package org.gz.struts.action;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import org.apache.struts.action.ActionForm;
import org.apache.struts.action.ActionForward;
import org.apache.struts.action.ActionMapping;
import org.apache.struts.actions.DispatchAction;
import org.gz.service.PlayService;
import org.gz.struts.form.NewsForm;
import org.gz.struts.form.PlayForm;
import org.gz.struts.form.UserForm;
import org.gz.util.Splitpageinfo;
public class PlayAction extends DispatchAction {
private PlayService playService;
public ActionForward firstPage(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
return this.getPage(mapping, request, 1);
}
public ActionForward upPage(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
return this.getPage(mapping, request, 2);
}
public ActionForward nextPage(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
return this.getPage(mapping, request, 3);
}
public ActionForward lastPage(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
return this.getPage(mapping, request, 4);
}
// 查询
public ActionForward selectPlay(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
PlayForm playForm = (PlayForm) form;
HttpSession session = request.getSession(true);
String condition = "";
condition += " and a.name like '%" + playForm.getName() + "%'";
if (playForm.getTypeitem_id() != -1)
condition += " and a.typeitem_id='" + playForm.getTypeitem_id()
+ "'";
if (playForm.getStatus() != -1)
condition += " and a.status = '" + playForm.getStatus() + "'";
PlayForm playInforForm = new PlayForm(playForm.getName(), playForm
.getTypeitem_id(), playForm.getStatus());
session.setAttribute("condition", condition);
session.setAttribute("playForm", playInforForm);
return mapping.findForward("splitPage");
}
// 页面显示公用方法
public ActionForward getPage(ActionMapping mapping,
HttpServletRequest request, int flag) {
// 得到默认路径
ActionForward forward = mapping.getInputForward();
// 获得Session对象
HttpSession session = request.getSession(true);
// 得到Condition
String condition = "";
String pre = request.getParameter("flag");
if ("pre".equals(pre)) {
condition += " and a.status=2 and a.startdate>getdate()";
}
// 首次进入需移除条件
if (request.getParameter("first") != null) {
session.removeAttribute("playForm");
session.removeAttribute("condition");
}
// 获取Select的条件Condition
if (session.getAttribute("condition") != null) {
condition = session.getAttribute("condition").toString();
}
if (session.getAttribute("user") != null) {
UserForm userForm = (UserForm) session.getAttribute("user");
List<PlayForm> types = playService.getAllType(userForm.getId());
List<Long> typeitems = new ArrayList<Long>();
for (PlayForm type : types) {
typeitems.add(type.getTypeitem_id());
}
if (!typeitems.contains(new Long(1)))
condition += " and a.user_id=" + userForm.getId();
}
// 得到分页信息
Splitpageinfo splitpageinfo = null;
if (request.getParameter("first") != null) {
splitpageinfo = new Splitpageinfo(1, playService
.getCount(condition));
session.setAttribute("splitpageinfo", splitpageinfo);
} else {
splitpageinfo = (Splitpageinfo) session
.getAttribute("splitpageinfo");
splitpageinfo.setTotalrecode(playService.getCount(condition));
}
int page = 1;
switch (flag) {
case 1:
page = 1;
break;
case 2:
page = splitpageinfo.getUppage();
break;
case 3:
page = splitpageinfo.getNextpage();
break;
case 4:
page = splitpageinfo.getTotalpage();
break;
}
splitpageinfo.setCurpage(page);
// 存住条件项显示在页面上
request.setAttribute("playForm", session.getAttribute("playForm"));
List<PlayForm> typeList = playService.getAllType(-1);
request.setAttribute("typeList", typeList);
List<PlayForm> playList = playService.getOnePage(condition, "id", page);
request.setAttribute("playList", playList);
if ("pre".equals(pre)) {
return mapping.findForward("prePlay");
}
if ("preResult".equals(pre)) {
List<PlayForm> resultList = playService.getSome(2, 0);
if (request.getParameter("first") != null) {
splitpageinfo = new Splitpageinfo(1, resultList.size());
session.setAttribute("splitpageinfo", splitpageinfo);
} else {
splitpageinfo = (Splitpageinfo) session.getAttribute("splitpageinfo");
splitpageinfo.setTotalrecode(resultList.size());
}
request.setAttribute("playList", resultList);
return mapping.findForward("preResult");
}
return forward;
}
public ActionForward addPlay(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
HttpSession session = request.getSession(true);
long user_id = -1;
if (session.getAttribute("user") != null) {
UserForm user = (UserForm) session.getAttribute("user");
user_id = user.getId();
}
List<PlayForm> typeList = playService.getAllType(user_id);
request.setAttribute("typeList", typeList);
request.setAttribute("flag", "insertPlay");
request.setAttribute("addResulte", true); // 相反,不能添加结果
return mapping.findForward("addPlay");
}
public ActionForward insertPlay(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
ActionForward forward = mapping.getInputForward();
PlayForm playForm = (PlayForm) form;
HttpSession session = request.getSession(true);
if (session.getAttribute("user") != null) {
UserForm user = (UserForm) session.getAttribute("user");
playForm.setUser_id(user.getId());
boolean flag = playService.savePlay(playForm);
if (flag)
forward = mapping.findForward("firstSplitpage");
else {
List<PlayForm> typeList = playService.getAllType(user.getId());
request.setAttribute("typeList", typeList);
forward = mapping.findForward("insertPlayFail");
}
}
return forward;
}
public ActionForward modifyPlay(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
long id = Long.parseLong(request.getParameter("id"));
PlayForm playForm = playService.getOnePlay(id);
List<PlayForm> typeList = playService.getAllType(playForm.getUser_id());
request.setAttribute("typeList", typeList);
request.setAttribute("playForm", playForm);
if (playForm.getEnddate().before(new Date())) {
request.setAttribute("flag", "addResult");
} else {
request.setAttribute("flag", "updatePlay");
}
return mapping.findForward("modifyPlay");
}
public ActionForward updatePlay(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
ActionForward forward = mapping.getInputForward();
PlayForm playForm = (PlayForm) form;
boolean flag = playService.updatePlay(playForm);
if (flag) {
forward = mapping.findForward("firstSplitpage");
} else {
forward = mapping.findForward("updatePlayFail");
}
return forward;
}
public ActionForward addResult(ActionMapping mapping, ActionForm form,
HttpServletRequest request, HttpServletResponse response) {
ActionForward forward = mapping.getInputForward();
PlayForm playForm = (PlayForm) form;
boolean flag = playService.addResult(playForm);
if (flag) {
forward = mapping.findForward("firstSplitpage");
} else {
forward = mapping.findForward("updatePlayFail");
}

GeekZFZ
- 粉丝: 51
- 资源: 20
最新资源
- 【创新无忧】基于雾凇优化算法RIME优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于雾凇优化算法RIME优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化广义神经网络GRNN实现光伏预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化广义神经网络GRNN实现数据回归预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化极限学习机KELM实现故障诊断附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化广义神经网络GRNN实现电机故障诊断附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化相关向量机RVM实现数据多输入单输出回归预测附matlab代码.rar
- 【创新无忧】基于向量加权平均算法INFO优化相关向量机RVM实现北半球光伏数据预测附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化极限学习机ELM实现乳腺肿瘤诊断附matlab代码.rar
- 【创新无忧】基于星雀优化算法NOA优化广义神经网络GRNN实现光伏预测附matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


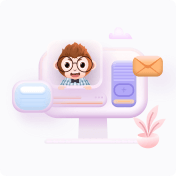