package cn.kfu.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import cn.kfu.entity.Student;
import cn.kfu.util.DBUtil;
public class StudentDAO implements BaseDAO<String, Student> {
private Connection conn;
private Statement stmt;
private PreparedStatement pStmt;
private ResultSet rs;
public int delete(Student obj) {
int result = 0;
String sql = "delete from 学生基本信息表 where 学号=?";
conn = DBUtil.getConnection();
boolean autoCommit = false;
try {
autoCommit = conn.getAutoCommit();
DBUtil.setAutoCommit(conn, false);
pStmt = conn.prepareStatement(sql);
pStmt.setString(1, obj.getStID());
result = pStmt.executeUpdate();
conn.commit();
DBUtil.setAutoCommit(conn, autoCommit);
} catch (SQLException e) {
e.printStackTrace();
}
finally{
try {
if(rs != null){
rs.close();
}
if(stmt != null){
stmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
DBUtil.close();
return result;
}//end method
public Student getObject(String key) {
String sql = "select * from 学生基本信息表 where 学号='" + key +"'";
conn = DBUtil.getConnection();
try {
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
while (rs.next()) {
Student st = new Student();
st.setStID(rs.getString("学号"));
st.setStName(rs.getString("姓名"));
st.setStSex(rs.getString("性别"));
st.setStAge(rs.getInt("年龄"));
st.setStBelief(rs.getString("政治面貌"));
st.setStTeam(rs.getInt("班级"));
st.setStAddress(rs.getString("地址"));
st.setStEamil(rs.getString("Email"));
st.setStPhone(rs.getString("电话"));
st.setStReamark(rs.getString("备注"));
return st;
}
} catch (SQLException e) {
e.printStackTrace();
}
finally{
try {
if(rs != null){
rs.close();
}
if(stmt != null){
stmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
DBUtil.close();
return null;
}//end method
public List<Student> getObjectsAll() {
ArrayList<Student> listStudent = new ArrayList<Student>();
String sql = "select * from 学生基本信息表";
conn = DBUtil.getConnection();
try {
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
while (rs.next()) {
Student st = new Student();
st.setStID(rs.getString("学号"));
st.setStName(rs.getString("姓名"));
st.setStSex(rs.getString("性别"));
st.setStAge(rs.getInt("年龄"));
st.setStBelief(rs.getString("政治面貌"));
st.setStTeam(rs.getInt("班级"));
st.setStAddress(rs.getString("地址"));
st.setStEamil(rs.getString("Email"));
st.setStPhone(rs.getString("电话"));
st.setStReamark(rs.getString("备注"));
listStudent.add(st);
}
return listStudent;
} catch (SQLException e) {
e.printStackTrace();
}
finally{
try {
if(rs != null){
rs.close();
}
if(stmt != null){
stmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
DBUtil.close();
return null;
}//end method
public List<Student> getObjectsAll(String start, String end) {
ArrayList<Student> listStudent = new ArrayList<Student>();
String sql = "select * from 学生基本信息表 where 学号 >= '" + start + "' and 学号 <= '" + end + "'";
conn = DBUtil.getConnection();
try {
stmt = conn.createStatement();
rs = stmt.executeQuery(sql);
while (rs.next()) {
Student st = new Student();
st.setStID(rs.getString("学号"));
st.setStName(rs.getString("姓名"));
st.setStSex(rs.getString("性别"));
st.setStAge(rs.getInt("年龄"));
st.setStBelief(rs.getString("政治面貌"));
st.setStTeam(rs.getInt("班级"));
st.setStAddress(rs.getString("地址"));
st.setStEamil(rs.getString("Email"));
st.setStPhone(rs.getString("电话"));
st.setStReamark(rs.getString("备注"));
listStudent.add(st);
}
return listStudent;
} catch (SQLException e) {
e.printStackTrace();
}
finally{
try {
if(rs != null){
rs.close();
}
if(stmt != null){
stmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
DBUtil.close();
return null;
}
public int save(Student obj) {
int result = 0;
String sql = "insert into 学生基本信息表(学号,姓名,性别,年龄,政治面貌,班级,地址,Email,电话,备注) values(?,?,?,?,?,?,?,?,?,?)";
conn = DBUtil.getConnection();
boolean autoCommit = false;
try {
autoCommit = conn.getAutoCommit();
DBUtil.setAutoCommit(conn, false);
pStmt = conn.prepareStatement(sql);
pStmt.setString(1, obj.getStID());
pStmt.setString(2, obj.getStName());
pStmt.setString(3, obj.getStSex());
pStmt.setInt(4, obj.getStAge());
pStmt.setString(5,obj.getStBelief());
pStmt.setInt(6, obj.getStTeam());
pStmt.setString(7, obj.getStAddress());
pStmt.setString(8, obj.getStEamil());
pStmt.setString(9, obj.getStPhone());
pStmt.setString(10, obj.getStReamark());
result = pStmt.executeUpdate();
conn.commit();
DBUtil.setAutoCommit(conn, autoCommit);
} catch (SQLException e) {
e.printStackTrace();
}
finally{
try {
if(rs != null){
rs.close();
}
if(stmt != null){
stmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
DBUtil.close();
return result;
}
public int update(Student obj) {
int result = 0;
String sql = "update 学生基本信息表 set 学号=?,姓名=?,性别=?,年龄=?,政治面貌=?,班级=?,地址=?,Email=?,电话=?,备注=? where 学号=?";
conn = DBUtil.getConnection();
boolean autoCommit = false;
try {
autoCommit = conn.getAutoCommit();
DBUtil.setAutoCommit(conn, false);
pStmt = conn.prepareStatement(sql);
pStmt.setString(1, obj.getStID());
pStmt.setString(2, obj.getStName());
pStmt.setString(3, obj.getStSex());
pStmt.setInt(4, obj.getStAge());
pStmt.setString(5,obj.getStBelief());
pStmt.setInt(6, obj.getStTeam());
pStmt.setString(7, obj.getStAddress());
pStmt.setString(8, obj.getStEamil());
pStmt.setString(9, obj.getStPhone());
pStmt.setString(10, obj.getStReamark());
pStmt.setString(11, obj.getStID());
result = pStmt.executeUpdate();
conn.commit();
DBUtil.setAutoCommit(conn, autoCommit);
} catch (SQLException e) {
e.printStackTrace();
}
finally{
try {
if(rs != null){
rs.close();
}
if(stmt != null){
stmt.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
DBUtil.close();
return result;
}
}//end StudentDAO
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
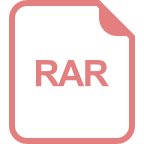
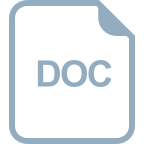
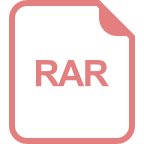
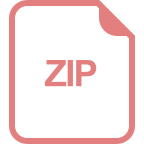
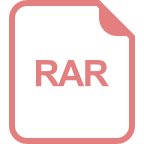
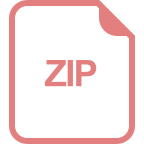
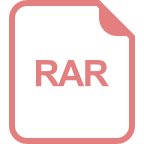
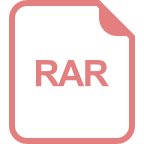
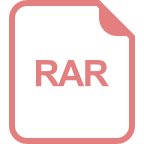
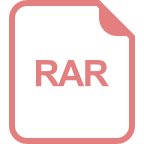
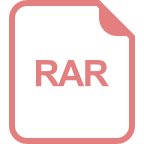
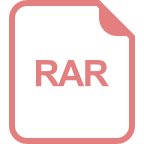
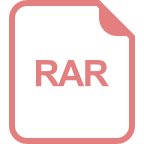
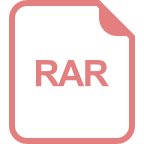
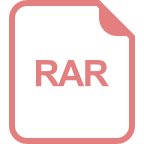
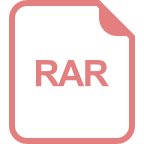
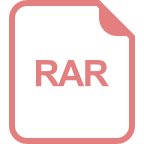
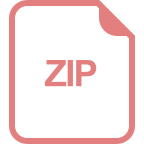
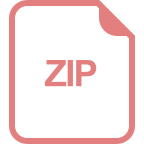
收起资源包目录


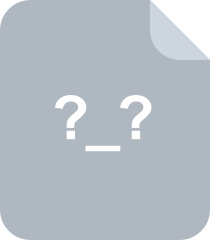

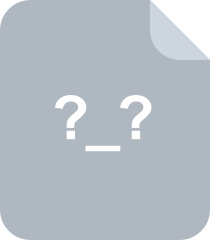
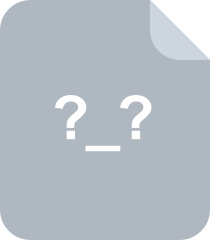


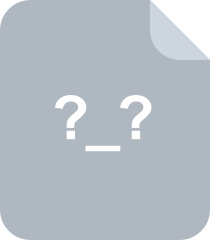

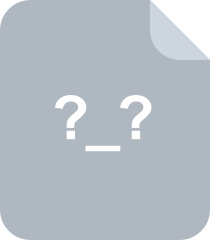
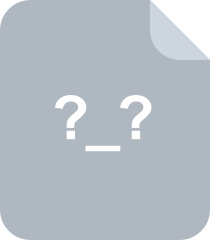

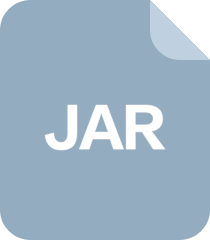
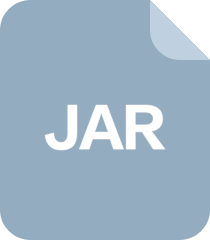
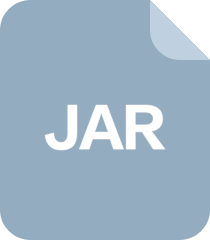




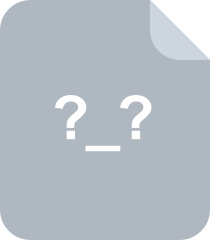
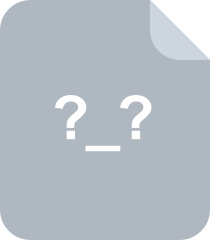
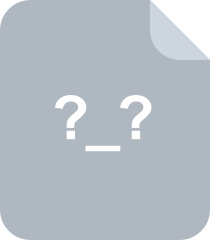
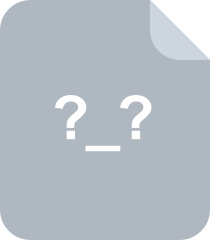

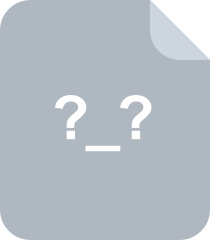

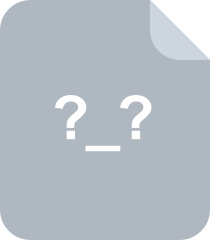
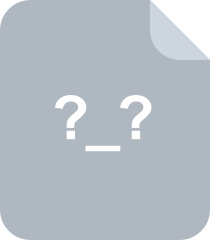
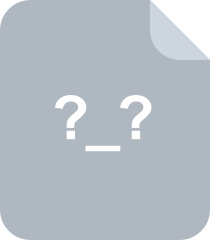
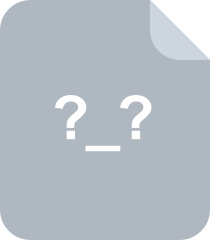
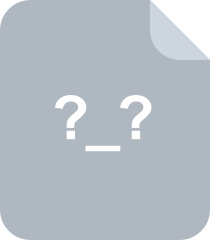
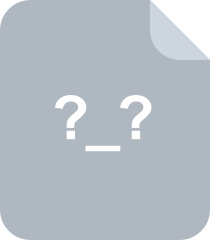
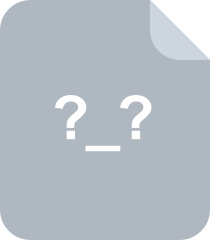

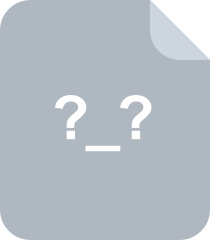
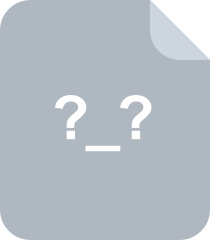

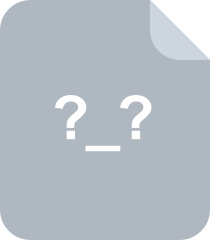

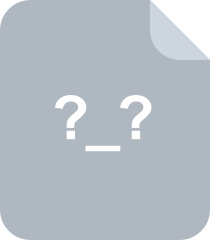

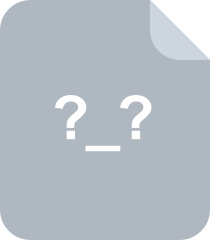
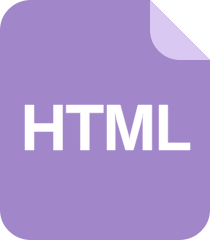





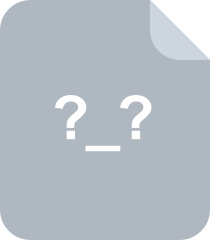
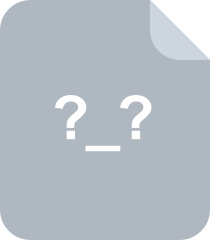
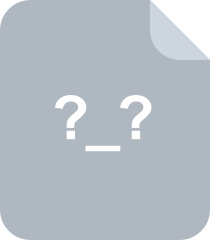
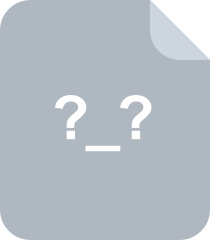

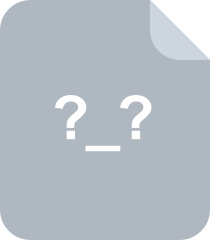

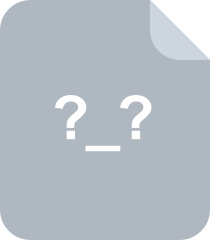
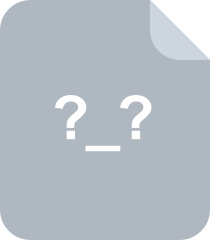
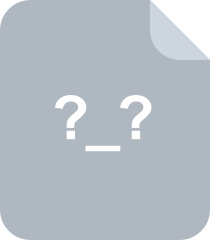
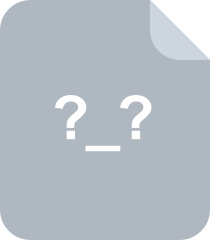
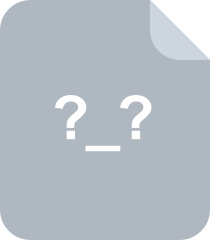
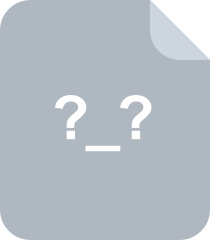
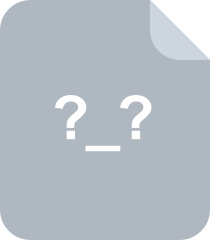

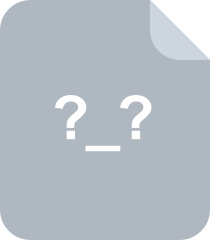
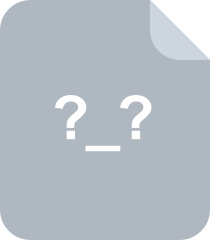

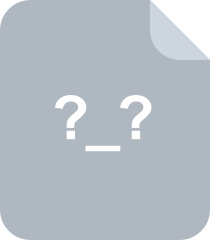
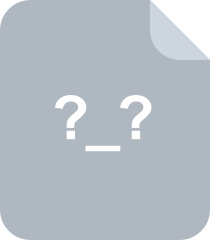
共 43 条
- 1
资源评论
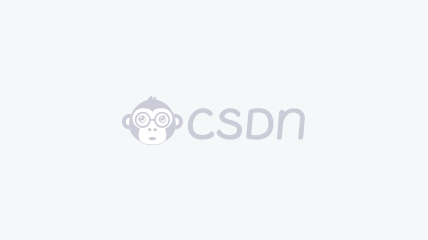
- zhaohpo2014-09-19很不错的资源,学习一下!
- yujian52o2014-01-17虽然还自己写的 但是能用不错
- wingsi2014-05-27不知道为什么不能运行
- ccut200917672013-09-21挺好的,能用

gelei1978
- 粉丝: 8
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

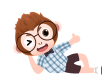
安全验证
文档复制为VIP权益,开通VIP直接复制
