package org.Test;
import org.usance.domain.BinarySource;
import java.util.ArrayList;
import java.util.List;
/**
* @author wuangjing
* @create 2020/5/16-15:41
* @Description:
*/
public class BinaryTreeTemporary {
private static BinarySource b = new BinarySource(null, 0, null);
private static List<BinarySource> list = new ArrayList<BinarySource>();
public static void main(String[] args) {
b = addBinaryNode(b, 1, 2);
BinarySource source1 = addBinaryNode(b.getSecondSource(), 11, 12);
BinarySource source2 = addBinaryNode(b.getSecondarySource(), 21, 22);
addBinaryNode(source1.getSecondSource(), 23, 45);
addBinaryNode(source1.getSecondarySource(), 35, 66);
addBinaryNode(source2.getSecondSource(), 46, 67);
addBinaryNode(source2.getSecondarySource(), 77, 89);
sequenceIterationBefore(b);
System.out.print("前序遍历结果:");
for (BinarySource source : list) {
System.out.println(source.getData());
}
System.out.println("------------");
System.out.println("树的深度:"+getTreeDepth(b));
}
/**
* 前序遍历
*
* @param binaryS
*/
public static void sequenceIterationBefore(BinarySource binaryS) {
list.add(binaryS);
if (binaryS.getSecondSource() != null) {
sequenceIterationBefore(binaryS.getSecondSource());
}
if (binaryS.getSecondarySource() != null) {
sequenceIterationBefore(binaryS.getSecondarySource());
}
return;
}
/**
* 中序遍历
*
* @param binaryS
*/
public static void inTheSequenceIteration(BinarySource binaryS) {
if (binaryS.getSecondSource() != null) {
inTheSequenceIteration(binaryS.getSecondSource());
}
list.add(binaryS);
if (binaryS.getSecondarySource() != null) {
inTheSequenceIteration(binaryS.getSecondarySource());
}
return;
}
/**
* 后序遍历
*
* @param binaryS
*/
public static void afterTheSequenceIteration(BinarySource binaryS) {
if (binaryS.getSecondSource() != null) {
inTheSequenceIteration(binaryS.getSecondSource());
}
if (binaryS.getSecondarySource() != null) {
inTheSequenceIteration(binaryS.getSecondarySource());
}
list.add(binaryS);
return;
}
/**
* 深度遍历
* @param binaryS
* @return
*/
public static int getTreeDepth(BinarySource binaryS) {
if (binaryS.getSecondSource() == null && binaryS.getSecondarySource() == null) {
return 1;
}
int left = 0, right = 0;
if (binaryS.getSecondSource() != null) {
left = getTreeDepth(binaryS.getSecondSource());
}
if (binaryS.getSecondarySource() != null) {
right = getTreeDepth(binaryS.getSecondarySource());
}
return left > right ? left + 1 : right + 1;
}
/**
* 链式添加结点
*
* @param binarySource
* @param LeftValue
* @param RightValue
* @return
*/
public static BinarySource addBinaryNode(BinarySource binarySource, Integer LeftValue, Integer RightValue) {
if (LeftValue != null) {
binarySource.setSecondSource(new BinarySource(null, LeftValue, null));
}
if (RightValue != null) {
binarySource.setSecondarySource(new BinarySource(null, RightValue, null));
}
return binarySource;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
java云盘后端服务器+前端electron前后端分离式+简单部署教程
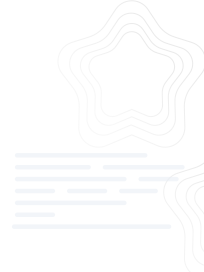
共749个文件
gif:154个
pak:112个
js:88个

需积分: 47 3 下载量 166 浏览量
2022-03-19
11:54:35
上传
评论
收藏 120.46MB 7Z 举报
温馨提示
云盘源码java后端服务器+前端electron前后端分离式自从学习了一点点electron基础命令(也就是几个标准单词),我就突发奇想,想写一款桌面基本的应用,但是也不知道该写什么demo,然后就这样子,想啊想…,直到发生了震惊国人pandownload事件,我痛恨百度云的机制,但也无法忘记它带给我们的资源, 所有我就想尝试写一个页面稍微可以上台面的桌面云盘应用。
资源详情
资源评论
资源推荐
收起资源包目录

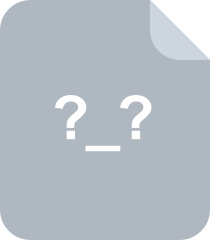
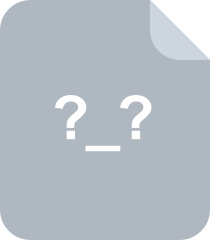
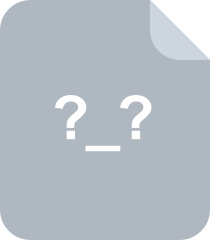
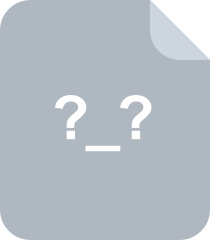
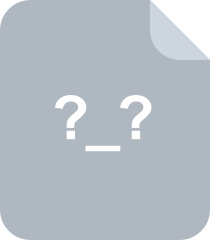
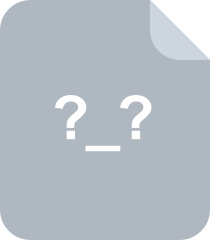
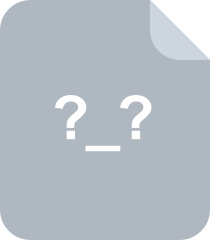
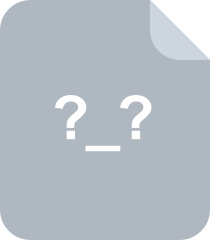
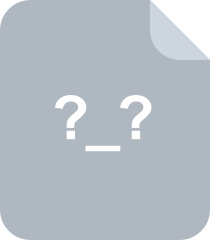
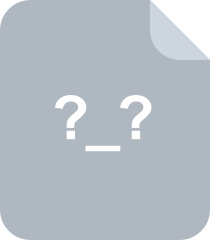
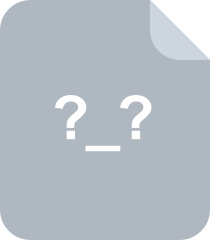
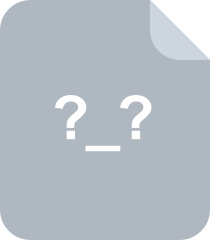
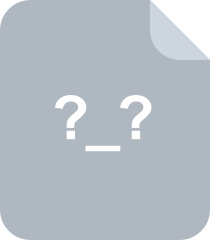
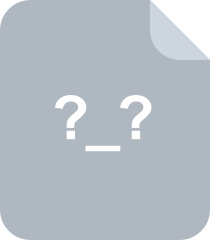
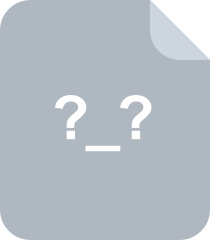
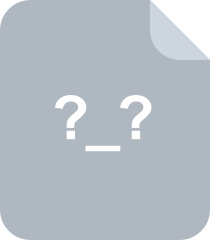
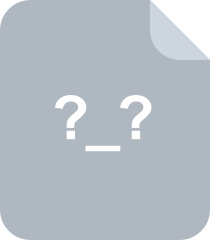
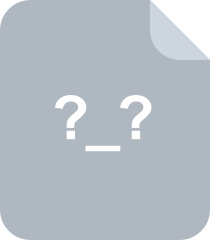
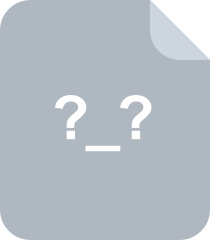
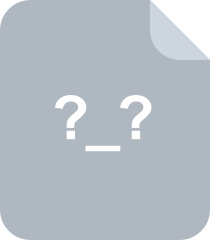
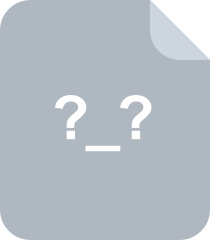
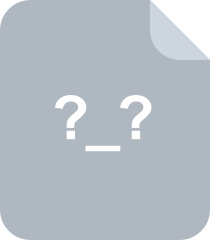
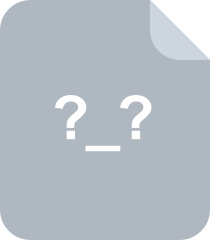
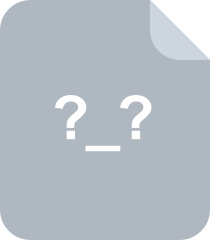
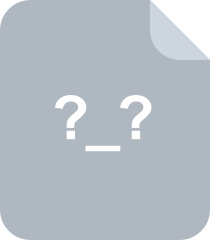
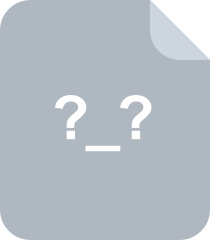
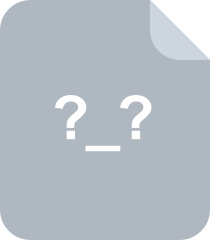
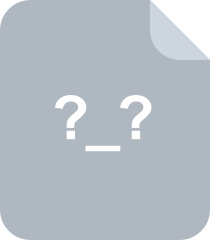
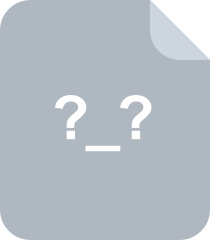
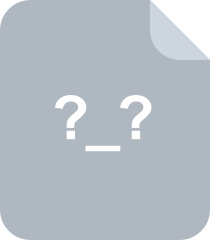
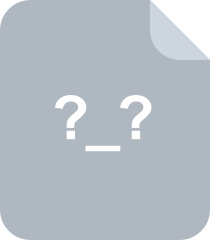
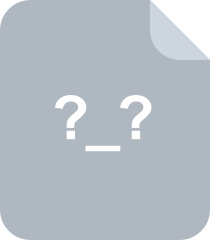
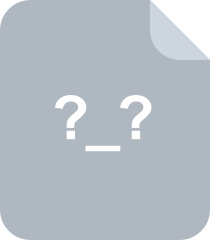
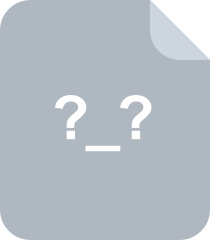
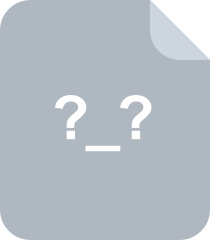
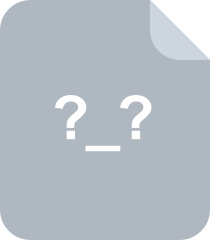
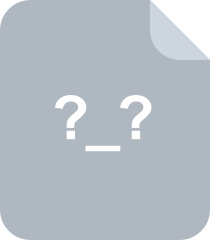
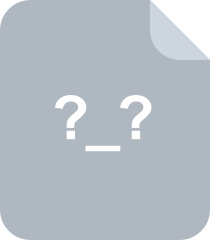
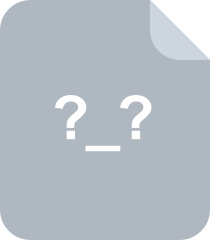
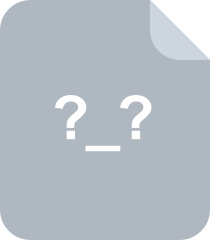
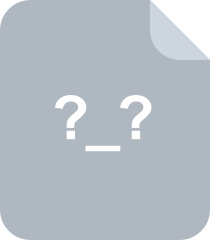
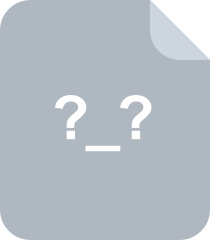
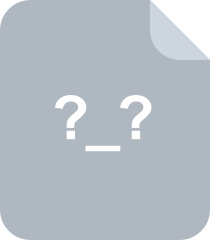
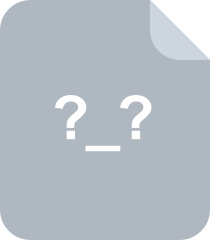
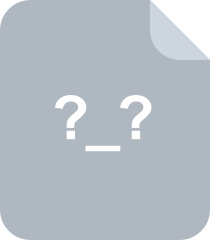
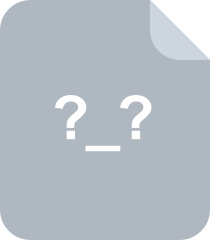
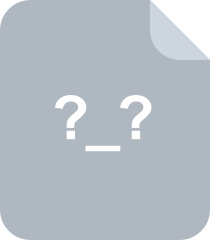
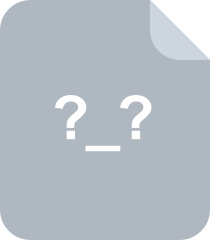
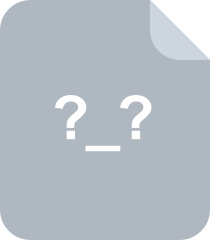
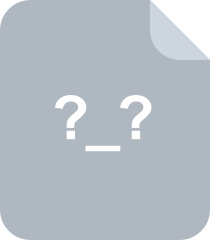
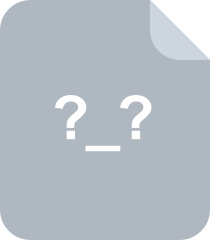
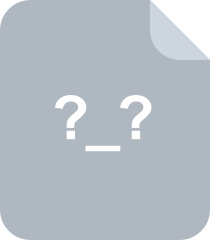
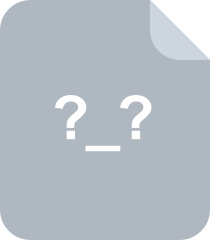
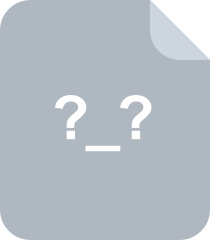
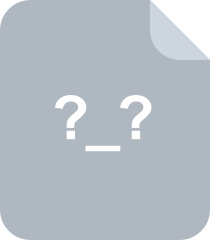
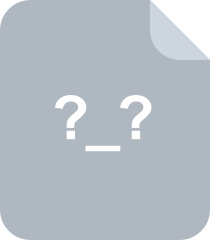
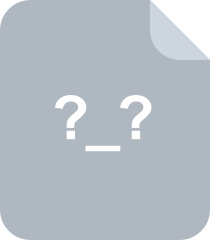
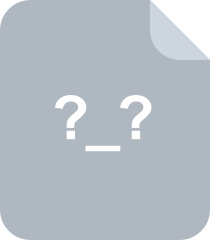
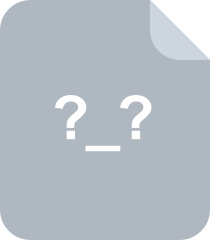
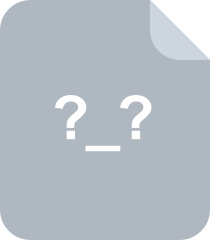
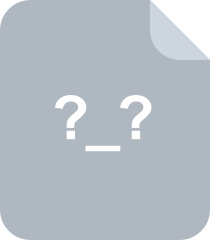
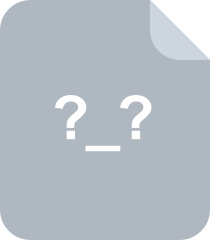
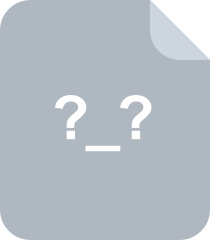
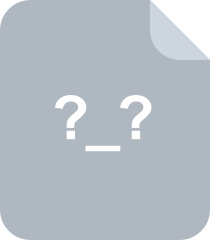
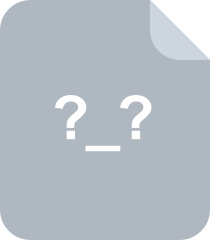
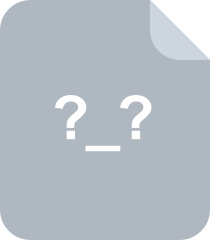
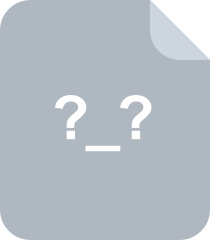
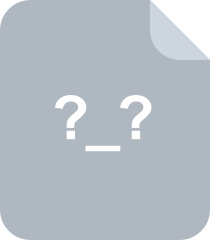
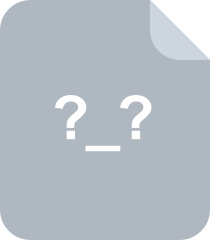
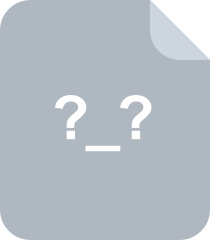
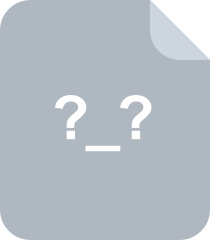
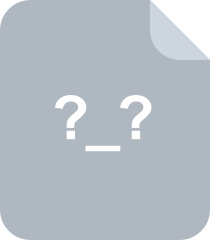
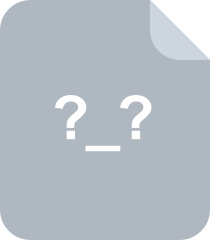
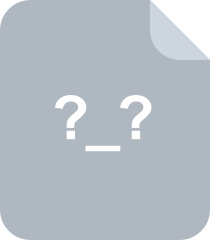
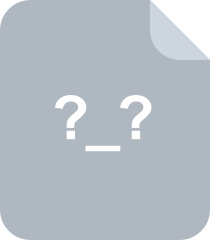
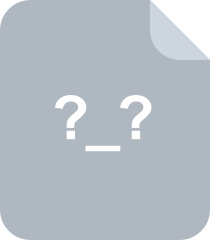
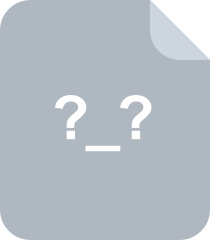
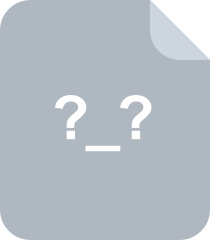
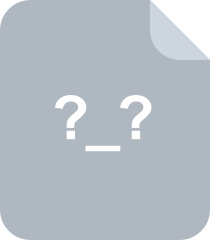
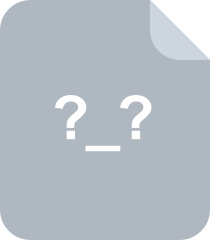
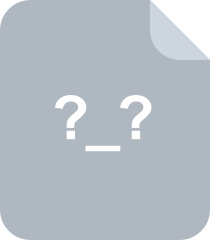
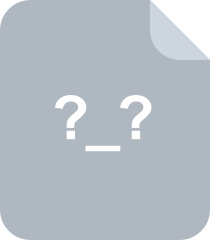
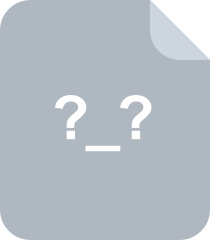
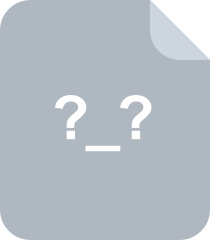
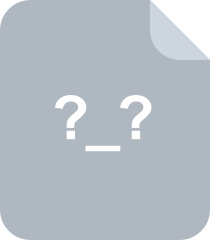
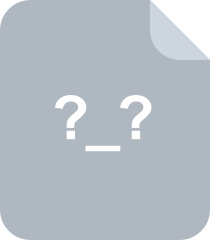
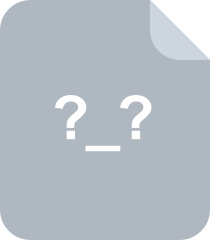
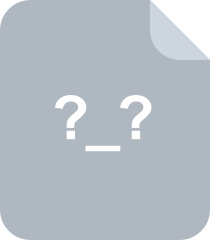
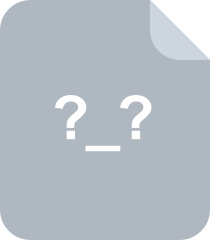
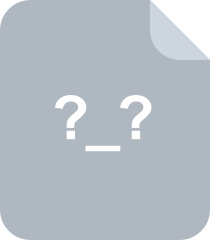
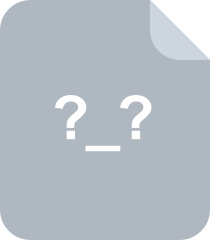
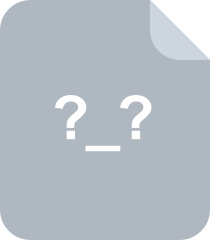
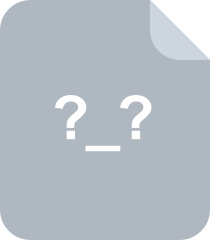
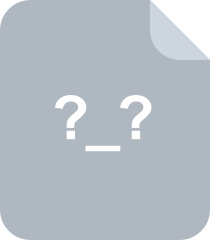
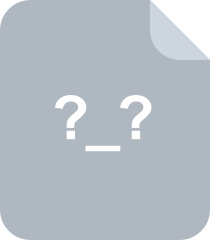
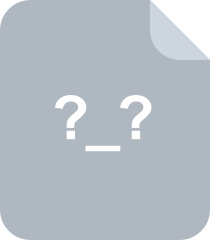
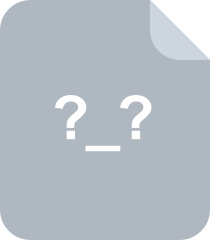
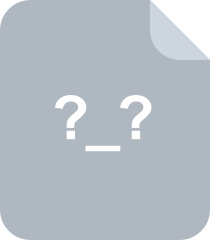
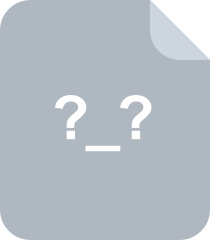
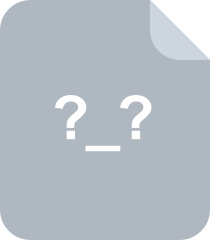
共 749 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
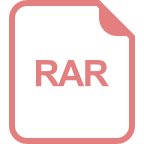
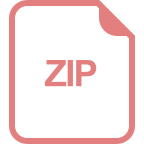
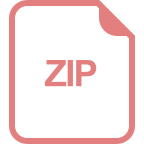
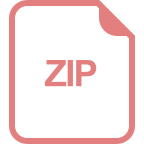
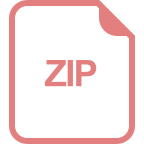
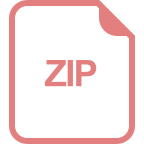
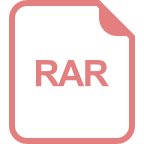
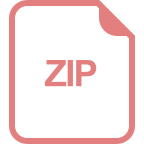
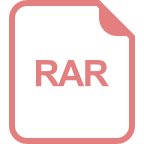
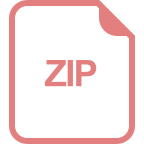
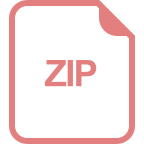
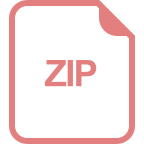
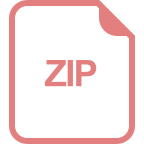
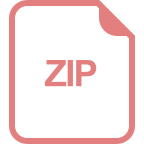
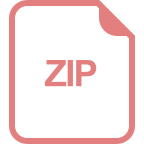
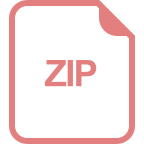
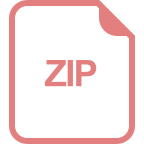
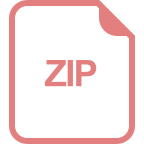
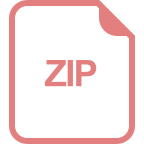
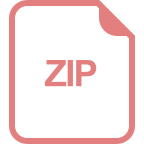
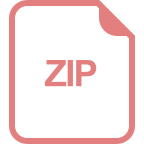
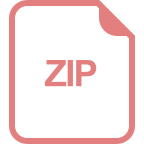
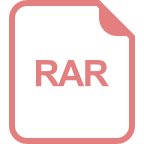
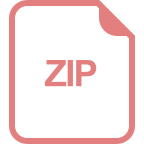
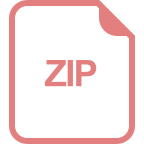
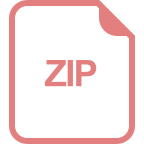

办公模板库素材蛙
- 粉丝: 1674
- 资源: 2299
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

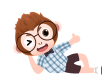
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


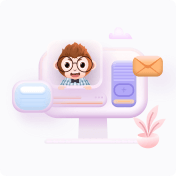
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0