iPhone编程解析xml
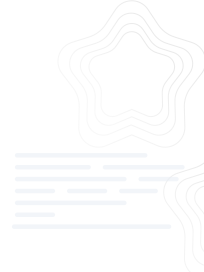


在iOS开发中,XML(eXtensible Markup Language)是一种常用的数据交换格式,它具有结构化、可扩展的特性,广泛应用于网络数据传输。本教程将深入探讨如何在iPhone应用程序中解析XML文件,主要涉及的技术点包括Objective-C编程、网络连接以及NSXMLParser类的使用。 我们要了解XML的基本结构。XML文档由元素、属性、文本内容等组成,通过层次化的标签来表达数据。在iPhone应用中,我们通常从服务器获取XML数据,这些数据可以是天气预报、新闻信息或者游戏数据等。 要解析XML,我们需要使用苹果提供的NSXMLParser类。NSXMLParser是一个基于事件驱动的解析器,它会在读取XML文档时触发一系列的回调方法。我们创建一个NSXMLParser的代理对象,实现这些方法,从而处理XML数据。 在开始解析之前,我们需要从网络获取XML数据。这可以通过URLSession或者第三方库如AFNetworking来实现。创建一个GET请求,获取到XML数据的URL,然后设置一个完成块,接收返回的XML字符串。 ```objc NSURL *url = [NSURL URLWithString:@"http://example.com/data.xml"]; NSURLSessionDataTask *task = [[NSURLSession sharedSession] dataTaskWithURL:url completionHandler:^(NSData * _Nullable data, NSURLResponse * _Nullable response, NSError * _Nullable error) { if (error) { NSLog(@"Error fetching XML: %@", error.localizedDescription); } else { // 创建XML解析器并开始解析 NSXMLParser *parser = [[NSXMLParser alloc] initWithData:data]; parser.delegate = self; // 设置代理 [parser parse]; // 开始解析 } }]; [task resume]; ``` 接着,我们需要实现NSXMLParserDelegate协议的方法。关键的方法有: 1. `parser:didStartElement:namespaceURI:qualifiedName:attributes:`:当解析器遇到开始标签时调用,可以在这里初始化数据结构,准备存储即将解析的数据。 2. `parser:foundCharacters:`:在解析到元素的文本内容时调用,将接收到的字符添加到当前元素的值中。 3. `parser:didEndElement:namespaceURI:qualifiedName:`:遇到结束标签时调用,可以在这里处理完当前元素的数据,并将其添加到适当的数据模型中。 4. `parser:parseErrorOccurred:`:如果解析过程中出现错误,这个方法会被调用,可以在这里捕获并处理错误。 例如,如果我们解析的XML如下: ```xml <items> <item id="1"> <title>Item 1</title> <description>Description for item 1</description> </item> <item id="2"> <title>Item 2</title> <description>Description for item 2</description> </item> </items> ``` 我们可以创建一个`Item`模型来存储解析的结果,并在代理方法中填充数据: ```objc @interface Item : NSObject @property (nonatomic, strong) NSString *itemId; @property (nonatomic, strong) NSString *title; @property (nonatomic, strong) NSString *description; @end @implementation Item // ... getters and setters @end @interface YourXMLParser : NSObject <NSXMLParserDelegate> @property (nonatomic, strong) NSMutableArray<Item *> *items; @end @implementation YourXMLParser - (void)parser:(NSXMLParser *)parser didStartElement:(NSString *)elementName namespaceURI:(NSString *)namespaceURI qualifiedName:(NSString *)qName attributes:(NSDictionary<NSString *, NSString *> *)attributeDict { if ([elementName isEqualToString:@"item"]) { Item *item = [[Item alloc] init]; item.itemId = attributeDict[@"id"]; self.currentItem = item; } else if (self.currentItem && [elementName isEqualToString:@"title"]) { self.currentItemTitle = @""; } else if (self.currentItem && [elementName isEqualToString:@"description"]) { self.currentItemDescription = @""; } } - (void)parser:(NSXMLParser *)parser foundCharacters:(NSString *)string { if (self.currentItemTitle) { self.currentItemTitle += string; } else if (self.currentItemDescription) { self.currentItemDescription += string; } } - (void)parser:(NSXMLParser *)parser didEndElement:(NSString *)elementName namespaceURI:(NSString *)namespaceURI qualifiedName:(NSString *)qName { if ([elementName isEqualToString:@"item"]) { self.items addObject:self.currentItem; self.currentItem = nil; } else if (self.currentItem && [elementName isEqualToString:@"title"]) { self.currentItem.title = self.currentItemTitle; self.currentItemTitle = nil; } else if (self.currentItem && [elementName isEqualToString:@"description"]) { self.currentItem.description = self.currentItemDescription; self.currentItemDescription = nil; } } @end ``` 完成XML解析后,你可以在应用中使用这些数据,比如显示在UITableView或者UICollectionView中。 HSXMLSample项目可能包含了一个完整的示例代码,你可以参考该项目来学习如何在iPhone应用中实现XML解析。通过这个项目,你可以了解到从网络获取数据、解析XML以及如何利用解析后的数据进行实际操作的具体步骤。记得在实际开发中,考虑到性能和内存管理,可以使用GCD异步加载XML数据,以及使用KVO(Key-Value Observing)或者通知来更新UI。
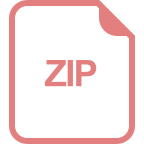
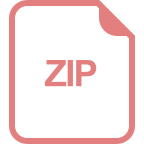
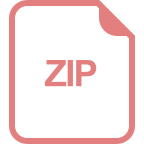
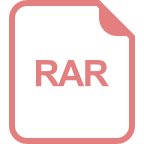
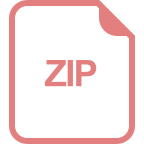
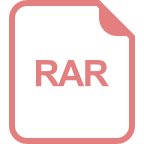
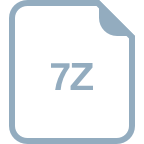
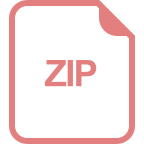
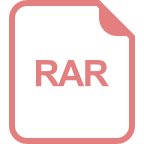
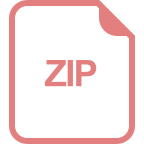
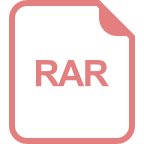
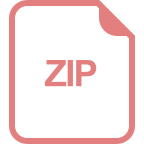
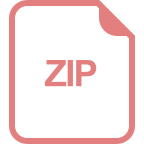
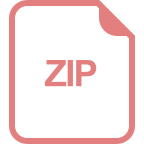
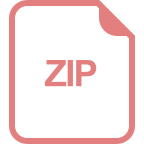
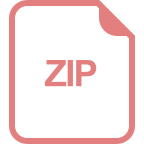
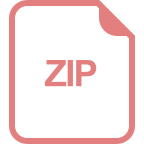
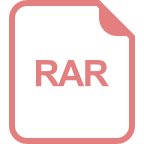
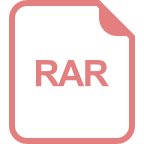
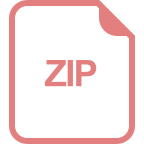
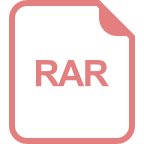
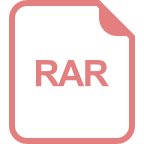
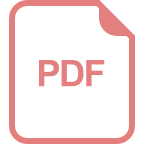
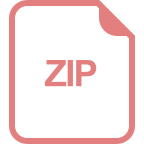
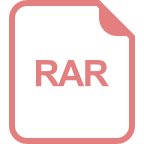



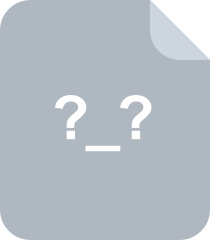
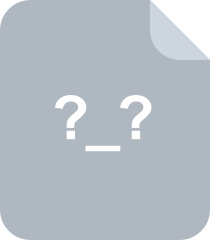
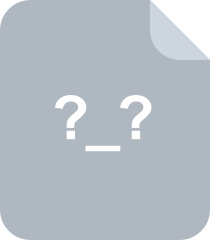
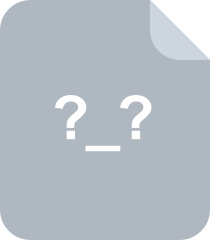
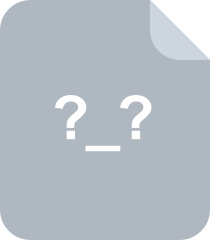
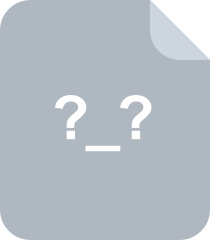
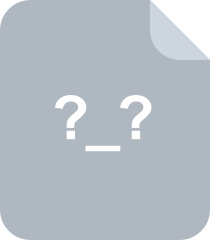

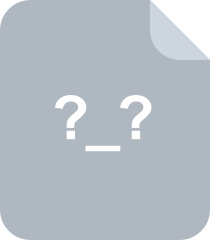

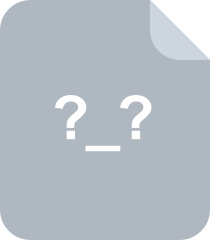

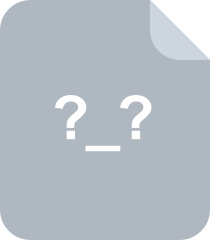
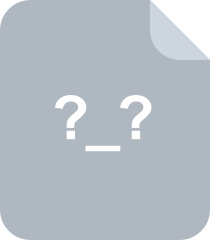
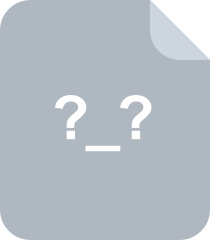
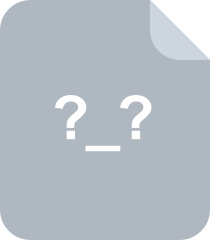
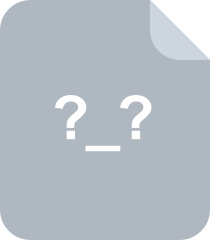
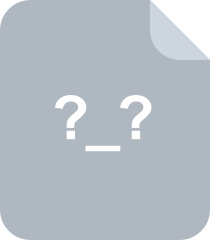
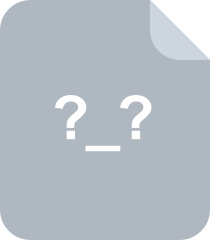
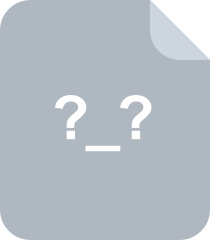
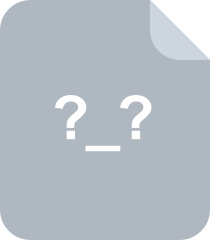
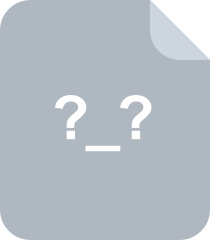
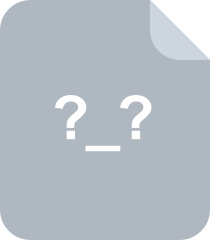
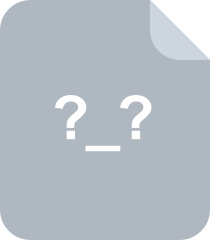



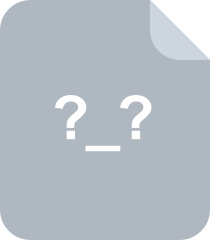




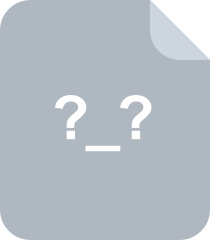
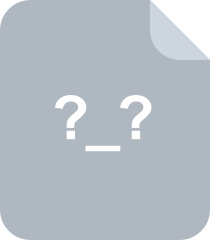


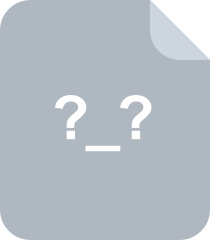

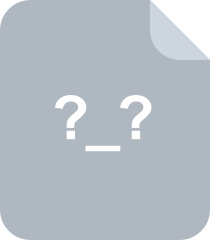

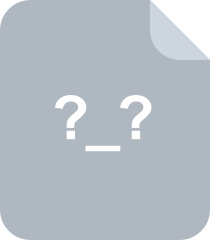


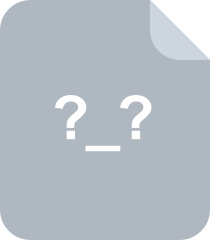

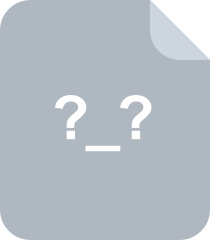

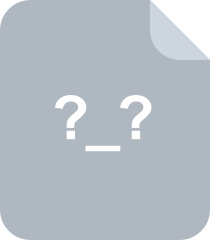

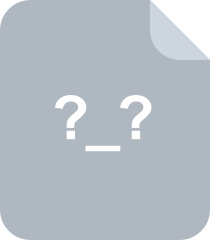

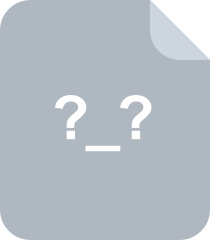


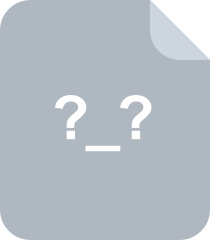

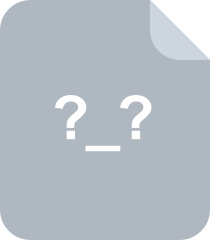

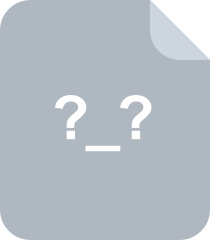

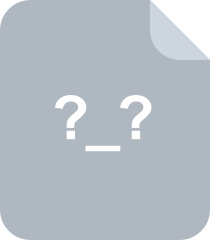

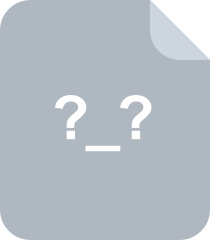
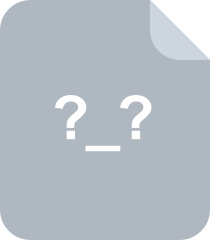
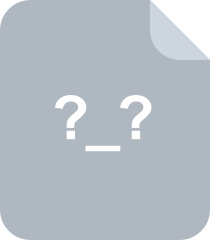
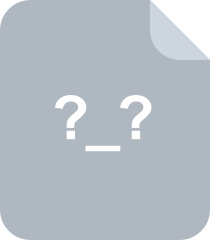


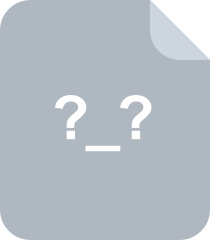


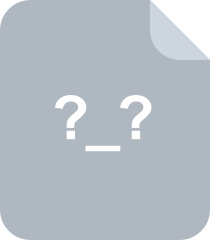
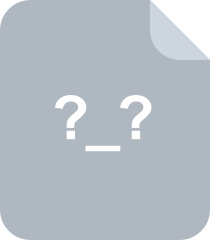
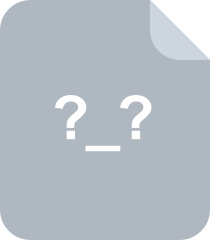



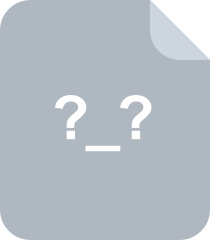
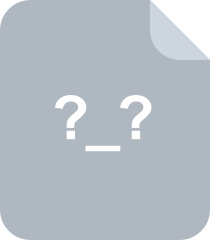
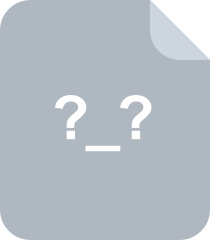
- 1
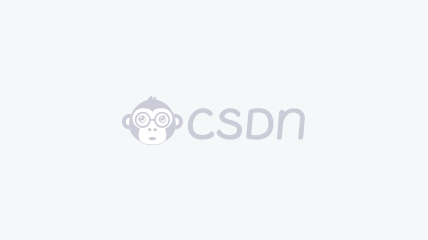
- taotao28162013-07-14这个资料很不完整,不知道解析对象是哪个
- gujigujifeifei2011-10-25是对哪个xml的解析?
- celf7772014-03-20这个资料很不完整,不知道解析对象是哪个

- 粉丝: 1086
- 资源: 22
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

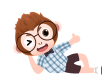
最新资源
- 新年倒计时网页基础教程
- Python编程初学者快速入门基础教程
- 新年倒计时编程基础教程
- 峰会报告自动化处理基础教程
- UE4UE5游戏开发基础教程:从零开始构建你的世界
- DataStructure-拓扑排序
- Front-end-learning-to-organize-notes-新年主题资源
- QPython Plus-Python资源
- baidulite-新年主题资源
- CnOCR-Python资源
- Golang_Puzzlers-新年主题资源
- Python开源扫雷游戏PyMine-Python资源
- Golang_Puzzlers-新年主题资源
- pyporter-Python资源
- Golang_Puzzlers-新年主题资源
- mulan-rework-Python资源

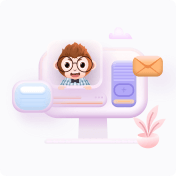
