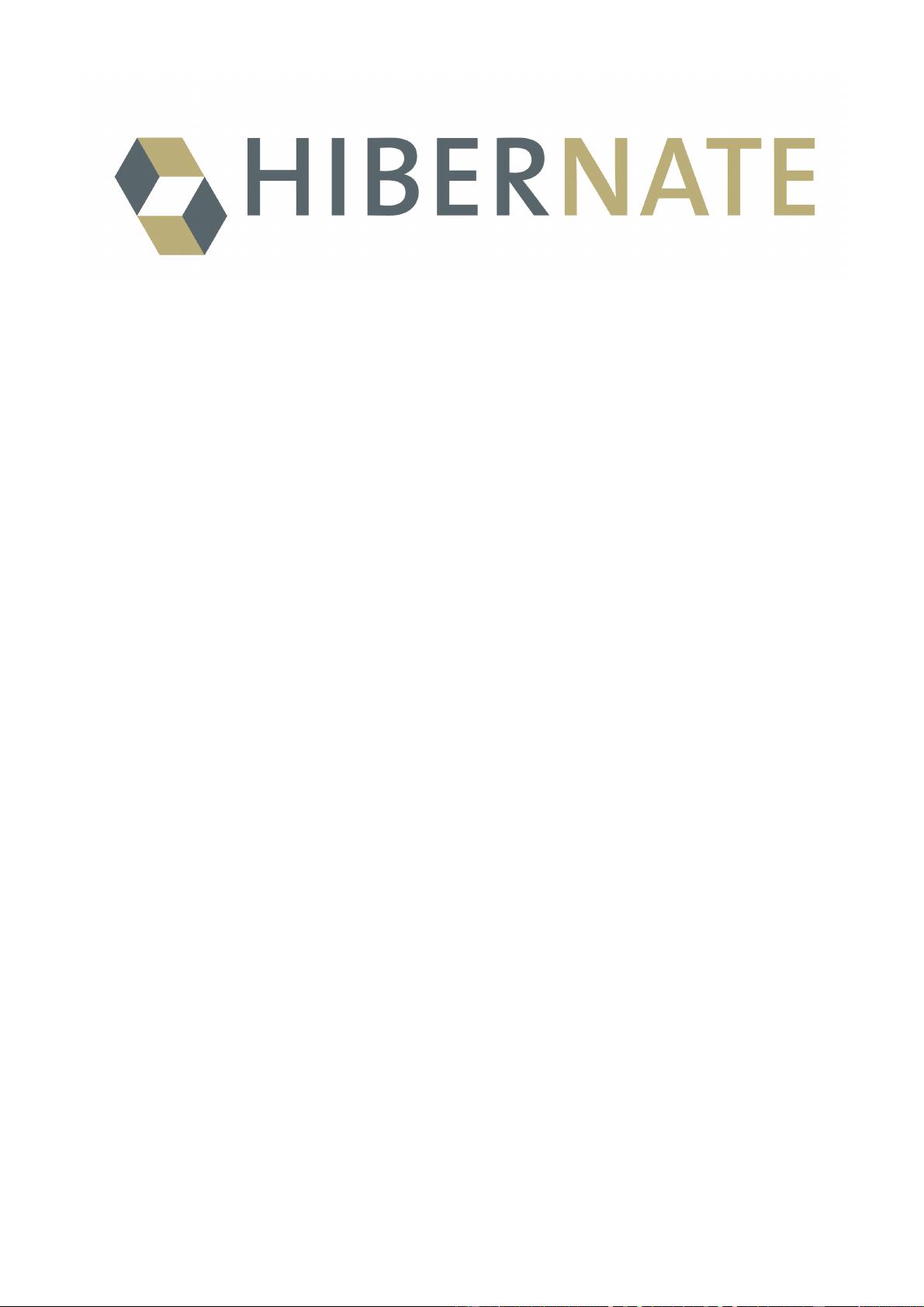
Hibernate EntityManager
User guide
Version: 3.2.0.GA
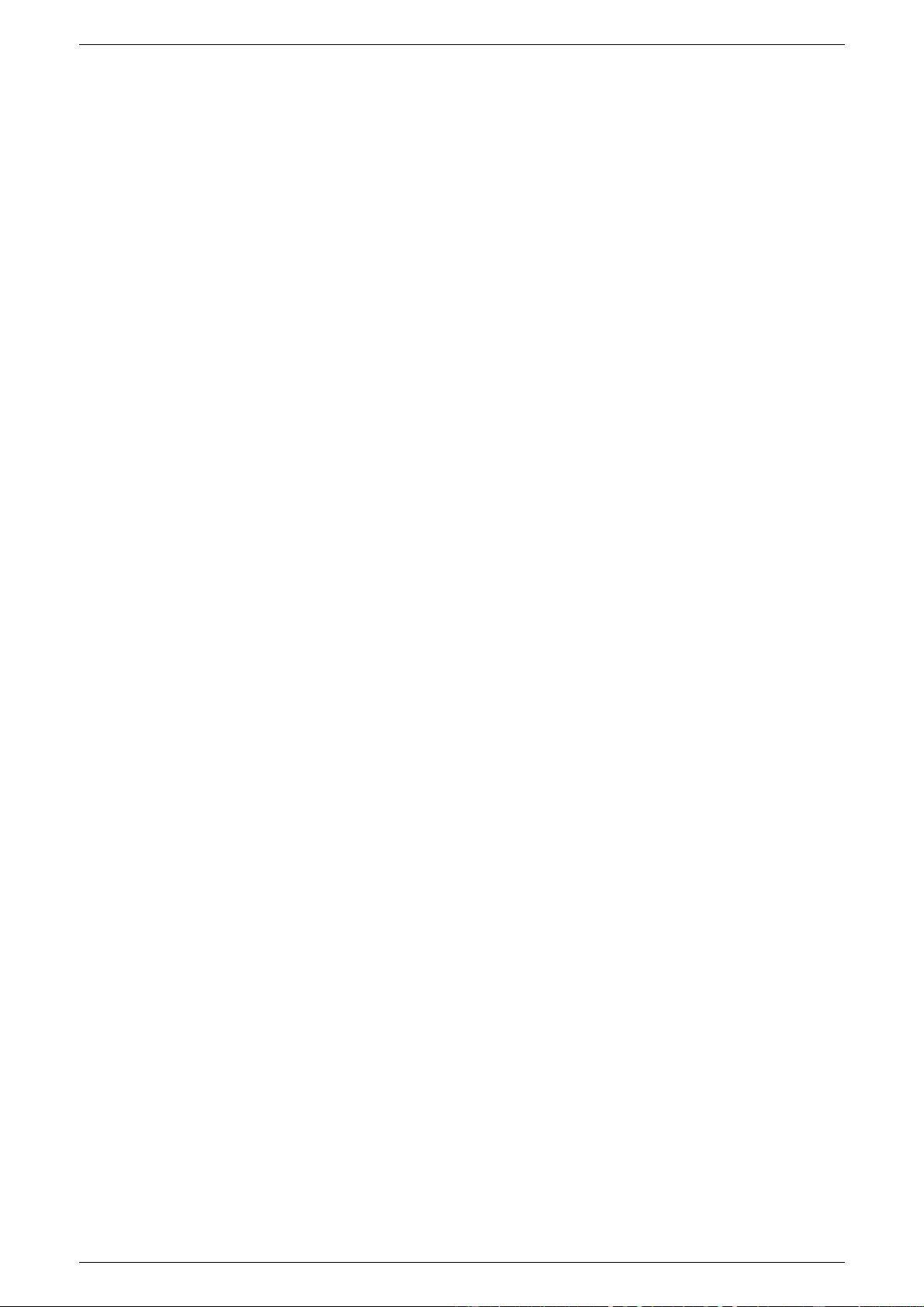
Table of Contents
Introducing EJB3 Persistence ...........................................................................................................iv
1. Architecture ................................................................................................................................1
1.1. Definitions .........................................................................................................................1
1.2. EJB container environment ................................................................................................. 1
1.2.1. Container-managed entity manager ...........................................................................1
1.2.2. Application-managed entity manager ........................................................................2
1.2.3. Persistence context scope ......................................................................................... 2
1.2.4. Persistence context propagation ................................................................................2
1.3. Java SE environments ......................................................................................................... 3
2. Setup and configuration .............................................................................................................. 4
2.1. Setup ................................................................................................................................. 4
2.2. Configuration and bootstrapping .........................................................................................4
2.2.1. Packaging ...............................................................................................................4
2.2.2. Bootstrapping .......................................................................................................... 5
2.3. Event listeners ...................................................................................................................8
2.4. Obtaining an EntityManager in a Java SE environment ......................................................... 9
2.5. Various .............................................................................................................................. 9
3. Working with objects ................................................................................................................ 10
3.1. Entity states ..................................................................................................................... 10
3.2. Making objects persistent ................................................................................................. 10
3.3. Loading an object ............................................................................................................. 10
3.4. Querying objects .............................................................................................................. 11
3.4.1. Executing queries .................................................................................................. 11
3.4.1.1. Projection ................................................................................................... 11
3.4.1.2. Scalar results .............................................................................................. 12
3.4.1.3. Bind parameters .......................................................................................... 12
3.4.1.4. Pagination .................................................................................................. 12
3.4.1.5. Externalizing named queries ........................................................................ 13
3.4.1.6. Native queries ............................................................................................ 13
3.4.1.7. Query hints ................................................................................................ 13
3.5. Modifying persistent objects ............................................................................................. 14
3.6. Modifying detached objects .............................................................................................. 14
3.7. Automatic state detection .................................................................................................. 15
3.8. Deleting managed objects ................................................................................................. 16
3.9. Flush the persistence context ............................................................................................. 16
3.9.1. In a transaction ...................................................................................................... 16
3.9.2. Outside a transaction ............................................................................................. 17
3.10. Transitive persistence ..................................................................................................... 17
3.11. Locking ......................................................................................................................... 18
4. Transactions and Concurrency ................................................................................................. 20
4.1. Entity manager and transaction scopes ............................................................................... 20
4.1.1. Unit of work ......................................................................................................... 20
4.1.2. Long units of work ................................................................................................ 21
4.1.3. Considering object identity .................................................................................... 22
4.1.4. Common concurrency control issues ....................................................................... 23
4.2. Database transaction demarcation ...................................................................................... 23
4.2.1. Non-managed environment .................................................................................... 24
4.2.1.1. EntityTransaction ....................................................................................... 24
Hibernate 3.2.0.GA ii
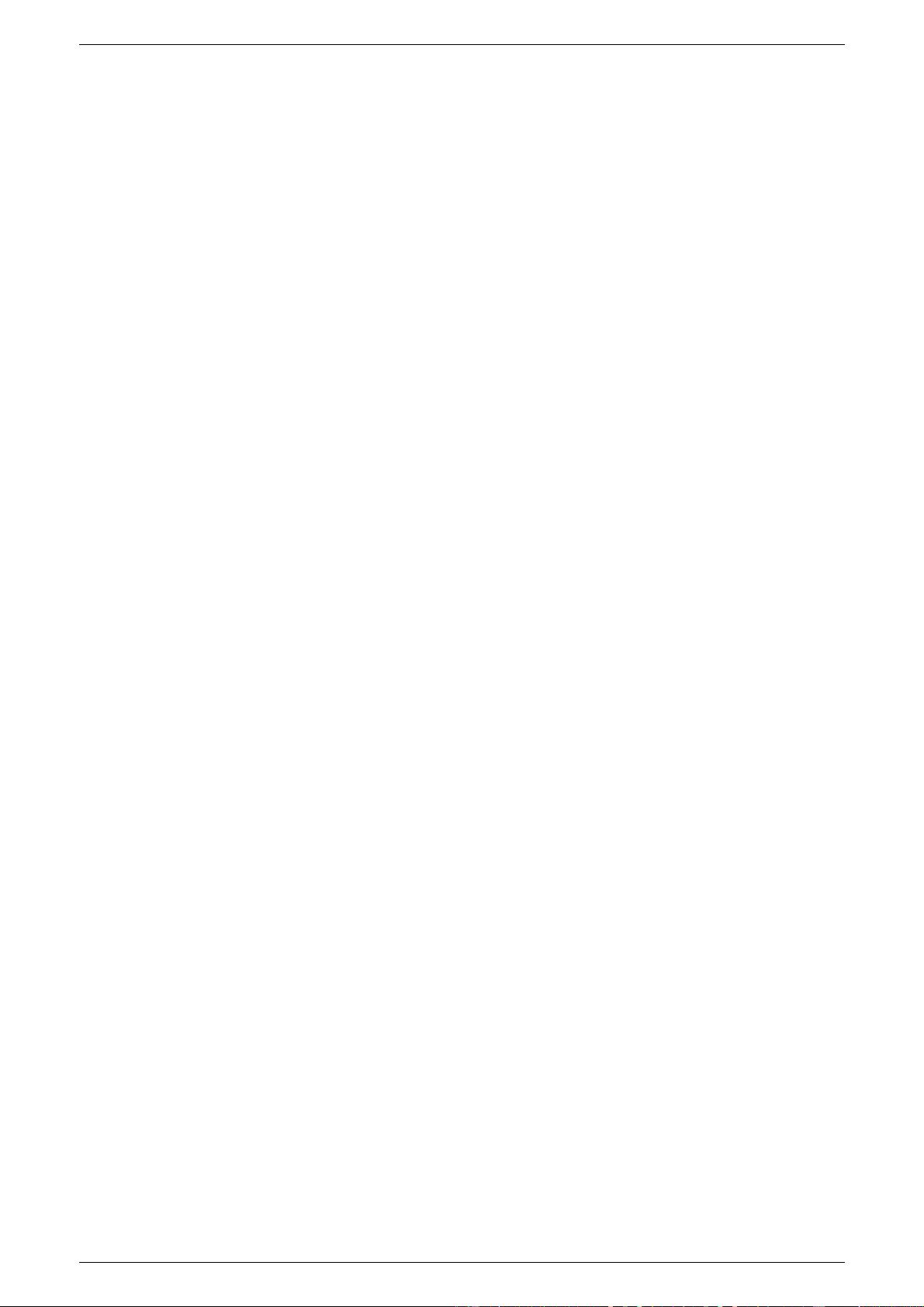
4.2.2. Using JTA ............................................................................................................ 25
4.2.3. Exception handling ................................................................................................ 26
4.3. EXTENDED Persistence Context ...................................................................................... 26
4.3.1. Container Managed Entity Manager ........................................................................ 27
4.3.2. Application Managed Entity Manager ..................................................................... 27
4.4. Optimistic concurrency control ......................................................................................... 27
4.4.1. Application version checking ................................................................................. 28
4.4.2. Extended entity manager and automatic versioning .................................................. 28
4.4.3. Detached objects and automatic versioning ............................................................. 29
5. Entity listeners and Callback methods ...................................................................................... 30
5.1. Definition ........................................................................................................................ 30
5.2. Callbacks and listeners inheritance .................................................................................... 31
5.3. XML definition ................................................................................................................ 32
6. Batch processing ....................................................................................................................... 33
6.1. Bulk update/delete ............................................................................................................ 33
7. EJB-QL: The Object Query Language ...................................................................................... 35
7.1. Case Sensitivity ............................................................................................................... 35
7.2. The from clause ............................................................................................................... 35
7.3. Associations and joins ...................................................................................................... 35
7.4. The select clause .............................................................................................................. 36
7.5. Aggregate functions ......................................................................................................... 37
7.6. Polymorphic queries ......................................................................................................... 38
7.7. The where clause .............................................................................................................. 38
7.8. Expressions ..................................................................................................................... 40
7.9. The order by clause .......................................................................................................... 42
7.10. The group by clause ....................................................................................................... 42
7.11. Subqueries ..................................................................................................................... 43
7.12. EJB-QL examples .......................................................................................................... 44
7.13. Bulk UPDATE & DELETE Statements ........................................................................... 45
7.14. Tips & Tricks ................................................................................................................. 45
8. Native query .............................................................................................................................. 47
8.1. Expressing the resultset .................................................................................................... 47
8.2. Using native SQL Queries ................................................................................................ 47
8.3. Named queries ................................................................................................................. 48
Hibernate EntityManager
Hibernate 3.2.0.GA iii
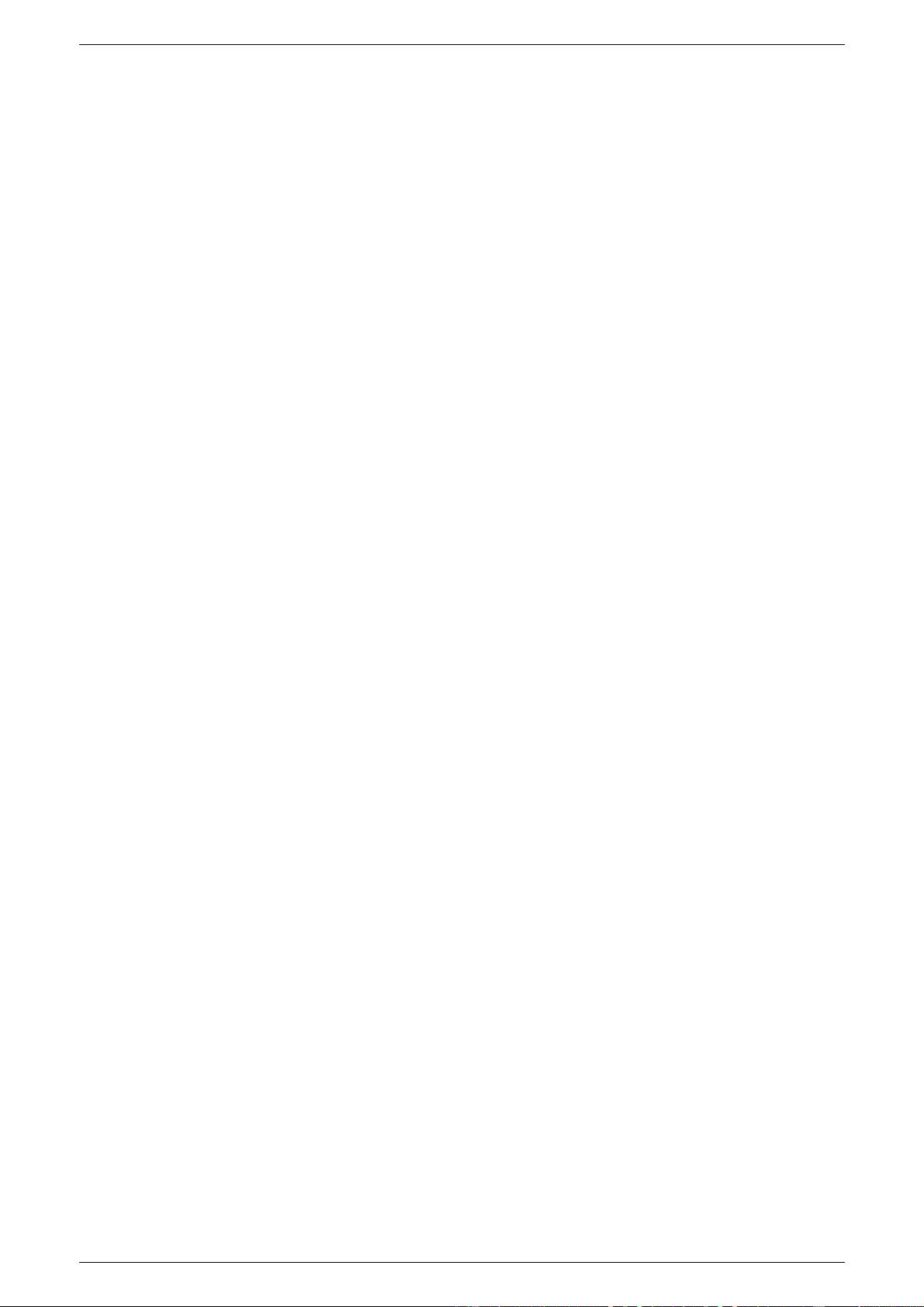
Introducing EJB3 Persistence
The EJB3 specification recognizes the interest and the success of the transparent object/relational mapping
paradigm. The EJB3 specification standardizes the basic APIs and the metadata needed for any object/relational
persistence mechanism. Hibernate EntityManager implements the programming interfaces and lifecycle rules
as defined by the EJB3 persistence specification. Together with Hibernate Annotations, this wrapper imple-
ments a complete (and standalone) EJB3 persistence solution on top of the mature Hibernate core. You may use
a combination of all three together, annotations without EJB3 programming interfaces and lifecycle, or even
pure native Hibernate, depending on the business and technical needs of your project. You can at all times fall
back to Hibernate native APIs, or if required, even to native JDBC and SQL.
Hibernate 3.2.0.GA iv
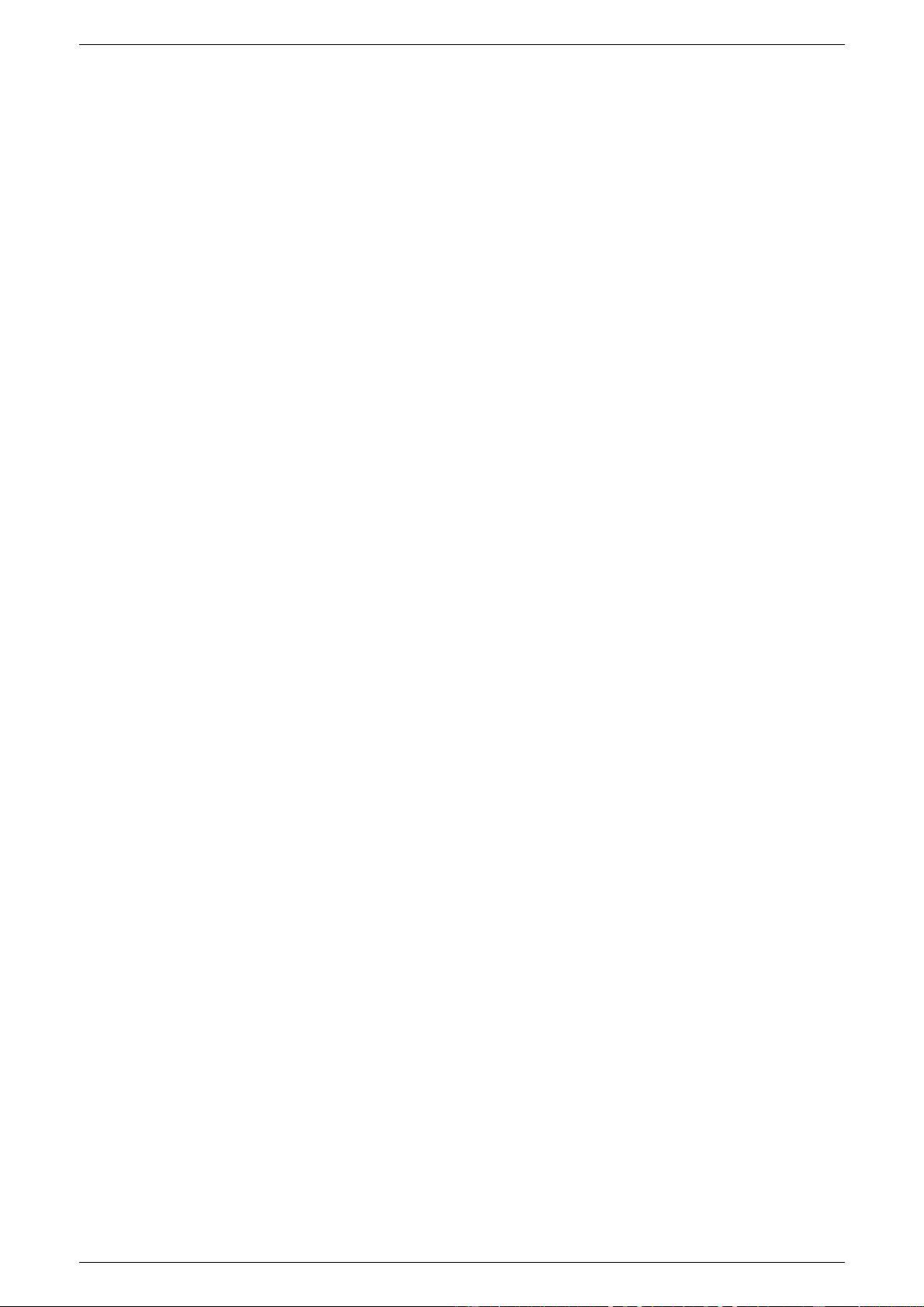
Chapter 1. Architecture
1.1. Definitions
EJB3 is part of the Java EE 5.0 platform. Persistence in EJB3 is available in EJB3 containers, as well as for
standalone J2SE applications that execute outside of a particular container. The following programming inter-
faces and artifacts are available in both environments.
EntityManagerFactory
An entity manager factory provides entity manager instances, all instances are configured to connect to the
same database, to use the same default settings as defined by the particular implementation, etc. You can
prepare several entity manager factories to access several data stores. This interface is similar to the Ses-
sionFactory in native Hibernate.
EntityManager
The EntityManager API is used to access a database in a particular unit of work. It is used to create and re-
move persistent entity instances, to find entities by their primary key identity, and to query over all entities.
This interface is similar to the Session in Hibernate.
Persistence context
A persistence context is a set of entity instances in which for any persistent entity identity there is a unique
entity instance. Within the persistence context, the entity instances and their lifecycle is managed by a par-
ticular entity manager. The scope of this context can either be the transaction, or an extended unit of work.
Persistence unit
The set of entity types that can be managed by a given entity manager is defined by a persistence unit. A
persistence unit defines the set of all classes that are related or grouped by the application, and which must
be collocated in their mapping to a single data store.
Container-managed entity manager
An Entity Manager whose lifecycle is managed by the container
Application-managed entity manager
An Entity Manager whose lifecycle is managed by the application.
JTA entity manager
Entity manager involved in a JTA transaction
Resource-local entity manager
Entity manager using a resource transaction (not a JTA transaction).
1.2. EJB container environment
1.2.1. Container-managed entity manager
The most common and widely used entity manager in a Java EE environment is the container-managed entity
manager. In this mode, the container is responsible for the opening and closing of the entity manager (this is
transparent to the application). It is also responsible for transaction boundaries. A container-managed entity
manager is obtained in an application through dependency injection or through JNDI lookup, A container-man-
Hibernate 3.2.0.GA 1