package com.itee.test.dao.impl;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.text.DecimalFormat;
import java.util.Iterator;
import java.util.List;
import java.util.Scanner;
import java.util.Set;
import javax.swing.JOptionPane;
import jxl.Cell;
import jxl.Sheet;
import jxl.Workbook;
import jxl.read.biff.BiffException;
import org.apache.poi.hssf.usermodel.HSSFCell;
import org.apache.poi.hssf.usermodel.HSSFRow;
import org.apache.poi.hssf.usermodel.HSSFSheet;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.poifs.filesystem.POIFSFileSystem;
import org.springframework.orm.hibernate3.support.HibernateDaoSupport;
import org.zkoss.zk.ui.Component;
import org.zkoss.zk.ui.Executions;
import org.zkoss.zul.Button;
import org.zkoss.zul.Combobox;
import org.zkoss.zul.Label;
import org.zkoss.zul.Listbox;
import org.zkoss.zul.Listcell;
import org.zkoss.zul.Listitem;
import org.zkoss.zul.Radiogroup;
import org.zkoss.zul.Textbox;
import com.itee.Excel.CreateXL;
import com.itee.test.dao.TestDao;
import com.itee.test.model.Choosablecourse;
import com.itee.test.model.Kecheng;
import com.itee.test.model.Student;
public class TestDaoImpl extends HibernateDaoSupport implements TestDao {
public String s1,s2;
public void insertObj(Object obj) {
this.getHibernateTemplate().save(obj);
}
public List queryAllByHql(String hql) {
hql = " from Student ";
List list = this.getHibernateTemplate().find(hql);
return list;
}
public void loginObj(Component position1,Component textbox1,Component textbox2) throws InterruptedException{
Textbox zhanghao,secret;
zhanghao=(Textbox)textbox1;
secret=(Textbox)textbox2;
Radiogroup position=(Radiogroup)position1;
s1=zhanghao.getText();
String hql="from Student s where s.xuehao='"+zhanghao.getText()+"'";
List list = this.getHibernateTemplate().find(hql);
if(list.isEmpty())
JOptionPane.showMessageDialog(null,"没有该账户");
for (Iterator a = list.iterator(); a.hasNext();) {
Student stu = (Student) a.next();
if(stu.getSecret().equals(secret.getText())){
if(stu.getQuanxian().equals(position.getSelectedItem().getLabel())){
if(stu.getQuanxian().equals("学生")){
s2=stu.getXingming();
Executions.getCurrent().sendRedirect("student.do");
}
else{
s2=stu.getXingming();
Executions.getCurrent().sendRedirect("admin.do");
}
}
else{
JOptionPane.showMessageDialog(null,"您没有权限");
}
}
else{
JOptionPane.showMessageDialog(null,"密码错误");
}
}
}
public void TianDelGhangeObj(Component self1, Component xuehao1, Student stu) throws InterruptedException {
// TODO Auto-generated method stub
Button button1=(Button)self1;
String s=button1.getLabel();//添加删除修改按钮标签
Textbox xuehao4;
xuehao4=(Textbox)xuehao1;
if(s.equals("添加")){
String s1=xuehao4.getText().trim();
String hql="from Student s where s.xuehao='"+s1+"'";
List list = this.getHibernateTemplate().find(hql);
if(!list.isEmpty()){
JOptionPane.showMessageDialog(null,"该学生已存在");
}
else {
this.getHibernateTemplate().save(stu);
JOptionPane.showMessageDialog(null,"添加成功");
}
}
else if(s.equals("删除")){
if(JOptionPane.showConfirmDialog(null, "确定", "取消", JOptionPane.YES_NO_OPTION)==0){
String s1=xuehao4.getText().trim();
String hql="from Kecheng s where s.xuehao='"+s1+"'";
List list = this.getHibernateTemplate().find(hql);
for (Iterator it = list.iterator(); it.hasNext();) {
Kecheng sc=(Kecheng)it.next();
this.getHibernateTemplate().delete(sc);
}
String hql1="from Student s where s.xuehao='"+s1+"'";
List list1 = this.getHibernateTemplate().find(hql1);
for (Iterator it = list1.iterator(); it.hasNext();) {
Student stu1=(Student)it.next();
this.getHibernateTemplate().delete(stu1);
}
JOptionPane.showMessageDialog(null,"删除成功");
}
}
else if(s.equals("修改")){
String s1=xuehao4.getText().trim();
String hql="from Student s where s.xuehao='"+s1+"'";
List list = this.getHibernateTemplate().find(hql);
if(list.isEmpty()){
JOptionPane.showMessageDialog(null,"该学生不存在");
}
else {
this.getHibernateTemplate().merge(stu);
JOptionPane.showMessageDialog(null,"修改成功");
}
}
}
public void changePersonalInfoObj(Component xuehao1, Component xingming1,
Component xuehao2, Component sexy1, Component birthday1,
Component xueyuan1, Component zhuanye1, Component dianhua1,
Component zhiwei1,Component mima) throws InterruptedException{
Textbox xingming,xuehao4,sexy,birthday,xueyuan,zhuanye,dianhua,zhiwei,mima1;
Textbox xuehao3;
xuehao3=(Textbox)xuehao1;
xingming=(Textbox)xingming1;
xuehao4=(Textbox)xuehao2;
sexy=(Textbox)sexy1;
birthday=(Textbox)birthday1;
xueyuan=(Textbox)xueyuan1;
zhuanye=(Textbox)zhuanye1;
dianhua=(Textbox)dianhua1;
zhiwei=(Textbox)zhiwei1;
mima1=(Textbox)mima;
String hql="from Student s where s.xuehao='"+xuehao3.getText()+"'";
List list = this.getHibernateTemplate().find(hql);
if(list.isEmpty()){
JOptionPane.showMessageDialog(null,"该学生不存在");
}
else{
for (Iterator it = list.iterator(); it.hasNext();) {
Student stu = (Student) it.next();
xingming.setValue(stu.getXingming());
xuehao4.setValue(stu.getXuehao().toString());
sexy.setValue(stu.getSexy());
birthday.setValue(stu.getBirthday());
xueyuan.setValue(stu.getXueyuan());
zhuanye.setValue(stu.getZhuanye());
dianhua.setValue(stu.getDianhua());
zhiwei.setValue(stu.getQuanxian());
mima1.setValue(stu.getSecret());
}
}
}
/* (non-Javadoc)
* @see com.itee.test.dao.TestDao#getChoosableCourseObj(org.zkoss.zk.ui.Component, org.zkoss.zk.ui.Component, org.zkoss.zk.ui.Component)
*/
public void getChoosableCourseObj(Component self1,Component seacherTextbox1,
Component CourseScoreList1) throws InterruptedException{
Button button1=(Button)self1;
Textbox choosableCourseTextbox=(Textbox)seacherTextbox1;
Listbox CourseScoreList=(Listbox)CourseScoreList1;
String s=button1.getLabel();
CourseScoreList.getItems().clear();
if(s.equals("按课程名称查询")){
String hql="from Choosablecourse s where s.theCourse='"+choosableCourseTextbox.getText()+"'";
List list = this.getHibernateTemplate().find(hql);
if(list.equals(null)){
JOptionPane.showMessageDialog(null,"没有您所要查询的课程信息");
}
else{
for (Iterator it=list.iterator();it.hasNext();) {
Choosablecourse sco=(Choosablecourse)it.next();
Listitem li = new Listitem();
Listcell lc1 = new Listcell();
Listcell lc2 = new Listcell();
Listcell lc3 = new Listcell();
lc1.setLabel(sco.getBiaoqian());
lc2.setLabel(sco.getTheCourse());
lc3.setLabel(sco.getTeacher());
li.appendChild(lc1);
li.appendChild(lc2);
li.appendChild(lc3);
CourseScoreList.appendChild(li);
}
}
}
else if(s.equals("全部查询")){
String hql="from Choosablecourse";
List list = this.getHibernateTemplate().find(hql);
if(list.equals(null)){
JOptionPane.showMessageDialog(null,"没有课程");
}
else{
for (Iterator it=list.iterator();it.hasNext();) {
Choosablecourse sco=(Choosablecourse)it.next();
Listitem li = new Listitem();
Listcell lc1 = new Listcell();
Listcell lc2 = new Listcell();
Listcell lc3 = new Listcell();
lc1.setLabel(sco.getBiaoqian());
lc2.setLabel(sco.get
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
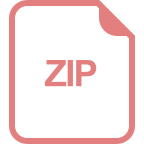
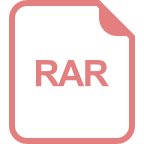
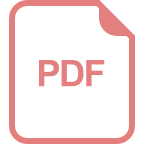
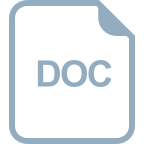
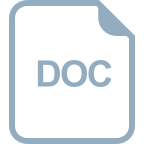
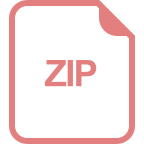
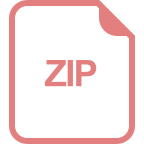
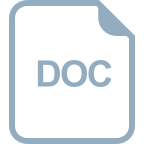
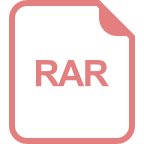
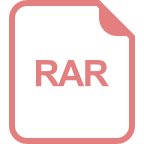
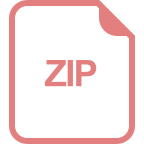
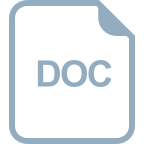
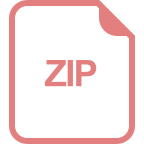
收起资源包目录

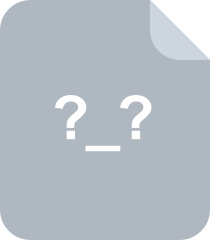
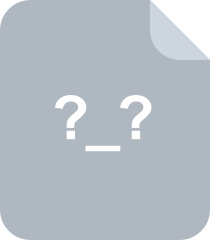
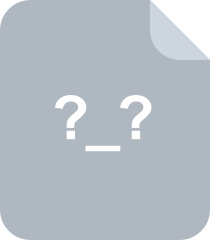
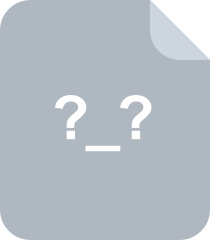
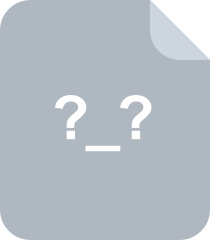
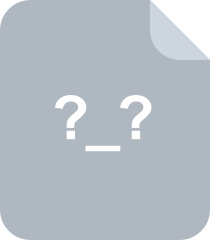
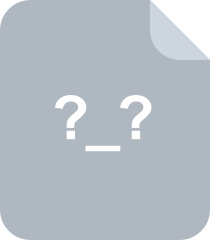
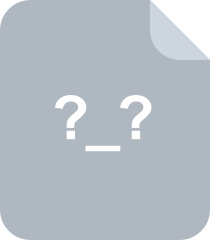
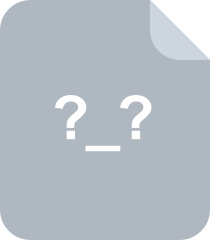
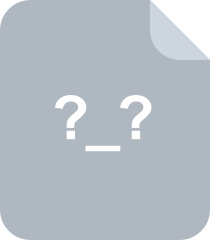
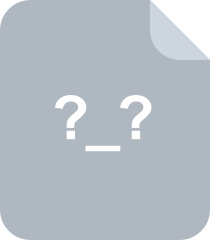
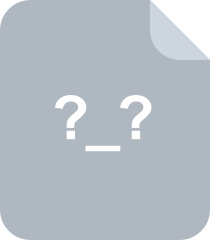
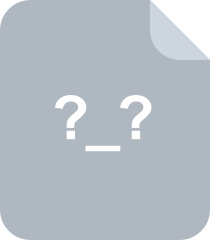
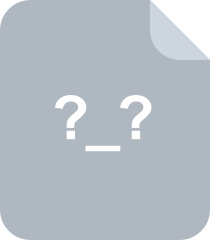
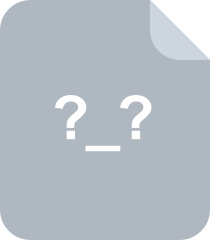
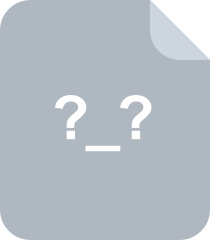
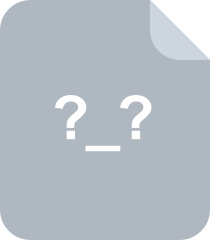
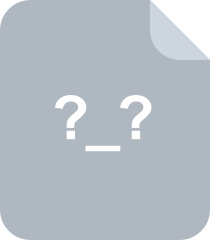
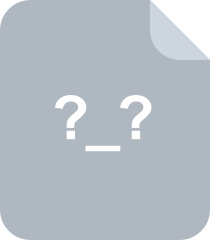
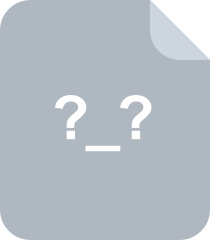
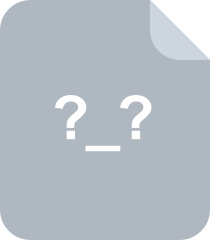
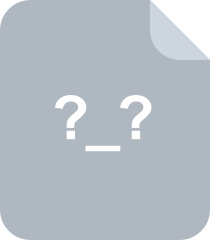
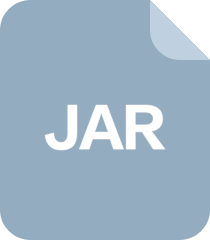
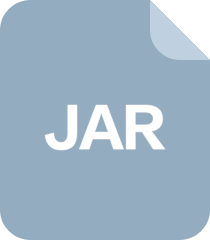
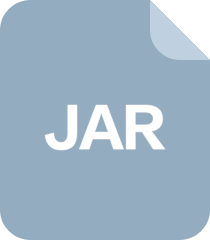
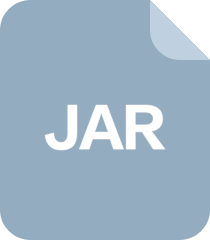
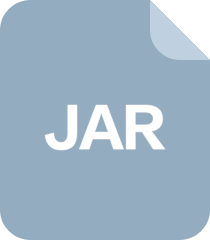
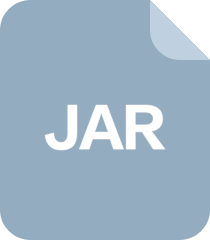
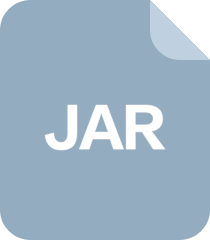
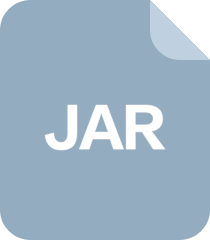
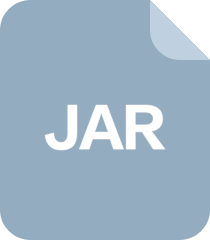
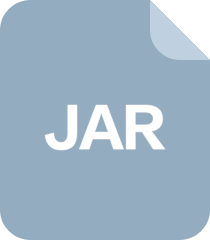
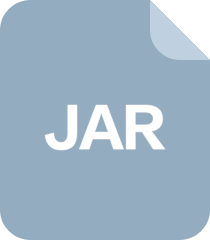
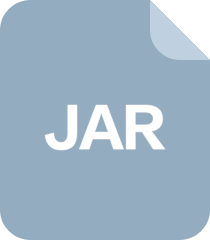
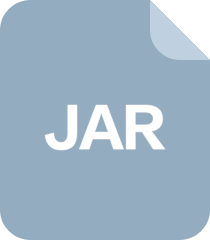
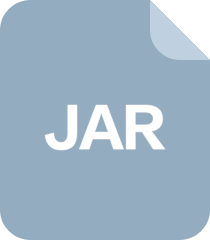
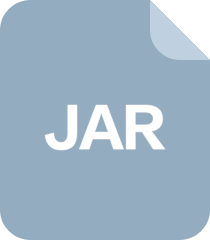
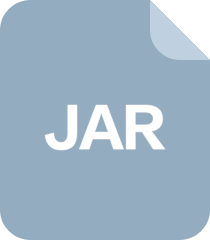
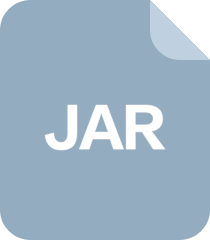
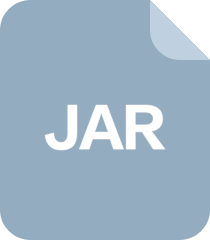
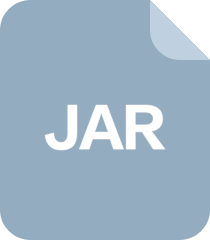
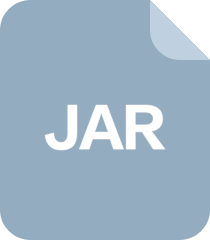
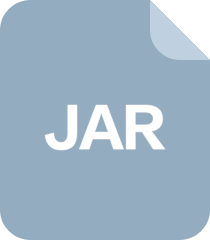
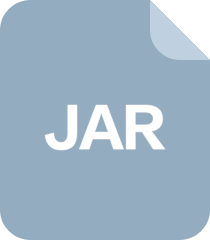
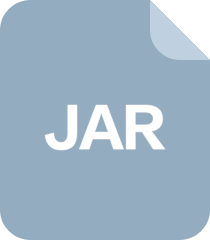
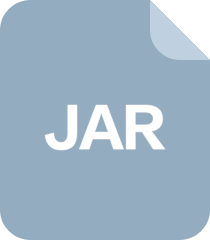
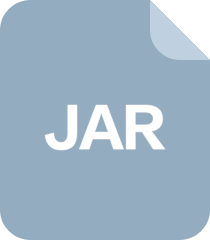
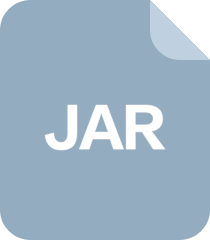
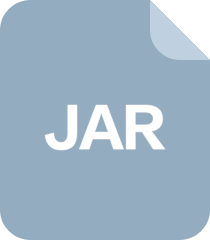
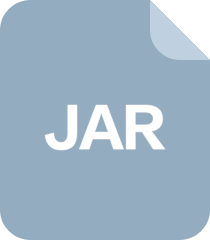
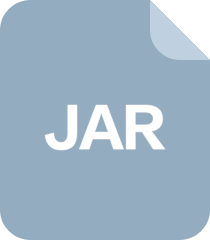
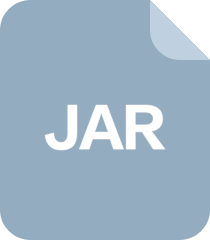
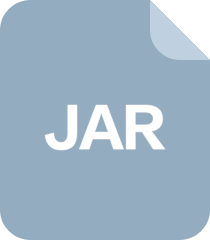
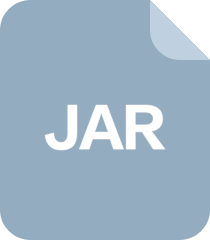
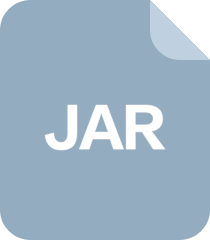
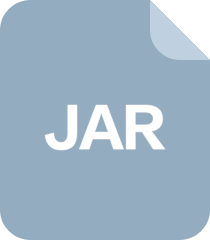
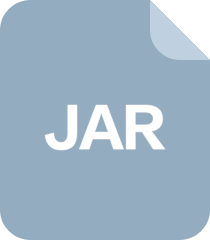
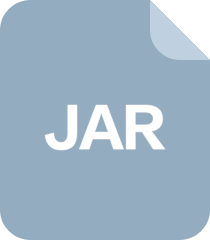
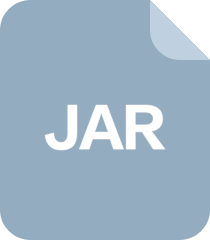
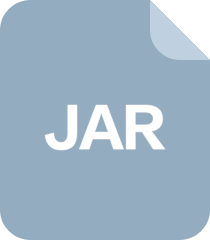
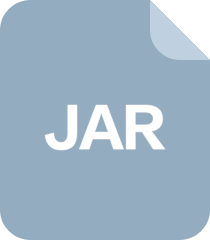
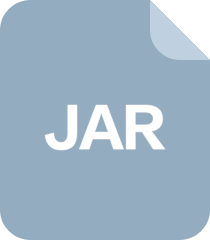
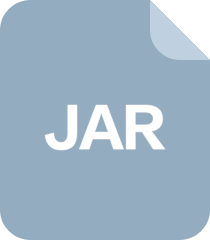
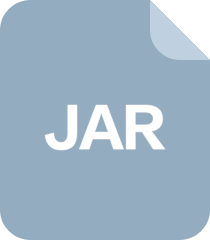
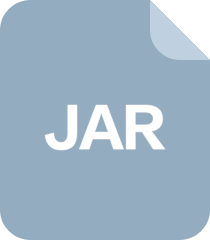
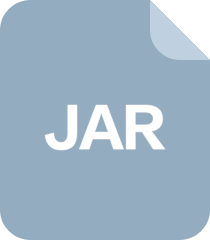
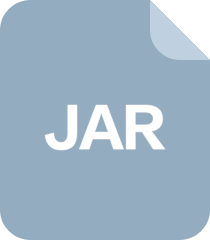
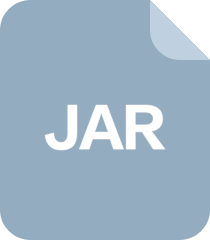
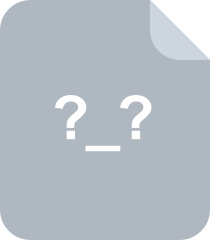
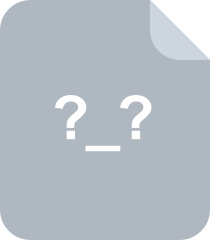
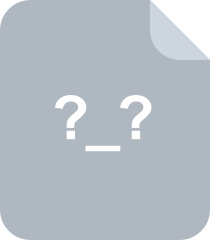
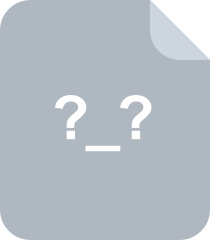
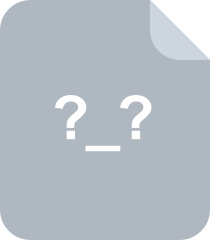
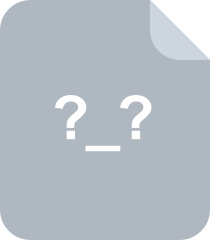
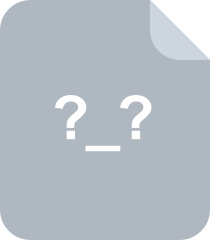
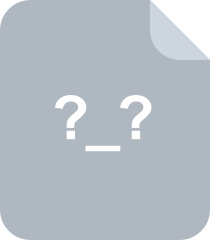
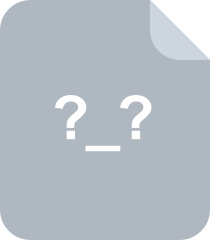
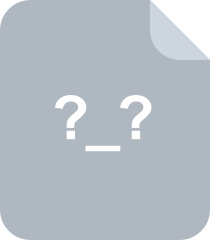
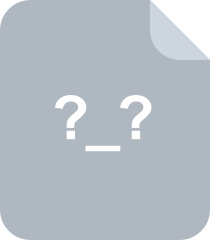
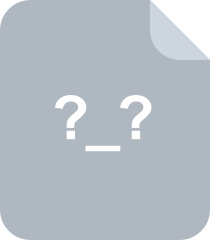
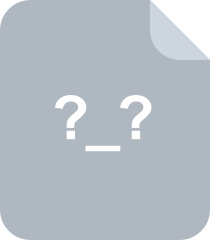
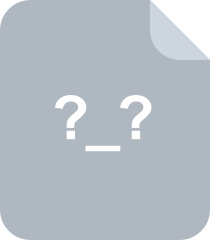
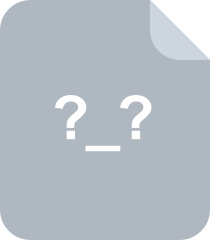
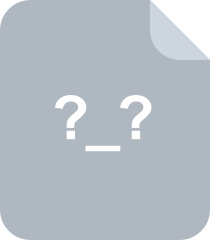
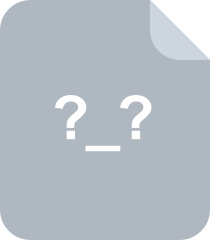
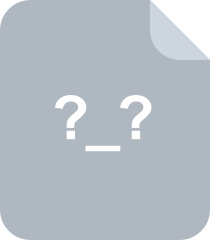
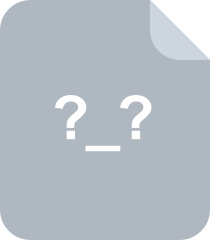
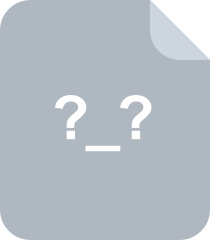
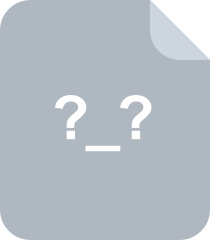
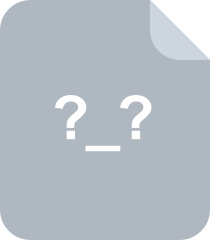
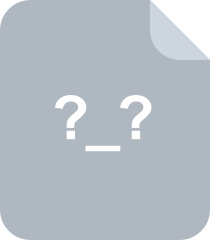
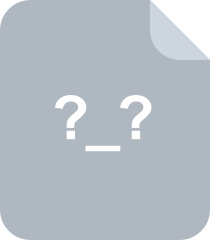
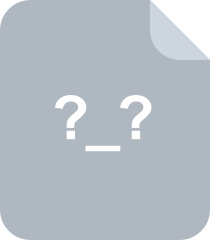
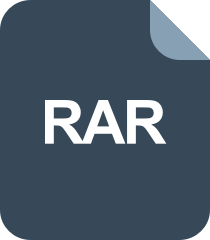
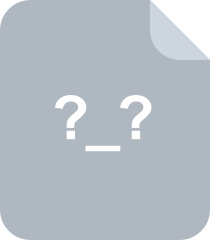
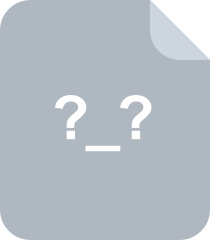
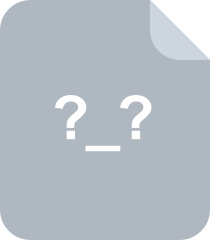
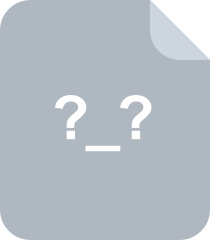
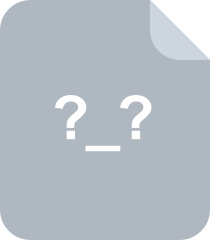
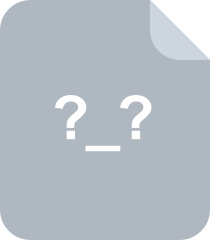
共 115 条
- 1
- 2

秀川001
- 粉丝: 3
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

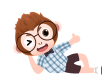
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


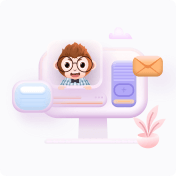
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页