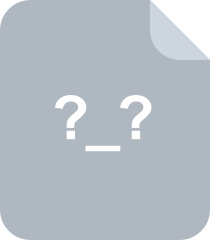
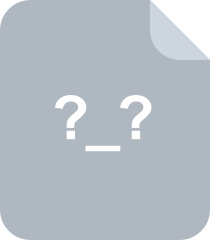
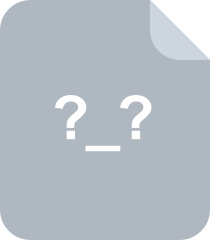
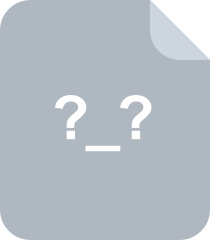
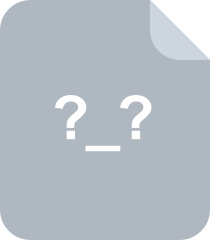
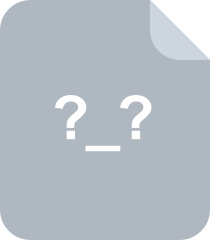
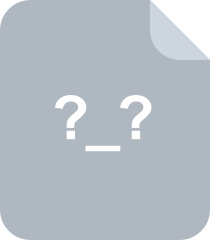
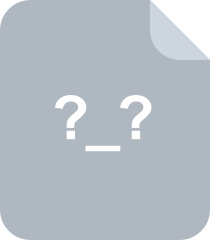
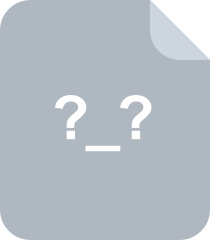
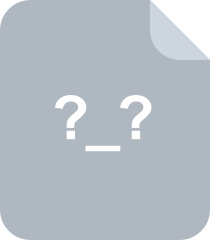
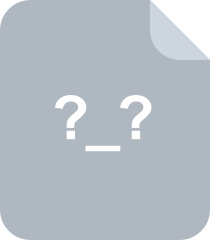
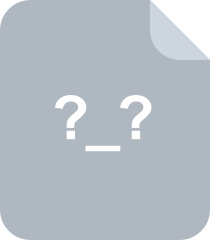
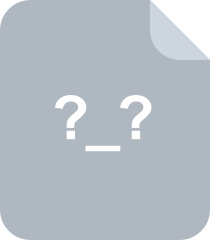
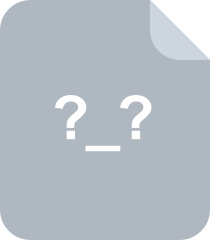
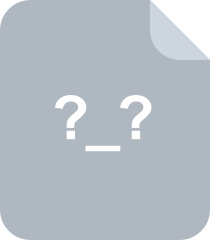
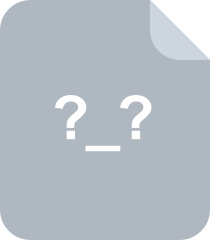
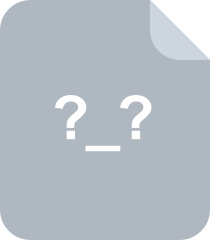
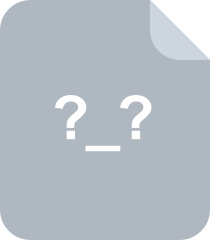
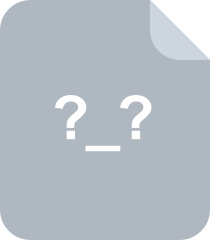
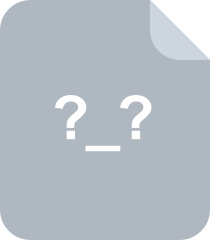
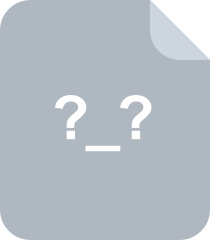
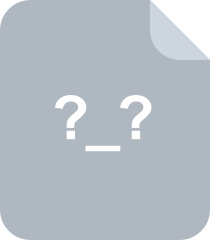
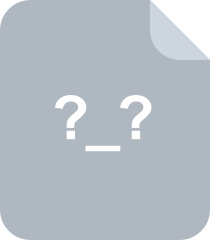
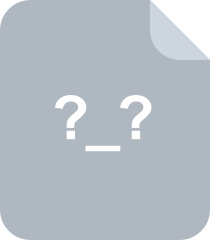
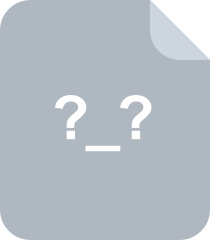
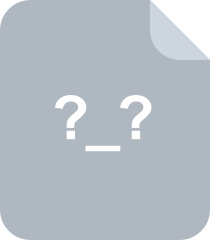
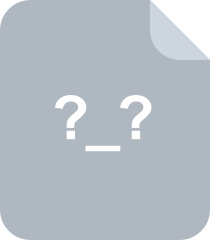
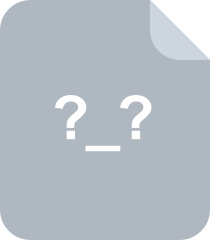
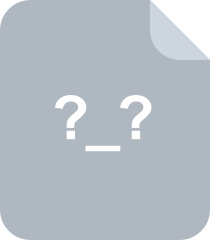
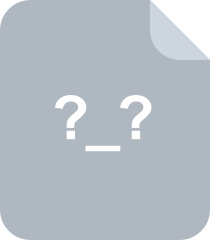
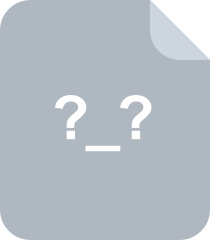
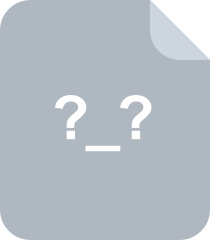
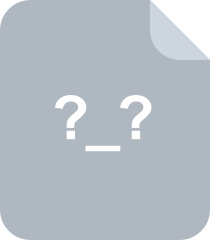
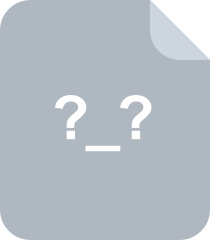
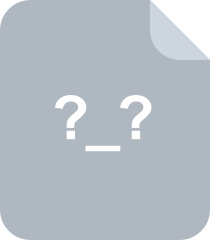
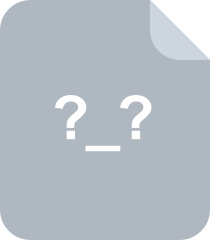
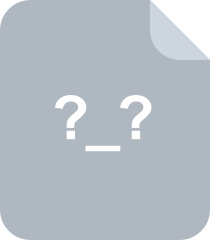
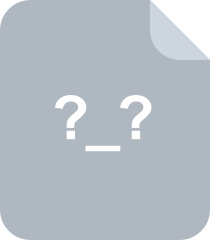
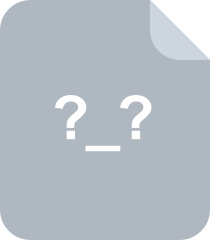
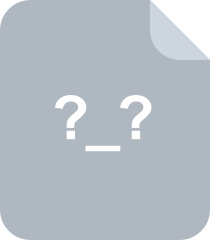
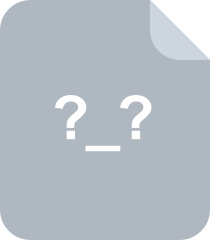
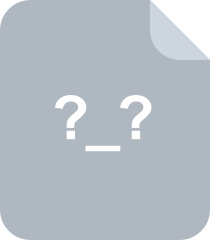
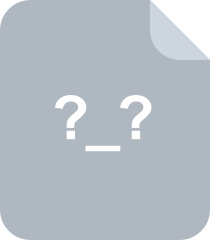
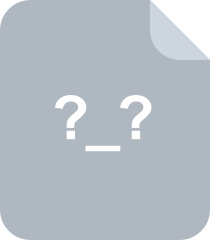
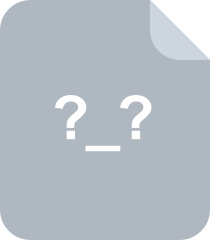
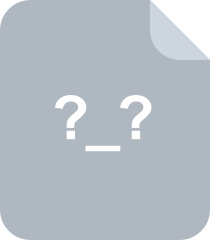
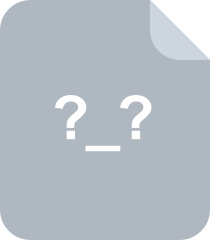
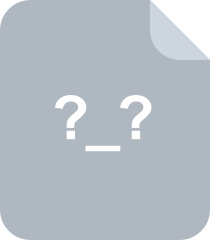
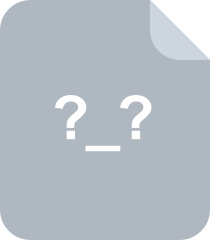
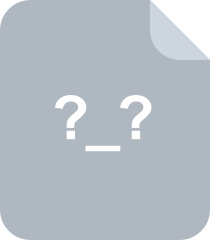
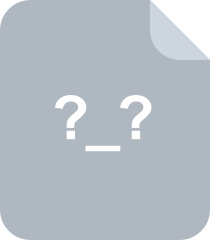
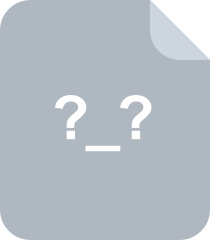
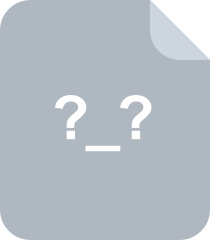
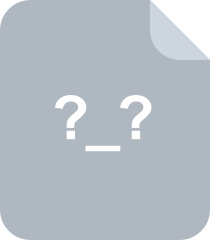
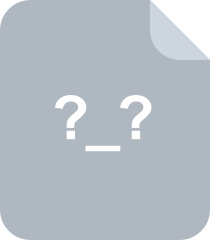
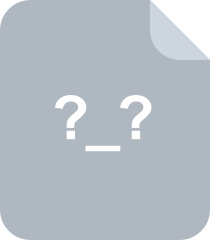
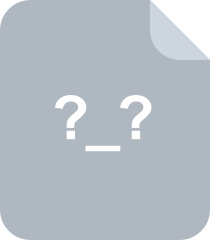
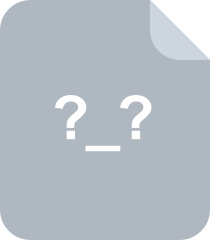
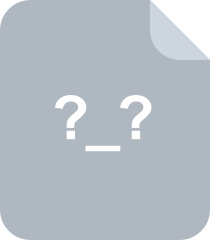
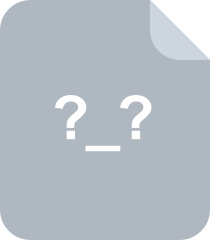
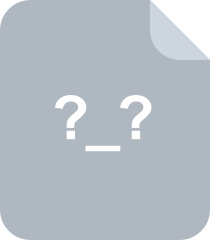
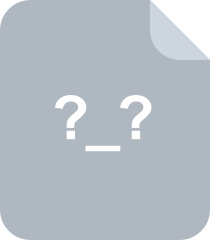
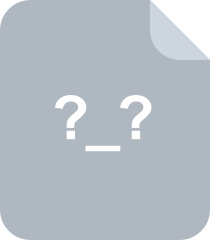
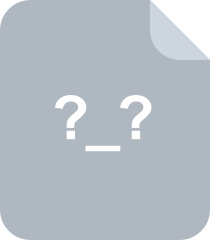
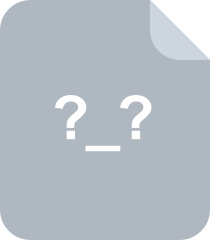
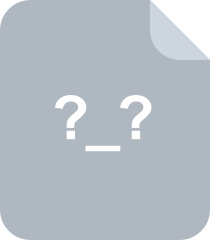
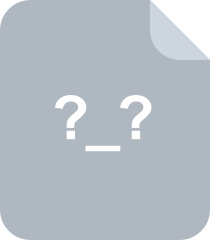
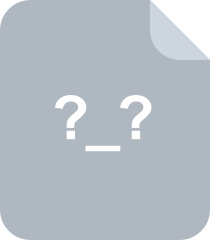
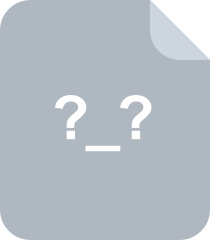
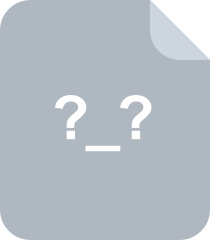
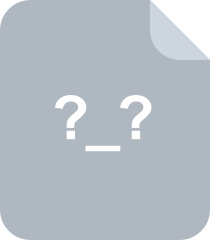
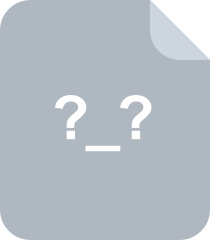
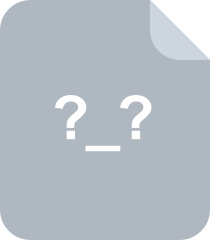
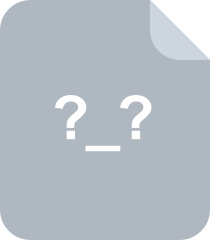
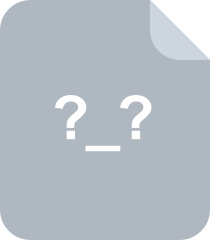
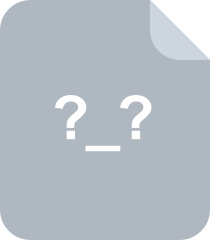
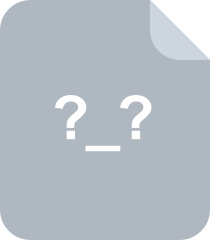
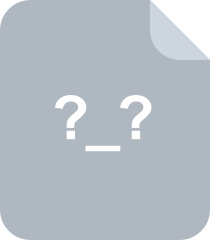
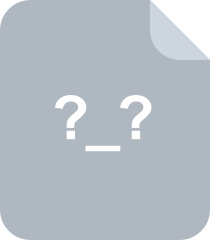
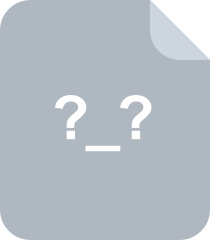
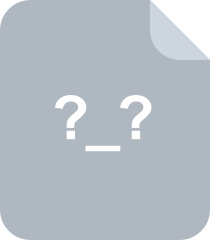
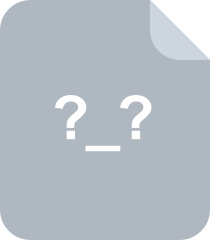
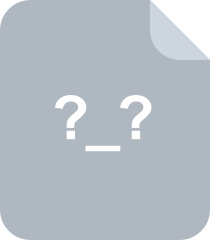
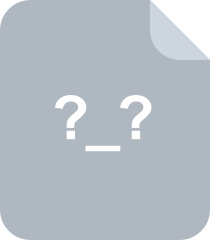
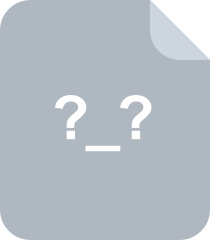
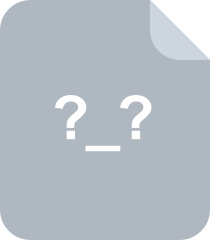
共 4939 条
- 1
- 2
- 3
- 4
- 5
- 6
- 50
资源评论
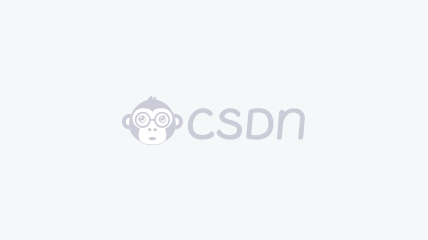

不怎么迷糊
- 粉丝: 23
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

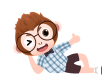
最新资源
- 环形导轨椭圆线体STEP全套设计资料100%好用.zip
- 第八章_焊接金相学.ppt
- 常用金属材料的焊接.ppt
- 管理者的目标计划执行.pptx
- 超(超)临界锅炉用新型耐热钢的焊接及热处理.ppt
- 第二章_焊接检验员安全须知.ppt
- 第七章_焊接检验中的公制英制单位制转换.ppt
- 第四章_焊接接头的几何形状及焊接符号.ppt
- 第一章_焊接检验及资格认证.ppt
- 典型焊接结构的生产工艺.ppt
- 第五章_焊接检验及资格认可的有关资料.ppt
- 钢制压力容器焊接工艺评定.ppt
- 过程装备制造Chapter 2 焊接变形与应力.ppt
- 过程装备制造Chapter 1 焊接接头与焊接规范.ppt
- 过程装备制造Chapter 4 焊接结构的断裂失效与防治.ppt
- 过程装备制造Chapter 3 焊接接头的强度计算.ppt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


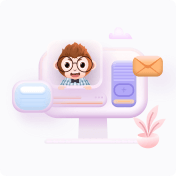
安全验证
文档复制为VIP权益,开通VIP直接复制
