bmp_基于像素统计的相似度检测.rar
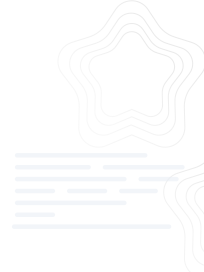


在图像处理领域,"基于像素统计的相似度检测"是一种常用的技术,用于比较两幅图像之间的相似性。这里,我们关注的是与BMP格式图像相关的应用。BMP(Bitmap)是一种未经压缩的位图文件格式,它存储了图像的原始像素数据。在“bmp_基于像素统计的相似度检测.rar”这个压缩包中,很可能包含了一些关于如何利用像素统计方法来评估BMP图像之间相似性的代码、文档或实例。 像素统计是通过分析图像中的每个像素值来理解图像特征的方法。在比较两幅图像的相似性时,我们可以计算它们在像素层面上的差异。常见的方法有以下几种: 1. **均方误差(Mean Squared Error, MSE)**: 这是最简单的相似度指标之一,通过计算两幅图像对应像素的平方差的平均值来评估它们的差异。MSE值越小,图像越相似。 2. **结构相似指数(Structural Similarity Index, SSIM)**: SSIM是一种更高级的相似度测量方法,它考虑了亮度、对比度和结构信息。SSIM值接近1表示两图像高度相似。 3. **归一化交叉相关(Normalized Cross-Correlation, NCC)**: NCC衡量两幅图像在像素级别的线性相关性。值越接近1,表示两图像越相似。 4. **汉明距离(Hamming Distance)**: 如果将图像看作二进制位图,汉明距离可以计算出在哪些位置上像素值不同,距离越小,相似度越高。 5. **余弦相似度(Cosine Similarity)**: 通过计算两个图像向量的夹角余弦值来评估它们的相似度,值越接近1,表示越相似。 6. **像素直方图比较**: 分别计算两幅图像的像素直方图,然后比较它们的差异,如直方图的重叠程度。 在处理BMP图像时,通常需要先读取图像数据,将其转化为合适的矩阵形式,然后用上述方法之一计算相似度。在实际应用中,可能还需要对图像进行预处理,如灰度化、归一化等,以减少噪声影响并提高比较效果。 “bmp_xiangsidu”可能是指一个示例程序或者脚本,用于实现上述的某种或多种像素统计方法。该文件可能包含了源代码、测试图像以及运行结果,供用户学习和参考。通过分析这个文件,你可以深入理解如何在实际项目中实施像素统计的相似度检测,对于理解和应用图像处理技术会有很大帮助。 在图像识别、内容检索、视频监控等领域,基于像素统计的相似度检测是非常关键的技术。它可以帮助我们找出数据库中与目标图像最接近的匹配项,或者检测图像序列中的变化。掌握这种方法,将有助于提升你在这些领域的专业能力。
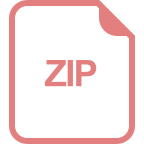
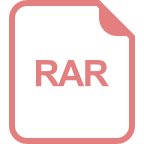
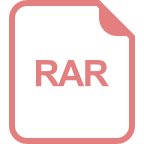
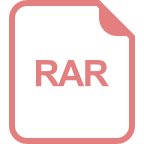
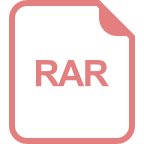
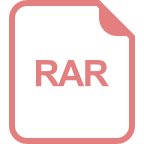
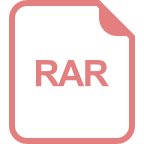
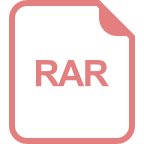
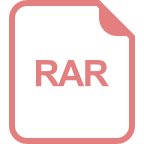
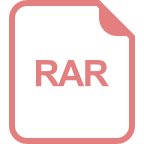
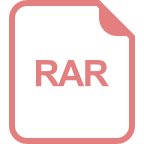
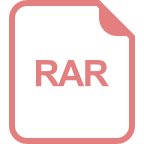
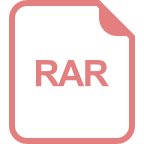
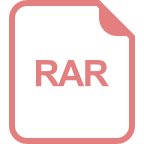
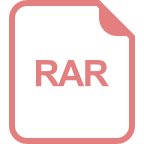
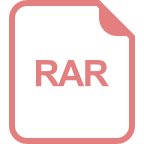
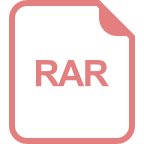
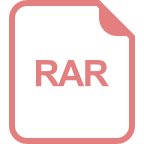
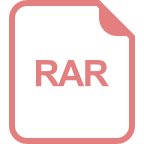
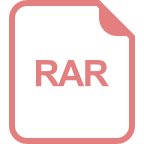
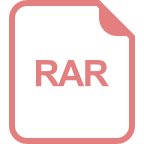
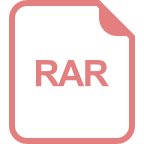
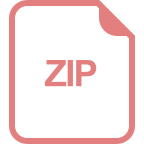
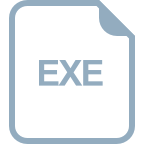


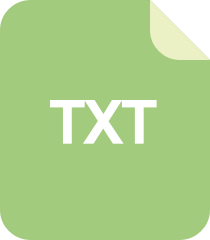
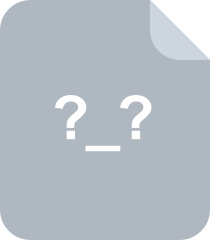


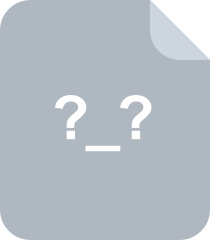
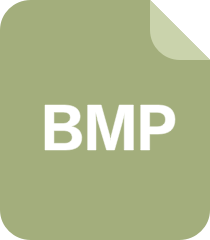
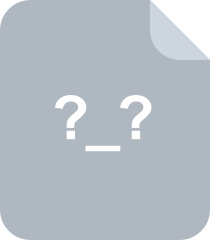
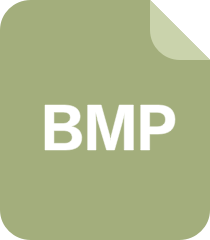
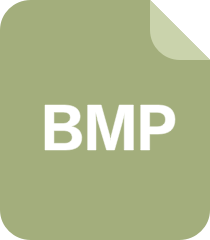
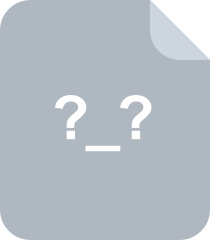
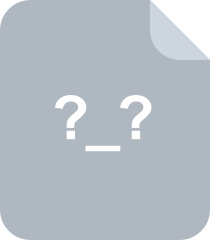
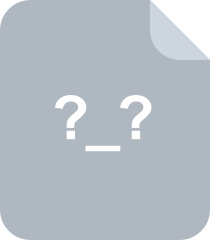
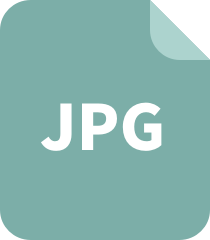
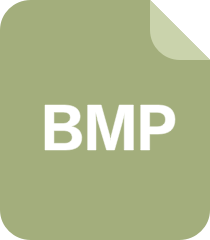
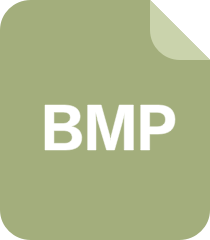
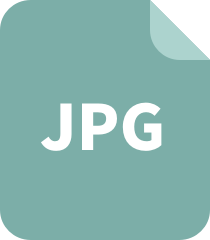
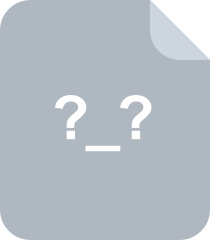

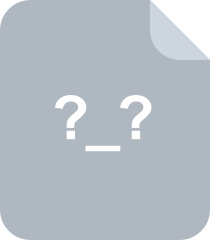
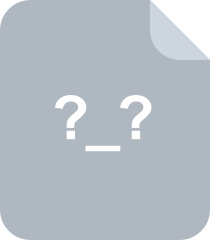
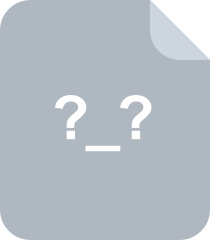
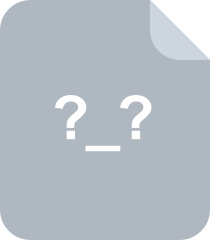
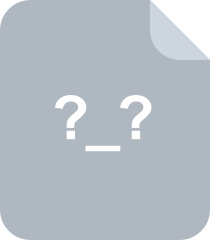
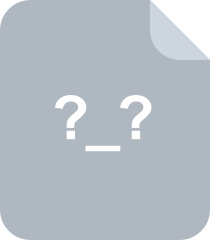
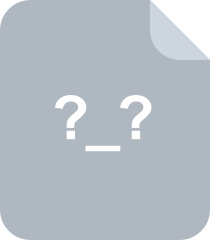
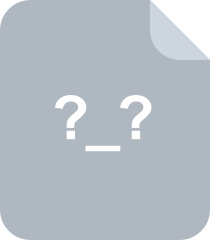
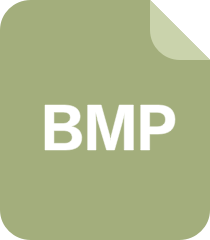
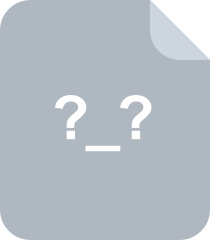
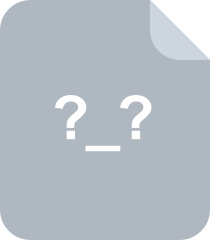

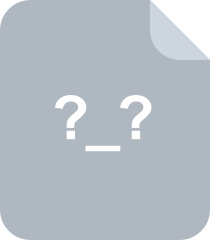
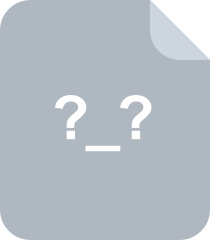
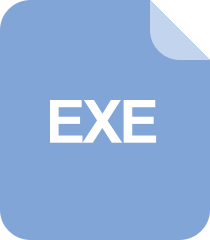
- 1
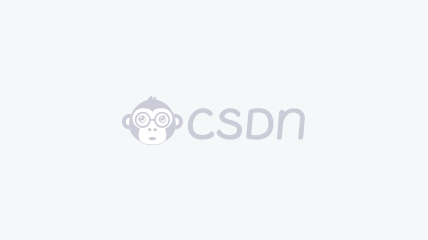
- 豆浆爱蟹蟹2014-10-06功能太简单了
- SrCMpunk2014-03-31运行不了!
- 转向kangqingyu博客2015-05-03质量一般般呀。。。不过价格也不高,还算合适。。

- 粉丝: 4
- 资源: 7
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

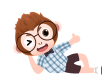
最新资源
- 中部槽双枪自动焊接专机的设计与应用.pdf
- 中东地区炼化工程现场焊接质量的控制.pdf
- 中国焊接工程师培养历程及国际接轨的发展展望.pdf
- 中厚板机器人焊接系统 (2).pdf
- 中厚度钢板单面焊全熔透焊缝焊接技术.pdf
- 中梁上盖板焊接反变形压型工艺探讨.pdf
- 中频电源对焊接工程车发电机干扰的解决方法.pdf
- 中小型安全PLC等安全保护产品在机器人焊接单元的应用.pdf
- 重叠式高压换热器复合金属材料的焊接方法.pdf
- 重型变速箱副箱焊接式行星架开发.pdf
- 中压锅炉过热器管排常用钢种焊接技术探讨.pdf
- 重要产品用低合金钢焊接性能综合评价.pdf
- 重整焊接板式换热器压力降升高原因和处理.pdf
- 轴承保持器焊接变形控制工艺.pdf
- 重载铁路75 kgm钢轨移动闪光焊焊接施工技术.pdf
- 珠光体钢与奥氏体钢异种钢焊接材料的选择与应用.pdf

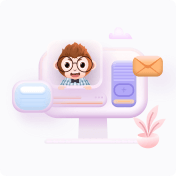
