// CodeGear C++Builder
// Copyright (c) 1995, 2015 by Embarcadero Technologies, Inc.
// All rights reserved
// (DO NOT EDIT: machine generated header) 'IKTypes.pas' rev: 29.00 (Windows)
#ifndef IktypesHPP
#define IktypesHPP
#pragma delphiheader begin
#pragma option push
#pragma option -w- // All warnings off
#pragma option -Vx // Zero-length empty class member
#pragma pack(push,8)
#include <System.hpp>
#include <SysInit.hpp>
#include <Winapi.Windows.hpp>
#include <Winapi.MMSystem.hpp>
#include <System.Classes.hpp>
#include <System.Math.hpp>
#include <System.TypInfo.hpp>
#include <System.SysUtils.hpp>
#include <Vcl.Graphics.hpp>
#include <Vcl.Imaging.pngimage.hpp>
#include <Winapi.DXGI.hpp>
#include <Winapi.D3D10.hpp>
#include <Winapi.D3D10_1.hpp>
#include <Winapi.DxgiFormat.hpp>
#include <Vcl.Themes.hpp>
#include <System.Rtti.hpp>
#include <System.UITypes.hpp>
#include <System.Types.hpp>
//-- user supplied -----------------------------------------------------------
namespace Iktypes
{
//-- forward type declarations -----------------------------------------------
struct TIKColor24;
struct TIKColor;
struct TIKColorF;
struct TIKPoint;
struct TIKPoint3D;
struct TIKVector;
struct TIKVector3D;
struct TIKMatrix;
struct TIKMatrix3D;
struct TIKSize;
struct TIKRect;
struct TIKVertex;
class DELPHICLASS TIKRenderWindow;
class DELPHICLASS TIKTexture;
class DELPHICLASS TIKMapTexture;
class DELPHICLASS TIKRenderTexture;
class DELPHICLASS TIKProgram;
class DELPHICLASS TIKDictionary;
class DELPHICLASS TIKObjectDictionary;
struct TIKValue;
class DELPHICLASS TIKPersistent;
struct TKernel;
//-- type declarations -------------------------------------------------------
enum DECLSPEC_DENUM TIKPixelFormat : unsigned int { pfNone, pfRGB, pfRGBX, pfRGBA, pfBGR, pfBGRX, pfBGRA };
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKColor24
{
public:
System::Byte B;
System::Byte G;
System::Byte R;
};
#pragma pack(pop)
typedef TIKColor24 *PIKColor24;
typedef System::StaticArray<TIKColor24, 536870911> TIKColor24Array;
typedef TIKColor24Array *PIKColor24Array;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKColor
{
union
{
struct
{
System::Byte B;
System::Byte G;
System::Byte R;
System::Byte A;
};
struct
{
System::Word HiWord;
System::Word LoWord;
};
struct
{
unsigned Color;
};
};
};
#pragma pack(pop)
typedef TIKColor *PIKColor;
typedef System::StaticArray<TIKColor, 536870911> TIKColorArray;
typedef TIKColorArray *PIKColorArray;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKColorF
{
public:
System::Uitypes::TColor __fastcall ToColor(void);
union
{
struct
{
float X;
float Y;
float Z;
float W;
};
struct
{
float R;
float G;
float B;
float A;
};
};
};
#pragma pack(pop)
typedef System::StaticArray<float, 2> TIKPointArray;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKPoint
{
union
{
struct
{
float X;
float Y;
};
struct
{
TIKPointArray V;
};
};
};
#pragma pack(pop)
typedef System::StaticArray<float, 3> TIKPoint3DArray;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKPoint3D
{
union
{
struct
{
float X;
float Y;
float Z;
};
struct
{
TIKPoint3DArray V;
};
};
};
#pragma pack(pop)
typedef System::StaticArray<float, 3> TIKVectorArray;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKVector
{
union
{
struct
{
float X;
float Y;
float W;
};
struct
{
TIKVectorArray V;
};
};
};
#pragma pack(pop)
typedef System::StaticArray<float, 4> TIKVector3DArray;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKVector3D
{
union
{
struct
{
TIKPoint3D P;
float PW;
};
struct
{
float X;
float Y;
float Z;
float W;
};
struct
{
TIKVector3DArray V;
};
};
};
#pragma pack(pop)
typedef System::StaticArray<TIKVector, 3> TIKMatrixArray;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKMatrix
{
union
{
struct
{
float m11;
float m12;
float m13;
float m21;
float m22;
float m23;
float m31;
float m32;
float m33;
};
struct
{
TIKMatrixArray M;
};
};
};
#pragma pack(pop)
typedef System::StaticArray<TIKVector3D, 4> TIKMatrix3DArray;
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKMatrix3D
{
union
{
struct
{
float m11;
float m12;
float m13;
float m14;
float m21;
float m22;
float m23;
float m24;
float m31;
float m32;
float m33;
float m34;
float m41;
float m42;
float m43;
float m44;
};
struct
{
TIKMatrix3DArray M;
};
};
};
#pragma pack(pop)
#pragma pack(push,1)
struct DECLSPEC_DRECORD TIKSize
{
public:
float Width;
float Height;
};
#pragma pack(pop)
struct DECLSPEC_DRECORD TIKRect
{
union
{
struct
{
TIKPoint Pos;
TIKSize Size;
};
struct
{
float X;
float Y;
float Width;
float Height;
};
};
};
struct DECLSPEC_DRECORD TIKVertex
{
public:
TIKPoint Pos;
TIKPoint UV;
};
typedef System::DynamicArray<TIKVertex> TIKVertexArray;
typedef System::StaticArray<TIKVertex, 134217727> TIKVertexStaticArray;
typedef TIKVertexStaticArray *PIKVertexArray;
typedef System::DynamicArray<System::Word> TIKIndexArray;
typedef System::StaticArray<System::Word, 1073741823> TKCStaticIndexArray;
typedef TKCStaticIndexArray *PIKIndexArray;
enum DECLSPEC_DENUM TIKCullMode : unsigned int { cmNone, cmFront, cmBack };
enum DECLSPEC_DENUM TIKBlendMode : unsigned int { bmDisable, bmNormal, bmAdditive };
enum DECLSPEC_DENUM TIKDepthTest : unsigned int { dtEnabled, dtDisabled };
enum DECLSPEC_DENUM TIKRenderWindowAttr : unsigned int { waMultisampling, waDepthStencil };
typedef System::Set<TIKRenderWindowAttr, TIKRenderWindowAttr::waMultisampling, TIKRenderWindowAttr::waDepthStencil> TIKRenderWindowAttrs;
#pragma pack(push,4)
class PASCALIMPLEMENTATION TIKRenderWindow : public System::TObject
{
typedef System::TObject inherited;
private:
TIKRenderWindowAttrs FAttrs;
int FWidth;
int FHeight;
protected:
virtual void __fastcall CreateResource(void);
virtual void __fastcall DestroyResource(void);
__property TIKRenderWindowAttrs Attrs = {read=FAttrs, nodefault};
__fastcall TIKRenderWindow(const TIKRenderWindowAttrs AAttrs, const int AWidth, const int AHeight);
public:
__fastcall virtual ~TIKRenderWindow(void);
void __fastcall Resize(const int AWidth, const int AHeight);
virtual void __fastcall Present(void);
__property int Width = {read=FWidth, nodefault};
__property int Height = {read=FHeight, nodefault};
};
#pragma pack(pop)
enum DECLSPEC_DENUM TIKTextureAttr : unsigned int { taAntialias, taMultisampling, taTileRepeat, taClampBorder, taDepthStencil };
typedef System::Set<TIKTextureAttr, TIKTextureAttr::taAntialias, TIKTextureAttr::taDepthStencil> TIKTextureAttrs;
#pragma pack(push,4)
class PASCALIMPLEMENTATION TIKTexture : public System::Classes::TPersistent
{
typedef System::Classes::TPersistent inherited;
private:
int FWidth;
int FHeight;
int FBytesPerPixel;
TIKPixelFormat FPixelFormat;
TIKTextureAttrs FAttrs;
System::Types::TRect __fastcall GetExtent(void);
protected:
virtual void __fastcall CreateResource(void);
virtual void __fastcall DestroyResource(void);
__property int BytesPerPixel = {read=FBytesPerPixel, nodefault};
public:
__fastcall TIKTexture(const TIKTextureAttrs AAttrs, const int AWidth, const int AHeight, const TIKPixelFormat APixelFormat);
__fastcall virtual ~TIKTexture(void);
virtual void __fastcall Activate(int Channel = 0x0);
virtual void __fastcall Deac
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
ImageKit是Almediadev的应用程序VCL,适用于Delphi和C++ Builder。VCL或Visual Component Library指的是用于设计Windows应用程序界面的基于图像的组件。ImageKit VCL是一个基于GPU的图像处理和图形渲染框架,可供Delphi语言开发人员使用。该组件的优势在于它隐藏了低级图形处理的细节和困难,并为编程提供了简单的界面。 该组件为您提供数十种预制的过滤器,您可以通过调整其输入参数来根据需要修改和调整每个过滤器。还可以将过滤器链接在一起,使一个过滤器的输出成为另一个输入,从而使您能够组合过滤器并充分利用它们。该组件还具有2D和3D转换器、高级DirectX 10渲染、支持64位体系结构和其他许多功能。
资源推荐
资源详情
资源评论
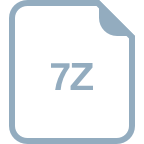
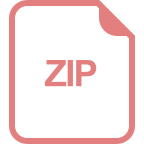
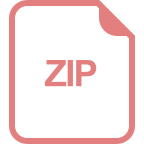
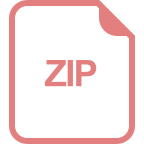
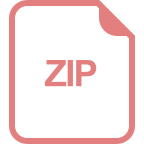
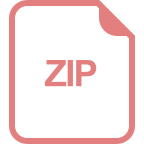
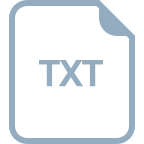
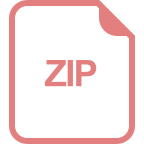
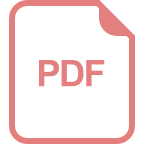
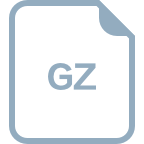
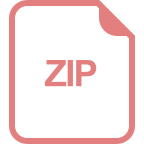
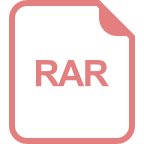
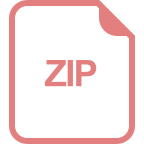
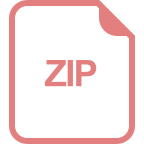
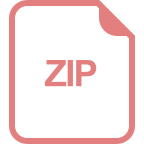
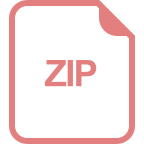
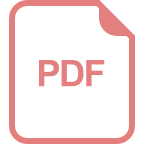
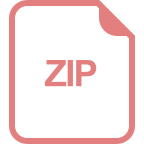
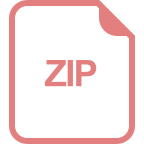
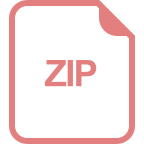
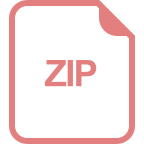
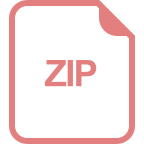
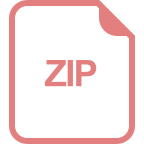
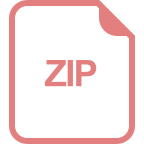
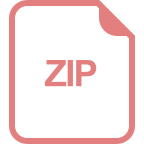
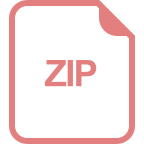
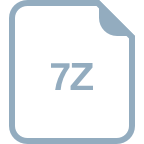
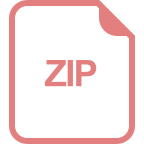
收起资源包目录

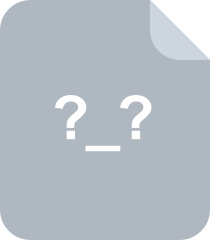
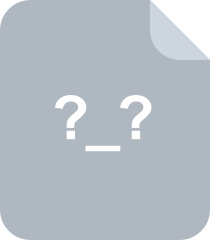
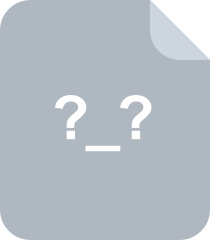
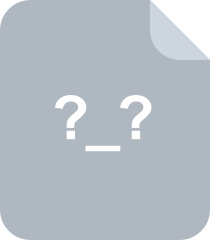
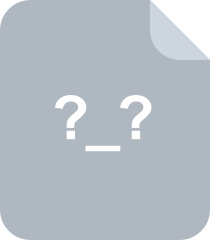
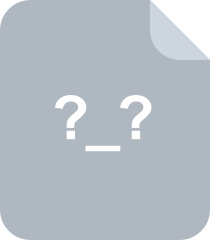
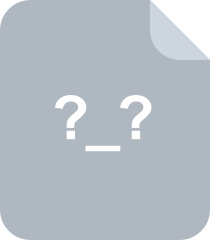
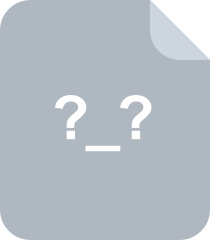
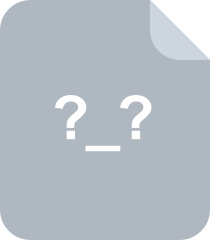
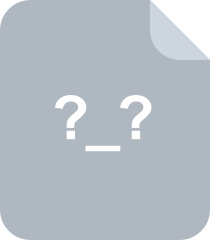
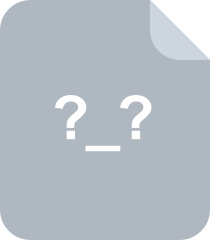
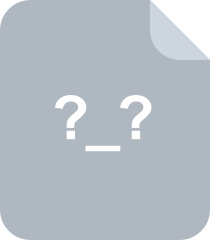
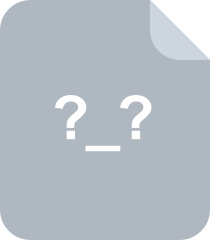
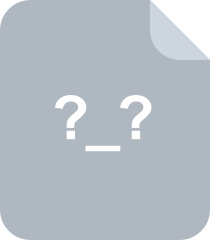
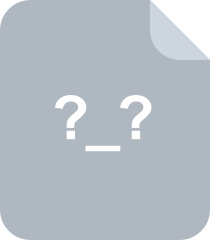
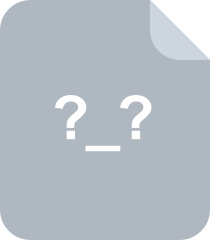
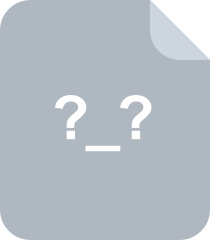
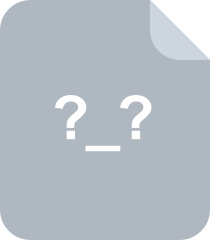
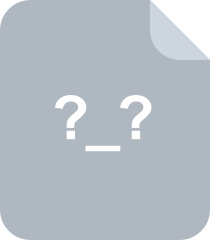
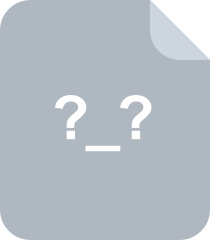
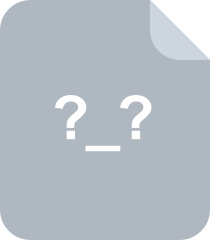
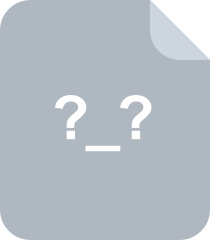
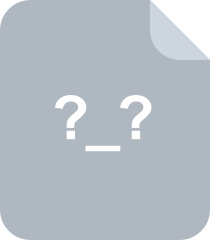
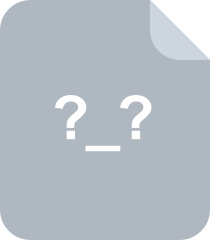
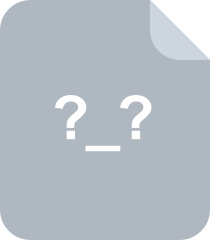
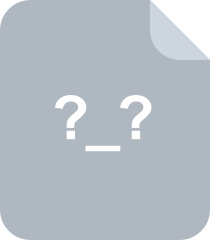
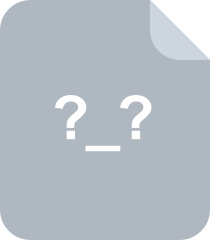
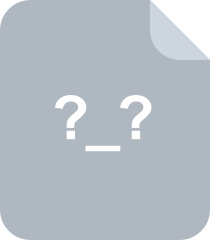
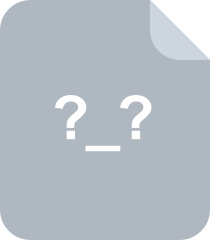
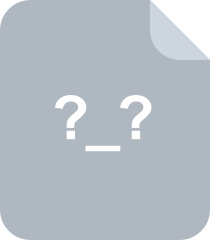
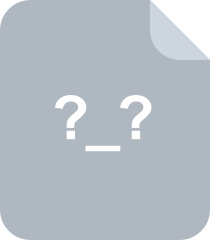
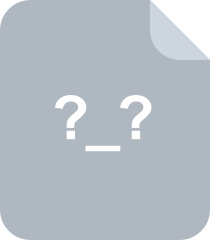
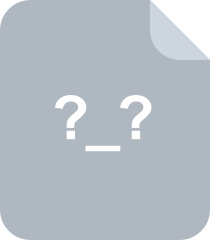
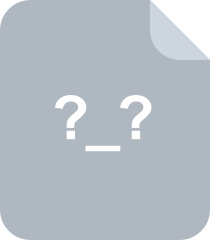
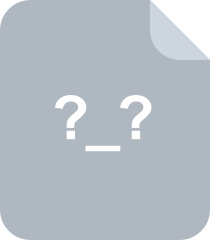
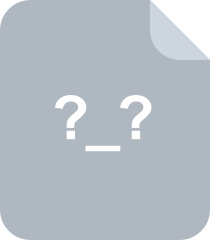
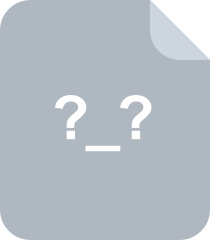
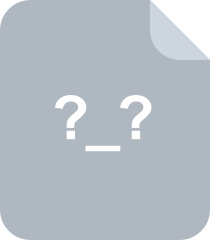
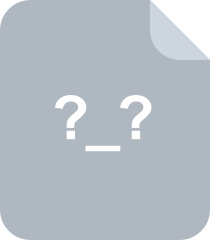
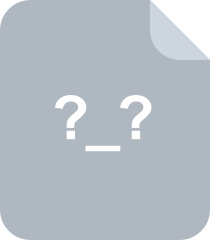
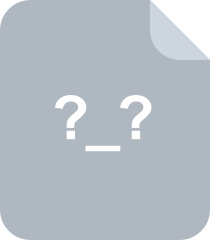
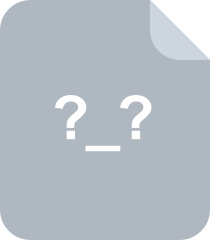
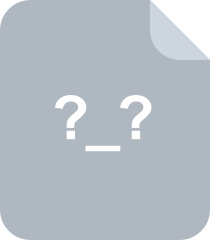
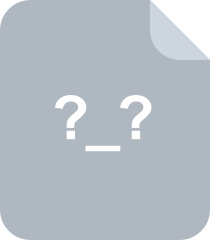
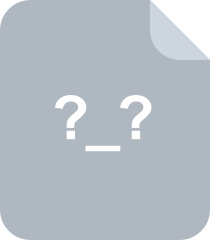
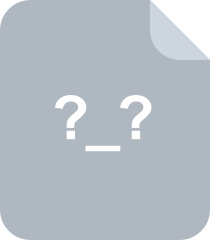
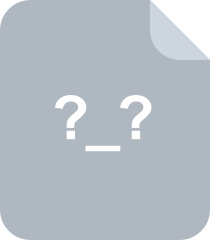
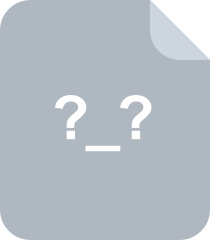
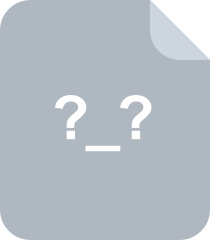
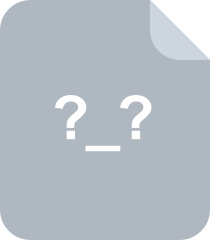
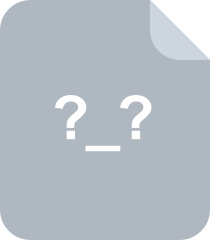
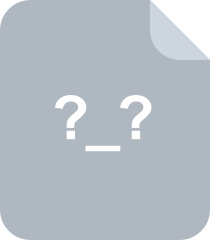
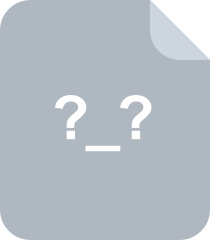
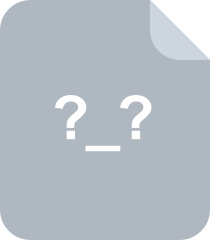
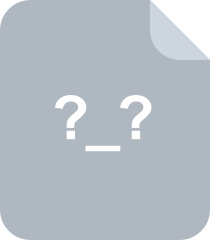
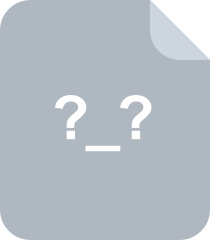
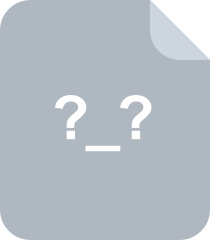
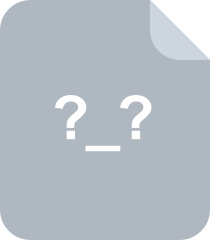
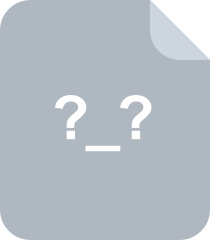
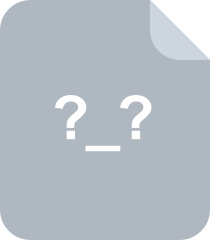
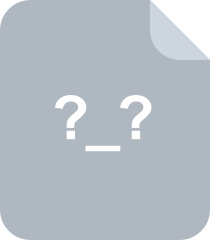
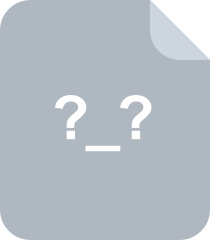
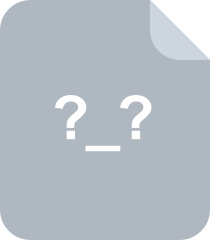
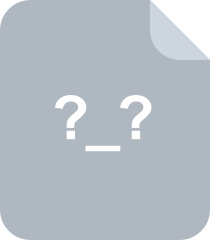
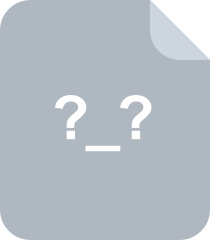
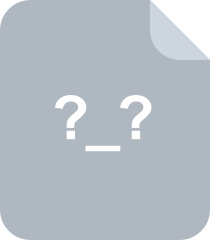
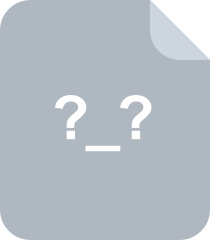
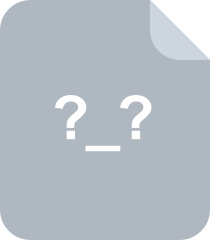
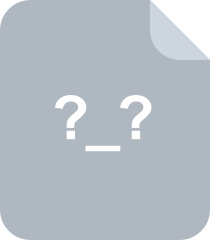
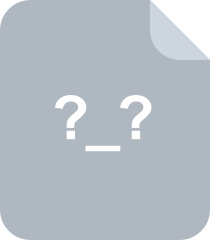
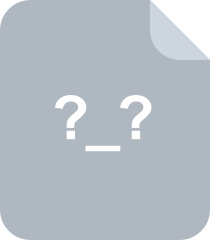
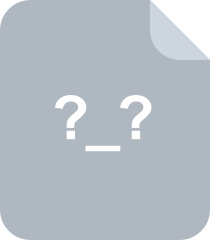
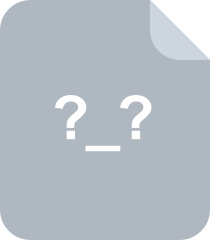
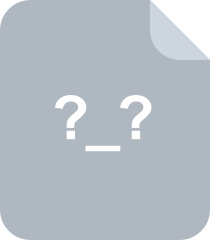
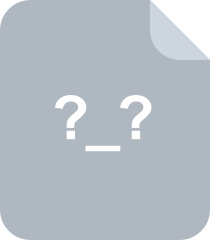
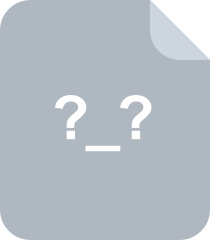
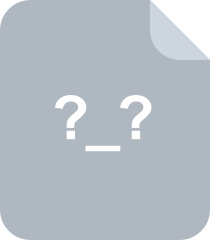
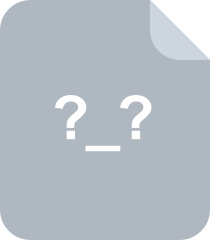
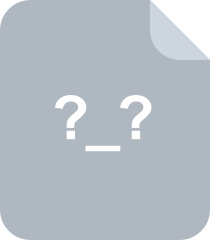
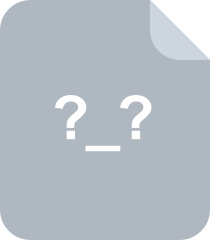
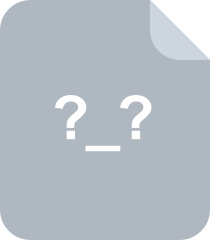
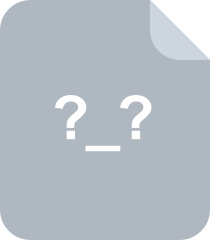
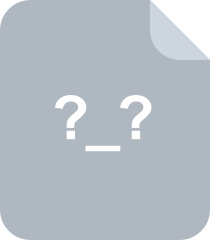
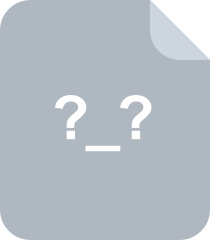
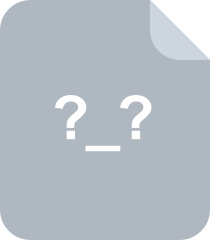
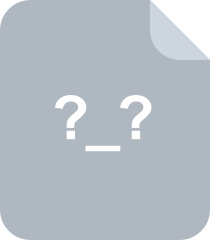
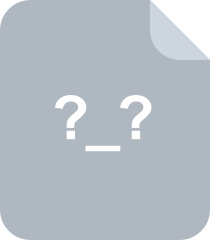
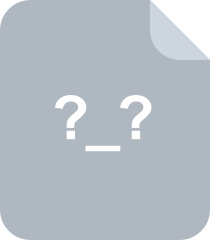
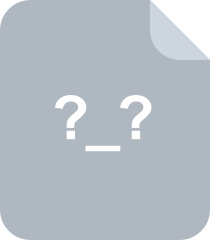
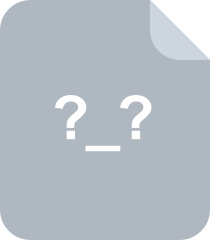
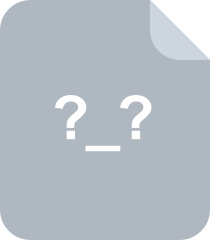
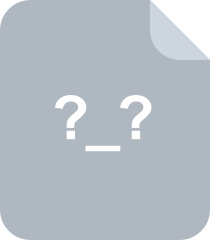
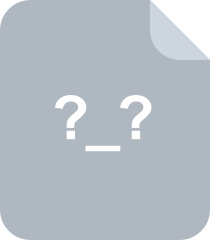
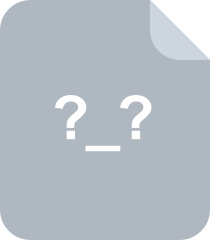
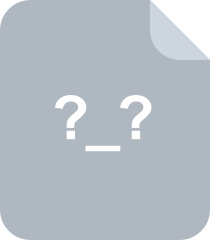
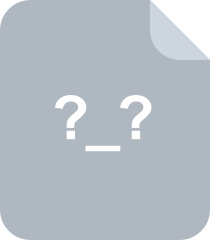
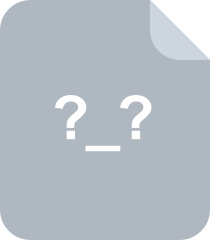
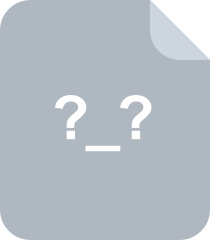
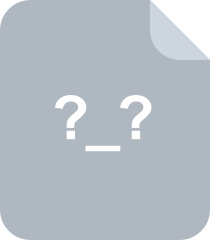
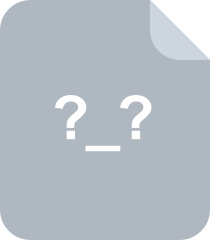
共 761 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
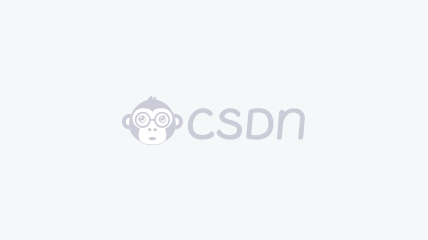

greenfarmer
- 粉丝: 0
- 资源: 33
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

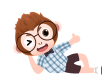
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


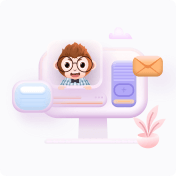
安全验证
文档复制为VIP权益,开通VIP直接复制
