package com.group.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class GroupDaoImpl implements GroupDaoInterface{
public void insertGroup(Group group) {
// TODO Auto-generated method stub
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DbUtils.getConnection();
String sql = "insert into [group](GROUPNAME,PRGROUPID,GROUPNOTE,USERID,SHAREFLG,ORDERDIS,CRTTIME) values(?,?,?,?,?,?,?)";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, group.getGroup_name());
pstmt.setString(2, group.getPrgroup_id());
pstmt.setString(3, group.getGroup_note());
pstmt.setInt(4, group.getUser_id());
pstmt.setString(5, group.getShareflg());
pstmt.setInt(6, group.getOrderdis());
pstmt.setDate(7, group.getGrttime());
pstmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
}
public void insertCards(Group group){
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DbUtils.getConnection();
String sql = "insert into group_card(GROUPID,MIDID) values(?,?)";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, group.getGroup_id());
pstmt.setInt(2, group.getMidid());
pstmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
}
public void updateGroup(Group group) {
// TODO Auto-generated method stub
Connection conn = null;
PreparedStatement pstmt = null;
try {
conn = DbUtils.getConnection();
String sql = "update [group] set GROUPNAME=?,PRGROUPID=?,GROUPNOTE=?,SHAREFLG=?,ORDERDIS=?,CRTTIME=? where GROUPID=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, group.getGroup_name());
pstmt.setString(2, group.getPrgroup_id());
pstmt.setString(3, group.getGroup_note());
pstmt.setString(4, group.getShareflg());
pstmt.setInt(5, group.getOrderdis());
pstmt.setDate(6, group.getGrttime());
pstmt.setInt(7, group.getGroup_id());
pstmt.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
}
public void deleteGroup(String group_id) {
// TODO Auto-generated method stub
Connection conn=null;
PreparedStatement pstmt=null;
try
{
conn=DbUtils.getConnection();
String sql="delete group_card where GROUPID=?;delete [group] where GROUPID=?";
pstmt=conn.prepareStatement(sql);
pstmt.setString(1,group_id);
pstmt.setString(2,group_id);
pstmt.executeUpdate();
}
catch(SQLException ex)
{
ex.printStackTrace();
}finally{
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
}
public void deleteCard(String midid) {
// TODO Auto-generated method stub
Connection conn=null;
PreparedStatement pstmt=null;
try
{
conn=DbUtils.getConnection();
String sql="delete group_card where MIDID=?";
pstmt=conn.prepareStatement(sql);
pstmt.setString(1,midid);
pstmt.executeUpdate();
}
catch(SQLException ex)
{
ex.printStackTrace();
}finally{
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
}
public List<Group> selectAllOwnGroups(int user_id) {
// TODO Auto-generated method stub
Connection conn = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
List<Group> groups=new ArrayList<Group>();
try {
conn = DbUtils.getConnection();
String sql = "select GROUPNAME from [group] where USERID=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1,user_id);
rs = pstmt.executeQuery();
while(rs.next()) {
Group group = new Group();
group.setGroup_name(rs.getString("GROUPNAME"));
groups.add(group);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeResult(rs);
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
return groups;
}
public List<Group> selectAllGroups(int user_id) {
// TODO Auto-generated method stub
Connection conn = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
List<Group> groups=new ArrayList<Group>();
try {
conn = DbUtils.getConnection();
String sql = "select GROUPNAME from [group] where USERID<>?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, user_id);
rs = pstmt.executeQuery();
while(rs.next()) {
Group group = new Group();
group.setGroup_name(rs.getString("GROUPNAME"));
groups.add(group);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeResult(rs);
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
return groups;
}
public List<Group> selectAllUsers(int user_id) {
// TODO Auto-generated method stub
Connection conn = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
List<Group> groups=new ArrayList<Group>();
try {
conn = DbUtils.getConnection();
String sql = "select USERNAME from [user] where USERID<>?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, user_id);
rs = pstmt.executeQuery();
while(rs.next()) {
Group group = new Group();
group.setUser_name(rs.getString("USERNAME"));
groups.add(group);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeResult(rs);
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
return groups;
}
public Group selectGroup_id(String group_name,int user_id){
Connection conn = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
Group group = new Group();
try {
conn = DbUtils.getConnection();
String sql = "select GROUPID from [group] where GROUPNAME=? and USERID=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, group_name);
pstmt.setInt(2, user_id);
rs = pstmt.executeQuery();
if(rs.next()) {
group.setGroup_id(rs.getInt("GROUPID"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeResult(rs);
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
return group;
}
public Group selectGroupById(int group_id) {
// TODO Auto-generated method stub
Connection conn = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
Group group = new Group();
try {
conn = DbUtils.getConnection();
String sql = "select * from [group] where GROUPID=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1,group_id);
rs = pstmt.executeQuery();
if(rs.next()) {
group.setGroup_id(rs.getInt("GROUPID"));
group.setGroup_name(rs.getString("GROUPNAME"));
group.setPrgroup_id(rs.getString("PRGROUPID"));
group.setGroup_note(rs.getString("GROUPNOTE"));
group.setShareflg(rs.getString("SHAREFLG"));
group.setOrderdis(rs.getInt("ORDERDIS"));
group.setGrttime(rs.getDate("CRTTIME"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
DbUtils.closeResult(rs);
DbUtils.closeStatement(pstmt);
DbUtils.closeConnection();
}
return group;
}
public List<Group> selectCardsById(String[] mid) {
// TODO Auto-generated method stub
Connection conn = null;
ResultSet rs = null;
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
这是我们学校为期两周的JavaEE实训,做一个客户资源管理系统。客户资源管理是对公司内部员工的名片进行管理的系统,其包括名片管理、组管理、访问日志管理、公司/部门视图、用户信息管理、文件导出等功能。 该资源非常详细,包含了需求分析,数据库,跟完整的代码,还有需要的一些jar包。 所用数据库为sql server 2008,程序运行环境为myeclipse,将以使用者,将jsp的编码方式改为utf-8,用到图片上传的组件jspsmart.jar,会存在路径不能显示的状况,建议使用IE浏览器(解决办法:打开浏览器-工具-Internet选项-安全(将安全级别降到最低)-自定义级别里面找到“将文件上传到服务器时包含本地目录路径”,选中“启动”-点击“确定”) 将SQL Server文件夹下的cardjava_Data.MDF附加到sql server中,将aa_3导入到myeclipse里,改一下数据库连接的IP地址(DbUtils.java,DBhelper.java),发布在tomcat下,就可以在浏览器查看了
资源推荐
资源详情
资源评论
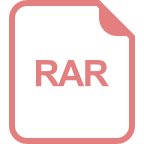
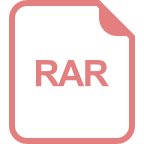
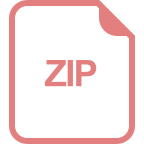
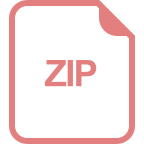
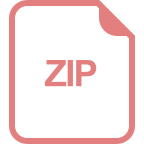
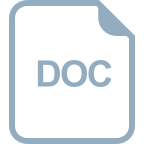
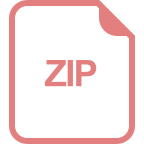
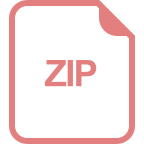
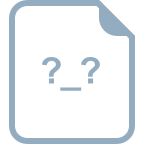
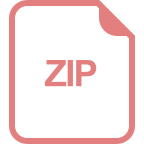
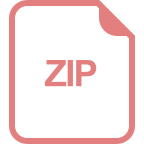
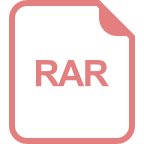
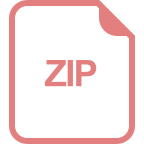
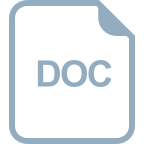
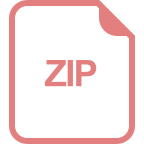
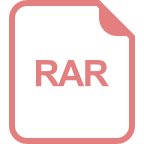
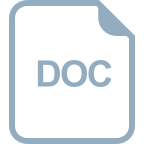
收起资源包目录

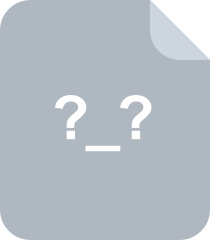
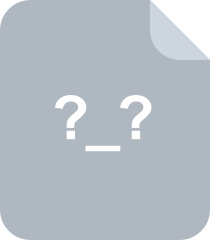
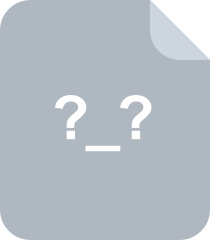
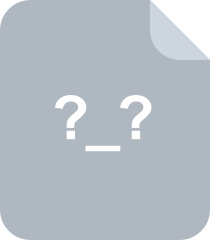
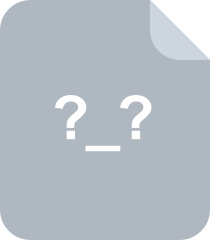
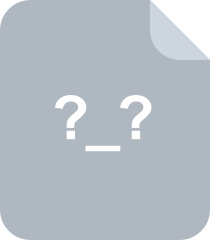
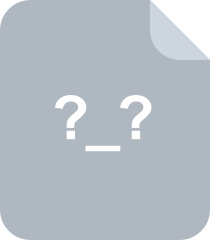
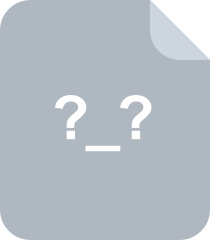
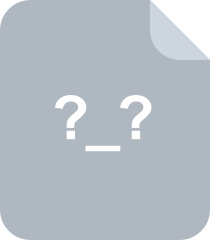
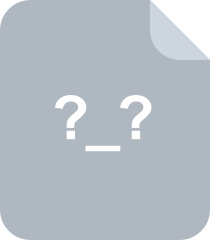
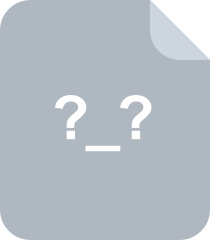
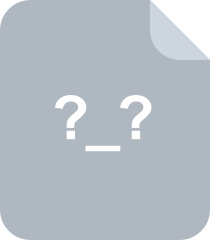
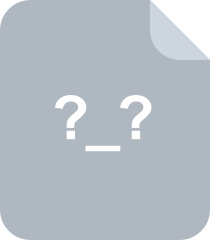
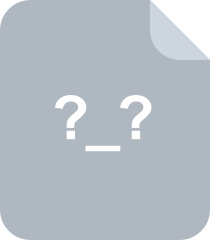
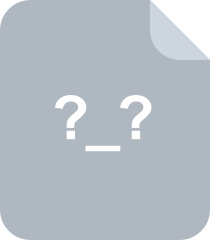
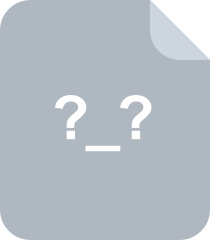
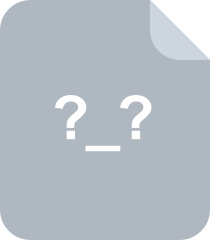
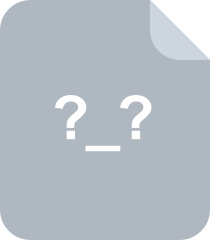
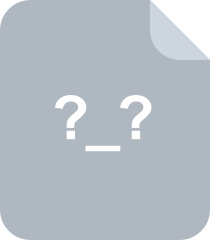
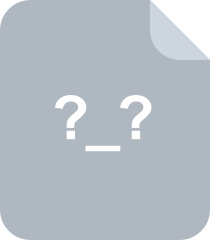
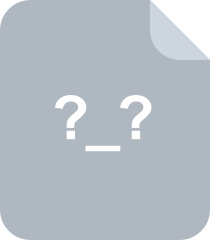
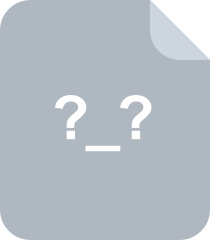
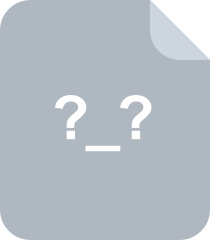
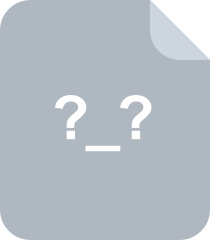
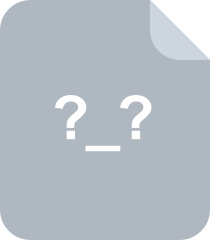
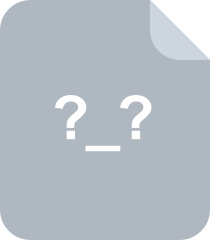
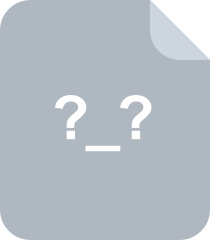
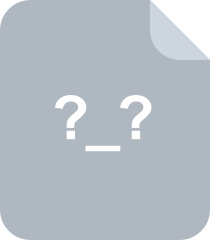
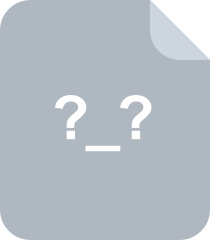
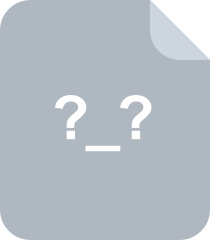
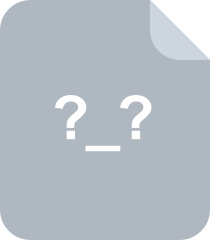
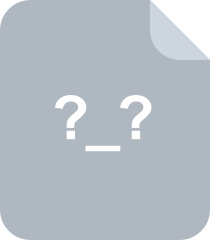
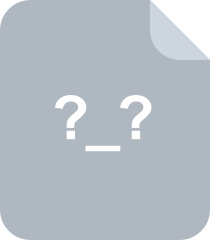
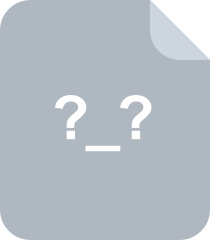
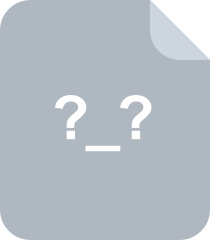
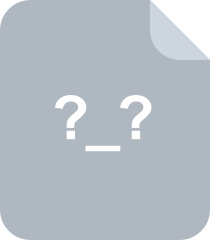
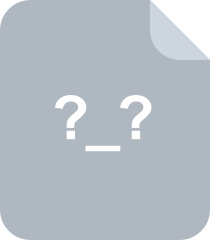
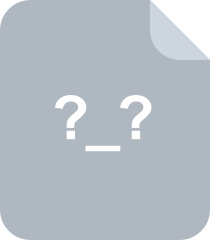
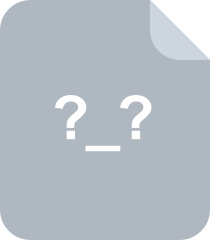
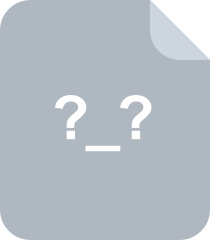
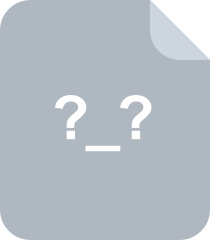
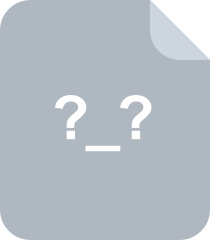
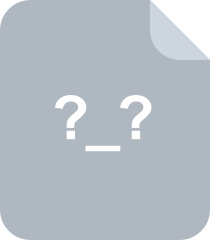
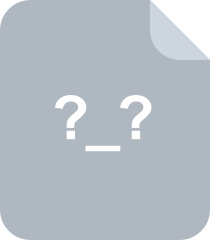
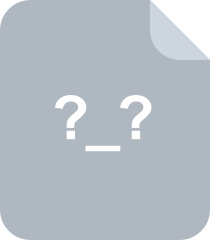
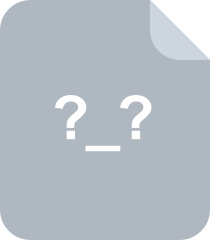
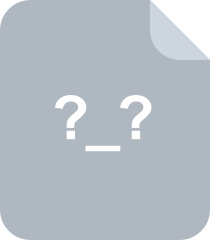
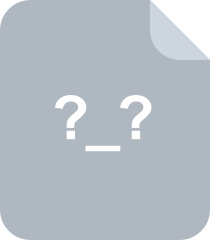
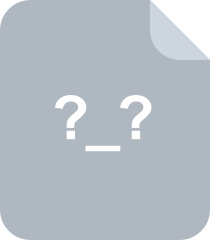
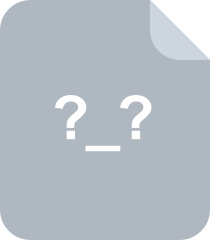
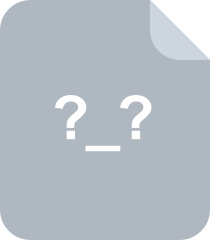
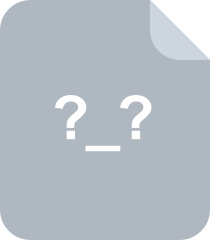
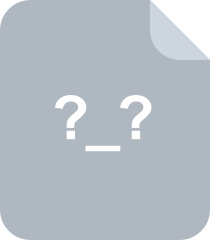
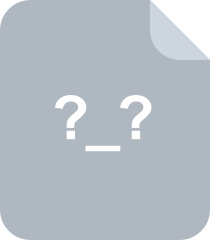
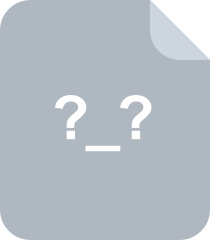
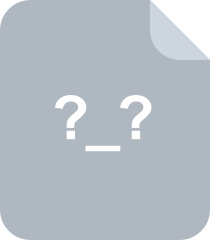
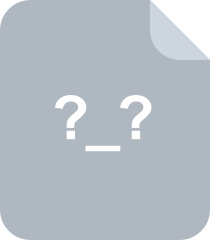
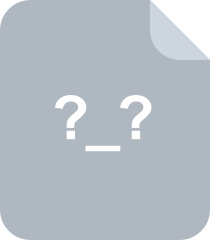
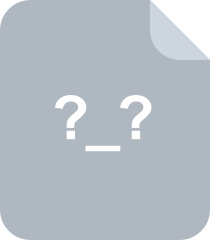
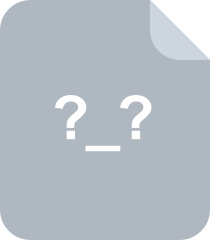
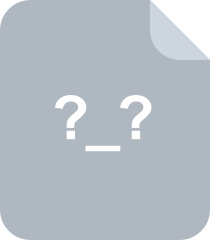
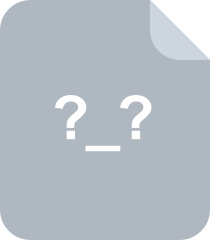
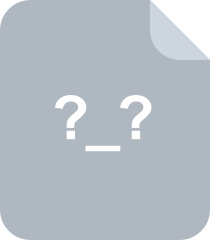
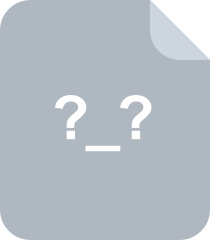
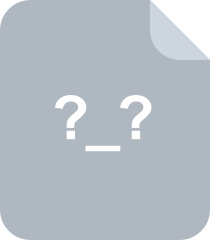
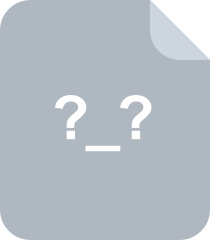
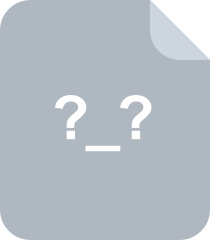
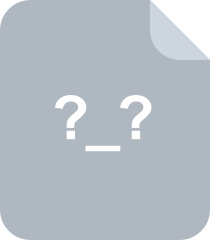
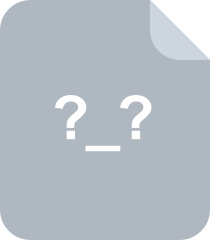
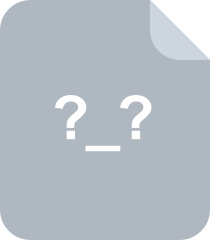
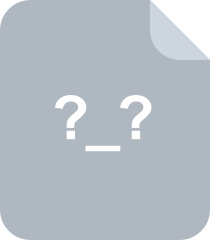
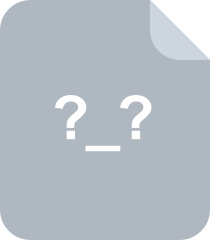
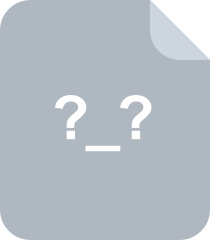
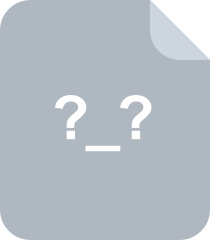
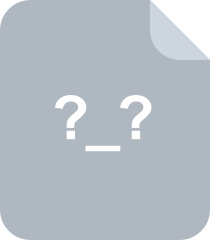
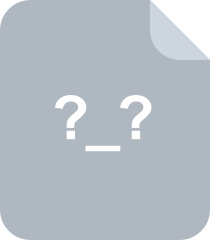
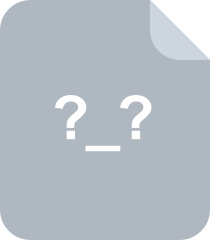
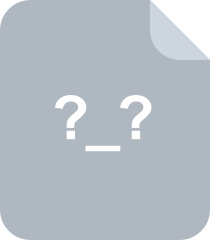
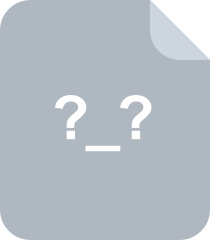
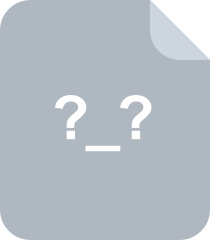
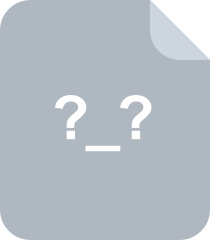
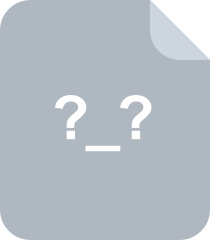
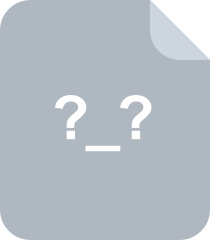
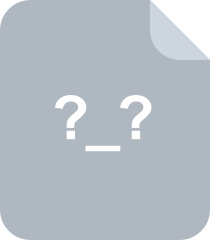
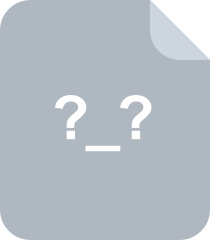
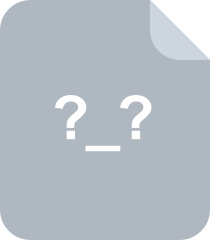
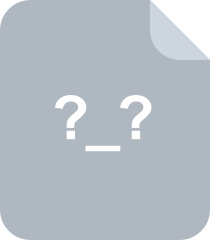
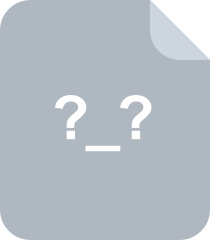
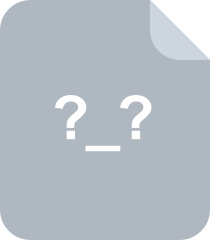
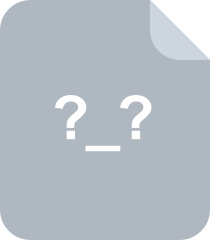
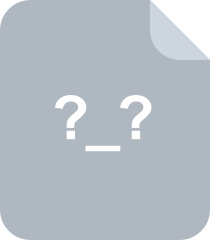
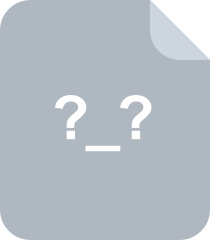
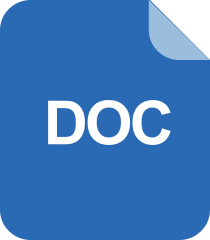
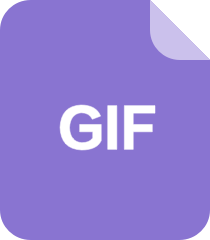
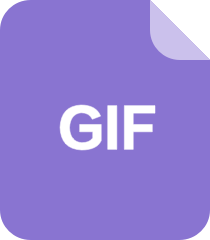
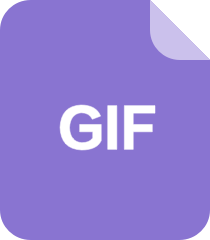
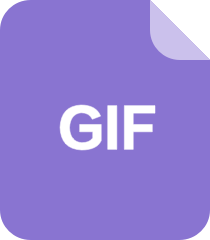
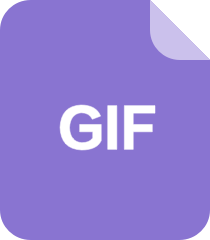
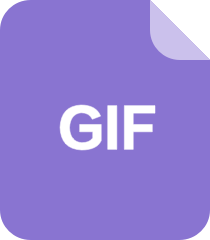
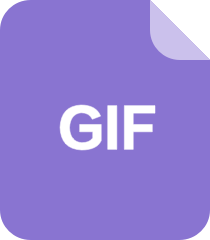
共 291 条
- 1
- 2
- 3

ggxiao1112
- 粉丝: 1
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

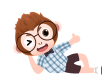
最新资源
- nuget 库官方下载包,可使用解压文件打开解压使用
- 非wine、原生Linux迅雷安装包deb文件,支持Ubuntu、UOS统信、深度Deepin、LinuxMint、Debain系通用
- KUKA机器人安装包,与PROFINET软件包
- 船舶燃料消耗和二氧化碳排放分析数据集,燃料消耗和碳排放关联分析数据
- req-sign、bd-ticket-ree-public加密算法(JS)
- 全自动批量建站快速养权重站系统【纯静态html站群版】:(GPT4.0自动根据关键词写文章+自动发布+自定义友链+自动文章内链+20%页面加提权词)
- 串联式、并联式、混联式混合动力系统simulink控制策略模型(串联式、并联式、混联式每个都是独立的需要单独说拿哪个,默认是混联式RB) 有基于逻辑门限值、状态机的规则控制策略(RB)、基于等效燃油
- 法码滋.exe法码滋2.exe法码滋3.exe
- python-geohash-0.8.5-cp38-cp38-win-amd64
- Matlab根据flac、pfc或其他软件导出的坐标及应力、位移数据再现云图 案例包括导出在flac6.0中导出位移的fish代码(也可以自己先准备软件导出的坐标数据及对应点的位移或应力数据,可根据需
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


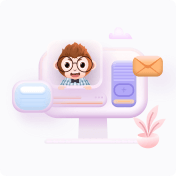
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页