/*
* Copyright 2004-2005 OpenSymphony
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
/*
* Previously Copyright (c) 2001-2004 James House
*/
package org.quartz.impl.jdbcjobstore;
import java.io.IOException;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Proxy;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.quartz.Calendar;
import org.quartz.CronTrigger;
import org.quartz.JobDataMap;
import org.quartz.JobDetail;
import org.quartz.JobPersistenceException;
import org.quartz.ObjectAlreadyExistsException;
import org.quartz.Scheduler;
import org.quartz.SchedulerConfigException;
import org.quartz.SchedulerException;
import org.quartz.SimpleTrigger;
import org.quartz.Trigger;
import org.quartz.core.SchedulingContext;
import org.quartz.spi.ClassLoadHelper;
import org.quartz.spi.JobStore;
import org.quartz.spi.SchedulerSignaler;
import org.quartz.spi.TriggerFiredBundle;
import org.quartz.utils.DBConnectionManager;
import org.quartz.utils.Key;
import org.quartz.utils.TriggerStatus;
/**
* <p>
* Contains base functionality for JDBC-based JobStore implementations.
* </p>
*
* @author <a href="mailto:jeff@binaryfeed.org">Jeffrey Wescott</a>
* @author James House
*/
public abstract class JobStoreSupport implements JobStore, Constants {
/*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*
* Constants.
*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
protected static final String LOCK_TRIGGER_ACCESS = "TRIGGER_ACCESS";
protected static final String LOCK_JOB_ACCESS = "JOB_ACCESS";
protected static final String LOCK_CALENDAR_ACCESS = "CALENDAR_ACCESS";
protected static final String LOCK_STATE_ACCESS = "STATE_ACCESS";
protected static final String LOCK_MISFIRE_ACCESS = "MISFIRE_ACCESS";
/*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*
* Data members.
*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
protected String dsName;
protected String tablePrefix = DEFAULT_TABLE_PREFIX;
protected boolean useProperties = false;
protected String instanceId;
protected String instanceName;
protected String delegateClassName;
protected Class delegateClass = StdJDBCDelegate.class;
protected HashMap calendarCache = new HashMap();
private DriverDelegate delegate;
private long misfireThreshold = 60000L; // one minute
private boolean dontSetAutoCommitFalse = false;
private boolean isClustered = false;
private boolean useDBLocks = false;
private boolean lockOnInsert = true;
private Semaphore lockHandler = null; // set in initialize() method...
private String selectWithLockSQL = null;
private long clusterCheckinInterval = 7500L;
private ClusterManager clusterManagementThread = null;
private MisfireHandler misfireHandler = null;
private ClassLoadHelper classLoadHelper;
private SchedulerSignaler signaler;
protected int maxToRecoverAtATime = 20;
private boolean setTxIsolationLevelSequential = false;
private boolean acquireTriggersWithinLock = false;
private long dbRetryInterval = 10000;
private boolean makeThreadsDaemons = false;
private boolean threadsInheritInitializersClassLoadContext = false;
private ClassLoader initializersLoader = null;
private boolean doubleCheckLockMisfireHandler = true;
private final Log log = LogFactory.getLog(getClass());
/*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*
* Interface.
*
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
*/
/**
* <p>
* Set the name of the <code>DataSource</code> that should be used for
* performing database functions.
* </p>
*/
public void setDataSource(String dsName) {
this.dsName = dsName;
}
/**
* <p>
* Get the name of the <code>DataSource</code> that should be used for
* performing database functions.
* </p>
*/
public String getDataSource() {
return dsName;
}
/**
* <p>
* Set the prefix that should be pre-pended to all table names.
* </p>
*/
public void setTablePrefix(String prefix) {
if (prefix == null) {
prefix = "";
}
this.tablePrefix = prefix;
}
/**
* <p>
* Get the prefix that should be pre-pended to all table names.
* </p>
*/
public String getTablePrefix() {
return tablePrefix;
}
/**
* <p>
* Set whether String-only properties will be handled in JobDataMaps.
* </p>
*/
public void setUseProperties(String useProp) {
if (useProp == null) {
useProp = "false";
}
this.useProperties = Boolean.valueOf(useProp).booleanValue();
}
/**
* <p>
* Get whether String-only properties will be handled in JobDataMaps.
* </p>
*/
public boolean canUseProperties() {
return useProperties;
}
/**
* <p>
* Set the instance Id of the Scheduler (must be unique within a cluster).
* </p>
*/
public void setInstanceId(String instanceId) {
this.instanceId = instanceId;
}
/**
* <p>
* Get the instance Id of the Scheduler (must be unique within a cluster).
* </p>
*/
public String getInstanceId() {
return instanceId;
}
/**
* Set the instance name of the Scheduler (must be unique within this server instance).
*/
public void setInstanceName(String instanceName) {
this.instanceName = instanceName;
}
/**
* Get the instance name of the Scheduler (must be unique within this server instance).
*/
public String getInstanceName() {
return instanceName;
}
/**
* <p>
* Set whether this instance is part of a cluster.
* </p>
*/
public void setIsClustered(boolean isClustered) {
this.isClustered = isClustered;
}
/**
* <p>
* Get whether this instance is part of a cluster.
* </p>
*/
public boolean isClustered() {
return isClustered;
}
/**
* <p>
* Get the frequency (in milliseconds) at which this instance "checks-in"
* with the other instances of the cluster. -- Affects the rate of
* detecting failed instances.
* </p>
*/
public long getClusterCheckinInterval() {
return clusterCheckinInterval;
}
/**
* <p>
* Set the frequency (in milliseconds) at which this instance "ch
没有合适的资源?快使用搜索试试~ 我知道了~
quartz-1.6.5 API
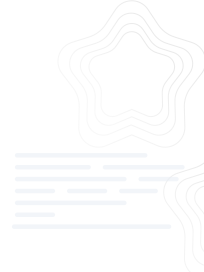
共1004个文件
html:545个
java:220个
gif:32个


温馨提示
Quartz是OpenSymphony开源组织在Job scheduling领域又一个开源项目,它可以与J2EE与J2SE应用程序相结合也可以单独使用。Quartz可 以用来创建简单或为运行十个,百个,甚至是好几万个Jobs这样复杂的日程序表。Jobs可以做成标准的Java组件或 EJBs。Quartz的最新 版本为Quartz 1.6.5。
资源推荐
资源详情
资源评论
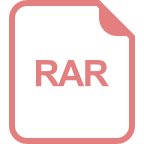
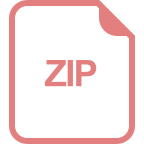
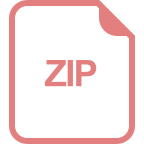
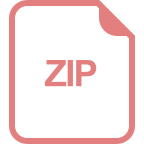
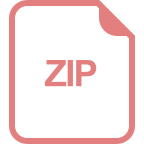
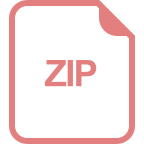
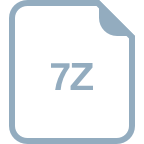
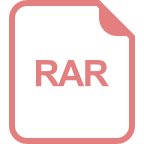
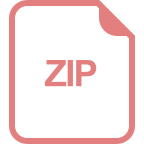
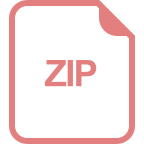
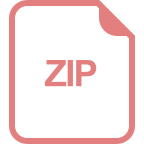
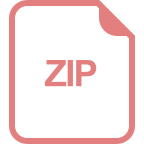
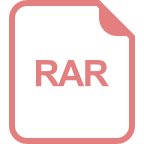
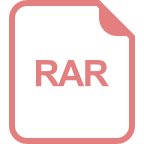
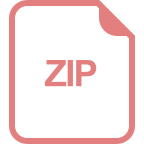
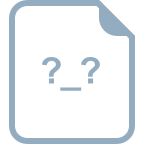
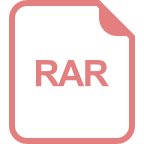
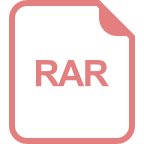
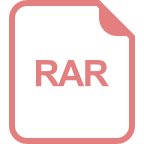
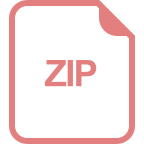
收起资源包目录

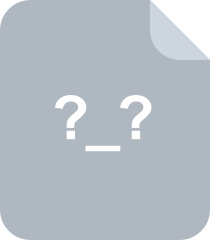
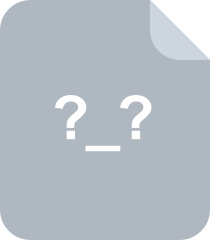
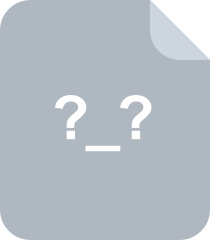
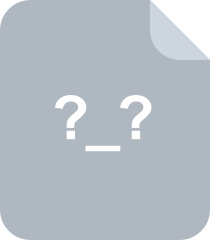
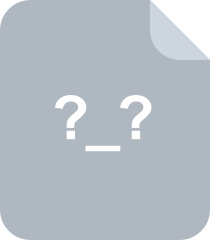
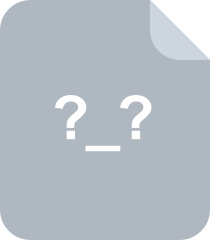
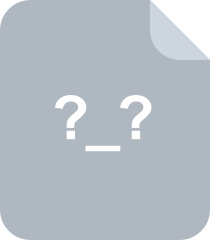
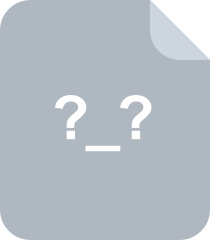
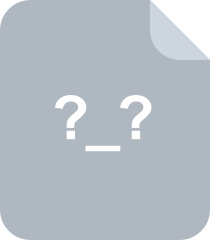
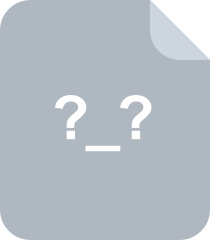
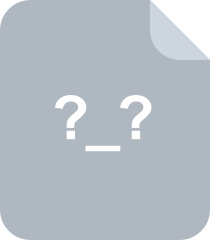
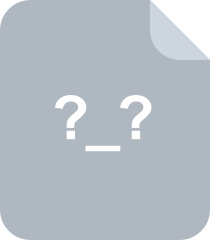
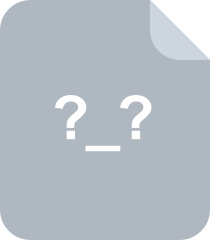
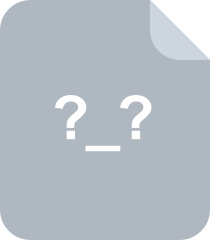
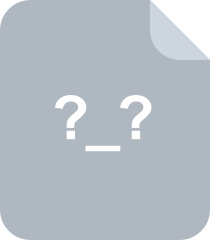
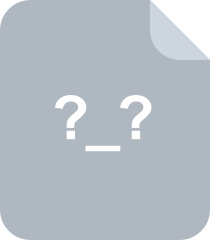
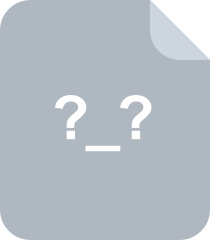
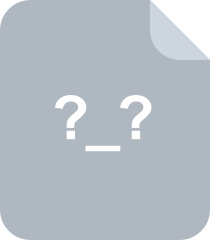
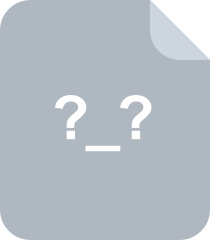
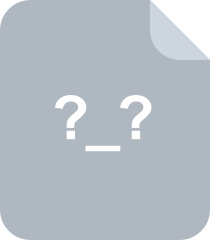
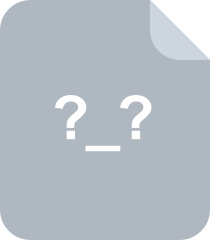
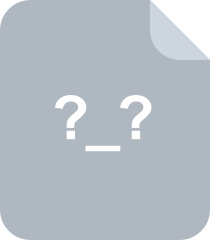
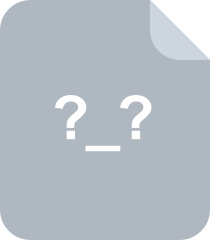
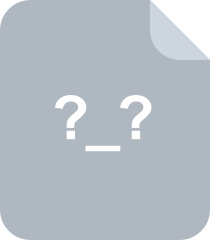
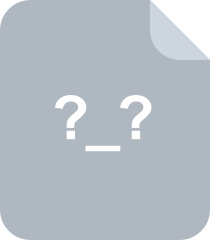
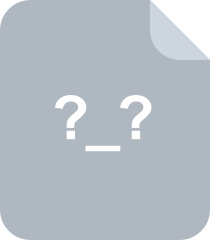
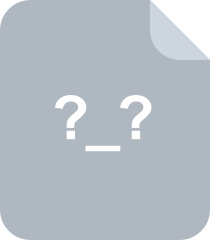
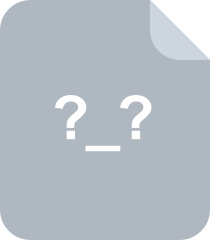
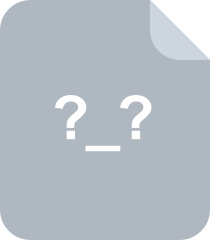
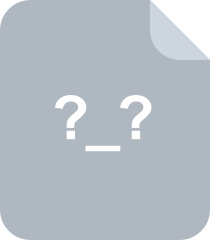
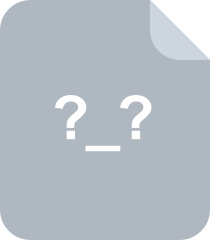
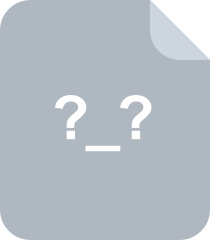
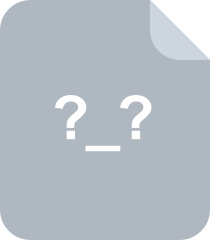
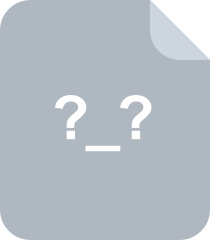
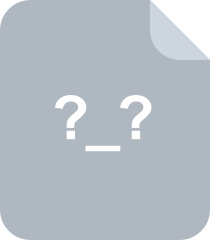
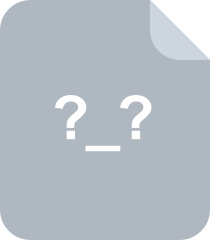
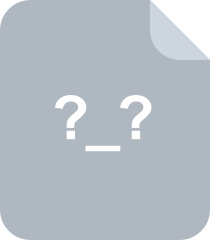
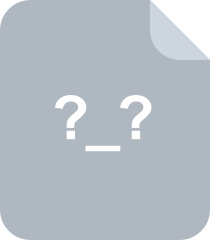
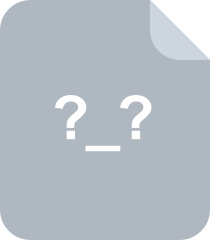
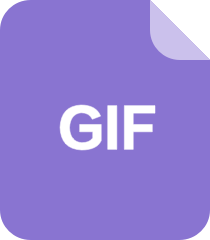
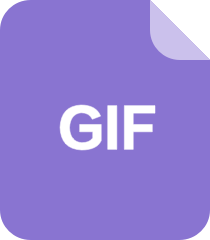
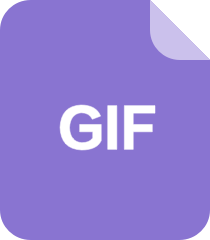
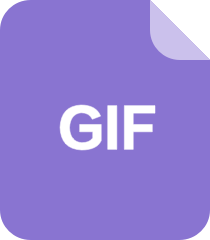
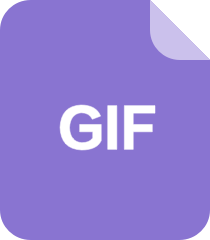
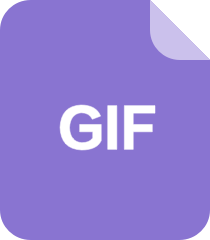
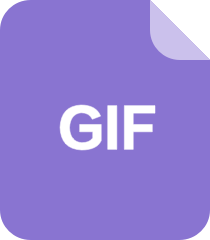
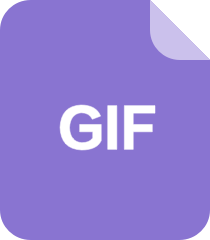
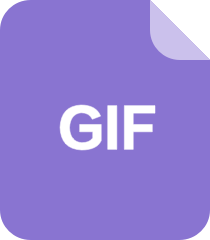
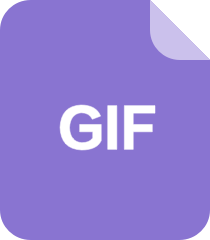
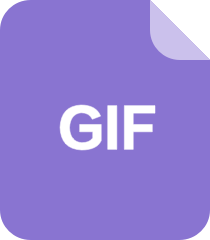
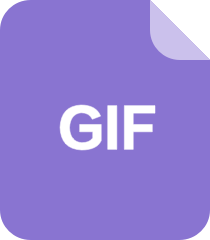
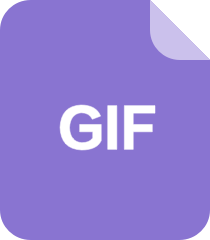
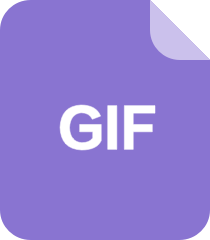
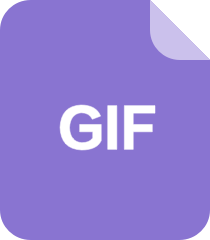
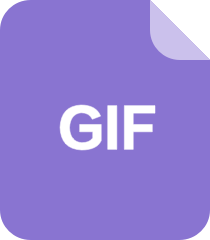
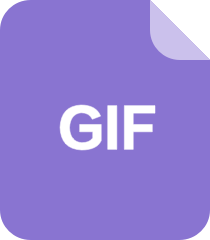
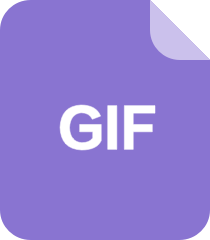
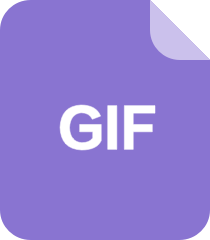
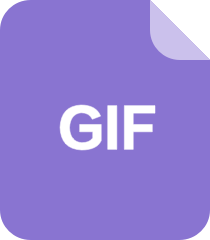
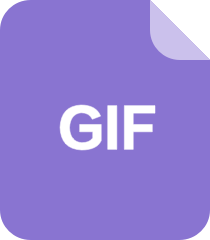
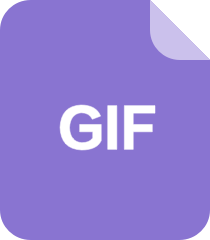
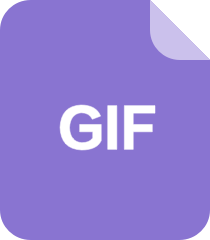
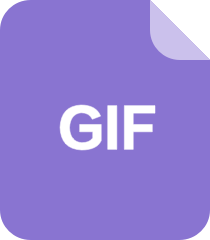
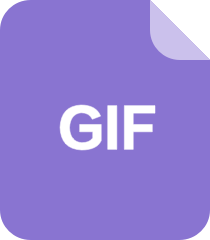
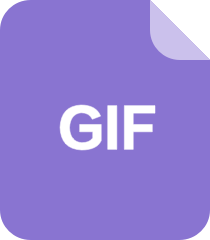
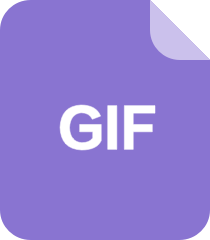
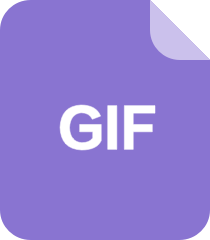
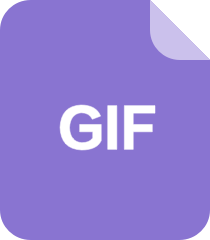
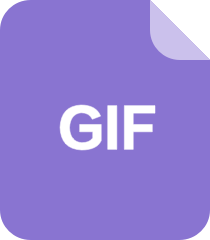
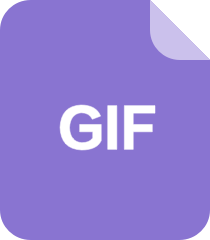
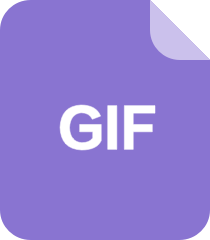
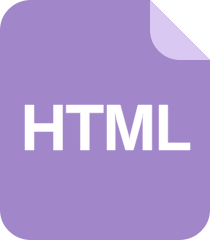
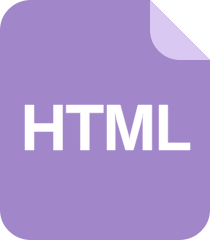
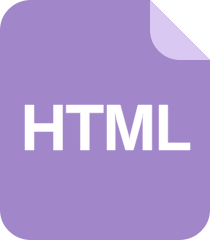
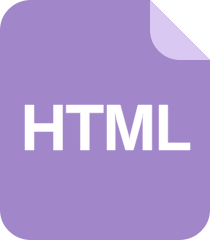
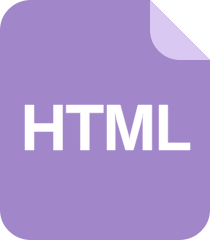
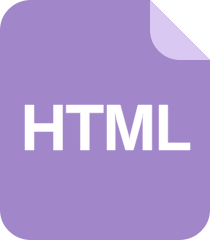
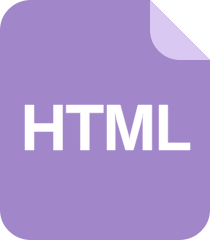
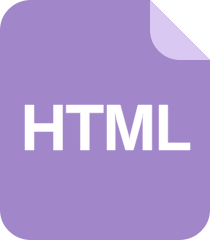
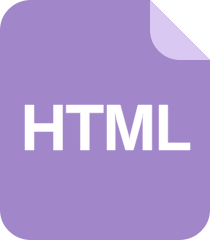
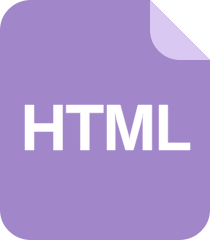
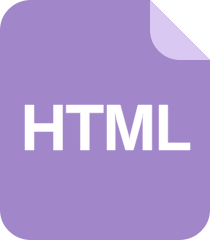
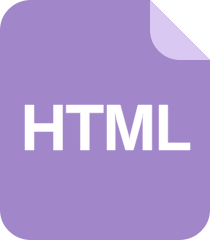
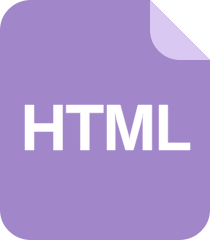
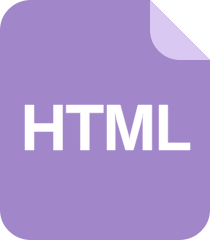
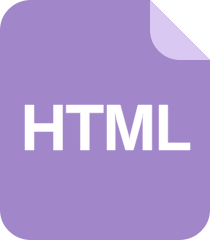
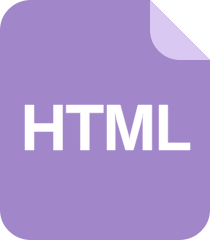
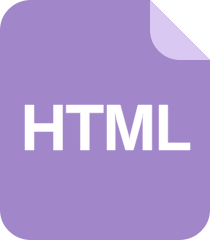
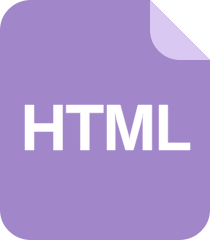
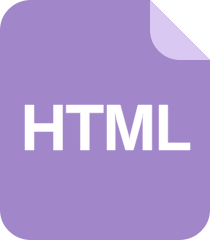
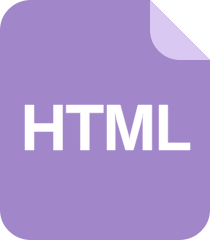
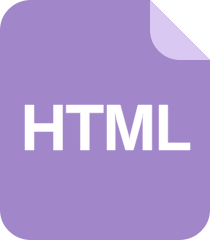
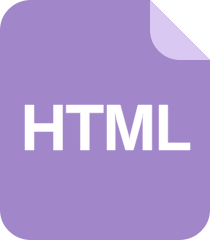
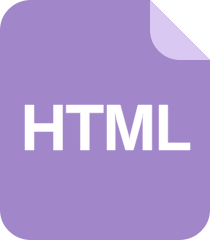
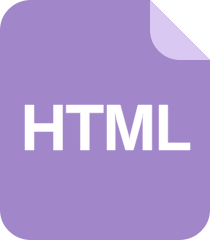
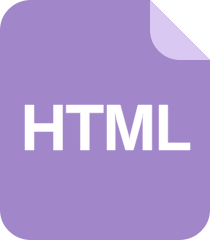
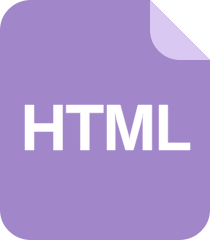
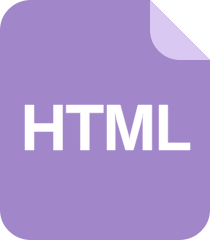
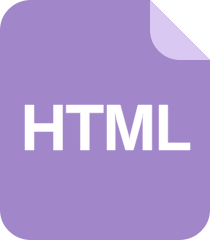
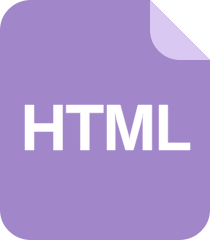
共 1004 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11

Andy_Tsinge
- 粉丝: 37
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

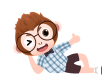
最新资源
- (源码)基于Java虚拟机(JVM)的内存管理与垃圾回收系统.zip
- (源码)基于QT和Python的熊猫检测系统.zip
- (源码)基于Spring Boot和Vue的直播数据可视化系统.zip
- (源码)基于Spring Boot和Vue的CRM客户管理系统.zip
- (源码)基于C#的影院票务管理系统.zip
- (源码)基于JSP和Java的校园论坛管理系统.zip
- (源码)基于Spring Boot和MyBatisPlus的在线茶叶销售系统.zip
- (源码)基于Avalonia框架的ECS管理系统.zip
- (源码)基于C#和STM32的WiFi无线门禁考勤系统.zip
- (源码)基于SSM框架的客户管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


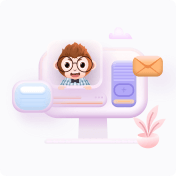
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页