/*
* LoginOn.java
*
* Created on __DATE__, __TIME__
*/
package LoginOn;
import java.awt.Dimension;
import java.awt.Toolkit;
import java.util.List;
import java.util.Locale;
import javax.swing.JLabel;
import javax.swing.ListSelectionModel;
import javax.swing.table.DefaultTableCellRenderer;
import javax.swing.table.DefaultTableModel;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import dao.BLLService;
import entity.Users;
/**
*
* @author __USER__
*/
public class LoginOn extends javax.swing.JFrame
{
/**
*
*/
private static final long serialVersionUID = 1L;
BLLService b = null;
Locale loc;
String loginOntitle;
String titInfo;
String colIdName;
String colUseName;
String colPwName;
String btnDel;
String btnAdd;
String labAdd;
String labUserName;
String labPassWord;
String labPassWord2;
String checkInfo1;
String checkInfo2;
String checkInfo3;
String checkInfo5;
/** Creates new form LoginOn */
public LoginOn()
{
}
public LoginOn(BLLService bll, Locale l)
{
this.b = bll;
this.loc = l;
this.i18n();
initComponents();
Toolkit theKit = this.getToolkit();
Dimension wndSize = theKit.getScreenSize();
this.setBounds(wndSize.width / 2 - this.getWidth() / 2, wndSize.height
/ 2 - this.getHeight() / 2, this.getWidth(), this.getHeight());
this.jTable1.getSelectionModel().setSelectionMode(
ListSelectionModel.SINGLE_SELECTION);
loadData();
}
private void loadData()
{
List<Users> liUsers = b.findAllUsers();
int count = liUsers.size();
DefaultTableModel dtm = (DefaultTableModel) this.jTable1.getModel();
int rowCount = dtm.getRowCount();
if (rowCount != 0)
{
for (int i = 0; i < rowCount; i++)
{
dtm.removeRow(0);
}
}
for (int i = 0; i < count; i++)
{
Object vRow[] = new Object[3];
vRow[0] = ((Users) liUsers.get(i)).getId();
vRow[1] = ((Users) liUsers.get(i)).getUserName();
vRow[2] = ((Users) liUsers.get(i)).getPassWord();
dtm.addRow(vRow);
}
DefaultTableCellRenderer r = new DefaultTableCellRenderer();
r.setHorizontalAlignment(JLabel.CENTER);
this.jTable1.setDefaultRenderer(Object.class, r);
}
private void i18n()
{
ApplicationContext ctx = new ClassPathXmlApplicationContext(
"messages.xml");
loginOntitle = ctx.getMessage("loginOntitle", null, loc);
titInfo = ctx.getMessage("titInfo", null, loc);
colIdName = ctx.getMessage("colIdName", null, loc);
colUseName = ctx.getMessage("colUseName", null, loc);
colPwName = ctx.getMessage("colPwName", null, loc);
btnDel = ctx.getMessage("btnDel", null, loc);
btnAdd = ctx.getMessage("btnAdd", null, loc);
labAdd = ctx.getMessage("labAdd", null, loc);
labUserName = ctx.getMessage("labUserName", null, loc);
labPassWord = ctx.getMessage("labPassWord", null, loc);
labPassWord2 = ctx.getMessage("labPassWord2", null, loc);
checkInfo1 = ctx.getMessage("checkInfo1", null, loc);
checkInfo2 = ctx.getMessage("checkInfo2", null, loc);
checkInfo3 = ctx.getMessage("checkInfo3", null, loc);
checkInfo5 = ctx.getMessage("checkInfo5", null, loc);
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
// GEN-BEGIN:initComponents
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents()
{
jScrollPane1 = new javax.swing.JScrollPane();
jTable1 = new javax.swing.JTable();
label1 = new java.awt.Label();
label2 = new java.awt.Label();
label3 = new java.awt.Label();
label4 = new java.awt.Label();
textField1 = new java.awt.TextField();
textField2 = new java.awt.TextField();
textField3 = new java.awt.TextField();
button1 = new java.awt.Button();
label5 = new java.awt.Label();
label6 = new java.awt.Label();
label7 = new java.awt.Label();
label8 = new java.awt.Label();
button2 = new java.awt.Button();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
setTitle(loginOntitle);
jTable1.setModel(new javax.swing.table.DefaultTableModel(
new Object[][] {}, new String[] { colIdName, colUseName,
colPwName }) {
/**
*
*/
private static final long serialVersionUID = 1L;
@SuppressWarnings("unchecked")
Class[] types = new Class[] { java.lang.String.class,
java.lang.String.class, java.lang.String.class };
boolean[] canEdit = new boolean[] { false, false, false };
@SuppressWarnings("unchecked")
public Class getColumnClass(int columnIndex)
{
return types[columnIndex];
}
public boolean isCellEditable(int rowIndex, int columnIndex)
{
return canEdit[columnIndex];
}
});
jScrollPane1.setViewportView(jTable1);
label1.setFont(new java.awt.Font("宋体", 1, 14));
label1.setText(labAdd);
label2.setText(labUserName);
label3.setText(labPassWord);
label4.setText(labPassWord2);
textField2.setEchoChar('*');
textField3.setEchoChar('*');
button1.setLabel(btnAdd);
button1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt)
{
button1ActionPerformed(evt);
}
});
label8.setText(titInfo);
button2.setLabel(btnDel);
button2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt)
{
button2ActionPerformed(evt);
}
});
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(
getContentPane());
getContentPane().setLayout(layout);
layout
.setHorizontalGroup(layout
.createParallelGroup(
javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(
layout
.createSequentialGroup()
.addGap(97, 97, 97)
.addGroup(
layout
.createParallelGroup(
javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(
layout
.createSequentialGroup()
.addComponent(
label1,
javax.swing.GroupLayout.PREFERRED_SIZE,
124,
javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(
237,
237,
237)
.addComponent(
button2,
javax.swing.GroupLayout.PREFERRED_SIZE,
javax.swing.GroupLayout.DEFAULT_SIZE,
javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(
93,
93,
93))
.addGroup(
layout
.createSequentialGroup()
.addGroup(
layout
.createParallelGroup(
javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(
javax.swing.GroupLayout.Alignment.LEADING,
layout
.createSequentialGroup()
.addGroup(
layout
.createParallelGroup(
javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(
label2,
javax.swing.GroupLayout.PREFERRED_SIZE,
javax.swing.GroupLayout.DEFAULT_SIZE,
javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
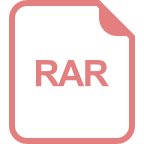
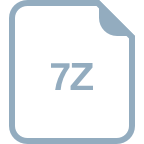
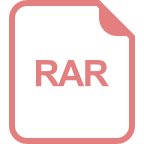
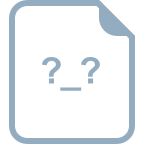
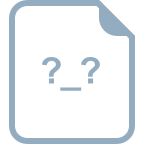
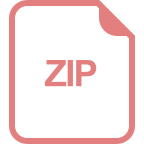
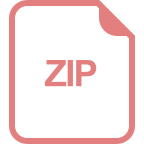
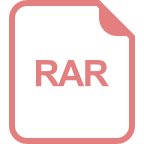
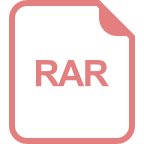
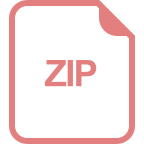
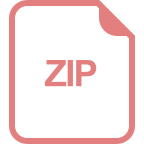
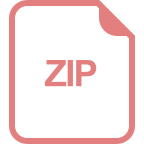
收起资源包目录


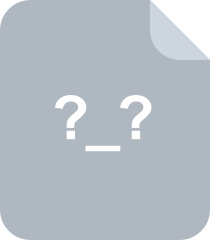




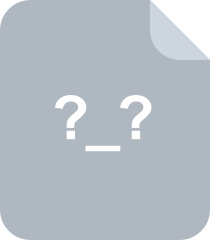

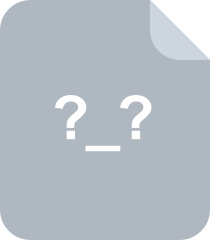
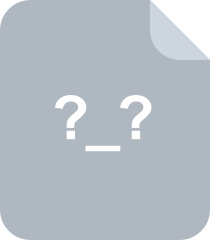
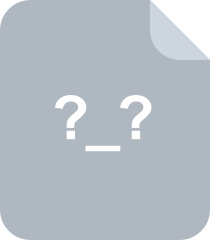
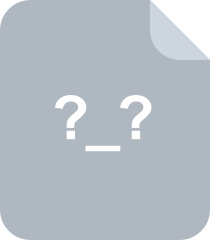
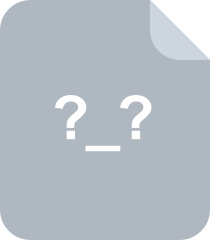
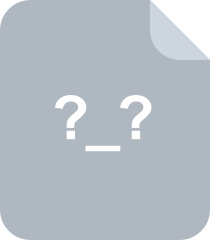
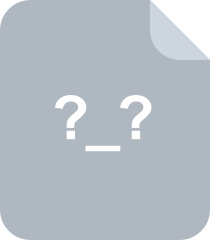

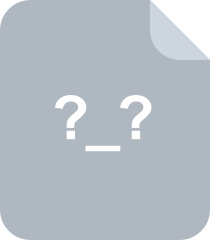

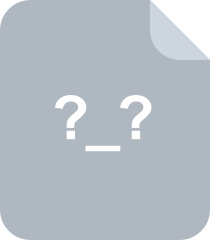

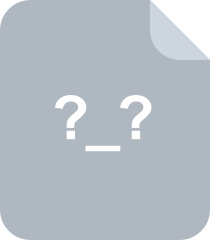
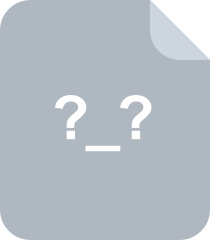
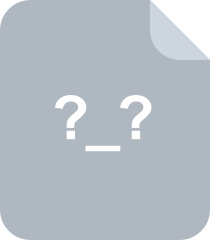
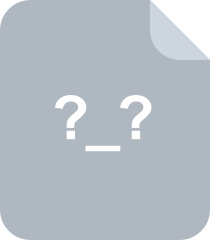

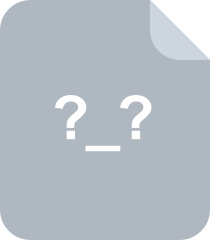
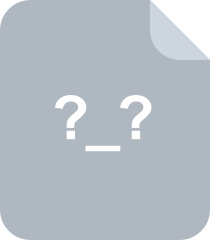
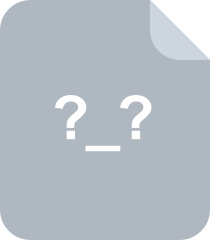
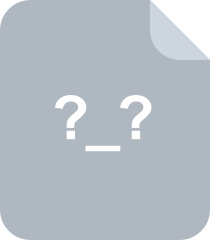
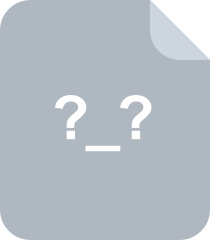
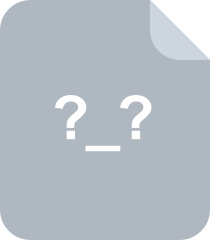
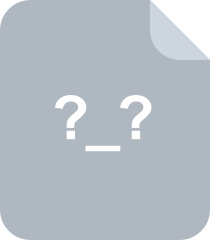

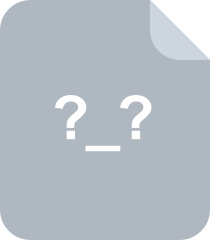
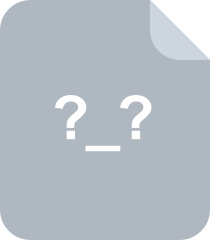
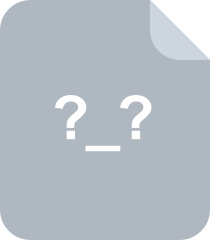
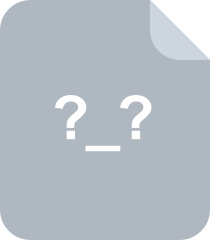
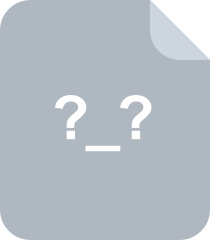
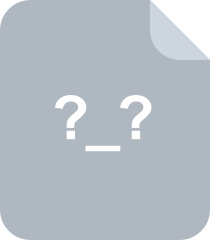
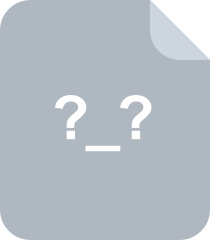
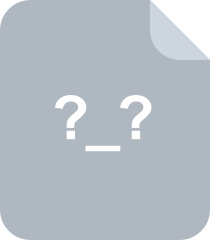
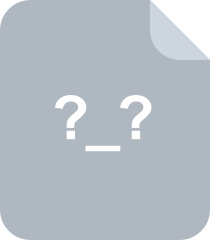
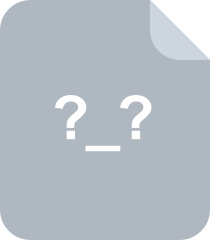
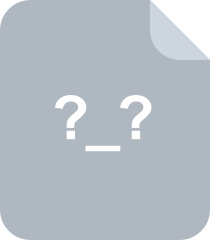

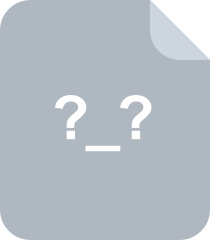
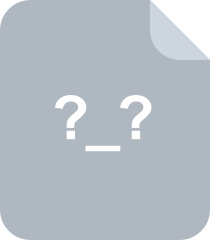
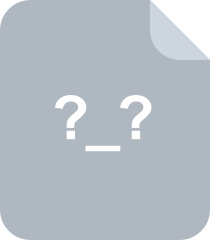

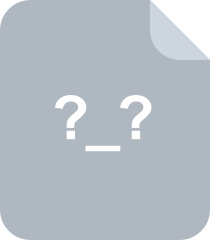

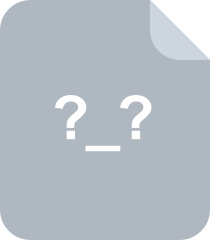

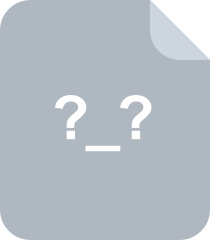
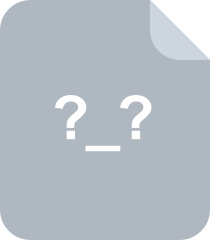

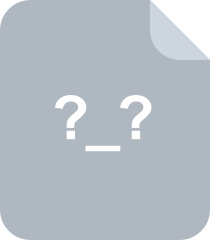
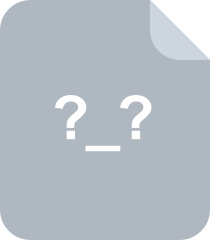
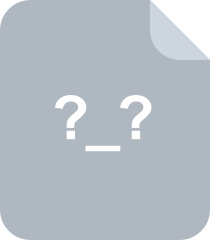
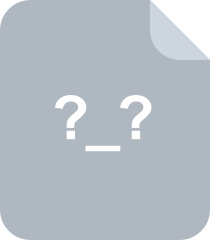
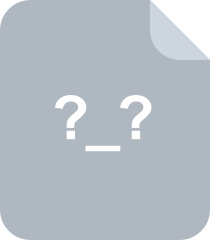

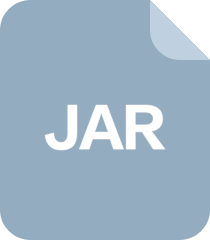
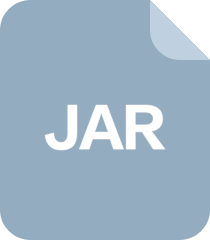
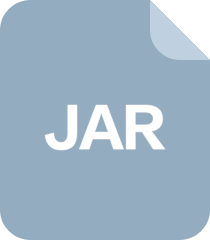
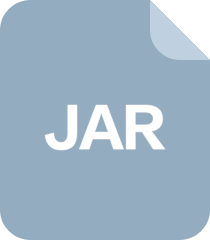
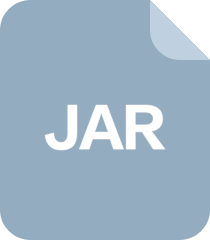
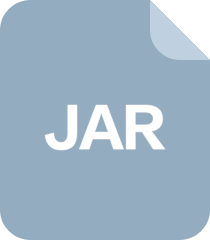
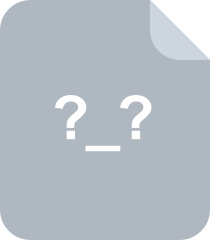
共 52 条
- 1

erdongritian
- 粉丝: 8
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

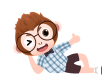
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页