/* Extended regular expression matching and search library,
version 0.12.
(Implements POSIX draft P10003.2/D11.2, except for
internationalization features.)
Copyright (C) 1993 Free Software Foundation, Inc.
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; either version 2, or (at your option)
any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA. */
/* AIX requires this to be the first thing in the file. */
#if defined (_AIX) && !defined (REGEX_MALLOC)
#pragma alloca
#endif
#define _GNU_SOURCE
/* We need this for `regex.h', and perhaps for the Emacs include files. */
#include <sys/types.h>
#ifdef HAVE_CONFIG_H
#include "config.h"
#endif
/* The `emacs' switch turns on certain matching commands
that make sense only in Emacs. */
#ifdef emacs
#include "lisp.h"
#include "buffer.h"
#include "syntax.h"
/* Emacs uses `NULL' as a predicate. */
#undef NULL
#else /* not emacs */
/* We used to test for `BSTRING' here, but only GCC and Emacs define
`BSTRING', as far as I know, and neither of them use this code. */
#if HAVE_STRING_H || STDC_HEADERS
#include <string.h>
#ifndef bcmp
#define bcmp(s1, s2, n) memcmp ((s1), (s2), (n))
#endif
#ifndef bcopy
#define bcopy(s, d, n) memcpy ((d), (s), (n))
#endif
#ifndef bzero
#define bzero(s, n) memset ((s), 0, (n))
#endif
#else
#include <strings.h>
#endif
#ifdef STDC_HEADERS
#include <stdlib.h>
#else
char *malloc ();
char *realloc ();
#endif
/* Define the syntax stuff for \<, \>, etc. */
/* This must be nonzero for the wordchar and notwordchar pattern
commands in re_match_2. */
#ifndef Sword
#define Sword 1
#endif
#ifdef SYNTAX_TABLE
extern char *re_syntax_table;
#else /* not SYNTAX_TABLE */
/* How many characters in the character set. */
#define CHAR_SET_SIZE 256
static char re_syntax_table[CHAR_SET_SIZE];
static void
init_syntax_once ()
{
register int c;
static int done = 0;
if (done)
return;
bzero (re_syntax_table, sizeof re_syntax_table);
for (c = 'a'; c <= 'z'; c++)
re_syntax_table[c] = Sword;
for (c = 'A'; c <= 'Z'; c++)
re_syntax_table[c] = Sword;
for (c = '0'; c <= '9'; c++)
re_syntax_table[c] = Sword;
re_syntax_table['_'] = Sword;
done = 1;
}
#endif /* not SYNTAX_TABLE */
#define SYNTAX(c) re_syntax_table[c]
#endif /* not emacs */
/* Get the interface, including the syntax bits. */
#include "regex.h"
/* isalpha etc. are used for the character classes. */
#include <ctype.h>
#ifndef isascii
#define isascii(c) 1
#endif
#ifdef isblank
#define ISBLANK(c) (isascii (c) && isblank (c))
#else
#define ISBLANK(c) ((c) == ' ' || (c) == '\t')
#endif
#ifdef isgraph
#define ISGRAPH(c) (isascii (c) && isgraph (c))
#else
#define ISGRAPH(c) (isascii (c) && isprint (c) && !isspace (c))
#endif
#define ISPRINT(c) (isascii (c) && isprint (c))
#define ISDIGIT(c) (isascii (c) && isdigit (c))
#define ISALNUM(c) (isascii (c) && isalnum (c))
#define ISALPHA(c) (isascii (c) && isalpha (c))
#define ISCNTRL(c) (isascii (c) && iscntrl (c))
#define ISLOWER(c) (isascii (c) && islower (c))
#define ISPUNCT(c) (isascii (c) && ispunct (c))
#define ISSPACE(c) (isascii (c) && isspace (c))
#define ISUPPER(c) (isascii (c) && isupper (c))
#define ISXDIGIT(c) (isascii (c) && isxdigit (c))
#ifndef NULL
#define NULL 0
#endif
/* We remove any previous definition of `SIGN_EXTEND_CHAR',
since ours (we hope) works properly with all combinations of
machines, compilers, `char' and `unsigned char' argument types.
(Per Bothner suggested the basic approach.) */
#undef SIGN_EXTEND_CHAR
#if __STDC__
#define SIGN_EXTEND_CHAR(c) ((signed char) (c))
#else /* not __STDC__ */
/* As in Harbison and Steele. */
#define SIGN_EXTEND_CHAR(c) ((((unsigned char) (c)) ^ 128) - 128)
#endif
/* Should we use malloc or alloca? If REGEX_MALLOC is not defined, we
use `alloca' instead of `malloc'. This is because using malloc in
re_search* or re_match* could cause memory leaks when C-g is used in
Emacs; also, malloc is slower and causes storage fragmentation. On
the other hand, malloc is more portable, and easier to debug.
Because we sometimes use alloca, some routines have to be macros,
not functions -- `alloca'-allocated space disappears at the end of the
function it is called in. */
#ifdef REGEX_MALLOC
#define REGEX_ALLOCATE malloc
#define REGEX_REALLOCATE(source, osize, nsize) realloc (source, nsize)
#else /* not REGEX_MALLOC */
/* Emacs already defines alloca, sometimes. */
#ifndef alloca
/* Make alloca work the best possible way. */
#ifdef __GNUC__
#define alloca __builtin_alloca
#else /* not __GNUC__ */
#if HAVE_ALLOCA_H
#include <alloca.h>
#else /* not __GNUC__ or HAVE_ALLOCA_H */
#ifndef _AIX /* Already did AIX, up at the top. */
char *alloca ();
#endif /* not _AIX */
#endif /* not HAVE_ALLOCA_H */
#endif /* not __GNUC__ */
#endif /* not alloca */
#define REGEX_ALLOCATE alloca
/* Assumes a `char *destination' variable. */
#define REGEX_REALLOCATE(source, osize, nsize) \
(destination = (char *) alloca (nsize), \
bcopy (source, destination, osize), \
destination)
#endif /* not REGEX_MALLOC */
/* True if `size1' is non-NULL and PTR is pointing anywhere inside
`string1' or just past its end. This works if PTR is NULL, which is
a good thing. */
#define FIRST_STRING_P(ptr) \
(size1 && string1 <= (ptr) && (ptr) <= string1 + size1)
/* (Re)Allocate N items of type T using malloc, or fail. */
#define TALLOC(n, t) ((t *) malloc ((n) * sizeof (t)))
#define RETALLOC(addr, n, t) ((addr) = (t *) realloc (addr, (n) * sizeof (t)))
#define REGEX_TALLOC(n, t) ((t *) REGEX_ALLOCATE ((n) * sizeof (t)))
#define BYTEWIDTH 8 /* In bits. */
#define STREQ(s1, s2) ((strcmp (s1, s2) == 0))
#define MAX(a, b) ((a) > (b) ? (a) : (b))
#define MIN(a, b) ((a) < (b) ? (a) : (b))
typedef char boolean;
#define false 0
#define true 1
/* These are the command codes that appear in compiled regular
expressions. Some opcodes are followed by argument bytes. A
command code can specify any interpretation whatsoever for its
arguments. Zero bytes may appear in the compiled regular expression.
The value of `exactn' is needed in search.c (search_buffer) in Emacs.
So regex.h defines a symbol `RE_EXACTN_VALUE' to be 1; the value of
`exactn' we use here must also be 1. */
typedef enum
{
no_op = 0,
/* Followed by one byte giving n, then by n literal bytes. */
exactn = 1,
/* Matches any (more or less) character. */
anychar,
/* Matches any one char belonging to specified set. First
following byte is number of bitmap bytes. Then come bytes
for a bitmap saying which chars are in. Bits in each byte
are ordered low-bit-first. A character is in the set if its
bit is 1. A character too large to have a bit in the map is
automatically not in the set. */
charset,
/* Same parameters as charset, but match any character that is
not one of those specified. */
charset_not,
/* Start remembering the text that is matched, for storing in a
register. Followed by one byte with the register number, in
the range 0 to one less than the pattern buffer's re_nsub
field. Then followed by one byte with the number of groups
inner to this one. (This last has to be part of the
start_memory only because we need i

fwxj813604
- 粉丝: 4
- 资源: 37
最新资源
- MATLAB: 双重改进的蚁狮优化算法-连续性边界收缩与动态权重系数的融合优化,MATLAB实现:双改进策略的蚁狮优化算法(IALO)与原始ALO算法的对比分析,matlab:一种改进的蚁狮优化算法
- 流程图-demo-总结的一些流程图
- 管家婆辉煌食品普及版TOP13.11.zip
- 基于人工势场算法的Matlab路径规划系统:自定义起始与目标点,栅格地图路径规划的优化实践,基于人工势场算法的Matlab路径规划算法研究与实现:栅格地图路径规划的自定义起始与目标点设置,人工势场算法
- 管家婆辉煌食品普及版TOP13.22.zip
- 管家婆辉煌食品普及版TOP13.32.zip
- AccessPort-ReceLend-2048
- 自定义A星算法路径规划与二维度码路径优化研究指南:附有详尽注释的代码,本地图自由更换指南 ,深入解析:自定义A星算法二维路径规划的代码实现与地图替换指南,A星算法路径规划 自己编写的Astar二维路
- 紫光拼音输入法-紫光拼音(Ziguang Pinyin)是一款中文拼音输入法软件,由清华大学和紫光集团联合开发
- osg、osgEarth加载tms瓦片数据只显示一个白球,缩放过程中图层消失
- 个人理财系统springboot+Thymeleaf+mysql
- Python网站自动登录项目+采用selenium+webdriver
- 基于A*算法的Matlab多AGV路径规划仿真系统:地图自定义导入,算法优化平滑路径,实时输出时空图与坐标信息 ,基于A*算法的Matlab多AGV路径规划仿真系统:地图自定义导入,优化路径平滑处理
- 基于混合整数二阶锥规划的配电网重构求解与代码详解-计及高比例清洁能源接入与需求响应,《高比例清洁能源接入下计及需求响应的配电网重构算法:混合整数二阶锥优化实现与代码解析》,EI复现高比例清洁能源接
- 管家婆辉煌食品版TOP+12.9.zip
- 管家婆辉煌食品普及版TOP15.0.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


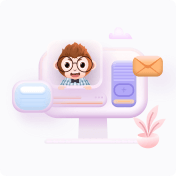