/********************************************************
* @author Airead Fan <fgh1987168@gmail.com> *
* @date 2011 9月 19 21:26:48 CST *
********************************************************
* after studying C 63 days *
* after studying APUE 28 days *
********************************************************/
#include <stdio.h>
#include <SDL/SDL.h>
#include <SDL/SDL_image.h>
#include <stdbool.h>
#include "xoox.h"
#include "button.h"
#include "timer.h"
#include "judgement.h"
#define TEST 1
int main(int argc, int *argv[])
{
bool quit = false; /* quit flag */
int i, j;
int play_stat = 0;
Timer fps; /* cap frame rate */
SDL_Event event;
SDL_Surface *mouseother;
SDL_Surface *wintitle[3];
SDL_Surface *screen; /* main screen */
SDL_Surface *chessboard; /* chess board */
Button pieces[CHESSBOARD_ROW][CHESSBOARD_COLUMN] = {{0}};
SDL_Surface *piece_stat_img[PIECE_STAT + 1] = {NULL}; /* pieces image */
int mouse_map[CHESSBOARD_ROW][CHESSBOARD_COLUMN] = {{0}}; /* click or not */
int pieces_map[CHESSBOARD_ROW][CHESSBOARD_COLUMN] = {{0}}; /* pieces state */
/* Init SDL lib*/
if(SDL_Init(SDL_INIT_EVERYTHING) == -1){
return 1;
}
/* Init screen */
screen = SDL_SetVideoMode(SCREEN_WIDTH, SCREEN_HEIGHT, SCREEN_BPP, SDL_SWSURFACE);
if(screen == NULL){
return 1;
}
SDL_WM_SetCaption("XO_OX", NULL);
/* Load resource */
piece_stat_img[1] = load_image("xooxres/chess1.png");
piece_stat_img[2] = load_image("xooxres/chess2.png");
piece_stat_img[3] = piece_stat_img[1]; /* for special show, but no time to do */
piece_stat_img[4] = piece_stat_img[2]; /* for special show, but no time to do */
chessboard = load_image("xooxres/chessboard.png");
mouseother = load_image("xooxres/mouseother.png");
wintitle[0] = load_image("xooxres/wintitle1.png");
wintitle[1] = load_image("xooxres/wintitle2.png");
wintitle[2] = load_image("xooxres/wintitle3.png");
/* Init timer */
timer_init(&fps);
/* Init button */
for(i = 0; i < CHESSBOARD_ROW; i++){
for(j = 0; j < CHESSBOARD_COLUMN; j++){
button_init(&pieces[i][j], SUBBOARD_WIDTH * i, SUBBOARD_HEIGHT * j, SUBBOARD_WIDTH , SUBBOARD_HEIGHT );
button_set_stat_img(&pieces[i][j], BUTTON_MOUSEOVER, NULL);
button_set_stat_img(&pieces[i][j], BUTTON_MOUSEOUT, mouseother);
button_set_stat_img(&pieces[i][j], BUTTON_MOUSEUP, mouseother);
button_set_stat_img(&pieces[i][j], BUTTON_MOUSEDOWN, mouseother);
}
}
/* Main loop */
while(quit == false){
/* timer start */
timer_start(&fps);
while(SDL_PollEvent(&event)){
/* Pieces input handle */
pieces_handle_event(pieces, mouse_map, &event);
/* User quit */
if(event.type == SDL_QUIT){
quit = true;
}
}
/* logic function */
play_stat = mouse_to_piece_map(mouse_map, pieces_map);
/* show chessboard */
subchessboard_show(piece_stat_img[get_leader()], mouseother, mouse_map, screen);
/* show pieces */
pieces_show(piece_stat_img, pieces_map, screen);
/* */
if(play_stat != 0){
printf("play_stat = %d\n", play_stat);
apply_surface((SCREEN_WIDTH - wintitle[play_stat - 1]->w) / 2, (SCREEN_HEIGHT - wintitle[play_stat - 1]->h) / 2, wintitle[play_stat - 1], screen);
}
/* Update screen */
if(SDL_Flip(screen) == -1){
return 1;
}
if(play_stat != 0){
SDL_Delay(3000);
quit = true;
}
/* cap frame rate */
if(timer_get_ticks(&fps) < 1000 / FRAME_PER_SECOND){
SDL_Delay((1000 / FRAME_PER_SECOND) - timer_get_ticks(&fps));
}
}
/* Free resource */
SDL_FreeSurface(chessboard);
SDL_FreeSurface(mouseother);
for(i = 0; i < 2; i++){
SDL_FreeSurface(piece_stat_img[i]);
}
for(i = 0; i < 3; i++){
SDL_FreeSurface(wintitle[i]);
}
SDL_Quit();
return 0;
}
/*
* Load a image to a temporary image, optimized the image
* and free the temporary image.
*
*/
SDL_Surface *load_image(char *filename)
{
SDL_Surface *img_tmp = NULL;
SDL_Surface *img_opt = NULL;
Uint32 colork = 0;
img_tmp = IMG_Load(filename);
if(img_tmp != NULL){
img_opt = SDL_DisplayFormat(img_tmp);
SDL_FreeSurface(img_tmp);
if(img_opt != NULL){
colork = SDL_MapRGB(img_opt->format, 0xFF, 0xFF, 0xFF);
SDL_SetColorKey(img_opt, SDL_SRCCOLORKEY, colork);
}
}
return img_opt;
}
/*
* Simple apply source to destination at (x, y)
*
*/
void apply_surface(int x, int y, SDL_Surface *source, SDL_Surface* destination)
{
SDL_Rect offset;
offset.x = x;
offset.y = y;
SDL_BlitSurface(source, NULL, destination, &offset);
}
/*
* every pieces handle event
* get mouse_map
*/
void pieces_handle_event(Button bt[][CHESSBOARD_COLUMN], int mouse_map[][CHESSBOARD_COLUMN], SDL_Event *event)
{
int i, j;
for(i = 0; i < CHESSBOARD_ROW; i++){
for(j = 0; j < CHESSBOARD_COLUMN; j++){
button_handle_event(&bt[i][j], event);
mouse_map[i][j] = bt[i][j].cur_stat;
}
}
}
/*
* show all pieces according to pieces map
*/
void pieces_show(SDL_Surface *piece_stat_img[], int pieces_map[][CHESSBOARD_COLUMN], SDL_Surface *screen)
{
int i, j;
for(i = 0; i < CHESSBOARD_ROW; i++){
for(j = 0; j < CHESSBOARD_COLUMN; j++){
if(pieces_map[i][j] != 0){
apply_surface(i * SUBBOARD_WIDTH + 4, j * SUBBOARD_HEIGHT + 4, piece_stat_img[pieces_map[i][j]], screen);
}
}
}
}
/*
* show chessboard
*/
void subchessboard_show(SDL_Surface *mouseover, SDL_Surface *mouseother, int mouse_map[][CHESSBOARD_COLUMN], SDL_Surface *screen)
{
int i, j;
for(i = 0; i < CHESSBOARD_ROW; i++){
for(j = 0; j < CHESSBOARD_COLUMN; j++){
if(mouse_map[i][j] & MOUSEOVER ){
apply_surface(i * SUBBOARD_WIDTH + 4, j * SUBBOARD_HEIGHT + 4, mouseover, screen);
}else{
apply_surface(i * SUBBOARD_WIDTH , j * SUBBOARD_HEIGHT , mouseother, screen);
}
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
XO_OX 0.0 <fgh1987168@gmail.com> 这些是“XO_OX"的注解。它们会让你全面了解这个游戏,并会说明如何安装它。 什么是“XO_OX"? “XO_OX"又名“五子棋”,五子棋则咸信是流传于古中国的传统棋种之一,至今仍在民间广泛流传,规则相当简单。或许因没有形成一套独立完整的棋种理论及文化内涵,更无制定公平完善的规则来解决黑白平衡问题,一直没有得到发展,所以没有像六博、格五、弹棋等传统棋类流传广泛,导致缺少可考古的棋具或文献,直到流传到外国才规则改革。 不管是哪种五子棋,棋手在先后手的观念、空间的思维及对棋形的理解都十分重要。 游戏规则: * 行棋:一人流轮一著下于棋盘空点处,下后不得移动。 * 胜负:先把五枚或以上己棋相连成任何横纵斜方向为胜。 * 和棋: o 行棋中一方提出和棋,另一方同意则判和棋。 o 棋子落满整张棋盘仍未分出胜负为和棋。 o 一方PASS后另一方下一手也PASS为和棋。 技术规格说明: 1、用C语言调用SDL实现; 2、基于LGPL协议。 3、程序中用到了SDL_image扩展包 如何安装: 1、在终端中运行make 2、在终端中运行make install 如何卸载: make uninstall 历史: 一、2011年8月15日 项目开始,谢红负责图形模块,赵梓辰负责游戏逻辑,吕玉飞负责事件响应,范人豪负责整体架构。 二、2011年8月17日 为了增加游戏的可玩性,项目由圈叉棋升级为五子棋。
资源推荐
资源详情
资源评论
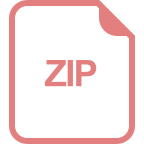
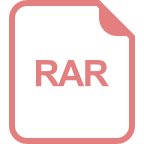
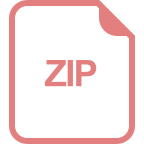
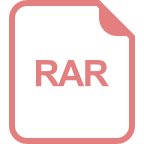
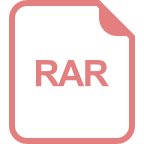
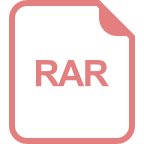
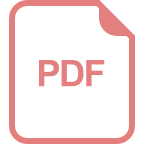
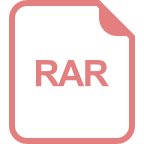
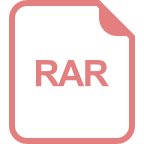
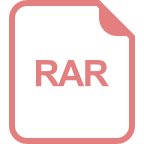
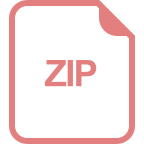
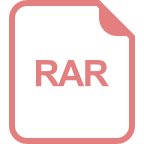
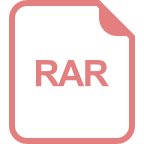
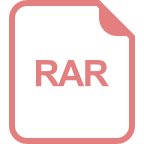
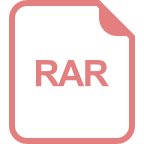
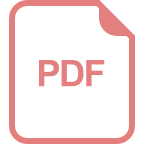
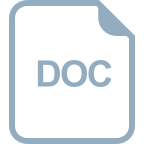
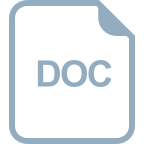
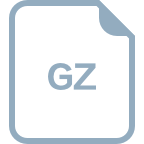
收起资源包目录


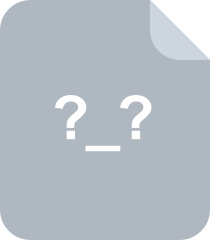
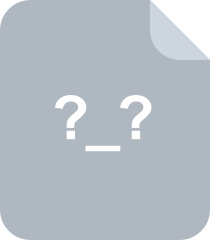
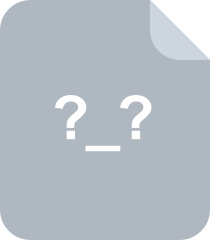

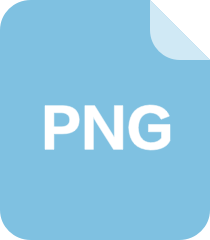
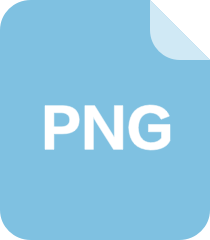
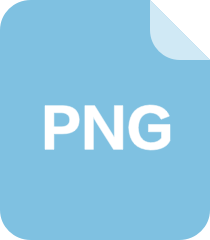
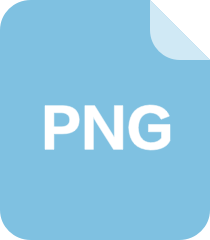
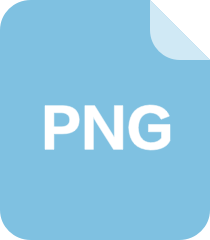
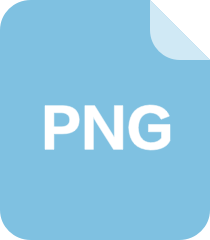
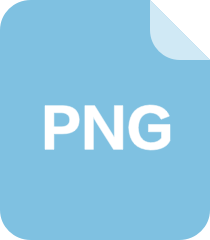
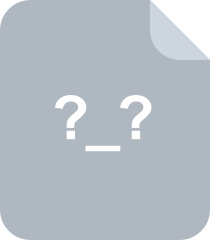
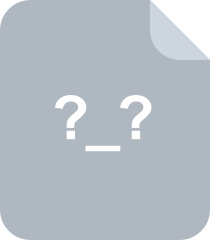
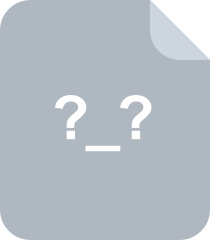
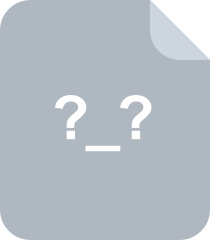
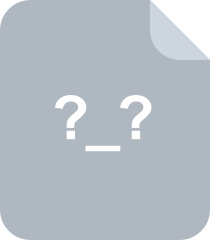
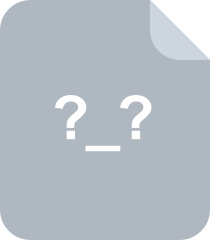
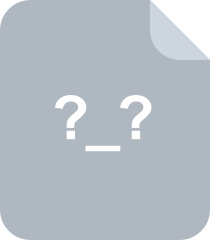
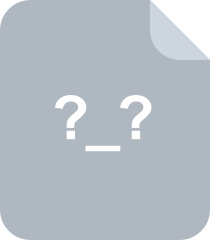
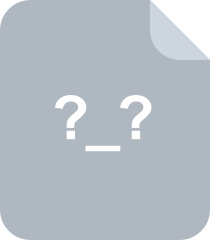
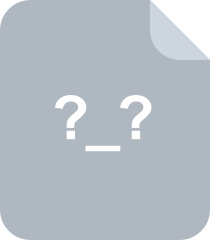
共 20 条
- 1

紫馨岚
- 粉丝: 6
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

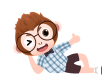
最新资源
- YOLOv8完整网络结构图详细visio
- LCD1602电子时钟程序
- 西北太平洋热带气旋【灾害风险统计】及【登陆我国次数评估】数据集-1980-2023
- 全球干旱数据集【自校准帕尔默干旱程度指数scPDSI】-190101-202312-0.5x0.5
- 基于Python实现的VAE(变分自编码器)训练算法源代码+使用说明
- 全球干旱数据集【标准化降水蒸发指数SPEI-12】-190101-202312-0.5x0.5
- C语言小游戏-五子棋-详细代码可运行
- 全球干旱数据集【标准化降水蒸发指数SPEI-03】-190101-202312-0.5x0.5
- spring boot aop记录修改前后的值demo
- 全球干旱数据集【标准化降水蒸发指数SPEI-01】-190101-202312-0.5x0.5
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


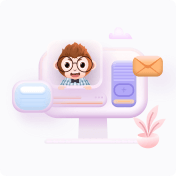
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页