dhtmlXVaultObject = function()
{
this.isUploadFile = "false";
this.isUploadFileAll = "false";
this.countRows = null;
this.idRowSelected = null;
this.sessionId = null;
//server handlers
this.pathUploadHandler = null;
this.pathGetInfoHandler = null;
this.pathGetIdHandler = null;
//demo
this.isDemo = true;
this.progressDemo = null;
//from PHP
this.MAX_FILE_SIZE = null;
this.UPLOAD_IDENTIFIER = null;
}
dhtmlXVaultObject.prototype.setServerHandlers = function(uploadHandler, getInfoHandler, getIdHandler)
{
this.pathUploadHandler = uploadHandler;
this.pathGetInfoHandler = getInfoHandler;
this.pathGetIdHandler = getIdHandler;
}
dhtmlXVaultObject.prototype.create = function(htmlObject)
{
this.parentObject = document.getElementById(htmlObject);
this.parentObject.style.position = "relative";
this.parentObject.innerHTML = "<iframe src='about:blank' id='dhtmlxVaultUploadFrame' name='dhtmlxVaultUploadFrame' style='display:none'></iframe>";
this.containerDiv = document.createElement("div");
this.containerDiv.style.cssText = "position:absolute;overflow-y:auto;height:190px;background-color:#FFFFFF;border:1px solid #878E95;top:10px;left:10px;z-index:10;width:410px";
this.parentObject.appendChild(this.containerDiv);
this.container = document.createElement("div");
this.container.style.position = "relative";
var str = "<table style='background-color:#EDEEEF;border: 1px solid #7A7C80;' border='0'>" +
"<tr><td style='width:420px' colspan=3 align='center' id = 'cellContainer' >" +
"<div style='height:200px;'></div>" +
"</td></tr>" +
"<tr><td style='width: 80px; height: 32px;' align='left'></td>" +
"<td style='width: 200px; height: 32px;' align='left'>" +
"<img _onclick='UploadControl.prototype.uploadAllItems()' _ID='ImageButton3' src='imgs/btn_upload.gif' style='cursor:pointer'/></td>" +
"<td style='width: 140px; height: 32px;' align='right'>" +
"<img _onclick='return UploadControl.prototype.removeAllItems()' _ID='ImageButton3' src='imgs/btn_clean.gif' style='cursor:pointer;margin-right:20px'/></td></tr></table>" +
"<div _id='fileContainer' style='width:84px;overflow:hidden;height:32px;left:0px;direction:rtl;position:absolute;top:211px'>" +
"<img style='z-index:2' src='imgs/btn_add.gif'/>" +
"<input type='file' id='file1' name='file1' value='' class='hidden' style='cursor:pointer;z-index:3;left:7px;position:absolute;height:25px;top:0px'/></div>";
this.container.innerHTML = str;
var self = this;
this.container.childNodes[0].rows[1].cells[1].childNodes[0].onclick = function() {
self.uploadAllItems()
};
this.container.childNodes[0].rows[1].cells[2].childNodes[0].onclick = function() {
self.removeAllItems()
};
this.fileContainer = this.container.childNodes[1];
this.fileContainer.childNodes[1].onchange = function() {
self.addFile()
};
this.uploadForm = document.createElement("form");
this.uploadForm.method = "post";
this.uploadForm.encoding = "multipart/form-data";
this.uploadForm.target = "dhtmlxVaultUploadFrame";
this.container.appendChild(this.uploadForm);
//from PHP
this.MAX_FILE_SIZE = document.createElement("input");
this.MAX_FILE_SIZE.type = "hidden";
this.MAX_FILE_SIZE.name = "MAX_FILE_SIZE";
this.MAX_FILE_SIZE.value = '200000000';
this.uploadForm.appendChild(this.MAX_FILE_SIZE);
this.UPLOAD_IDENTIFIER = document.createElement("input");
this.UPLOAD_IDENTIFIER.type = "hidden";
this.UPLOAD_IDENTIFIER.name = "UPLOAD_IDENTIFIER";
this.uploadForm.appendChild(this.UPLOAD_IDENTIFIER);
this.parentObject.appendChild(this.container);
this.tblListFiles = null;
this.currentFile = this.fileContainer.childNodes[1];
//if demo
if (this.isDemo)
{
this.progressDemo = this.createProgressDemo();
}
}
dhtmlXVaultObject.prototype.createXMLHttpRequest = function()
{
var xmlHttp = null;
if (window.ActiveXObject)
{
xmlHttp = new ActiveXObject("Microsoft.XMLHTTP");
}
else if (window.XMLHttpRequest)
{
xmlHttp = new XMLHttpRequest();
}
return xmlHttp
}
//get file name
dhtmlXVaultObject.prototype.getFileName = function(path)
{
var arr = path.split("\\");
return arr[arr.length - 1];
}
dhtmlXVaultObject.prototype.selectItemInExplorer = function(currentId)
{
var currentRow = this.getCurrentRowListFiles(currentId);
if (this.idRowSelected)
{
var row = this.getCurrentRowListFiles(this.idRowSelected);
if (row)
{
if (row.id != currentRow.id)
{
currentRow.style.background = "#F3F3F3";
this.idRowSelected = currentId;
row.style.background = "#FFFFFF";
}
else
{
currentRow.style.background = "#FFFFFF";
this.idRowSelected = "";
}
}
else
{
currentRow.style.background = "#F3F3F3";
this.idRowSelected = currentId;
}
} else
{
currentRow.style.background = "#F3F3F3";
this.idRowSelected = currentId;
}
}
dhtmlXVaultObject.prototype.selectItemInMozilla = function(currentId)
{
var currentRow = this.getCurrentRowListFiles(currentId);
if (this.idRowSelected)
{
var row = this.getCurrentRowListFiles(this.idRowSelected);
if (row)
{
if (row.id != currentRow.id)
{
currentRow.style.background = "#F3F3F3";
this.idRowSelected = currentId;
row.style.background = "#FFFFFF";
}
else
{
currentRow.style.background = "#FFFFFF";
this.idRowSelected = "";
}
}
else
{
currentRow.style.background = "#F3F3F3";
this.idRowSelected = currentId;
}
} else
{
currentRow.style.background = "#F3F3F3";
this.idRowSelected = currentId;
}
}
// add item in "upload control"
dhtmlXVaultObject.prototype.addFile = function()
{
var currentId = this.createId();
var file = this.currentFile;
file.disabled = true;
file.style.display = "none";
this.uploadForm.appendChild(file);
var newInputFile = document.createElement("input");
newInputFile.type = "file";
newInputFile.className = "hidden";
newInputFile.style.cssText = "cursor:pointer;z-index:3;left:7px;position:absolute;height:30px";
newInputFile.id = "file" + (currentId + 1);
newInputFile.name = "file" + (currentId + 1);
this.currentFile = newInputFile;
var self = this;
newInputFile.onchange = function() {
return self.addFile()
};
this.fileContainer.appendChild(newInputFile);
var fileName = this.getFileName(file.value);
var imgFile = this.getImgFile(fileName);
//create table ListFiles
var containerData = this.containerDiv;
if (this.tblListFiles == null)
{
this.tblListFiles = this.createTblListFiles();
containerData.appendChild(this.tblListFiles);
}
var rowListFiles = this.tblListFiles.insertRow(this.tblListFiles.rows.length);
rowListFiles.setAttribute("fileItemId", currentId);
rowListFiles.setAttribute("id", "rowListFiles" + currentId);
rowListFiles.setAttribute("isUpload", "false");
if (navigator.appName.indexOf("Explorer") != -1)
{
rowListFiles.onclick = function() {
self.selectItemIn

fhygogo
- 粉丝: 2
- 资源: 3
最新资源
- 电子学习资料设计作品全资料RCC电路间歇振荡的研究资料
- 基于SpringBoot在线学习平台的设计与实现
- 基于springboot框架实现的微信公众号推送天气信息完整源码
- 电子学习资料设计作品全资料八位数字密码锁资料
- 冬天就快来了,n 只鼠鼠偷了一大堆奶酪放到老鼠洞里,约定第二天平分 这些鼠鼠很崇拜鼠王杰瑞,所以都想给它留一些奶酪 可没过一会,第一只鼠鼠悄悄来到老鼠洞里,把奶酪平均分成 n 份,把剩下的 m 个奶酪
- Java8 JDK8 8u202 Windows版
- 电子学习资料设计作品全资料笔记本电脑的智能底座设计
- 基于jdk17,mysql8,redis7,spring boot3,spring cloud Alibaba ,nacos,openfigen,resilience4j等搭建的微服务框架
- 电子学习资料设计作品全资料步进电机
- java8 jdk8-8u181-windows-x64
- javaweb项目:基于servlet+jsp实现的登录注册功能分前后台完整代码
- 电子学习资料设计作品全资料步进电机调速系统设计资料
- jdk8-8u202-linux-x64.tar
- 电子学习资料设计作品全资料采用实时时钟芯片DS1302+AT89C2051的红外遥控LED电子钟
- jdk8-8u202-linux-i586.tar
- 基于Springboot农产品研究报告管理系统的设计与实现
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


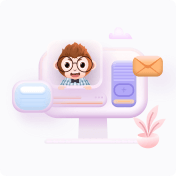
- 1
- 2
- 3
- 4
- 5
- 6
前往页