Purpose
--------------
GLView is a collection of classes designed to make it as easy as possible to get up and running with OpenGL functionality within an iOS app.
The GLImage and GLImageView classes make it possible to load and display PVR formatted images and video clips in your app without needing to know any OpenGL whatsoever. See more about PVR images and video below.
The GLModel and GLModelView classes allow you to load a 3D model using the popular WaveFront .obj format and display it in a view, again without needing to know anything about OpenGL.
The GLView library is modular. If you don't want to render 3D models you can omit the Models classes and the rest of the library will still work. If you aren't interested in loading and displaying images and just want a basic OpenGL context set up for you, you can omit the Images and Models classes.
Supported OS & SDK Versions
-----------------------------
* Supported build target - iOS 6.0 / Mac OS 10.8 (Xcode 4.5.2, Apple LLVM compiler 4.1)
* Earliest supported deployment target - iOS 5.0 / Mac OS 10.7
* Earliest compatible deployment target - iOS 4.3 / Mac OS 10.6
NOTE: 'Supported' means that the library has been tested with this version. 'Compatible' means that the library should work on this iOS version (i.e. it doesn't rely on any unavailable SDK features) but is no longer being tested for compatibility and may require tweaking or bug fixes to run correctly.
ARC Compatibility
------------------
As of version 1.5, GLView requires ARC. If you wish to use GLView in a non-ARC project, just add the -fobjc-arc compiler flag to all of the GLView class files. To do this, go to the Build Phases tab in your target settings, open the Compile Sources group, double-click each of the GLView-related .m files in the list and type -fobjc-arc into the popover.
If you wish to convert your whole project to ARC, comment out the #error line in GLUtils.m, then run the Edit > Refactor > Convert to Objective-C ARC... tool in Xcode and make sure all files that you wish to use ARC for (including all of the GLView files) are checked.
Installation
---------------
To use GLView, just drag the class files into your project and add the QuartzCore and OpenGLES frameworks. If you are using the optional GZIP library then you will also need to add the libz.dylib.
Classes
---------------
The GLView library currently includes the following classes:
- GLUtils - this is a collection of useful class extensions and global methods that are used by the GLView library.
- GLView - this is a general-purpose UIView subclass for displaying OpenGL graphics on screen. It doesn't display anything by default, but if you are familiar with OpenGL you can use it for raw OpenGL drawing.
- GLImage - this is a class for loading image files as OpenGL textures. It supports all the same image formats as UIImage, as well as a number of PVR image formats.
- GLImageMap - this is a class for loading image maps, also known as image atlases or spritemaps.
- GLImageView - this is a subclass of GLView, specifically designed for displaying GLImages. It behaves in a similar way to UIImageView and mostly mirrors its interface.
- GLModel - this is a class for loading 3D mesh geometry for rendering in a GLView. It currently supports only Wavefront .obj files and the bespoke "WWDC2010" model format used in the Apple GLEssentials sample code, but will support additional formats in future.
- GLModelView - this is a subclass of GLView, specifically designed for displaying GLModels as simply and easily as possible.
- GLLight - this class represents a light source and is used for illuminating GLModel objects in the GLModelView.
Global methods
------------------
void CGRectGetGLCoords(CGRect rect, GLfloat *coords);
This method is used by the GLView library for converting GLRects into OpenGL vertices. It receives a pointer to an array of 8 GLfloats, and it populates the array with the coordinates for the 4 corners of the rect.
UIColor extensions
---------------------
These methods extend UIColor to make it easier to integrate with OpenGL code.
- (void)getGLComponents:(GLfloat *)rgba;
Pass in a pointer to a C array of four GLfloats and this method will populate them with the red, green, blue and alpha components of the UIColor. Works with monochromatic and RGB UIColors, but will fail if the color contains a pattern image, or some other unsupported color format.
- (void)bindGLClearColor;
Binds the UIColor as the current OpenGL clear color.
- (void)bindGLBlendColor;
Binds the UIColor as the current OpenGL blend color.
- (void)bindGLColor;
Binds the UIColor as the current OpenGL color.
GLView properties
----------------
@property (nonatomic, assign) CGFloat fov;
This is the vertical field of view, in radians. This defaults to zero, which gives an orthographic projection (no perspective) but set it to around Pi/2 (90 degrees) for a perspective projection.
@property (nonatomic, assign) CGFloat near;
The near clipping plane. This is used by OpenGL to clip geometry that is too close to the camera. In orthographic projection mode, this value can be negative, but in perspective mode the value must be greater than zero.
@property (nonatomic, assign) CGFloat far;
The far clipping plane. This is used by OpenGL to clip geometry that is too far from the camera. A smaller value for the far plane improves the precision of the z-buffer and reduces rendering artefacts, so try to set this value as low as possible (Note: it must be larger than the near value).
@property (nonatomic, assign) NSTimeInterval frameInterval;
This property controls the frame rate of the view animation. The default value is 1.0/60.0 (60 frames per second). You may wish to reduce this to 30 fps if your animation becomes choppy.
@property (nonatomic, assign) NSTimeInterval elapsedTime;
This property is used when animating the view. It indicates the total animation time elapsed since the startAnimating method was first called. It is not reset when stop is called, but you can reset it manually at any time as it is a read/write property.
GLView methods
----------------
GLView has the following methods:
- (void)setUp
Called by `initWithCoder: and `initWithFrame:` to initialise the view. Override this method to do setup code in your own GLView subclasses. Remember to call [super setUp] or the view won't work properly.
- (void)display;
If you are managing drawing yourself using an update loop, you can call this method to call the drawRect: method immediately instead of waiting for the next OS frame update.
- (void)drawRect:(CGRect)rect;
This method (inherited from UIView) is responsible for drawing the view contents. The default implementation does nothing, but you can override this method in a GLView subclass to perform your own OpenGL rendering logic.
- (void)bindFramebuffer;
This method is used to bind the GLView before attempting any drawing using OpenGL commands. This method is called automatically prior to the `drawRect:` method, so in most cases you won't ever need to call it yourself.
- (BOOL)presentRenderbuffer;
Call this method to update the view and display the result of any previous drawing commands. This method is called automatically after the `drawRect:` method, so in most cases you won't ever need to call it yourself.
- (void)startAnimating;
Begin animating the view. This will cause the `step:` method to be called repeatedly every 60th second, allowing you to do frame-by-frame animation of your OpenGL geometry.
- (void)stopAnimating;
Pauses the animation. The elapsedTime property is not automatically reset so calling `startAnimating` again will resume the animation from the point at which it stopped.
- (BOOL)isAnimating;
Returns YES if the view is currently animating and NO if it isn't.
- (void)step:(NSTimeInterval)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
GLView封装了很多OpenGL的功能,能够让开发者很方便地在iOS编程中使用OpenGL来处理图像、三维模型以及视频。 其中GLImage 和 GLImageView能够方便地加载和显示PVR格式的图像或者视频剪辑。 而GLModel 和 GLModelView能够让开发者加载.obj格式的三维模型。 这两个模块是独立的,如果你仅仅想处理图片或者视频,那么就不需要GLModel,反之则反。 注:GLView支持ARC和non-ARC,
资源推荐
资源详情
资源评论
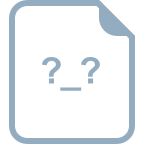
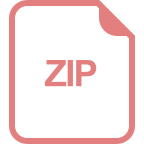
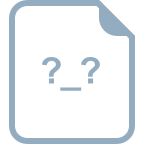
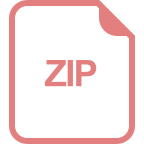
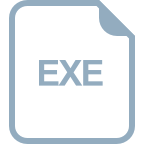
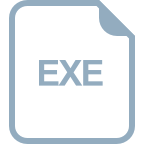
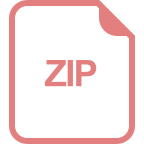
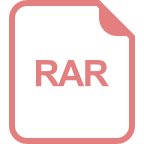
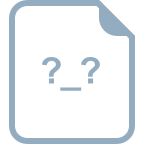
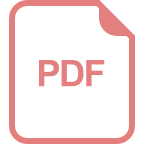
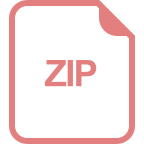
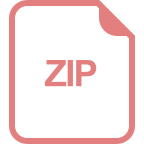
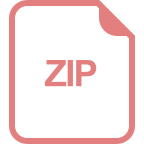
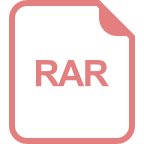
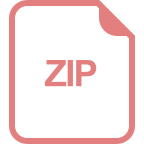
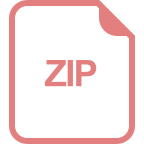
收起资源包目录





































































































共 729 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
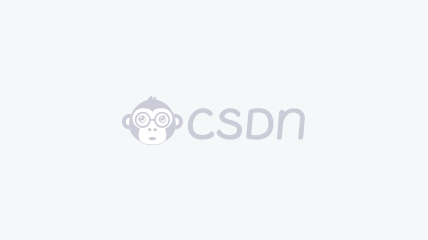
- 9彩虹糖12013-04-29不知道该怎么用啊,要是有说明就好啦
- shihliangou2013-03-27很实用, 帮助极大
- play_more2014-04-04很有用,不过还是要对IOS架构比较熟悉就快能上手的代码

ernest201210
- 粉丝: 49
- 资源: 43
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

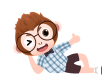
安全验证
文档复制为VIP权益,开通VIP直接复制
