### AJAX完成分页程序知识点详解 #### 一、引言 在现代Web开发中,为了提升用户体验并减轻服务器压力,异步加载数据成为了一种常见的技术手段。AJAX(Asynchronous JavaScript and XML)就是实现这一目标的重要技术之一。本文将详细介绍如何使用AJAX来完成分页功能,并对代码进行逐行解析。 #### 二、AJAX分页概述 AJAX分页是指通过AJAX技术来实现页面数据的动态加载,用户在浏览过程中无需刷新整个页面即可查看不同页的数据。这种方式可以极大地提高网页的响应速度和用户体验。 #### 三、核心代码分析 我们来看一下`PostPaginate`构造函数: ```javascript function PostPaginate(pageSize, isCallAllItems, result) { if (result) this.result = result; this.totalNum = 0; // all items' length this.pageSize = 5; // default value. this.start = 0; // start index of items this.end = 0; // end index of items this.curPage = 0; // current page index this.totalPage = 0; // all page count this.isCallAllItems = isCallAllItems; this.init(0, pageSize, result.itemCount); } ``` **1. 构造函数解析:** - `pageSize`: 每页显示的记录数量。 - `isCallAllItems`: 是否调用所有项。 - `result`: 包含当前请求结果的对象。 **2. 初始化属性:** - `totalNum`: 总记录数。 - `pageSize`: 页面大小。 - `start`: 当前页起始索引。 - `end`: 当前页结束索引。 - `curPage`: 当前页码。 - `totalPage`: 总页数。 - `isCallAllItems`: 是否调用所有项目。 接下来是`init`方法: ```javascript PostPaginate.prototype.init = function(currentPage, onePageSize, totalNum) { window.scroll(0, 0); this.pageSize = onePageSize; this.totalNum = totalNum; this.totalPage = Math.ceil(this.totalNum / this.pageSize); if (currentPage >= 0) { if (currentPage >= Math.ceil(this.totalNum / this.pageSize)) { this.curPage = this.totalPage - 1; } else { this.curPage = currentPage; } } else { this.curPage = 0; } this.start = this.curPage * this.pageSize; this.end = this.start + this.pageSize; if (this.end > this.totalNum) { this.end = this.totalNum; } // 控制按钮状态 this.controllButtonsStatus(); // 填充HTML this.fillDataWithCurrentPageItems(); // 更新描述 this.fillPaginateDescription(); }; ``` **3. init 方法解析:** - 调整窗口滚动位置到顶部。 - 设置页面大小、总记录数、总页数等属性。 - 计算当前页的起始与结束索引。 - 调用`controllButtonsStatus`控制按钮状态。 - 调用`fillDataWithCurrentPageItems`填充当前页数据。 - 调用`fillPaginateDescription`更新描述信息。 **4. 数据获取函数:** ```javascript function callbackGetItemsForPaginate(result, exception) { if (exception) { showError("An error has occurred: " + exception.message); } else { // 对title进行转义 for (var i = 0; i < result.items.length; i++) { var item = result.items[i]; if (item.categoryInformation) { item.categoryInformation.title = dojo.string.escape("html", item.categoryInformation.title); } } paginate.result = result; paginate.init(paginate.curPage, paginate.pageSize, result.itemCount); } } ``` - `callbackGetItemsForPaginate`: 异步请求回调函数。 - 处理异常情况。 - 对数据进行预处理,如对标题进行转义。 - 更新分页对象的状态并重新渲染页面。 **5. 数据请求方法:** ```javascript PostPaginate.prototype.fetchData = function(curPage) { if (!checkTreeNodeExisted(selectWhichNode)) { alert("Sorry, this feed was previously removed from your feed tree."); return; } if (this.isCallAllItems) { this.result = jsonrpc.subscribe.getItems(callbackGetItemsForPaginate, true, selectWhichNode, selectedTime, true, curPage, false, this.totalNum); } else { this.result = jsonrpc.subscribe.getItems(callbackGetItemsForPaginate, false, selectWhichNode, selectedTime, true, curPage, false, this.pageSize); } }; ``` - `fetchData`: 请求数据的方法。 - 验证节点是否存在。 - 根据`isCallAllItems`决定是否请求所有项。 - 发送异步请求获取数据。 #### 四、总结 本文通过一个具体的分页实现案例,详细解析了使用AJAX完成分页的主要过程。从初始化分页对象、设置分页参数、获取数据、处理数据到最终展示数据,每个步骤都进行了细致的说明。通过学习这些内容,可以帮助开发者更好地理解并实现基于AJAX的分页功能。
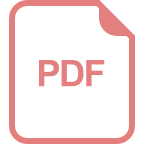
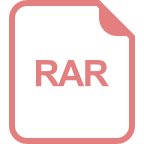
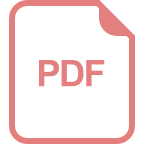
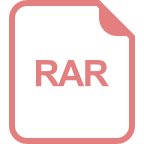
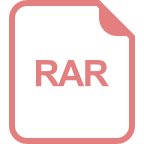
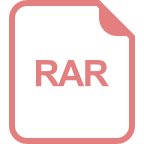
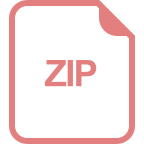
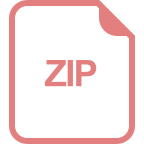
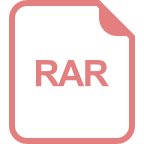
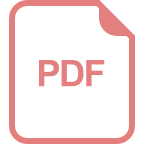
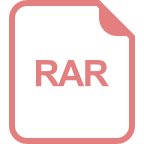
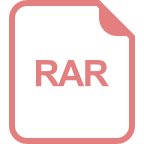
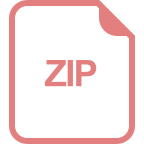
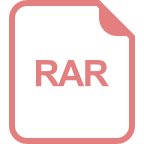
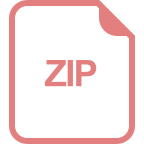
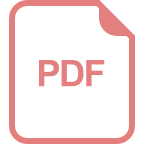
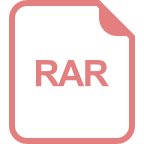
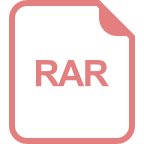
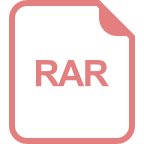
js 代码
function PostPaginate(pageSize, isCallAllItems, result){
if (result)
this.result = result;
this.totalNum = 0; // all items' length
this.pageSize = 5; // default value.
this.start = 0; // start index of items
this.end = 0; // end index of items
this.curPage = 0; // current page index
this.totalPage = 0; // all page count
this.isCallAllItems = isCallAllItems;
this.init(0, pageSize, result.itemCount);
}
// prepay all need data, ie totalNum, start, end .... also it would do something others,
// fill the data to html page, controll the first, previous, next, last buttons status.
PostPaginate.prototype.init=function(currentPage, onePageSize, totalNum){
window.scroll(0, 0);
this.pageSize = onePageSize;
this.totalNum = totalNum;
this.totalPage = Math.ceil(this.totalNum / this.pageSize);
if (currentPage >=0){
if (currentPage >= Math.ceil(this.totalNum / this.pageSize)){
this.curPage = this.totalPage-1;
}else{
}
}else{
this.curPage = 0;
}
this.start = this.curPage * this.pageSize;
this.end = this.start + this.pageSize;
if (this.end > this.totalNum){
this.end = this.totalNum;
}
// controll buttons
this.controllButtonsStatus();
// fill html
this.fillDataWithCurrentPageItems();
//update the description
this.fillPaginateDescription();
};
function callbackGetItemsForPaginate(result, exception){
if (exception) {
showError("An error has occurred: " + exception.message);
} else {
// escape titles
for (var i = 0; i < result.items.length; i++) {
var item = result.items[i];
if (item.categoryInformation) {
item.categoryInformation.title = dojo.string.escape("html", item.categoryInformation.title);
剩余9页未读,继续阅读
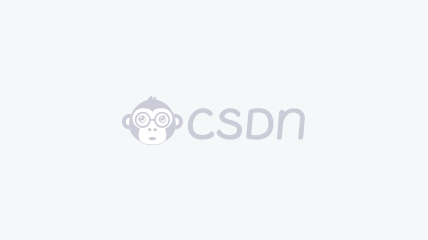

- 粉丝: 2
- 资源: 24
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

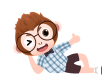
最新资源

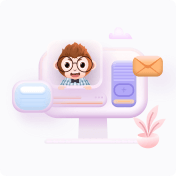
