/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.commons.dbcp;
import java.math.BigDecimal;
import java.sql.Array;
import java.sql.Blob;
import java.sql.Clob;
import java.sql.Ref;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.sql.SQLWarning;
import java.sql.Statement;
import java.util.Calendar;
/**
* A dummy {@link ResultSet}, for testing purposes.
*
* @author Rodney Waldhoff
* @author Dirk Verbeeck
* @version $Revision: 479142 $ $Date: 2006-11-25 09:31:27 -0700 (Sat, 25 Nov 2006) $
*/
public class TesterResultSet implements ResultSet {
public TesterResultSet(Statement stmt) {
_statement = stmt;
}
public TesterResultSet(Statement stmt, Object[][] data) {
_statement = stmt;
_data = data;
}
public TesterResultSet(Statement stmt, Object[][] data, int type, int concurrency) {
_statement = stmt;
_data = data;
_type = type;
_concurrency = concurrency;
}
protected int _type = ResultSet.TYPE_FORWARD_ONLY;
protected int _concurrency = ResultSet.CONCUR_READ_ONLY;
protected Object[][] _data = null;
protected int _currentRow = -1;
protected Statement _statement = null;
protected int _rowsLeft = 2;
protected boolean _open = true;
public boolean next() throws SQLException {
checkOpen();
if (_data != null) {
_currentRow++;
return _currentRow < _data.length;
}
else {
if(--_rowsLeft > 0) {
return true;
} else {
return false;
}
}
}
public void close() throws SQLException {
checkOpen();
((TesterStatement)_statement)._resultSet = null;
_open = false;
}
public boolean wasNull() throws SQLException {
checkOpen();
return false;
}
public String getString(int columnIndex) throws SQLException {
checkOpen();
if (columnIndex == -1) {
throw new SQLException("broken connection");
}
if (_data != null) {
return (String) getObject(columnIndex);
}
return "String" + columnIndex;
}
public boolean getBoolean(int columnIndex) throws SQLException {
checkOpen();
return true;
}
public byte getByte(int columnIndex) throws SQLException {
checkOpen();
return (byte)columnIndex;
}
public short getShort(int columnIndex) throws SQLException {
checkOpen();
return (short)columnIndex;
}
public int getInt(int columnIndex) throws SQLException {
checkOpen();
return (short)columnIndex;
}
public long getLong(int columnIndex) throws SQLException {
checkOpen();
return (long)columnIndex;
}
public float getFloat(int columnIndex) throws SQLException {
checkOpen();
return (float)columnIndex;
}
public double getDouble(int columnIndex) throws SQLException {
checkOpen();
return (double)columnIndex;
}
/** @deprecated */
public BigDecimal getBigDecimal(int columnIndex, int scale) throws SQLException {
checkOpen();
return new BigDecimal((double)columnIndex);
}
public byte[] getBytes(int columnIndex) throws SQLException {
checkOpen();
return new byte[] { (byte)columnIndex };
}
public java.sql.Date getDate(int columnIndex) throws SQLException {
checkOpen();
return null;
}
public java.sql.Time getTime(int columnIndex) throws SQLException {
checkOpen();
return null;
}
public java.sql.Timestamp getTimestamp(int columnIndex) throws SQLException {
checkOpen();
return null;
}
public java.io.InputStream getAsciiStream(int columnIndex) throws SQLException {
checkOpen();
return null;
}
/** @deprecated */
public java.io.InputStream getUnicodeStream(int columnIndex) throws SQLException {
checkOpen();
return null;
}
public java.io.InputStream getBinaryStream(int columnIndex) throws SQLException {
checkOpen();
return null;
}
public String getString(String columnName) throws SQLException {
checkOpen();
return columnName;
}
public boolean getBoolean(String columnName) throws SQLException {
checkOpen();
return true;
}
public byte getByte(String columnName) throws SQLException {
checkOpen();
return (byte)(columnName.hashCode());
}
public short getShort(String columnName) throws SQLException {
checkOpen();
return (short)(columnName.hashCode());
}
public int getInt(String columnName) throws SQLException {
checkOpen();
return (columnName.hashCode());
}
public long getLong(String columnName) throws SQLException {
checkOpen();
return (long)(columnName.hashCode());
}
public float getFloat(String columnName) throws SQLException {
checkOpen();
return (float)(columnName.hashCode());
}
public double getDouble(String columnName) throws SQLException {
checkOpen();
return (double)(columnName.hashCode());
}
/** @deprecated */
public BigDecimal getBigDecimal(String columnName, int scale) throws SQLException {
checkOpen();
return new BigDecimal((double)columnName.hashCode());
}
public byte[] getBytes(String columnName) throws SQLException {
checkOpen();
return columnName.getBytes();
}
public java.sql.Date getDate(String columnName) throws SQLException {
checkOpen();
return null;
}
public java.sql.Time getTime(String columnName) throws SQLException {
checkOpen();
return null;
}
public java.sql.Timestamp getTimestamp(String columnName) throws SQLException {
checkOpen();
return null;
}
public java.io.InputStream getAsciiStream(String columnName) throws SQLException {
checkOpen();
return null;
}
/** @deprecated */
public java.io.InputStream getUnicodeStream(String columnName) throws SQLException {
checkOpen();
return null;
}
public java.io.InputStream getBinaryStream(String columnName) throws SQLException {
checkOpen();
return null;
}
public SQLWarning getWarnings() throws SQLException {
checkOpen();
return null;
}
public void clearWarnings() throws SQLException {
checkOpen();
}
public String getCursorName() throws SQLException {
checkOpen();
return null;
}
public ResultSetMetaData getMetaData() throws SQLException {
checkOpen();
return null;
}
public Object getObject(int columnIndex) throws SQLException {
checkOpen();
if (_data != null) {
return _data[_currentRow][columnIndex-1];
}
return new Object();
}
public Object getObject(String columnName) throws SQLException {
checkOpen();
return columnName;
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
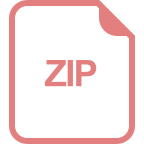
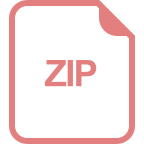
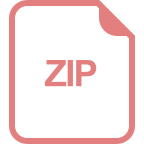
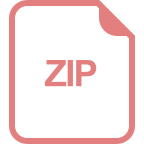
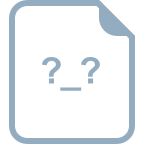
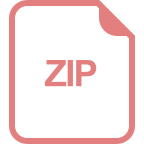
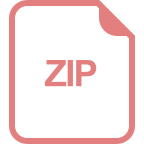
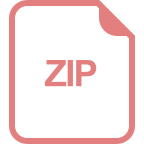
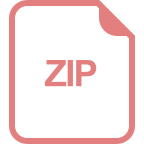
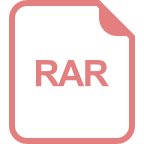
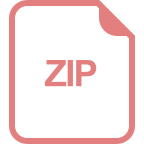
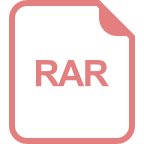
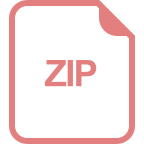
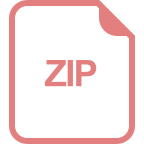
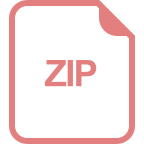
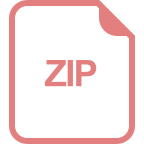
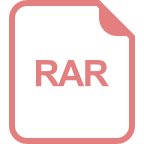
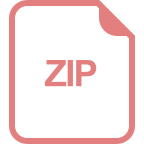
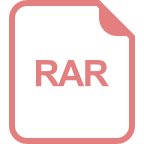
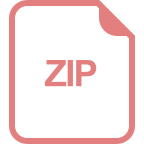
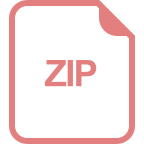
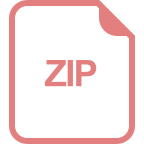
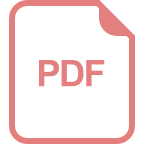
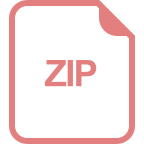
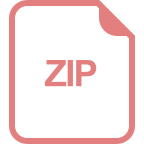
收起资源包目录

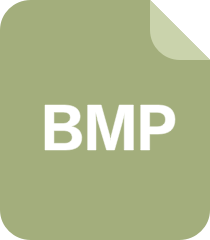
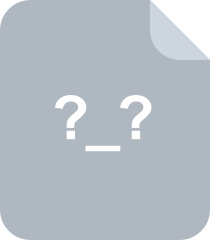
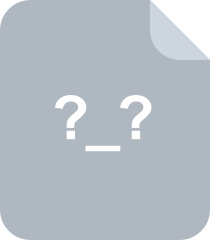
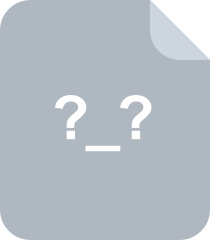
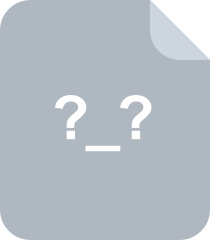
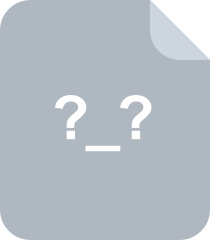
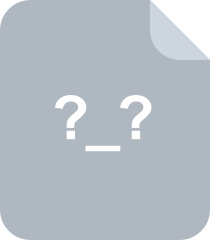
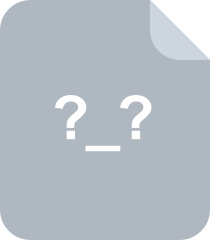
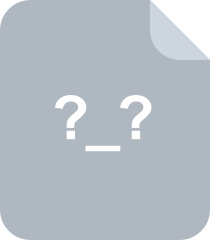
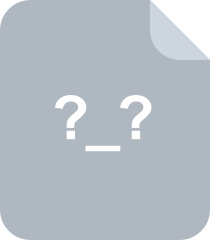
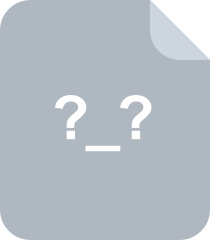
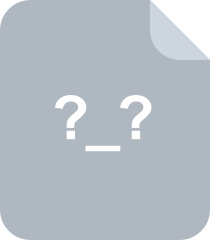
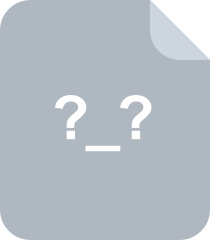
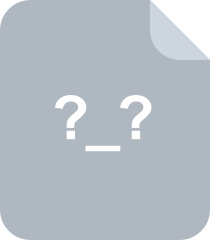
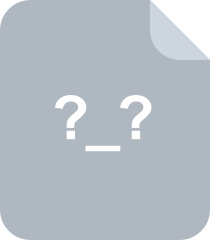
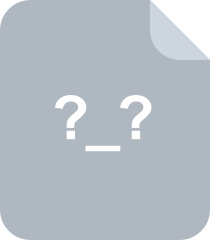
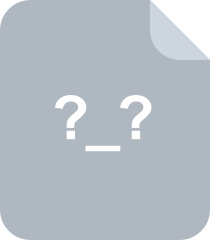
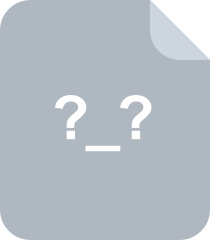
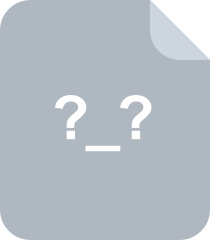
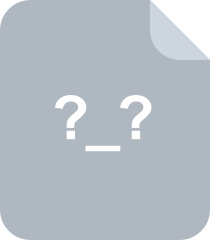
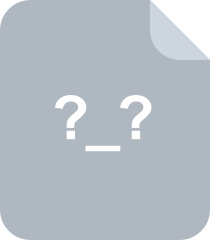
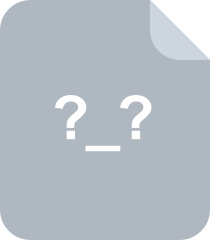
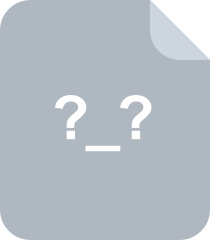
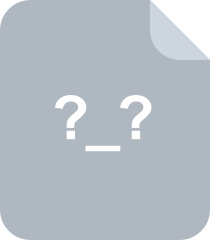
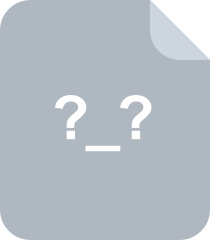
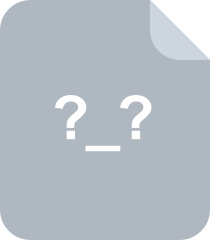
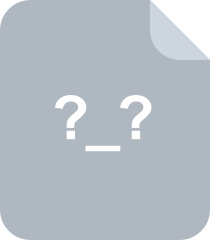
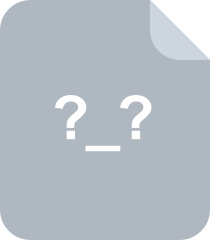
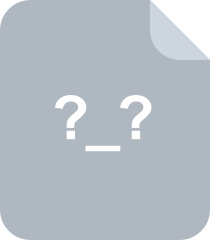
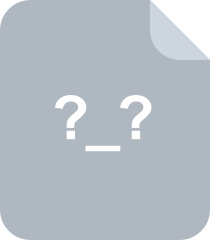
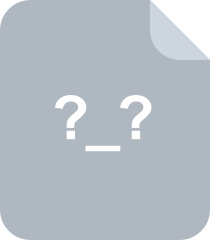
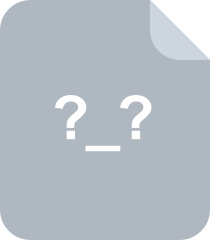
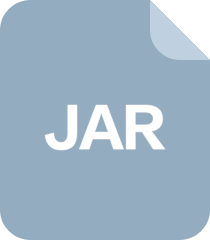
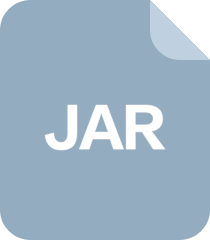
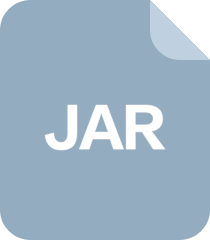
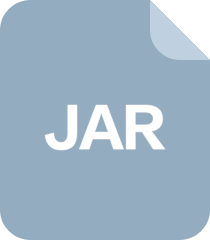
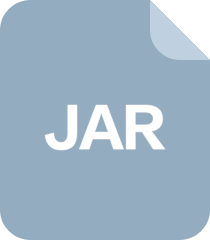
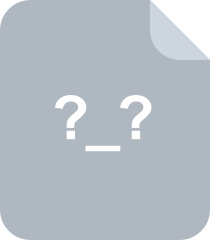
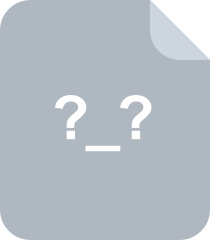
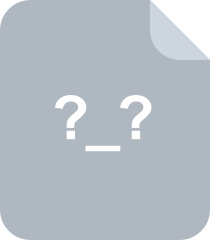
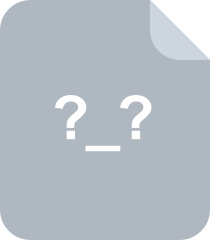
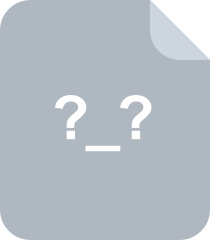
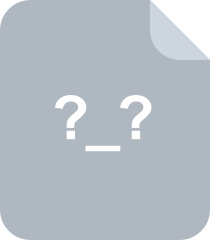
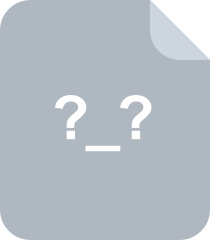
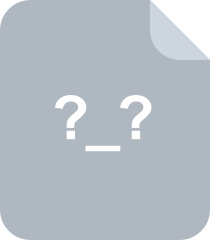
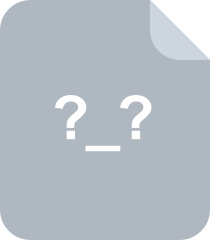
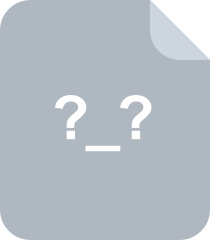
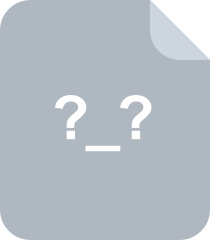
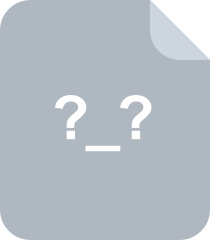
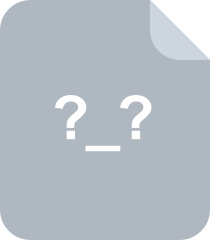
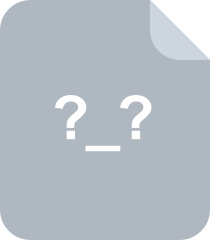
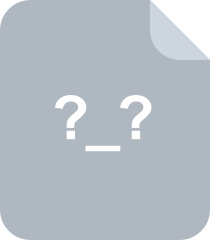
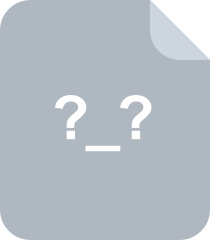
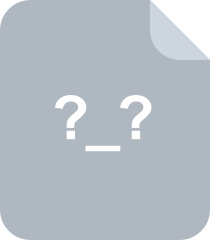
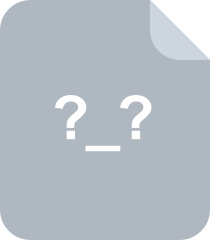
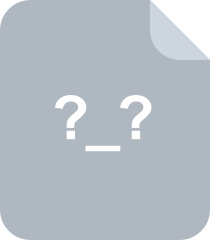
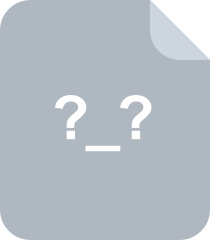
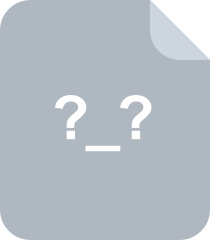
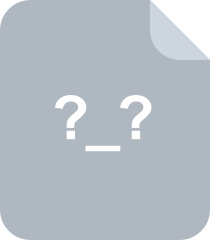
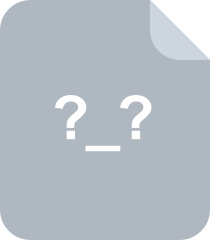
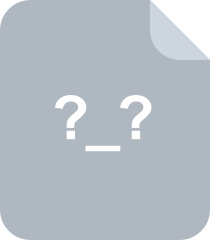
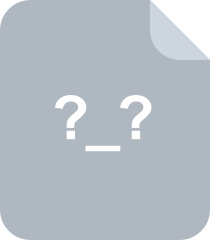
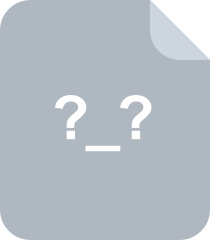
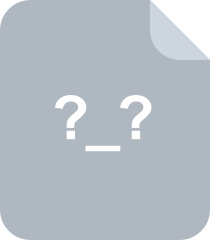
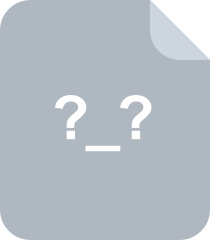
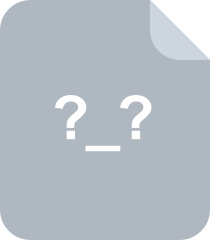
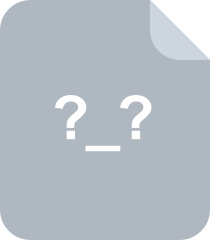
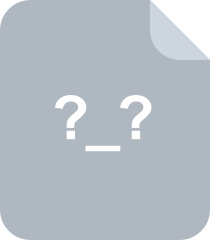
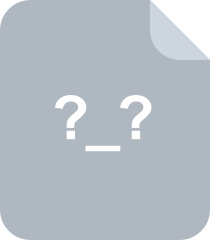
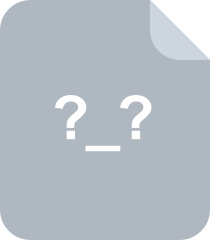
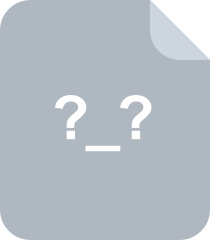
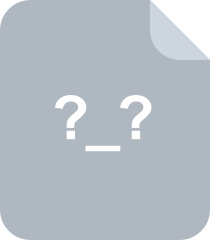
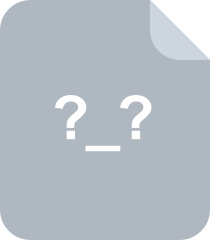
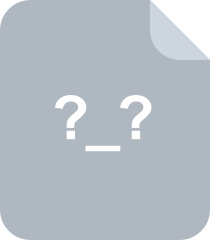
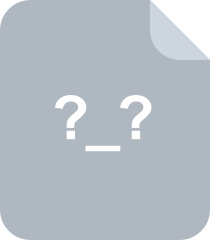
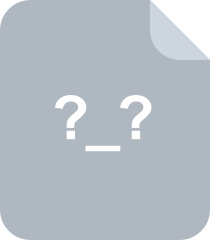
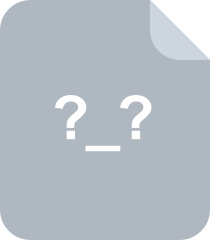
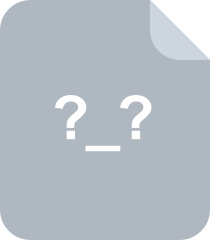
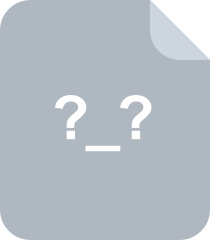
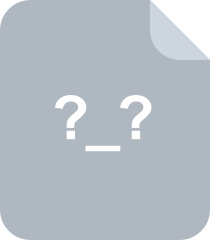
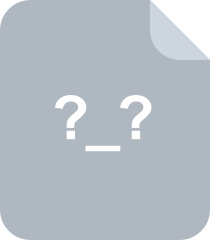
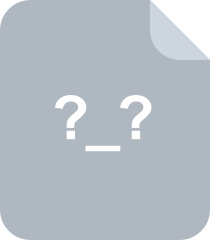
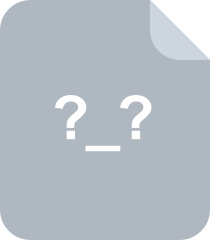
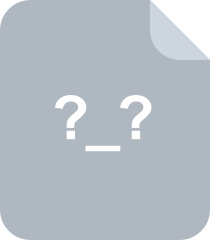
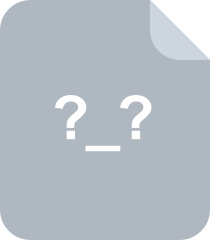
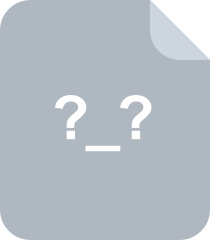
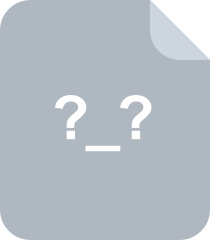
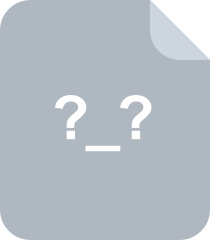
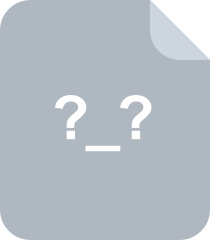
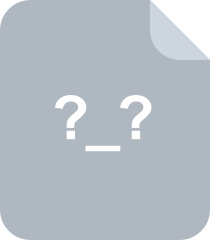
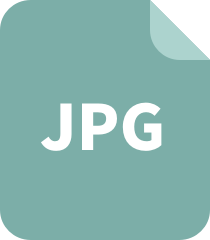
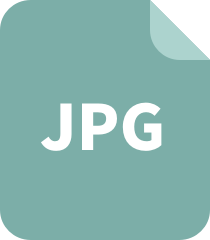
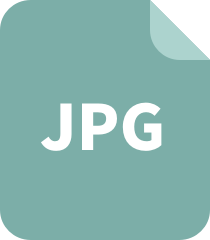
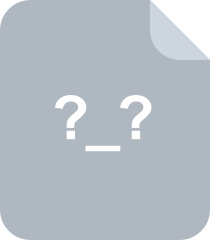
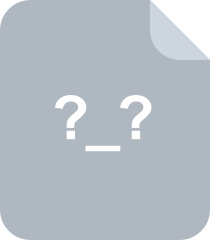
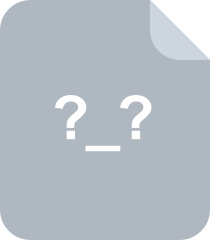
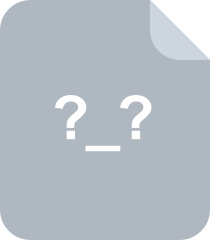
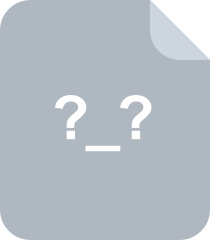
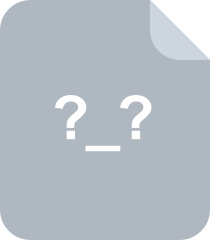
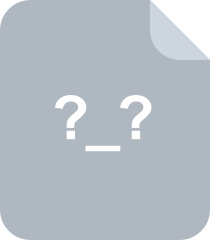
共 129 条
- 1
- 2
资源评论
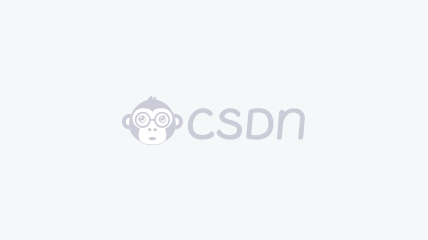
- zhouguoq2013-05-18用来做前端模板,不太好用,没什么可以借鉴之处

fartherway
- 粉丝: 17
- 资源: 271
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

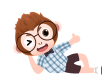
安全验证
文档复制为VIP权益,开通VIP直接复制
