/*******************************************************************************
* DTBase.cpp *
*------------*
* Description:
* This module contains the CDXBaseNTo1 transform
*-------------------------------------------------------------------------------
* Created By: Edward W. Connell Date: 07/28/97
* Copyright (C) 1997 Microsoft Corporation
* All Rights Reserved
*
*-------------------------------------------------------------------------------
* Revisions:
*
*******************************************************************************/
//--- Additional includes
#include "stdafx.h"
#include <DXTrans.h>
#include "DTBase.h"
#include "new.h"
//--- Initialize static member of debug scope class
#ifdef _DEBUG
CDXTDbgFlags CDXTDbgScope::m_DebugFlags;
#endif
//--- This should only be used locally in this file. We duplicated this GUID
// value to avoid having to include DDraw.
static const IID IID_IDXDupDirectDraw =
{ 0x6C14DB80,0xA733,0x11CE, { 0xA5,0x21,0x00,0x20,0xAF,0x0B,0xE5,0x60 } };
static const IID IID_IDXDupDDrawSurface =
{ 0x6C14DB81,0xA733,0x11CE, { 0xA5,0x21,0x00,0x20,0xAF,0x0B,0xE5,0x60 } };
static const IID IID_IDXDupDirect3DRM =
{0x2bc49361, 0x8327, 0x11cf, {0xac, 0x4a, 0x0, 0x0, 0xc0, 0x38, 0x25, 0xa1 } };
static const IID IID_IDXDupDirect3DRM3 =
{0x4516ec83, 0x8f20, 0x11d0, {0x9b, 0x6d, 0x00, 0x00, 0xc0, 0x78, 0x1b, 0xc3 } };
static const IID IID_IDXDupDirect3DRMMeshBuilder3 =
{ 0x4516ec82, 0x8f20, 0x11d0, { 0x9b, 0x6d, 0x00, 0x00, 0xc0, 0x78, 0x1b, 0xc3} };
HRESULT CDXDataPtr::Assign(BOOL bMesh, IUnknown * pObject, IDXSurfaceFactory *pSurfaceFactory)
{
HRESULT hr = S_OK;
if (pObject)
{
IUnknown *pNative = NULL;
if (!bMesh)
{
//--- Try to get a DX surface
hr = pObject->QueryInterface( IID_IDXSurface, (void **)&pNative );
if( FAILED( hr ) )
{
IDirectDrawSurface *pSurf;
//--- Try to get a DDraw surface
hr = pObject->QueryInterface( IID_IDXDupDDrawSurface, (void **)&pSurf );
if( SUCCEEDED( hr ) )
{
//--- Create a DXSurface from the DDraw surface
hr = pSurfaceFactory->CreateFromDDSurface(
pSurf, NULL, 0, NULL, IID_IDXSurface,
(void **)&pNative );
pSurf->Release();
}
}
}
else // Must be a mesh builder
{
hr = pObject->QueryInterface(IID_IDXDupDirect3DRMMeshBuilder3, (void **)&pNative);
}
if (SUCCEEDED(hr))
{
Release();
m_pNativeInterface = pNative;
pObject->AddRef();
m_pUnkOriginalObject = pObject;
if (SUCCEEDED(pNative->QueryInterface(IID_IDXBaseObject, (void **)&m_pBaseObj)))
{
m_pBaseObj->GetGenerationId(&m_dwLastDirtyGenId);
m_dwLastDirtyGenId--;
}
if (!bMesh)
{
((IDXSurface *)pNative)->GetPixelFormat(NULL, &m_SampleFormat);
}
}
else
{
if (hr == E_NOINTERFACE)
{
hr = E_INVALIDARG;
}
}
}
else
{
Release();
}
return hr;
} /* CDXDataPtr::Assign */
bool CDXDataPtr::IsDirty(void)
{
if (m_pBaseObj)
{
DWORD dwOldId = m_dwLastDirtyGenId;
m_pBaseObj->GetGenerationId(&m_dwLastDirtyGenId);
return dwOldId != m_dwLastDirtyGenId;
}
else
{
return false;
}
}
DWORD CDXDataPtr::GenerationId(void)
{
if (m_pBaseObj)
{
DWORD dwGenId;
m_pBaseObj->GetGenerationId(&dwGenId);
return dwGenId;
}
else
{
return 0;
}
}
bool CDXDataPtr::UpdateGenerationId(void)
{
if (m_pBaseObj)
{
DWORD dwOldId = m_dwLastUpdGenId;
m_pBaseObj->GetGenerationId(&m_dwLastUpdGenId);
return dwOldId != m_dwLastUpdGenId;
}
else
{
return false;
}
} /* CDXDataPtr::UpdateGenerationId */
ULONG CDXDataPtr::ObjectSize(void)
{
ULONG ulSize = 0;
if (m_pBaseObj)
{
m_pBaseObj->GetObjectSize(&ulSize);
}
return ulSize;
}
/*****************************************************************************
* CDXBaseNTo1::CDXBaseNTo1 *
*--------------------------*
* Description:
* Constructor
*-----------------------------------------------------------------------------
* Created By: Ed Connell Date: 07/28/97
*-----------------------------------------------------------------------------
* Parameters:
*****************************************************************************/
CDXBaseNTo1::CDXBaseNTo1() :
m_aInputs(NULL),
m_ulNumInputs(0),
m_ulNumProcessors(1), // Default to one until task manager is set
m_dwGenerationId(1),
m_dwCleanGenId(0),
m_Duration(1.0f),
m_StepResolution(0.0f),
m_Progress(0.0f),
m_dwBltFlags(0),
m_bPickDoneByBase(false),
m_bInMultiThreadWorkProc(FALSE),
m_fQuality(0.5f), // Default to normal quality.
// Wait forever before timing out on a lock by default
m_ulLockTimeOut(INFINITE),
//
// Override these flags if your object does not support one or more of these options.
// Typically, 3-D effects should set this member to 0.
//
m_dwMiscFlags(DXTMF_BLEND_WITH_OUTPUT | DXTMF_DITHER_OUTPUT |
DXTMF_BLEND_SUPPORTED | DXTMF_DITHER_SUPPORTED | DXTMF_BOUNDS_SUPPORTED | DXTMF_PLACEMENT_SUPPORTED),
//
// If your object has a different number of objects or a different number of
// required objects than 1, simply set these members in the body of your
// constructor or in FinalConstruct(). For every input that is > the number
// required, that input will be reported as optional.
//
// If your transform takes 2 required inputs, set both to 2.
// If your transform takes 2 optional inputs, set MaxInputs = 2, NumInRequired = 0
// If your transform takes 1 required and 2 optional inputs,
// set MaxInputs = 2, NumInRequired = 1
//
// For more complex combinations of optinal/required, you will need to override
// the OnSetup method of this base class, and override the methods
// GetInOutInfo
//
m_ulMaxInputs(1),
m_ulNumInRequired(1),
//
// If the intputs or output types are not surfaces then set appropriate object type
//
m_dwOptionFlags(0), // Inputs and output are surfaces, don't have to be the same size
m_ulMaxImageBands(DXB_MAX_IMAGE_BANDS)
{
DXTDBG_FUNC( "CDXBaseNTo1::CDXBaseNTo1" );
//
// Set event handles to NULL.
//
memset(m_aEvent, 0, sizeof(m_aEvent));
} /* CDXBaseNTo1::CDXBaseNTo1 */
/*****************************************************************************
* CDXBaseNTo1::~CDXBaseNTo1 *
*---------------------------*
* Description:
* Constructor
*-----------------------------------------------------------------------------
* Created By: Ed Connell Date: 07/28/97
*-----------------------------------------------------------------------------
* Parameters:
*****************************************************************************/
CDXBaseNTo1::~CDXBaseNTo1()
{
DXTDBG_FUNC( "CDXBaseNTo1::~CDXBaseNTo1" );
_ReleaseReferences();
delete[] m_aInputs;
//--- Release event objects
for(ULONG i = 0; i < DXB_MAX_IMAGE_BANDS; ++i )
{
if( m_aEvent[i] ) ::CloseHandle( m_aEvent[i] );
}
} /* CDXBaseNTo1::~CDXBaseNTo1 */
没有合适的资源?快使用搜索试试~ 我知道了~
BCB6(BorlandC++Builder6.0编译器)
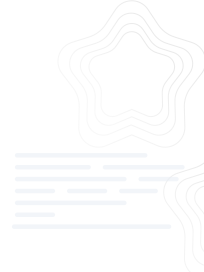
共1725个文件
h:1372个
lib:185个
inl:23个


温馨提示
Borland C++Builder 6为开发人员创建支持新兴Web服务的高效应用提供了一个稳固,高效率的电子商务开发环境.BizSnap Web服务开发平台能使开发人员轻松地创建业界标准SOAP/XML Web服务和连接,从而简化了企业到企业集成.开发人员可以利用基于组件的Web应用开发平台WebSnap提高开发过程的功能,速度和效率,利用DataSnap建立与许多商务进程和许多业务伙伴集成的强大数据存取中间件解决方案.
资源推荐
资源详情
资源评论
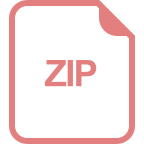
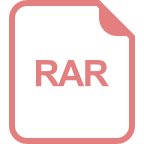
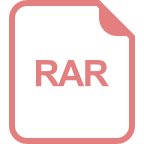
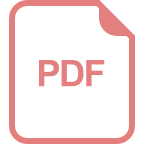
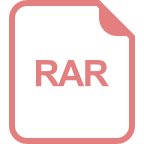
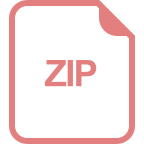
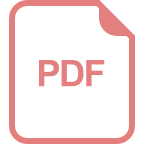
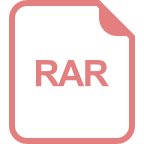
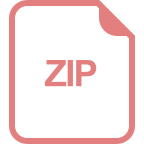
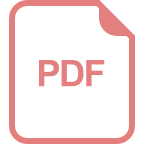
收起资源包目录

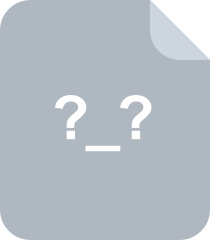
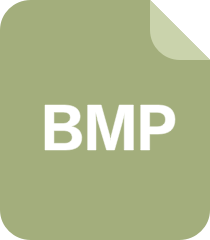
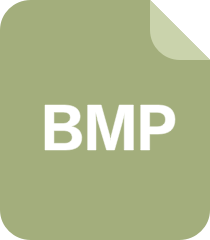
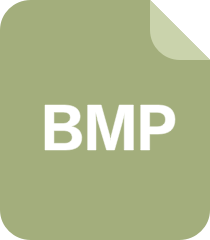
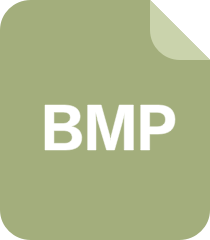
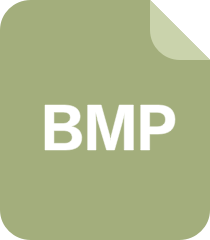
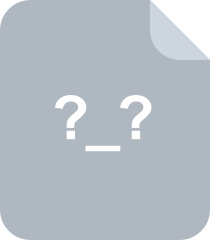
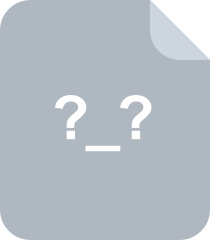
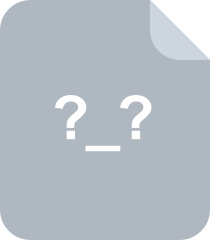
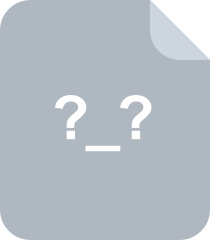
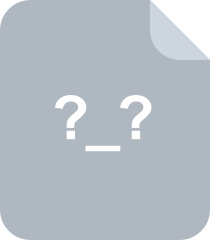
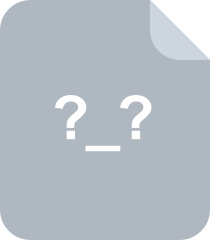
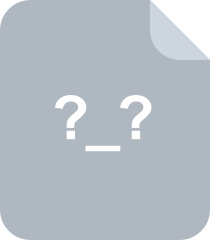
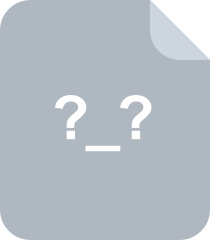
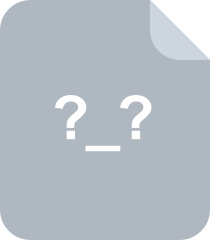
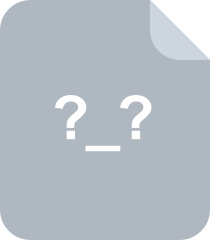
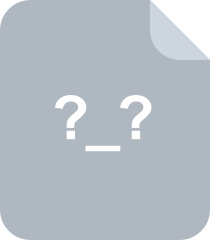
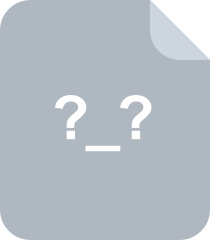
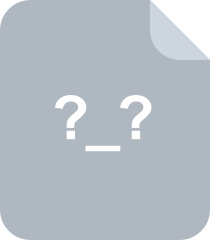
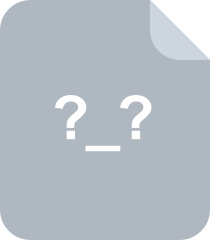
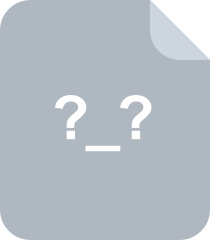
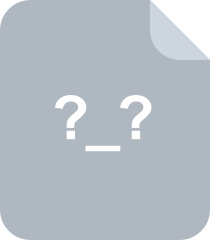
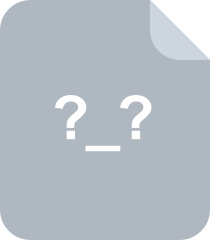
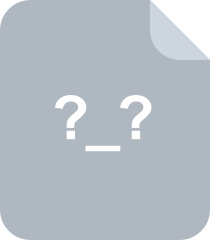
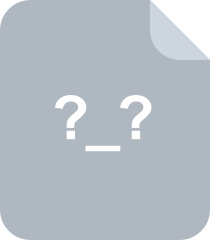
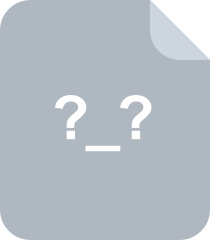
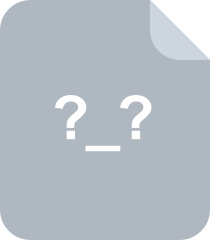
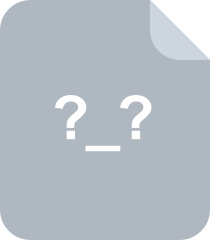
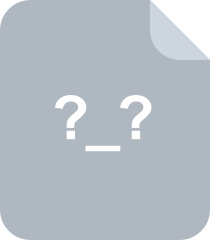
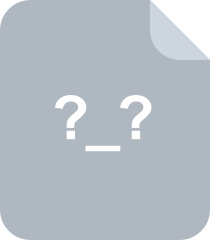
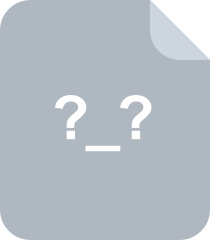
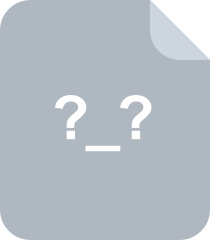
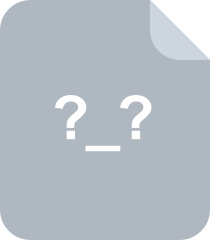
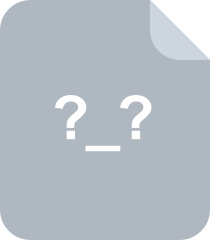
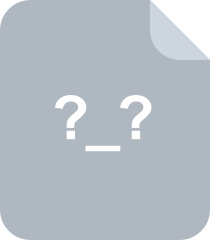
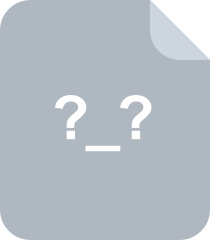
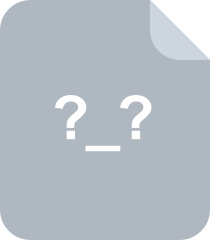
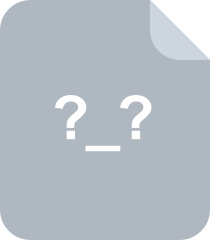
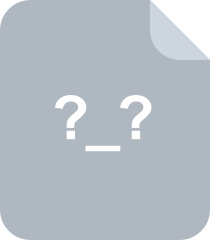
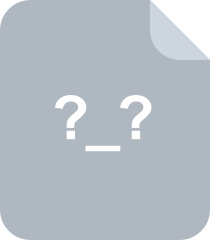
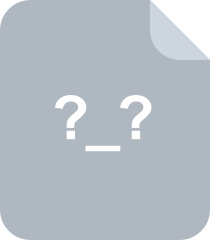
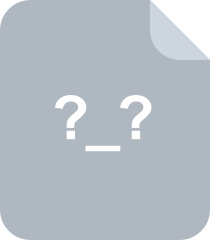
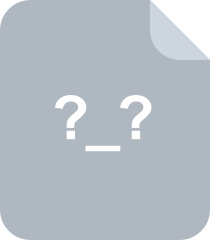
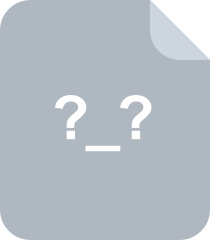
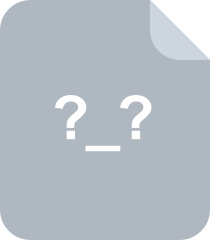
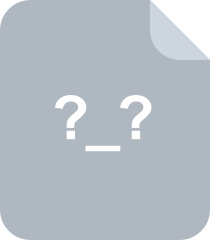
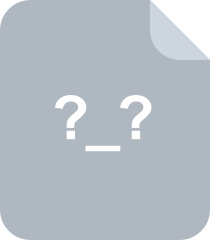
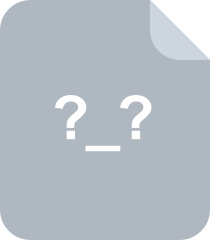
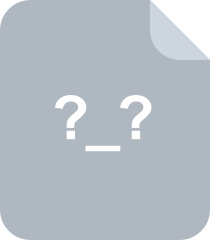
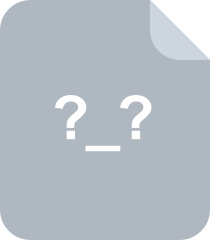
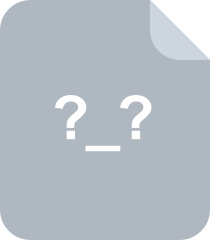
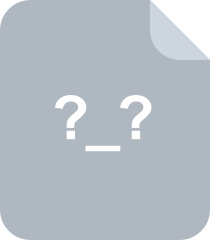
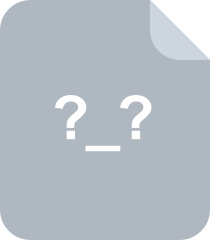
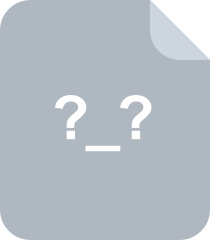
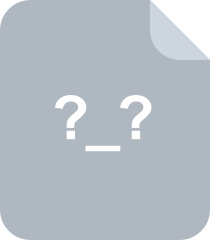
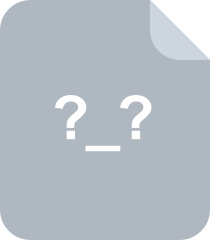
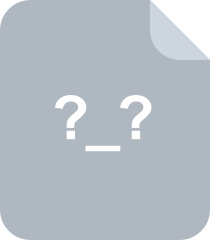
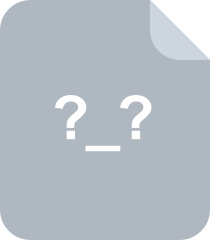
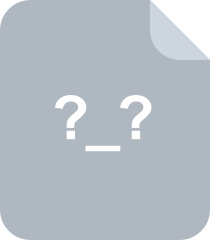
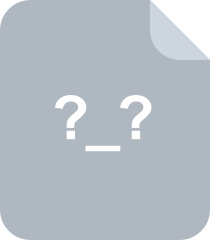
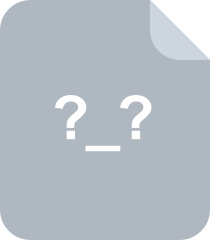
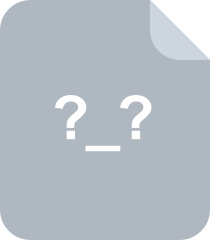
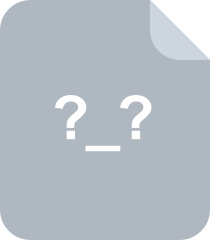
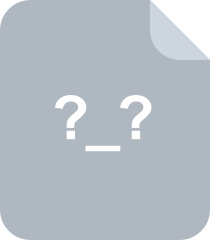
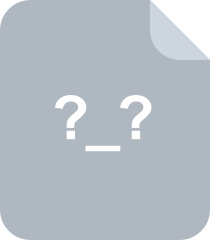
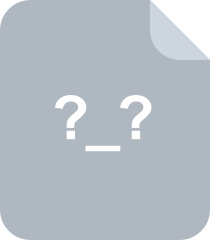
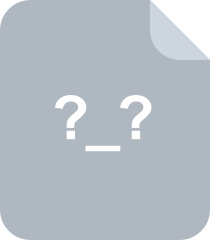
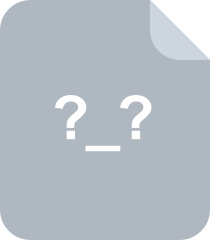
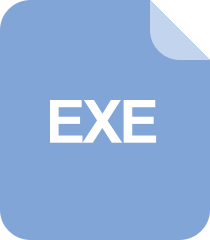
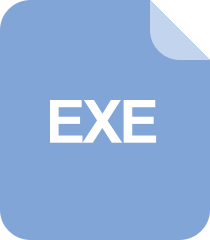
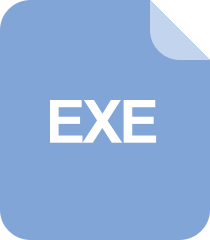
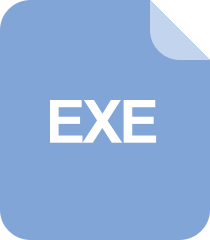
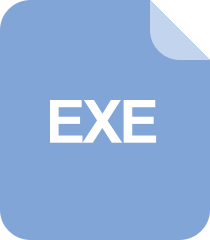
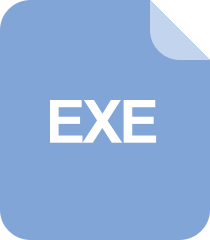
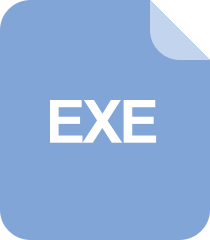
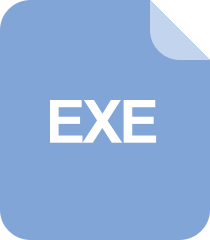
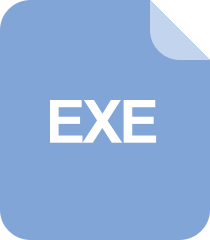
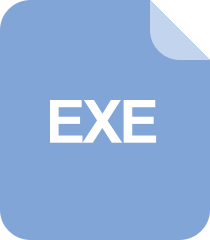
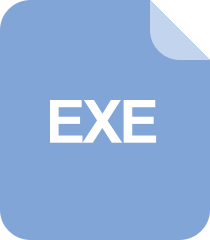
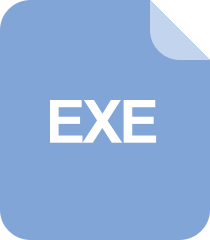
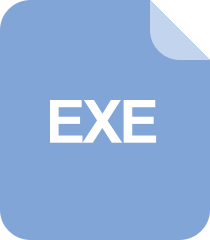
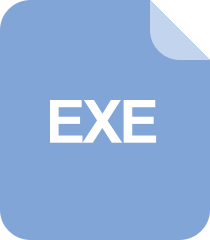
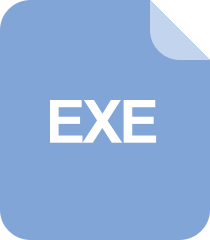
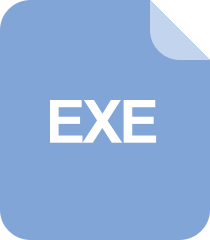
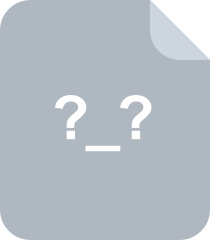
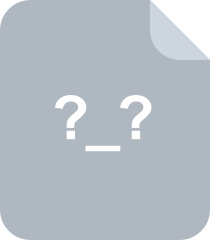
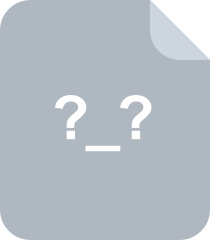
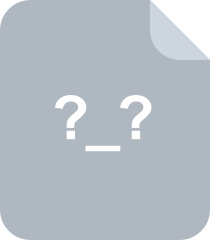
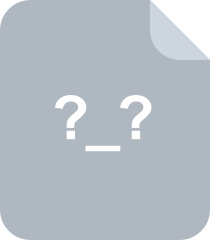
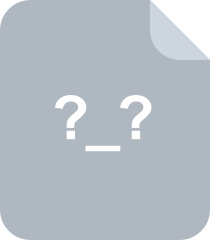
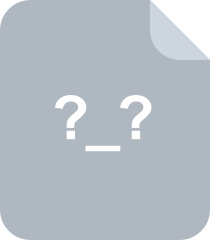
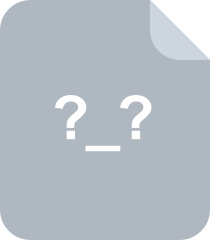
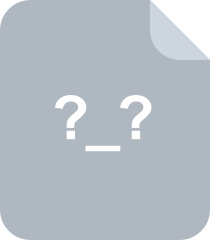
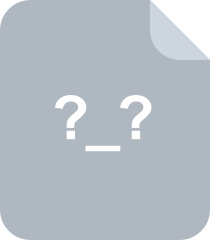
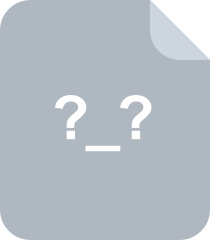
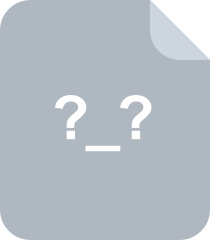
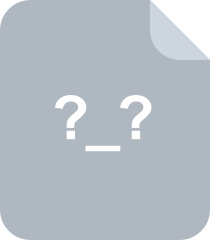
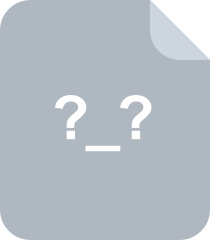
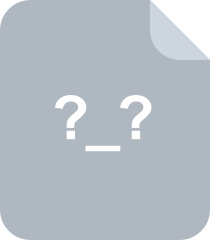
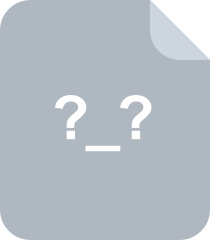
共 1725 条
- 1
- 2
- 3
- 4
- 5
- 6
- 18

烨炜带火
- 粉丝: 25
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

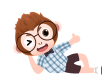
最新资源
- C# winform置托盘图标并闪烁演示源码.zip
- 打包和分发Rust工具.pdf
- SQL中的CREATE LOGFILE GROUP 语句.pdf
- C语言-leetcode题解之第172题阶乘后的零.zip
- C语言-leetcode题解之第171题Excel列表序号.zip
- C语言-leetcode题解之第169题多数元素.zip
- ocr-图像识别资源ocr-图像识别资源
- 图像识别:基于Resnet50 + VGG16模型融合的人体细胞癌症分类模型实现-图像识别资源
- C语言-leetcode题解之第168题Excel列表名称.zip
- C语言-leetcode题解之第167题两数之和II-输入有序数组.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


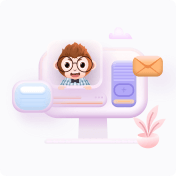
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页