C++矩阵运算
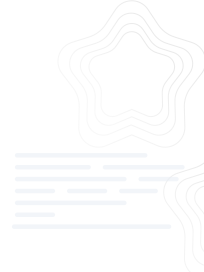


在C++编程中,矩阵运算是一项重要的任务,特别是在科学计算、图像处理以及各种工程应用中。本主题将深入探讨如何使用C++实现矩阵的加法、减法、乘法和除法运算。我们需要理解矩阵的基本概念和运算规则。 矩阵是由若干行和列构成的元素数组,通常用大写字母表示,如A、B等。矩阵的加减运算遵循对应位置元素相加减的原则。例如,两个同型矩阵A和B相加,新矩阵C的每个元素cij等于aij+bij,其中aij和bij分别是A和B中对应位置的元素。减法与此类似,只是bij变为bij-aij。 矩阵乘法则更为复杂,它不是简单的对应元素相乘,而是基于“线性组合”的概念。对于两个矩阵A(m×n)和B(n×p),它们可以相乘得到一个新矩阵C(m×p),其中C的每个元素cij是通过将A的第i行与B的第j列对应元素相乘并求和得到的。具体公式为cij = Σ(aki * bkj),ki从1到n,其中aki是A的第i行第k列元素,bkj是B的第k行第j列元素。 在C++中,我们可以使用类来封装矩阵的属性和操作。下面是一个简单的矩阵类设计示例: ```cpp #include <iostream> #include <vector> class Matrix { public: int rows, cols; std::vector<std::vector<int>> data; // 构造函数 Matrix(int r, int c) : rows(r), cols(c) { data.resize(rows, std::vector<int>(cols, 0)); } // 初始化矩阵 void init(int (*arr)[], int size) { for (int i = 0; i < rows; ++i) { for (int j = 0; j < cols; ++j) { data[i][j] = arr[i*cols + j]; } } } // 加法 Matrix operator+(const Matrix& other) const { if (rows != other.rows || cols != other.cols) { throw std::runtime_error("Matrices cannot be added due to different dimensions."); } Matrix result(rows, cols); for (int i = 0; i < rows; ++i) { for (int j = 0; j < cols; ++j) { result.data[i][j] = data[i][j] + other.data[i][j]; } } return result; } // 减法 Matrix operator-(const Matrix& other) const { if (rows != other.rows || cols != other.cols) { throw std::runtime_error("Matrices cannot be subtracted due to different dimensions."); } Matrix result(rows, cols); for (int i = 0; i < rows; ++i) { for (int j = 0; j < cols; ++j) { result.data[i][j] = data[i][j] - other.data[i][j]; } } return result; } // 乘法 Matrix operator*(const Matrix& other) const { if (cols != other.rows) { throw std::runtime_error("Matrix multiplication is not possible due to incompatible dimensions."); } Matrix result(rows, other.cols); for (int i = 0; i < rows; ++i) { for (int j = 0; j < other.cols; ++j) { for (int k = 0; k < cols; ++k) { result.data[i][j] += data[i][k] * other.data[k][j]; } } } return result; } // 输出矩阵 friend std::ostream& operator<<(std::ostream& os, const Matrix& m) { for (int i = 0; i < m.rows; ++i) { for (int j = 0; j < m.cols; ++j) { os << m.data[i][j] << " "; } os << "\n"; } return os; } }; ``` 这个类定义了矩阵的基本操作,包括构造、初始化、加法、减法和乘法。注意,加减法使用了重载的`operator+`和`operator-`,而乘法则使用了`operator*`。此外,我们还定义了一个友元函数`operator<<`用于方便地打印矩阵。 在实际编程中,你可以根据需要添加更多的功能,如读写矩阵文件、矩阵转置、求逆、求行列式等。对于矩阵除法,由于矩阵除法(即求逆矩阵乘以另一个矩阵)在C++中通常不直接表示,而是通过求逆矩阵来实现。你可以使用高斯消元法或LU分解等方法来求解逆矩阵。 在压缩包文件"矩阵加、减、乘"中,可能包含了一些具体的矩阵运算示例代码,你可以根据这些示例进一步学习和实践矩阵运算的实现。通过不断地练习和优化,你将能够熟练地在C++中处理各种复杂的矩阵运算问题。
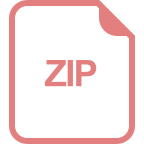
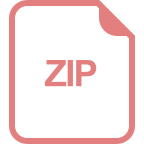
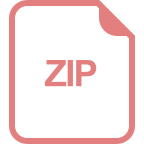
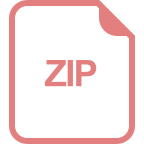
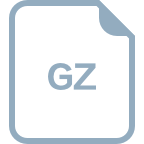
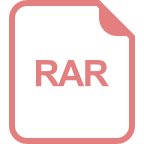
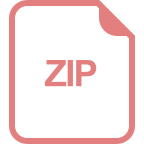
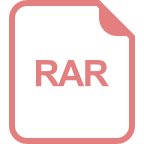
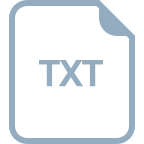
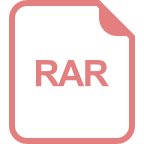
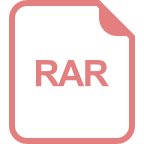
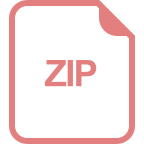


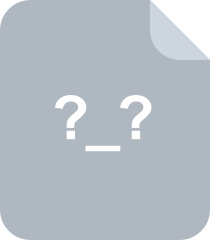


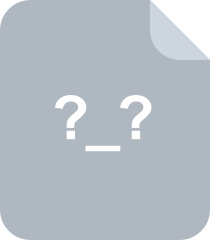


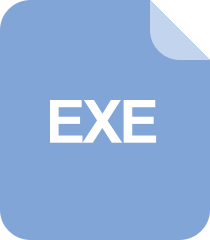
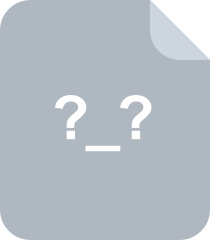
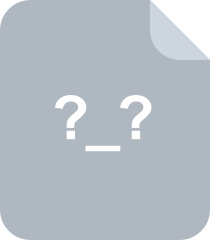
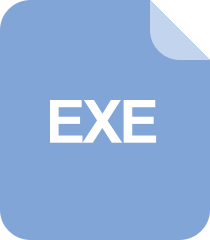
- 1
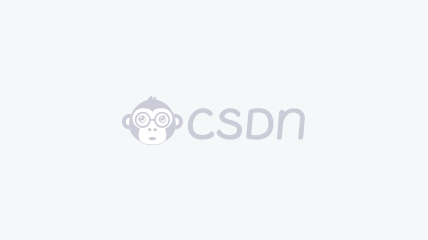
- Eru's2019-12-02对我有很大帮助,感谢!

- 粉丝: 2
- 资源: 14
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

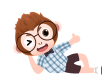
最新资源
- C语言-leetcode题解之70-climbing-stairs.c
- C语言-leetcode题解之68-text-justification.c
- C语言-leetcode题解之66-plus-one.c
- C语言-leetcode题解之64-minimum-path-sum.c
- C语言-leetcode题解之63-unique-paths-ii.c
- C语言-leetcode题解之62-unique-paths.c
- C语言-leetcode题解之61-rotate-list.c
- C语言-leetcode题解之59-spiral-matrix-ii.c
- C语言-leetcode题解之58-length-of-last-word.c
- 计算机编程课程设计基础教程

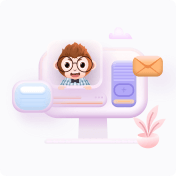
