/*
* 电力检测嵌入式端入口主程序-主界面显示
*
*
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <math.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <sys/time.h>
#include <signal.h>
#include <sys/wait.h>
#include <time.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <sys/ioctl.h>
#include <linux/rtc.h>
#include <errno.h> /*错误号定义*/
#define MWINCLUDECOLORS
#include "nano-X.h"
#include "Elec.h" //包含自定义头文件
//创建共享内存区
char *shmBuf = NULL;
char *shmCfgBuf = NULL;
struct ConfigData *confData;
char chShmID[10],chShmCfgID[10];
int main(int argc,char *argv[])
{
int shmID,shmCfgID;
int pid;
int retval;
if((shmID = shmget(IPC_PRIVATE,sizeof(struct StatData),0666)) < 0)
{
if(DEBUG)
{
printf("Warning:Couldn't shmget!\n");
exit(-1);
}
}
if((shmCfgID = shmget(IPC_PRIVATE,sizeof(struct ConfigData),0666)) < 0)
{
if(DEBUG)
{
printf("Warning:Couldn't shmget config!\n");
exit(-1);
}
}
sprintf(chShmID,"%d",shmID);
sprintf(chShmCfgID,"%d",shmCfgID);
//定义信号处理函数
Signal_Init();
//退出处理
atexit(Close_atexit);
//读取CMOS时间
GetDateTime();
//创建数据采集进程
if((pidData=fork()) == -1)
{
Status_Data = SIGNAL_DATA_PROECESS_ERROR;
if(DEBUG)
{
printf("Warning:Couldn't fork Data process!\n");
exit(-1);
}
}
if(pidData == 0 )//数据采集进程
{
if (execl("./Data","Data",chShmID,NULL)<0)
{
kill(getppid(),SIGNAL_DATA_PROECESS_ERROR);
printf("Warning:execl data process error!\n");
}
}
else
{
////挂载USB盘
//retval = system("mount -t vfat /dev/sda1 /usb");
//if(retval == 127)
// printf("Warning:mount usb /bin/sh not available!\n");
//else if(retval == -1)
// printf("Warning:mount usb error!\n");
//else if(retval != 0)
// printf("Warning:mount usb error!\n");
//else
// printf("OK:mount usb successed!\n");
//创建远程通讯进程
if((pidRemote=fork())==-1)
{
Status_Remote = SIGNAL_REMOTE_PROECESS_ERROR;
if(DEBUG)
{
printf("Warning:Couldn't fork Remote process!\n");
exit(-1);
}
}
if(pidRemote==0)
{
if (execl("./Remote","Remote",chShmID,chShmCfgID,NULL)<0)
{
kill(getppid(),SIGNAL_REMOTE_PROECESS_ERROR);
printf("Warning:Execl remote process error!\n");
}
}
else//主界面程序
{
//附加共享内存区
if((shmBuf = shmat(shmID,0,0))<(char *)0)
{
if(DEBUG)
{
printf("Warning:MainGUI shmat error!\n");
exit(-1);
}
}
dataMem = shmBuf;
//dataMem->Fresh = 0;
dataMem->Busy = 1;
if((shmCfgBuf = shmat(shmCfgID,0,0))<(char *)0)
{
if(DEBUG)
{
printf("Warning:MainGUI shmat config error!\n");
exit(-1);
}
}
//读写内存
confData = shmCfgBuf;
//读取通讯配置文件
if(GetConfig()<=0)
{
//发送错误信号
pid = getpid();
//kill(pid,SIGNAL_CONFIG_ERROR);
exit(-1);
}
InitGUI();
//定时
signal(SIGALRM,(void *)Signal_handle);
SetTimer(1);
//创建界面显示
ShowGUI();
}
}
exit(0);
}
//时间设置
int GetDateTime()
{
int fd, retval;
struct rtc_time rtctime;
struct tm *timer;
time_t t;
//时间设置
fd = open ("/dev/nx8025", O_RDONLY|O_NOCTTY);
if (fd == -1)
{
perror("/dev/nx8025");
exit(errno);
}
retval = ioctl(fd, RTC_RD_TIME, &rtctime);
if (retval == -1)
{
perror("Get DateTime Error!\n");
exit(errno);
}
time(&t);
timer = localtime(&t);
/*printf("nx8025_set_datetime: tm_year = %d\n", rtctime.tm_year+1900);
printf("nx8025_set_datetime: tm_mon = %d\n", rtctime.tm_mon);
printf("nx8025_set_datetime: tm_mday = %d\n", rtctime.tm_mday);
printf("nx8025_set_datetime: tm_hour = %d\n", rtctime.tm_hour);
printf("nx8025_set_datetime: tm_min = %d\n", rtctime.tm_min);
printf("nx8025_set_datetime: tm_sec = %d\n", rtctime.tm_sec);*/
timer->tm_sec = rtctime.tm_sec;
timer->tm_min = rtctime.tm_min;
timer->tm_hour = rtctime.tm_hour;
timer->tm_mday = rtctime.tm_mday;
timer->tm_mon = rtctime.tm_mon;
timer->tm_year = rtctime.tm_year;
close(fd);
SetDateTime(timer);
}
int SetDateTime(struct tm *timer)
{
time_t t;
t = mktime(timer);
stime(&t);
}
int SaveDateTime(struct tm *timer)
{
int fd, retval;
struct rtc_time rtctime;
//时间设置
fd = open ("/dev/nx8025", O_RDONLY|O_NOCTTY);
if (fd == -1)
{
perror("/dev/nx8025");
exit(errno);
}
rtctime.tm_sec = timer->tm_sec ;
rtctime.tm_min = timer->tm_min;
rtctime.tm_hour = timer->tm_hour;
rtctime.tm_mday = timer->tm_mday;
rtctime.tm_mon = timer->tm_mon;
rtctime.tm_year = timer->tm_year;
/*printf ("%d-%d-%d %d:%d:%d\n",timer->tm_year,timer->tm_mon, timer->tm_mday, timer->tm_hour, timer->tm_min, timer->tm_sec);
printf("nx8025_set_datetime: tm_year = %d\n", rtctime.tm_year+1900);
printf("nx8025_set_datetime: tm_mon = %d\n", rtctime.tm_mon);
printf("nx8025_set_datetime: tm_mday = %d\n", rtctime.tm_mday);
printf("nx8025_set_datetime: tm_hour = %d\n", rtctime.tm_hour);
printf("nx8025_set_datetime: tm_min = %d\n", rtctime.tm_min);
printf("nx8025_set_datetime: tm_sec = %d\n", rtctime.tm_sec);*/
retval = ioctl(fd, RTC_SET_TIME, &rtctime);
if (retval == -1)
{
perror("ioctl");
exit(errno);
}
close(fd);
GetDateTime();
}
//******************************界面显示函数**************************************************************//
//加载图片
void LoadImage()
{
if(iidBackImage == NULL)
{
if(!(iidBackImage = GrLoadImageFromFile("Backimage.gif", 0))) {
fprintf(stderr, "Failed to load image \n");
return;
}
imagewindow = GrNewPixmap(WIDTH,HEIGHT, NULL);
GrDrawImageToFit(imagewindow, gc, 0, 0, WIDTH, HEIGHT, iidBackImage);
}
}
void InitGUI()
{
GR_REGION_ID regionid;
GR_FONT_INFO info;
//图形初始化
#if CLIP_POLYGON
GR_POINT points[] = { {0, 0}, {WIDTH, 0}, {WIDTH, HEIGHT}, {0, HEIGHT} };
#else
GR_RECT clip_rect = { 0, 0, WIDTH, HEIGHT };
#endif
if (GrOpen() < 0) //打开
{
printf("Couldn't connect to Nano-X server\n");
exit(6);
}
//创建主窗口
window = GrNewWindowEx(GR_WM_PROPS_APPWINDOW,
"Elec",
GR_ROOT_WINDOW_ID, 0, 0, WIDTH, HEIGHT, BGCOLOR);
//创建临时窗口-绘图时先绘制到临时窗口,再Copy到主窗口
offwindow = GrNewPixmap(WIDTH, HEIGHT, NULL);
GrSelectEvents(window,
GR_EVENT_MASK_EXPOSURE | GR_EVENT_MASK_CLOSE_REQ | GR_EVENT_MASK_KEY_DOWN ); //set window event
GrMapWindow(window); //show window
gc = GrNewGC();
GrSetGCUseBackground(gc, GR_FALSE);
GrSetGCBackground(gc, BGCOLOR);
//设置GC区域
#if CLIP_POLYGON
/* polygon clip region */
regionid = GrNewPolygonRegion(MWPOLY_EVENODD, 3, points);
#else
/* rectangle clip region */
regionid = GrNewRegion();
GrUnionRectWithRegion(regionid, &clip_rect);
#endif
GrSetGCRegion(gc, regionid);
//设置显示字体
fontid = GrCreateFont(fontname, 0, NULL);
GrSetFontAttr(fontid, GR_TFKERNING | GR_TFANTIALIAS, 0);
GrSetFontSize(fontid,3);
GrSetGCFont(gc, fontid);
GrGetFontInfo(fontid, &info);
font_height=info.maxwidth;
LoadImage();
}
void ShowGUI()
{
//GrSetFontSize(fontid,1);
//GrSetFontRotation(fontid, 330); /* 33 degrees */
//消息循环
while (1)
{
GR_EVENT event;
//显示主菜单
MainMenu();
GrGetNextEvent(&event);
switch(event.type)
{
case GR_EVENT_TYPE_CLOSE_REQ:
GrClose();
case GR_EVENT_TYPE_KEY_DOWN:
{
//putchar(event.keystroke.ch);
//printf("\nKey:%c\n",event.keystroke.ch);
switch(event.keystroke.ch)
{
case KEY_F1: //谐波显示
ClearWindow(offwindow);
ShowXBMenu();
break;
case KEY_F2: //波形显示
ClearWindow(offwindow);
ShowWaveMenu();
break;
ca
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
这是一个用ARM9开发版开发的一个嵌入式linux图形界面系统(nano-X实现,即microwindows)-支持socket通讯,GPRS拨号通讯,Modem拨号通讯和串口通讯(已去掉此功能),支持usb设备。<br> 公布源代码可以方便大家学习嵌入式系统编程,收取资源分,是因为不得已,本人的资源分已经用完,下不了自己想要的资源,只能先收取部分资源分,已缓解压力,如有不便,还请原谅。
资源推荐
资源详情
资源评论
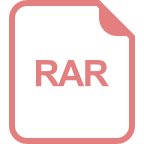
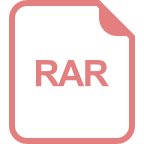
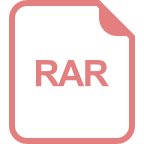
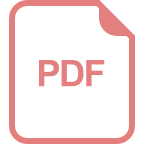
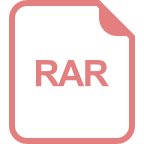
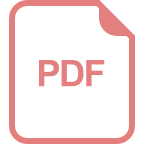
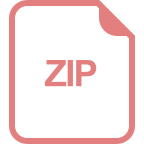
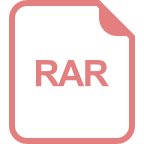
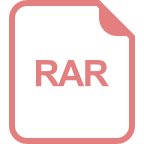
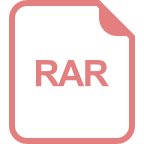
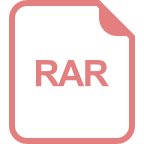
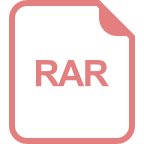
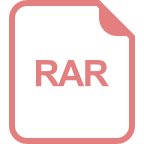
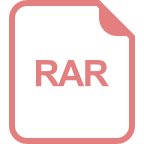
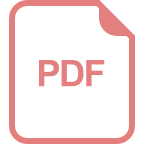
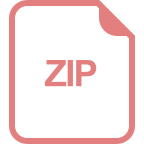
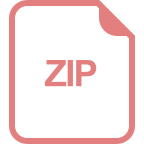
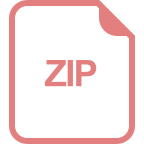
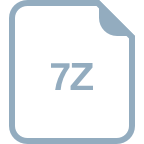
收起资源包目录


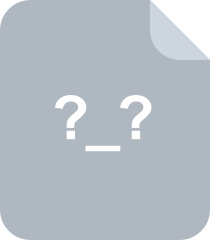
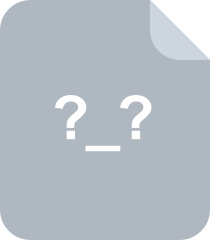

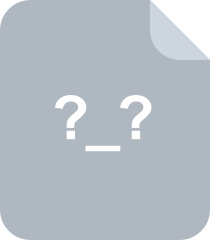
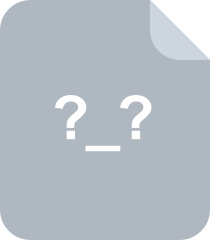
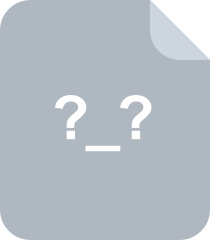
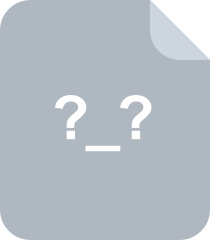

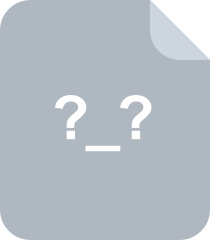
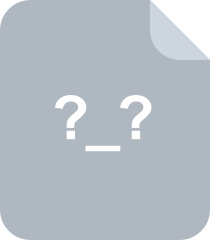


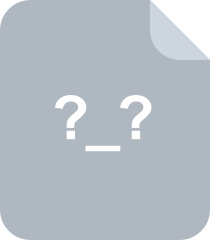
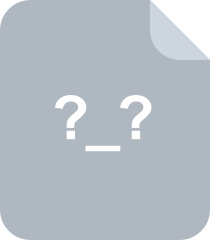
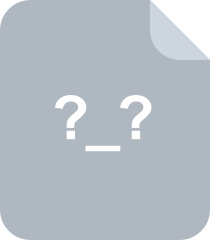
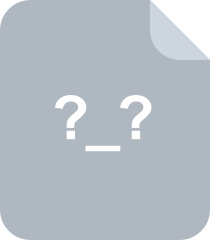
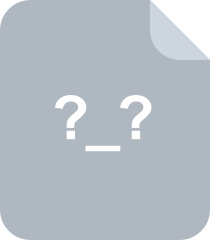

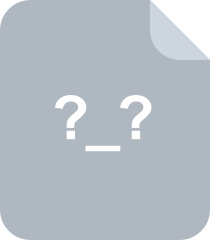
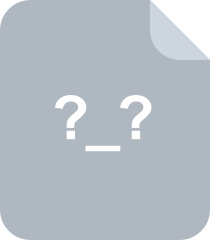
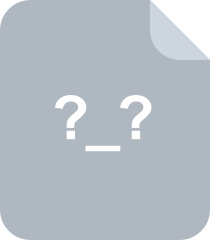
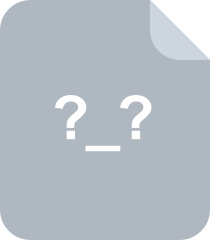
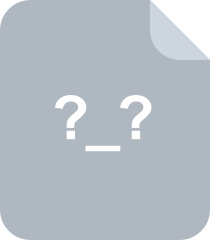

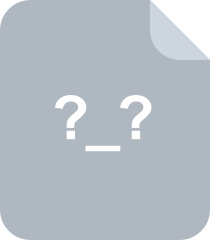
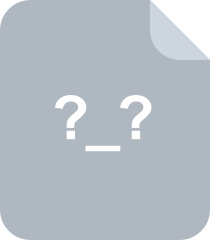
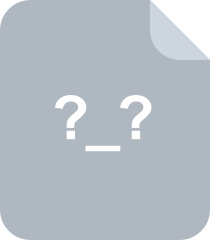
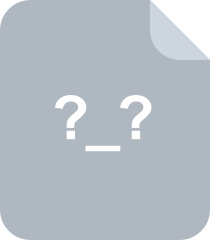


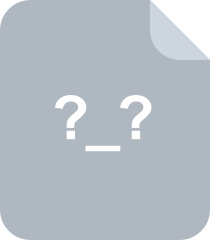
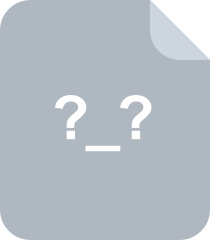
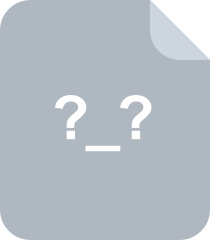
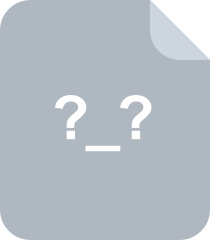
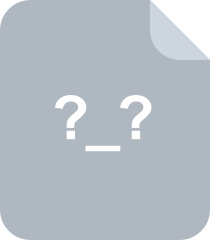
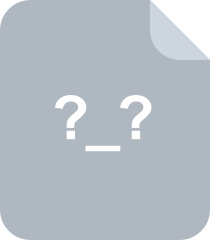
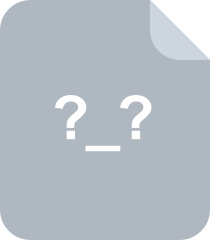
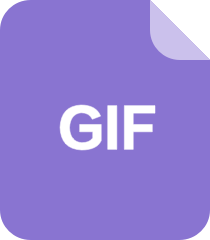
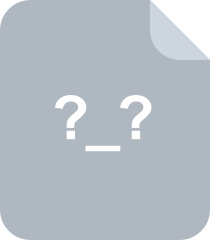
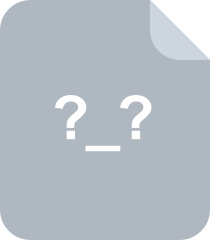


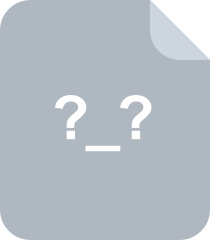

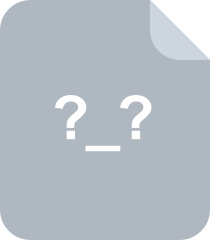
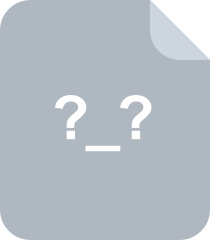

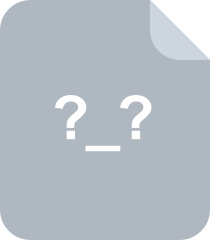

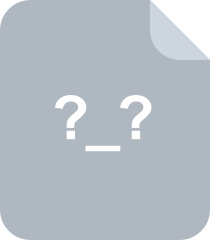
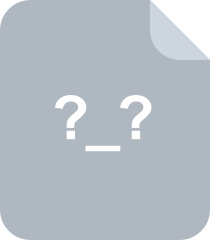
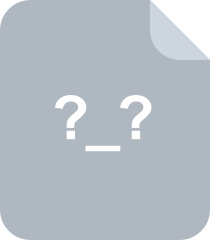
共 39 条
- 1
资源评论
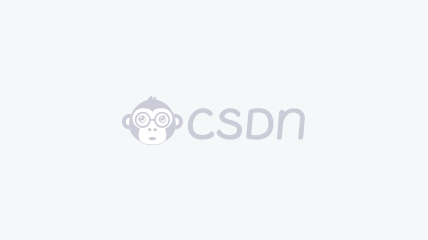
- slsir2014-07-23不错,可以学习一下
- laseren2013-01-03还不错,部分可用。呵呵。
- QT初学者20222013-11-25还不错,部分可用。呵呵。
- 查尔斯风2015-10-02非常好,刚刚好可以拿来参考!

flybird_lt
- 粉丝: 6
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

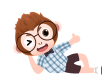
最新资源
- JSP-JTBC-CMS(SQLITE).rar
- MC3362和MC145151调频无线接收器的设计.pdf
- MiniRenamer-v100.0一款简单易用的批量文件重命名工具(已注册PRO版本).rar
- 小狐狸Ai系统 小狐狸ai付费创作系统V2.8.0 ChatGPT智能机器人
- 公孙离-内衣-肚兜.zipgsl
- 快慢指针判断链表是否有环-go 语言实现
- 学生成绩管理系统的设计与实现-收藏备用.pdf
- JSP+SQL网站流量统计管理系统(源代码+论文).rar
- IBM-PC-XT微机过程...道中模拟量数据的采集和处理.pdf
- JSP+SQL网上选课系统(源代码+论文+答辩PPT).rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


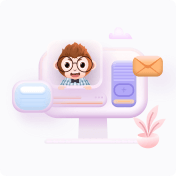
安全验证
文档复制为VIP权益,开通VIP直接复制
