VC编写定时关机程序
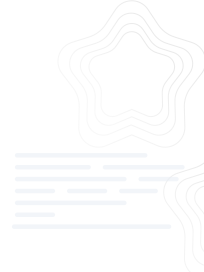


在本文中,我们将深入探讨如何使用Microsoft Foundation Class (MFC) 库在Visual C++ (VC++)环境中编写一个定时关机程序。MFC是微软提供的一套C++类库,它封装了Windows API,使开发者能更方便地构建Windows应用程序。 我们需要理解定时关机的核心机制。在Windows操作系统中,可以通过发送一个特定的系统消息来实现电脑的定时关机。这个消息通常是`WM_SHUTDOWN`。然而,在实际编程中,我们不能直接发送这个消息,而是要通过调用API函数来执行此操作。其中,`ExitWindowsEx`函数是最常用的,它可以安全地关闭或重新启动计算机。 创建一个MFC应用程序的第一步是启动Visual Studio,并选择“新建项目” -> “MFC应用程序”。在向导中,你可以选择“基于对话框”的应用程序模板,因为定时关机程序通常需要用户界面来设置关机时间。 接下来,我们需要添加一个时间选择控件,如DateTimePicker,让用户能够选择关机的日期和时间。在资源编辑器中,右键单击对话框资源,选择“插入” -> “DateTimePicker”,并为其分配一个ID(例如IDD_SHUTDOWN_TIME)。 然后,我们需要处理DateTimePicker的`ON_WM_COMMAND`消息,当用户选择一个时间时,获取所选的时间值。在`CMyApp::OnInitDialog()`方法中,可以添加以下代码: ```cpp void CMyApp::OnInitDialog() { CDialogEx::OnInitDialog(); // 获取DateTimePicker的控件指针 CDateTimePickerCtrl* pDateTimePicker = (CDateTimePickerCtrl*)GetDlgItem(IDC_SHUTDOWN_TIME); // 设置初始时间,如果需要的话 // ...其他初始化代码 } ``` 接着,定义一个定时器来触发关机操作。在`ON_BN_CLICKED`消息处理程序中,为按钮添加以下代码: ```cpp void CMyApp::OnBnClickedShutdownButton() { // 获取DateTimePicker中的时间 SYSTEMTIME systemTime; CDateTimePickerCtrl* pDateTimePicker = (CDateTimePickerCtrl*)GetDlgItem(IDC_SHUTDOWN_TIME); pDateTimePicker->GetSystemTime(&systemTime); // 计算从当前时间到关机时间的毫秒数 ULONGLONG millisecondsToShutdown = GetTimeDifferenceInMilliseconds(&systemTime); // 创建定时器 SetTimer(NULL, 1, (UINT)millisecondsToShutdown, NULL); } ``` 这里,`GetTimeDifferenceInMilliseconds`是一个自定义函数,用于计算两个时间之间的差值。实现如下: ```cpp ULONGLONG CMyApp::GetTimeDifferenceInMilliseconds(const SYSTEMTIME* shutdownTime) { FILETIME fileTime1, fileTime2; ULARGE_INTEGER largeInt1, largeInt2; // 将当前时间转换为FILETIME GetSystemTimeAsFileTime(&fileTime1); // 将关机时间转换为FILETIME SystemTimeToFileTime(shutdownTime, &fileTime2); // 转换为ULARGE_INTEGER largeInt1.LowPart = fileTime1.dwLowDateTime; largeInt1.HighPart = fileTime1.dwHighDateTime; largeInt2.LowPart = fileTime2.dwLowDateTime; largeInt2.HighPart = fileTime2.dwHighDateTime; // 计算差值 ULONGLONG difference = largeInt2.QuadPart - largeInt1.QuadPart; // 转换为毫秒 return difference / 10000; } ``` 我们需要处理定时器的`ON_TIMER`消息,当定时器触发时调用`ExitWindowsEx`函数: ```cpp void CMyApp::OnTimer(UINT_PTR nIDEvent) { if (nIDEvent == 1) { // 关闭计算机 ExitWindowsEx(EWX_SHUTDOWN, 0); KillTimer(NULL, 1); // 停止定时器 } CDialogEx::OnTimer(nIDEvent); } ``` 在实际应用中,可能还需要添加错误处理和取消关机的功能。这只是一个基本的实现,你可以根据需求扩展功能,例如添加通知提示、允许用户取消关机等。 通过以上步骤,我们就成功地使用MFC和Visual C++编写了一个简单的定时关机程序。这个程序利用了MFC的对话框、控件以及Windows API,展示了如何在Windows环境中集成用户界面和系统级别的功能。在开发过程中,熟悉MFC类库和Windows API是至关重要的,这将有助于创建更复杂的应用程序。
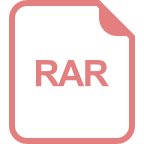
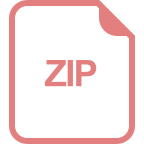
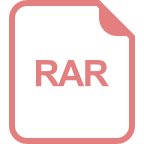
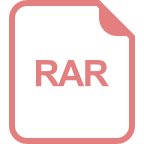
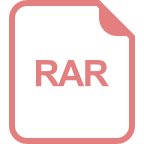
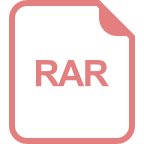
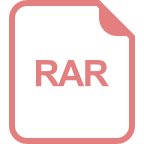
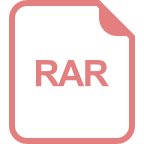
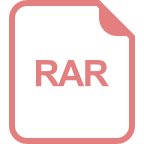
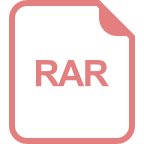
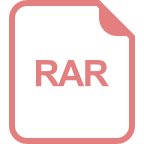



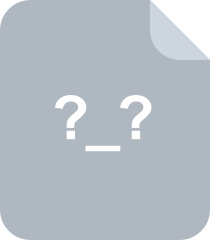
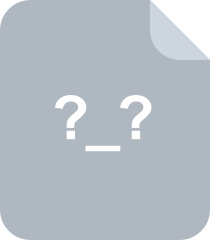
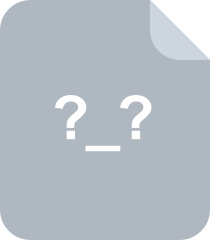
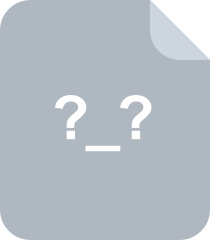
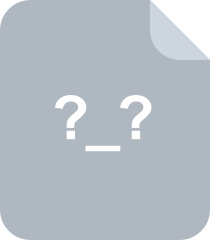
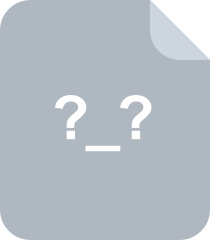
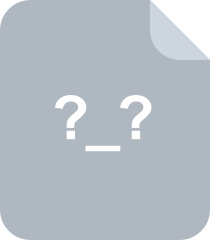
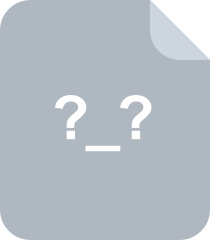
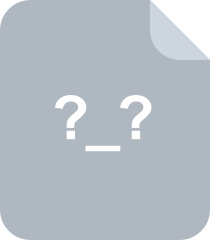
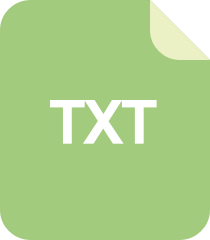
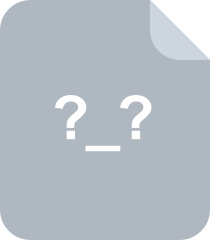
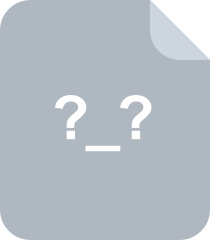
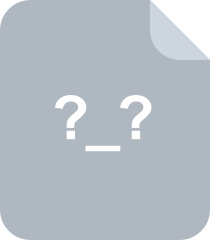
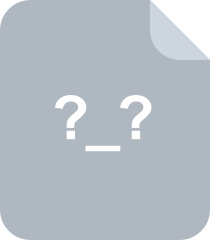
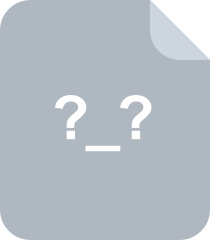

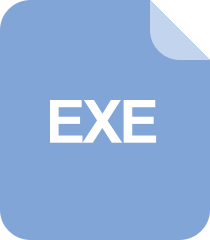
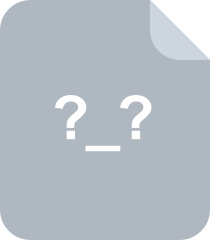
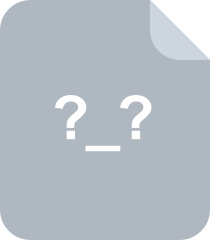
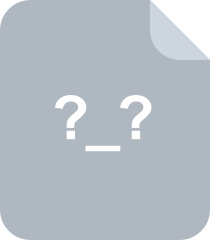
- 1
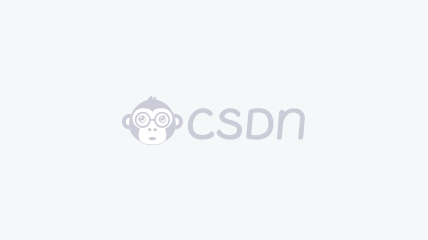
- xuejishu20122012-03-30程序是自动重启啊,不是关机
- u0105871742014-09-11学习一下代码,还可以
- thtfpcuser2014-06-26NT上关机需要权限的。还需要打开token。
- sjz_hh2013-05-21学习一下代码

- 粉丝: 2
- 资源: 16
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

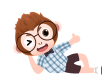
最新资源

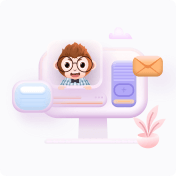
