在PHP中,文件上传是网站开发中常见的功能,无论是单文件还是多文件上传,都是为了方便用户上传数据到服务器。本篇文章将详细讲解如何实现多文件和单文件上传,并涉及支持格式限制以及图片缩略图的生成。 单文件上传在PHP中主要通过`<input type="file">`标签来实现,用户选择一个文件后,表单提交到服务器,PHP通过全局变量`$_FILES`来获取上传的信息。例如: ```php <?php if(isset($_FILES['file'])) { $file = $_FILES['file']; // 检查文件是否上传成功 if($file['error'] == UPLOAD_ERR_OK) { // 文件名、临时路径、类型、大小等信息 $fileName = $file['name']; $tmpName = $file['tmp_name']; $fileType = $file['type']; $fileSize = $file['size']; // 进行文件移动、验证或处理 } } ?> ``` 多文件上传则需要使用HTML5的`multiple`属性,配合PHP的循环处理: ```html <input type="file" name="files[]" multiple> ``` PHP端代码: ```php <?php if(isset($_FILES['files']) && count($_FILES['files']['name']) > 0) { foreach ($_FILES['files']['name'] as $key => $value) { $file = $_FILES['files']; // 检查每个文件的错误码 if($file['error'][$key] == UPLOAD_ERR_OK) { $fileName = $file['name'][$key]; $tmpName = $file['tmp_name'][$key]; $fileType = $file['type'][$key]; $fileSize = $file['size'][$key]; // 对每个文件进行处理 } } } ?> ``` 在上传过程中,为了确保安全和防止恶意文件,我们需要限制上传文件的类型。这可以通过检查文件扩展名实现: ```php $allowedTypes = array('jpg', 'jpeg', 'png', 'gif'); $fileTypeExt = pathinfo($fileName, PATHINFO_EXTENSION); if(!in_array($fileTypeExt, $allowedTypes)) { echo "只允许上传 {$allowedTypes} 类型的文件"; } ``` 对于图片文件,我们还可能需要生成缩略图。PHP可以使用GD库或者Imagick来实现。以GD库为例,创建缩略图的步骤如下: 1. 加载原图: ```php $image = imagecreatefromstring(file_get_contents($tmpName)); ``` 2. 获取原图宽高: ```php $width = imagesx($image); $height = imagesy($image); ``` 3. 计算缩略图的宽高,保持比例: ```php $thumbWidth = 100; // 缩略图宽度 $ratio = $width / $height; if($ratio > 1) { $thumbHeight = $thumbWidth / $ratio; } else { $thumbWidth = $thumbHeight * $ratio; } ``` 4. 创建缩略图: ```php $thumbnail = imagecreatetruecolor($thumbWidth, $thumbHeight); ``` 5. 复制并调整原图到缩略图: ```php imagecopyresampled($thumbnail, $image, 0, 0, 0, 0, $thumbWidth, $thumbHeight, $width, $height); ``` 6. 保存缩略图: ```php imagejpeg($thumbnail, 'thumbnail_' . $fileName); ``` 7. 释放内存: ```php imagedestroy($image); imagedestroy($thumbnail); ``` 通过以上代码,你可以实现多文件和单文件的上传,同时限制上传文件的类型,并生成缩略图。在实际应用中,还需要考虑错误处理、文件重命名、大小限制等问题,以提高文件上传功能的稳定性和安全性。记得在服务器端设置合适的文件上传大小限制,避免因文件过大导致的上传失败。
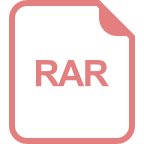
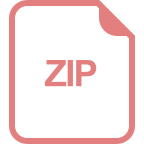
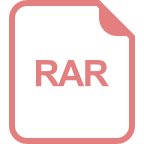
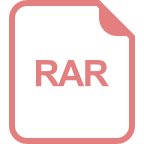
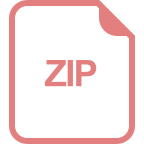
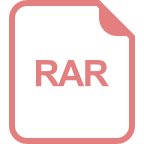
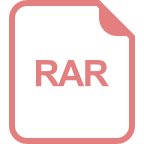
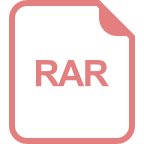
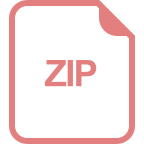
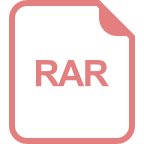
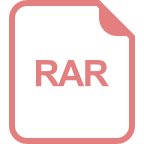
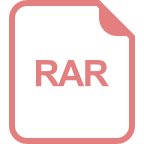
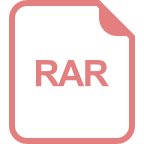
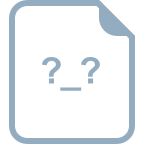
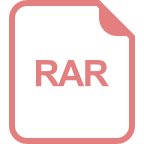
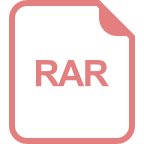
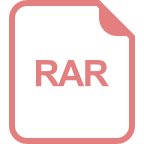
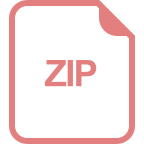
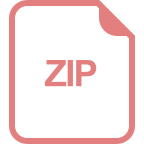
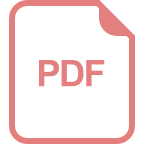
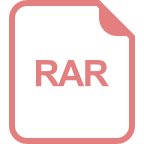
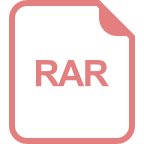
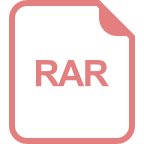
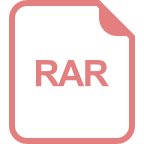
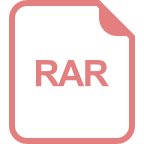
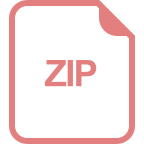
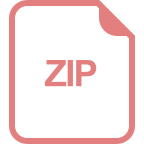
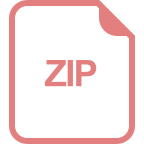
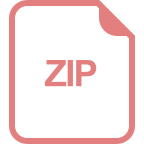

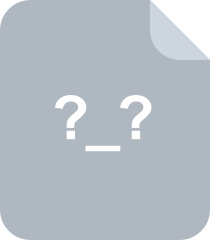
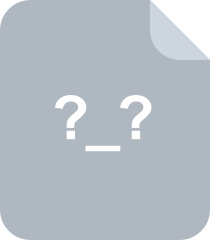
- 1
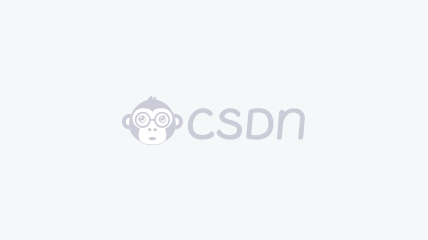

- 粉丝: 368
- 资源: 40
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

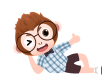
最新资源

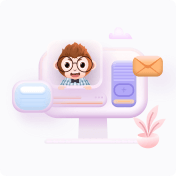
