tableExport.jquery.plugin
=========================
<h3>Export HTML Table to</h3>
<ul>
<li> CSV
<li> DOC
<li> JSON
<li> PDF
<li> PNG
<li> SQL
<li> TSV
<li> TXT
<li> XLS (Excel 2000 HTML format)
<li> XLSX (Excel 2007 Office Open XML format)
<li> XML (Excel 2003 XML Spreadsheet format)
<li> XML (Raw xml)
</ul>
Installation
============
To save the generated export files on client side, include in your html code:
```html
<script type="text/javascript" src="libs/FileSaver/FileSaver.min.js"></script>
```
To export the table in XLSX (Excel 2007+ XML Format) format, you need to include additionally:
```html
<script type="text/javascript" src="libs/js-xlsx/xlsx.core.min.js"></script>
```
To export an html table to a PDF file, you can use jsPDF-AutoTable as a PDF producer:
```html
<script type="text/javascript" src="libs/jsPDF/jspdf.min.js"></script>
<script type="text/javascript" src="libs/jsPDF-AutoTable/jspdf.plugin.autotable.js"></script>
```
Many HTML stylings can be converted to PDF with jsPDF, but support for non-western character sets is almost non-existent. Especially if you want to export Arabic or Chinese characters to your PDF file, you can use pdfmake as an alternative PDF producer. The disadvantage compared to jspdf is that using pdfmake has a reduced styling capability. To use pdfmake enable the pdfmake option and instead of the jsPDF files include
```html
<script type="text/javascript" src="libs/pdfmake/pdfmake.min.js"></script>
<script type="text/javascript" src="libs/pdfmake/vfs_fonts.js"></script>
<!-- To export arabic characters include mirza_fonts.js _instead_ of vfs_fonts.js
<script type="text/javascript" src="libs/pdfmake/mirza_fonts.js"></script>
-->
<!-- For a chinese font include either gbsn00lp_fonts.js or ZCOOLXiaoWei_fonts.js _instead_ of vfs_fonts.js
<script type="text/javascript" src="libs/pdfmake/gbsn00lp_fonts.js"></script>
-->
```
To export the table in PNG format, you need to include:
```html
<!-- For IE support include es6-promise before html2canvas -->
<script type="text/javascript" src="libs/es6-promise/es6-promise.auto.min.js"></script>
<script type="text/javascript" src="libs/html2canvas/html2canvas.min.js"></script>
```
Regardless of the desired format, finally include:
```html
<script type="text/javascript" src="tableExport.min.js"></script>
```
Please keep this include order.
Dependencies
============
Library | Version
--------|--------
[jQuery](https://github.com/jquery/jquery) | \>= 1.9.1
[es6-promise](https://github.com/stefanpenner/es6-promise) | \>= 4.2.4
[FileSaver](https://github.com/hhurz/tableExport.jquery.plugin/blob/master/libs/FileSaver/FileSaver.min.js) | \>= 1.2.0
[html2canvas](https://github.com/niklasvh/html2canvas) | \>= 0.5.0-beta4
[jsPDF](https://github.com/MrRio/jsPDF) | \>=1.3.4
[jsPDF-AutoTable](https://github.com/simonbengtsson/jsPDF-AutoTable) | 2.0.14 or 2.0.17
[pdfmake](https://github.com/bpampuch/pdfmake) | 0.1.65
[SheetJS](https://github.com/SheetJS/js-xlsx) | \>= 0.12.5
Examples
========
```
// CSV format
$('#tableID').tableExport({type:'csv'});
```
```
// Excel 2000 html format
$('#tableID').tableExport({type:'excel'});
```
```
// XML Spreadsheet 2003 file format with multiple worksheet support
$('table').tableExport({type:'excel',
mso: {fileFormat:'xmlss',
worksheetName: ['Table 1','Table 2', 'Table 3']}});
```
```
// PDF export using jsPDF only
$('#tableID').tableExport({type:'pdf',
jspdf: {orientation: 'p',
margins: {left:20, top:10},
autotable: false}
});
```
```
// PDF format using jsPDF and jsPDF Autotable
$('#tableID').tableExport({type:'pdf',
jspdf: {orientation: 'l',
format: 'a3',
margins: {left:10, right:10, top:20, bottom:20},
autotable: {styles: {fillColor: 'inherit',
textColor: 'inherit'},
tableWidth: 'auto'}
}
});
```
```
// PDF format with callback example
function DoCellData(cell, row, col, data) {}
function DoBeforeAutotable(table, headers, rows, AutotableSettings) {}
$('table').tableExport({fileName: sFileName,
type: 'pdf',
jspdf: {format: 'bestfit',
margins: {left:20, right:10, top:20, bottom:20},
autotable: {styles: {overflow: 'linebreak'},
tableWidth: 'wrap',
tableExport: {onBeforeAutotable: DoBeforeAutotable,
onCellData: DoCellData}}}
});
```
```
// PDF export using pdfmake
$('#tableID').tableExport({type:'pdf',
pdfmake:{enabled:true,
docDefinition:{pageOrientation:'landscape'}}
});
```
Options (Default settings)
=======
```
csvEnclosure: '"'
csvSeparator: ','
csvUseBOM: true
date: html: 'dd/mm/yyyy'
displayTableName: false (Deprecated)
escape: false (Deprecated)
exportHiddenCells: false
fileName: 'tableExport'
htmlContent: false
htmlHyperlink: 'content'
ignoreColumn: []
ignoreRow: []
jsonScope: 'all'
jspdf: orientation: 'p'
unit:'pt'
format: 'a4'
margins: left: 20
right: 10
top: 10
bottom: 10
onDocCreated: null
autotable: styles: cellPadding: 2
rowHeight: 12
fontSize: 8
fillColor: 255
textColor: 50
fontStyle: 'normal'
overflow: 'ellipsize'
halign: 'inherit'
valign: 'middle'
headerStyles: fillColor: [52, 73, 94]
textColor: 255
fontStyle: 'bold'
halign: 'inherit'
valign: 'middle'
alternateRowStyles: fillColor: 245
tableExport: doc: null
onAfterAutotable: null
onBeforeAutotable: null
onAutotableText: null
onTable: null
outputImages: true
mso: fileFormat: 'xlshtml'
onMsoNumberFormat: null
pageFormat: 'a4'
pageOrientation: 'portrait'
rtl: false
styles: []
worksheetName: ''
xslx: formatId: date: 14
numbers: 2
numbers: html: decimalMark: '.'
thousandsSeparator: ','
output: decimalMark: '.',
thousandsSeparator: ','
onAfterSaveToFile: null
onBeforeSaveToFile: null
onCellData: null
onCellHtmlData: null
onCellHtmlHyperlink: null
onIgnoreRow: null
onTableExportBegin: null
onTableExportEnd: null
outputMode: 'file'
pdfmake: enabled: false
docDefinition: pageSize: 'A4'
pageOrientation: 'portrait'
styles: header: background: '#34495E'
color: '#FFFFFF'
bold: true
alignment: 'center'
fillColor: '#34495E
alternateRow: fillColor: '#f5f5f5'
defaultStyle: color: '#000000'
fontSize: 8
font: 'Roboto'
fonts: {}
preserve: leadingWS: false
trail

办公模板库素材蛙
- 粉丝: 1698
- 资源: 2319
最新资源
- 基于Spring Boot和SSM框架的ERP进销存管理系统源码:单据流转、精细化的物流信息管理,前端细节设计,权限管理,透明背景与关联单据优化,基于Spring Boot与SSM框架的ERP进销存管
- 燃料电池与超级电容复合能量管理策略:Simulink仿真模型研究及其在混合储能系统中的应用,燃料电池与超级电容复合能量管理策略:Simulink仿真模型研究及其在混合储能系统中的应用,燃料电池电池超级
- 西门子PLC1500控制Fanuc机器人汽车焊装生产线自动化:Profinet通讯与多种模块集成应用,西门子PLC1500大型程序掌控汽车焊装生产线:涵盖Fanuc机器人、触摸屏、Profinet通讯
- 基于MATLAB的综合能源系统优化调度:结合需求响应与碳交易机制研究,综合能源系统优化调度:Matlab程序制定与碳交易机制下的综合需求响应应用,matlab程序制定,综合能源系统优化调度,综合需求响
- 电力市场出清程序:基于IEEE 14节点输电阻塞分析的机组与节点边际电价求解,利用拉格朗日乘子计算最优发电成本,采用MATLAB linprog函数实现,具备广泛参考价值 ,电力市场出清程序:基于IE
- 永磁同步电机双矢量模型预测控制仿真研究:MATLAB下的PMSM控制策略探索,永磁同步电机双矢量模型预测控制仿真研究:基于MATLAB平台的PMSM控制策略探索,永磁同步电机双矢量模型预测控制仿真PM
- 【毕业设计】Python的Django-html开放领域事件抽取系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html旅游城市关键词分析系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html旅游景点方面级别情感分析语料库与模型源码(完整前后端+mysql+说明文档+LW+PPT).zip
- LabVIEW利用DLL接口操作结构体指针:获取嵌套指针与混合类型数据处理策略,LabVIEW利用DLL获取结构体指针内嵌套指针元素及多种类型数据的实践方法,LabVIEW通过dll获取结构体指针中的
- 【毕业设计】Python的Django-html某大学学生影响力分析系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html棉花数据平台建设与可视化系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html小波变换的数字水印研究系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】Python的Django-html语音识别的智能垃圾分类系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 基于蒙特卡洛模拟的分布式电源(风光)概率潮流Matlab仿真研究:IEEE 33节点系统的分析与应用(包括牛拉法潮流计算与电压特性前后对比),基于蒙特卡洛方法的分布式电源(风光)概率潮流计算Matla
- 【毕业设计】Python的Django-html知识图谱的百科知识问答平台源码(完整前后端+mysql+说明文档+LW+PPT).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


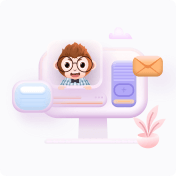