/**
* Copyright (c) 2015 迅维web端团队 All rights reserved
*
* Base on awframework,powered by xunwei web team
*
* Licensed under the Apache License, Version 2.0 (the "License");
*/
package com.xunwei.netalarm.xx.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import org.apache.commons.lang3.time.DateFormatUtils;
/**
* 日期工具类, 继承org.apache.commons.lang.time.DateUtils类
*
* @author 迅维web端团队
* @version 2013-3-15
*/
public class DateUtil extends org.apache.commons.lang3.time.DateUtils {
public static final String YYMMDDHHMMSS = "yyyyMMddHHmmss";
/**
* 得到当前日期字符串 格式(yyyy-MM-dd)
*/
public static String getDate() {
return getDate("yyyy-MM-dd");
}
/**
* 得到当前日期字符串 格式(yyyy-MM-dd) pattern可以为:"yyyy-MM-dd" "HH:mm:ss" "E"
*/
public static String getDate(final String pattern) {
return DateFormatUtils.format(new Date(), pattern);
}
/**
* 得到日期字符串 默认格式(yyyy-MM-dd) pattern可以为:"yyyy-MM-dd" "HH:mm:ss" "E"
*/
public static String formatDate(final Date date, final Object... pattern) {
String formatDate = null;
if (pattern != null && pattern.length > 0) {
formatDate = DateFormatUtils.format(date, pattern[0].toString());
} else {
formatDate = DateFormatUtils.format(date, "yyyy-MM-dd");
}
return formatDate;
}
/**
* 得到日期时间字符串,转换格式(yyyy-MM-dd HH:mm:ss)
*/
public static String formatDateTime(final Date date) {
return formatDate(date, "yyyy-MM-dd HH:mm:ss");
}
/**
* 得到当前时间字符串 格式(HH:mm:ss)
*/
public static String getTime() {
return formatDate(new Date(), "HH:mm:ss");
}
/**
* 得到当前日期和时间字符串 格式(yyyy-MM-dd HH:mm:ss)
*/
public static String getDateTime() {
return formatDate(new Date(), "yyyy-MM-dd HH:mm:ss");
}
/**
* 得到当前年份字符串 格式(yyyy)
*/
public static String getYear() {
return formatDate(new Date(), "yyyy");
}
/**
* 得到当前月份字符串 格式(MM)
*/
public static String getMonth() {
return formatDate(new Date(), "MM");
}
/**
* 得到当天字符串 格式(dd)
*/
public static String getDay() {
return formatDate(new Date(), "dd");
}
/**
* 得到当前星期字符串 格式(E)星期几
*/
public static String getWeek() {
return formatDate(new Date(), "E");
}
/**
* 获取过去的天数
*
* @param date
* @return
*/
public static long pastDays(final Date date) {
final long t = new Date().getTime() - date.getTime();
return t / (24 * 60 * 60 * 1000);
}
public static Date getDateStart(Date date) {
if (date == null)
return null;
final SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
date = sdf.parse(formatDate(date, "yyyy-MM-dd") + " 00:00:00");
} catch (final ParseException e) {
e.printStackTrace();
}
return date;
}
public static Date getDateEnd(Date date) {
if (date == null)
return null;
final SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
date = sdf.parse(formatDate(date, "yyyy-MM-dd") + " 23:59:59");
} catch (final ParseException e) {
e.printStackTrace();
}
return date;
}
/**
* 按星期比较日期大小,是今天以前日期小于0,以后日期大于0
*
* @param target
* @return
*/
public static int compareToByWeek(final Date target) {
try {
int returnInt = 0;
final Calendar calendar = Calendar.getInstance();
final Calendar targetCal = Calendar.getInstance();
targetCal.setTime(target);
calendar.add(Calendar.DATE, -calendar.get(Calendar.DAY_OF_WEEK) + 2);
calendar.get(Calendar.DAY_OF_YEAR);
targetCal.get(Calendar.DAY_OF_YEAR);
calendar.get(Calendar.YEAR);
targetCal.get(Calendar.YEAR);
if (targetCal.get(Calendar.YEAR) < calendar.get(Calendar.YEAR)) {
returnInt = -1;
} else if (calendar.get(Calendar.YEAR) < targetCal.get(Calendar.YEAR)) {
returnInt = 1;
} else {
if (targetCal.get(Calendar.DAY_OF_YEAR) < calendar.get(Calendar.DAY_OF_YEAR)) {
returnInt = -1;
} else if (calendar.get(Calendar.DAY_OF_YEAR) < targetCal.get(Calendar.DAY_OF_YEAR)) {
returnInt = 1;
} else {
returnInt = 0;
}
}
return returnInt;
} catch (final Exception e) {
return 0;
}
}
/**
* @param args
* @throws ParseException
*/
public static void main(final String[] args) throws ParseException {
// System.out.println(formatDate(parseDate("2010/3/6")));
// System.out.println(getDate("yyyyMMddHH:mm:ss"));
// long time = new Date().getTime()-parseDate("2012-11-19").getTime();
// System.out.println(time/(24*60*60*1000));
System.out.println(getDate("HHmmss"));
}
}

若鱼1919
- 粉丝: 3949
- 资源: 51
最新资源
- 操作系统实验ucore lab3
- DG储能选址定容模型matlab 程序采用改进粒子群算法,考虑时序性得到分布式和储能的选址定容模型,程序运行可靠 这段程序是一个改进的粒子群算法,主要用于解决电力系统中的优化问题 下面我将对程序进行详
- final_work_job1(1).sql
- 区块链与联邦学习结合:FedChain项目详细复现指南
- 西门子S7 和 S7 Plus 协议开发示例
- 模块化多电平变流器 MMC 的VSG控制 同步发电机控制 MATLAB–Simulink仿真模型 5电平三相MMC,采用VSG控制 受端接可编辑三相交流源,直流侧接无穷大电源提供调频能量 设置频率
- 微电网(两台)主从控制孤岛-并网平滑切的分析 分析了: 1.孤岛下VF控制 2.并网下PQ控制 3.孤岛下主从控制 4.孤岛到并网的平滑切控制 5.除模型外还对分布式发电与主动配电网一些常见问题做了
- 第四组二手产品.zip
- 基于小程序的智慧物业平台源代码(java+小程序+mysql+LW).zip
- MVIMG_20241222_194113.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


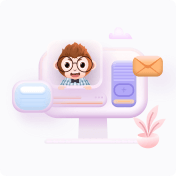
- 1
- 2
前往页