根据给定的文件标题、描述、标签以及部分内容,我们可以总结并扩展以下相关的IT知识点: ### 一、同时汇出多个Excel的关键技术要点 #### 1. 多个Excel文件的生成 - **目的**:在实际业务场景中,可能需要将不同类别的数据分别导出到不同的Excel文件中,以便于后续的数据分析或分享。 - **实现方法**: - 使用Java编程语言中的相关库(如Apache POI)来创建和操作Excel文件。 - 遍历所有需要导出的数据集合,为每个集合创建一个单独的Excel文件。 #### 2. 文件打包与压缩 - **目的**:将多个Excel文件打包成一个压缩文件(通常为ZIP格式),便于传输和存储。 - **实现方法**: - 使用`ZipOutputStream`类来进行文件的压缩处理。 - 将每个Excel文件添加到压缩包中,并设置相应的文件名。 #### 3. 用户下载功能 - **目的**:允许用户通过Web应用下载打包好的压缩文件。 - **实现方法**: - 将压缩文件作为HTTP响应的一部分返回给客户端。 - 设置正确的HTTP头部信息,确保浏览器能够正确识别文件类型并触发下载行为。 ### 二、关键代码解读 #### 1. 方法签名及参数解释 ```java public void exportMatch(String type, int year, OutputStream out) throws IOException { ``` - **参数说明**: - `type`:指定导出数据的类型。 - `year`:指定导出数据的年份。 - `out`:输出流对象,用于将最终压缩文件写入到客户端。 #### 2. 数据准备与处理 ```java List<SportAgeGroup> deleteAgeGroups = new ArrayList<SportAgeGroup>(); List<SportAgeGroup> ageGroups = sportAgeGroupDao.findAll(); Map<Long, List<SportEventRound>> ageGroupRounds = getAgeGroupRounds(type, year, ageGroups); ``` - **过程说明**: - 调用DAO层的方法获取所有的`SportAgeGroup`对象列表。 - 使用自定义方法`getAgeGroupRounds`获取每个年龄组下的赛事轮次信息。 #### 3. 创建压缩流与数据输出流 ```java ZipOutputStream zip = new ZipOutputStream(out); DataOutputStream data = new DataOutputStream(zip); ``` - **作用**:初始化压缩输出流和数据输出流,以便将Excel文件逐个添加到压缩包中。 #### 4. 循环生成Excel文件并添加到压缩包 ```java for (SportAgeGroup ageGroup : ageGroups) { // ...省略部分代码... HSSFWorkbook workBook = new HSSFWorkbook(); workBook = exportMatchTest(workBook, f, eventRounds, type, year); ZipEntry entry = new ZipEntry(filename); zip.putNextEntry(entry); zip.setEncoding("GBK"); FileInputStream inputStream = new FileInputStream("c:/" + ageGroup.getName() + ".xls"); byte[] buf = new byte[1024]; while (inputStream.read(buf) > 0) { data.write(buf); } zip.closeEntry(); } ``` - **过程说明**: - 对于每个`SportAgeGroup`,创建一个对应的Excel文件。 - 调用`exportMatchTest`方法填充Excel文件的内容。 - 将填充好的Excel文件添加到压缩包中。 #### 5. 清理资源 ```java } finally { zip.close(); deleteFile(deleteAgeGroups); } ``` - **过程说明**: - 关闭压缩输出流。 - 清除临时生成的Excel文件。 ### 三、总结 以上代码示例展示了如何在Java环境中实现多个Excel文件的同时导出,并将其打包压缩后提供给用户下载。该过程涉及到了数据的获取、Excel文件的创建与填充、文件的压缩打包以及最终的文件传输等关键步骤。通过这种方式,可以有效地提升数据导出的效率和用户体验。
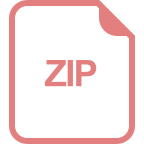
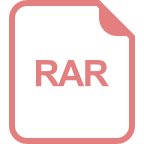
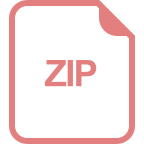
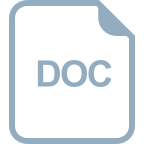
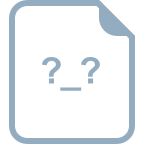
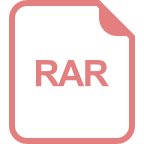
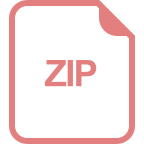
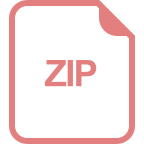
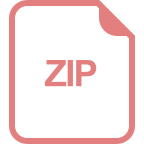
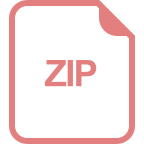
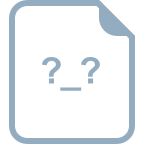
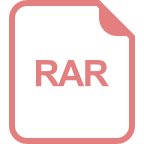
throws IOException {
List<SportAgeGroup> deleteAgeGroups = new ArrayList<SportAgeGroup>();
List<SportAgeGroup> ageGroups = sportAgeGroupDao.findAll();
Map<Long, List<SportEventRound>> ageGroupRounds = getAgeGroupRounds(
type, year, ageGroups);
ZipOutputStream zip = new ZipOutputStream(out);
DataOutputStream data = new DataOutputStream(zip);
try {
for (SportAgeGroup ageGroup : ageGroups) {
FileOutputStream f = new FileOutputStream("c:/"
+ ageGroup.getName() + ".xls");
String filename = ageGroup.getName() + ".xls";
List<SportEventRound> eventRounds = ageGroupRounds.get(ageGroup
.getId());
if (eventRounds.size() > 0) {
deleteAgeGroups.add(ageGroup);
HSSFWorkbook workBook = new HSSFWorkbook();
workBook = exportMatchTest(workBook, f, eventRounds, type,
year);
ZipEntry entry = new ZipEntry(filename);
zip.putNextEntry(entry);
zip.setEncoding("GBK");
FileInputStream inputStream = new FileInputStream("c:/"
+ ageGroup.getName() + ".xls");
byte[] buf = new byte[1024];
while (inputStream.read(buf) > 0) {
data.write(buf);
}
zip.closeEntry();
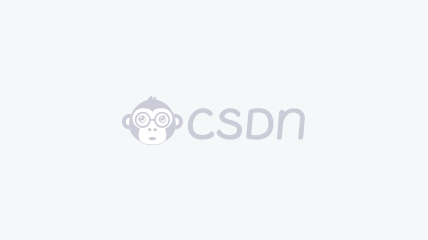

- 粉丝: 6
- 资源: 14
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

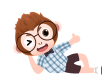
最新资源
- 快速定制中国传统节日头像(源码)
- hcia 复习内容的实验
- 准Z源光伏并网系统MATLAB仿真模型,采用了三次谐波注入法SPWM调制,具有更高的电压利用效率 并网部分采用了电压外环电流内环 电池部分采用了扰动观察法,PO Z源并网和逆变器研究方向的同学可
- 海面目标检测跟踪数据集.zip
- 欧美风格, 节日主题模板
- 西门子1200和三菱FXU通讯程序
- 11种概率分布的拟合与ks检验,可用于概率分析,可靠度计算等领域 案例中提供11种概率分布,具体包括:gev、logistic、gaussian、tLocationScale、Rayleigh、Log
- 机械手自动排列控制PLC与触摸屏程序设计
- uDDS源程序publisher
- 中国风格, 节日 主题, PPT模板

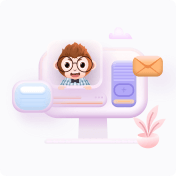
