#!/bin/bash
# -*- coding: utf-8 -*-
# Author: CIASM
# update 2023/06/12
<<!
The system supports the Sql Server 2019 2022 deployment in rpm mode,ubuntu(20.04, not included 22.04), centos,redhat,oracle(7,8,9),rockylinux,almalinux(8,9), suse(12,15)
# Official website automated script reference
https://learn.microsoft.com/en-us/sql/linux/sample-unattended-install-redhat?view=sql-server-ver16
# download Linux all sql server package
https://packages.microsoft.com/
# SQL server Linux repo
https://packages.microsoft.com/config/
# ubuntu To execute this script
ln -sf bash /bin/sh
!
install_sqlserver() {
# Check if the script is being run as root
if [ "$(id -u)" != "0" ]; then
echo "This script must be run as root."
exit 1
fi
# Check the CentOS/Red Hat version
if [[ -f /etc/redhat-release ]]; then
OS=$(cat /etc/*release* | grep "^NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+\.[0-9]+' | head -n1)
# Check the oralce Linux version
elif [[ -f /etc/oracle-release ]]; then
OS=$(cat /etc/*release* | grep "^NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+\.[0-9]+' | head -n1)
# Check the Rock Linux version
elif [[ -f /etc/rocky-release ]]; then
OS=$(cat /etc/*release* | grep "^NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+\.[0-9]+' | head -n1)
# Check the AlmaLinux version
elif [[ -f /etc/almalinux-release ]]; then
OS=$(cat /etc/*release* | grep "^NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+\.[0-9]+' | head -n1)
# Check the ubuntu version
elif [[ -f /etc/os-release ]]; then
OS=$(cat /etc/*release* | grep "^NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+\.[0-9]+' | head -n1)
# Check the suse version
elif [[ -f /etc/SuSE-release ]]; then
OS=$(cat /etc/*release* | grep "^PRETTY_NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+\.[0-9]+' | head -n1)
# Check the Debian version
elif [[ -f /etc/os-release ]]; then
OS=$(cat /etc/*release* | grep "^NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+' | head -n1)
# Check the Fedora version
elif [[ -f /etc/fedora-release ]]; then
OS=$(cat /etc/*release* | grep "^NAME=" | cut -d'=' -f2- | tr -d '"')
VERSION=$(cat /etc/*release* | grep -oE '[0-9]+' | head -n1)
else
echo -e "\033[31mThis script only supports $OS $VERSION...\033[0m"
exit 1
fi
# Check the SQL Server version
#if ! command -v sqlservr &> /dev/null; then
if [ ! -d "/opt/mssql" ]; then
echo -e "\033[32mInstalling SQL Server for $OS $VERSION...\033[0m"
case $VERSION in
# CentOS/RedHat/oracle 7 install Sql Server 2019
7.?)
sudo curl -o /etc/yum.repos.d/mssql-server.repo https://packages.microsoft.com/config/rhel/7/mssql-server-2019.repo
sudo curl -o /etc/yum.repos.d/msprod.repo https://packages.microsoft.com/config/rhel/7/prod.repo
echo Installing SQL Server...
sudo yum install -y net-tools vim
sudo yum install -y bzip2 cyrus-sasl cyrus-sasl-gssapi gdb libatomic libsss_nss_idmap libtirpc python3 python3-libs python3-pip python3-setuptools cyrus-sasl-lib
sudo yum install -y mssql-server
sudo ACCEPT_EULA=Y yum install -y mssql-tools unixODBC unixODBC-devel
sudo yum install -y mssql-server-fts
firewall-cmd --zone=public --add-port=1433/tcp --permanent && firewall-cmd --reload
;;
# CentOS/RedHat/oracle/RockLinux/AlmaLinux 8 install Sql Server 2022
8.?)
sudo curl -o /etc/yum.repos.d/mssql-server.repo https://packages.microsoft.com/config/rhel/8/mssql-server-2022.repo
sudo curl -o /etc/yum.repos.d/msprod.repo https://packages.microsoft.com/config/rhel/8/prod.repo
echo Installing SQL Server...
sudo yum install -y net-tools vim
sudo yum install -y gc gdb libatomic_ops libipt python3-pip gdb-headless python36 bzip2 cyrus-sasl-gssapi cyrus-sasl libatomic libbabeltrace python3-setuptools chkconfig guile platform-python-pip
sudo yum install -y mssql-server
sudo ACCEPT_EULA=Y yum install -y mssql-tools unixODBC unixODBC-devel
sudo yum install -y mssql-server-fts
firewall-cmd --zone=public --add-port=1433/tcp --permanent && firewall-cmd --reload
;;
# CentOS/RedHat/oracle/RockLinux/AlmaLinux 9 install Sql Server 2022
9.?)
sudo curl -o /etc/yum.repos.d/mssql-server.repo https://packages.microsoft.com/config/rhel/9.0/mssql-server-2022.repo
sudo curl -o /etc/yum.repos.d/msprod.repo https://packages.microsoft.com/config/rhel/9.0/prod.repo
echo Installing SQL Server...
sudo yum install -y net-tools vim
sudo yum install -y gc gdb libatomic_ops libipt python3-pip gdb-headless python36 bzip2 cyrus-sasl-gssapi cyrus-sasl libatomic libbabeltrace python3-setuptools chkconfig guile platform-python-pip
sudo yum install -y mssql-server
sudo ACCEPT_EULA=Y yum install -y mssql-tools unixODBC unixODBC-devel
sudo yum install -y mssql-server-fts
firewall-cmd --zone=public --add-port=1433/tcp --permanent && firewall-cmd --reload
;;
# ubuntu 20.04,22.04 Linux install Sql Server 2019
20.0?|22.0?)
sudo curl https://packages.microsoft.com/keys/microsoft.asc | sudo apt-key add -
repoargs="$(curl https://packages.microsoft.com/config/ubuntu/20.04/mssql-server-2019.list)"
sudo add-apt-repository "${repoargs}"
repoargs="$(curl https://packages.microsoft.com/config/ubuntu/20.04/prod.list)"
sudo add-apt-repository "${repoargs}"
sudo apt-get install -y gdb gdbserver libatomic1 libbabeltrace1 libc++1 libc++1-10 libc++abi1-10 libc6 libc6-dbg libcc1-0 libdw1 libsasl2-modules-gssapi-mit libsss-nss-idmap0
sudo apt-get install -y autoconf automake autotools-dev binutils binutils-common binutils-x86-64-linux-gnu cpp cpp-9 gcc gcc-9 gcc-9-base libasan5 libbinutils libc-dev-bin libc6-dev libcrypt-dev libctf-nobfd0 libctf0 libgcc-9-dev libgomp1 libisl22 libitm1 liblsan0 libltdl-dev libmpc3 libodbc1 libquadmath0 libtool libtsan0 libubsan1 linux-libc-dev m4 manpages-dev msodbcsql17 odbcinst odbcinst1debian2
sudo apt-get install -y net-tools vim
sudo apt-get install -y mssql-server
sudo ACCEPT_EULA=Y apt-get install -y mssql-tools unixodbc unixodbc-dev
sudo apt-get install -y mssql-server-fts
sudo ufw allow 1433/tcp
sudo ufw reload
;;
# Debian 10, 11
10|11)
echo "Not supported by official website"
;;
# Fedora 31,32,33
31|32|33)
echo "Not supported by official website"
;;
# SUSE 12 install Sql Server 2019
12.?)
sudo zypper addrepo -fc https://packages.microsoft.com/config/sles/12/mssql-server-2019.repo
sudo zypper addrepo -fc https://packages.microsoft.com/config/sles/12/prod.repo
sudo zypper --gpg-auto-import-keys refresh
sudo SUSEConnect -p sle-sdk/12.4/x86_64
echo Installing SQL Server...
sudo zypper install -y net-tools vim
sudo zypper install -y mssql-server
sudo ACCEPT_EULA=Y zypper install -y mssql-tools unixODBC-devel
sudo zypper install -y mssql-server-fts
sudo SuSEfirewall2 open INT TCP 1433
sudo SuSEfirewall2 stop
sudo SuSEfirewall2 start
;;
# SUSE 15 install Sql Server 2022
15.?)
sudo zypper addrepo -fc https://packages.microsoft.com/config/sles/15/mssql-server-2022.repo
sudo zypper addrepo -fc https://packages.microsoft.com/config/sles/15/prod.repo
sudo zypper --gpg-auto-import-keys refresh
sudo SUSEConnect -p sle-sdk/15.3/x86_64
echo Installing SQL Server...
sudo zypper install -y net-tools vim
sudo zypper install -y mssql-server
sudo ACCEPT_EULA=Y zypper install -y mssql-tools unixODBC-devel
sudo zypper install -y mssql-server-fts
sudo SuSEfirewall2 open INT TCP 1433
sudo SuSEfirewall2 stop
sudo SuSEfirewall2 start
;;
*)
echo -e "\033[31mUnsupported $OS $VERSION...\033[0m"
exit 1
;;
esac
#initialized_database
echo "SQL Server initialized_database $OS $VERSION..."
initialized_database
echo -e "\033[32mSQL Server for $OS $VERSION successful

CIAS
- 粉丝: 1174
- 资源: 299
最新资源
- 基于模型预测控制的永磁同步电机并网发电控制系统 simulink仿真,效果非常好
- matlab 小波阈值降噪,经典信号分解及降噪程序,模态
- 基于comsol的储层降压开采过程中的渗流-应力耦合算例 提供基于comsol的储层降压开采过程中的渗流-应力耦合算例,可在此基础上熟悉降压开采过程中的渗流-应力耦合计算方法
- 固高运动控制卡GTS400系列用C#语言写的二轴取放料学习模板,扩展性强,轴参数,登录界面,轴点位数据都用INI配置文件保存读取,是学习用C#运动控制的好案例,代码有注释,便于理解吸收, 录制的,超级
- 深度神经网络(DNN)做多特征输入单输出的二分类及多分类模型 程序内注释详细,直接替数据就可以用 程序语言为matlab 程序可出分类效果图,迭代优化图,混淆矩阵图具体效果如下所示 PS:以
- 永磁同步电机PMSM的MTPA+弱磁控制 包含参考资料,搭建步骤
- 自动驾驶基于阿克曼模型的控制算法仿真测试 , 基于ROS扣取单独的阿克曼控制算法模块进行测试,能够帮助朋友们学习基于阿克曼模型的控制算法实现以及对该控制算法的理解 阿克曼实现部分带有代码注释,帮助您
- 汇川H5U系列PLC程序 汇川H5U PLC程序,搭配汇川伺服驱动器,运动控制总线轴运动 PLC程序+昆仑通态触摸屏程序 ,模板程序,高端大气上档次UI设计,工控模板 优秀的触摸屏模板
- MATLAB对矩阵数据输出二维图和三维图m文件源码资料包 便于目视判读
- SiC MOSFET碳化硅MOS管驱动电路设计与Pspice仿真(基于同步整流电路,具有防直通互锁、米勒钳位、短路电流保护、负压关断等功能) 原理图和pcb满足减少寄生电感等优化布局,还有buck、
- matlab simulink光伏储能并网交直流发电系统仿真模型,2018a版本,2021a版本 1)光伏采用扰动观察法最大功率跟踪 2)蓄电池为双向DC-DC变器,采用电压环和电流环控制的双闭环控制
- 遗传算法优化用于分类 回归 时序预测 遗传算法优化支持向量机SVM,最小二乘支持向量机LSSVM,随机森林RF,极限学习机ELM,核极限学习机KELM,深度极限学习机DELM,BP神经网络,长短时记忆
- MATLAB代码:基于主从博弈理论的共享储能与综合能源微网优化运行研究 关键词:主从博弈 共享储能 综合能源微网 优化调度 参考文档:《基于主从博弈理论的共享储能与综合能源微网优化运行研究》完全复现
- ZZU物联网工程专业数据结构相关整合
- MATLAB代码:基于遗传算法的电动汽车有序充放电优化 关键词:遗传算法 电动汽车 有序充电 优化调度 参考文档:《精英自适应混合遗传算法及其实现-江建》算法部分;电动汽车建模部分相关文档太多,自
- MATLAB代码:基于储能电站服务的冷热电多微网系统双层优化配置 关键词:储能电站 共享储能电站 冷热电多微网 双层优化配置 参考文档:《基于储能电站服务的冷热电多微网系统双层优化配置》完全复
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


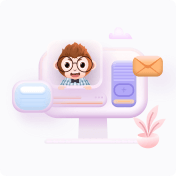