protues模拟红外发射接收
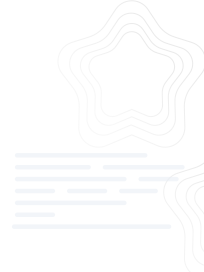


Protues是一款强大的虚拟原型设计工具,它允许用户在软件中构建和模拟电子电路,而无需实际搭建硬件。在“protues模拟红外发射接收”的主题中,我们将深入探讨如何使用Protues来模拟红外(IR)通信系统,这在电子工程的学习和实验中非常常见。 红外发射与接收是无线通信的一种方式,广泛应用于遥控器、传感器和数据传输等领域。红外信号由红外发射器发出,通过空气传播,然后被红外接收器捕获并解码为可理解的信号。 在Protues中模拟这个过程,你需要创建两个主要部分:红外发射电路和红外接收电路。发射电路通常包含一个微控制器(如Arduino或51单片机),用于编码和驱动红外LED。接收电路则包括红外光敏二极管和相应的滤波及放大电路,用于接收并解码红外信号。 在描述中提到的"附有源代码",这意味着可能提供了微控制器的编程代码,用于控制红外发射和接收。这些代码可能使用C语言编写,其中发射部分可能包含了特定的编码算法(如NEC或RC5协议),接收部分则包含了解码逻辑。 "Last Loaded 根据网上重做我自己的.DBK、根据网上重做我自己的.DSN、根据网上重做我自己的.PWI、发射2、接收、发射"这些文件名表明它们可能是Protues工程文件或相关资源。`.DBK`和`.DSN`是Protues的工程文件格式,`.PWI`可能是保存工作界面或设置的文件。"发射2"和"接收"可能代表不同的电路设计或代码文件,分别对应红外发射和接收功能。 在实际操作中,你需要在Protues中打开`.DSN`文件加载电路,然后运行`.DBK`项目文件来启动模拟。`.PWI`文件可以帮助恢复工作环境。同时,你需要理解并适配源代码,确保它们与你的Protues模拟电路相匹配。 模拟红外发射接收的过程中,关键步骤包括: 1. **建立电路**:在Protues中添加微控制器、红外LED(发射端)、红外光敏二极管(接收端)以及必要的电阻和电容等元件。 2. **编写代码**:根据提供的源代码,理解并修改以适应你的模拟需求。发射端代码会生成特定的红外脉冲序列,而接收端代码则负责解析这些脉冲。 3. **配置模拟**:设置好Protues的模拟参数,如时间步长、模拟时长等。 4. **运行模拟**:启动模拟后,你可以观察发射和接收端的行为,检查信号是否正确发送和接收。 5. **调试**:如果模拟结果不符合预期,可以检查代码或电路设计是否有误,并进行相应调整。 通过这样的模拟,学习者可以深入理解红外通信的工作原理,无需实际硬件,就能进行反复试验和调试,对理论知识有更直观的认识。同时,这也是一个很好的实践练习,有助于提升电子设计和编程技能。
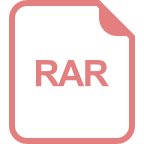
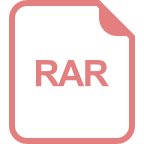
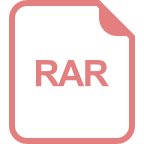
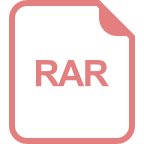
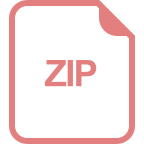
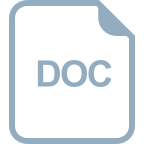
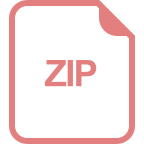
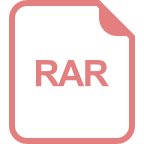
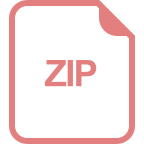
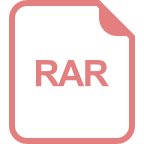
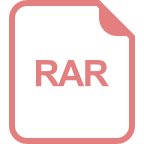


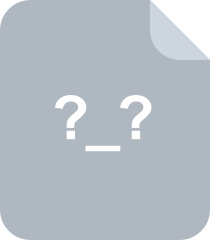
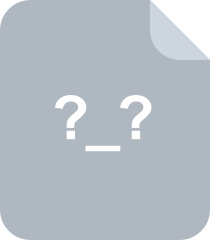
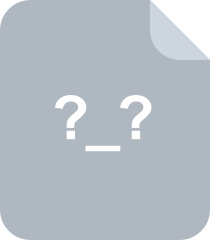
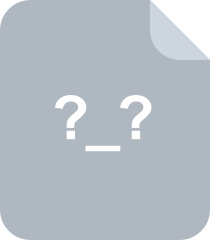
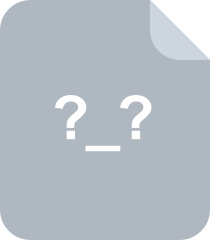
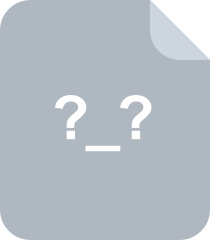
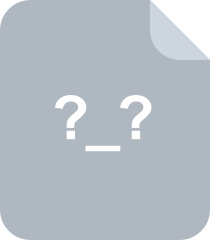
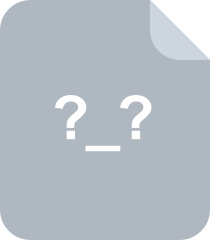
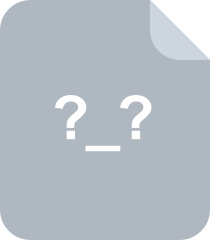
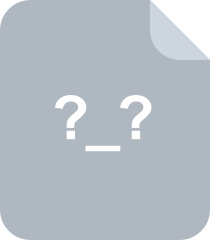
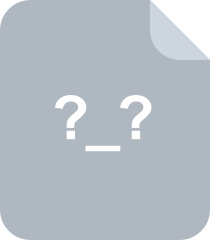
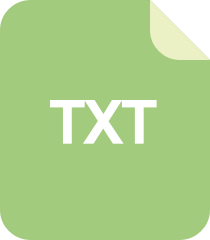
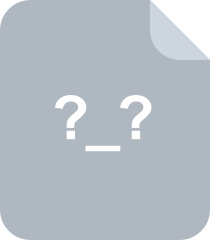
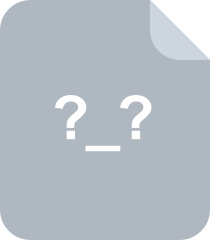

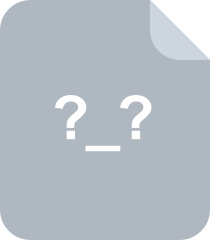
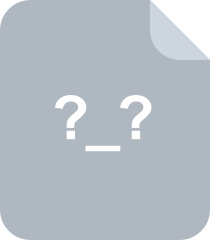
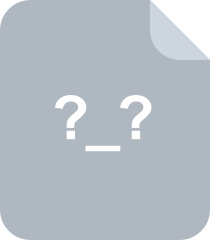
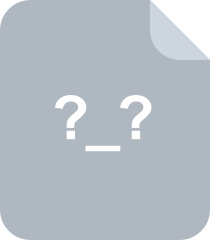
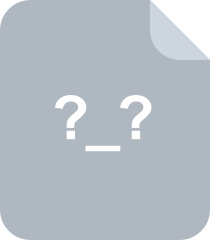
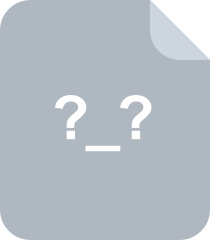
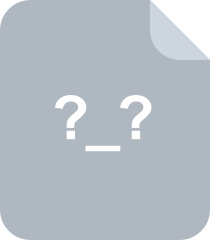
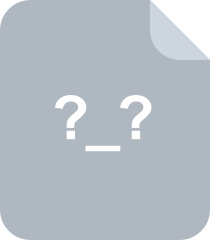
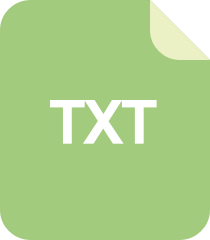
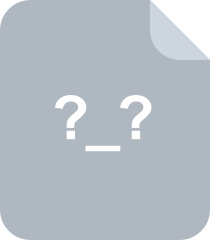
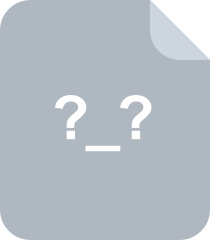
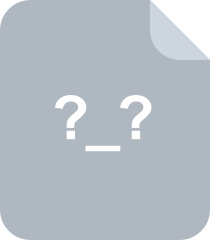
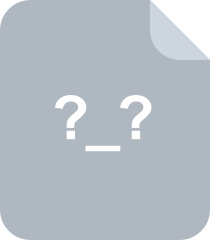

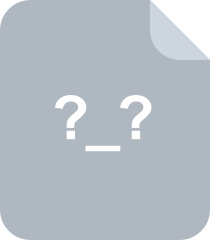
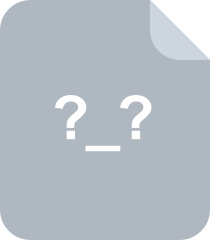
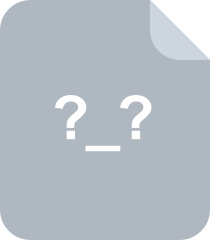
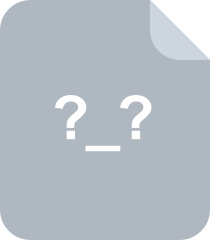
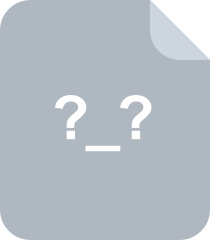
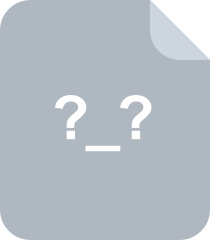
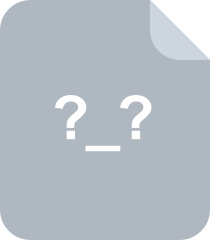
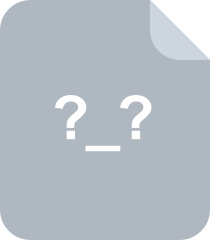
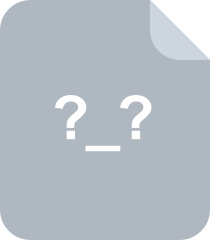
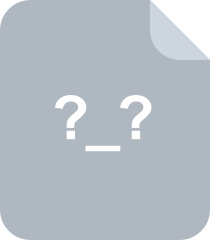
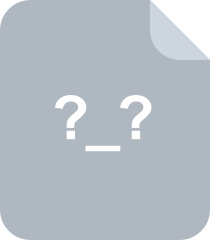
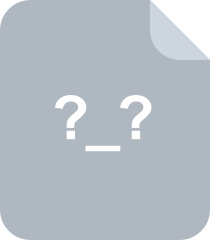
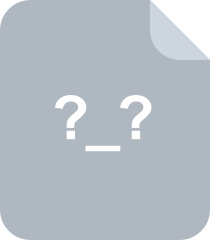
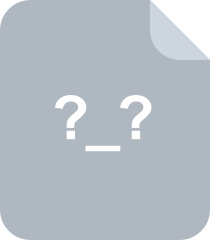
- 1

- 粉丝: 1
- 资源: 19
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

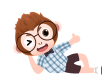
最新资源
- (源码)基于Qt和AVR的FestosMechatronics系统终端.zip
- (源码)基于Java的DVD管理系统.zip
- (源码)基于Java RMI的共享白板系统.zip
- (源码)基于Spring Boot和WebSocket的毕业设计选题系统.zip
- (源码)基于C++的机器人与船舶管理系统.zip
- (源码)基于WPF和Entity Framework Core的智能货架管理系统.zip
- SAP Note 532932 FAQ Valuation logic with active material ledger
- (源码)基于Spring Boot和Redis的秒杀系统.zip
- (源码)基于C#的计算器系统.zip
- (源码)基于ESP32和ThingSpeak的牛舍环境监测系统.zip

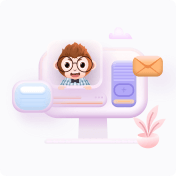

- 1
- 2
- 3
- 4
前往页