package org.hadoop;
import java.io.IOException;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.SequenceFileOutputFormat;
import org.apache.mahout.math.RandomAccessSparseVector;
import org.apache.mahout.math.Vector;
import org.apache.mahout.math.VectorWritable;
import org.fz.algorithm.hadoop.model.Text2vectorVo;
import util.hadoop.HadoopUtil;
/**
* transform the text to vector
* @author fansy
*
*/
public class Text2VectorDriver {
private final static String SPLIT ="split";
private static Log log=LogFactory.getLog(Text2VectorDriver.class);
public boolean run(Text2vectorVo text2vectorVo) throws IOException, ClassNotFoundException, InterruptedException{
Configuration conf=new Configuration();
conf.set("mapred.job.tracker", HadoopUtil.getJobTracker());
conf.set(SPLIT, text2vectorVo.getSplit());
Job job=new Job(conf,"text2vector with input"+text2vectorVo.getInput());
job.setJarByClass(Text2VectorDriver.class);
job.setOutputFormatClass(SequenceFileOutputFormat.class);
job.setReducerClass(T2VReducer.class);
job.setMapperClass(T2VMapper.class);
job.setMapOutputKeyClass(LongWritable.class);
job.setMapOutputValueClass(VectorWritable.class);
job.setOutputKeyClass(LongWritable.class);
job.setOutputValueClass(VectorWritable.class);
FileInputFormat.addInputPath(job, new Path(text2vectorVo.getInput()));
SequenceFileOutputFormat.setOutputPath(job, new Path(text2vectorVo.getOutput()));
boolean flag=job.waitForCompletion(true);
return flag;
}
public static class T2VMapper extends Mapper<LongWritable,Text,LongWritable,VectorWritable>{
private String split=",";
@Override
public void setup(Context cxt){
if(cxt.getConfiguration().get(SPLIT)!=null){
split=cxt.getConfiguration().get(SPLIT);
}
}
@Override
public void map(LongWritable key,Text line,Context cxt)throws IOException,InterruptedException{
String[] str=line.toString().split(split); // split data
Vector vector=new RandomAccessSparseVector(str.length);
for(int i=0;i<str.length;i++){
double tempVal=-1;
try {
tempVal = Double.parseDouble(str[i]);
} catch (Exception e) {
log.info("Exception when parsing string to double,with error:"+e.getMessage());
return;
}
vector.set(i, tempVal);
}
VectorWritable va=new VectorWritable(vector);
cxt.write(key, va);
}
}
public static class T2VReducer extends Reducer<LongWritable,VectorWritable,LongWritable,VectorWritable>{
public void reduce(LongWritable key,Iterable<VectorWritable> values,Context context)throws IOException,InterruptedException{
for(VectorWritable v:values){
context.write(key, v);
}
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
web 工程调用hadoop集群1.3版本,使用structs2框架,把WEB-INF/lib下面的hadoop-fz1.3.jar拷贝到hadoop集群的lib下面,然后就可以运行了,暂时只支持text2vector算法。具体参考http://blog.csdn.net/fansy1990中相应blog。
资源推荐
资源详情
资源评论
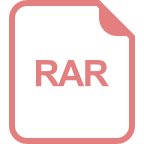
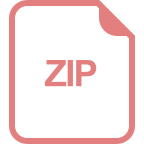
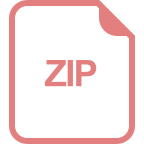
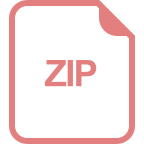
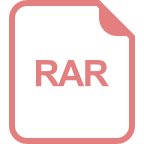
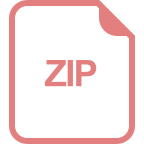
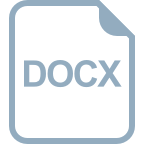
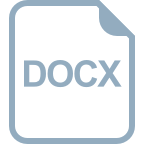
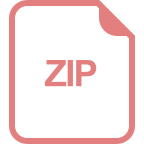
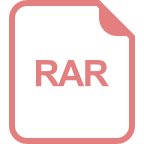
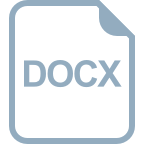
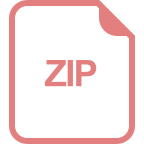
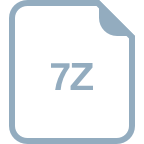
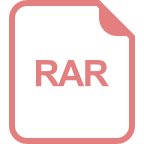
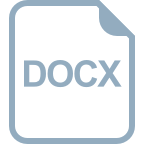
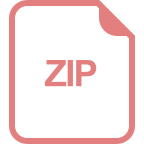
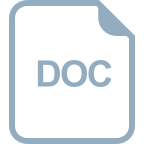
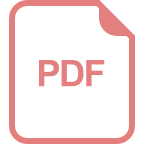
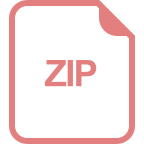
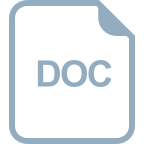
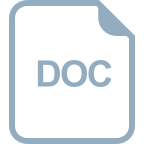
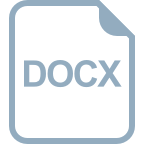
收起资源包目录

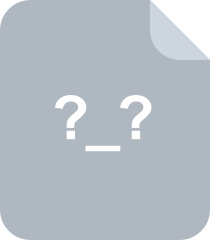
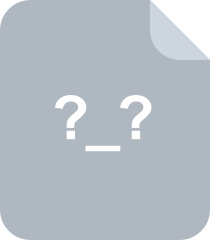
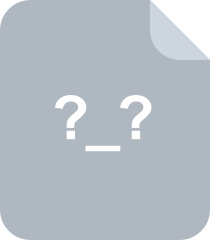
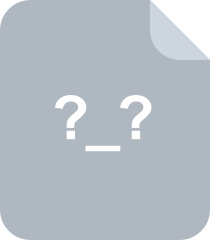
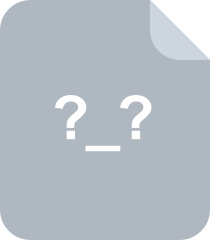
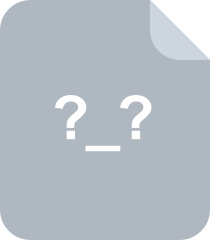
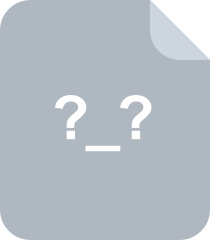
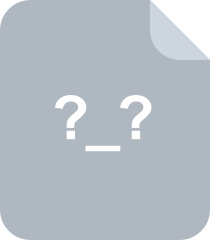
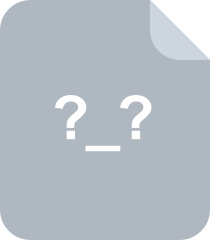
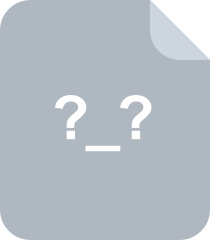
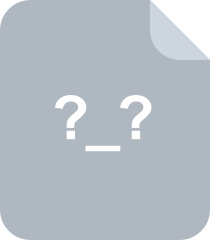
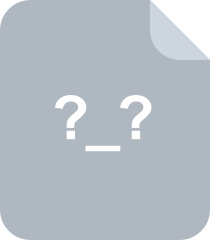
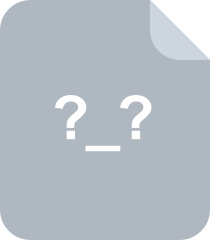
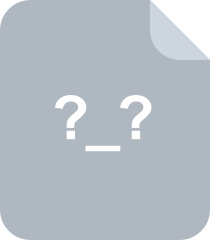
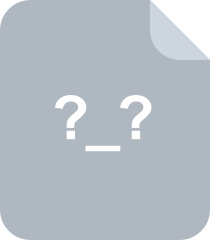
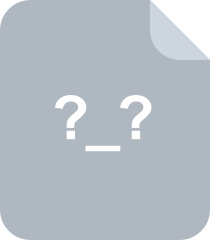
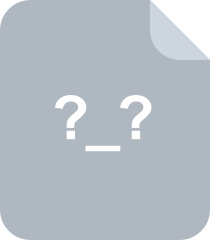
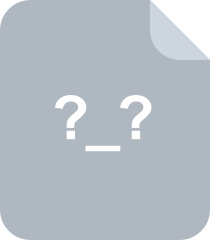
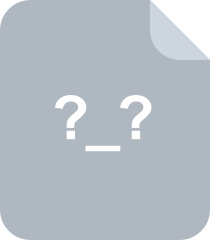
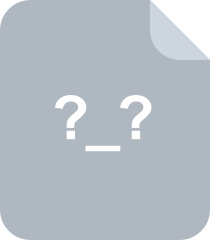
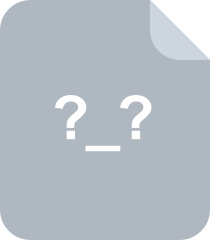
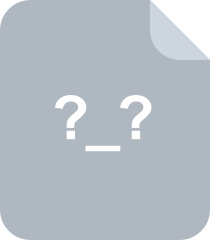
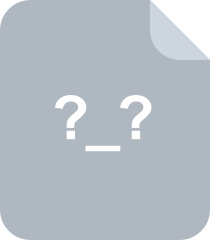
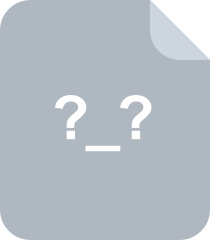
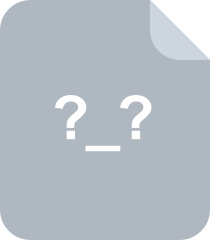
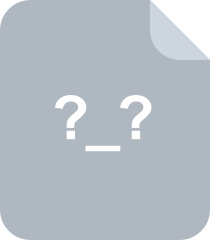
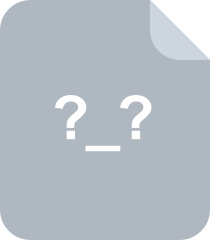
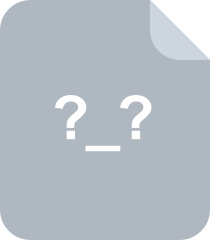
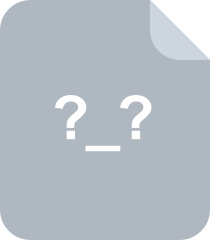
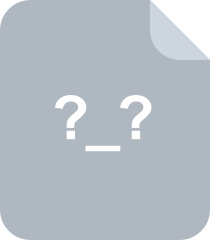
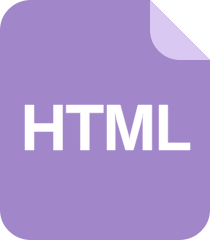
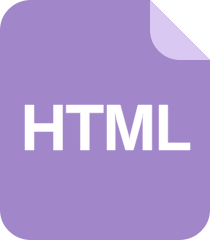
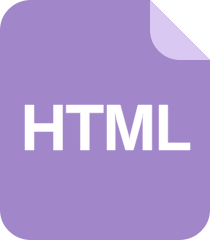
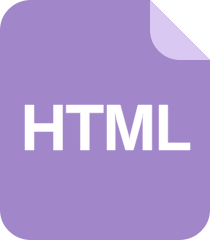
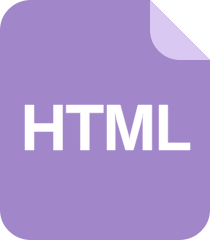
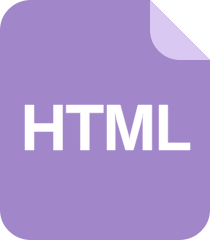
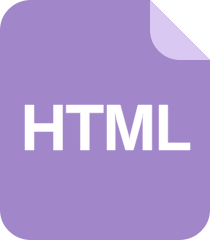
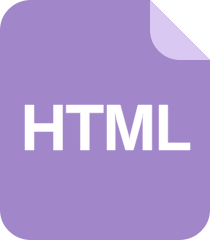
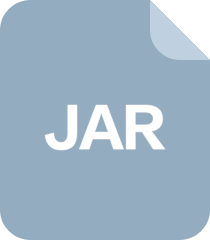
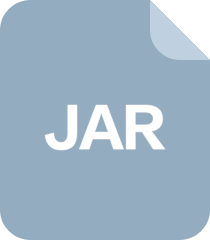
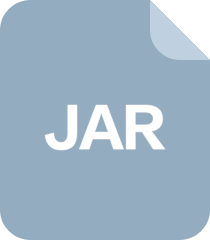
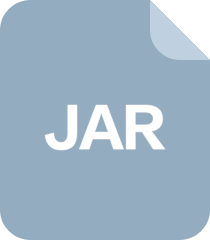
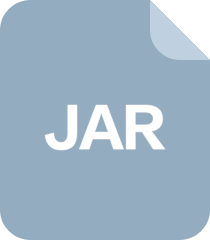
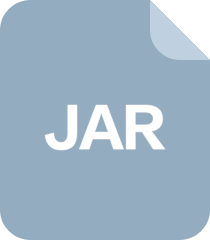
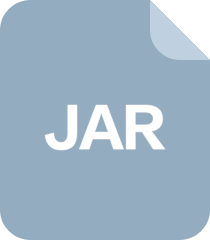
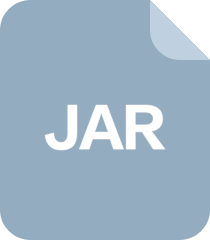
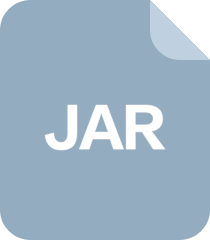
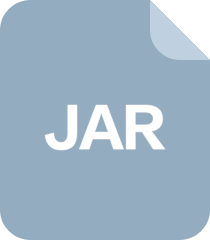
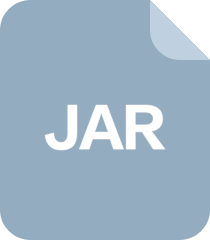
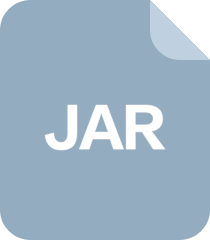
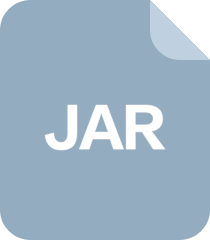
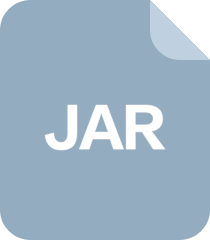
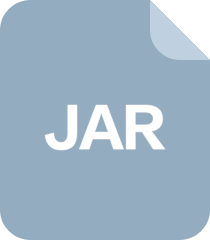
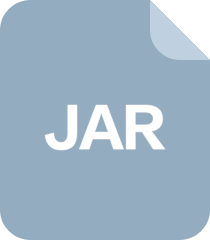
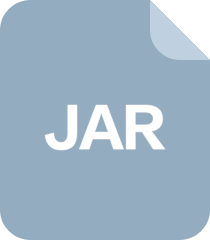
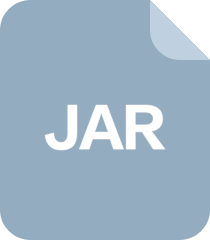
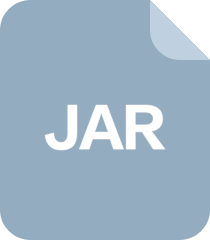
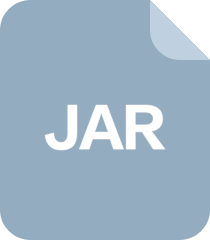
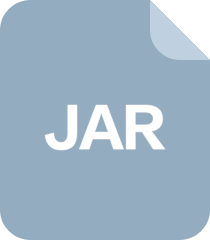
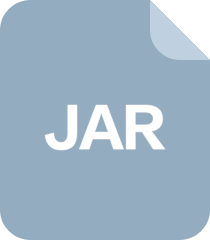
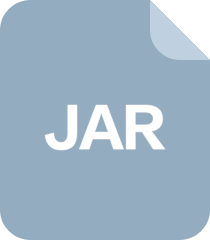
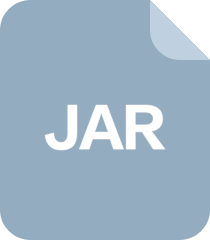
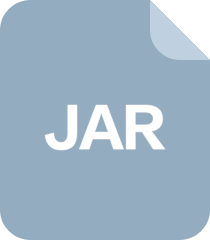
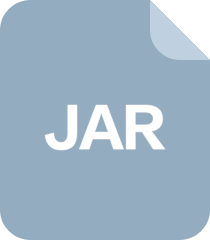
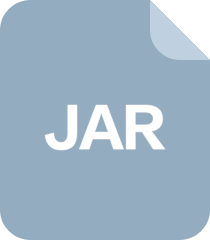
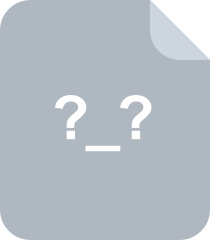
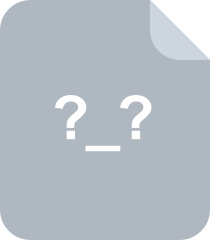
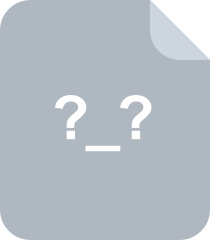
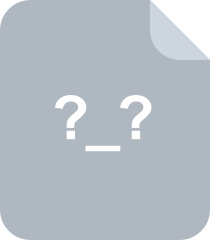
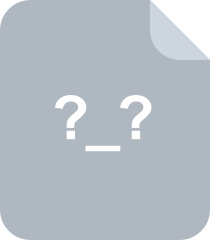
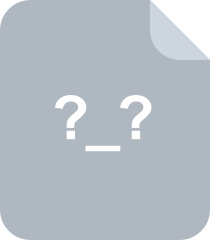
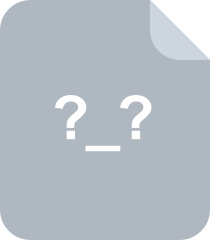
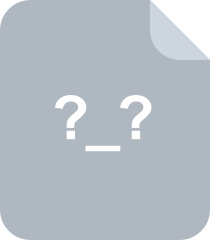
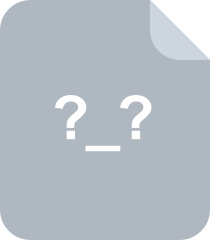
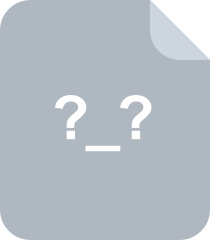
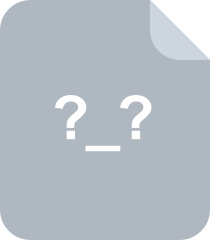
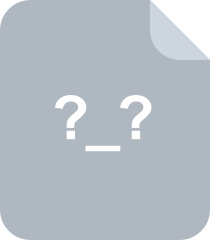
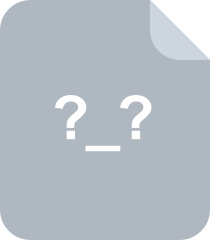
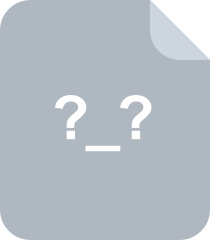
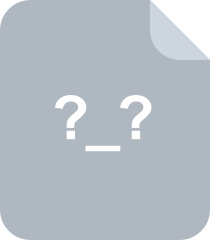
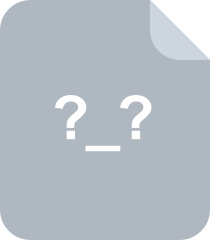
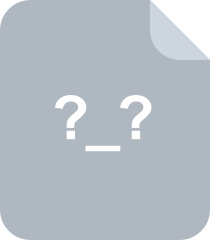
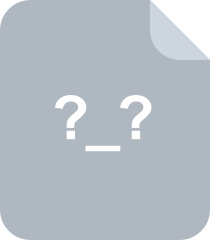
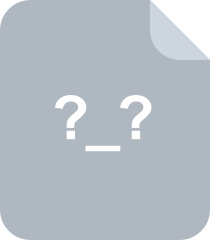
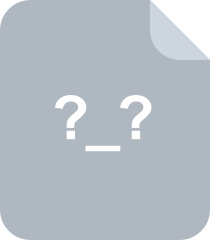
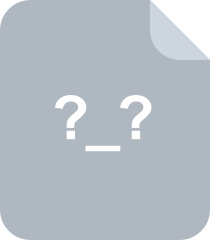
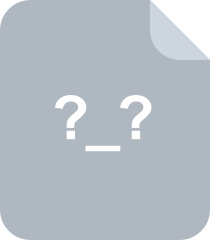
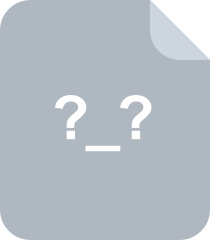
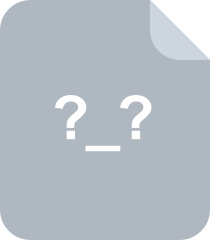
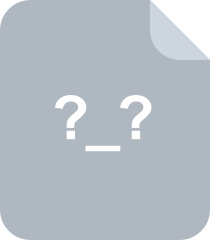
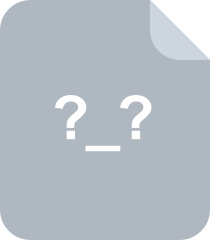
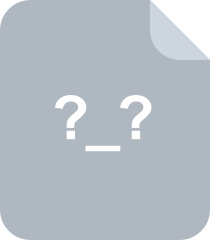
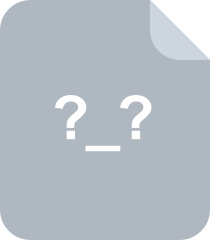
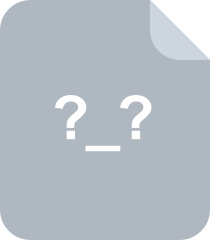
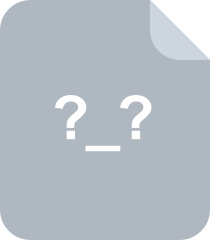
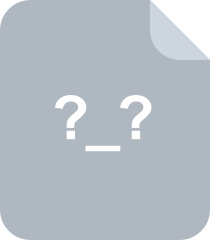
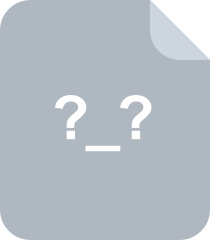
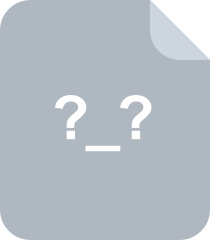
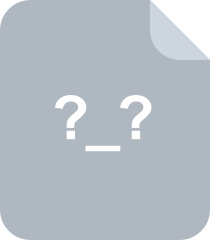
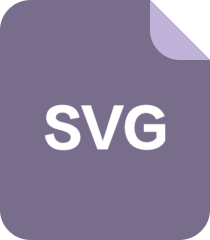
共 106 条
- 1
- 2

fansy1990
- 粉丝: 1664
- 资源: 60
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

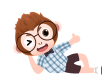
最新资源
- java学籍管理系统源码带本地搭建教程数据库 MySQL源码类型 WebForm
- php+mysql学生成绩查询(系统)
- Bazel 的 Go 规则.zip
- Android ListView下拉刷新 Demo.zip
- Python实现基于一维卷积神经网络(1D-CNN)的多元时间序列分类源码+文档说明
- java基于springBoot课程评价系统源码数据库 MySQL源码类型 WebForm
- 5G工业无线路由器说明书
- SunshineLife个人博客是基于django+mysql+layui的小型个人博客网站
- 5G终端拔号脚本(AT), 适用于MT5701
- 基于Python + Django的生鲜超市系统 调用alipay沙盒系统支付
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


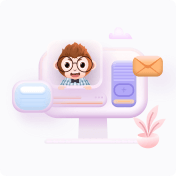
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页