根据提供的文件信息,“C++经典代码大全”似乎是一份汇集了C++编程语言中的经典案例与实用代码片段的资料。由于具体内容部分并没有提供实际的代码示例或详细的知识点介绍,这里将根据标题和描述来生成相关的C++知识点,并尽可能地丰富内容。 ### C++经典代码大全知识点概览 #### 1. 基础语法 - **变量声明与初始化**:在C++中,声明变量时需要指定其类型,例如`int`、`float`等。例如: ```cpp int age = 25; float height = 1.80; ``` - **控制结构**:包括`if...else`语句、`switch`语句、循环(`for`、`while`、`do...while`)等。 - **if...else**语句用于条件判断: ```cpp if (age > 18) { cout << "成年人" << endl; } else { cout << "未成年人" << endl; } ``` - **循环**用于重复执行一段代码直到满足某个条件: ```cpp for (int i = 0; i < 10; i++) { cout << i << " "; } ``` #### 2. 函数与模块化编程 - **函数定义与调用**:函数是C++程序的基本组成单元之一,用于封装一组执行特定任务的语句。 ```cpp void greet(string name) { cout << "Hello, " << name << "!" << endl; } int main() { string name = "Alice"; greet(name); // 调用greet函数 return 0; } ``` - **递归函数**:一种特殊的函数,它会在函数体内部调用自身来解决问题。 ```cpp int factorial(int n) { if (n == 1) { return 1; } else { return n * factorial(n - 1); } } ``` #### 3. 数据结构 - **数组**:数组是一种存储相同类型数据的集合。 ```cpp int numbers[5] = {1, 2, 3, 4, 5}; ``` - **指针**:指针是一种特殊的变量,它存储的是另一个变量的地址。 ```cpp int x = 10; int* ptr = &x; // ptr指向x的地址 cout << *ptr << endl; // 输出10 ``` - **字符串处理**:C++提供了多种方式处理字符串,如标准库中的`std::string`类。 ```cpp string s = "hello world"; s = s.substr(0, 5); // 截取前5个字符 cout << s << endl; // 输出"hello" ``` #### 4. 类与对象 - **类的定义**:类是面向对象编程的基础,用于定义对象的属性和行为。 ```cpp class Person { private: string name; int age; public: Person(string n, int a) : name(n), age(a) {} void sayHello() { cout << "Hello, my name is " << name << ", and I am " << age << " years old." << endl; } }; int main() { Person p("Bob", 30); p.sayHello(); return 0; } ``` - **继承与多态**:继承允许一个类继承另一个类的属性和方法;多态则允许子类重写父类的方法。 ```cpp class Animal { public: virtual void makeSound() { cout << "Some sound" << endl; } }; class Dog : public Animal { public: void makeSound() override { cout << "Woof woof" << endl; } }; int main() { Animal* animal = new Dog(); animal->makeSound(); // 输出"Woof woof" delete animal; return 0; } ``` #### 5. 文件操作与异常处理 - **文件读写**:C++提供了`fstream`库来进行文件的读写操作。 ```cpp #include <fstream> std::ofstream file("example.txt"); file << "Hello, World!"; file.close(); ``` - **异常处理**:异常处理机制用于处理运行时错误。 ```cpp try { int x = 10; int y = 0; if (y == 0) { throw "除数不能为零"; } int result = x / y; cout << "结果:" << result << endl; } catch (const char* msg) { cout << "捕获到异常:" << msg << endl; } ``` 通过上述知识点的介绍,我们可以看出C++作为一种强大的编程语言,在基础语法、函数、数据结构、类与对象等方面都有着非常丰富的特性和应用场景。希望这些知识点能够帮助初学者更好地理解和学习C++。
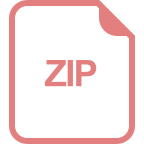
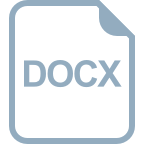
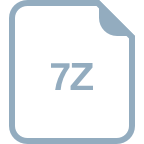
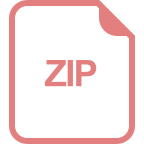
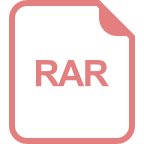
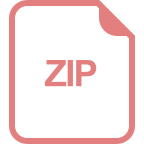
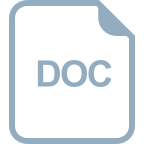
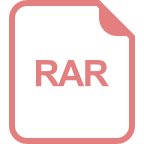
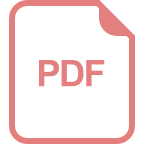
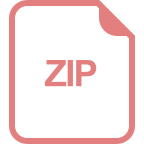
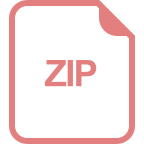
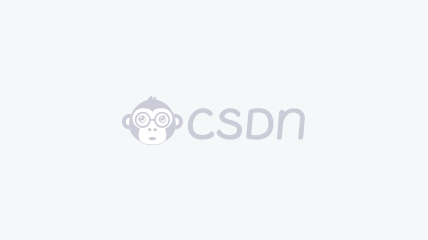
- tzj3498323182013-03-10还可以,可以学到不少

- 粉丝: 0
- 资源: 21
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

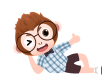
最新资源
- 智慧校园管理系统快速导入配置类工具类实体类(1)config&util&pojo.zip
- 智慧校园管理系统快速导入配置类与工具类 config&util.zip
- JrebelBrainsLicenseServerforJava-1.0-SNAPSHOT-jar-with-dependenc
- IMG_1641.PNG
- guanyumayasmoothweights
- 控制流平坦化还原示例代码,采用ast技术
- jdk - 21.0.4 - windows
- jdk21 - 21.0.4 - macos
- jdk21 - 21.0.4 - linux
- ffmpeg-6.1.2-full-build-with-mingw32

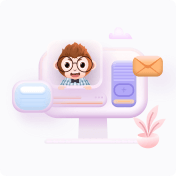
