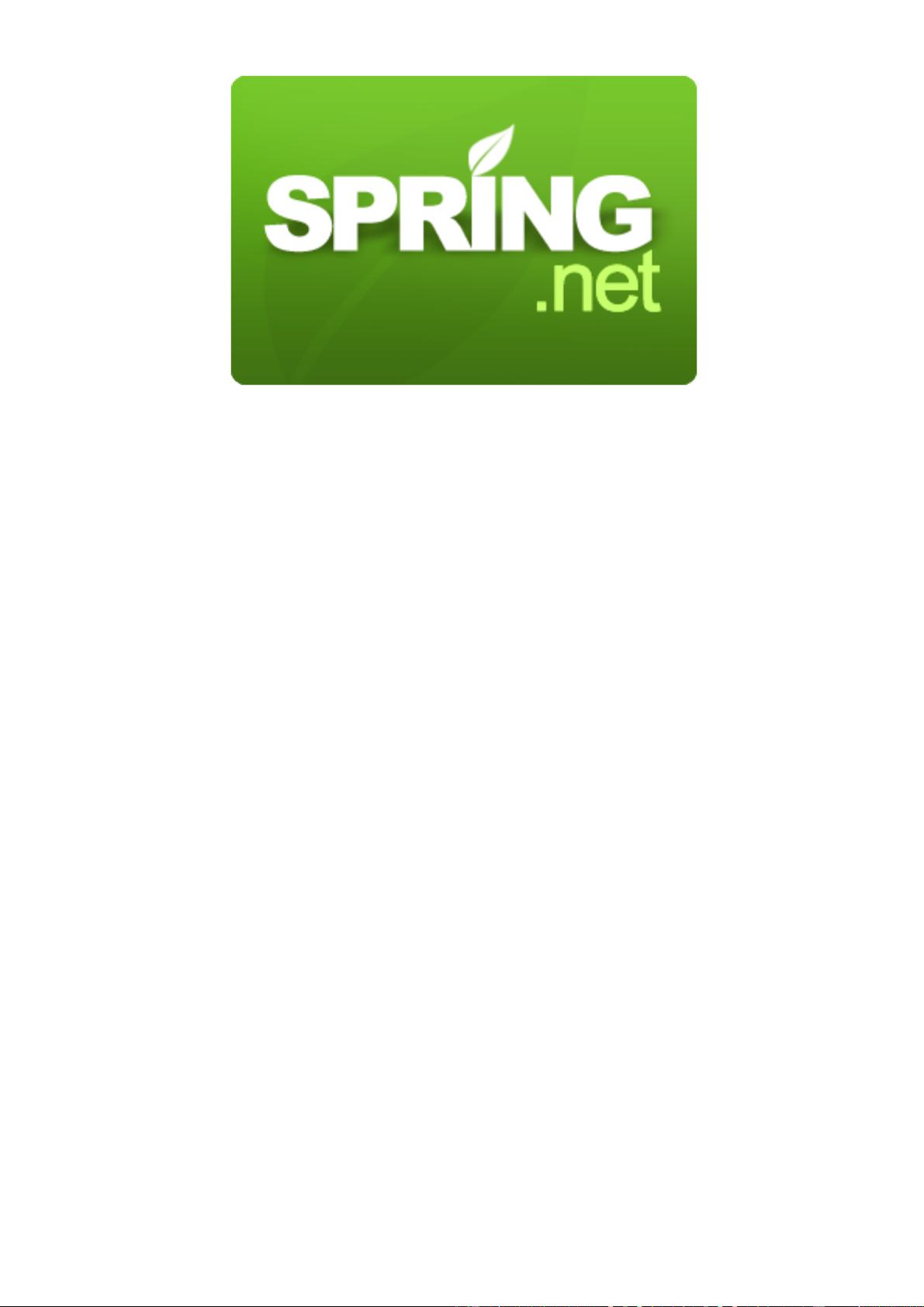
Reference Documentation
Version 1.3.2
Last Updated August 1, 2011 (Latest documentation)
Copyright © 2004-2008 Mark Pollack, Rick Evans, Aleksandar Seovic, Bruno
Baia, Erich Eichinger, Federico Spinazzi, Rob Harrop, Griffin Caprio, Ruben
Bartelink, Choy Rim, Erez Mazor, Stephen Bohlen, The Spring Java Team
Copies of this document may be made for your own use and for distribution to others,
provided that you do not charge any fee for such copies and further provided that
each copy contains this Copyright Notice, whether distributed in print or electronically.
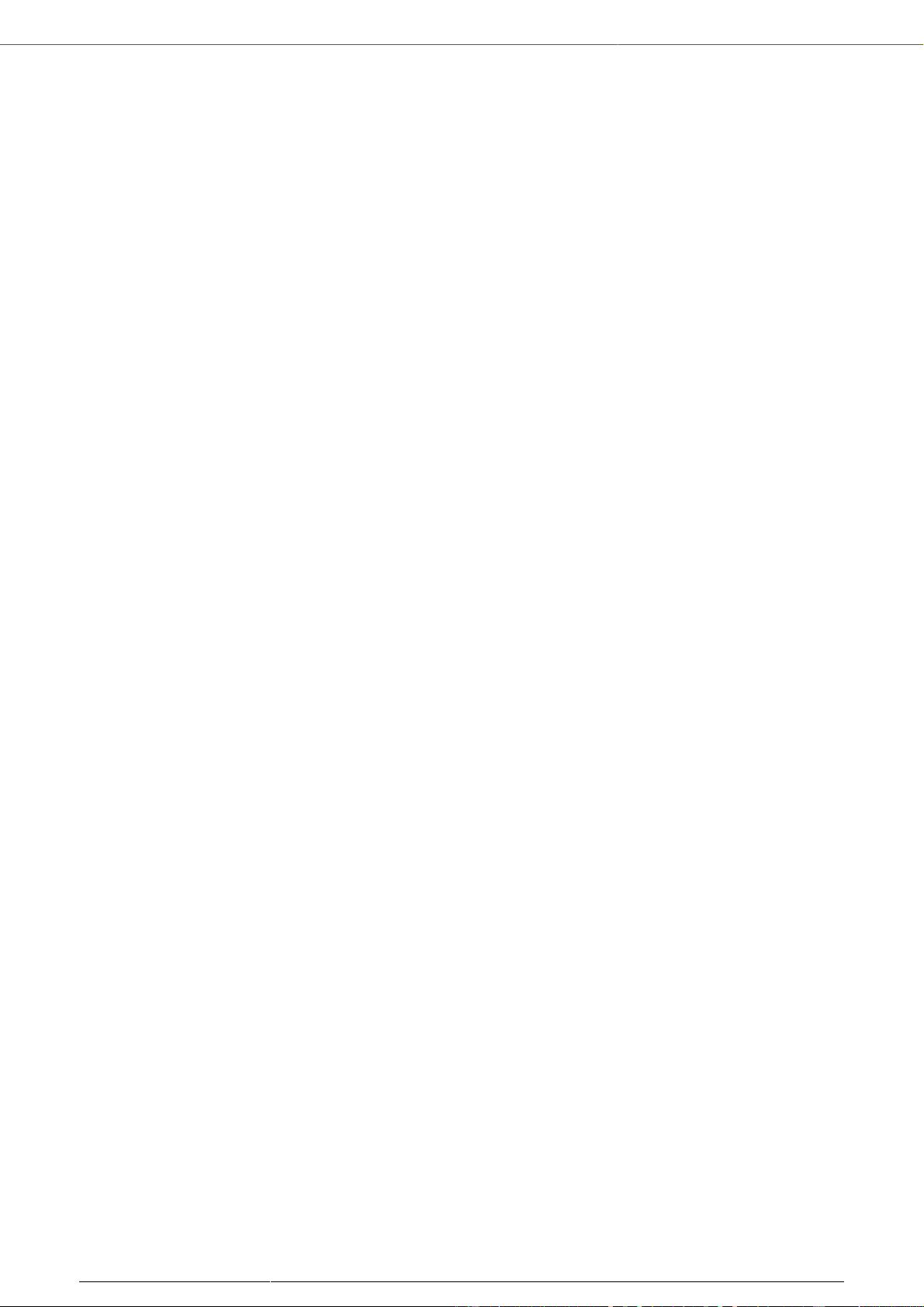
Spring Framework (Version 1.3.2) ii
1. Preface ................................................................................................................................................ 1
2. Introduction ......................................................................................................................................... 2
2.1. Overview .................................................................................................................................... 2
2.2. Background ................................................................................................................................. 2
2.3. Modules ...................................................................................................................................... 3
2.4. Usage Scenarios .......................................................................................................................... 4
2.5. Quickstart applications ................................................................................................................. 5
2.6. Associated Spring.NET Projects ................................................................................................... 6
2.7. License Information ..................................................................................................................... 6
2.8. Support ....................................................................................................................................... 6
3. Background information ....................................................................................................................... 7
3.1. Inversion of Control .................................................................................................................... 7
4. Migrating from 1.1 M2 ........................................................................................................................ 8
4.1. Introduction ................................................................................................................................. 8
4.2. Important Changes ...................................................................................................................... 8
4.2.1. Namespaces .................................................................................................................. 8
4.2.2. Core ............................................................................................................................. 9
4.2.3. Web ............................................................................................................................. 9
4.2.4. Data ............................................................................................................................. 9
4.3. Support for .NET 4 ...................................................................................................................... 9
I. Core Technologies .............................................................................................................................. 10
5. The IoC container ........................................................................................................................ 11
5.1. Introduction ................................................................................................................... 11
5.2. Container overview ........................................................................................................ 11
5.2.1. Configuration metadata ............................................................................. 12
5.2.2. Instantiating a container ............................................................................ 13
5.2.3. Using the container ................................................................................... 17
5.2.4. Object definition overview ........................................................................ 17
5.2.5. Instantiating objects .................................................................................. 19
5.2.6. Object creation of generic types ................................................................. 21
5.3. Dependencies ................................................................................................................ 23
5.3.1. Dependency injection ................................................................................ 23
5.3.2. Dependencies and configuration in detail .................................................... 30
5.3.3. Declarative Event Listener Registration ...................................................... 39
5.3.4. Using depends-on ..................................................................................... 41
5.3.5. Lazily-initialized objects ............................................................................ 41
5.3.6. Autowiring collaborators ........................................................................... 42
5.3.7. Checking for dependencies ........................................................................ 43
5.3.8. Method injection ....................................................................................... 44
5.3.9. Setting a reference using the members of other objects and classes. ............... 47
5.3.10. Provided IFactoryObject implementations ................................................. 51
5.4. Object Scopes ............................................................................................................... 51
5.4.1. The singleton scope .................................................................................. 52
5.4.2. The prototype scope .................................................................................. 52
5.4.3. Singleton objects with prototype-object dependencies .................................. 53
5.4.4. Request, session and web application scopes ............................................... 53
5.5. Type conversion ............................................................................................................ 53
5.5.1. Type Conversion for Enumerations ............................................................ 54
5.5.2. Built-in TypeConverters ............................................................................ 54
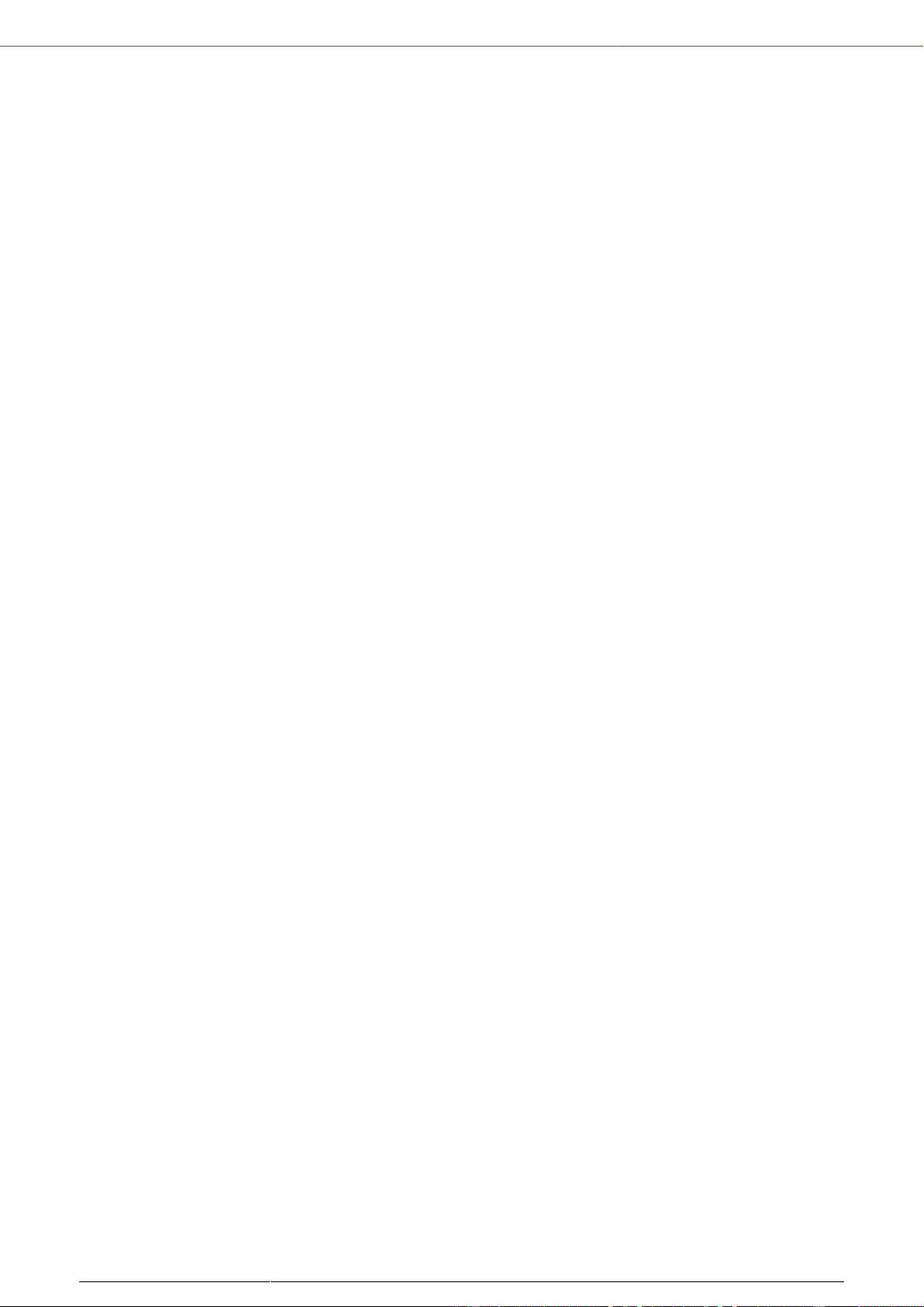
The Spring.NET Framework
Spring Framework (Version 1.3.2) iii
5.5.3. Custom Type Conversion .......................................................................... 55
5.6. Customizing the nature of an object ................................................................................ 57
5.6.1. Lifecycle interfaces ................................................................................... 57
5.6.2. IApplicationContextAware and IObjectNameAware .................................... 58
5.7. Object definition inheritance .......................................................................................... 59
5.8. Container extension points ............................................................................................. 60
5.8.1. Obtaining an IFactoryObject, not its product ............................................... 61
5.9. Container extension points ............................................................................................. 61
5.9.1. Customizing objects with IObjectPostProcessors ......................................... 61
5.9.2. Customizing configuration metadata with IObjectFactoryPostProcessors ....... 66
5.9.3. Customizing instantiation logic using IFactoryObjects ................................. 74
5.10. The IApplicationContext .............................................................................................. 75
5.10.1. IObjectFactory or IApplicationContext? .................................................... 76
5.11. Configuration of IApplicationContext ........................................................................... 77
5.11.1. Registering custom parsers ...................................................................... 78
5.11.2. Registering custom resource handlers ....................................................... 78
5.11.3. Registering Type Aliases ......................................................................... 79
5.11.4. Registering Type Converters .................................................................... 80
5.12. Added functionality of the IApplicationContext ............................................................. 80
5.12.1. Context Hierarchies ................................................................................. 80
5.12.2. Using IMessageSource ............................................................................ 81
5.12.3. Using resources within Spring.NET .......................................................... 83
5.12.4. Loosely coupled events ........................................................................... 83
5.12.5. Event notification from IApplicationContext ............................................. 84
5.13. Customized behavior in the ApplicationContext ............................................................. 86
5.13.1. The IApplicationContextAware marker interface ....................................... 86
5.13.2. The IObjectPostProcessor ........................................................................ 86
5.13.3. The IObjectFactoryPostProcessor ............................................................. 86
5.13.4. The PropertyPlaceholderConfigurer .......................................................... 86
5.14. Configuration of ApplicationContext without using XML ............................................... 87
5.15. Service Locator access ................................................................................................. 87
5.16. Stereotype attributes ..................................................................................................... 88
6. The IObjectWrapper and Type conversion ..................................................................................... 89
6.1. Introduction ................................................................................................................... 89
6.2. Manipulating objects using the IObjectWrapper ............................................................... 89
6.2.1. Setting and getting basic and nested properties ............................................ 89
6.2.2. Other features worth mentioning ................................................................ 91
6.3. Type conversion ............................................................................................................ 91
6.3.1. Type Conversion for Enumerations ............................................................ 92
6.4. Built-in TypeConverters ................................................................................................. 92
6.4.1. Custom type converters ............................................................................. 93
7. Resources .................................................................................................................................... 94
7.1. Introduction ................................................................................................................... 94
7.2. The IResource interface ................................................................................................. 94
7.3. Built-in IResource implementations ................................................................................ 95
7.3.1. Registering custom IResource implementations ........................................... 95
7.4. The IResourceLoader ..................................................................................................... 96
7.5. The IResourceLoaderAware interface ............................................................................. 96
7.6. Application contexts and IResource paths ....................................................................... 97
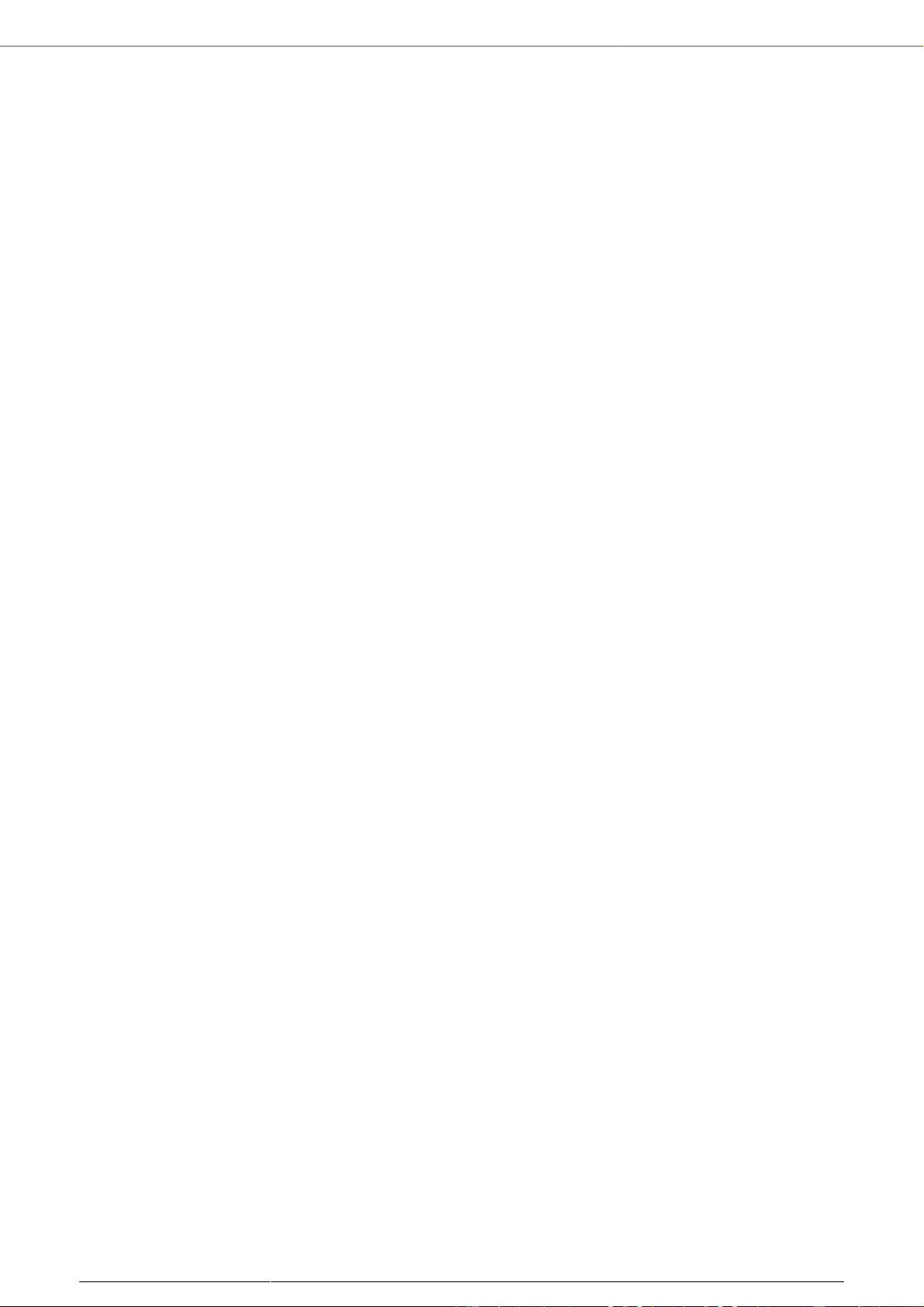
The Spring.NET Framework
Spring Framework (Version 1.3.2) iv
8. Threading and Concurrency Support ............................................................................................. 98
8.1. Introduction ................................................................................................................... 98
8.2. Thread Local Storage ..................................................................................................... 98
8.3. Synchronization Primitives ............................................................................................. 99
8.3.1. ISync ........................................................................................................ 99
8.3.2. SyncHolder ............................................................................................... 99
8.3.3. Latch ...................................................................................................... 100
8.3.4. Semaphore .............................................................................................. 100
9. Object Pooling ........................................................................................................................... 102
9.1. Introduction ................................................................................................................. 102
9.2. Interfaces and Implementations ..................................................................................... 102
10. Spring.NET miscellanea ............................................................................................................ 103
10.1. Introduction ............................................................................................................... 103
10.2. PathMatcher ............................................................................................................... 103
10.2.1. General rules ......................................................................................... 103
10.2.2. Matching filenames ............................................................................... 103
10.2.3. Matching subdirectories ......................................................................... 104
10.2.4. Case does matter, slashes don't ............................................................... 104
11. Expression Evaluation ............................................................................................................... 106
11.1. Introduction ............................................................................................................... 106
11.2. Evaluating Expressions ............................................................................................... 106
11.3. Language Reference ................................................................................................... 107
11.3.1. Literal expressions ................................................................................. 107
11.3.2. Properties, Arrays, Lists, Dictionaries, Indexers ....................................... 108
11.3.3. Methods ................................................................................................ 109
11.3.4. Operators .............................................................................................. 109
11.3.5. Assignment ........................................................................................... 112
11.3.6. Expression lists ..................................................................................... 112
11.3.7. Types .................................................................................................... 112
11.3.8. Type Registration .................................................................................. 113
11.3.9. Constructors .......................................................................................... 113
11.3.10. Variables ............................................................................................. 113
11.3.11. Ternary Operator (If-Then-Else) ........................................................... 114
11.3.12. List Projection and Selection ................................................................ 114
11.3.13. Collection Processors and Aggregators .................................................. 115
11.3.14. Spring Object References ..................................................................... 118
11.3.15. Lambda Expressions ............................................................................ 119
11.3.16. Delegate Expressions ........................................................................... 120
11.3.17. Null Context ....................................................................................... 120
11.4. Classes used in the examples ...................................................................................... 120
12. Validation Framework .............................................................................................................. 122
12.1. Introduction ............................................................................................................... 122
12.2. Example Usage .......................................................................................................... 122
12.3. Validator Groups ....................................................................................................... 124
12.4. Validators .................................................................................................................. 124
12.4.1. Condition Validator ............................................................................... 124
12.4.2. Required Validator ................................................................................ 125
12.4.3. Regular Expression Validator ................................................................. 125
12.4.4. Generic Validator .................................................................................. 126
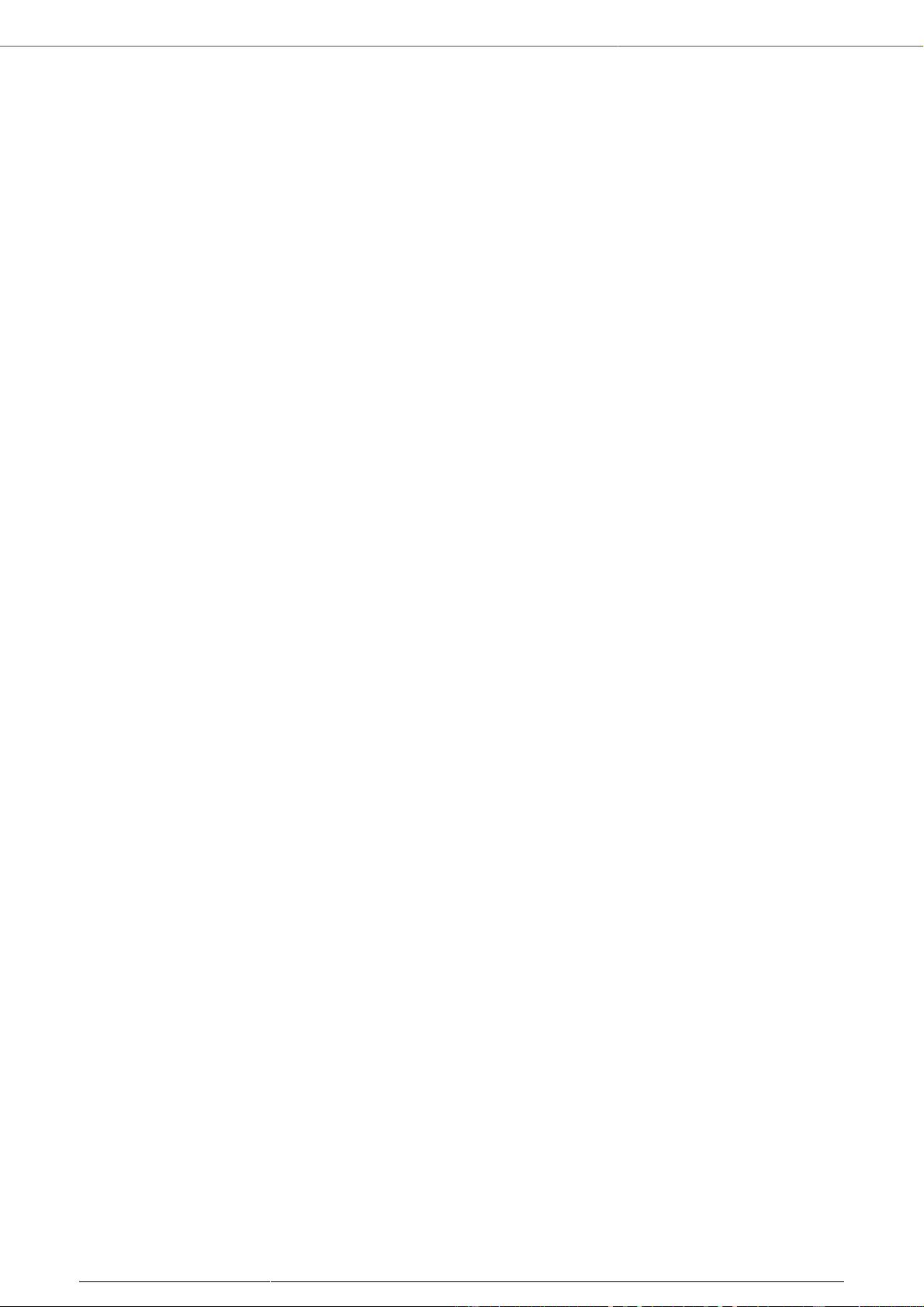
The Spring.NET Framework
Spring Framework (Version 1.3.2) v
12.4.5. Conditional Validator Execution ............................................................. 126
12.5. Validator Actions ....................................................................................................... 127
12.5.1. Error Message Action ............................................................................ 127
12.5.2. Exception Action ................................................................................... 127
12.5.3. Generic Actions .................................................................................... 128
12.6. Validator References .................................................................................................. 128
12.7. Progammatic usage .................................................................................................... 129
12.8. Usage tips within ASP.NET ....................................................................................... 129
12.8.1. Rendering Validation Errors ................................................................... 130
12.8.2. How Validate() and Validation Controls play together ............................. 131
13. Aspect Oriented Programming with Spring.NET ........................................................................ 133
13.1. Introduction ............................................................................................................... 133
13.1.1. AOP concepts ....................................................................................... 133
13.1.2. Spring.NET AOP capabilities ................................................................. 134
13.1.3. AOP Proxies in Spring.NET .................................................................. 135
13.2. Pointcut API in Spring.NET ....................................................................................... 135
13.2.1. Concepts ............................................................................................... 136
13.2.2. Operations on pointcuts ......................................................................... 136
13.2.3. Convenience pointcut implementations ................................................... 137
13.2.4. Custom pointcuts ................................................................................... 139
13.3. Advice API in Spring.NET ......................................................................................... 140
13.3.1. Advice Lifecycle ................................................................................... 140
13.3.2. Advice types ......................................................................................... 140
13.4. Advisor API in Spring.NET ....................................................................................... 145
13.5. Using the ProxyFactoryObject to create AOP proxies ................................................... 145
13.5.1. Basics ................................................................................................... 146
13.5.2. ProxyFactoryObject Properties ............................................................... 146
13.5.3. Proxying Interfaces ................................................................................ 147
13.5.4. Proxying Classes ................................................................................... 149
13.5.5. Concise proxy definitions ...................................................................... 149
13.6. Proxying mechanisms ................................................................................................. 150
13.6.1. InheritanceBasedAopConfigurer ............................................................. 151
13.7. Creating AOP Proxies Programatically with the ProxyFactory ...................................... 151
13.8. Manipulating Advised Objects .................................................................................... 152
13.9. Using the "autoproxy" facility .................................................................................... 153
13.9.1. Autoproxy object definitions .................................................................. 153
13.9.2. Using attribute-driven auto-proxying ...................................................... 158
13.10. Using AOP Namespace ............................................................................................ 158
13.11. Using TargetSources ................................................................................................ 159
13.11.1. Hot swappable target sources ............................................................... 160
13.11.2. Pooling target sources .......................................................................... 160
13.11.3. Prototype target sources ....................................................................... 161
13.11.4. ThreadLocal target sources ................................................................... 162
13.12. Defining new Advice types ....................................................................................... 162
13.13. Further reading and resources ................................................................................... 162
14. Aspect Library .......................................................................................................................... 163
14.1. Introduction ............................................................................................................... 163
14.2. Caching ..................................................................................................................... 163
14.3. Exception Handling .................................................................................................... 165