DataGridView的进度条ProgressBar
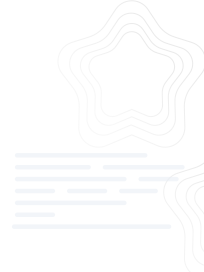


在.NET框架中,`DataGridView`控件是用于展示表格数据的标准组件,而`ProgressBar`控件则用于显示任务的进度状态。将`ProgressBar`集成到`DataGridView`中,可以为用户呈现数据处理或加载时的实时进度,提高用户体验。本文将深入探讨如何在`DataGridView`中实现进度条功能,以及在实际开发中可能遇到的问题和解决方案。 我们需要创建一个自定义的`DataGridView`列类型,这通常通过继承`DataGridViewColumn`并重写其行为来完成。例如,我们可以创建一个名为`DataGridViewProgressColumn`的新类,它包含一个内嵌的`ProgressBar`控件。这个自定义列将在每个单元格中显示进度条。 ```csharp public class DataGridViewProgressColumn : DataGridViewColumn { public DataGridViewProgressColumn() : base(new DataGridViewProgressCell()) { } // 其他属性和方法... } ``` 接下来,我们需要创建一个对应的单元格类`DataGridViewProgressCell`,它将承载`ProgressBar`控件。在这个类中,我们将设置`ProgressBar`的样式、范围和当前值,同时确保它能在`DataGridView`中正确显示和更新。 ```csharp public class DataGridViewProgressCell : DataGridViewTextBoxCell { private ProgressBar progressBar; public override void InitializeEditingControl(int rowIndex, object initialFormattedValue, DataGridViewCellStyle dataGridViewCellStyle) { base.InitializeEditingControl(rowIndex, initialFormattedValue, dataGridViewCellStyle); progressBar = (ProgressBar)EditingControl; progressBar.Style = ProgressBarStyle.Continuous; progressBar.Minimum = 0; progressBar.Maximum = 100; } protected override void OnDataGridViewChanged() { base.OnDataGridViewChanged(); if (DataGridView != null && EditingControl != null) { progressBar.Value = (int)DataGridView.Rows[RowIndex].Cells[OwningColumn.Index].Value; } } } ``` 为了在`DataGridView`中使用这个新列,我们需要在代码中添加它,并为每个行设置进度值。例如: ```csharp dataGridView1.Columns.Add(new DataGridViewProgressColumn { Name = "Progress" }); // 假设我们有100行数据 for (int i = 0; i < 100; i++) { dataGridView1.Rows.Add(i + 1); // 添加行 dataGridView1.Rows[i].Cells["Progress"].Value = i * 10; // 设置进度值 } ``` 在实际应用中,进度条通常与后台处理任务关联。例如,如果你正在加载大量数据或执行长时间操作,可以在后台线程中更新进度,并通过`Invoke`方法同步更新UI(因为UI操作必须在主线程中进行)。 ```csharp private async Task LoadDataAsync() { for (int i = 0; i <= 100; i++) { await Task.Delay(100); // 模拟耗时操作 // 更新进度条 dataGridView1.Invoke(new Action(() => dataGridView1.Rows[i].Cells["Progress"].Value = i)); } } ``` 请注意,由于多线程访问UI的安全性问题,务必确保在更新`DataGridView`时使用`Invoke`或`BeginInvoke`方法。此外,考虑使用异步编程技术(如`async/await`)以避免阻塞UI线程。 总结,将`ProgressBar`整合到`DataGridView`中是一项实用的功能,可以提升用户对数据处理过程的感知。通过创建自定义列和单元格,我们可以根据需求定制进度条的样式和行为,同时确保在后台操作中正确地同步更新界面。这种技术广泛应用于各种需要显示实时进度的应用场景,如数据导入、导出、分析等。
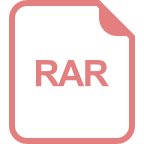
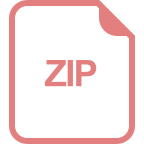
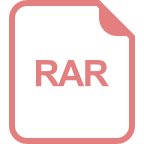
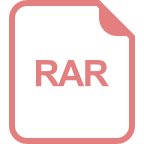
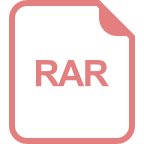
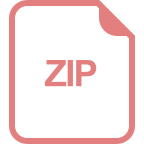
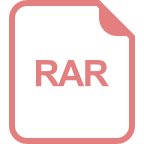
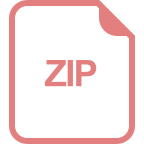
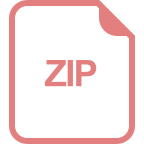
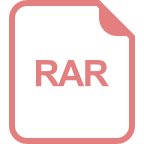
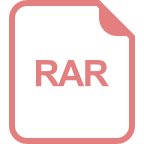
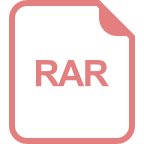
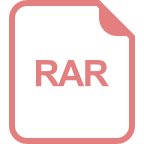
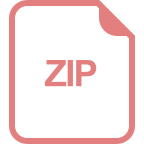
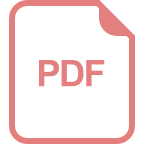
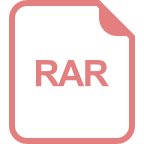



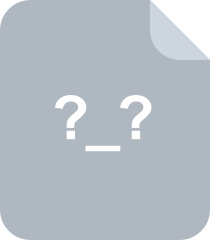


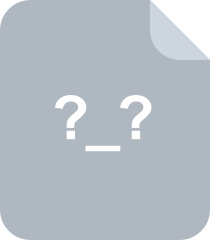
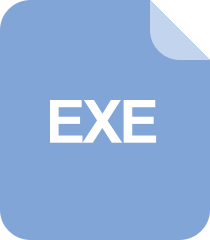
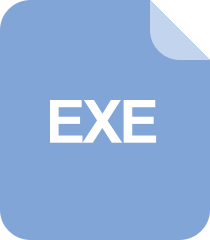


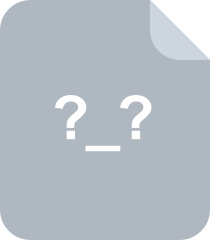
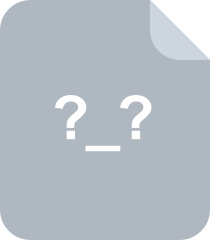


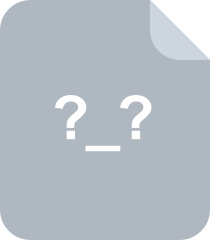
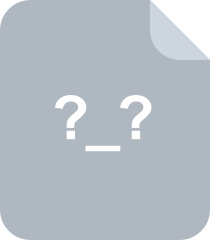
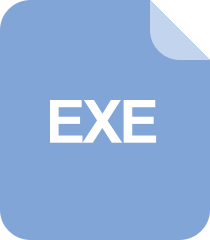
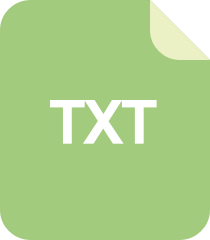

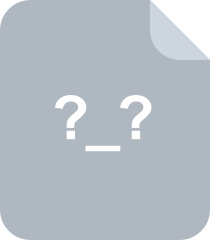
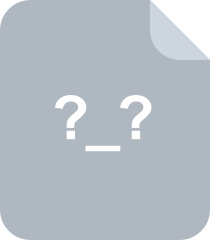
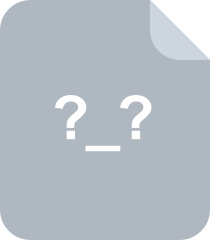
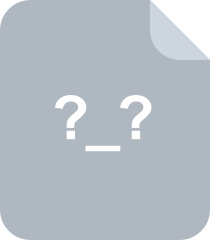
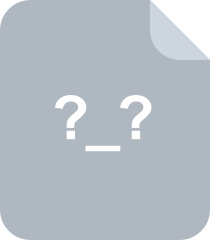
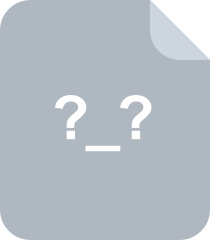
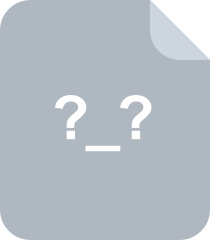
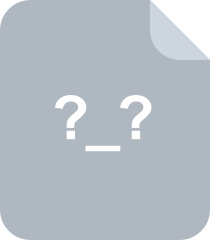
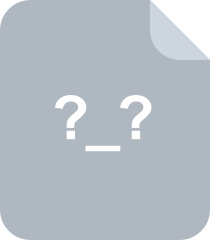
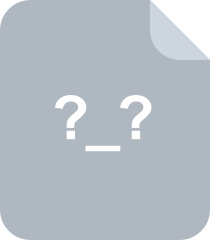
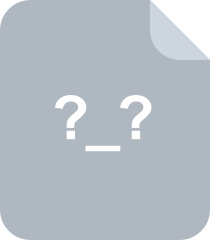
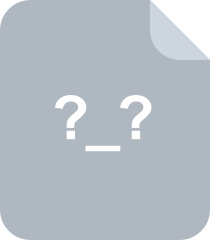
- 1
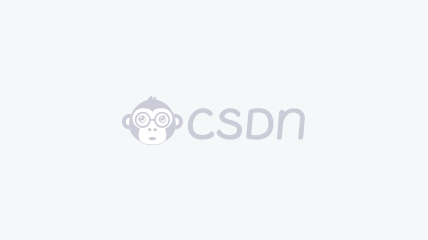
- 忠诚2012-12-04可以用,但很丑了
- 多大的试试2015-11-17测试通过 可以用谢谢
- Softboy0822012-06-15测试通过 可以用谢谢
- feng_36302013-06-22编译,测试能用
- 玛克洛斯2012-07-19编译,测试能用

- 粉丝: 708
- 资源: 641
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

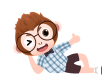
最新资源
- 此存储库收集了所有有趣的 Python 单行代码 欢迎随意提交你的代码!.zip
- 高考志愿智能推荐-JAVA-基于springBoot高考志愿智能推荐系统设计与实现
- 标准 Python 记录器的 Json 格式化程序.zip
- kernel-5.15-rc7.zip
- 来自我在 Udemy 上的完整 Python 课程的代码库 .zip
- 来自微软的免费 Edx 课程.zip
- c++小游戏猜数字(基础)
- 金铲铲S13双城之战自动拿牌助手
- x64dbg-development-2022-09-07-14-52.zip
- 多彩吉安红色旅游网站-JAVA-基于springBoot多彩吉安红色旅游网站的设计与实现

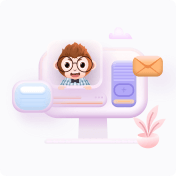
