c++String类的重写
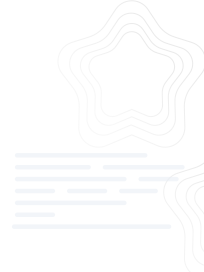


在C++编程语言中,`std::string` 类是用于处理字符串的重要工具,它提供了丰富的功能,如拼接、查找、替换等。然而,为了满足特定需求或优化性能,我们有时需要对`std::string`类进行重写,即自定义它的行为。这通常涉及到运算符重载和构造函数重载。 ### 运算符重载 **1. 赋值运算符(=)**:`std::string`类中已经有一个默认的赋值运算符,但如果你希望在复制字符串时执行特殊操作(例如深拷贝),你可以重写这个运算符。例如: ```cpp class MyString : public std::string { public: MyString& operator=(const MyString& other) { // 执行自定义操作 std::string::operator=(other); return *this; } }; ``` **2. 索引运算符([])**:允许通过索引访问字符串中的字符,例如`myStr[i]`。重写它可以帮助添加边界检查或其他行为: ```cpp class MyString : public std::string { public: char& operator[](size_t index) { assert(index < size()); // 添加边界检查 return std::string::operator[](index); } const char& operator[](size_t index) const { assert(index < size()); return std::string::operator[](index); } }; ``` **3. 连接运算符(+)**:用于连接两个字符串。如果需要自定义连接策略,可以重写这个运算符: ```cpp class MyString : public std::string { public: MyString operator+(const MyString& other) const { MyString result(*this); result.append(other); return result; } }; ``` ### 构造函数重载 **1. 默认构造函数**:创建一个空字符串。你可以根据需要添加额外的行为: ```cpp class MyString : public std::string { public: MyString() : std::string() { // 自定义行为,例如设置默认容量 } }; ``` **2. 从C风格字符串构造**:从`const char*`创建字符串: ```cpp class MyString : public std::string { public: MyString(const char* str) : std::string(str) { // 自定义行为,例如检查输入是否为NULL } }; ``` **3. 从字符数组构造**:允许传入特定长度的字符数组: ```cpp class MyString : public std::string { public: MyString(const char* str, size_t len) : std::string(str, len) { // 自定义行为,例如检查长度 } }; ``` ### 其他重写 除了运算符和构造函数,还可以考虑重写其他成员函数,如`append()`、`insert()`、`erase()`等,以适应特定的需求。同时,注意保持与`std::string`基类的兼容性,确保代码的可移植性和互操作性。 在实际应用中,重写`std::string`类通常是为了实现特定的性能优化、错误检查或添加额外的功能。不过,除非有明确的理由,否则不推荐这样做,因为标准库的实现通常经过了大量优化,自定义可能会引入新的bug或降低性能。在进行这样的重写时,一定要谨慎,并进行充分的测试。
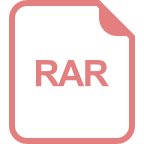
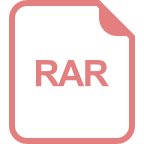
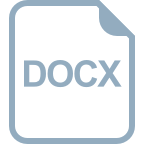
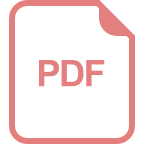
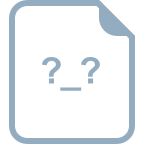
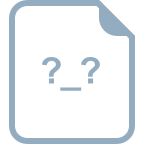
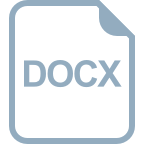
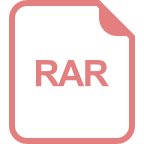
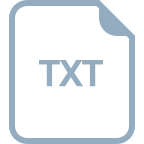
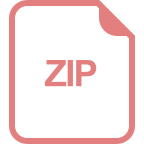
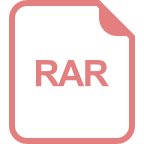
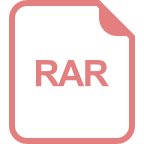
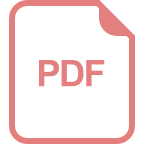


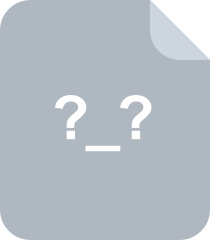
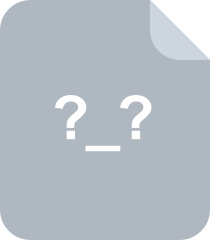
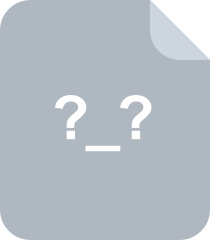


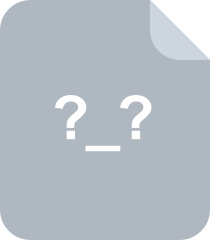

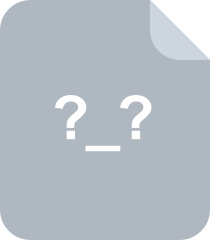
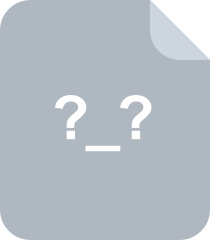
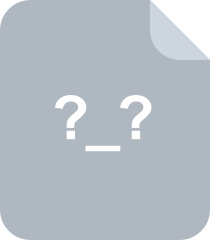
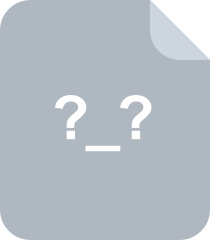
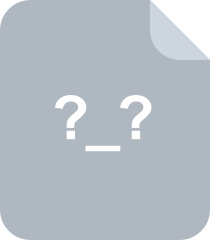
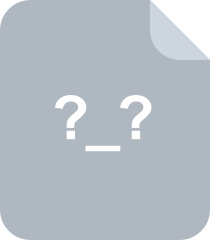
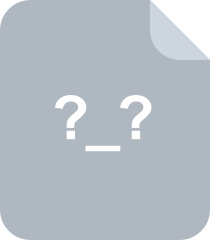
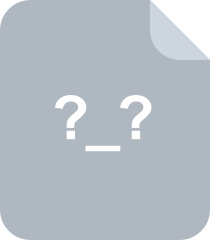
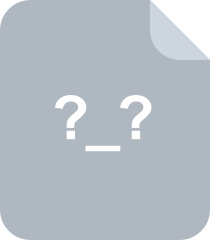

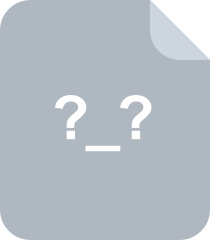
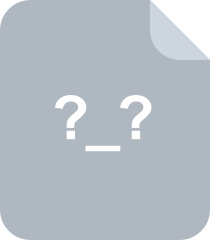
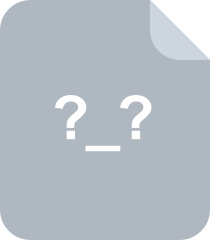
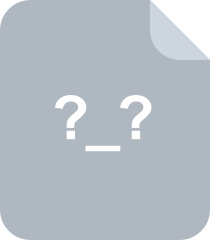
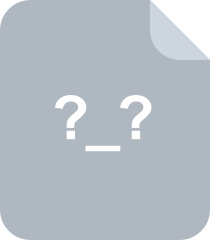
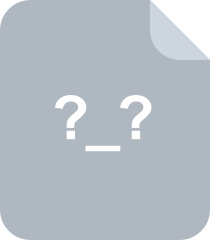
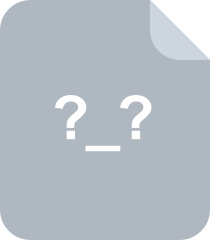
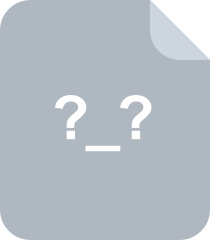
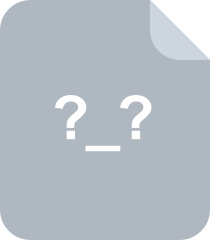
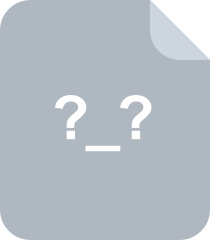
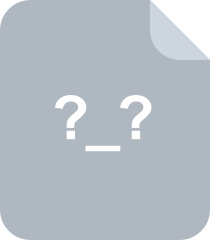
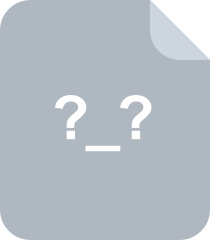
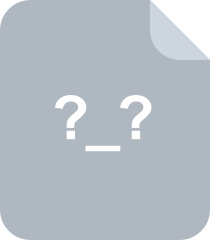
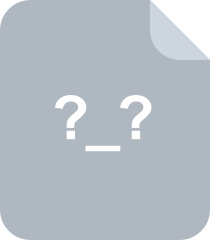
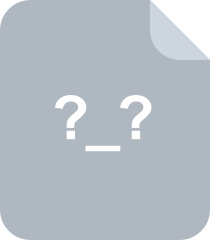
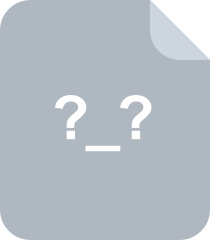
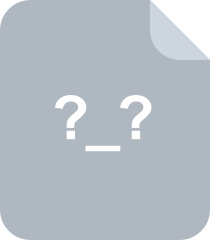
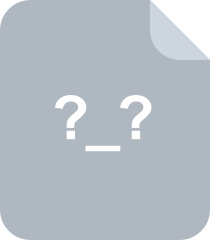
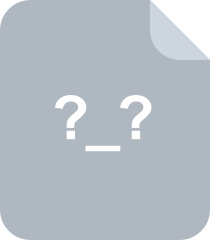
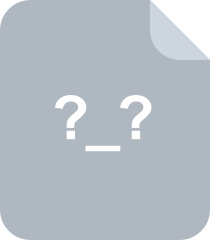
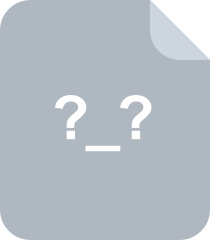
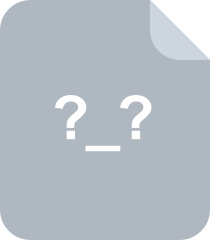
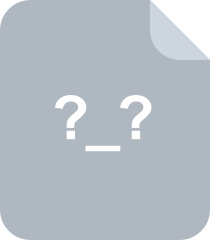
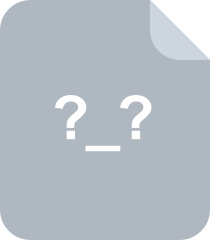
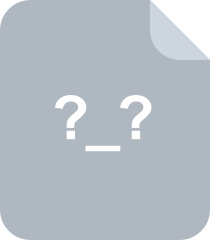
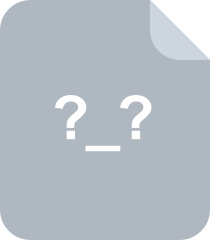
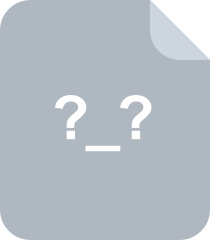
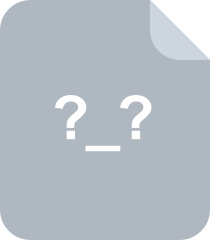
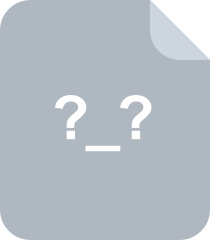
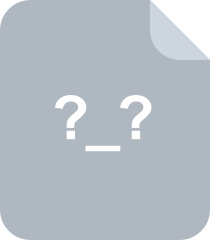
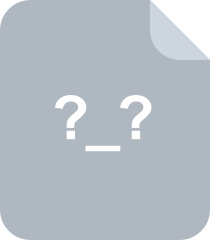
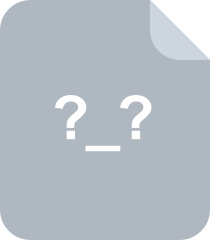
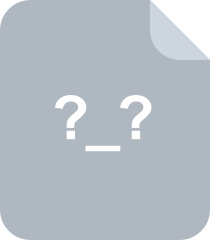
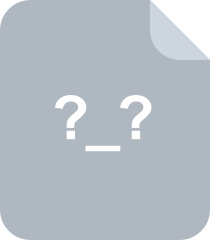
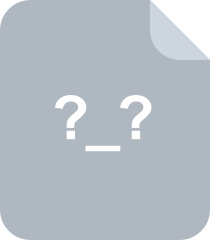
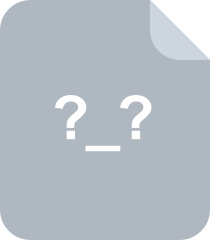
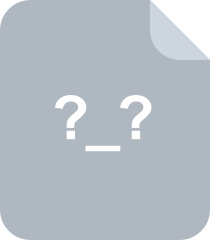
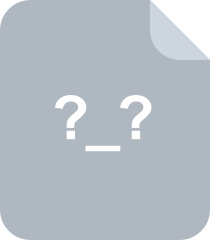
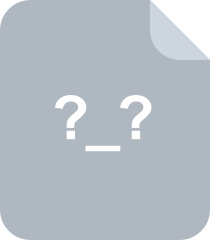
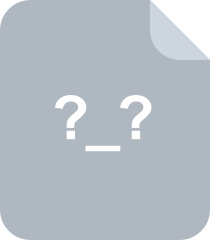
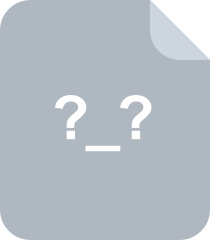
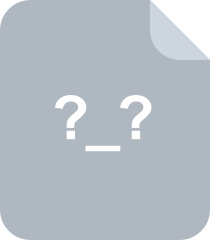
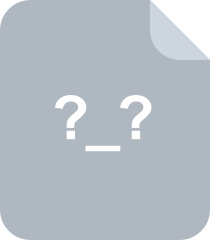
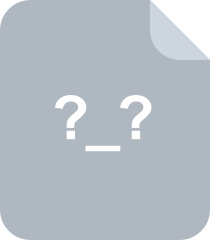
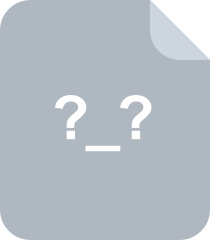
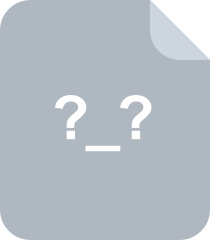
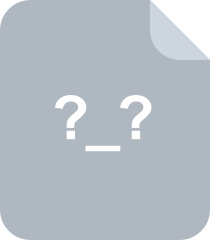
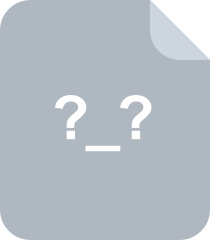
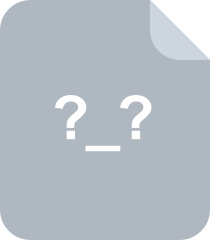
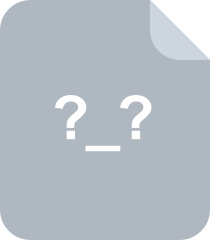
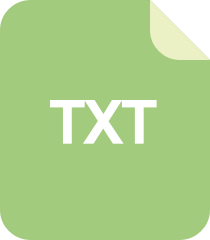

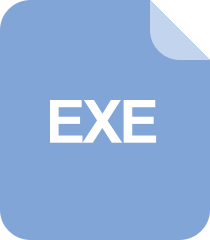
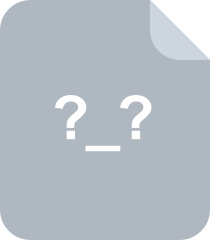
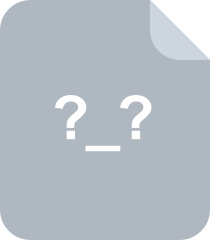
- 1
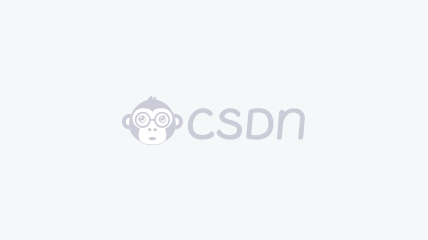
- zhgfan1232013-07-05代码很规范,不过只重载了一下运算符
- Genius_deng2013-11-26写的一般...没有想象中的好

- 粉丝: 0
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

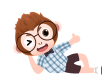
最新资源
- GD32 123456789
- 机器学习实现贷款违约行为预测
- 【java毕业设计】智慧社区养老照护系统.zip
- 【java毕业设计】智慧社区在线教育平台.zip
- 【java毕业设计】智慧社区就业服务门户.zip
- 【java毕业设计】智慧社区心理咨询服务.zip
- 【java毕业设计】智慧社区法律咨询服务网.zip
- 【java毕业设计】智慧社区金融服务网络.zip
- 【java毕业设计】智慧社区旅游服务网络.zip
- 【java毕业设计】智慧社区餐饮服务平台网.zip
- 【java毕业设计】智慧社区医疗健康门户.zip
- 【java毕业设计】智慧社区邻里社交平台网.zip
- 【java毕业设计】智慧社区文化艺术展示网.zip
- 【java毕业设计】智慧社区体育健身网络.zip
- 【java毕业设计】智慧社区公共信息显示系统.zip
- 【java毕业设计】智慧社区居民意见门户.zip

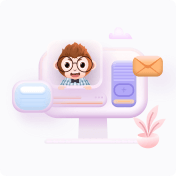
