(global["webpackJsonp"] = global["webpackJsonp"] || []).push([
["common/vendor"],
[
/* 0 */
,
/* 1 */
/*!************************************************************!*\
!*** ./node_modules/@dcloudio/uni-mp-weixin/dist/index.js ***!
\************************************************************/
/*! no static exports found */
/***/
(function (module, exports, __webpack_require__) {
"use strict";
/* WEBPACK VAR INJECTION */
(function (global) {
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.createApp = createApp;
exports.createComponent = createComponent;
exports.createPage = createPage;
exports.createPlugin = createPlugin;
exports.createSubpackageApp = createSubpackageApp;
exports.default = void 0;
var _vue = _interopRequireDefault(__webpack_require__( /*! vue */ 3));
var _uniI18n = __webpack_require__( /*! @dcloudio/uni-i18n */ 4);
function _interopRequireDefault(obj) {
return obj && obj.__esModule ? obj : {
default: obj
};
}
function ownKeys(object, enumerableOnly) {
var keys = Object.keys(object);
if (Object.getOwnPropertySymbols) {
var symbols = Object.getOwnPropertySymbols(object);
if (enumerableOnly) symbols = symbols.filter(function (sym) {
return Object.getOwnPropertyDescriptor(object, sym).enumerable;
});
keys.push.apply(keys, symbols);
}
return keys;
}
function _objectSpread(target) {
for (var i = 1; i < arguments.length; i++) {
var source = arguments[i] != null ? arguments[i] : {};
if (i % 2) {
ownKeys(Object(source), true).forEach(function (key) {
_defineProperty(target, key, source[key]);
});
} else if (Object.getOwnPropertyDescriptors) {
Object.defineProperties(target, Object.getOwnPropertyDescriptors(source));
} else {
ownKeys(Object(source)).forEach(function (key) {
Object.defineProperty(target, key, Object.getOwnPropertyDescriptor(source, key));
});
}
}
return target;
}
function _slicedToArray(arr, i) {
return _arrayWithHoles(arr) || _iterableToArrayLimit(arr, i) || _unsupportedIterableToArray(arr, i) || _nonIterableRest();
}
function _nonIterableRest() {
throw new TypeError("Invalid attempt to destructure non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.");
}
function _iterableToArrayLimit(arr, i) {
if (typeof Symbol === "undefined" || !(Symbol.iterator in Object(arr))) return;
var _arr = [];
var _n = true;
var _d = false;
var _e = undefined;
try {
for (var _i = arr[Symbol.iterator](), _s; !(_n = (_s = _i.next()).done); _n = true) {
_arr.push(_s.value);
if (i && _arr.length === i) break;
}
} catch (err) {
_d = true;
_e = err;
} finally {
try {
if (!_n && _i["return"] != null) _i["return"]();
} finally {
if (_d) throw _e;
}
}
return _arr;
}
function _arrayWithHoles(arr) {
if (Array.isArray(arr)) return arr;
}
function _defineProperty(obj, key, value) {
if (key in obj) {
Object.defineProperty(obj, key, {
value: value,
enumerable: true,
configurable: true,
writable: true
});
} else {
obj[key] = value;
}
return obj;
}
function _toConsumableArray(arr) {
return _arrayWithoutHoles(arr) || _iterableToArray(arr) || _unsupportedIterableToArray(arr) || _nonIterableSpread();
}
function _nonIterableSpread() {
throw new TypeError("Invalid attempt to spread non-iterable instance.\nIn order to be iterable, non-array objects must have a [Symbol.iterator]() method.");
}
function _unsupportedIterableToArray(o, minLen) {
if (!o) return;
if (typeof o === "string") return _arrayLikeToArray(o, minLen);
var n = Object.prototype.toString.call(o).slice(8, -1);
if (n === "Object" && o.constructor) n = o.constructor.name;
if (n === "Map" || n === "Set") return Array.from(o);
if (n === "Arguments" || /^(?:Ui|I)nt(?:8|16|32)(?:Clamped)?Array$/.test(n)) return _arrayLikeToArray(o, minLen);
}
function _iterableToArray(iter) {
if (typeof Symbol !== "undefined" && Symbol.iterator in Object(iter)) return Array.from(iter);
}
function _arrayWithoutHoles(arr) {
if (Array.isArray(arr)) return _arrayLikeToArray(arr);
}
function _arrayLikeToArray(arr, len) {
if (len == null || len > arr.length) len = arr.length;
for (var i = 0, arr2 = new Array(len); i < len; i++) {
arr2[i] = arr[i];
}
return arr2;
}
var realAtob;
var b64 = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/=';
var b64re = /^(?:[A-Za-z\d+/]{4})*?(?:[A-Za-z\d+/]{2}(?:==)?|[A-Za-z\d+/]{3}=?)?$/;
if (typeof atob !== 'function') {
realAtob = function realAtob(str) {
str = String(str).replace(/[\t\n\f\r ]+/g, '');
if (!b64re.test(str)) {
throw new Error("Failed to execute 'atob' on 'Window': The string to be decoded is not correctly encoded.");
}
// Adding the padding if missing, for semplicity
str += '=='.slice(2 - (str.length & 3));
var bitmap;
var result = '';
var r1;
var r2;
var i = 0;
for (; i < str.length;) {
bitmap = b64.indexOf(str.charAt(i++)) << 18 | b64.indexOf(str.charAt(i++)) << 12 |
(r1 = b64.indexOf(str.charAt(i++))) << 6 | (r2 = b64.indexOf(str.charAt(i++)));
result += r1 === 64 ? String.fromCharCode(bitmap >> 16 & 255) :
r2 === 64 ? String.fromCharCode(bitmap >> 16 & 255, bitmap >> 8 & 255) :
String.fromCharCode(bitmap >> 16 & 255, bitmap >> 8 & 255, bitmap & 255);
}
return result;
};
} else {
// 注意atob只能在全局对象上调用,例如:`const Base64 = {atob};Base64.atob('xxxx')`是错误的用法
realAtob = atob;
}
function b64DecodeUnicode(str) {
return decodeURIComponent(realAtob(str).split('').map(function (c) {
return '%' + ('00' + c.charCodeAt(0).toString(16)).slice(-2);
}).join(''));
}
function getCurrentUserInfo() {
var token = wx.getStorageSync('uni_id_token') || '';
var tokenArr = token.split('.');
if (!token || tokenArr.length !== 3) {
return {
uid: null,
role: [],
permission: [],
tokenExpired: 0
};
}
var userInfo;
try {
userInfo = JSON.parse(b64DecodeUnicode(tokenArr[1]));
} catch (error) {
throw new Error('获取当前用户信息出错,详细错误信息为:' + error.message);
}
userInfo.tokenExpired = userInfo.exp * 1000;
delete userInfo.exp;
delete userInfo.iat;
return userInfo;
}
function uniIdMixin(Vue) {
Vue.prototype.uniIDHasRole = function (roleId) {
var _getCurrentUserInf
没有合适的资源?快使用搜索试试~ 我知道了~
天气预报微信小程序源码
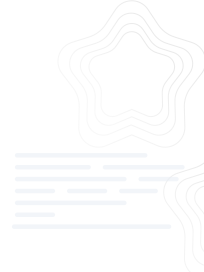
共64个文件
js:16个
json:15个
wxss:14个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
为您提供实时全国天气气象信息,及时发布天气预报、灾害预警、气象云图、旅游天气、台风、暴雨雪等气象信息,为我国的生产生活提供全面精确的气象服务 功能: 1. 实时天气获取 2. 未来三天天气展示 3. 高温显示 4. 小程序背景颜色修改(默认4个) 技术: 1. vue 2. uniapp 代码说明: 1. 替换 project.config.json 文件中 第 44行 appid 为 你的wx小程序id 2. 替换 .\common 目录下 vendor.js 文件第 22050 行“applicationKey”、“qweatherKey”对应的key applicationKey : 腾讯云地图api 申请地址:注册 (qq.com) qweatherKey: 和风天气api 申请地址 :注册和风天气 部署时,小程序后台-开发设置-服务器域名 添加 https://apis.map.qq.com https://devapi.qweather.com
资源推荐
资源详情
资源评论
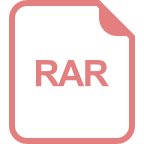
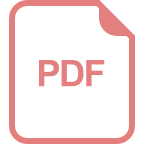
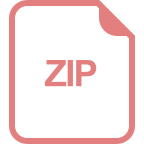
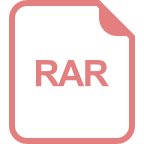
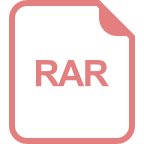
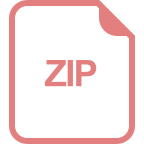
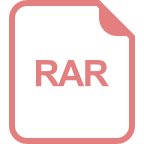
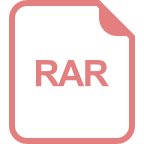
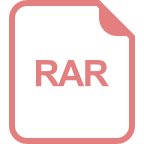
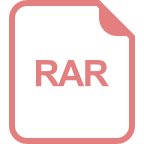
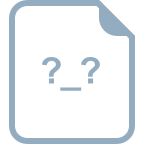
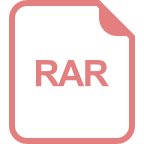
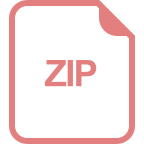
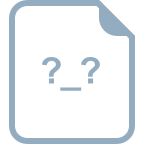
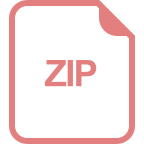
收起资源包目录

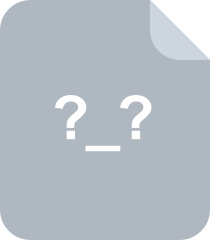


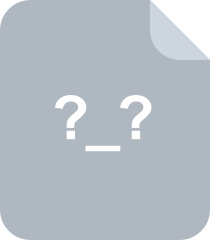
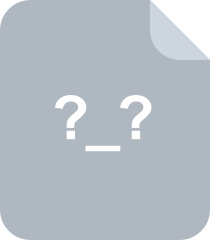
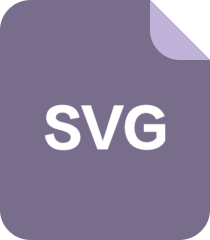
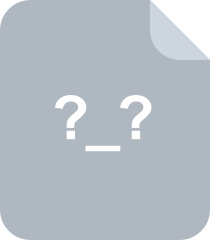
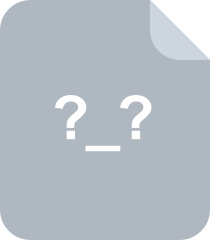
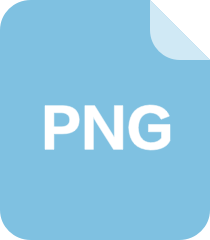


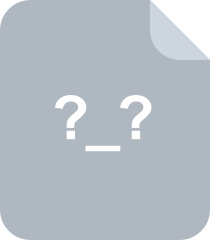
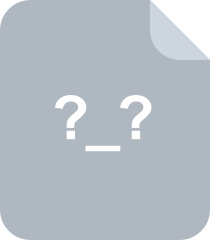
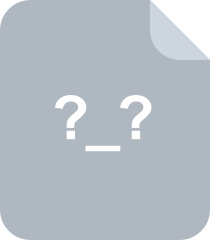
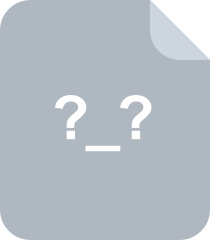

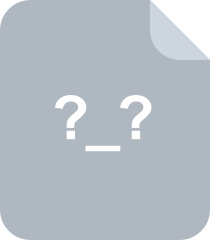
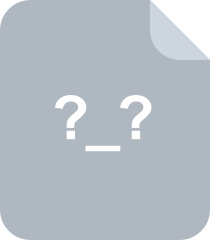
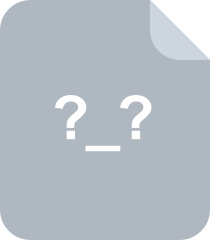
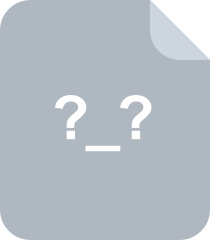




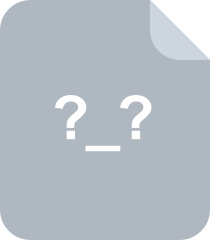
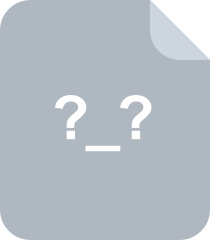
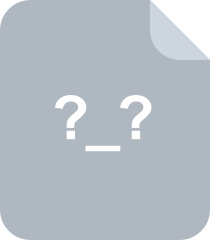
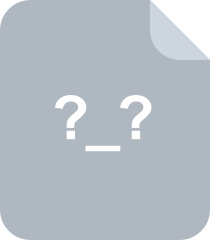

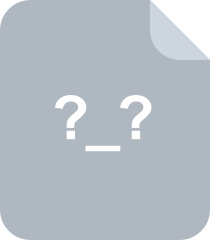
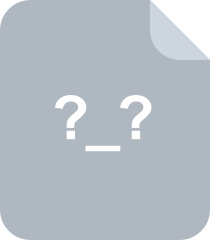
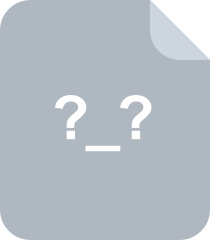
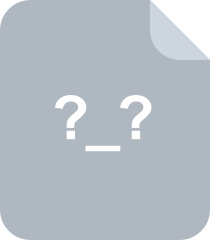

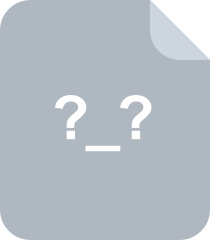
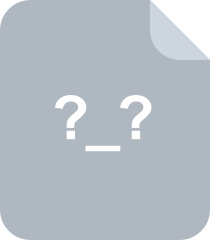
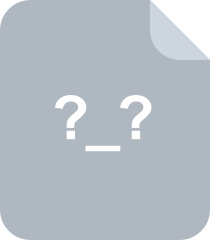
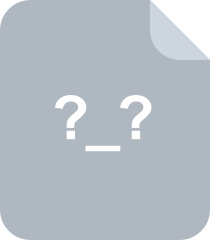

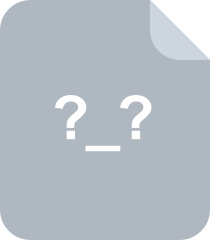
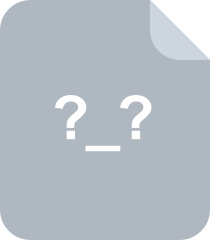
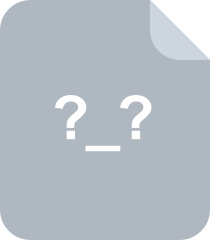
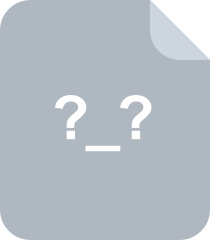

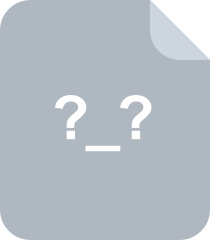
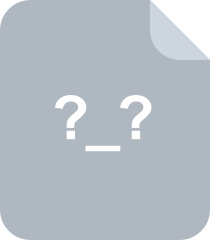
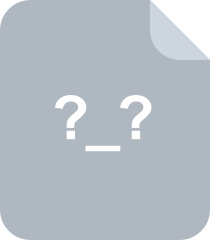
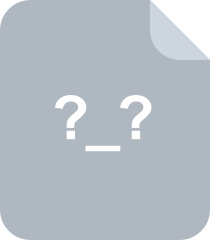

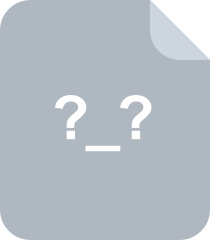
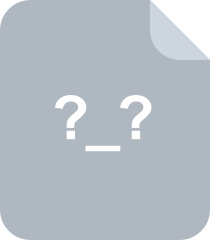
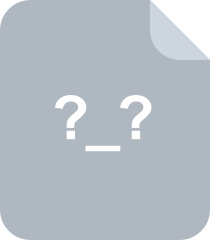
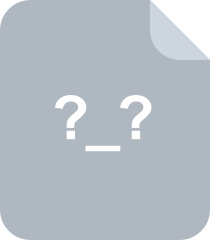

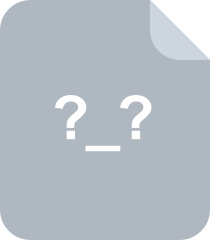
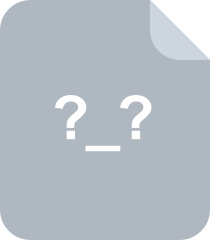
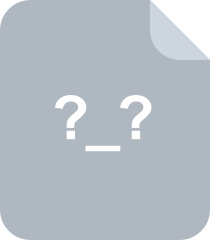
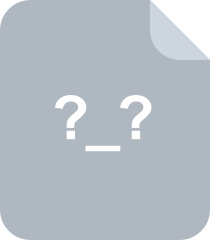

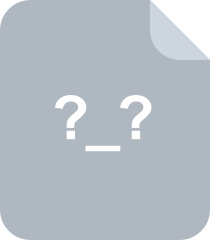
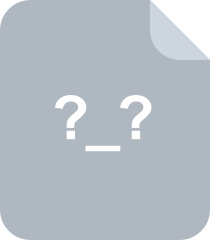
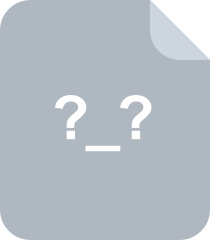
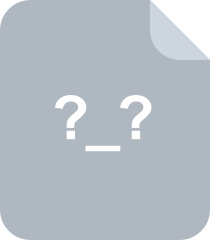

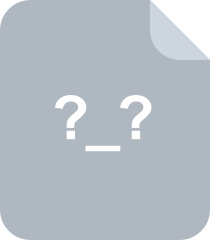
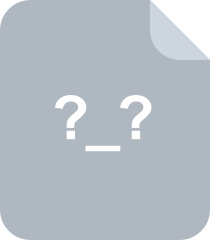
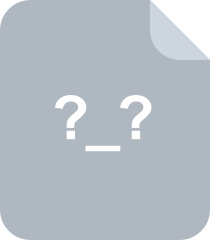
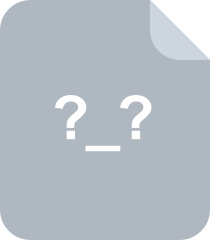

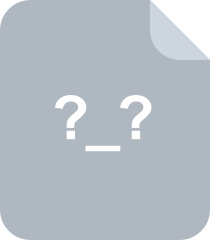
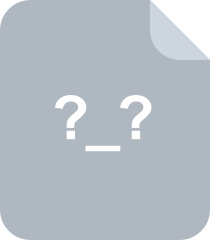
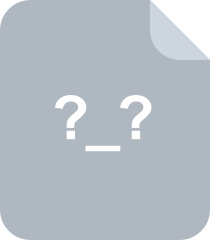
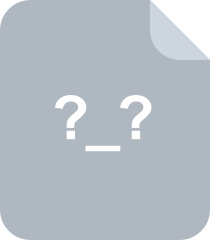

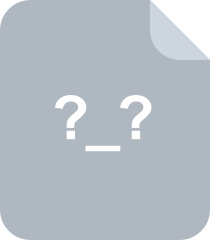
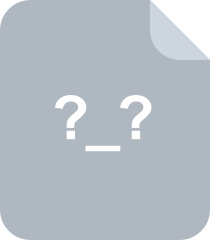
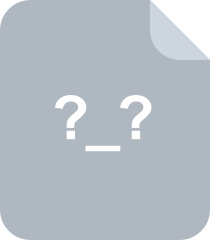
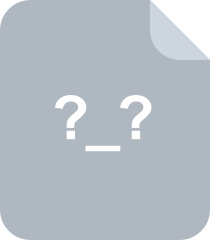
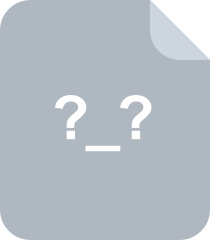
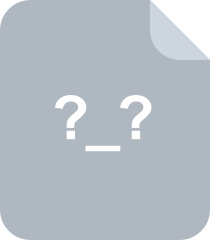
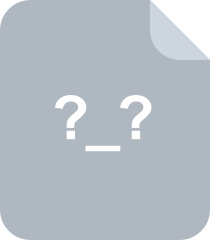
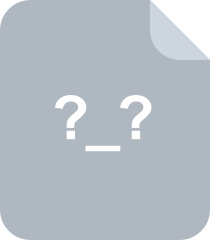
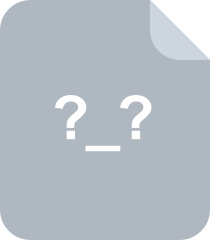
共 64 条
- 1

办公模板库素材蛙
- 粉丝: 1660
- 资源: 2299
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

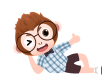
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


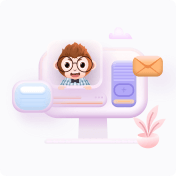
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页