package com.controller;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.annotation.IgnoreAuth;
import com.entity.YishengEntity;
import com.entity.view.YishengView;
import com.service.YishengService;
import com.service.TokenService;
import com.utils.PageUtils;
import com.utils.R;
import com.utils.MPUtil;
import com.utils.CommonUtil;
/**
* 医生
* 后端接口
* @author
* @email
* @date 2020-09-23 17:33:51
*/
@RestController
@RequestMapping("/yisheng")
public class YishengController {
@Autowired
private YishengService yishengService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
YishengEntity user = yishengService.selectOne(new EntityWrapper<YishengEntity>().eq("gonghao", username));
if(user==null || !user.getMima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(), username,"yisheng", "医生" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody YishengEntity yisheng){
//ValidatorUtils.validateEntity(yisheng);
YishengEntity user = yishengService.selectOne(new EntityWrapper<YishengEntity>().eq("gonghao", yisheng.getGonghao()));
if(user!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
yisheng.setId(uId);
yishengService.insert(yisheng);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
YishengEntity user = yishengService.selectById(id);
return R.ok().put("data", user);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
YishengEntity user = yishengService.selectOne(new EntityWrapper<YishengEntity>().eq("gonghao", username));
if(user==null) {
return R.error("账号不存在");
}
user.setMima("123456");
yishengService.updateById(user);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,YishengEntity yisheng, HttpServletRequest request){
EntityWrapper<YishengEntity> ew = new EntityWrapper<YishengEntity>();
PageUtils page = yishengService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yisheng), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,YishengEntity yisheng, HttpServletRequest request){
EntityWrapper<YishengEntity> ew = new EntityWrapper<YishengEntity>();
PageUtils page = yishengService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, yisheng), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( YishengEntity yisheng){
EntityWrapper<YishengEntity> ew = new EntityWrapper<YishengEntity>();
ew.allEq(MPUtil.allEQMapPre( yisheng, "yisheng"));
return R.ok().put("data", yishengService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(YishengEntity yisheng){
EntityWrapper< YishengEntity> ew = new EntityWrapper< YishengEntity>();
ew.allEq(MPUtil.allEQMapPre( yisheng, "yisheng"));
YishengView yishengView = yishengService.selectView(ew);
return R.ok("查询医生成功").put("data", yishengView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") String id){
YishengEntity yisheng = yishengService.selectById(id);
return R.ok().put("data", yisheng);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") String id){
YishengEntity yisheng = yishengService.selectById(id);
return R.ok().put("data", yisheng);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody YishengEntity yisheng, HttpServletRequest request){
yisheng.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yisheng);
YishengEntity user = yishengService.selectOne(new EntityWrapper<YishengEntity>().eq("gonghao", yisheng.getGonghao()));
if(user!=null) {
return R.error("用户已存在");
}
yisheng.setId(new Date().getTime());
yishengService.insert(yisheng);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody YishengEntity yisheng, HttpServletRequest request){
yisheng.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(yisheng);
YishengEntity user = yishengService.selectOne(new EntityWrapper<YishengEntity>().eq("gonghao", yisheng.getGonghao()));
if(user!=null) {
return R.error("用户已存在");
}
yisheng.setId(new Date().getTime());
yishengService.insert(yisheng);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody YishengEntity yisheng, HttpServletRequest request){
//ValidatorUtils.validateEntity(yisheng);
yishengService.updateById(yisheng);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
yishengService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new D
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
(1)管理员功能:系统首页、个人中心、用户管理、医生管理、疫情公告管理、行动轨迹管理、异样报告管理、科室信息管理、异常报告管理、系统管理、医院信息管理、预约信息管理、在线聊天管理、聊天回复管理、我的收藏管理。 (2)用户功能:系统首页、个人中心、行动轨迹管理、异样报告管理、预约信息管理、在线聊天管理、聊天回复管理、我的收藏管理。 (3)医生功能:系统首页、个人中心、预约信息管理、在线聊天管理、聊天回复管理。 环境说明: 开发语言:Java 后端框架:ssm 前端框架:vue JDK版本:JDK1.8 数据库:mysql 5.7+ 部署容器:tomcat7+ 数据库工具:Navicat11+ 开发软件:eclipse/myeclipse/idea(推荐idea) Maven包:Maven3.3.9
资源推荐
资源详情
资源评论
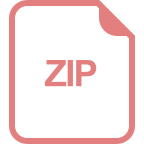
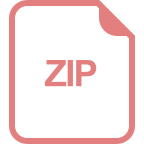
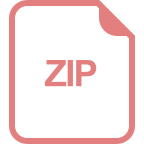
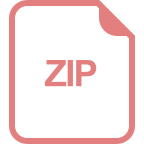
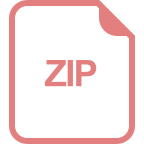
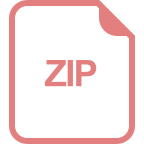
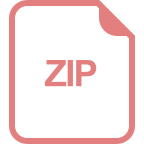
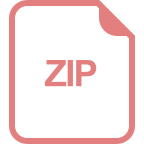
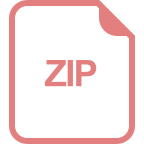
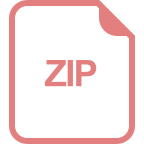
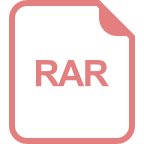
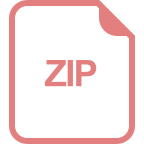
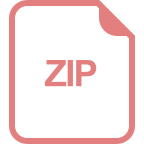
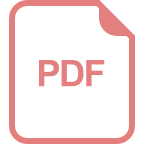
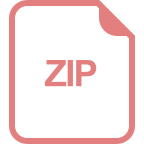
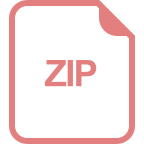
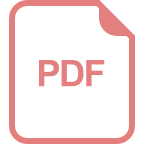
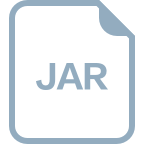
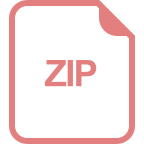
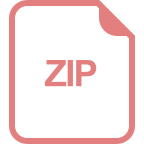
收起资源包目录

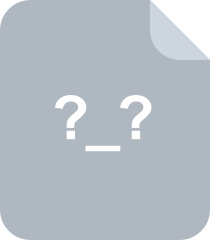
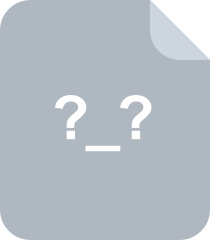
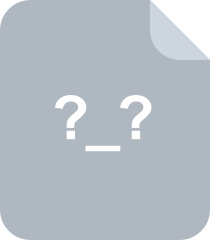
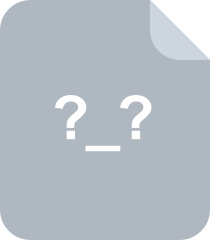
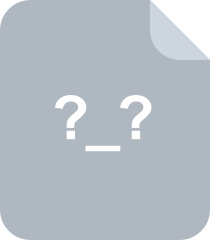
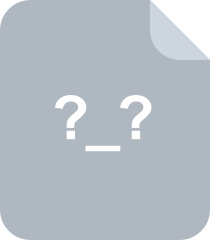
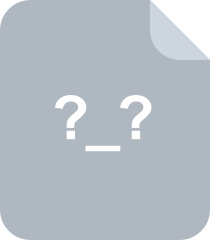
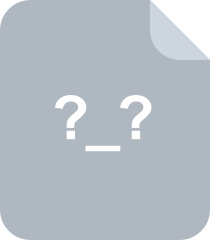
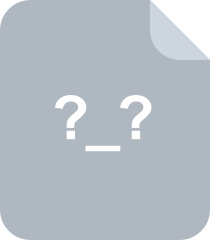
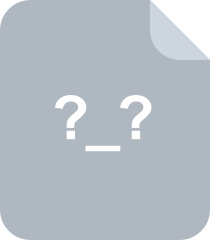
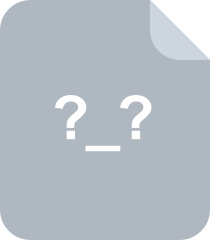
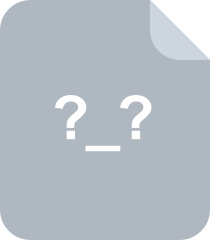
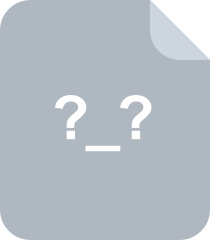
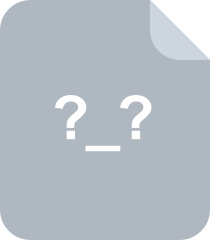
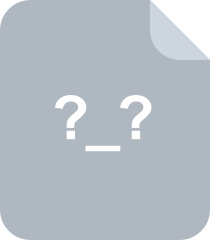
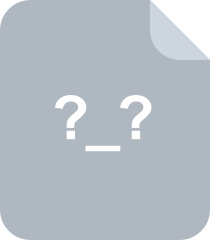
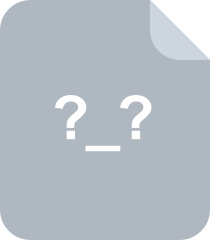
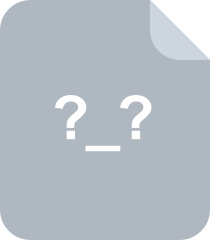
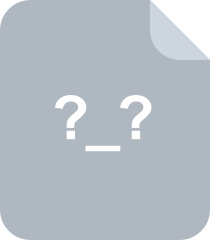
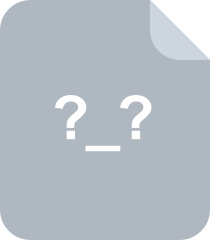
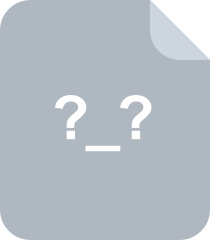
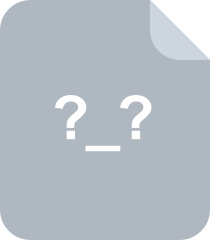
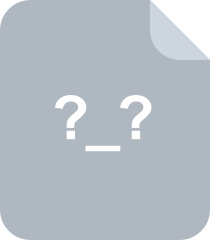
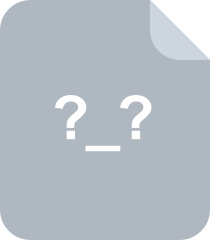
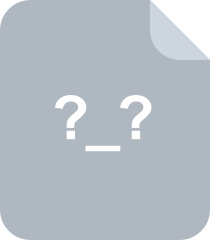
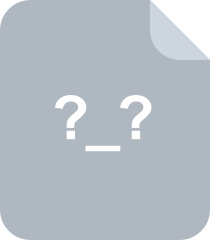
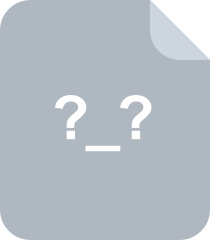
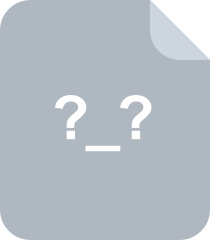
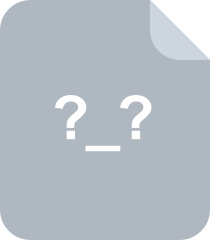
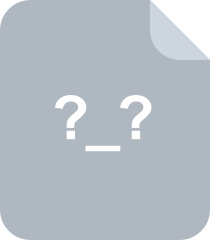
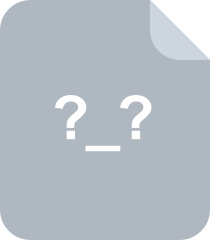
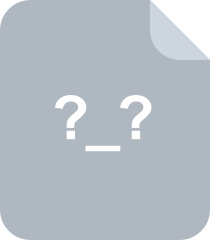
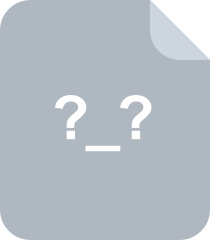
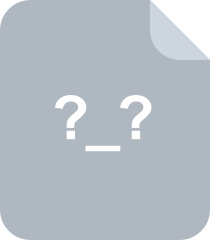
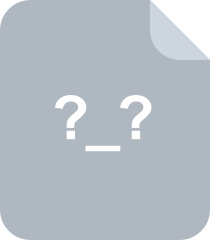
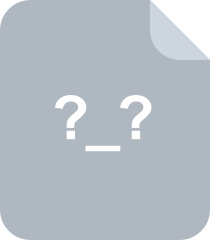
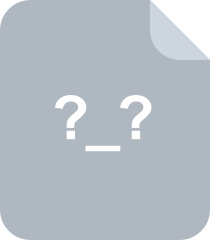
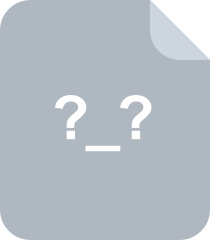
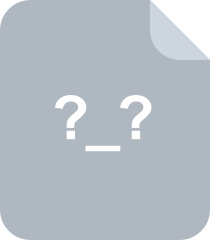
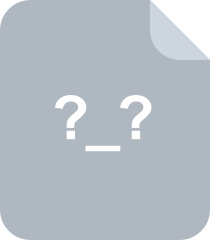
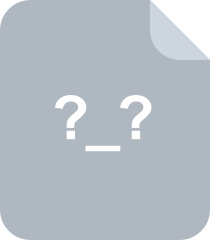
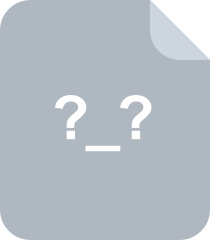
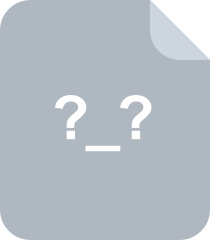
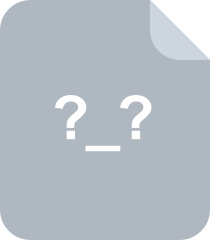
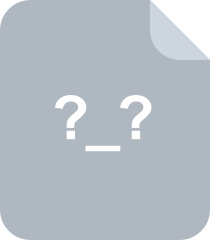
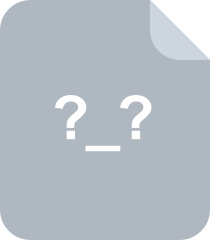
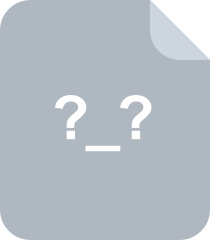
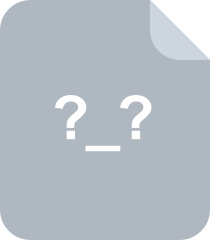
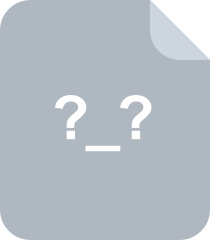
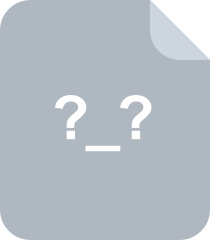
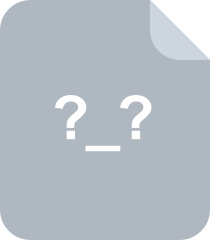
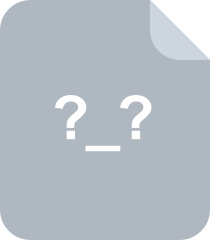
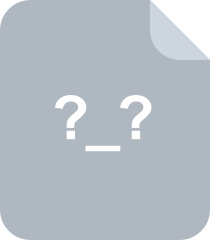
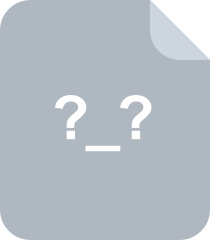
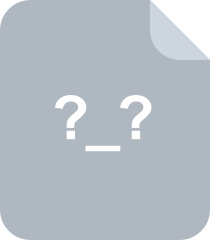
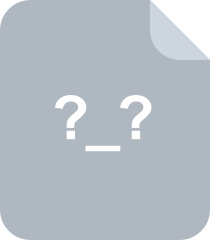
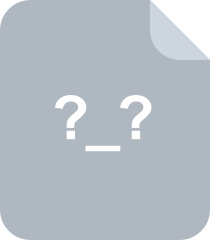
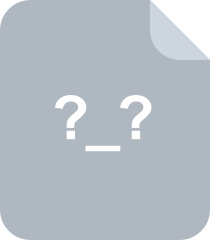
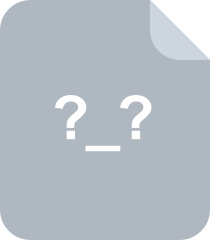
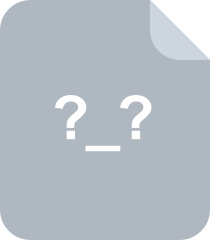
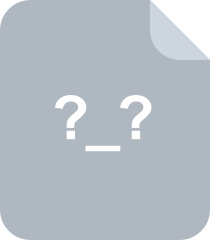
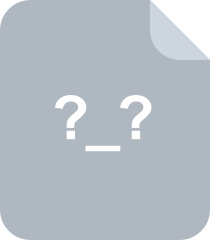
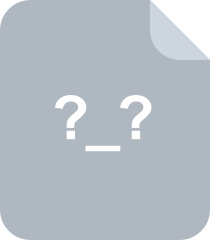
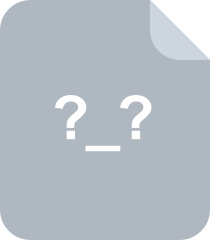
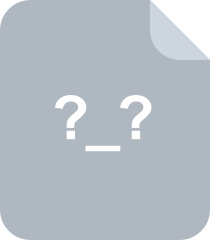
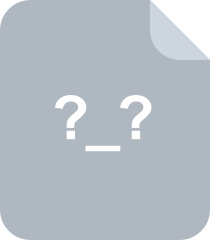
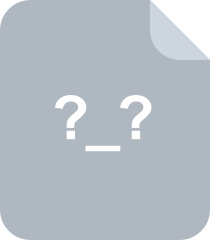
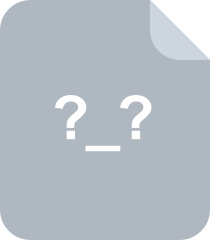
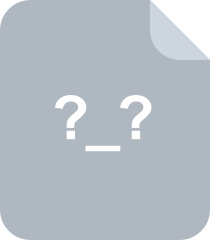
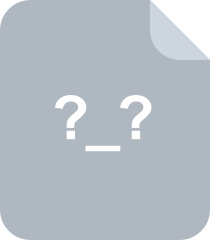
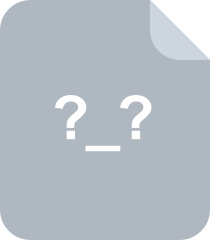
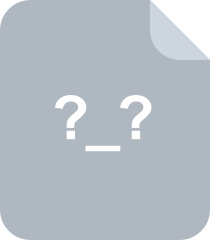
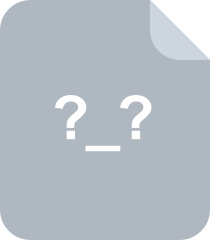
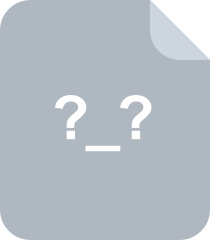
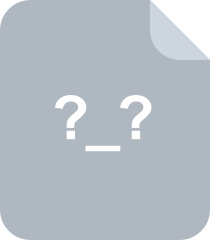
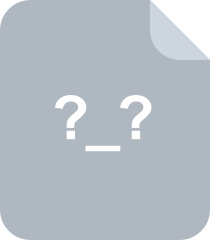
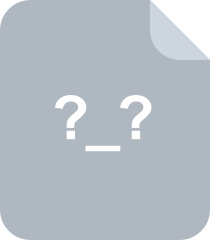
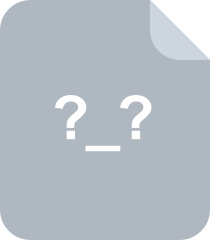
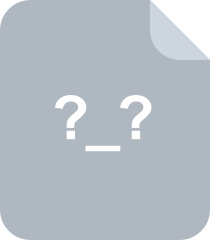
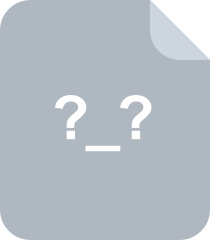
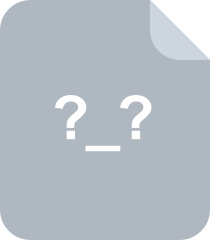
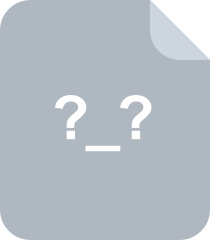
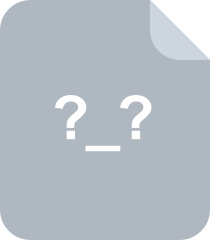
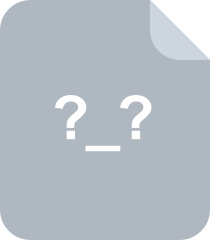
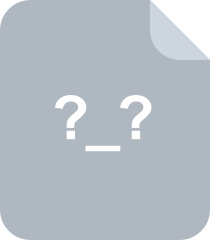
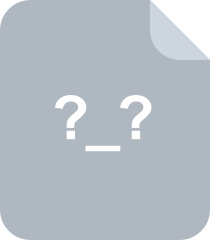
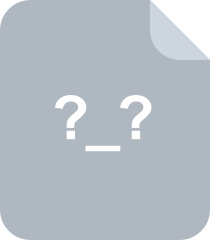
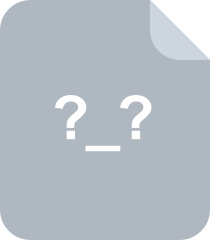
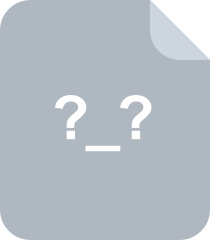
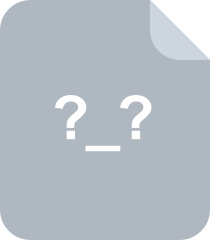
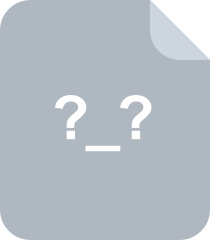
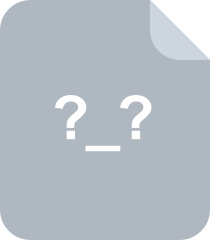
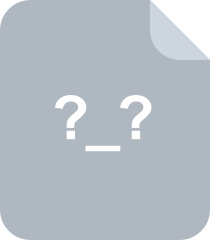
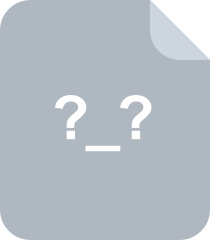
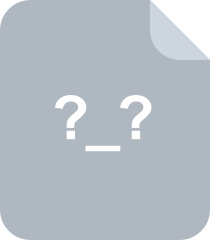
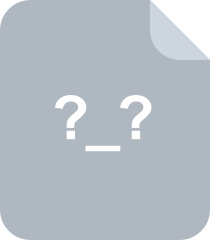
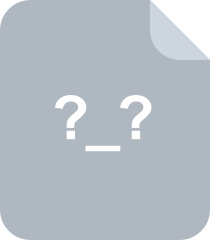
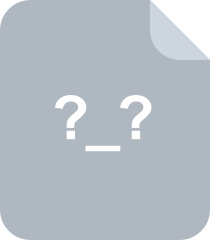
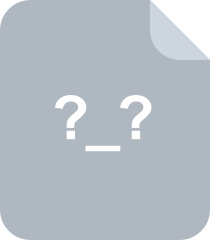
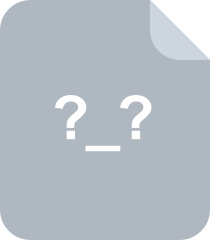
共 878 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
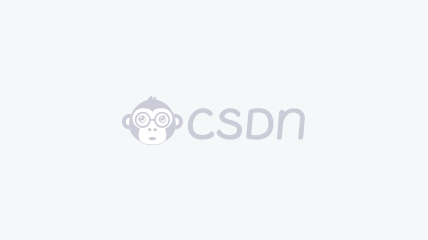

大学生资源网
- 粉丝: 137
- 资源: 1334
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

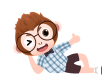
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


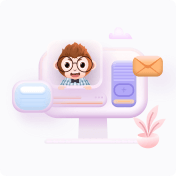
安全验证
文档复制为VIP权益,开通VIP直接复制
