# ----------------------------------------------------------------------------
# Root CMake file for OpenCV
#
# From the off-tree build directory, invoke:
# $ cmake <PATH_TO_OPENCV_ROOT>
#
# ----------------------------------------------------------------------------
# Disable in-source builds to prevent source tree corruption.
if(" ${CMAKE_SOURCE_DIR}" STREQUAL " ${CMAKE_BINARY_DIR}")
message(FATAL_ERROR "
FATAL: In-source builds are not allowed.
You should create a separate directory for build files.
")
endif()
include(cmake/OpenCVMinDepVersions.cmake)
if(CMAKE_GENERATOR MATCHES Xcode AND XCODE_VERSION VERSION_GREATER 4.3)
cmake_minimum_required(VERSION 3.0 FATAL_ERROR)
elseif(CMAKE_SYSTEM_NAME MATCHES WindowsPhone OR CMAKE_SYSTEM_NAME MATCHES WindowsStore)
cmake_minimum_required(VERSION 3.1 FATAL_ERROR)
#Required to resolve linker error issues due to incompatibility with CMake v3.0+ policies.
#CMake fails to find _fseeko() which leads to subsequent linker error.
#See details here: http://www.cmake.org/Wiki/CMake/Policies
cmake_policy(VERSION 2.8)
else()
cmake_minimum_required(VERSION "${MIN_VER_CMAKE}" FATAL_ERROR)
endif()
option(ENABLE_PIC "Generate position independent code (necessary for shared libraries)" TRUE)
set(CMAKE_POSITION_INDEPENDENT_CODE ${ENABLE_PIC})
set(OPENCV_MATHJAX_RELPATH "https://cdnjs.cloudflare.com/ajax/libs/mathjax/2.7.0" CACHE STRING "URI to a MathJax installation")
# Following block can break build in case of cross-compiling
# but CMAKE_CROSSCOMPILING variable will be set only on project(OpenCV) command
# so we will try to detect cross-compiling by the presence of CMAKE_TOOLCHAIN_FILE
if(NOT DEFINED CMAKE_INSTALL_PREFIX)
if(NOT CMAKE_TOOLCHAIN_FILE)
# it _must_ go before project(OpenCV) in order to work
if(WIN32)
set(CMAKE_INSTALL_PREFIX "${CMAKE_BINARY_DIR}/install" CACHE PATH "Installation Directory")
else()
set(CMAKE_INSTALL_PREFIX "/usr/local" CACHE PATH "Installation Directory")
endif()
else()
#Android: set output folder to ${CMAKE_BINARY_DIR}
set(LIBRARY_OUTPUT_PATH_ROOT ${CMAKE_BINARY_DIR} CACHE PATH "root for library output, set this to change where android libs are compiled to" )
# any cross-compiling
set(CMAKE_INSTALL_PREFIX "${CMAKE_BINARY_DIR}/install" CACHE PATH "Installation Directory")
endif()
endif()
if(POLICY CMP0026)
cmake_policy(SET CMP0026 NEW)
endif()
if(POLICY CMP0042)
cmake_policy(SET CMP0042 NEW)
endif()
if(POLICY CMP0046)
cmake_policy(SET CMP0046 NEW)
endif()
if(POLICY CMP0051)
cmake_policy(SET CMP0051 NEW)
endif()
if(POLICY CMP0054) # CMake 3.1: Only interpret if() arguments as variables or keywords when unquoted.
cmake_policy(SET CMP0054 NEW)
endif()
if(POLICY CMP0056)
cmake_policy(SET CMP0056 NEW)
endif()
if(POLICY CMP0067)
cmake_policy(SET CMP0067 NEW)
endif()
if(POLICY CMP0068)
cmake_policy(SET CMP0068 NEW) # CMake 3.9+: `RPATH` settings on macOS do not affect `install_name`.
endif()
include(cmake/OpenCVUtils.cmake)
ocv_cmake_reset_hooks()
ocv_check_environment_variables(OPENCV_CMAKE_HOOKS_DIR)
if(DEFINED OPENCV_CMAKE_HOOKS_DIR)
foreach(__dir ${OPENCV_CMAKE_HOOKS_DIR})
get_filename_component(__dir "${__dir}" ABSOLUTE)
ocv_cmake_hook_register_dir(${__dir})
endforeach()
endif()
ocv_cmake_hook(CMAKE_INIT)
# must go before the project command
ocv_update(CMAKE_CONFIGURATION_TYPES "Debug;Release" CACHE STRING "Configs" FORCE)
if(DEFINED CMAKE_BUILD_TYPE)
set_property( CACHE CMAKE_BUILD_TYPE PROPERTY STRINGS ${CMAKE_CONFIGURATION_TYPES} )
endif()
enable_testing()
project(OpenCV CXX C)
if(CMAKE_SYSTEM_NAME MATCHES WindowsPhone OR CMAKE_SYSTEM_NAME MATCHES WindowsStore)
set(WINRT TRUE)
endif()
if(WINRT OR WINCE)
add_definitions(-DNO_GETENV)
endif()
if(WINRT)
add_definitions(-DWINRT)
# Making definitions available to other configurations and
# to filter dependency restrictions at compile time.
if(CMAKE_SYSTEM_NAME MATCHES WindowsPhone)
set(WINRT_PHONE TRUE)
add_definitions(-DWINRT_PHONE)
elseif(CMAKE_SYSTEM_NAME MATCHES WindowsStore)
set(WINRT_STORE TRUE)
add_definitions(-DWINRT_STORE)
endif()
if(CMAKE_SYSTEM_VERSION MATCHES 10)
set(WINRT_10 TRUE)
add_definitions(-DWINRT_10)
elseif(CMAKE_SYSTEM_VERSION MATCHES 8.1)
set(WINRT_8_1 TRUE)
add_definitions(-DWINRT_8_1)
elseif(CMAKE_SYSTEM_VERSION MATCHES 8.0)
set(WINRT_8_0 TRUE)
add_definitions(-DWINRT_8_0)
endif()
endif()
if(MSVC)
set(CMAKE_USE_RELATIVE_PATHS ON CACHE INTERNAL "" FORCE)
endif()
ocv_cmake_eval(DEBUG_PRE ONCE)
ocv_clear_vars(OpenCVModules_TARGETS)
include(cmake/OpenCVDownload.cmake)
set(BUILD_LIST "" CACHE STRING "Build only listed modules (comma-separated, e.g. 'videoio,dnn,ts')")
# ----------------------------------------------------------------------------
# Break in case of popular CMake configuration mistakes
# ----------------------------------------------------------------------------
if(NOT CMAKE_SIZEOF_VOID_P GREATER 0)
message(FATAL_ERROR "CMake fails to determine the bitness of the target platform.
Please check your CMake and compiler installation. If you are cross-compiling then ensure that your CMake toolchain file correctly sets the compiler details.")
endif()
# ----------------------------------------------------------------------------
# Detect compiler and target platform architecture
# ----------------------------------------------------------------------------
include(cmake/OpenCVDetectCXXCompiler.cmake)
ocv_cmake_hook(POST_DETECT_COMPILER)
# Add these standard paths to the search paths for FIND_LIBRARY
# to find libraries from these locations first
if(UNIX AND NOT ANDROID)
if(X86_64 OR CMAKE_SIZEOF_VOID_P EQUAL 8)
if(EXISTS /lib64)
list(APPEND CMAKE_LIBRARY_PATH /lib64)
else()
list(APPEND CMAKE_LIBRARY_PATH /lib)
endif()
if(EXISTS /usr/lib64)
list(APPEND CMAKE_LIBRARY_PATH /usr/lib64)
else()
list(APPEND CMAKE_LIBRARY_PATH /usr/lib)
endif()
elseif(X86 OR CMAKE_SIZEOF_VOID_P EQUAL 4)
if(EXISTS /lib32)
list(APPEND CMAKE_LIBRARY_PATH /lib32)
else()
list(APPEND CMAKE_LIBRARY_PATH /lib)
endif()
if(EXISTS /usr/lib32)
list(APPEND CMAKE_LIBRARY_PATH /usr/lib32)
else()
list(APPEND CMAKE_LIBRARY_PATH /usr/lib)
endif()
endif()
endif()
# Add these standard paths to the search paths for FIND_PATH
# to find include files from these locations first
if(MINGW)
if(EXISTS /mingw)
list(APPEND CMAKE_INCLUDE_PATH /mingw)
endif()
if(EXISTS /mingw32)
list(APPEND CMAKE_INCLUDE_PATH /mingw32)
endif()
if(EXISTS /mingw64)
list(APPEND CMAKE_INCLUDE_PATH /mingw64)
endif()
endif()
# ----------------------------------------------------------------------------
# OpenCV cmake options
# ----------------------------------------------------------------------------
OCV_OPTION(OPENCV_ENABLE_NONFREE "Enable non-free algorithms" OFF)
# 3rd party libs
OCV_OPTION(OPENCV_FORCE_3RDPARTY_BUILD "Force using 3rdparty code from source" OFF)
OCV_OPTION(BUILD_ZLIB "Build zlib from source" (WIN32 OR APPLE OR OPENCV_FORCE_3RDPARTY_BUILD) )
OCV_OPTION(BUILD_TIFF "Build libtiff from source" (WIN32 OR ANDROID OR APPLE OR OPENCV_FORCE_3RDPARTY_BUILD) )
OCV_OPTION(BUILD_JASPER "Build libjasper from source" (WIN32 OR ANDROID OR APPLE OR OPENCV_FORCE_3RDPARTY_BUILD) )
OCV_OPTION(BUILD_JPEG "Build libjpeg from source" (WIN32 OR ANDROID OR APPLE OR OPENCV_FORCE_3RDPARTY_BUILD) )
OCV_OPTION(BUILD_PNG "Build libpng from source" (WIN32 OR ANDROID OR APPLE OR OPENCV_FORCE_3RDPARTY_BUILD) )
OCV_OPTION(BUILD_OPENEXR "Build openexr from source" (((WIN32 OR ANDROID OR APPLE) AND NOT WINRT) OR OPENCV_FORCE_3RDPARTY_BUILD) )
OCV_OPTION(BUILD_WEBP "Build WebP from source" (((WIN32 OR AND
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
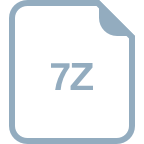
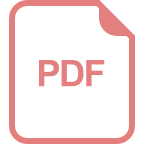
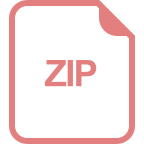
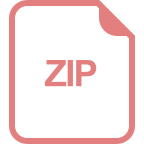
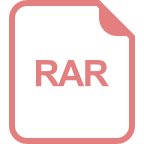
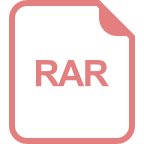
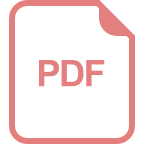
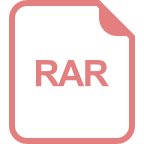
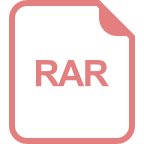
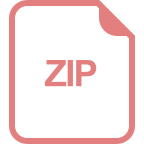
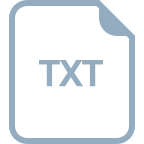
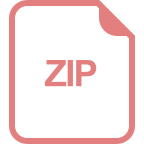
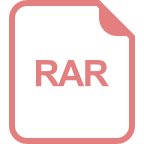
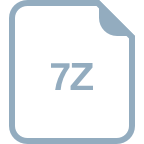
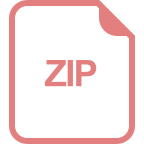
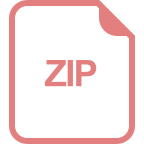
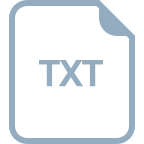
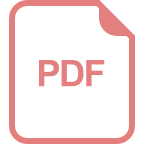
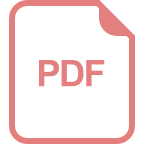
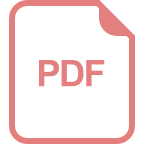
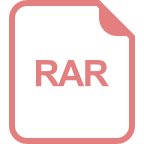
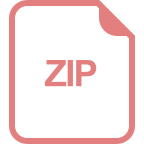
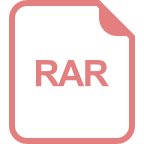
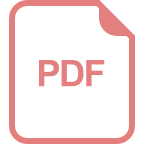
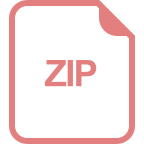
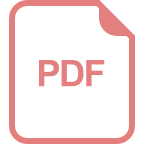
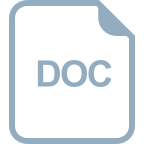
收起资源包目录

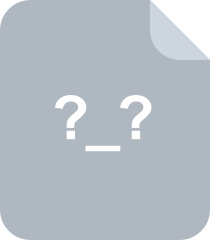
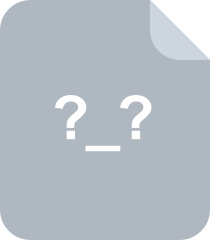
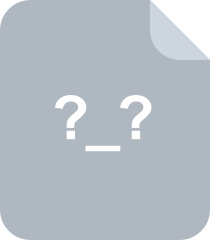
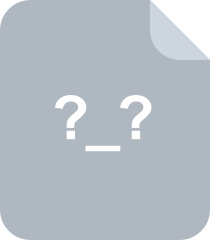
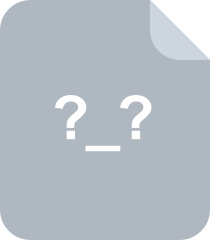
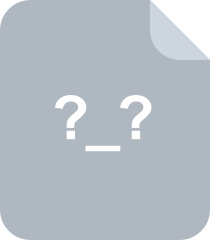
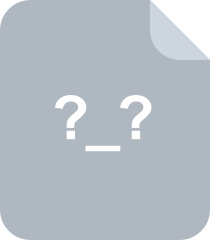
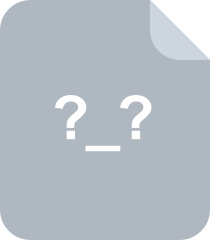
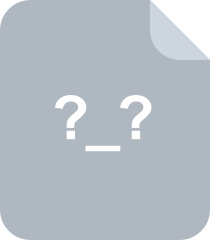
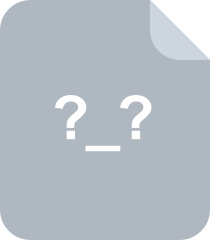
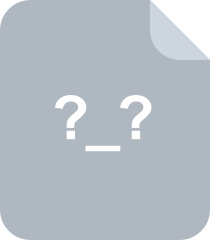
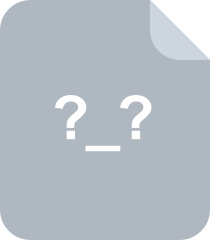
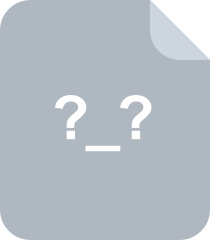
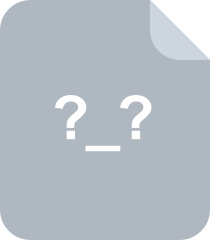
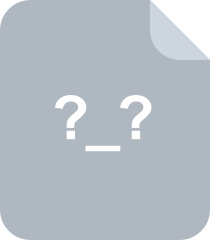
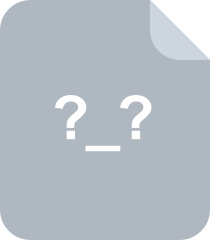
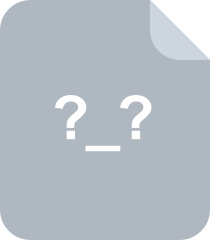
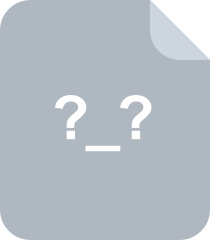
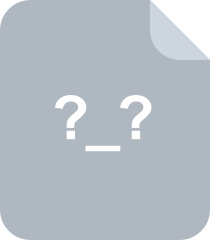
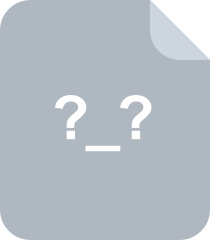
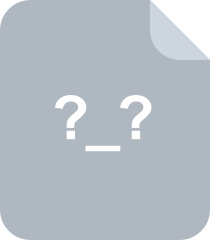
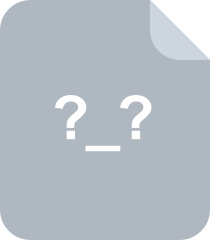
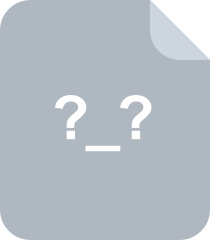
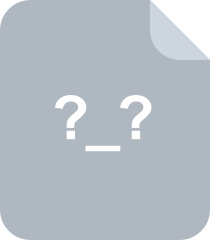
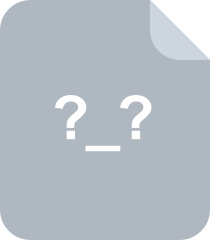
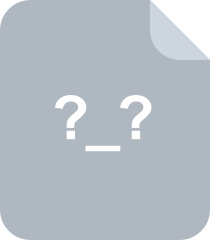
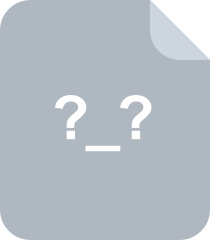
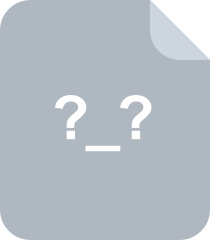
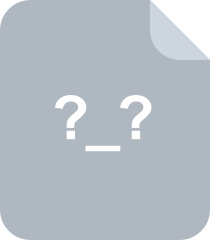
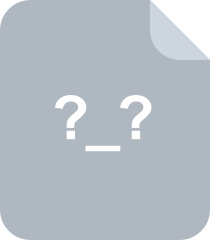
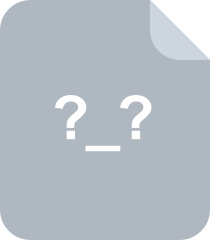
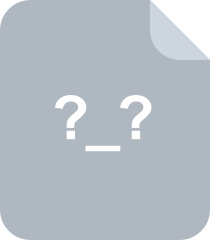
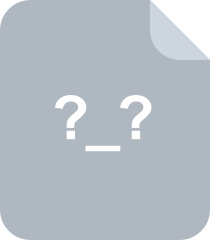
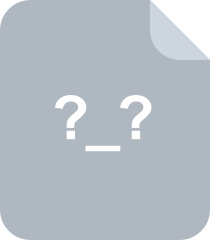
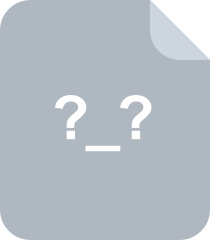
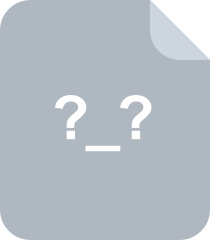
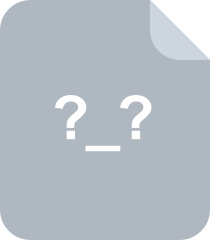
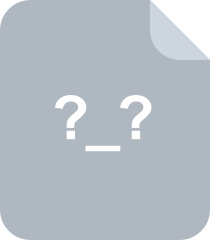
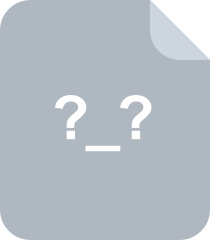
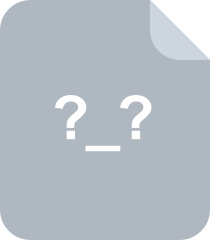
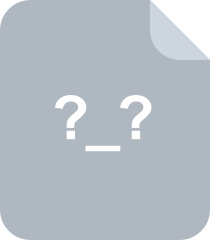
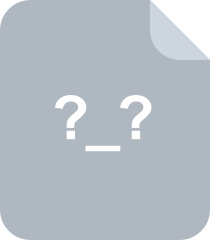
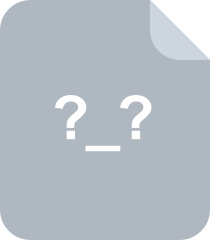
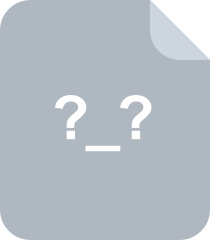
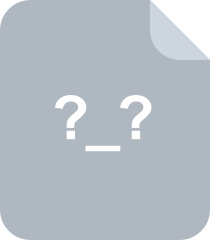
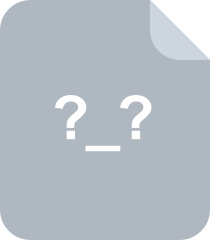
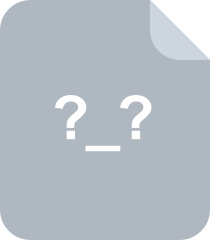
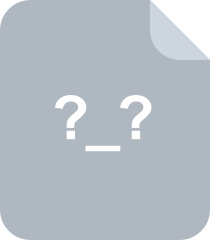
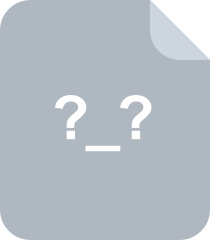
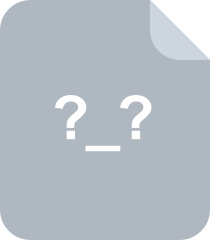
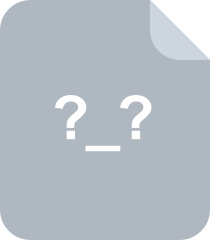
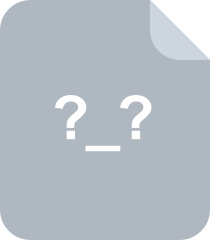
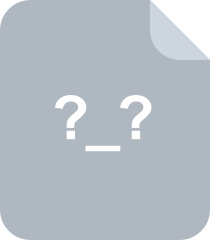
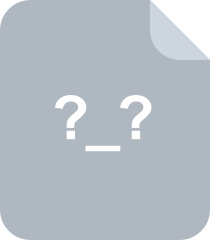
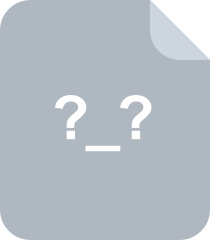
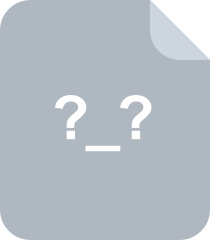
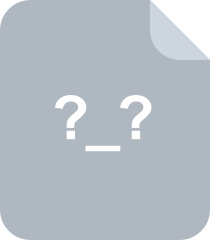
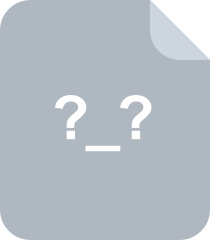
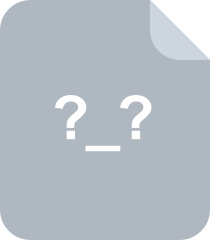
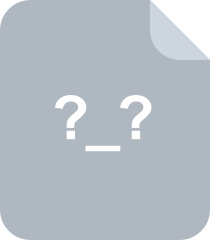
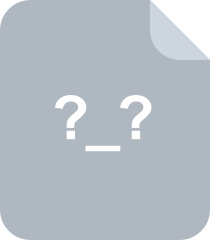
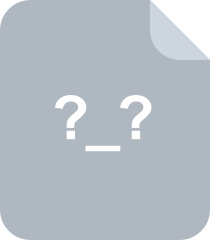
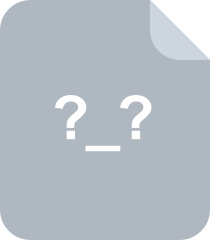
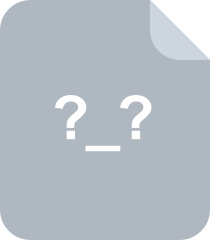
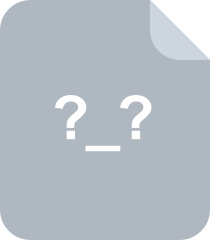
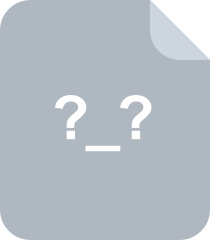
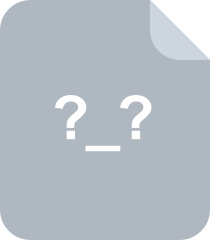
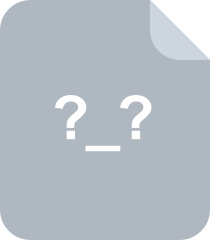
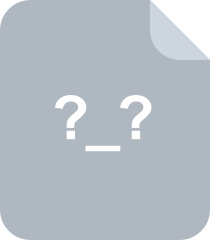
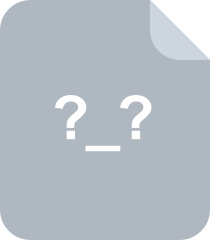
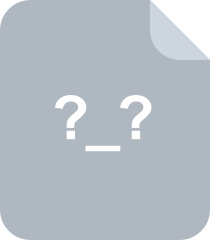
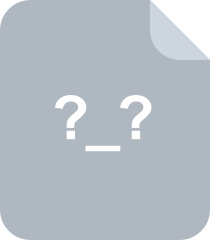
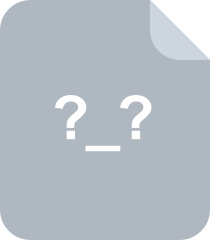
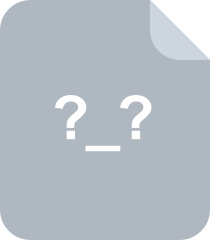
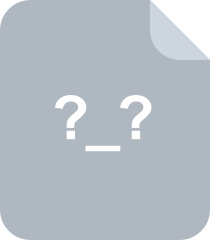
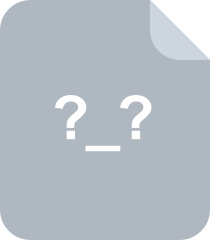
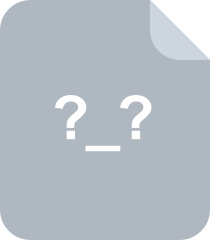
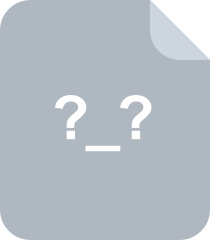
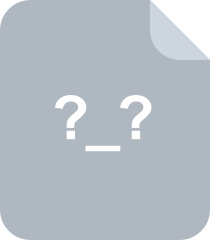
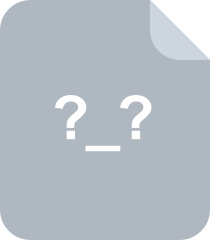
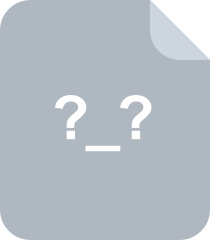
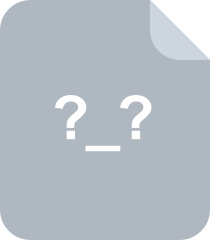
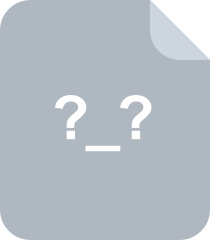
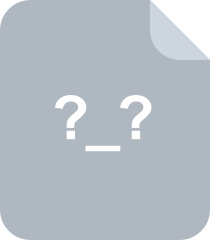
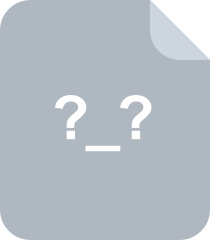
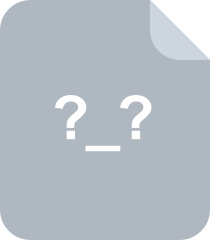
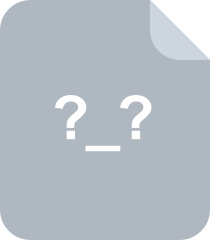
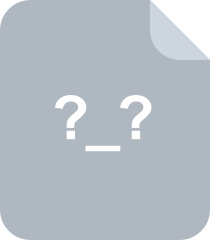
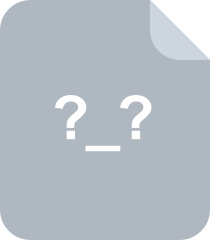
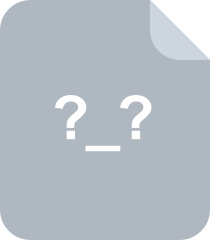
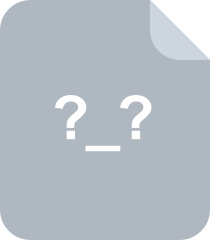
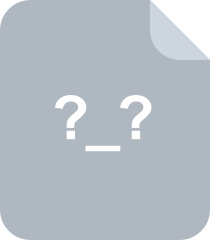
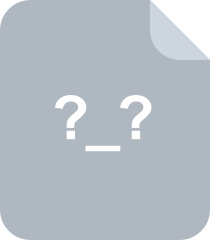
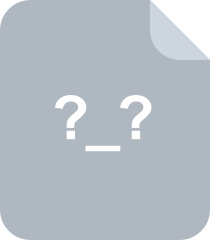
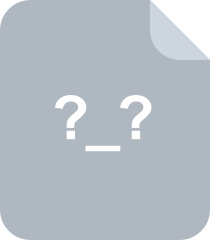
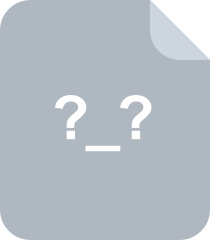
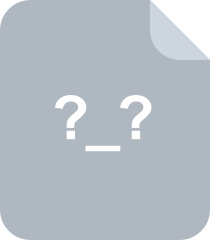
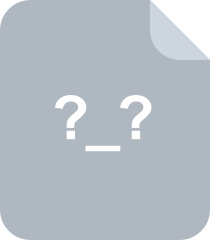
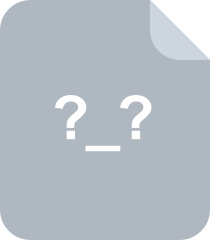
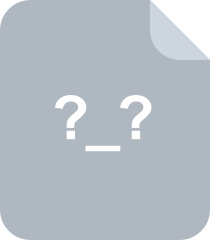
共 2010 条
- 1
- 2
- 3
- 4
- 5
- 6
- 21
资源评论
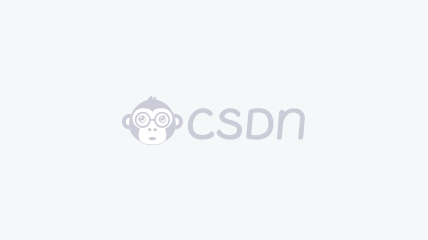

hakesashou
- 粉丝: 6764
- 资源: 1678
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

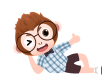
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


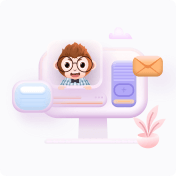
安全验证
文档复制为VIP权益,开通VIP直接复制
