package com.ideabobo.tool;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.channels.FileChannel;
import android.content.Context;
import android.content.Intent;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Environment;
import android.util.Log;
public class FileTool extends AsyncTask<String, Void, Integer>{
private static final String COMMAND_BACKUP = "backupDatabase";
public static final String COMMAND_RESTORE = "restroeDatabase";
private Context mContext;
public FileTool(Context context) {
this.mContext = context;
}
@Override
public Integer doInBackground(String... params) {
// TODO Auto-generated method stub
// 获得正在使用的数据库路径,我的是 sdcard 目录下的 /dlion/db_dlion.db
// 默认路径是 /data/data/(包名)/databases/*.db
// File dbFile = mContext.getDatabasePath(Environment
// .getExternalStorageDirectory().getAbsolutePath()
// + "/dlion/db_dlion.db");
File dbFileDir = mContext.getDatabasePath("/data/data/com.ideabobo.gap/");
File exportDir = new File(Environment.getExternalStorageDirectory(),
"ideaback/");
if (!exportDir.exists()) {
exportDir.mkdirs();
}
String command = params[0];
if (command.equals(COMMAND_BACKUP)) {
try {
copyDirectiory(dbFileDir.getAbsolutePath(), exportDir.getAbsolutePath());
return Log.d("backup", "ok");
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
return Log.d("backup", "fail");
}
} else if (command.equals(COMMAND_RESTORE)) {
try {
copyDirectiory(exportDir.getAbsolutePath(), dbFileDir.getAbsolutePath());
return Log.d("restore", "success");
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
return Log.d("restore", "fail");
}
} else {
return null;
}
}
private void fileCopy(File dbFile, File backup) throws IOException {
// TODO Auto-generated method stub
FileChannel inChannel = new FileInputStream(dbFile).getChannel();
FileChannel outChannel = new FileOutputStream(backup).getChannel();
try {
inChannel.transferTo(0, inChannel.size(), outChannel);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
if (inChannel != null) {
inChannel.close();
}
if (outChannel != null) {
outChannel.close();
}
}
}
// 复制文件夹
public static void copyDirectiory(String sourceDir, String targetDir) throws IOException {
// 新建目标目录
(new File(targetDir)).mkdirs();
// 获取源文件夹当前下的文件或目录
File[] file = (new File(sourceDir)).listFiles();
for (int i = 0; i < file.length; i++) {
if (file[i].isFile()) {
// 源文件
File sourceFile = file[i];
// 目标文件
File targetFile = new File(new File(targetDir).getAbsolutePath() + File.separator + file[i].getName());
copyFile(sourceFile, targetFile);
}
else if (file[i].isDirectory()) {
// 准备复制的源文件夹
String dir1 = sourceDir + "/" + file[i].getName();
// 准备复制的目标文件夹
String dir2 = targetDir + "/" + file[i].getName();
copyDirectiory(dir1, dir2);
}
}
}
// 复制文件
public static void copyFile(File sourceFile, File targetFile) throws IOException {
BufferedInputStream inBuff = null;
BufferedOutputStream outBuff = null;
try {
// 新建文件输入流并对它进行缓冲
inBuff = new BufferedInputStream(new FileInputStream(sourceFile));
// 新建文件输出流并对它进行缓冲
outBuff = new BufferedOutputStream(new FileOutputStream(targetFile));
// 缓冲数组
byte[] b = new byte[1024 * 5];
int len;
while ((len = inBuff.read(b)) != -1) {
outBuff.write(b, 0, len);
}
// 刷新此缓冲的输出流
outBuff.flush();
} finally {
// 关闭流
if (inBuff != null)
inBuff.close();
if (outBuff != null)
outBuff.close();
}
}
public static void delFolder(String folderPath) {
try {
delAllFile(folderPath); //删除完里面所有内容
String filePath = folderPath;
filePath = filePath.toString();
java.io.File myFilePath = new java.io.File(filePath);
myFilePath.delete(); //删除空文件夹
} catch (Exception e) {
e.printStackTrace();
}
}
//删除指定文件夹下所有文件
//param path 文件夹完整绝对路径
public static boolean delAllFile(String path) {
boolean flag = false;
File file = new File(path);
if (!file.exists()) {
return flag;
}
if (!file.isDirectory()) {
return flag;
}
String[] tempList = file.list();
File temp = null;
for (int i = 0; i < tempList.length; i++) {
if (path.endsWith(File.separator)) {
temp = new File(path + tempList[i]);
} else {
temp = new File(path + File.separator + tempList[i]);
}
if (temp.isFile()) {
temp.delete();
}
else if (temp.isDirectory()) {
delAllFile(path + "/" + tempList[i]);//先删除文件夹里面的文件
delFolder(path + "/" + tempList[i]);//再删除空文件夹
flag = true;
}
}
return flag;
}
public static String getSDPath(){
File sdDir = null;
boolean sdCardExist = Environment.getExternalStorageState()
.equals(android.os.Environment.MEDIA_MOUNTED); //判断sd卡是否存在
if(sdCardExist){
sdDir = Environment.getExternalStorageDirectory();//获取跟目录
}
return sdDir.toString();
}
public static final String[][] MIME_MapTable={
//{后缀名, MIME类型}
{".3gp", "video/3gpp"},
{".apk", "application/vnd.android.package-archive"},
{".asf", "video/x-ms-asf"},
{".avi", "video/x-msvideo"},
{".bin", "application/octet-stream"},
{".bmp", "image/bmp"},
{".c", "text/plain"},
{".class", "application/octet-stream"},
{".conf", "text/plain"},
{".cpp", "text/plain"},
{".doc", "application/msword"},
{".docx", "application/vnd.openxmlformats-officedocument.wordprocessingml.document"},
{".xls", "application/vnd.ms-excel"},
{".xlsx", "application/vnd.openxmlformats-officedocument.spreadsheetml.shee
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
源码描述: 一、源码介绍 城市公交线路查询系统系统包括app客户端和后台管理端,采用Android+JSP技术设计,基于C/S+B/S设计模式,以MySQL数据库来存储系统的数据。 二、主要功能 app客户端实现的功能包括:注册登录、换乘查询、线路查询、站点查询以及个人设置; 后台管理端实现的功能包括:登录、公交数据管理以及用户管理等 三、注意事项 开发环境为eclipse,myeclipse,数据库为 mysql
资源推荐
资源详情
资源评论
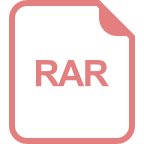
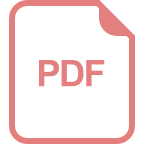
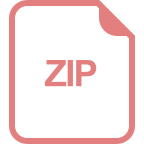
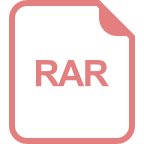
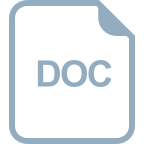
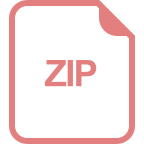
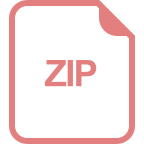
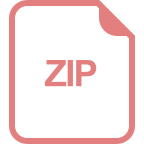
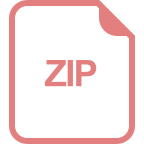
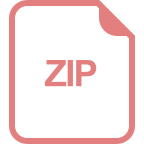
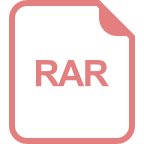
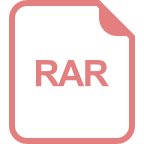
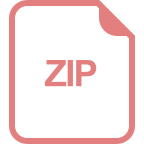
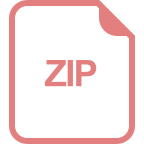
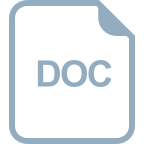
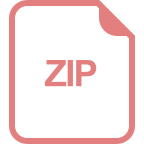
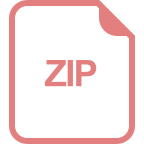
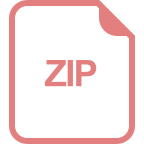
收起资源包目录

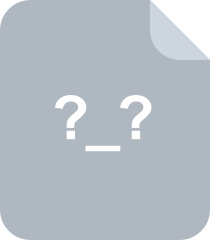
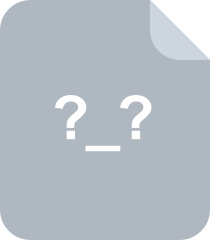
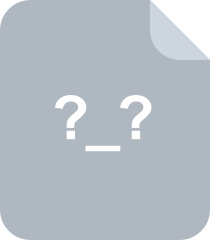
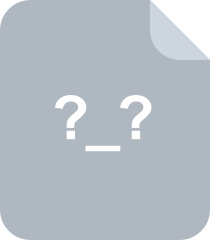
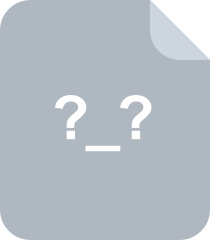
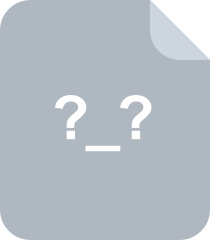
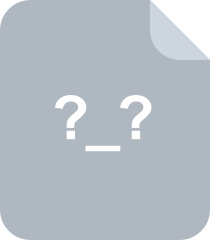
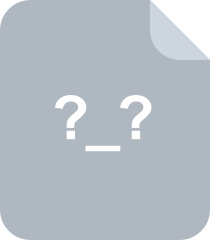
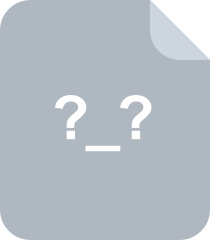
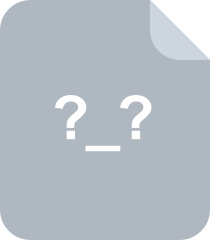
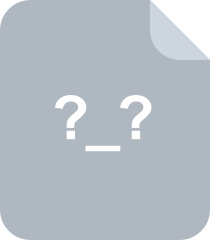
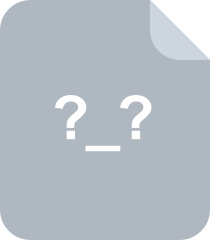
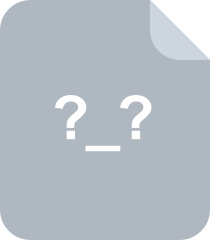
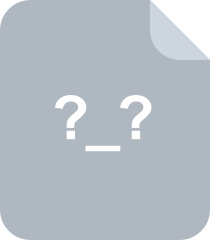
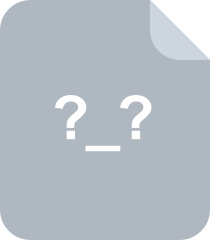
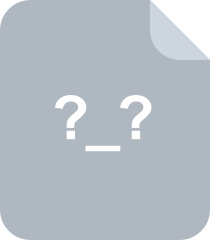
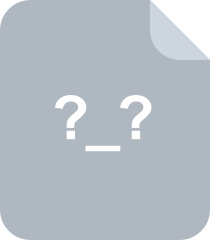
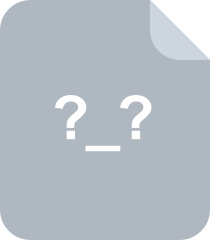
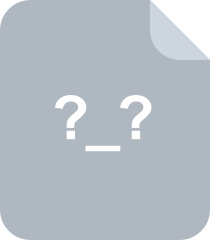
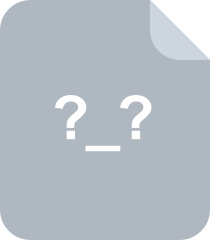
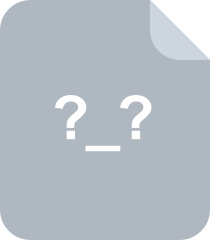
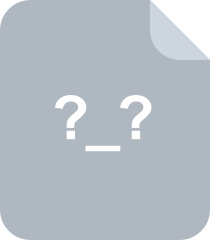
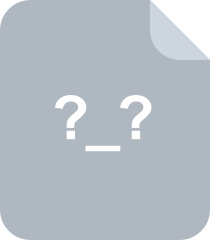
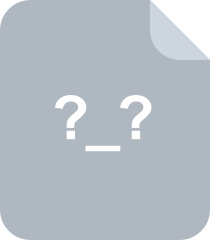
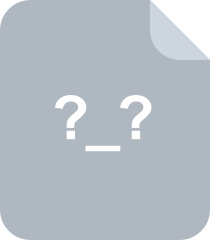
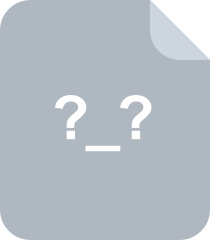
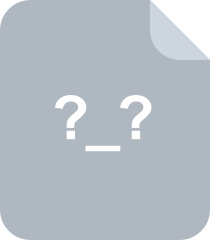
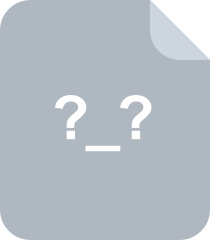
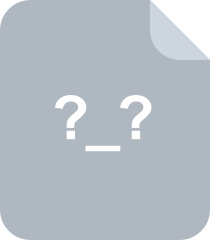
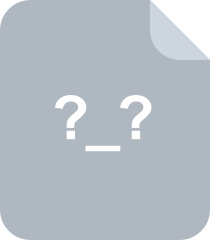
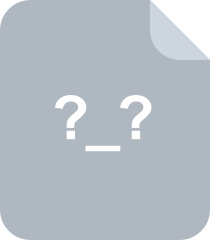
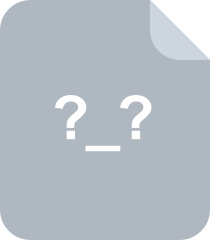
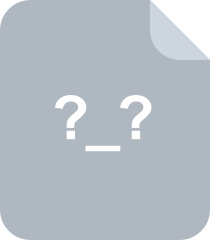
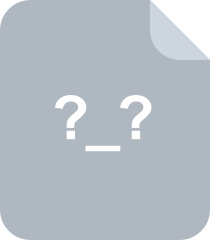
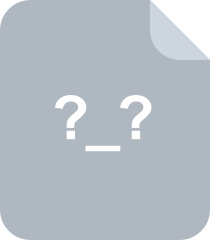
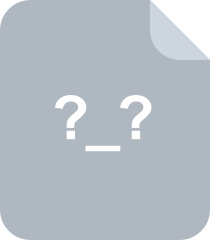
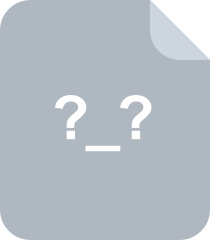
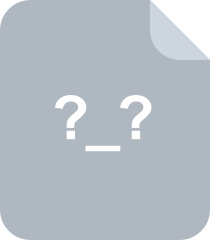
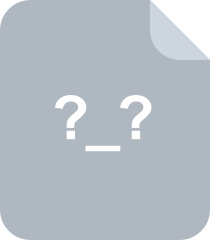
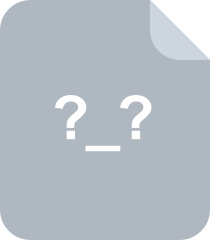
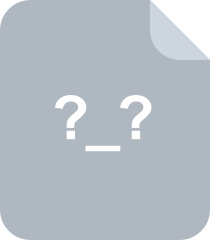
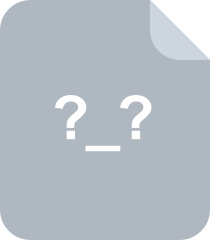
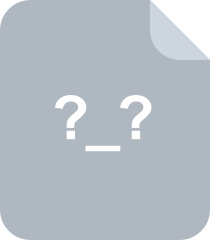
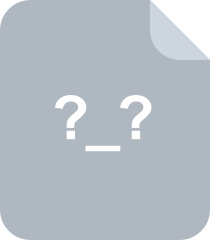
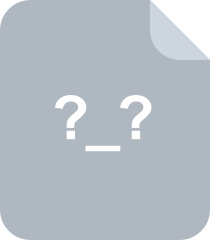
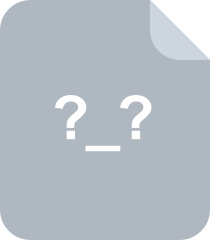
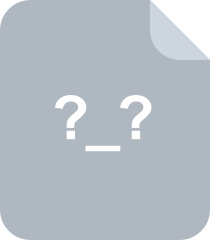
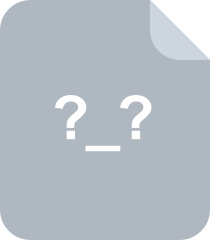
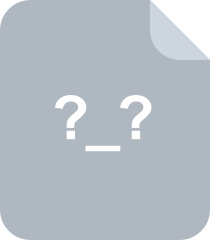
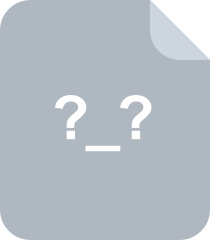
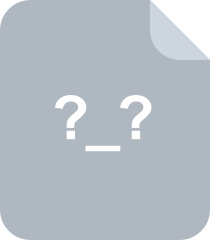
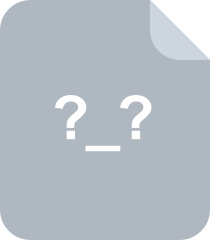
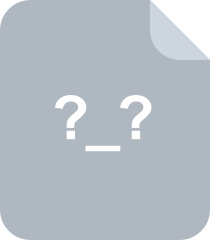
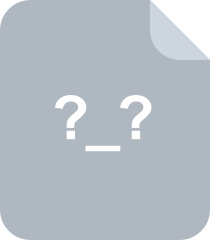
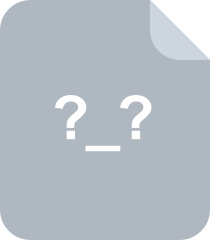
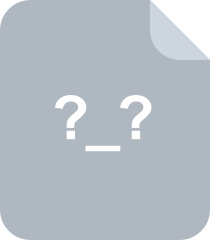
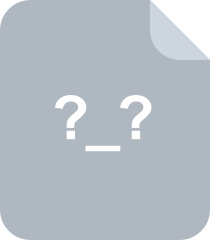
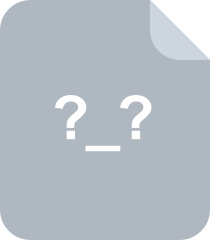
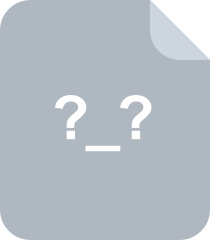
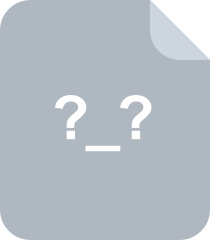
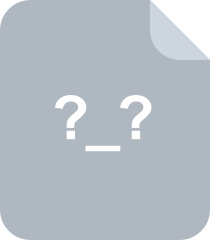
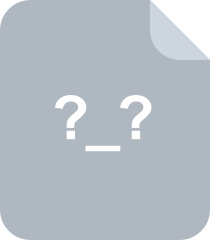
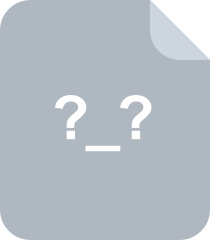
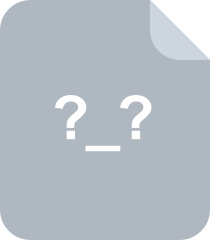
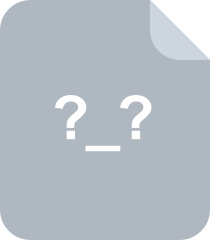
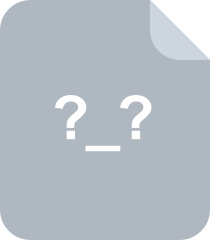
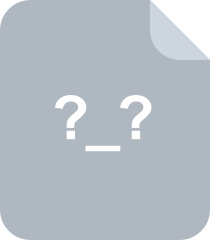
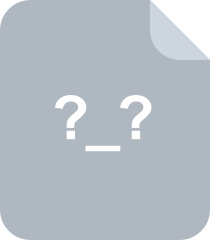
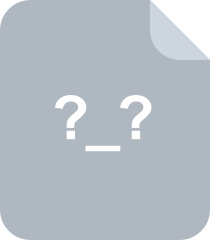
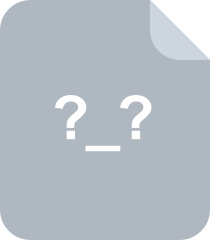
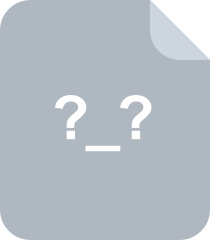
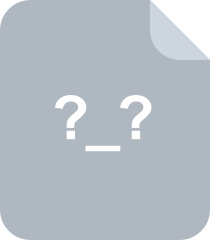
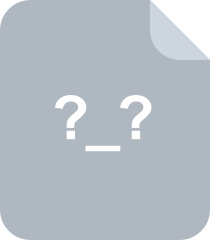
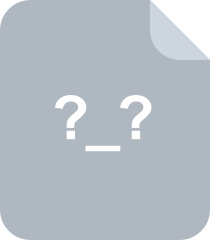
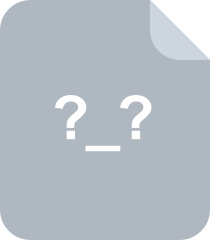
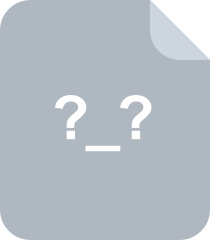
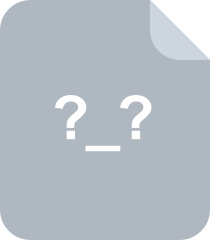
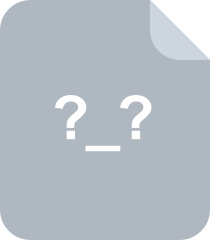
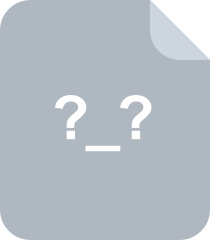
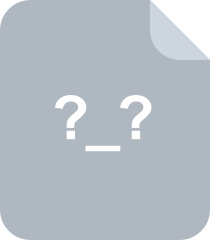
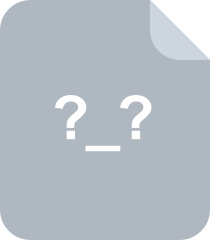
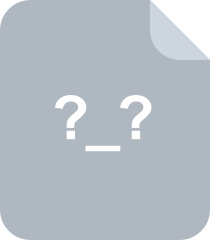
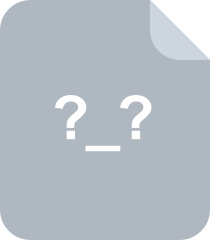
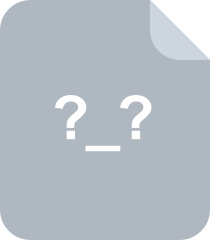
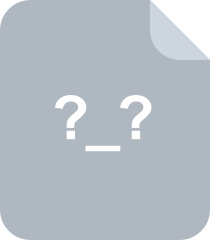
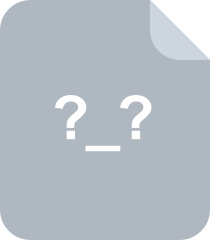
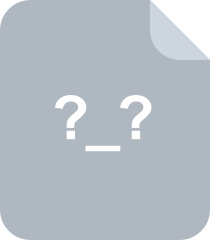
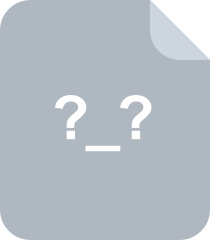
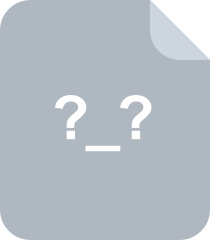
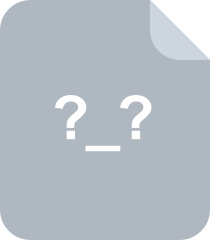
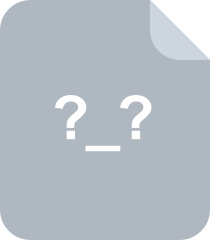
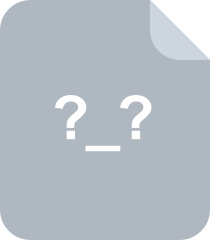
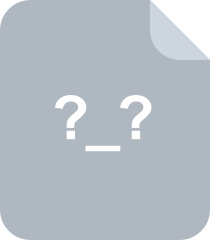
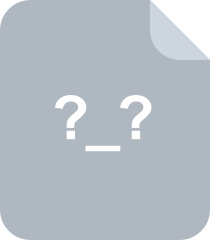
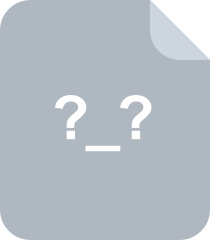
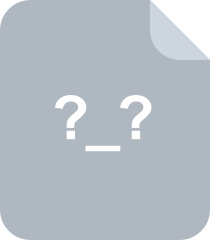
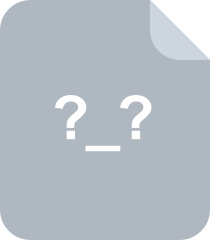
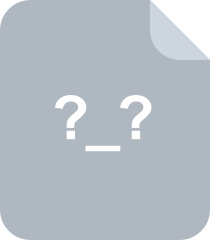
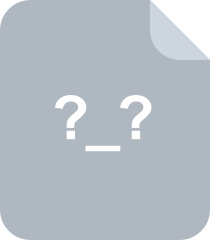
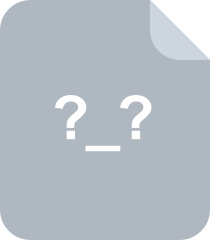
共 1709 条
- 1
- 2
- 3
- 4
- 5
- 6
- 18
资源评论
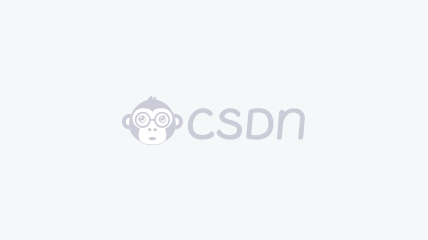
- ShenWW_2022-04-26用户下载后在一定时间内未进行评价,系统默认好评。

办公模板库素材蛙
- 粉丝: 1682
- 资源: 2301
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

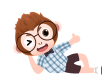
最新资源
- 线性块码实现汉明码(7,4)Matlab代码.rar
- 相位偏移键控调制 8PSK附matlab代码.rar
- 相移键控 8PSK 调制Matlab代码.rar
- 信道编码中使用的两种卷积码的误码率(BER)比较Matlab代码.rar
- 研究正交幅度调制(QAM)中的相位误差检测.rar
- 一个用于FSK调制和解调方案的MATLAB代码.rar
- 一个模拟Alamouti空间时间码的Matlab函数.rar
- 循环前缀和直接序列扩频用于BPSK、QPSK和16QAM调制Matlab代码.rar
- 选择性无线信道中模拟了OFDM系统。同时模拟了相干和非相干情况Matlab代码.rar
- 选择性无线信道中模拟了OFDM系统。同时模拟了相干和非相干情况Matlanb代码.rar
- 硬决策块码BPSK的BER曲线Matlab代码.rar
- 用于 ASK 调制和解调的 MATLAB 代码.rar
- 医学影像阅读器和查看器Matlab代码.rar
- 用于 BPSK、QPSK 和 16QAM 调制的直接序列扩频 (DSSS)Matlab代码.rar
- 用于 FSK 调制和解调的 MATLAB 代码.rar
- 用于 MIMO 仿真的空间信道模型。基于 3GPP TR 25.996 v.6.1.0 的基于 Ray 的模拟器Matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


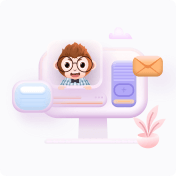
安全验证
文档复制为VIP权益,开通VIP直接复制
