from django.shortcuts import render, redirect
from django.views import View
from django.contrib import messages
from django.db.models import Q
from mylibrary.models import User, Book, Borrow, Log
from mylibrary.forms import LoginForm, RegisterForm, SearchForm
from datetime import datetime, timedelta
import hashlib
class IndexView(View):
"""
主页
"""
def get(self, request):
return redirect('/login/')
class LoginView(View):
"""
登录
"""
def get(self, request):
if request.session.get('is_login', None):
return redirect('/home/')
login_form = LoginForm()
return render(request, 'login.html', locals())
def post(self, request):
login_form = LoginForm(request.POST)
message = '请检查填写的内容!'
if login_form.is_valid():
user_id = login_form.cleaned_data['user_id']
password = login_form.cleaned_data['password']
user = User.objects.filter(id=user_id).first()
if user and user.password == hashcode(password, user_id):
request.session['is_login'] = True
request.session['user_id'] = user.id
request.session['user_name'] = user.name
Log.objects.create(user_id=user_id, action='登录')
return redirect('/home/')
else:
message = '用户名或密码错误!'
return render(request, 'login.html', locals())
class LogoutView(View):
"""
登出
"""
def get(self, request):
if request.session.get('is_login', None):
Log.objects.create(user_id=request.session['user_id'], action='登出')
request.session.flush()
messages.success(request, '登出成功!')
return redirect('/login/')
class RegisterView(View):
"""
注册
"""
def get(self, request):
register_form = RegisterForm()
return render(request, 'register.html', locals())
def post(self, request):
register_form = RegisterForm(request.POST)
message = '请检查填写的内容!'
if register_form.is_valid():
user_name = register_form.cleaned_data['user_name']
user_id = register_form.cleaned_data['user_id']
password1 = register_form.cleaned_data['password1']
password2 = register_form.cleaned_data['password2']
if password1 == password2:
same_id_users = User.objects.filter(id=user_id)
if same_id_users:
message = '该学号已被注册!'
else:
User.objects.create(id=user_id, name=user_name, password=hashcode(password1, user_id))
Log.objects.create(user_id=user_id, action='注册')
messages.success(request, '注册成功!')
return redirect('/login/')
else:
message = '两次输入的密码不一致!'
return render(request, 'register.html', locals())
class HomeView(View):
"""
个人中心
"""
def get(self, request):
if not request.session.get('is_login', None):
messages.error(request, '请先登录!')
return redirect('/login/')
user_id = request.session['user_id']
borrow_entries = Borrow.objects.filter(user_id=user_id)
return render(request, 'home.html', locals())
class SearchView(View):
"""
借书
"""
def get(self, request):
if not request.session.get('is_login', None):
messages.error(request, '请先登录!')
return redirect('/login/')
search_form = SearchForm(request.GET)
if search_form.is_valid():
keyword = search_form.cleaned_data['keyword']
books = Book.objects.filter(Q(name__icontains=keyword) | Q(author__icontains=keyword) | Q(publisher__icontains=keyword))
if not books:
message = '未查询到相关书籍!'
return render(request, "search.html", locals())
class BorrowView(View):
"""
借书操作
"""
def get(self, request):
if not request.session.get('is_login', None):
messages.error(request, '请先登录!')
return redirect('/login/')
user_id = request.session['user_id']
book_id = request.GET.get('book_id')
books = Book.objects.filter(id=book_id, is_available=True)
if books:
book = books.first()
borrow_time = datetime.now()
return_ddl = borrow_time + timedelta(days=90)
Borrow.objects.create(user_id=user_id, book_id=book_id, borrow_time=borrow_time, return_ddl=return_ddl)
book.is_available = False
book.save()
Log.objects.create(user_id=user_id, book_id=book_id, action='借书')
messages.success(request, '借书成功!')
else:
messages.error(request, '借书失败:此书不存在或已借出!')
return redirect('/search/')
class ReturnView(View):
"""
还书操作
"""
def get(self, request):
if not request.session.get('is_login', None):
messages.error(request, '请先登录!')
return redirect('/login/')
user_id = request.session['user_id']
book_id = request.GET.get('book_id')
borrow_entries = Borrow.objects.filter(user_id=user_id, book_id=book_id)
if borrow_entries:
borrow_entry = borrow_entries.first()
delta = -(borrow_entry.borrow_time - datetime.now()) # 负天数不足一天算一天
exceed_days = delta.days - 90
if exceed_days > 0:
fine = exceed_days * 0.5
messages.warning(request, '已逾期 {} 天,需缴纳罚金 {} 元!'.format(exceed_days, fine))
borrow_entry.delete()
book = Book.objects.get(id=book_id)
book.is_available = True
book.save()
Log.objects.create(user_id=user_id, book_id=book_id, action='还书')
messages.success(request, '还书成功!')
else:
messages.error(request, '还书失败:您未借过此书!')
return redirect('/home/')
class TestView(View):
"""
for test
"""
def get(self, request):
search_form = SearchForm()
return render(request, 'test.html', locals())
def hashcode(s, salt='17373252'):
s += salt
h = hashlib.sha256()
h.update(s.encode())
return h.hexdigest()
没有合适的资源?快使用搜索试试~ 我知道了~
Python 毕业设计-图书借阅管理系统 - 附报告源码,保证可用.zip
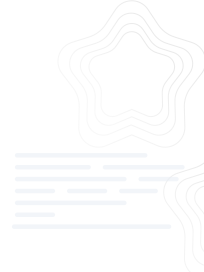
共103个文件
py:32个
pyc:28个
css:10个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
Python 毕业设计-图书借阅管理系统,可作为毕业设计 1. 注册 用户打开注册页面,输入个人信息,RegisterView 获得后向 User 查询该用户是否不存在,若是则在 User 中增添该用户,并将本次注册操作写入 Log,然后返回注册成功信息。 2. 登录 用户打开登录页面,输入用户名和密码,LoginView 获得后向 User 查询该用户是否存在,若是则将本次登录操作写入 Log,然后跳转页面到用户的个人中心。HomeView 向 Borrow 查询该用户的借阅信息,并返回借阅列表。 3. 借书 用户打开查询界面,输入想要借的书籍的关键字,SearchView 获得后向 Book 查询相关书籍,并返回书籍列表。用户点击列表项目的借书按钮,BorrowView 向 Borrow 中增添一条该用户的借书记录,然后在 Book 中将该书籍设置为不可借,并将本次借书操作写入 Log,最后返回借书成功信息。 4. 还书 用户打开个人中心页面,HomeView 向 Borrow 查询该用户的借阅信息,并返回借阅列
资源推荐
资源详情
资源评论
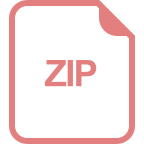
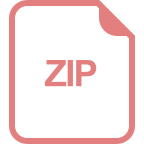
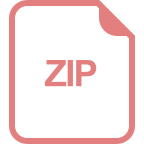
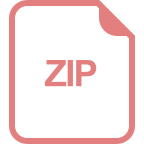
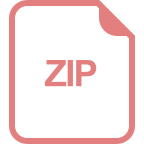
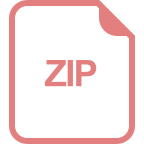
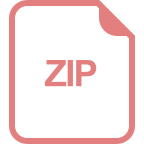
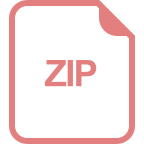
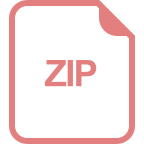
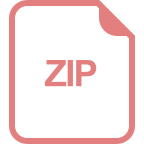
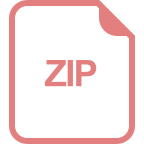
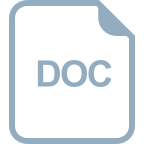
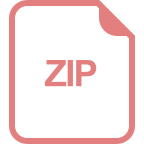
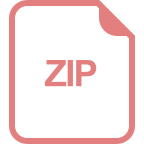
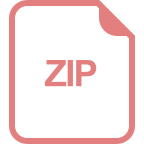
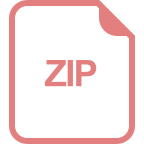
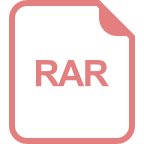
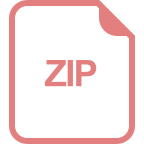
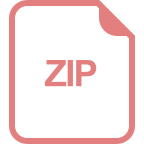
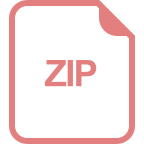
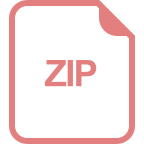
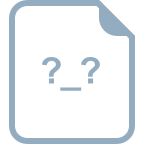
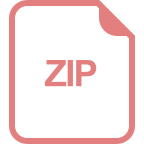
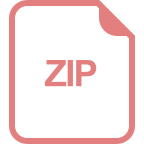
收起资源包目录

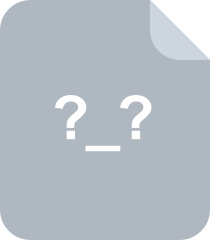
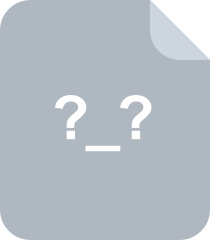
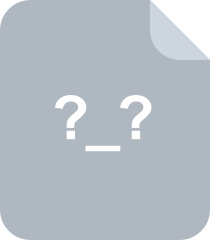
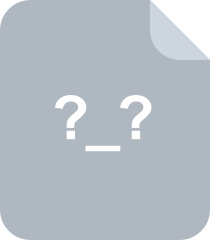
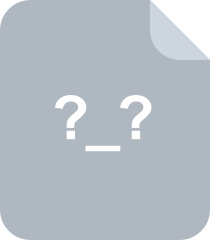
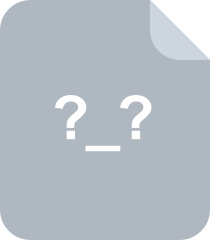
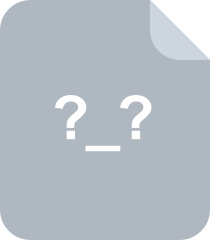
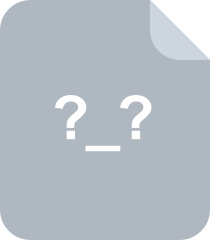
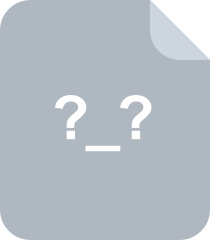
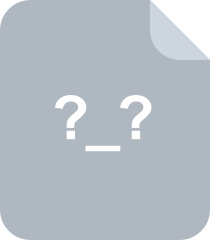
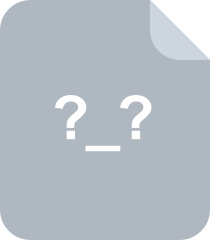
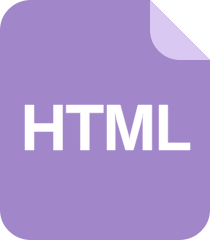
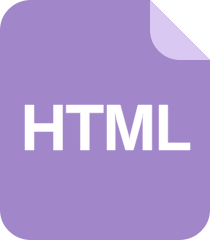
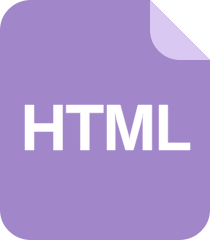
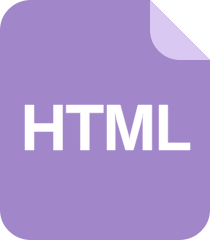
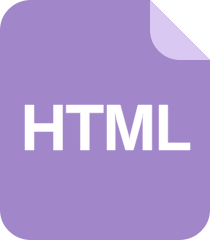
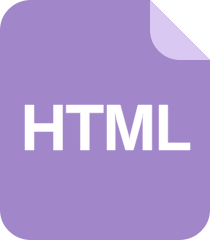
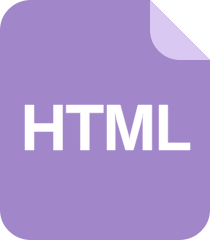
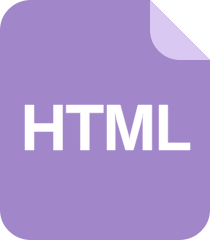
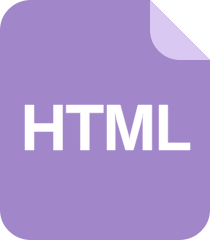
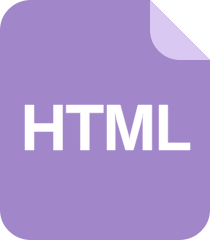
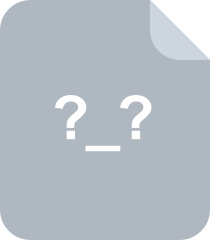
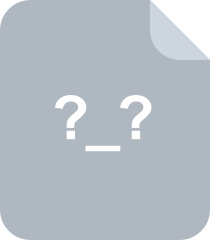
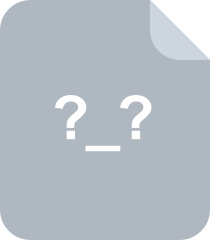
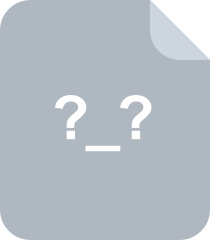
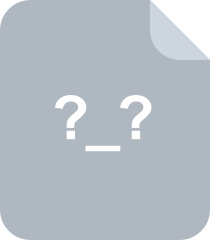
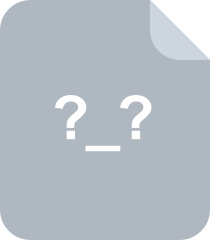
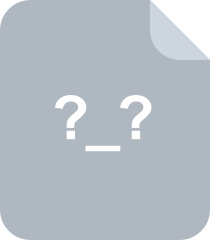
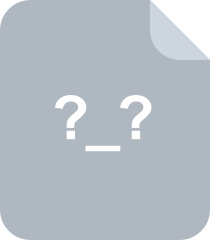
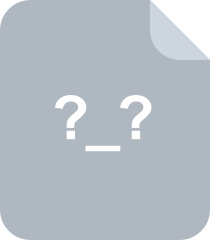
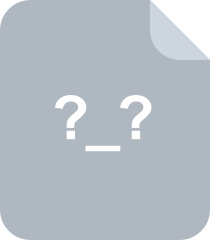
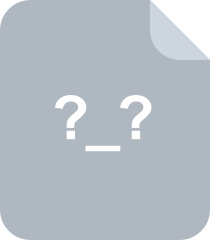
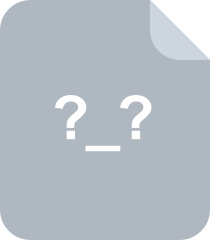
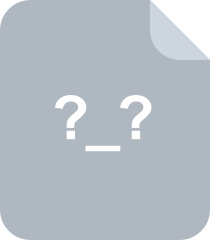
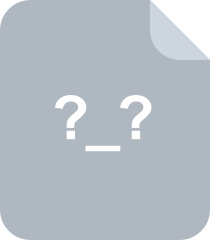
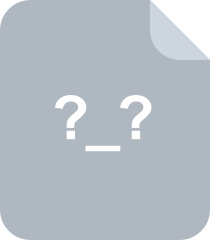
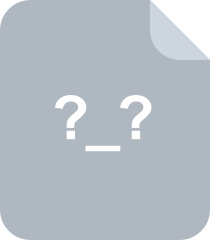
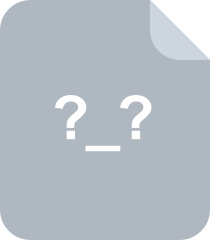
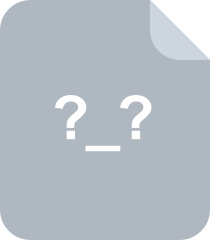
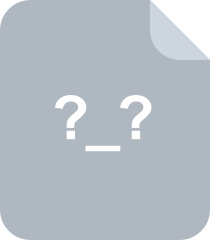
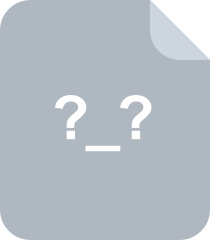
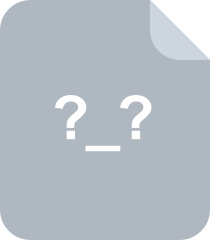
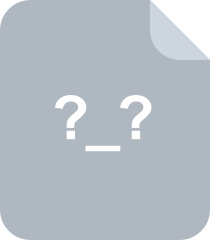
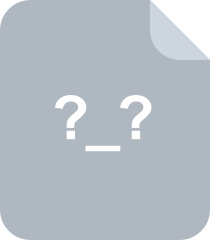
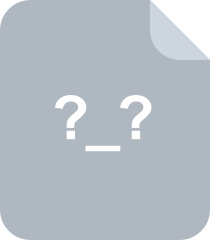
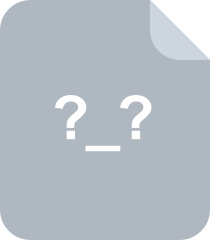
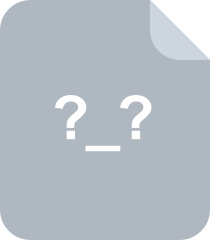
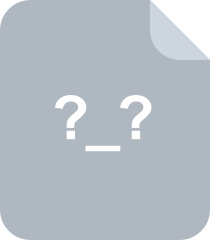
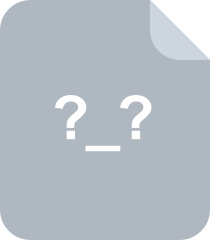
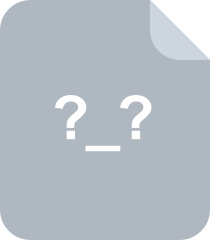
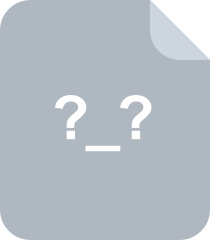
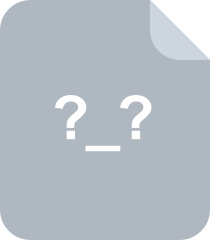
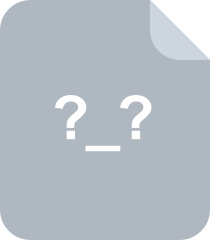
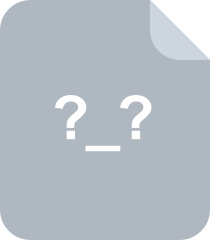
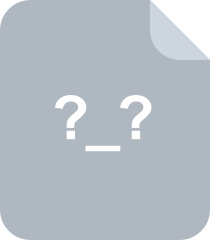
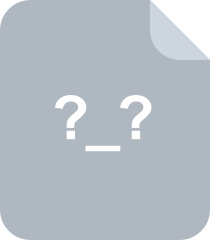
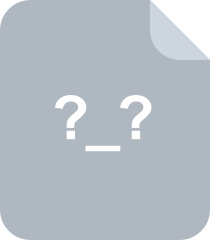
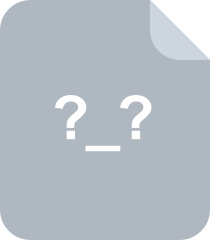
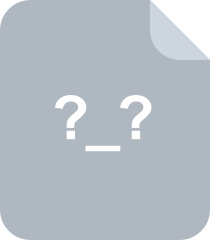
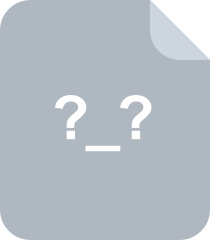
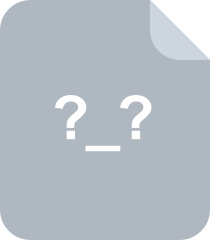
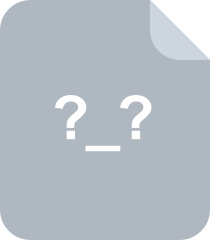
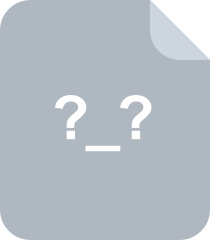
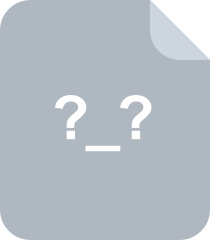
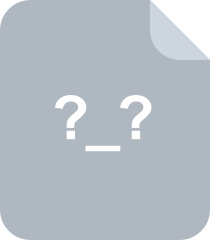
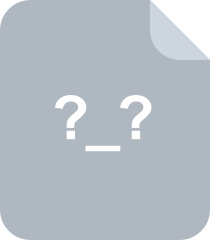
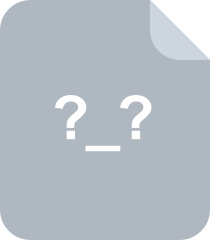
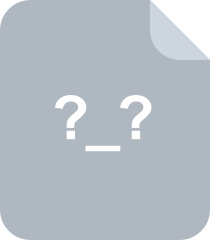
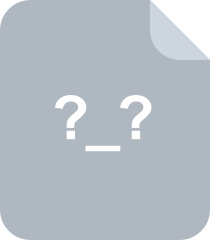
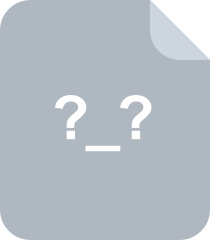
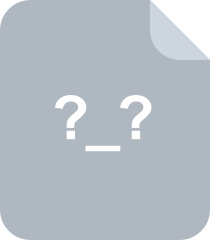
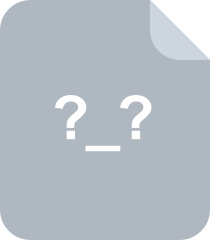
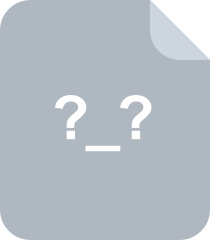
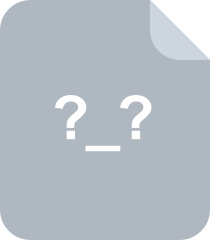
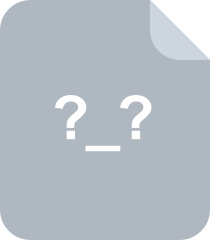
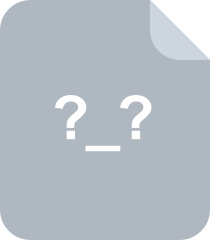
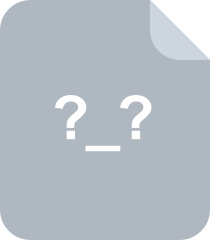
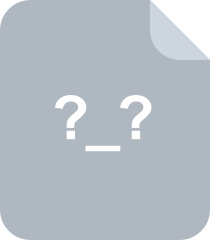
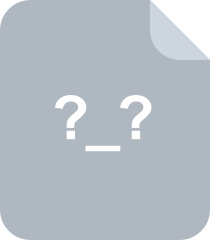
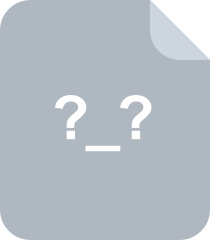
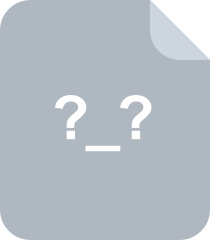
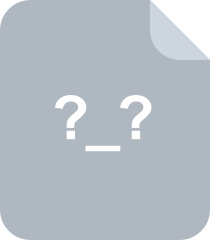
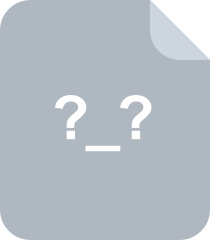
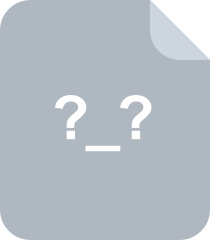
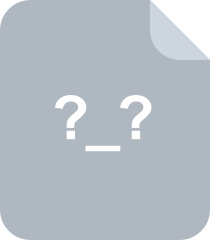
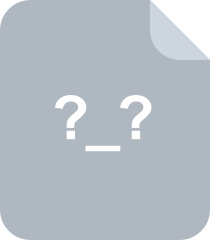
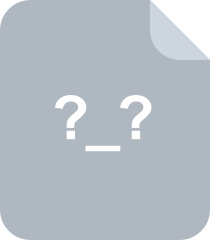
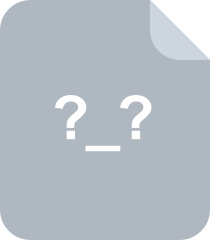
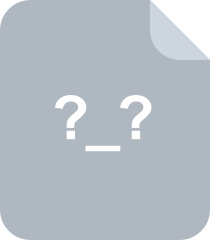
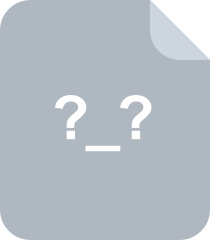
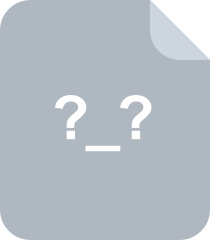
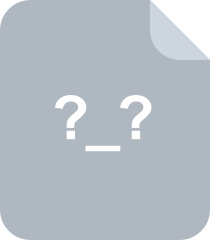
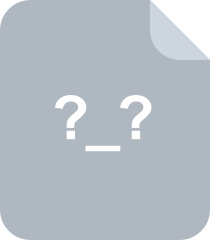
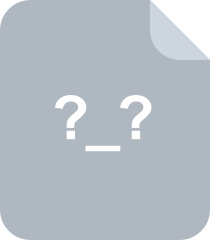
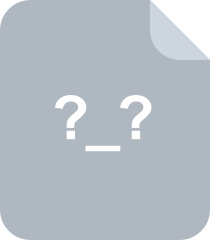
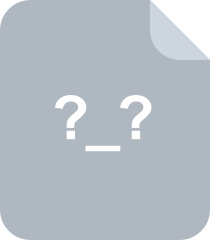
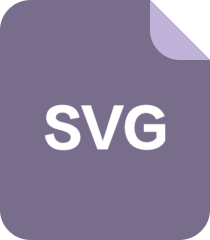
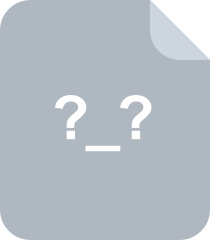
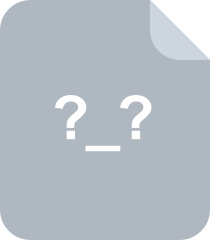
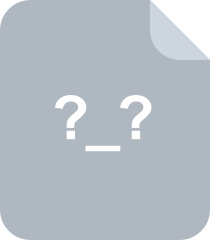
共 103 条
- 1
- 2

gdutxiaoxu
- 粉丝: 1543
- 资源: 3119
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

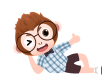
最新资源
- 白色大气风格的室内装修设计网站模板下载.zip
- 白色大气风格的手机电脑商城模板下载.zip
- 白色大气风格的手机软件公司html5模板下载.zip
- 白色大气风格的手机端HTML5企业网站模板.zip
- 白色大气风格的水疗按摩网页模板下载.zip
- 白色大气风格的双屏个人主页模板.zip
- 白色大气风格的数据研究公司模板下载.zip
- 白色大气风格的探险文化企业网站模板下载.zip
- 白色大气风格的投资企业CSS3网站模板.zip
- 白色大气风格的投资网站CSS3模板.zip
- 白色大气风格的图片设计类网站模板下载.zip
- 白色大气风格的网上购物CSS3整站网站模板.zip
- 白色大气风格的土建设计公司模板下载.zip
- 白色大气风格的纹身企业网站模板.zip
- 白色大气风格的温馨舒适家具bootstrap模板.zip
- 白色大气风格的五星级酒店集团模板下载.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


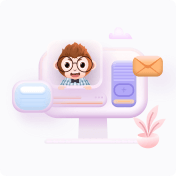
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页