package com.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.YuangonggongziEntity;
import com.entity.view.YuangonggongziView;
import com.service.YuangonggongziService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MD5Util;
import com.utils.MPUtil;
import com.utils.CommonUtil;
import java.io.IOException;
/**
* 员工工资
* 后端接口
* @author
* @email
* @date 2022-04-02 16:46:23
*/
@RestController
@RequestMapping("/yuangonggongzi")
public class YuangonggongziController {
@Autowired
private YuangonggongziService yuangonggongziService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YuangonggongziEntity yuangonggongzi,
HttpServletRequest request){
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("yuangong")) {
yuangonggongzi.setYuangonggonghao((String)request.getSession().getAttribute("username"));
}
EntityWrapper<YuangonggongziEntity> ew = new EntityWrapper<YuangonggongziEntity>();
PageUtils page = yuangonggongziService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yuangonggongzi), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YuangonggongziEntity yuangonggongzi,
HttpServletRequest request){
EntityWrapper<YuangonggongziEntity> ew = new EntityWrapper<YuangonggongziEntity>();
PageUtils page = yuangonggongziService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yuangonggongzi), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( YuangonggongziEntity yuangonggongzi){
EntityWrapper<YuangonggongziEntity> ew = new EntityWrapper<YuangonggongziEntity>();
ew.allEq(MPUtil.allEQMapPre( yuangonggongzi, "yuangonggongzi"));
return R.ok().put("data", yuangonggongziService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(YuangonggongziEntity yuangonggongzi){
EntityWrapper< YuangonggongziEntity> ew = new EntityWrapper< YuangonggongziEntity>();
ew.allEq(MPUtil.allEQMapPre( yuangonggongzi, "yuangonggongzi"));
YuangonggongziView yuangonggongziView = yuangonggongziService.selectView(ew);
return R.ok("查询员工工资成功").put("data", yuangonggongziView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
YuangonggongziEntity yuangonggongzi = yuangonggongziService.selectById(id);
return R.ok().put("data", yuangonggongzi);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
YuangonggongziEntity yuangonggongzi = yuangonggongziService.selectById(id);
return R.ok().put("data", yuangonggongzi);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YuangonggongziEntity yuangonggongzi, HttpServletRequest request){
yuangonggongzi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yuangonggongzi);
yuangonggongziService.insert(yuangonggongzi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody YuangonggongziEntity yuangonggongzi, HttpServletRequest request){
yuangonggongzi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yuangonggongzi);
yuangonggongziService.insert(yuangonggongzi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody YuangonggongziEntity yuangonggongzi, HttpServletRequest request){
//ValidatorUtils.validateEntity(yuangonggongzi);
yuangonggongziService.updateById(yuangonggongzi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
yuangonggongziService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
Wrapper<YuangonggongziEntity> wrapper = new EntityWrapper<YuangonggongziEntity>();
if(map.get("remindstart")!=null) {
wrapper.ge(columnName, map.get("remindstart"));
}
if(map.get("remindend")!=null) {
wrapper.le(columnName, map.get("remindend"));
}
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("yuangong")) {
wrapper.eq("yuangonggonghao", (String)request.getSession().getAttribute("username"));
}
int count = yuangonggongziService.selectCount(wrapper);
return R.ok().put("count", count);
}
/**
* (按值统计)
*/
@RequestMapping("/value/{xColumnName}/{yColumnName}")
public R value(@PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnName,HttpServletRequest request) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("xColumn", xColumnName);
params.put("yColumn", yColumnName);
EntityWrapper<YuangonggongziEntity> ew = new EntityWrapper<YuangonggongziEntity>();
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("yuangong")) {
ew.eq("yuangonggonghao", (String)request.getSession().getAttribute("usernam
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
SpringBoot ,Java, Vue 毕业设计,基于 SpringBoot 开发的,含有代码注释,新手也可看懂。毕业设计、期末大作业、课程设计、高分必看,已获高分通过项目。 包含:项目源码、数据库脚本、软件工具、项目说明等,该项目可以作为毕设、课程设计使用。 该系统功能完善、界面美观、操作简单、功能齐全、管理便捷,具有很高的实际应用价值。 里面有部署教程,项目都经过严格调试,确保可以运行! 1. 技术组成 后台框架:SpringBoot 前端:Vue 数据库:MySQL Maven 开发环境:JDK、IDEA、Tomcat 2. 部署教程 https://blog.junxu666.top/p/49037.html 如果需要指导,也可以私信联系我
资源推荐
资源详情
资源评论
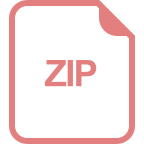
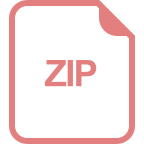
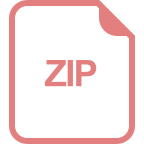
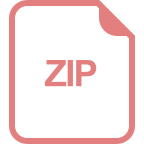
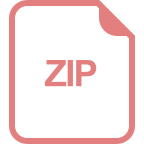
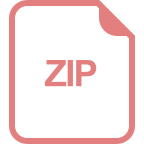
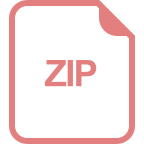
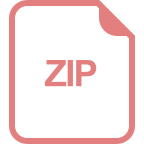
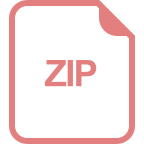
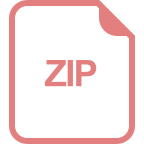
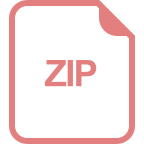
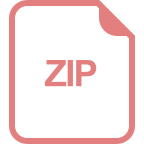
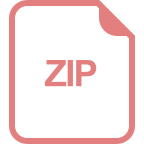
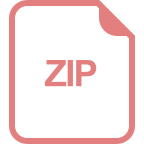
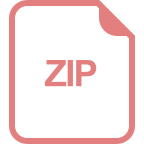
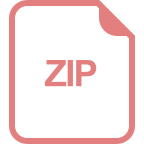
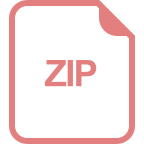
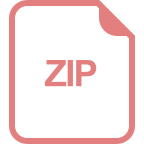
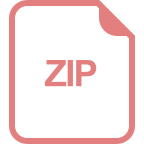
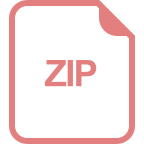
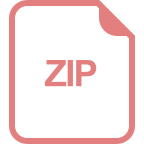
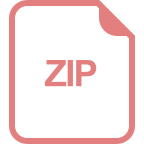
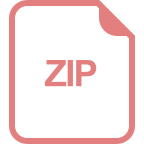
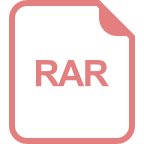
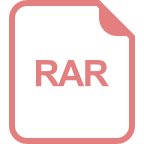
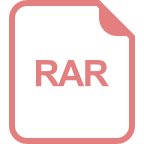
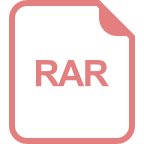
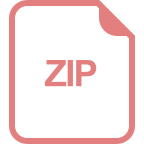
收起资源包目录

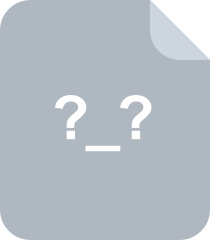
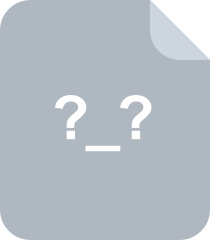
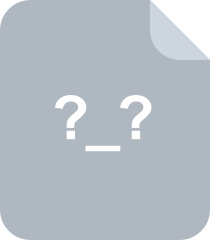
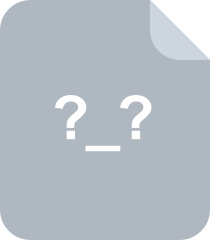
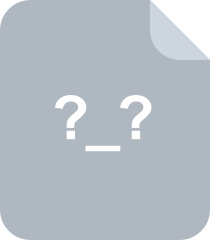
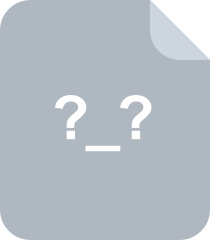
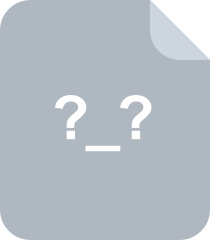
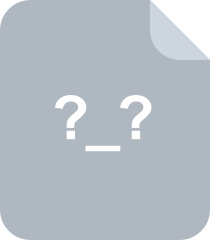
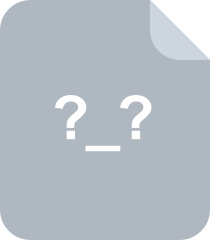
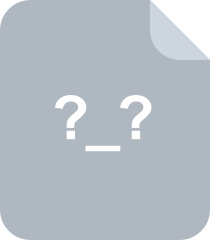
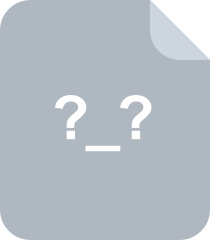
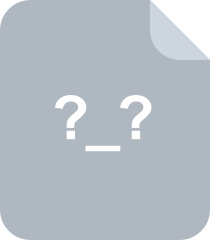
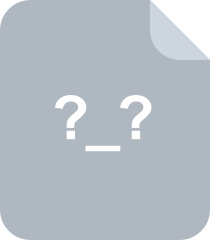
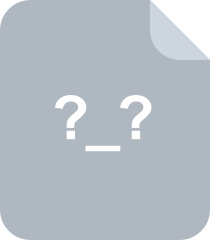
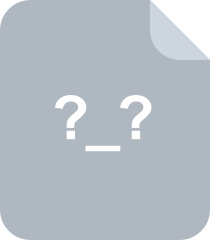
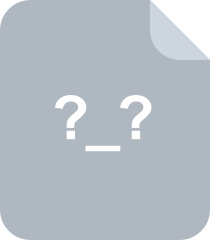
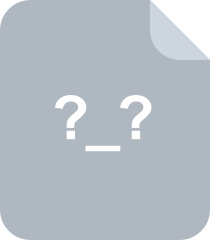
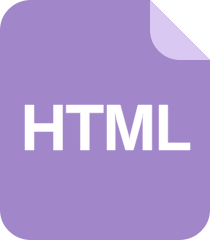
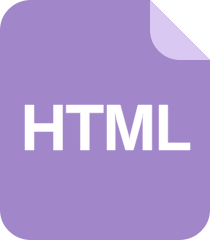
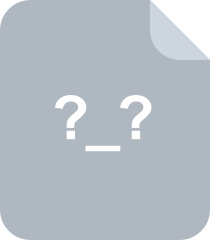
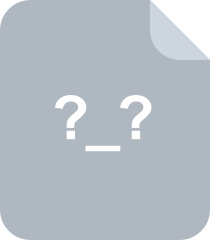
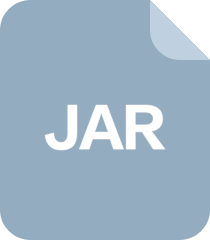
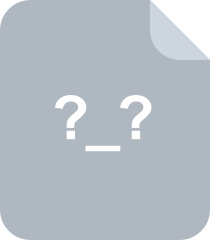
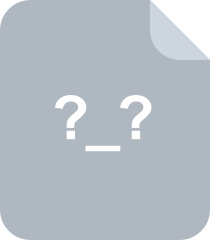
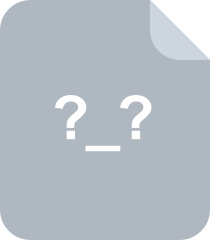
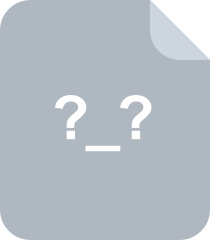
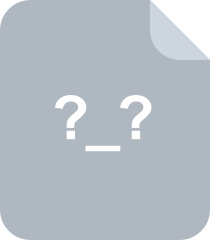
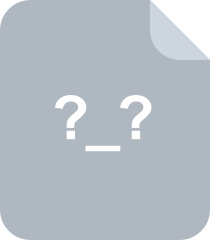
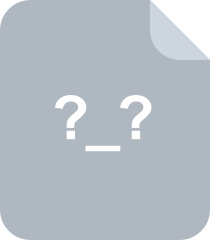
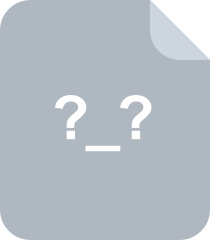
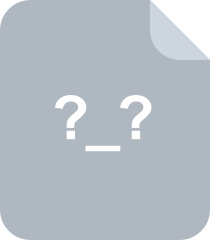
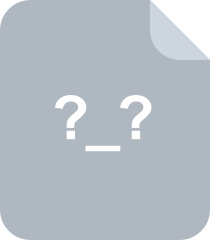
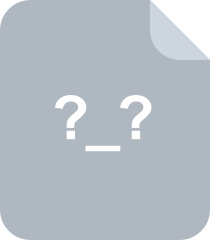
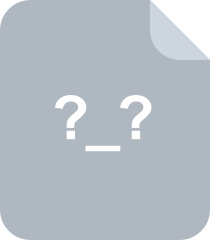
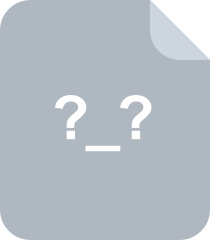
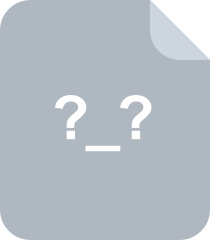
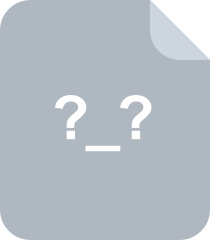
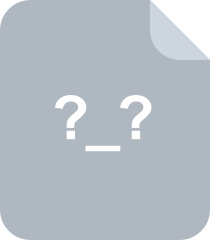
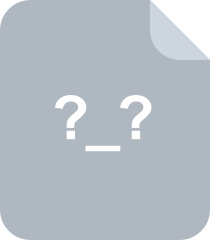
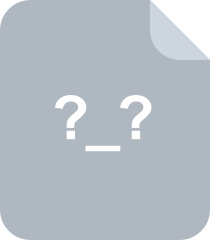
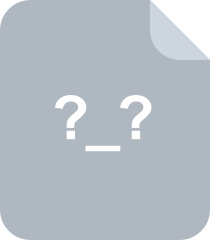
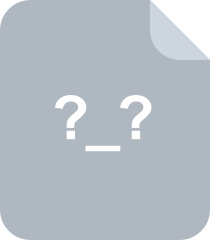
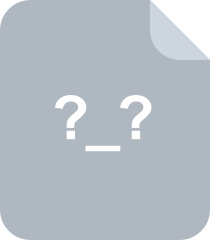
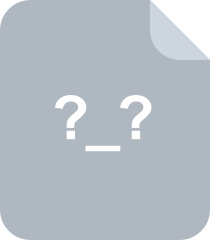
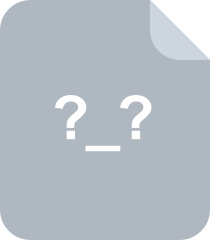
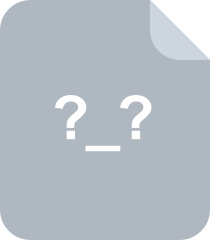
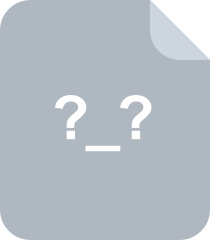
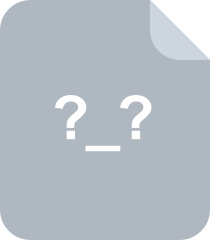
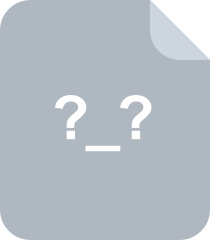
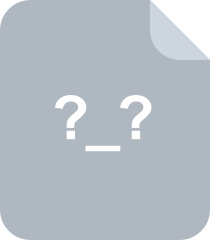
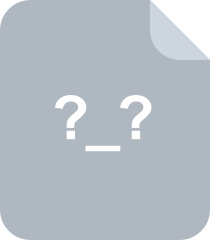
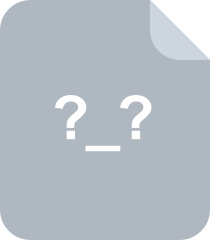
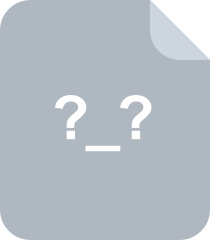
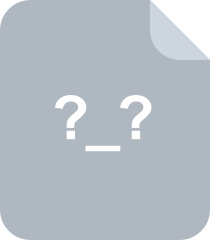
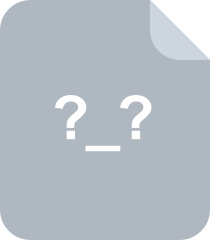
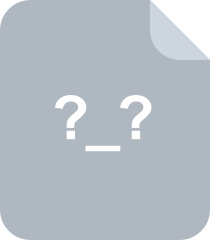
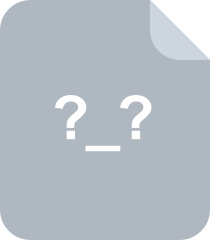
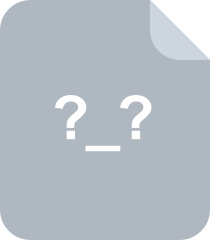
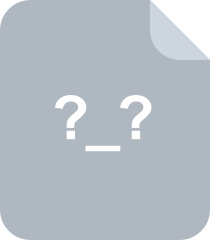
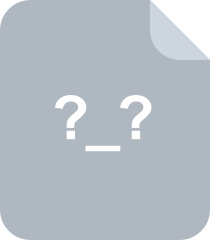
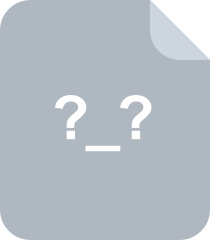
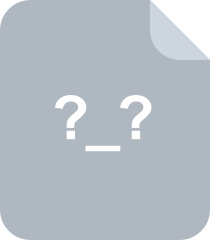
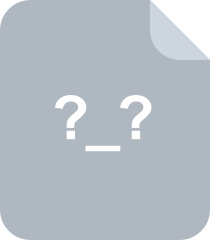
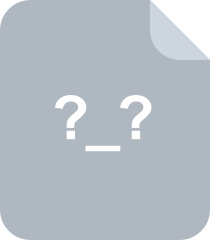
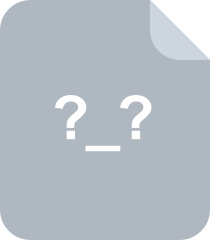
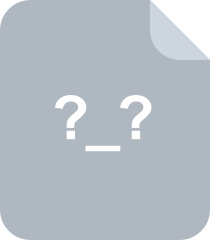
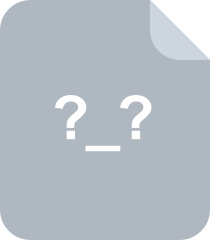
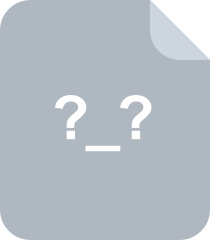
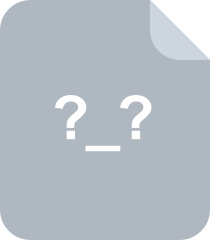
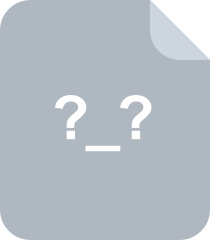
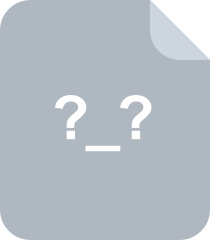
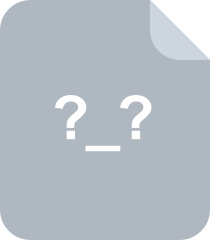
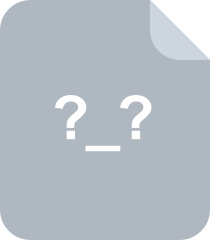
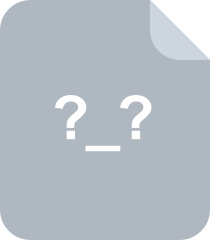
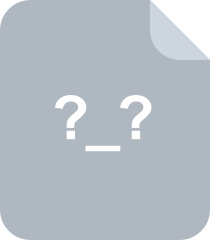
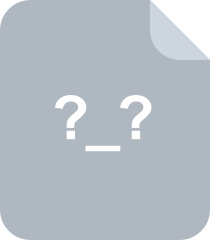
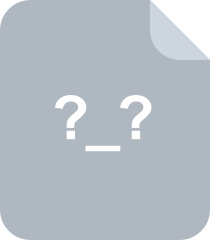
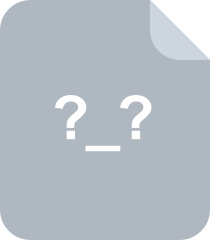
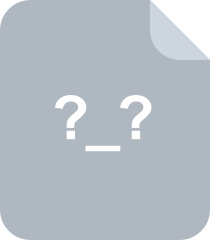
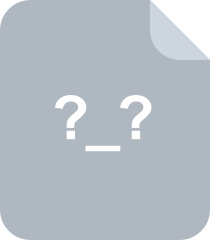
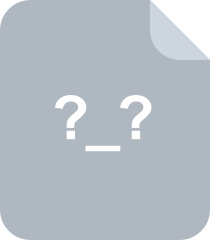
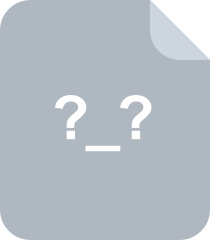
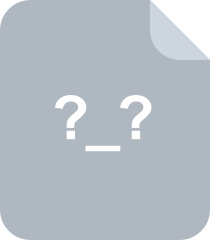
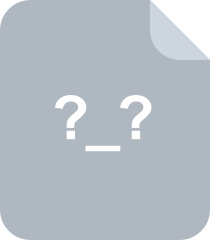
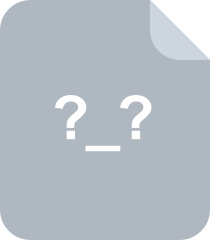
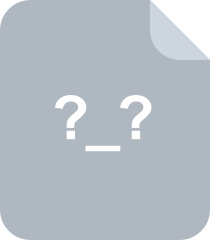
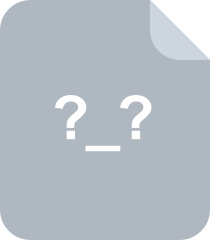
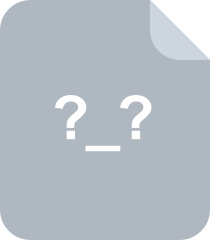
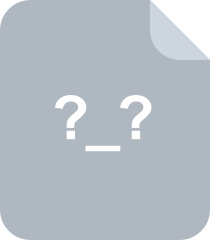
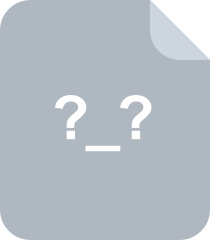
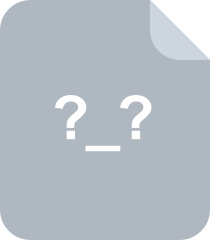
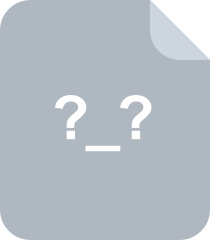
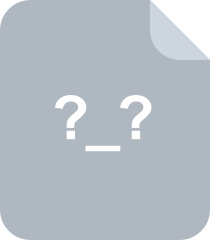
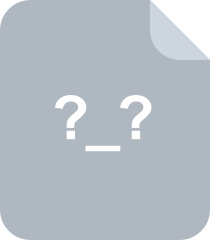
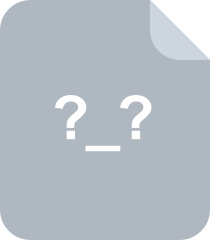
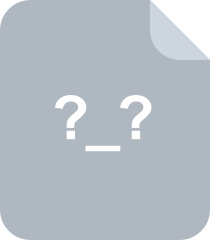
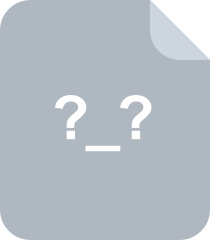
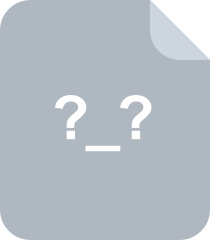
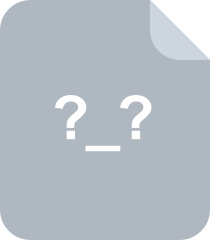
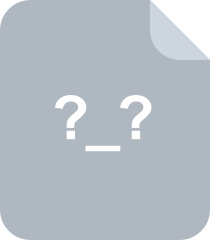
共 447 条
- 1
- 2
- 3
- 4
- 5
资源评论
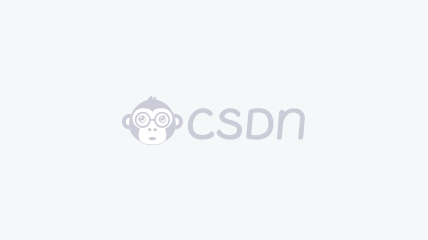
- wjw_202023-08-22感谢资源主的分享,很值得参考学习,资源价值较高,支持!

gdutxiaoxu
- 粉丝: 1540
- 资源: 3119
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

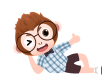
最新资源
- 【答题卡识别】hough变换答题卡判定与成绩统计【含GUI Matlab仿真 752期】.zip
- 【火灾检测】火灾检测【含GUI Matlab仿真 249期】.zip
- 【人脸表情识别】CNN人脸表情识别【含GUI Matlab仿真 787期】.zip
- 【手势识别】SIFT+SVM算法手势识别【含GUI Matlab仿真 1789期】.zip
- 【缺陷检测】形态学PCB电路板缺陷检测【含GUI Matlab仿真 821期】.zip
- 【水果识别】苹果质量检测及分级系统【含GUI Matlab仿真 896期】.zip
- 【条形码识别】二维条形码识别【含GUI Matlab仿真 607期】.zip
- 【手写数字识别】贝叶斯+线性分类器手写数字识别【含GUI Matlab仿真 828期】.zip
- 【图像配准】Brox+sift图像配准(含相关性)【含GUI Matlab仿真 747期】.zip
- 【图像分割】遗传算法Otsu图像分割【含GUI Matlab仿真 734期】.zip
- 【图像加密】正交拉丁方置乱算法图像加解密【含GUI Matlab仿真 182期】.zip
- 【图像去噪】加权+绝对差分中值滤波图像去噪(含PNSR)【含GUI Matlab仿真 1880期】.zip
- 【图像融合】增强随机游走算法多焦点图像融合【含Matlab仿真 1975期】.zip
- 【图像隐写】DWT+DCT+LSB数字水印隐藏提取比较【含Matlab仿真 1623期】.zip
- 【图像隐写】LSB匹配图像隐写【含GUI Matlab仿真 812期】.zip
- 【表盘识别】Hough变换钟表表盘识别【含Matlab仿真 1069期】.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


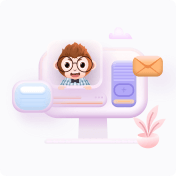
安全验证
文档复制为VIP权益,开通VIP直接复制
