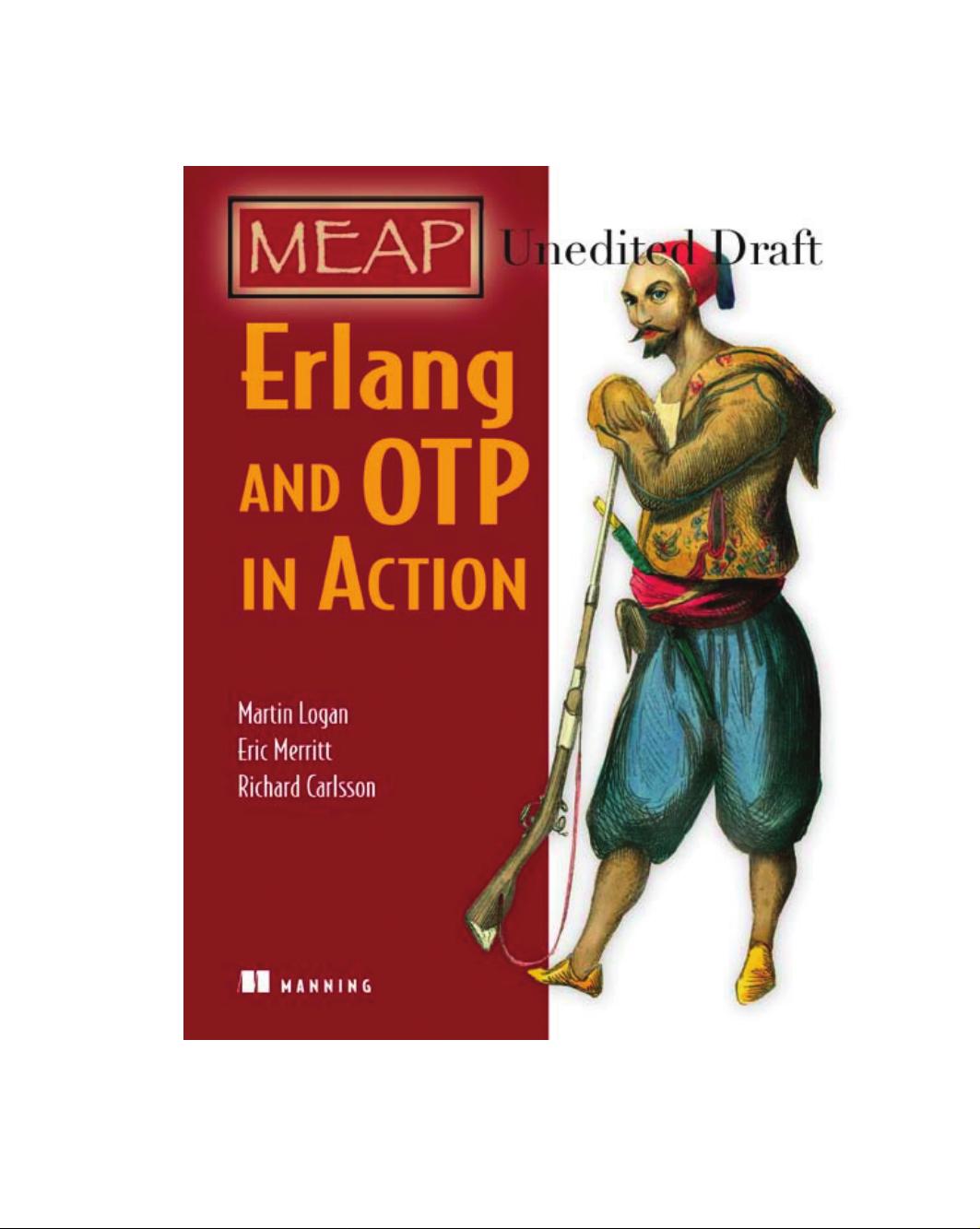
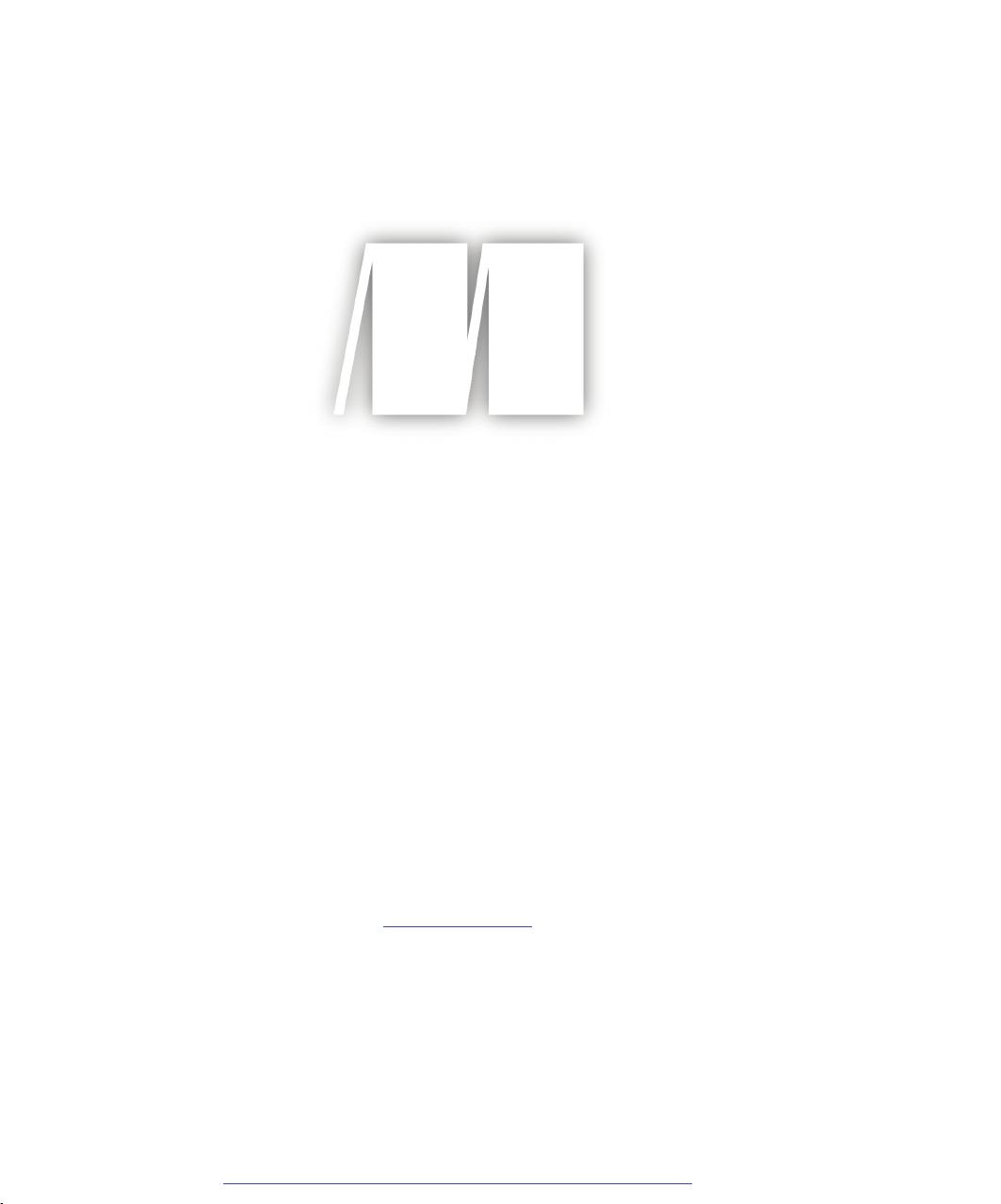
2
©Manning Publications Co. Please post comments or corrections to the Author Online forum:
http://www.manning-sandbox.com/forum.jspa?forumID=454
MEAP Edition
Manning Early Access Program
Copyright 2009 Manning Publications
For more information on this and other Manning titles go to
www.manning.com
Licensed to Wow! eBook <www.wowebook.com>
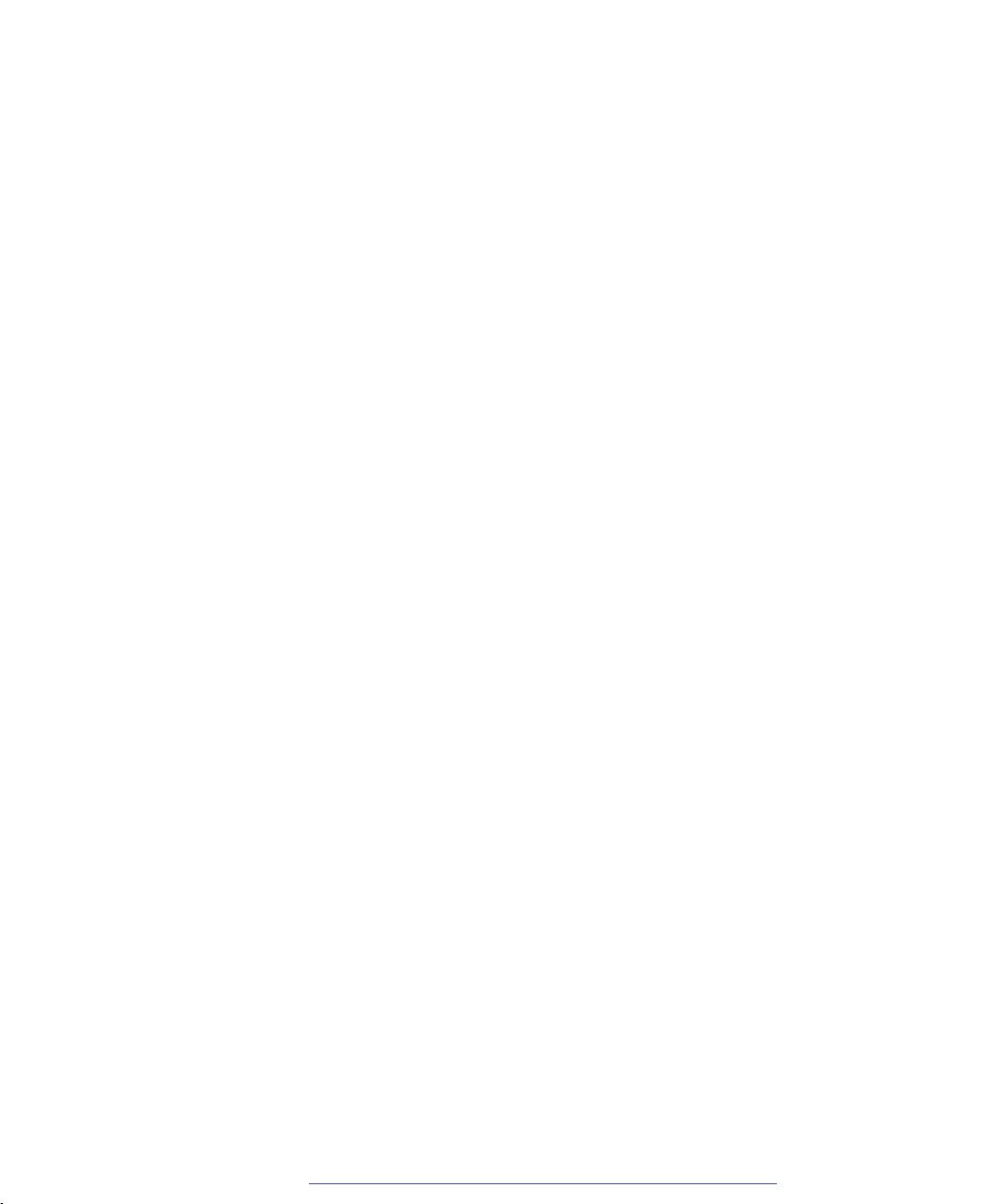
3
©Manning Publications Co. Please post comments or corrections to the Author Online forum:
http://www.manning-sandbox.com/forum.jspa?forumID=454
Table of Contents
Part One: Getting Past Pure Erlang; The OTP Basics
Chapter One: The Foundations of Erlang/OTP
Chapter Two: Erlang Essentials
Chapter Three: Writing a TCP based RPC Service
Chapter Four: OTP Packaging and Organization
Chapter Five: Processes, Linking and the Platform
Part Two: Building A Production System
Chapter Six: Implementing a Caching System
Chapter Seven: Logging and Eventing the Erlang/OTP way
Chapter Eight: Introducing Distributed Erlang/OTP way
Chapter Nine: Converting the Cache into a Distributed Application
Chapter Ten: Packaging, Services and Deployment
Part Three: Working in a Modern Environment
Chapter Eleven: Non-native Erlang Distribution with TCP and REST
Chapter Twelve: Drivers and Multi-Language Interfaces
Chapter Thirteen: Communication between Erlang and Java via JInterface
Chapter Fourteen: Optimization and Performance
Chapter Fifteen: Make it Faster
Appendix A – Installing Erlang
Appendix B – Lists and Referential Transparency
Licensed to Wow! eBook <www.wowebook.com>
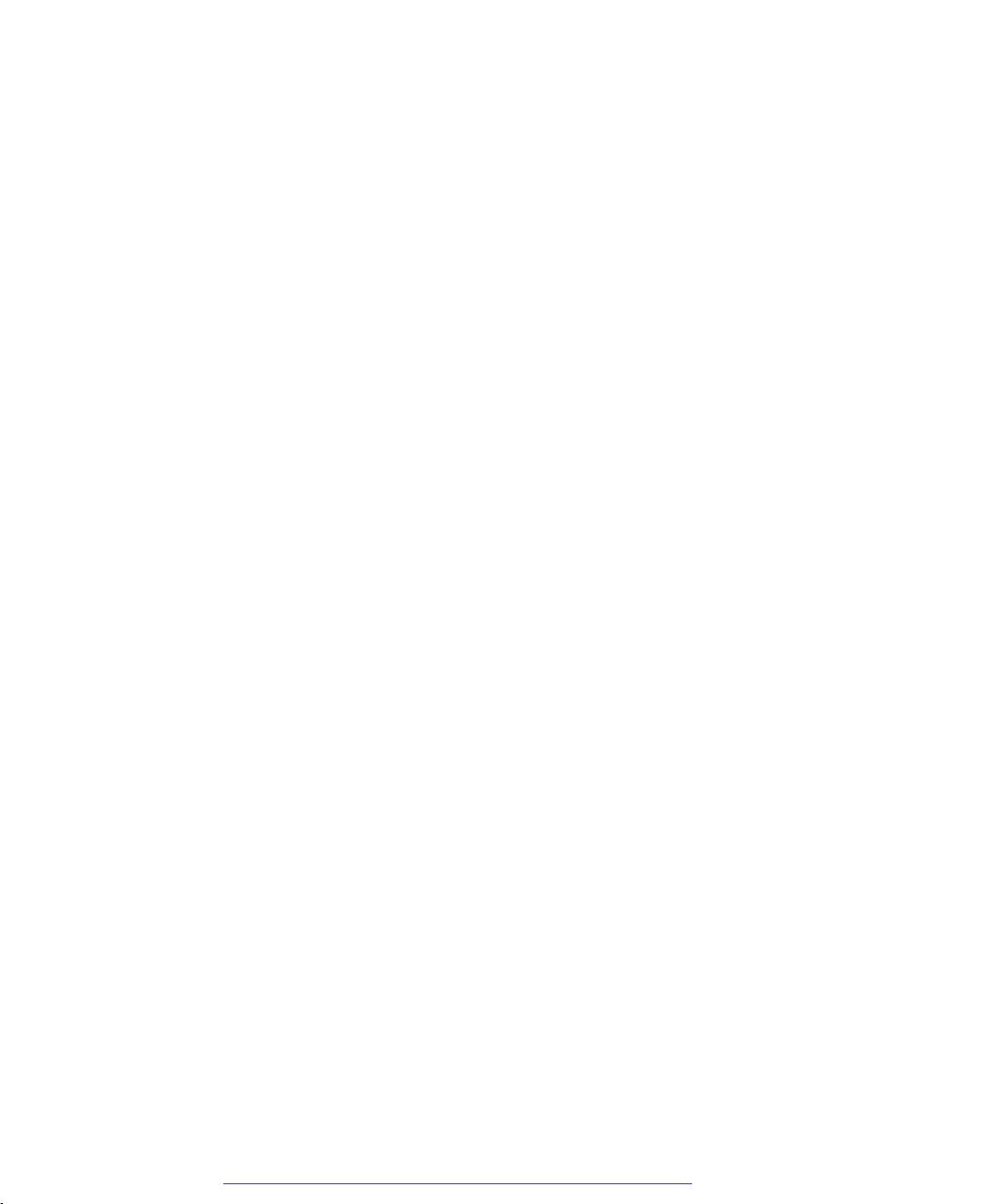
4
©Manning Publications Co. Please post comments or corrections to the Author Online forum:
http://www.manning-sandbox.com/forum.jspa?forumID=454
1
The foundations of Erlang/OTP
Welcome to our book about Erlang and OTP in action! You probably know already that Erlang
is a programming language—and as such it is pretty interesting in itself—but our focus here
will be on the practical and the “in action”, and for that we also need the OTP framework.
This is always included in any Erlang distribution, and is actually such an integral part of
Erlang these days that it is hard to say where the line is drawn between OTP and the plain
standard libraries; hence, one often writes “Erlang/OTP” to refer to either or both.
But why should we learn to use the OTP framework, when we could just hack away,
rolling our own solutions as we go? Well, these are some of the main points of OTP:
Productivity
Using OTP makes it possible to produce production-quality systems in very short time.
Stability
Code written on top of OTP can focus on the logic, and avoid error prone re-implementations
of the typical things that every real-world system will need: process management, servers,
state machines, etc.
Supervision
The application structure provided by the framework makes it simple to supervise and
control the running systems, both automatically and through graphical user interfaces.
Upgradability
The framework provides patterns for handling code upgrades in a systematic way.
Reliable code base
The code for the OTP framework itself is rock-solid and has been thoroughly battle tested.
Licensed to Wow! eBook <www.wowebook.com>
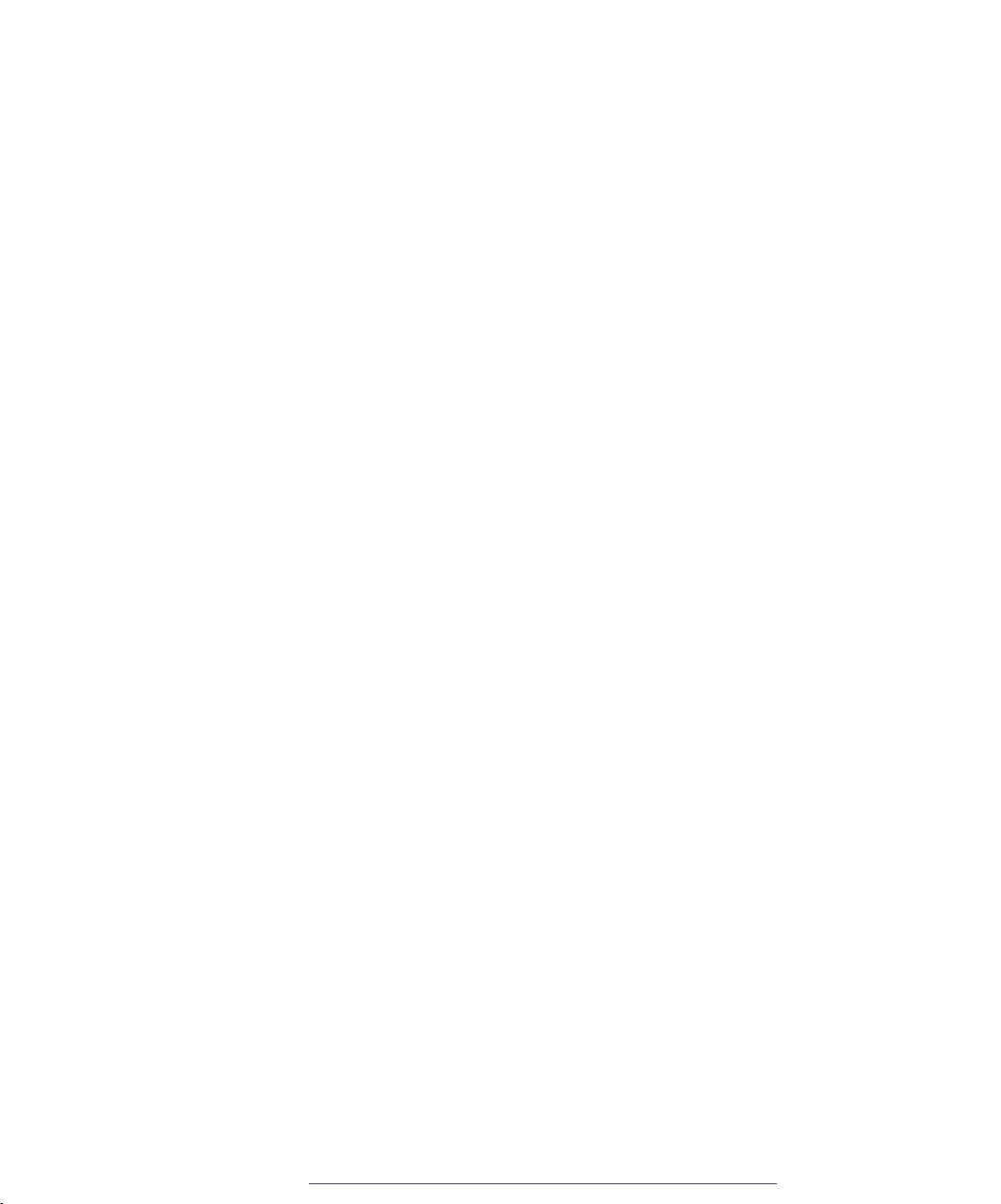
5
©Manning Publications Co. Please post comments or corrections to the Author Online forum:
http://www.manning-sandbox.com/forum.jspa?forumID=454
Despite these advantages, it is probably true to say that to most Erlang programmers,
OTP is still something of a secret art, learned partly by osmosis and partly by poring over the
more impenetrable sections of the documentation. We would like to change this. This is to
our knowledge the first book focused on learning to use OTP, and we want to show that it
can be a much easier experience than you might think. We are sure you won’t regret it.
In this first chapter, we will present the core features on which Erlang/OTP is built, and
that are provided by the Erlang programming language and run-time system:
Processes and concurrency
Fault tolerance
Distributed programming
Erlang's core functional language
The point here is to get you acquainted with the thinking behind all the concrete stuff
we’ll be diving into from chapter 2 onwards, rather than starting off by handing you a whole
bunch of facts up front. Erlang is different, and many of the things you will see in this book
will take some time to get accustomed to. With this chapter, we hope to give you some idea
of why things work the way they do, before we get into technical details.
1.1 – Understanding processes and concurrency
Erlang was designed for concurrency—having multiple tasks running simultaneously—from
the ground up; it was a central concern when the language was designed. Its built-in support
for concurrency, which uses the process concept to get a clean separation between tasks,
allows us to create fault tolerant architectures and fully utilize the multi-core hardware that
is available to us today.
Before we go any further, we should explain exactly what we mean by the words
“process” and “concurrency”.
1.1.1 – Processes
Processes are at the heart of concurrency. A process is the embodiment of an ongoing
activity: an agent that is running a piece of program code, concurrent to other processes
running their own code, at their own pace.
Licensed to Wow! eBook <www.wowebook.com>