/**
* @Title: MyBatisPaginationPlugin.java
* @Package
* @Description postgresql 数据库表生成XML的插件类
* @author
* @date
* @version V1.0
*/
package com.tinet.MyBatisGeneratorTool;
import java.util.List;
import org.mybatis.generator.api.CommentGenerator;
import org.mybatis.generator.api.IntrospectedTable;
import org.mybatis.generator.api.PluginAdapter;
import org.mybatis.generator.api.dom.java.Field;
import org.mybatis.generator.api.dom.java.FullyQualifiedJavaType;
import org.mybatis.generator.api.dom.java.JavaVisibility;
import org.mybatis.generator.api.dom.java.Method;
import org.mybatis.generator.api.dom.java.Parameter;
import org.mybatis.generator.api.dom.java.TopLevelClass;
import org.mybatis.generator.api.dom.xml.Attribute;
import org.mybatis.generator.api.dom.xml.Document;
import org.mybatis.generator.api.dom.xml.TextElement;
import org.mybatis.generator.api.dom.xml.XmlElement;
public class MyBatisPaginationPlugin extends PluginAdapter {
@Override
public boolean modelExampleClassGenerated(TopLevelClass topLevelClass,
IntrospectedTable introspectedTable) {
// add field, getter, setter for limit clause
addPage(topLevelClass, introspectedTable, "page");
return super.modelExampleClassGenerated(topLevelClass,
introspectedTable);
}
@Override
public boolean sqlMapDocumentGenerated(Document document,
IntrospectedTable introspectedTable) {
XmlElement parentElement = document.getRootElement();
// 产生分页语句
XmlElement paginationPrefixElement = new XmlElement("sql");
paginationPrefixElement.addAttribute(new Attribute("id", "PostgresqlPaging"));
XmlElement pageStart = new XmlElement("if");
pageStart.addAttribute(new Attribute("test", "page != null"));
pageStart.addElement(new TextElement( "limit #{page.size} offset #{page.start}"));
paginationPrefixElement.addElement(pageStart);
parentElement.addElement(paginationPrefixElement);
// 产生getCount语句
// XmlElement getCountPrefixElement = new XmlElement("select");
// getCountPrefixElement.addAttribute(new Attribute("id", "getCountBy"));
// getCountPrefixElement.addAttribute(new Attribute("parameterType", "java.util.Map"));
// getCountPrefixElement.addAttribute(new Attribute("resultType", "INTEGER"));
// XmlElement getCountStart = new XmlElement("if");
// getCountStart.addAttribute(new Attribute("test", "page != null"));
// getCountStart.addElement(new TextElement( "limit #{page.size}, #{page.start}"));
// getCountPrefixElement.addElement(pageStart);
// parentElement.addElement(getCountPrefixElement);
//
//
// <select id="getCountBy" parameterType="java.util.Map" resultType="INTEGER">
// SELECT
// COUNT(*) AS C
// FROM
// <include refid="tableNameSql" />
// p
// <include refid="varSql" />
// </select>
// 添加缓存
//XmlElement cacheElement = new XmlElement("cache");
//parentElement.addElement(cacheElement);
return super.sqlMapDocumentGenerated(document, introspectedTable);
}
@Override
public boolean sqlMapSelectByExampleWithoutBLOBsElementGenerated(
XmlElement element, IntrospectedTable introspectedTable) {
XmlElement isNotNullElement = new XmlElement("include"); //$NON-NLS-1$
isNotNullElement.addAttribute(new Attribute("refid",
"PostgresqlPaging"));
element.getElements().add(isNotNullElement);
return super.sqlMapUpdateByExampleWithoutBLOBsElementGenerated(element,
introspectedTable);
}
/**
* @param topLevelClass
* @param introspectedTable
* @param name
*/
private void addPage(TopLevelClass topLevelClass,
IntrospectedTable introspectedTable, String name) {
topLevelClass.addImportedType(new FullyQualifiedJavaType(
"core.entity.Page"));
CommentGenerator commentGenerator = context.getCommentGenerator();
Field field = new Field();
field.setVisibility(JavaVisibility.PROTECTED);
field.setType(new FullyQualifiedJavaType("core.entity.Page"));
field.setName(name);
commentGenerator.addFieldComment(field, introspectedTable);
topLevelClass.addField(field);
char c = name.charAt(0);
String camel = Character.toUpperCase(c) + name.substring(1);
Method method = new Method();
method.setVisibility(JavaVisibility.PUBLIC);
method.setName("set" + camel);
method.addParameter(new Parameter(new FullyQualifiedJavaType(
"core.entity.Page"), name));
method.addBodyLine("this." + name + "=" + name + ";");
commentGenerator.addGeneralMethodComment(method, introspectedTable);
topLevelClass.addMethod(method);
method = new Method();
method.setVisibility(JavaVisibility.PUBLIC);
method.setReturnType(new FullyQualifiedJavaType(
"core.entity.Page"));
method.setName("get" + camel);
method.addBodyLine("return " + name + ";");
commentGenerator.addGeneralMethodComment(method, introspectedTable);
topLevelClass.addMethod(method);
}
/**
* This plugin is always valid - no properties are required
*/
public boolean validate(List<String> warnings) {
return true;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
mybatis使用虽然灵活简单,但mapper.xml的配置却很繁琐。如果项目的实体表比较多,手工配置是不现实的。这个工具可以帮助自动后成model,dao,mapper.xml 使开发者从繁琐的mapper.xml映射中解放出来,把更多的精力投入到项目的业务层中去。首先配置好generatorConfig_zongfenji.xml,之后运行MyBatisGeneratorTool. 这是一个完整的带源码的小工具,maven方式管理。所需jar包在pom.xml中有配置。联网更新依赖会自动下载。
资源推荐
资源详情
资源评论
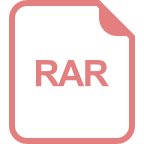
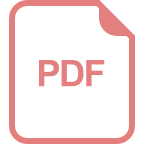
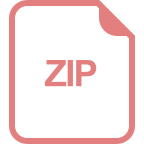
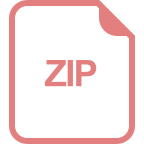
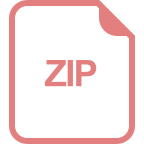
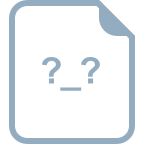
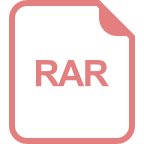
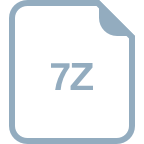
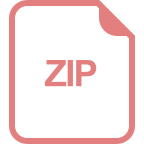
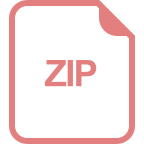
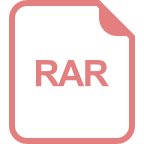
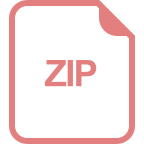
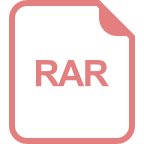
收起资源包目录

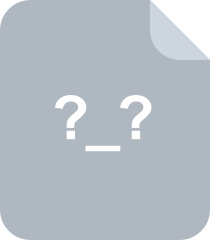
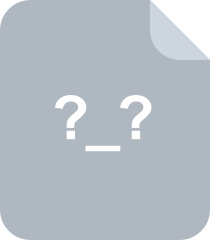
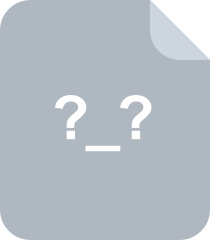
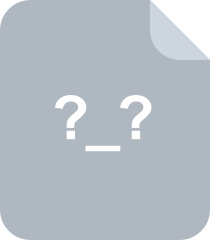
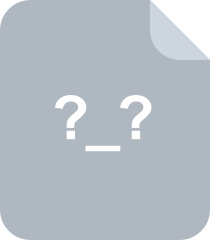
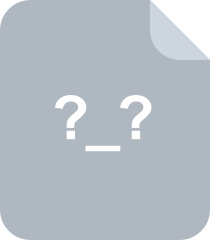
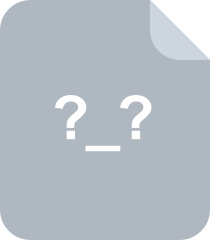
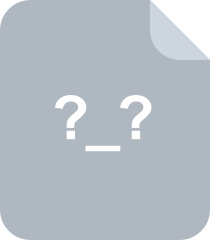
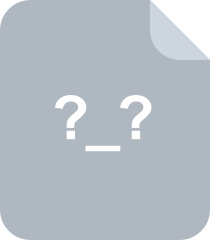
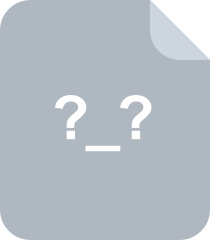
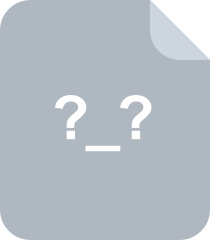
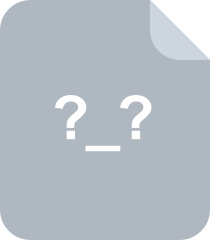
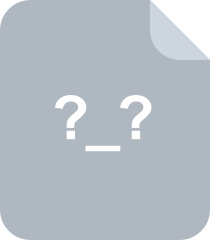
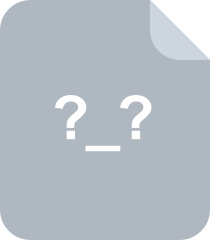
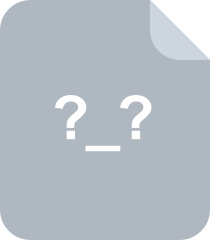
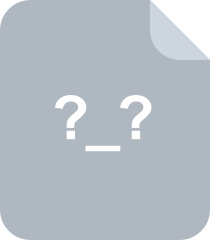
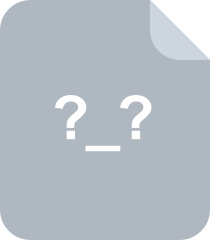
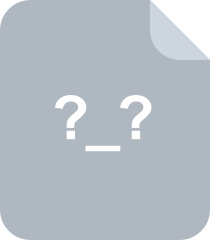
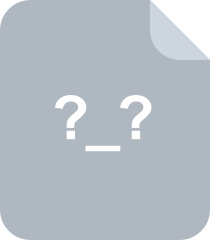
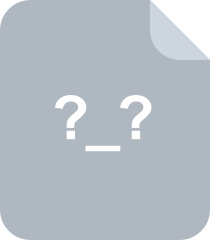
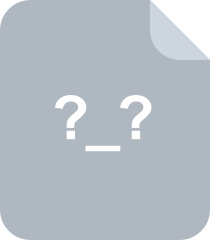
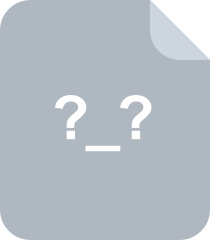
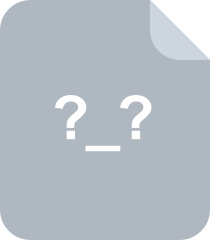
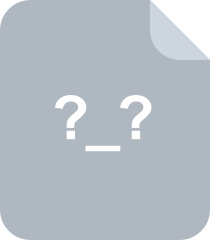
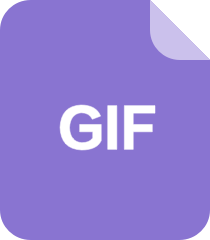
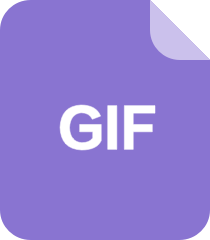
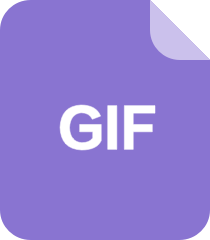
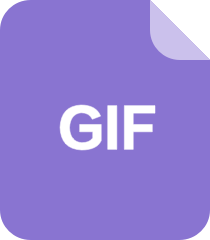
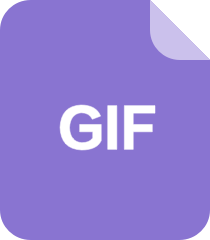
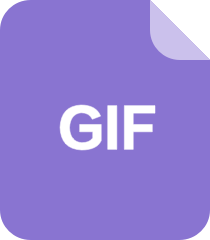
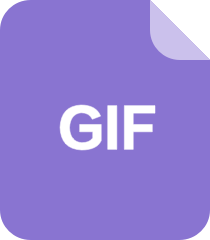
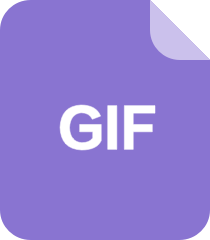
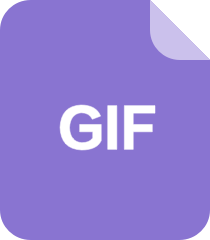
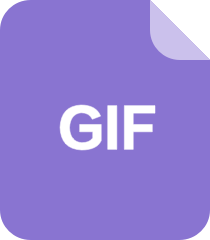
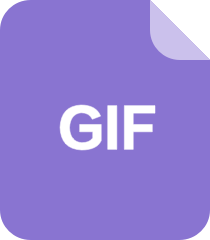
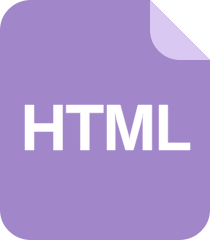
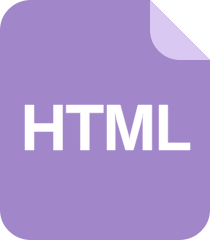
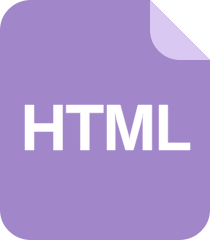
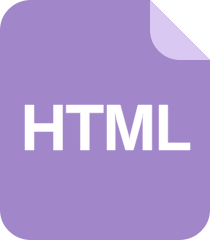
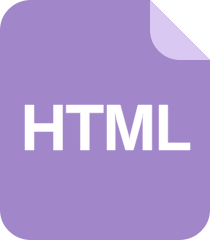
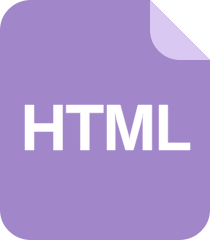
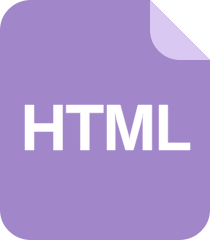
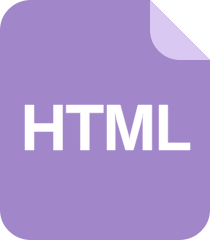
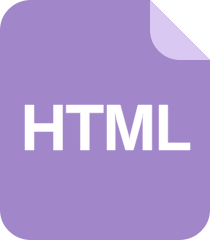
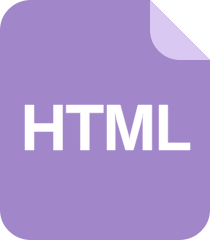
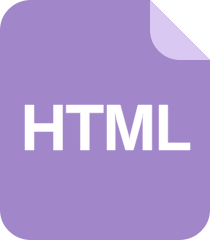
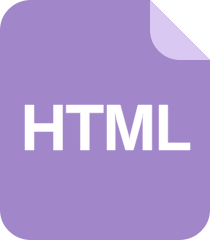
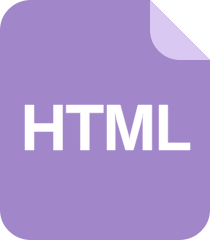
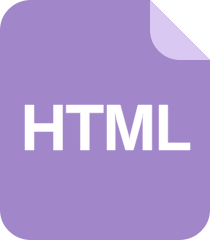
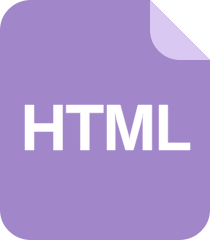
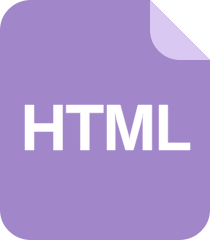
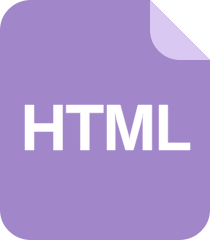
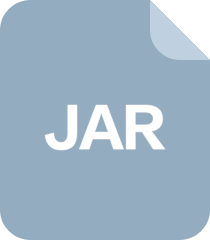
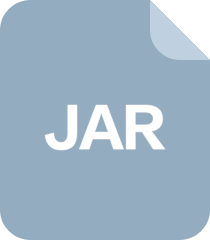
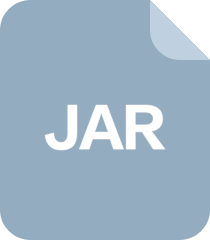
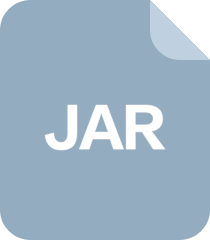
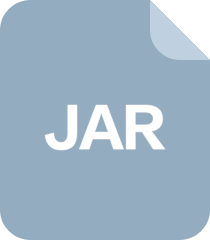
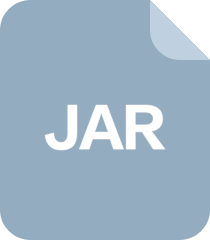
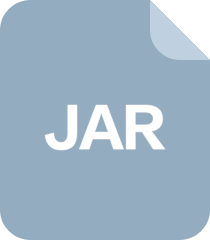
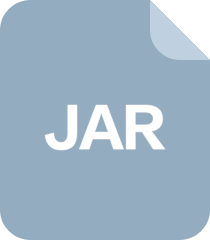
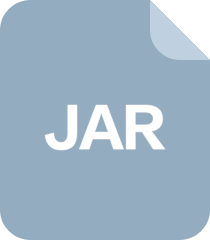
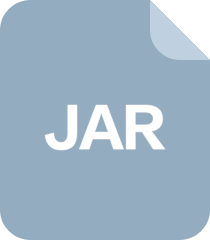
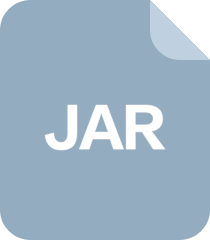
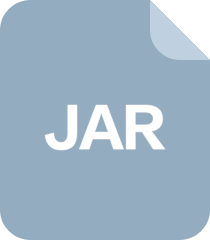
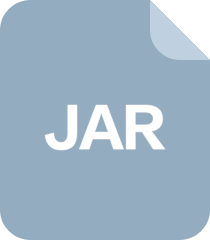
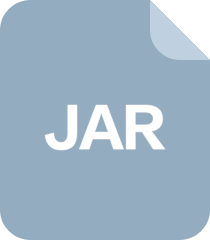
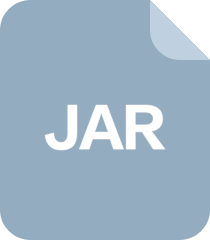
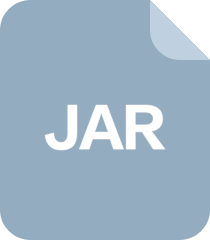
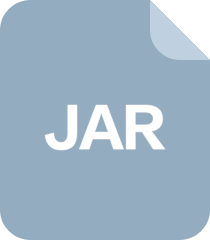
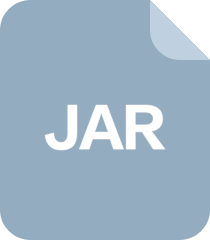
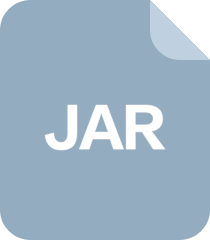
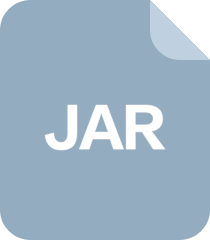
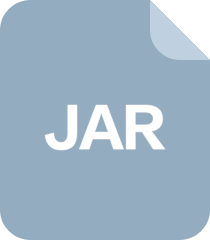
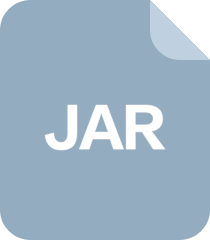
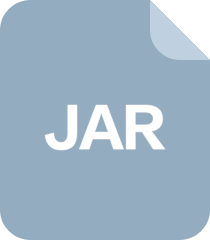
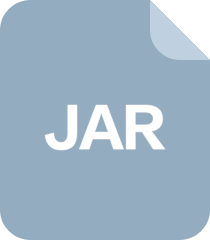
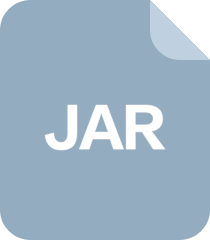
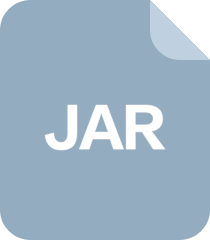
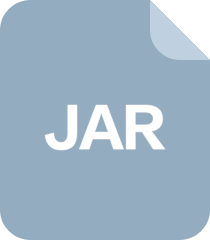
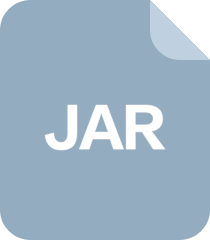
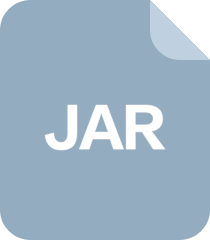
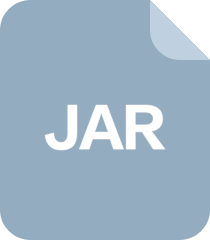
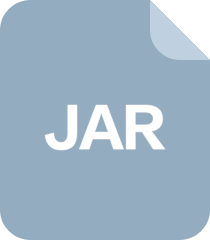
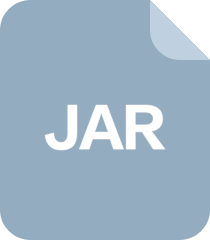
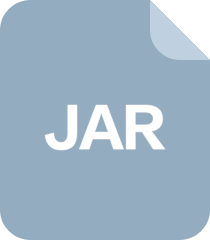
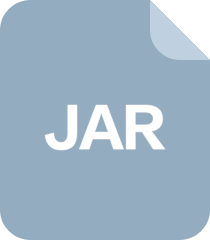
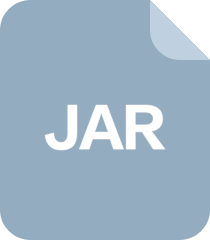
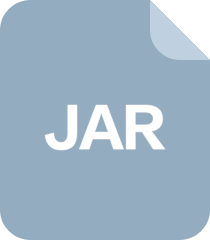
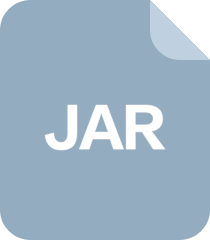
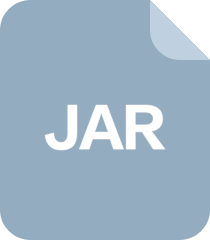
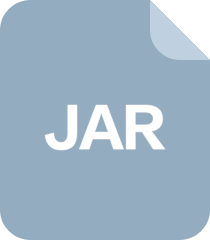
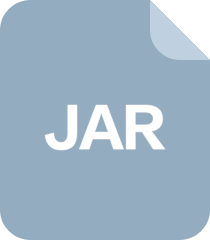
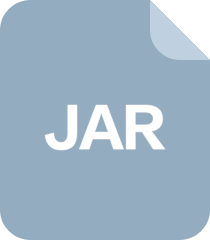
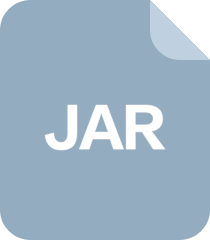
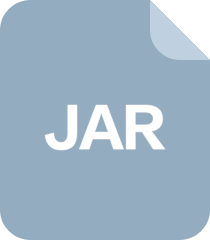
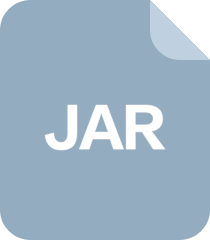
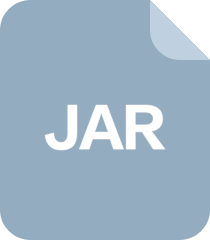
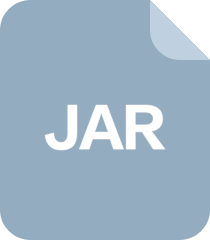
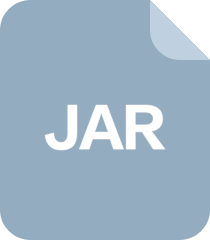
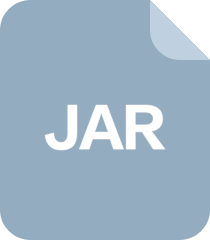
共 375 条
- 1
- 2
- 3
- 4
资源评论
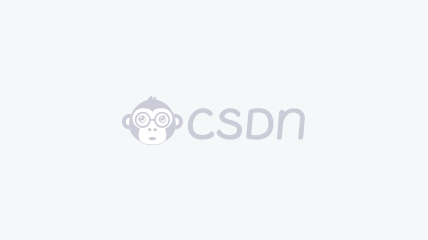
- lmrdabendan12015-11-10非常好 非常棒 解决了我常年困扰的难题
- jollon2016-11-04资源很不错,值得学习

guiyu_2008
- 粉丝: 0
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

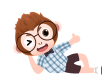
最新资源
- 两相步进电机FOC矢量控制Simulink仿真模型 1.采用针对两相步进电机的SVPWM控制算法,实现FOC矢量控制,DQ轴解耦控制~ 2.转速电流双闭环控制,电流环采用PI控制,转速环分别采用PI和
- VMware虚拟机USB驱动
- Halcon手眼标定简介(1)
- (175128050)c&c++课程设计-图书管理系统
- 视频美学多任务学习中PyTorch的多回归实现-含代码及解释
- 基于ssh员工管理系统
- 5G SRM815模组原理框图.jpg
- T型3电平逆变器,lcl滤波器滤波器参数计算,半导体损耗计算,逆变电感参数设计损耗计算 mathcad格式输出,方便修改 同时支持plecs损耗仿真,基于plecs的闭环仿真,电压外环,电流内环
- 毒舌(解锁版).apk
- 显示HEX、S19、Bin、VBF等其他汽车制造商特定的文件格式
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


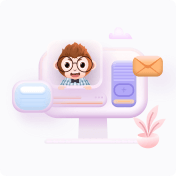
安全验证
文档复制为VIP权益,开通VIP直接复制
