package yh.Iwind;
import yh.Common.HttpDownloader;
import yh.Common.Statics;
import android.app.Activity;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.DashPathEffect;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.graphics.PathEffect;
import android.graphics.PointF;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.Typeface;
import android.os.Bundle;
import android.util.DisplayMetrics;
import android.util.FloatMath;
import android.util.Log;
import android.view.KeyEvent;
import android.view.MotionEvent;
import android.view.View;
import android.widget.Toast;
import yh.Common.Statics;
public class KLine extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
DisplayMetrics metrics = new DisplayMetrics();
this.getWindowManager().getDefaultDisplay().getMetrics(metrics);
// 屏幕的分辨率
int width = metrics.widthPixels;
int height = metrics.heightPixels;
setContentView(new MyCircle(this,this, width, height));
}
class MyCircle extends View {
private Context context;
/**
* 屏幕的宽
*/
private int width;
/**
* 屏幕的高
*/
private int height;
private Activity myact;
public DisplayMetrics metrics;
public int displayKValuenum=30;//当前显示多少值
public int MaxDisplayKvalueNum=40;//最大选择多少个
public int MinDisplayKvalueNum=5;//最少选择多少个
public int StockValueCount=0;//所有 股票值一共多少
public int startpos=0;//开始地址
public int endpos=0;//结束地址
public int leftrightpos=0;//左右偏移地址
public MyCircle(Context context, Activity mytt, int width, int height) {
super(context);
this.context = context;
this.width = width;
this.height = height;
myact=mytt;
setFocusable(true);
System.out.println("width="+width+"<---->height="+height);
}
@Override
protected void onDraw(Canvas canvas) {
metrics= new DisplayMetrics();
myact.getWindowManager().getDefaultDisplay().getMetrics(metrics);
// 屏幕的分辨率
int width = metrics.widthPixels;
int height = metrics.heightPixels;
/*文字开始*/
String ShangZhengZhiShu="3621.00";//上证 指数
String ShangZhiColor="R";//
String ShenZhengZHiShu="12161.21";//深证指数
String ShenZhiColor="G";//
String StockName="东北证券";//股票名称
String StockCode="000686";//股票代码
String NewPrice="6.33";//最新价
String UpPercent="1.1%";//上涨比例
String UpPrice="0.22";//上涨价格
String HighPrice="7.00";//最高价
String LowPrice="6.00";//最低价
String VOL="60000";//成交量
String Amount="1200000";//成交额
String OpenPrice="6.21";//今开
String ClosePrice="6.11";//昨收
Bitmap bitmaplogo = BitmapFactory.decodeResource( this.context.getResources(),yh.Iwind.R.drawable.icon);
int LogoHeight= bitmaplogo.getHeight();//Logo高度
HttpDownloader httpDownloader = new HttpDownloader();
String PriceListURLGet=getResources().getString(R.string.root_www)+"iwind/RealTimeList/GetNowPrice.ashx?stockcode="+Statics.MKT+Statics.CurStockCode;
String AllPriceStr= httpDownloader.download(PriceListURLGet);
String[] RtStr=AllPriceStr.split(",");
StockCode =RtStr[0];////0证券代码
StockName = RtStr[1];////1证券简称
ClosePrice = String.format("%1.2f", Float.parseFloat(RtStr[2]));////2昨日收盘价
OpenPrice = String.format("%1.2f", Float.parseFloat(RtStr[3]));////3今日开盘价
NewPrice = String.format("%1.2f", Float.parseFloat(RtStr[4]));////4最近成交价
VOL = String.format("%1.2f", Float.parseFloat(RtStr[5])/100/1000)+"万";////5成交数量
Amount = String.format("%1.2f", Float.parseFloat(RtStr[6])/100/1000)+"万";////6成交金额
HighPrice = String.format("%1.2f", Float.parseFloat(RtStr[8]));////8最高成交价
LowPrice= String.format("%1.2f", Float.parseFloat(RtStr[9]));////9最低成交价
float zhangdie=Float.parseFloat(RtStr[4])- Float.parseFloat(RtStr[2]);//涨跌
float zhangfu= (zhangdie)/Float.parseFloat(RtStr[2]);//涨幅
UpPercent=String.format("%1.2f",zhangfu)+"%";
UpPrice=String.format("%1.2f",zhangdie);
String LeftDesc1Str="7.0";//左大1
String LeftDesc2Str="6.7";//左大2
String LeftDesc3Str="6.5";//左大3
String LeftDesc4Str="6.3";//左大4
String LeftDesc5Str="6.0";//左大5
//指数
String ZSURLGet=getResources().getString(R.string.root_www)+"iwind/RealTimeList/BackZhiShu.ashx";
String szszzhishu= httpDownloader.download(ZSURLGet);
String[] ZSRtStr=szszzhishu.split(",");
ShangZhengZhiShu=ZSRtStr[0];//上证 指数
ShangZhiColor=ZSRtStr[1];//
ShenZhengZHiShu=ZSRtStr[2];//深证指数
ShenZhiColor=ZSRtStr[3];//
/*文字结束*/
//设置线条粗细
float alltop=60;
float allleft=10;
float allwidth=310;//整个宽度
float allheight=280;//整个高度
float descheight=60;//说明高度
float allscreen=Float.valueOf(String.valueOf(height));//整个屏幕高度 89为上文字 35为标
float allscreenw=Float.valueOf(String.valueOf(width));
float alllogoheight=Float.valueOf(String.valueOf(LogoHeight));
allheight=allscreen-alltop-descheight-alllogoheight-45;
allwidth=allscreenw;
float everyNodestep=allwidth/240;//每分钟的步长
allwidth=everyNodestep*240;
/*前期逻辑值计算*/
//间距以及柱的宽度
float xstepvalue=allwidth/(displayKValuenum);
String URLGetCount=getResources().getString(R.string.root_www)+"iwind/realtimelist/GetCount.ashx?stockcode="+Statics.CurStockCode;
String DataCountStr=httpDownloader.download(URLGetCount);
StockValueCount=Integer.parseInt(DataCountStr);//所有的个数
if(StockValueCount>displayKValuenum)//如果所有的个数大于一页
{
startpos=StockValueCount-Statics.KLineCurNum*displayKValuenum;
if(startpos>displayKValuenum)
{
endpos=startpos+displayKValuenum+leftrightpos;
Log.e("YH",String.valueOf(leftrightpos));
}
else//最后一页
{
endpos=startpos;
startpos=0;
}
}
else//如果所有的个数小于一页
{
startpos=0;
endpos=StockValueCount;
}
/*文字解析开始*/
String URLGetContent=getResources().getString(R.string.root_www)+ "iwind/realtimelist/AndroidBackKLine.ashx?stockcode="+Statics.CurStockCode+"&start="+St
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
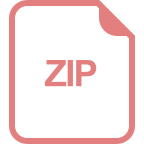
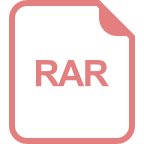
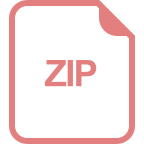
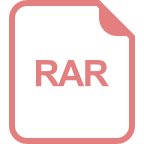
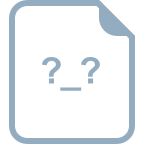
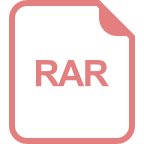
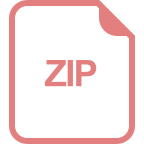
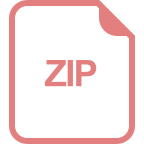
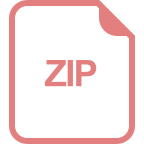
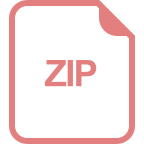
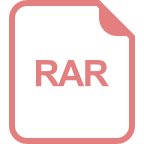
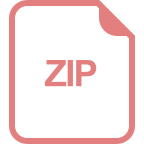
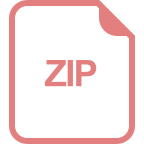
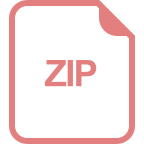
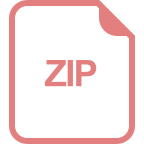
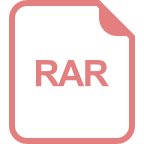
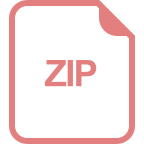
收起资源包目录

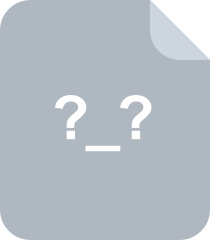
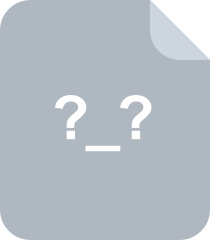

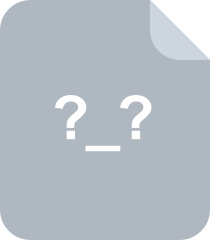
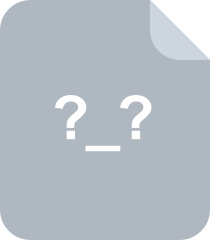


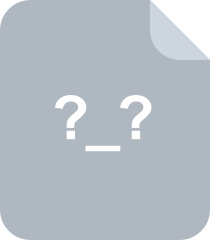
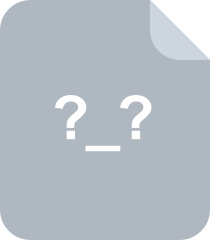
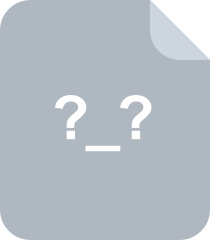
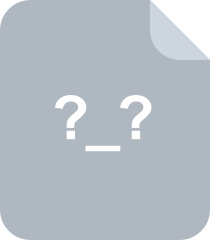
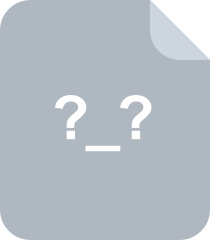
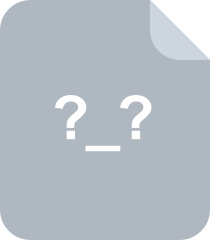
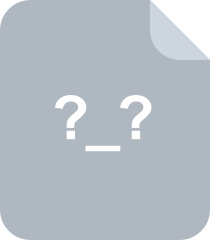
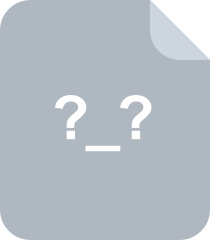
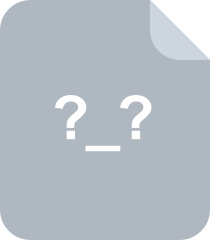
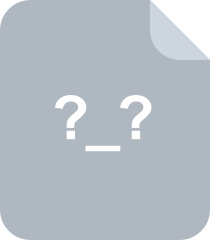
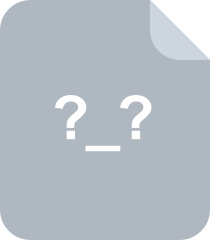
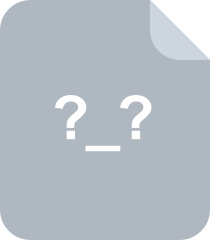
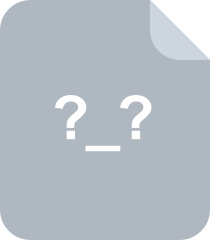
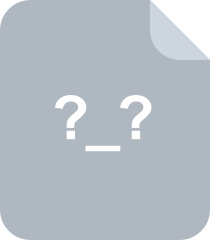
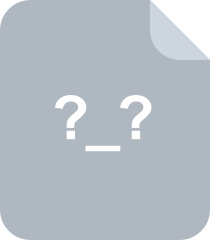
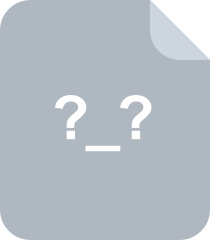
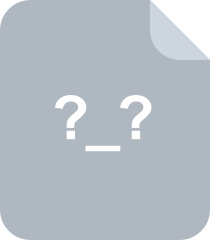
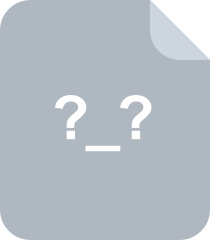
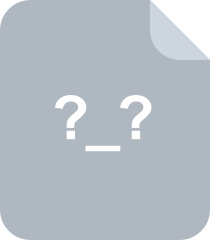
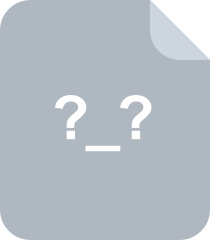
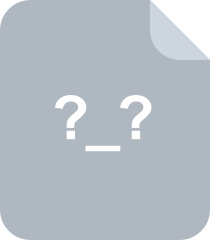
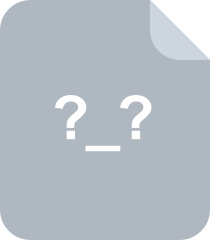

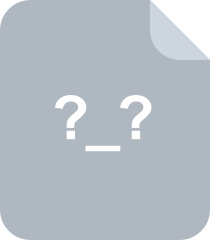
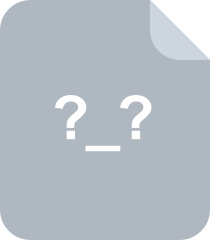
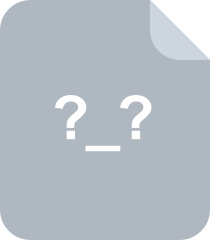
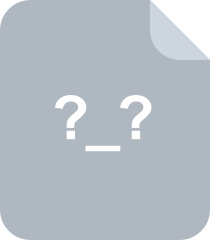
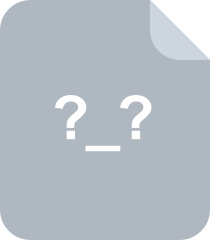

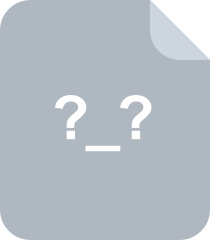
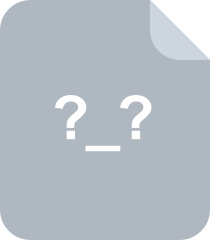
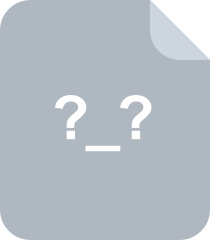
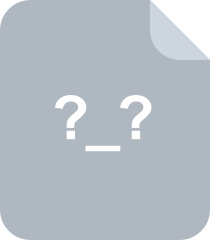
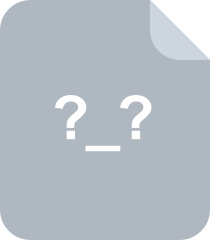
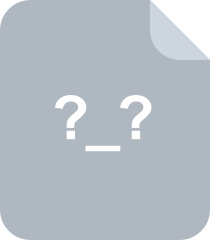



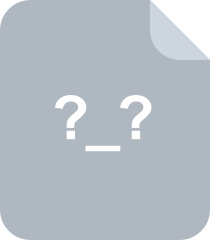
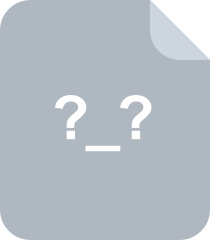
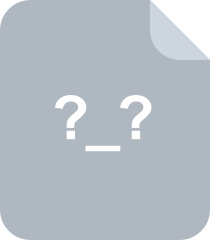
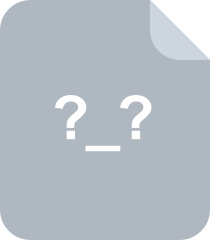
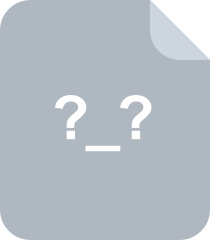
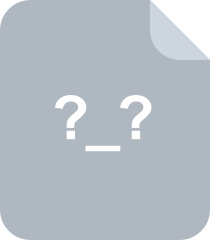

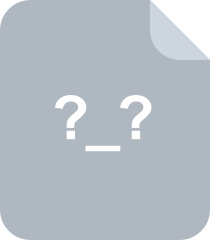
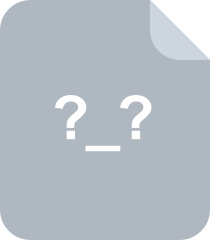
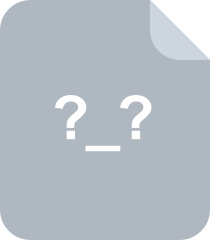
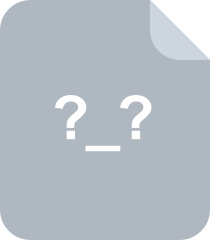
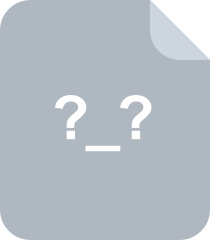

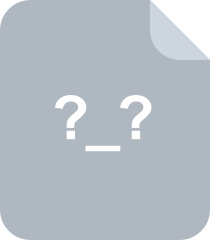
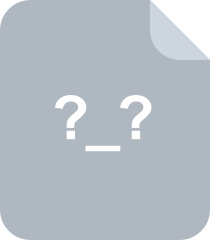
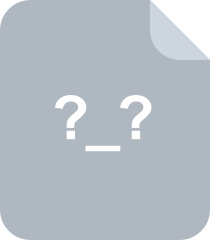


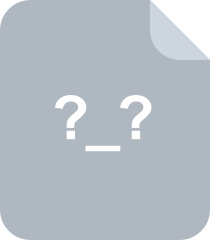

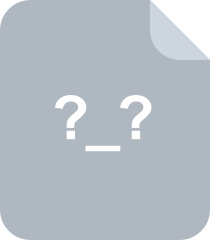
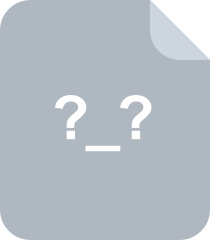
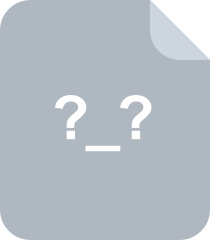
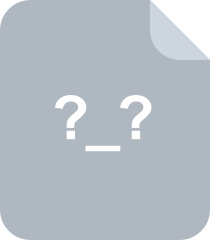
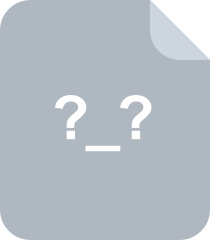

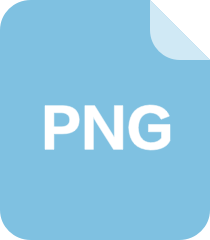

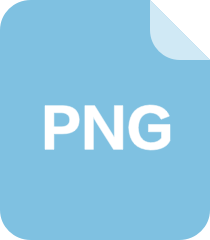
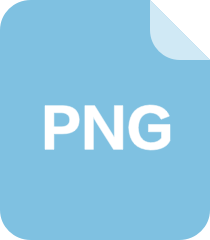
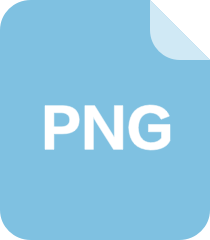
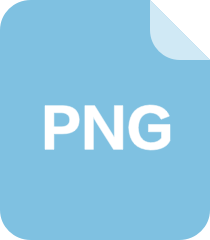
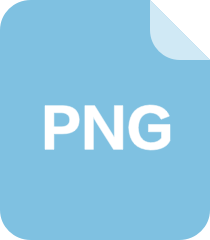
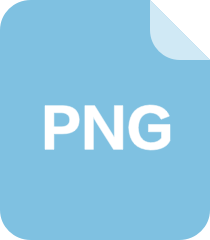
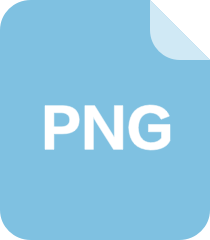
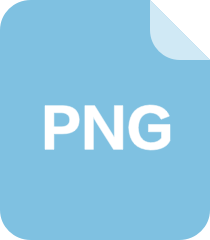
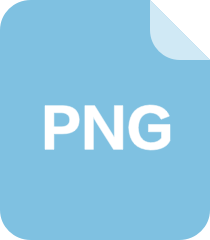
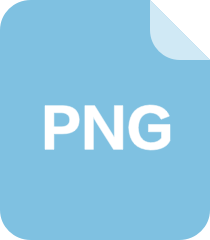
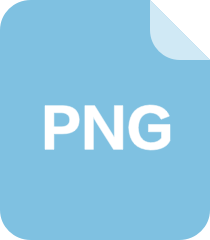
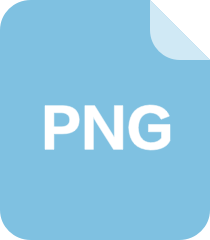
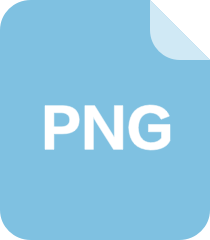
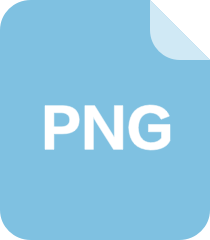
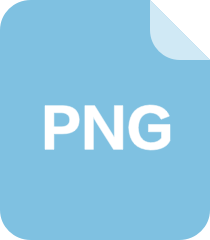
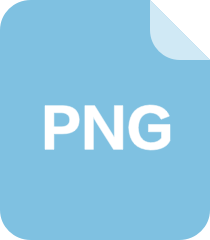
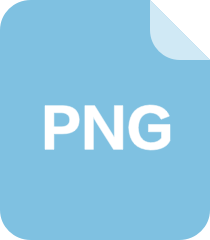
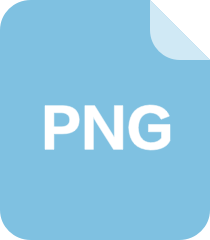
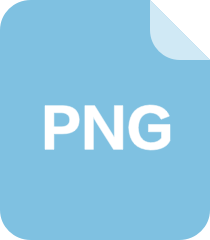
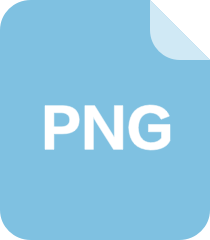
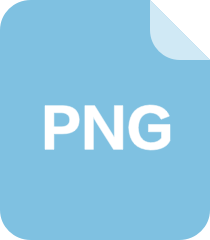

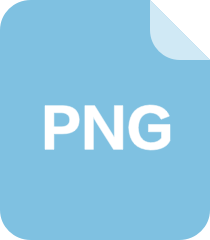
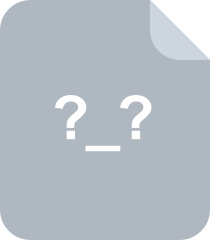



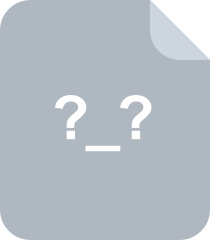


共 82 条
- 1

杨航AI
- 粉丝: 710
- 资源: 641
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

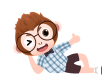
最新资源
- Matlab根据flac、pfc或其他软件导出的坐标及应力、位移数据再现云图 案例包括导出在flac6.0中导出位移的fish代码(也可以自己先准备软件导出的坐标数据及对应点的位移或应力数据,可根据需
- 拳皇97.exe拳皇972.exe拳皇973.exe
- 捕鱼达人1.exe捕鱼达人2.exe捕鱼达人3.exe
- 医疗骨折摄像检测29-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma数据集合集.rar
- ks滑块加密算法与源代码
- 医护人员检测23-YOLOv8数据集合集.rar
- 1.电力系统短路故障引起电压暂降 2.不对称短路故障分析 包括:共两份自编word+相应matlab模型 1.短路故障的发生频次以及不同类型短路故障严重程度,本文选取三类典型的不对称短路展开研究
- C#连接sap NCO组件 X64版
- 开源基于51单片机的多功能智能闹钟设计,课设毕设借鉴参考
- 深度强化学习电气工程复现文章,适合小白学习 关键词:能量管理 深度学习 强化学习 深度强化学习 能源系统 优化调度 编程语言:python平台 主题:用于能源系统优化调度的深度强化学习算法的性能比较
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


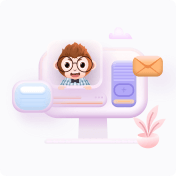
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页